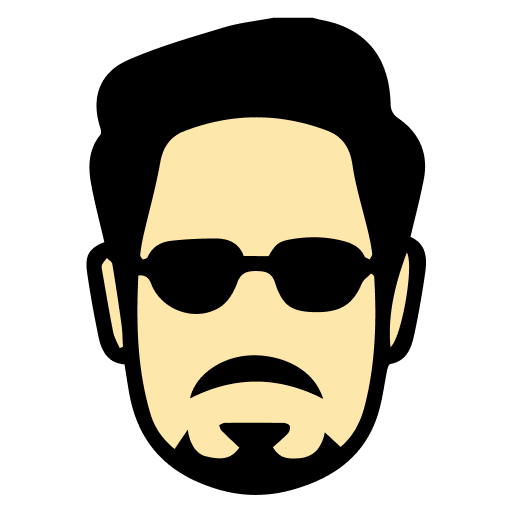
Vue.js, a progressive JavaScript framework, is popular for building interactive user interfaces, making it a vital skill for frontend developers and UI engineers. Stark.ai offers a curated collection of Vue.js interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Vue.js is a progressive JavaScript framework for building user interfaces. Key features include reactive data...
Create Vue projects using Vue CLI with 'vue create project-name' or quick start using CDN. Vue CLI provides project...
Vue 3 introduces Composition API, improved TypeScript support, multiple root elements, Teleport, Fragments, and...
Configure Vue application using createApp() method. Set global options, plugins, and components. Configure via...
Vue instance goes through creation (setup data observation), mounting (DOM compilation), updating (re-render), and...
Define data using data() function returning object in Options API or ref()/reactive() in Composition API. Data...
Computed properties are cached, reactive data properties that update when dependencies change. Define using computed...
Handle events using v-on directive or @ shorthand. Support event modifiers, arguments, and method calls. Example:...
Directives are special attributes with v- prefix that apply reactive behavior to DOM. Common directives include...
Use v-if, v-else-if, v-else for conditional rendering. v-show for toggle visibility. v-if removes/adds elements from...
Use watch option or watch() function to react to data changes. Support deep watching, immediate execution. Handle...
Register components globally using app.component() or locally in components option. Support async components. Handle...
Create mixins to share component logic. Handle mixin merging. Support global mixins. Implement mixin lifecycle....
Create plugins using install method. Handle plugin options. Support global features. Implement plugin API. Handle...
Create filters for value transformation in Vue 2. Replace with methods or computed properties in Vue 3. Handle...
Use component element with :is binding. Handle component switching. Support keep-alive. Implement lazy loading....
Implement async data fetching in created/mounted hooks or setup(). Handle loading states. Support error handling....
Use render functions for programmatic template creation. Handle virtual DOM nodes. Support JSX. Implement render...
Use provide/inject for dependency injection. Handle injection inheritance. Support reactive injection. Implement...
Create custom reactive systems. Handle dependency tracking. Support reactivity transformation. Implement custom...
Design plugin systems. Handle plugin compatibility. Support plugin composition. Implement plugin lifecycle. Handle...
Implement error tracking systems. Handle error recovery. Support error reporting. Implement error boundaries. Handle...
Create custom rendering systems. Handle render optimization. Support virtual scrolling. Implement render caching....
Implement complex state patterns. Handle state persistence. Support state synchronization. Implement state machines....
Create complex event systems. Handle cross-component communication. Support event buses. Implement communication...
Implement deployment strategies. Handle build optimization. Support continuous integration. Implement deployment...
Components are reusable Vue instances with template, script, and style blocks. They encapsulate HTML, JavaScript,...
Create .vue files with template, script, and style sections. Support component logic, template syntax, and scoped...
Props are custom attributes for passing data to child components. Define using props option or defineProps. Support...
Use $emit method or defineEmits to emit custom events. Parent components listen using v-on or @. Support event...
Slots provide content distribution points in component templates. Support default content, named slots, and scoped...
Register globally using app.component() or locally in components option. Support async components and dynamic...
ref wraps primitive values, reactive creates reactive objects. ref requires .value for access in script. Both...
Implement v-model using modelValue prop and update:modelValue event. Support custom v-model modifiers. Handle form...
Hooks run at specific stages of component lifecycle. Include created, mounted, updated, unmounted. Support setup and...
setup runs before component creation. Returns reactive state and methods. Replaces Options API features. Access...
Define async components using defineAsyncComponent. Support loading and error states. Handle component lazy loading....
Use provide/inject for dependency injection. Handle reactivity. Support injection key management. Implement...
Pass data to slot content using scoped slots. Support slot props. Handle template refs. Implement slot fallback...
Create reusable composition functions. Handle state sharing. Support composition hooks. Implement composition...
Use emits option or defineEmits. Support event validation. Handle event modifiers. Implement event patterns....
Use ref attribute and ref() function. Access DOM elements and component instances. Handle template refs. Implement...
Extend components using extends option. Handle inheritance chain. Support mixin inheritance. Implement inheritance patterns.
Create complex slot systems. Handle slot composition. Support dynamic slots. Implement slot patterns. Handle slot...
Implement component test strategies. Handle unit testing. Support integration testing. Implement test utilities....
Create complex composition patterns. Handle state sharing. Support composition hooks. Implement advanced patterns....
Design scalable component systems. Handle component organization. Support architecture patterns. Implement design...
Implement security measures. Handle XSS prevention. Support content security. Implement security patterns. Handle...
Reactivity is Vue's system for automatically updating the DOM when data changes. Uses Proxy-based reactivity in Vue...
Computed properties are cached reactive values that update when dependencies change. Define using computed() or...
Watchers observe reactive data changes and execute callbacks. Use watch option or watch() function. Support deep...
Use array methods that trigger reactivity (push, pop, splice). Avoid directly setting array indices. Use spread...
ref creates reactive reference to value. Access via .value in script. Automatically unwrapped in templates. Handles...
Use reactive() for objects, ref() for primitives. Handle nested reactivity. Support deep reactivity. Implement...
shallowRef and shallowReactive create shallow reactive references. Only top-level properties are reactive. Useful...
Use triggerRef() for refs, trigger component updates with nextTick(). Force component re-render. Handle manual...
Create readonly versions of reactive objects using readonly(). Prevent mutations. Support readonly refs. Implement...
