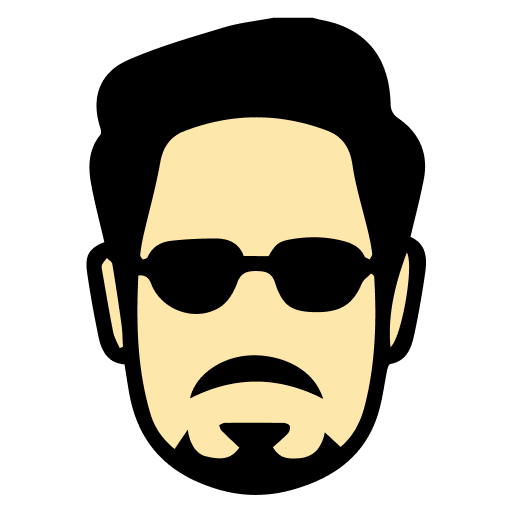
Routing in Laravel refers to defining URL paths that users can access and mapping them to specific controller...
Laravel supports common HTTP methods: GET for retrieving data, POST for creating new resources, PUT/PATCH for...
A controller is a PHP class that handles business logic and acts as an intermediary between Models and Views....
Controllers can be created using the Artisan command: php artisan make:controller ControllerName. This creates a new...
Route parameters are dynamic segments in URLs that capture and pass values to controller methods. They are defined...
Routes can be named using the name() method: Route::get('/profile', [ProfileController::class,...
web.php contains routes for web interface and includes session state and CSRF protection. api.php is for stateless...
Request data can be accessed using the Request facade or by type-hinting Request in controller methods. Example:...
Route grouping allows you to share route attributes (middleware, prefixes, namespaces) across multiple routes....
Redirects can be performed using redirect() helper or Route::redirect(). Examples: return redirect('/home') or...
Resource controllers handle CRUD operations for a resource. Created using 'php artisan make:controller...
Route model binding automatically resolves Eloquent models from route parameters. It can be implicit (type-hint the...
Controller middleware filter HTTP requests before they reach controllers. They can be assigned using the middleware...
File uploads are handled using the $request->file() method. Files can be stored using Storage facade or move()...
Route constraints limit how route parameters may be matched using regex patterns. They can be applied using where()...
CORS can be handled using the cors middleware and configuration in config/cors.php. Laravel provides built-in CORS...
Dependency injection in controllers automatically resolves class dependencies in constructor or method parameters...
API versioning can be implemented using route prefixes, subdomains, or headers. Common approaches include using...
Signed routes are URLs with a signature hash to verify they haven't been