Use watchers instead of computed for side effects. Handle async computed. Support computed caching. Implement...
Use deep reactivity with reactive(). Handle nested objects and arrays. Support deep watching. Implement nested patterns.
Cache computed results. Handle cache invalidation. Support cache strategies. Implement caching patterns. Handle...
Use Vue devtools. Track reactive dependencies. Support debugging tools. Implement debugging strategies. Handle...
Create reusable reactive patterns. Handle state sharing. Support composition. Implement pattern libraries. Handle...
Use reactive Maps and Sets. Handle collection methods. Support collection reactivity. Implement collection patterns.
Create persistent reactive state. Handle storage sync. Support storage patterns. Implement storage strategies....
Implement middleware for reactive updates. Handle transformation pipeline. Support middleware chain. Implement...
Create complex reactive architectures. Handle advanced patterns. Support system composition. Implement advanced strategies.
Optimize reactive updates. Handle large datasets. Support performance monitoring. Implement optimization strategies.
Create reactive test suites. Handle test scenarios. Support integration testing. Implement test strategies. Handle...
Implement security measures. Handle data protection. Support secure patterns. Implement security strategies.
Track reactive system metrics. Handle performance monitoring. Support debugging tools. Implement monitoring strategies.
Design scalable reactive systems. Handle system organization. Support architecture patterns. Implement design principles.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement...
Handle system upgrades. Support version migration. Implement migration strategies. Handle compatibility issues.
Directives are special attributes with v- prefix that apply reactive behavior to DOM. Built-in directives include...
v-bind dynamically binds attributes or props to expressions. Shorthand is :. Example: v-bind:href or :href. Supports...
v-if conditionally renders elements by adding/removing from DOM. v-show toggles display CSS property. v-if supports...
v-for iterates over arrays/objects. Syntax: v-for='item in items' or v-for='(value, key) in object'. Requires :key...
v-model creates two-way data binding on form inputs. Automatically handles different input types. Supports modifiers...
v-on listens to DOM events. Shorthand is @. Example: v-on:click or @click. Supports event modifiers and method...
Template expressions use {{ }} syntax for data binding. Support JavaScript expressions. Access component properties...
Modifiers are special postfixes denoted by dot. Example: .prevent, .stop, .capture. Modify directive behavior....
v-pre skips compilation for element and children. Displays raw mustache tags. Useful for displaying template syntax....
v-once renders element/component once only. Skips future updates. Improves performance for static content. Content...
Custom directives use app.directive() or locally in component directives option. Define hooks like mounted, updated....
Directive hooks include beforeMount, mounted, beforeUpdate, updated, beforeUnmount, unmounted. Access element and...
Use square brackets for dynamic arguments. Example: v-bind:[attributeName]. Support dynamic event names. Handle null...
Use v-slot directive for named slots. Support scoped slots. Handle slot props. Implement slot fallback content....
Use v-bind:class with object/array syntax. Support multiple classes. Handle dynamic classes. Implement conditional...
Use v-bind:style with object/array syntax. Support multiple styles. Handle vendor prefixes. Implement dynamic...
Use v-model for different input types. Handle checkboxes, radio buttons, selects. Support array/boolean bindings....
Use v-if, v-else-if, v-else. Handle template v-if. Support key management. Implement conditional groups. Handle...
Use v-for with key attribute. Handle array mutations. Support object iteration. Implement filtered/sorted lists....
Use v-on directive with modifiers. Handle method events. Support inline handlers. Implement event objects. Handle...
Create complex directive systems. Handle advanced cases. Support pattern composition. Implement reusable patterns....
Optimize directive performance. Handle caching strategies. Support lazy evaluation. Implement optimization patterns....
Create directive test suites. Handle test scenarios. Support integration testing. Implement test strategies. Handle...
Implement security measures. Handle XSS prevention. Support content security. Implement security patterns. Handle...
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems....
Implement semantic versioning. Handle backwards compatibility. Support version migration. Implement version control....
Create reusable directive libraries. Handle distribution. Support theming. Implement documentation. Handle...
Design scalable directive systems. Handle organization. Support architecture patterns. Implement design systems....
Track directive usage and performance. Handle monitoring integration. Support analytics. Implement monitoring strategies.
Vue Router is the official routing library for Vue.js. Enables navigation between pages, supports nested routes,...
Install using npm/yarn, create router instance with createRouter(), configure routes array with path and component...
Dynamic segments in route path marked with colon. Example: /user/:id. Access via useRoute().params or $route.params....
Define child routes in children array of parent route. Use router-view for nested components. Support multiple...
Middleware functions that guard navigations. Include beforeEach, beforeResolve, afterEach globally, and beforeEnter...
Component for declarative navigation. Supports to prop for destination, active class styling. Example: <router-link...
Navigate using router.push() or router.replace(). Support path object notation. Handle navigation with useRouter()...
Access via useRoute().query or $route.query. Support multiple parameters. Handle URL encoding. Persist across...
Routes with name property for reference. Use in router-link :to='{ name: 'route' }'. Support params binding. Avoid...
Custom fields in meta property of routes. Access via route.meta. Use for route-specific data. Handle authorization,...
Use transition component around router-view. Support enter/leave transitions. Handle per-route transitions....
Configure scrollBehavior in router options. Handle scroll position restoration. Support smooth scrolling. Implement...
Combine multiple guards. Handle guard chain. Support async guards. Implement guard utilities. Handle guard ordering.
Define alias property in routes. Support multiple aliases. Handle SEO considerations. Implement redirect patterns....
Enable props in route configuration. Support boolean mode, object mode, function mode. Handle prop types. Implement...
Handle data fetching in navigation guards or setup(). Support loading states. Implement error handling. Handle data...
Use regex patterns in route paths. Support parameter constraints. Handle wildcard routes. Implement matching strategies.
Implement error pages. Handle navigation errors. Support error recovery. Implement error boundaries. Handle error logging.
Create complex routing systems. Handle advanced scenarios. Support pattern composition. Implement routing strategies.
Optimize route configuration. Handle route caching. Support code splitting. Implement performance strategies. Handle...
Create router test suites. Handle navigation testing. Support guard testing. Implement test utilities. Handle test coverage.
Implement route protection. Handle authentication flows. Support authorization. Implement security patterns. Handle...
Track navigation metrics. Handle analytics integration. Support performance monitoring. Implement monitoring strategies.
Design scalable routing systems. Handle route organization. Support architecture patterns. Implement routing hierarchy.
Implement deployment strategies. Handle server configuration. Support hosting platforms. Implement deployment patterns.
Handle route structure changes. Support path migrations. Implement redirects. Handle compatibility. Manage migration state.
Vuex and Pinia are state management patterns/libraries for Vue.js applications. Vuex is traditional with mutations,...
Vuex has five core concepts: State, Getters, Mutations, Actions, and Modules. State holds data, Getters compute...
Pinia offers simpler API without mutations, better TypeScript support, multiple stores without modules, automatic...
Use defineStore with unique id and options or setup syntax. Contains state, getters, and actions. Example:...
Mutations are synchronous functions that modify state. Only way to change state in Vuex. Committed using commit...
In Vuex: use mapState helper or $store.state. In Pinia: use store instance directly or storeToRefs. Both support...
Actions handle asynchronous operations. Can commit mutations (Vuex) or directly modify state (Pinia). Support...
Getters compute derived state. Access other getters and state. Cache results based on dependencies. Similar to...
Modules split store into sub-stores. Support namespacing. Handle state separation. Implement module registration....
Compose multiple stores. Handle store dependencies. Support store inheritance. Implement composition patterns....
Create plugins for additional functionality. Handle state subscriptions. Support plugin options. Implement plugin...
Support store hot reloading. Handle state preservation. Implement HMR handlers. Support development workflow. Handle...
Test store components separately. Handle action testing. Support mutation testing. Implement test utilities. Handle...
Initialize store with default state. Handle async initialization. Support dynamic registration. Implement...
Subscribe to state changes. Handle mutation/action subscriptions. Support watchers. Implement subscription patterns.
Create middleware for store operations. Handle action middleware. Support middleware chain. Implement middleware patterns.
Manage error handling in store. Handle global errors. Support error recovery. Implement error patterns. Handle error...
Create complex store architectures. Handle advanced scenarios. Support pattern composition. Implement advanced strategies.
Optimize store performance. Handle large state trees. Support selective updates. Implement optimization strategies.
Handle store access control. Implement state protection. Support security patterns. Handle sensitive data.
Implement state migrations. Handle version updates. Support migration strategies. Handle backwards compatibility.
Track store operations. Handle performance monitoring. Support debugging tools. Implement monitoring strategies.
Design scalable store systems. Handle store organization. Support architecture patterns. Implement design principles.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement...
Create comprehensive test suites. Handle integration testing. Support unit testing. Implement test strategies.
Props are custom attributes for passing data from parent to child components. Defined using props option or...
Use $emit method or defineEmits to send events to parent components. Parents listen with v-on or @. Support event...
Provide/inject enables dependency injection between components. Parent provides data/methods, descendants inject...
v-model creates two-way binding between parent and child. Combines prop and event. Component must emit...
Refs provide direct access to child component instances. Use template ref attribute and ref(). Access methods and...
Slots pass template content to child components. Support default and named slots. Enable component composition. Pass...
Define prop types, required status, default values. Use validator functions. Handle prop constraints. Support type...
Modifiers customize event handling. Include .prevent, .stop, .once. Chain multiple modifiers. Support custom...
Watch props for changes. Handle prop updates. Support reactive props. Implement update logic. Example: watch(() =>...
attrs contains non-prop attributes passed to component. Access via $attrs or useAttrs(). Handle attribute...
Create centralized event bus for component communication. Handle event subscription. Support event broadcasting....
Use promises or async/await. Handle loading states. Support error handling. Implement async patterns. Handle...
Define methods using defineExpose. Access through template refs. Handle method calls. Support method parameters....
Pass data to slot content. Access slot props. Handle slot scope. Support slot defaults. Example: v-slot='slotProps'.
Transform props before use. Handle computed props. Support prop formatting. Implement transformation logic. Handle...
Validate event payload. Define event contracts. Handle validation errors. Implement validation logic. Support event types.
Define injection types. Handle type safety. Support type inference. Implement type validation. Handle type errors.
Implement prop forwarding. Handle prop chains. Support inheritance patterns. Implement inheritance logic. Handle...
Create event processing middleware. Handle event transformation. Support middleware chain. Implement middleware patterns.
Test component interaction. Handle event testing. Support prop testing. Implement test utilities. Handle test scenarios.
Create complex communication systems. Handle advanced scenarios. Support pattern composition. Implement advanced strategies.
Optimize component interaction. Handle performance bottlenecks. Support optimization strategies. Implement...
Handle secure communication. Implement data protection. Support security patterns. Handle sensitive data. Implement...
Track component interaction. Handle performance monitoring. Support debugging tools. Implement monitoring strategies.
Design scalable communication systems. Handle system organization. Support architecture patterns. Implement design...
Implement version control for APIs. Handle backwards compatibility. Support version migration. Implement versioning...
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement...
Create comprehensive test suites. Handle integration testing. Support unit testing. Implement test strategies....
Lifecycle hooks are special methods that allow executing code at specific stages of a component's life. Include...
created runs after instance is created, reactive data is set up. Can access reactive data and events. Cannot access...
mounted executes when component is mounted to DOM. Can access DOM elements and refs. Good for DOM manipulations and...
updated runs after data changes and DOM updates. Can access updated DOM. Should avoid changing state to prevent...
unmounted executes when component is removed from DOM. Used for cleanup (removing event listeners, canceling...
beforeCreate runs before instance initialization. Cannot access reactive data or events. Used for plugin...
beforeMount executes before DOM mounting begins. Template is compiled but not mounted. Cannot access DOM elements....
beforeUpdate runs before DOM updates after data change. Can access old DOM state. Good for saving state before...
beforeUnmount executes before component unmounting starts. Component still fully functional. Good for cleanup...
Composition API uses on* prefixed functions for lifecycle hooks. Must be called synchronously in setup(). Support...
Use async/await or promises. Handle loading states. Support error handling. Implement cleanup for async operations....
Return cleanup function from setup hooks. Remove event listeners. Clear timers. Cancel subscriptions. Handle...
activated and deactivated hooks for cached components. Handle component activation. Support cache lifecycle....
Use errorCaptured hook or try/catch. Handle error states. Support error recovery. Implement error boundaries. Handle...
Mixin hooks merge with component hooks. Handle hook ordering. Support hook composition. Implement mixin strategies.
Test hook execution order. Handle async testing. Support component mounting. Implement test utilities. Handle test scenarios.
Handle loading states. Support error states. Implement loading component. Handle async lifecycle. Support component loading.
Use Vue Devtools. Handle hook debugging. Support logging. Implement debugging strategies. Handle hook inspection.
Minimize hook operations. Handle heavy computations. Support performance optimization. Implement efficient patterns.
Create complex hook systems. Handle advanced scenarios. Support pattern composition. Implement advanced strategies.
Compose multiple hooks. Handle hook dependencies. Support hook reuse. Implement composition patterns.
Create hook-based plugins. Handle plugin lifecycle. Support plugin options. Implement plugin patterns.
Implement secure hook patterns. Handle sensitive operations. Support security measures. Implement security strategies.
Track hook execution. Handle performance monitoring. Support debugging tools. Implement monitoring strategies.
Design scalable hook systems. Handle hook organization. Support architecture patterns. Implement design principles.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement...
Create comprehensive test suites. Handle integration testing. Support unit testing. Implement test strategies.
Implement version updates. Handle migration strategies. Support backwards compatibility. Implement migration patterns.
v-model creates two-way data binding between form inputs and state. Works with input, textarea, select elements....
Use @submit.prevent for form submission. Access form data through refs or v-model. Handle validation before...
Modifiers customize input behavior: .lazy for change events, .number for numeric conversion, .trim for whitespace...
Use v-model with boolean or array values. Support multiple selections. Handle value binding. Work with...
Use v-model with single value binding. Handle radio groups. Support value attribute. Work with dynamic values....
Use v-model for single/multiple selection. Handle option groups. Support dynamic options. Work with value binding....
Validate form inputs before submission. Handle validation rules. Display validation errors. Support custom...
Use input type='file'. Access files through event.target.files. Handle file upload. Support multiple files....
Use form.reset() or set model values to initial state. Handle form clearing. Support partial reset. Implement reset...
Use computed for derived form values. Handle complex validation. Support dependent fields. Implement computed...
Create components with v-model support. Handle value prop and input events. Support validation. Implement custom...
Integrate validation libraries like Vuelidate or VeeValidate. Handle validation rules. Support async validation....
Generate form fields from data. Handle dynamic validation. Support field addition/removal. Implement dynamic...
Manage form state in Vuex/Pinia. Handle form persistence. Support state updates. Implement state patterns. Handle...
Create multi-step forms. Handle step validation. Support navigation. Implement progress tracking. Handle state persistence.
Manage arrays of form inputs. Handle dynamic fields. Support array operations. Implement array validation. Handle...
Add ARIA attributes. Handle keyboard navigation. Support screen readers. Implement accessible labels. Handle focus...
Display validation errors. Handle error styling. Support error messages. Implement error handling. Handle error recovery.
Test form validation. Handle input testing. Support submission testing. Implement test utilities. Handle test scenarios.
Create complex form systems. Handle advanced validation. Support form composition. Implement advanced strategies.
Optimize form performance. Handle large forms. Support form caching. Implement optimization strategies.
Handle CSRF protection. Implement data validation. Support security measures. Handle sensitive data.
Support multiple languages. Handle validation messages. Implement i18n patterns. Support RTL layouts.
Track form usage. Handle analytics integration. Support performance monitoring. Implement monitoring strategies.
Design scalable form systems. Handle form organization. Support architecture patterns. Implement design principles.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement...
Create comprehensive test suites. Handle integration testing. Support unit testing. Implement test strategies.
Implement version updates. Handle migration strategies. Support backwards compatibility. Implement migration patterns.
Vue.js is a progressive JavaScript framework for building user interfaces. Key features include reactive data binding, component-based architecture, virtual DOM, directives, computed properties, and lifecycle hooks. Supports both Options API and Composition API.
Create Vue projects using Vue CLI with 'vue create project-name' or quick start using CDN. Vue CLI provides project scaffolding, development tools, and build setup. Also supports Vite with 'npm init vue@latest'.
Vue 3 introduces Composition API, improved TypeScript support, multiple root elements, Teleport, Fragments, and better performance. Also includes improved reactivity system based on Proxy, and smaller bundle size.
Configure Vue application using createApp() method. Set global options, plugins, and components. Configure via vue.config.js for CLI projects. Handle environment variables and build settings.
Vue instance goes through creation (setup data observation), mounting (DOM compilation), updating (re-render), and destruction phases. Each phase has associated lifecycle hooks like created(), mounted(), updated(), and unmounted().
Define data using data() function returning object in Options API or ref()/reactive() in Composition API. Data properties are reactive and trigger view updates when changed.
Computed properties are cached, reactive data properties that update when dependencies change. Define using computed option or computed() function. More efficient than methods for derived values.
Handle events using v-on directive or @ shorthand. Support event modifiers, arguments, and method calls. Example: @click='handleClick' or v-on:submit.prevent='handleSubmit'.
Directives are special attributes with v- prefix that apply reactive behavior to DOM. Common directives include v-if, v-for, v-bind, v-model, v-on. Can create custom directives.
Use v-if, v-else-if, v-else for conditional rendering. v-show for toggle visibility. v-if removes/adds elements from DOM, v-show toggles display property.
Use watch option or watch() function to react to data changes. Support deep watching, immediate execution. Handle async operations. Implement cleanup functions.
Register components globally using app.component() or locally in components option. Support async components. Handle component naming conventions. Implement dynamic registration.
Create mixins to share component logic. Handle mixin merging. Support global mixins. Implement mixin lifecycle. Handle mixin conflicts.
Create plugins using install method. Handle plugin options. Support global features. Implement plugin API. Handle plugin initialization.
Create filters for value transformation in Vue 2. Replace with methods or computed properties in Vue 3. Handle filter chaining. Implement global filters.
Use component element with :is binding. Handle component switching. Support keep-alive. Implement lazy loading. Handle transition effects.
Implement async data fetching in created/mounted hooks or setup(). Handle loading states. Support error handling. Implement data caching.
Use render functions for programmatic template creation. Handle virtual DOM nodes. Support JSX. Implement render helpers. Handle render optimization.
Use provide/inject for dependency injection. Handle injection inheritance. Support reactive injection. Implement injection key management.
Create custom reactive systems. Handle dependency tracking. Support reactivity transformation. Implement custom observers. Handle reactive cleanup.
Design plugin systems. Handle plugin compatibility. Support plugin composition. Implement plugin lifecycle. Handle plugin dependencies.
Implement error tracking systems. Handle error recovery. Support error reporting. Implement error boundaries. Handle error patterns.
Create custom rendering systems. Handle render optimization. Support virtual scrolling. Implement render caching. Handle render strategies.
Implement complex state patterns. Handle state persistence. Support state synchronization. Implement state machines. Handle state optimization.
Create complex event systems. Handle cross-component communication. Support event buses. Implement communication patterns. Handle event optimization.
Implement deployment strategies. Handle build optimization. Support continuous integration. Implement deployment automation. Handle environment configuration.
Components are reusable Vue instances with template, script, and style blocks. They encapsulate HTML, JavaScript, and CSS. Support props, events, and slots for composition. Can be registered globally or locally.
Create .vue files with template, script, and style sections. Support component logic, template syntax, and scoped styles. Example: <template>, <script>, and <style> blocks in one file.
Props are custom attributes for passing data to child components. Define using props option or defineProps. Support type checking, default values, and required props. Example: props: ['title'] or defineProps(['title']).
Use $emit method or defineEmits to emit custom events. Parent components listen using v-on or @. Support event arguments and validation. Example: this.$emit('change', value) or emit('change', value).
Slots provide content distribution points in component templates. Support default content, named slots, and scoped slots. Enable component composition and content injection. Example: <slot name='header'>.
Register globally using app.component() or locally in components option. Support async components and dynamic registration. Example: components: { 'my-component': MyComponent }.
ref wraps primitive values, reactive creates reactive objects. ref requires .value for access in script. Both trigger reactivity. Use ref for primitives, reactive for objects.
Implement v-model using modelValue prop and update:modelValue event. Support custom v-model modifiers. Handle form input components. Example: v-model='value' or defineModel().
Hooks run at specific stages of component lifecycle. Include created, mounted, updated, unmounted. Support setup and cleanup operations. Example: onMounted(() => {}).
setup runs before component creation. Returns reactive state and methods. Replaces Options API features. Access props and context. Example: setup(props, context).
Define async components using defineAsyncComponent. Support loading and error states. Handle component lazy loading. Implement loading strategies.
Use provide/inject for dependency injection. Handle reactivity. Support injection key management. Implement injection inheritance. Example: provide('key', value).
Pass data to slot content using scoped slots. Support slot props. Handle template refs. Implement slot fallback content. Example: v-slot='slotProps'.
Create reusable composition functions. Handle state sharing. Support composition hooks. Implement composition patterns. Example: useFeature().
Use emits option or defineEmits. Support event validation. Handle event modifiers. Implement event patterns. Example: emits: ['update'].
Use ref attribute and ref() function. Access DOM elements and component instances. Handle template refs. Implement ref callback.
Extend components using extends option. Handle inheritance chain. Support mixin inheritance. Implement inheritance patterns.
Create complex slot systems. Handle slot composition. Support dynamic slots. Implement slot patterns. Handle slot optimization.
Implement component test strategies. Handle unit testing. Support integration testing. Implement test utilities. Handle test coverage.
Create complex composition patterns. Handle state sharing. Support composition hooks. Implement advanced patterns. Handle composition testing.
Design scalable component systems. Handle component organization. Support architecture patterns. Implement design systems. Handle maintainability.
Implement security measures. Handle XSS prevention. Support content security. Implement security patterns. Handle vulnerability prevention.
Reactivity is Vue's system for automatically updating the DOM when data changes. Uses Proxy-based reactivity in Vue 3, Object.defineProperty in Vue 2. Tracks dependencies and triggers updates automatically.
Computed properties are cached reactive values that update when dependencies change. Define using computed() or computed option. More efficient than methods for derived values.
Watchers observe reactive data changes and execute callbacks. Use watch option or watch() function. Support deep watching and immediate execution. Handle side effects.
Use array methods that trigger reactivity (push, pop, splice). Avoid directly setting array indices. Use spread operator for immutable updates. Handle array reactivity limitations.
ref creates reactive reference to value. Access via .value in script. Automatically unwrapped in templates. Handles primitive values and objects. Example: const count = ref(0).
Use reactive() for objects, ref() for primitives. Handle nested reactivity. Support deep reactivity. Implement reactive patterns. Example: reactive({ count: 0 }).
shallowRef and shallowReactive create shallow reactive references. Only top-level properties are reactive. Useful for large objects. Better performance for specific cases.
Use triggerRef() for refs, trigger component updates with nextTick(). Force component re-render. Handle manual reactivity. Example: triggerRef(myRef).
Create readonly versions of reactive objects using readonly(). Prevent mutations. Support readonly refs. Implement immutable patterns. Example: readonly(state).
Use watchers instead of computed for side effects. Handle async computed. Support computed caching. Implement computed patterns.
Use deep reactivity with reactive(). Handle nested objects and arrays. Support deep watching. Implement nested patterns.
Cache computed results. Handle cache invalidation. Support cache strategies. Implement caching patterns. Handle cache management.
Use Vue devtools. Track reactive dependencies. Support debugging tools. Implement debugging strategies. Handle reactivity issues.
Create reusable reactive patterns. Handle state sharing. Support composition. Implement pattern libraries. Handle pattern management.
Use reactive Maps and Sets. Handle collection methods. Support collection reactivity. Implement collection patterns.
Create persistent reactive state. Handle storage sync. Support storage patterns. Implement storage strategies. Handle storage management.
Implement middleware for reactive updates. Handle transformation pipeline. Support middleware chain. Implement middleware patterns.
Create complex reactive architectures. Handle advanced patterns. Support system composition. Implement advanced strategies.
Optimize reactive updates. Handle large datasets. Support performance monitoring. Implement optimization strategies.
Create reactive test suites. Handle test scenarios. Support integration testing. Implement test strategies. Handle test coverage.
Implement security measures. Handle data protection. Support secure patterns. Implement security strategies.
Track reactive system metrics. Handle performance monitoring. Support debugging tools. Implement monitoring strategies.
Design scalable reactive systems. Handle system organization. Support architecture patterns. Implement design principles.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement deployment patterns.
Handle system upgrades. Support version migration. Implement migration strategies. Handle compatibility issues.
Directives are special attributes with v- prefix that apply reactive behavior to DOM. Built-in directives include v-if, v-for, v-bind, v-on, v-model. Support modifiers and arguments.
v-bind dynamically binds attributes or props to expressions. Shorthand is :. Example: v-bind:href or :href. Supports dynamic values and object syntax for multiple bindings.
v-if conditionally renders elements by adding/removing from DOM. v-show toggles display CSS property. v-if supports v-else and has higher toggle cost. v-show better for frequent toggles.
v-for iterates over arrays/objects. Syntax: v-for='item in items' or v-for='(value, key) in object'. Requires :key for list rendering. Supports index parameter.
v-model creates two-way data binding on form inputs. Automatically handles different input types. Supports modifiers like .lazy, .number, .trim. Example: v-model='message'.
v-on listens to DOM events. Shorthand is @. Example: v-on:click or @click. Supports event modifiers and method handlers. Can pass event object and arguments.
Template expressions use {{ }} syntax for data binding. Support JavaScript expressions. Access component properties and methods. Limited to single expressions.
Modifiers are special postfixes denoted by dot. Example: .prevent, .stop, .capture. Modify directive behavior. Support chaining multiple modifiers.
v-pre skips compilation for element and children. Displays raw mustache tags. Useful for displaying template syntax. Improves compilation performance for static content.
v-once renders element/component once only. Skips future updates. Improves performance for static content. Content treated as static after initial render.
Custom directives use app.directive() or locally in component directives option. Define hooks like mounted, updated. Access element and binding values. Support directive arguments.
Directive hooks include beforeMount, mounted, beforeUpdate, updated, beforeUnmount, unmounted. Access element and binding values. Handle lifecycle events.
Use square brackets for dynamic arguments. Example: v-bind:[attributeName]. Support dynamic event names. Handle null values. Implement dynamic behavior.
Use v-slot directive for named slots. Support scoped slots. Handle slot props. Implement slot fallback content. Example: v-slot:header.
Use v-bind:class with object/array syntax. Support multiple classes. Handle dynamic classes. Implement conditional classes. Example: :class="{ active: isActive }".
Use v-bind:style with object/array syntax. Support multiple styles. Handle vendor prefixes. Implement dynamic styles. Example: :style="{ color: activeColor }".
Use v-model for different input types. Handle checkboxes, radio buttons, selects. Support array/boolean bindings. Implement custom input components.
Use v-if, v-else-if, v-else. Handle template v-if. Support key management. Implement conditional groups. Handle transition effects.
Use v-for with key attribute. Handle array mutations. Support object iteration. Implement filtered/sorted lists. Handle list updates.
Use v-on directive with modifiers. Handle method events. Support inline handlers. Implement event objects. Handle multiple events.
Create complex directive systems. Handle advanced cases. Support pattern composition. Implement reusable patterns. Handle edge cases.
Optimize directive performance. Handle caching strategies. Support lazy evaluation. Implement optimization patterns. Handle render optimization.
Create directive test suites. Handle test scenarios. Support integration testing. Implement test strategies. Handle test coverage.
Implement security measures. Handle XSS prevention. Support content security. Implement security patterns. Handle vulnerability prevention.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems. Handle maintenance.
Implement semantic versioning. Handle backwards compatibility. Support version migration. Implement version control. Handle deprecation.
Create reusable directive libraries. Handle distribution. Support theming. Implement documentation. Handle versioning and maintenance.
Design scalable directive systems. Handle organization. Support architecture patterns. Implement design systems. Handle maintainability.
Track directive usage and performance. Handle monitoring integration. Support analytics. Implement monitoring strategies.
Vue Router is the official routing library for Vue.js. Enables navigation between pages, supports nested routes, dynamic route matching, navigation guards, and route meta fields. Integrates with Vue.js transitions.
Install using npm/yarn, create router instance with createRouter(), configure routes array with path and component mappings. Use history mode (createWebHistory) or hash mode.
Dynamic segments in route path marked with colon. Example: /user/:id. Access via useRoute().params or $route.params. Support optional parameters and custom regex patterns.
Define child routes in children array of parent route. Use router-view for nested components. Support multiple levels of nesting. Handle nested layouts.
Middleware functions that guard navigations. Include beforeEach, beforeResolve, afterEach globally, and beforeEnter per route. Handle authentication and authorization.
Component for declarative navigation. Supports to prop for destination, active class styling. Example: <router-link to='/path'>. Handles both literal and dynamic paths.
Navigate using router.push() or router.replace(). Support path object notation. Handle navigation with useRouter() in composition API. Support navigation parameters.
Access via useRoute().query or $route.query. Support multiple parameters. Handle URL encoding. Persist across navigations. Example: /search?q=term.
Routes with name property for reference. Use in router-link :to='{ name: 'route' }'. Support params binding. Avoid hard-coded URLs. Example: name: 'user'.
Custom fields in meta property of routes. Access via route.meta. Use for route-specific data. Handle authorization, transitions, or any custom data.
Use transition component around router-view. Support enter/leave transitions. Handle per-route transitions. Implement transition modes. Handle dynamic transitions.
Configure scrollBehavior in router options. Handle scroll position restoration. Support smooth scrolling. Implement scroll preservation. Handle async scrolling.
Combine multiple guards. Handle guard chain. Support async guards. Implement guard utilities. Handle guard ordering.
Define alias property in routes. Support multiple aliases. Handle SEO considerations. Implement redirect patterns. Handle alias conflicts.
Enable props in route configuration. Support boolean mode, object mode, function mode. Handle prop types. Implement prop validation.
Handle data fetching in navigation guards or setup(). Support loading states. Implement error handling. Handle data dependencies.
Use regex patterns in route paths. Support parameter constraints. Handle wildcard routes. Implement matching strategies.
Implement error pages. Handle navigation errors. Support error recovery. Implement error boundaries. Handle error logging.
Create complex routing systems. Handle advanced scenarios. Support pattern composition. Implement routing strategies.
Optimize route configuration. Handle route caching. Support code splitting. Implement performance strategies. Handle route priorities.
Create router test suites. Handle navigation testing. Support guard testing. Implement test utilities. Handle test coverage.
Implement route protection. Handle authentication flows. Support authorization. Implement security patterns. Handle security policies.
Track navigation metrics. Handle analytics integration. Support performance monitoring. Implement monitoring strategies.
Design scalable routing systems. Handle route organization. Support architecture patterns. Implement routing hierarchy.
Implement deployment strategies. Handle server configuration. Support hosting platforms. Implement deployment patterns.
Handle route structure changes. Support path migrations. Implement redirects. Handle compatibility. Manage migration state.
Vuex and Pinia are state management patterns/libraries for Vue.js applications. Vuex is traditional with mutations, while Pinia is newer with simpler API. Both handle centralized state management with reactive updates.
Vuex has five core concepts: State, Getters, Mutations, Actions, and Modules. State holds data, Getters compute derived state, Mutations change state synchronously, Actions handle async operations.
Pinia offers simpler API without mutations, better TypeScript support, multiple stores without modules, automatic code splitting. More lightweight and intuitive than Vuex.
Use defineStore with unique id and options or setup syntax. Contains state, getters, and actions. Example: defineStore('counter', { state: () => ({ count: 0 }) }).
Mutations are synchronous functions that modify state. Only way to change state in Vuex. Committed using commit method. Example: commit('increment', payload).
In Vuex: use mapState helper or $store.state. In Pinia: use store instance directly or storeToRefs. Both support computed properties for reactivity.
Actions handle asynchronous operations. Can commit mutations (Vuex) or directly modify state (Pinia). Support business logic and API calls. Can return promises.
Getters compute derived state. Access other getters and state. Cache results based on dependencies. Similar to computed properties for stores.
Modules split store into sub-stores. Support namespacing. Handle state separation. Implement module registration. Support dynamic modules.
Compose multiple stores. Handle store dependencies. Support store inheritance. Implement composition patterns. Handle state sharing.
Create plugins for additional functionality. Handle state subscriptions. Support plugin options. Implement plugin patterns. Handle plugin lifecycle.
Support store hot reloading. Handle state preservation. Implement HMR handlers. Support development workflow. Handle module updates.
Test store components separately. Handle action testing. Support mutation testing. Implement test utilities. Handle mock data.
Initialize store with default state. Handle async initialization. Support dynamic registration. Implement initialization patterns.
Subscribe to state changes. Handle mutation/action subscriptions. Support watchers. Implement subscription patterns.
Create middleware for store operations. Handle action middleware. Support middleware chain. Implement middleware patterns.
Manage error handling in store. Handle global errors. Support error recovery. Implement error patterns. Handle error reporting.
Create complex store architectures. Handle advanced scenarios. Support pattern composition. Implement advanced strategies.
Optimize store performance. Handle large state trees. Support selective updates. Implement optimization strategies.
Handle store access control. Implement state protection. Support security patterns. Handle sensitive data.
Implement state migrations. Handle version updates. Support migration strategies. Handle backwards compatibility.
Track store operations. Handle performance monitoring. Support debugging tools. Implement monitoring strategies.
Design scalable store systems. Handle store organization. Support architecture patterns. Implement design principles.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement deployment patterns.
Create comprehensive test suites. Handle integration testing. Support unit testing. Implement test strategies.
Props are custom attributes for passing data from parent to child components. Defined using props option or defineProps. Support type checking, default values, and validation. Example: defineProps(['title']) or props: { title: String }.
Use $emit method or defineEmits to send events to parent components. Parents listen with v-on or @. Support event arguments. Example: emit('update', value) or this.$emit('update', value).
Provide/inject enables dependency injection between components. Parent provides data/methods, descendants inject them. Works across component layers. Alternative to prop drilling.
v-model creates two-way binding between parent and child. Combines prop and event. Component must emit update:modelValue event. Support custom v-model modifiers.
Refs provide direct access to child component instances. Use template ref attribute and ref(). Access methods and properties. Handle component communication.
Slots pass template content to child components. Support default and named slots. Enable component composition. Pass data back through scoped slots.
Define prop types, required status, default values. Use validator functions. Handle prop constraints. Support type checking. Example: prop: { type: String, required: true }.
Modifiers customize event handling. Include .prevent, .stop, .once. Chain multiple modifiers. Support custom modifiers. Example: @click.prevent.stop.
Watch props for changes. Handle prop updates. Support reactive props. Implement update logic. Example: watch(() => props.value).
attrs contains non-prop attributes passed to component. Access via $attrs or useAttrs(). Handle attribute inheritance. Support fallthrough attributes.
Create centralized event bus for component communication. Handle event subscription. Support event broadcasting. Implement event patterns.
Use promises or async/await. Handle loading states. Support error handling. Implement async patterns. Handle response handling.
Define methods using defineExpose. Access through template refs. Handle method calls. Support method parameters. Example: defineExpose({ method }).
Pass data to slot content. Access slot props. Handle slot scope. Support slot defaults. Example: v-slot='slotProps'.
Transform props before use. Handle computed props. Support prop formatting. Implement transformation logic. Handle prop updates.
Validate event payload. Define event contracts. Handle validation errors. Implement validation logic. Support event types.
Define injection types. Handle type safety. Support type inference. Implement type validation. Handle type errors.
Implement prop forwarding. Handle prop chains. Support inheritance patterns. Implement inheritance logic. Handle prop conflicts.
Create event processing middleware. Handle event transformation. Support middleware chain. Implement middleware patterns.
Test component interaction. Handle event testing. Support prop testing. Implement test utilities. Handle test scenarios.
Create complex communication systems. Handle advanced scenarios. Support pattern composition. Implement advanced strategies.
Optimize component interaction. Handle performance bottlenecks. Support optimization strategies. Implement performance patterns.
Handle secure communication. Implement data protection. Support security patterns. Handle sensitive data. Implement security measures.
Track component interaction. Handle performance monitoring. Support debugging tools. Implement monitoring strategies.
Design scalable communication systems. Handle system organization. Support architecture patterns. Implement design principles.
Implement version control for APIs. Handle backwards compatibility. Support version migration. Implement versioning strategies.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement deployment patterns.
Create comprehensive test suites. Handle integration testing. Support unit testing. Implement test strategies. Handle test coverage.
Lifecycle hooks are special methods that allow executing code at specific stages of a component's life. Include creation, mounting, updating, and destruction phases. Available in both Options API and Composition API.
created runs after instance is created, reactive data is set up. Can access reactive data and events. Cannot access DOM/this.$el. Good for initial data fetching. Composition API equivalent: onCreated.
mounted executes when component is mounted to DOM. Can access DOM elements and refs. Good for DOM manipulations and third-party library initialization. Composition API: onMounted.
updated runs after data changes and DOM updates. Can access updated DOM. Should avoid changing state to prevent infinite loops. Composition API: onUpdated.
unmounted executes when component is removed from DOM. Used for cleanup (removing event listeners, canceling timers). All directives unbound, watchers stopped. Composition API: onUnmounted.
beforeCreate runs before instance initialization. Cannot access reactive data or events. Used for plugin initialization. Composition API setup() runs before this hook.
beforeMount executes before DOM mounting begins. Template is compiled but not mounted. Cannot access DOM elements. Composition API: onBeforeMount.
beforeUpdate runs before DOM updates after data change. Can access old DOM state. Good for saving state before update. Composition API: onBeforeUpdate.
beforeUnmount executes before component unmounting starts. Component still fully functional. Good for cleanup initialization. Composition API: onBeforeUnmount.
Composition API uses on* prefixed functions for lifecycle hooks. Must be called synchronously in setup(). Support multiple calls. Return cleanup functions.
Use async/await or promises. Handle loading states. Support error handling. Implement cleanup for async operations. Consider component lifecycle.
Return cleanup function from setup hooks. Remove event listeners. Clear timers. Cancel subscriptions. Handle resource cleanup.
activated and deactivated hooks for cached components. Handle component activation. Support cache lifecycle. Implement caching strategies.
Use errorCaptured hook or try/catch. Handle error states. Support error recovery. Implement error boundaries. Handle error reporting.
Mixin hooks merge with component hooks. Handle hook ordering. Support hook composition. Implement mixin strategies.
Test hook execution order. Handle async testing. Support component mounting. Implement test utilities. Handle test scenarios.
Handle loading states. Support error states. Implement loading component. Handle async lifecycle. Support component loading.
Use Vue Devtools. Handle hook debugging. Support logging. Implement debugging strategies. Handle hook inspection.
Minimize hook operations. Handle heavy computations. Support performance optimization. Implement efficient patterns.
Create complex hook systems. Handle advanced scenarios. Support pattern composition. Implement advanced strategies.
Compose multiple hooks. Handle hook dependencies. Support hook reuse. Implement composition patterns.
Create hook-based plugins. Handle plugin lifecycle. Support plugin options. Implement plugin patterns.
Implement secure hook patterns. Handle sensitive operations. Support security measures. Implement security strategies.
Track hook execution. Handle performance monitoring. Support debugging tools. Implement monitoring strategies.
Design scalable hook systems. Handle hook organization. Support architecture patterns. Implement design principles.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement deployment patterns.
Create comprehensive test suites. Handle integration testing. Support unit testing. Implement test strategies.
Implement version updates. Handle migration strategies. Support backwards compatibility. Implement migration patterns.
v-model creates two-way data binding between form inputs and state. Works with input, textarea, select elements. Supports modifiers like .lazy, .number, .trim. Automatically handles different input types.
Use @submit.prevent for form submission. Access form data through refs or v-model. Handle validation before submission. Support async submission. Implement loading states.
Modifiers customize input behavior: .lazy for change events, .number for numeric conversion, .trim for whitespace removal. Can be chained. Example: v-model.lazy.trim.
Use v-model with boolean or array values. Support multiple selections. Handle value binding. Work with true-value/false-value attributes. Support checkbox groups.
Use v-model with single value binding. Handle radio groups. Support value attribute. Work with dynamic values. Implement radio button components.
Use v-model for single/multiple selection. Handle option groups. Support dynamic options. Work with value binding. Implement custom select components.
Validate form inputs before submission. Handle validation rules. Display validation errors. Support custom validation. Implement validation strategies.
Use input type='file'. Access files through event.target.files. Handle file upload. Support multiple files. Implement file validation.
Use form.reset() or set model values to initial state. Handle form clearing. Support partial reset. Implement reset strategies.
Use computed for derived form values. Handle complex validation. Support dependent fields. Implement computed validation. Handle computed states.
Create components with v-model support. Handle value prop and input events. Support validation. Implement custom behavior. Handle component state.
Integrate validation libraries like Vuelidate or VeeValidate. Handle validation rules. Support async validation. Implement validation messages.
Generate form fields from data. Handle dynamic validation. Support field addition/removal. Implement dynamic behavior. Handle state management.
Manage form state in Vuex/Pinia. Handle form persistence. Support state updates. Implement state patterns. Handle form actions.
Create multi-step forms. Handle step validation. Support navigation. Implement progress tracking. Handle state persistence.
Manage arrays of form inputs. Handle dynamic fields. Support array operations. Implement array validation. Handle array state.
Add ARIA attributes. Handle keyboard navigation. Support screen readers. Implement accessible labels. Handle focus management.
Display validation errors. Handle error styling. Support error messages. Implement error handling. Handle error recovery.
Test form validation. Handle input testing. Support submission testing. Implement test utilities. Handle test scenarios.
Create complex form systems. Handle advanced validation. Support form composition. Implement advanced strategies.
Optimize form performance. Handle large forms. Support form caching. Implement optimization strategies.
Handle CSRF protection. Implement data validation. Support security measures. Handle sensitive data.
Support multiple languages. Handle validation messages. Implement i18n patterns. Support RTL layouts.
Track form usage. Handle analytics integration. Support performance monitoring. Implement monitoring strategies.
Design scalable form systems. Handle form organization. Support architecture patterns. Implement design principles.
Create comprehensive documentation. Generate API docs. Support example usage. Implement documentation systems.
Implement deployment strategies. Handle environment configuration. Support production optimization. Implement deployment patterns.
Create comprehensive test suites. Handle integration testing. Support unit testing. Implement test strategies.
Implement version updates. Handle migration strategies. Support backwards compatibility. Implement migration patterns.
Understand composition API, props, and emit patterns.
Work with Vuex, Pinia, and reactive state patterns.
Explore computed properties, watchers, and lazy loading.
Expect discussions about component organization and routing.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Vue.js questions, mock interviews, and more to secure your dream role.
Start Preparing now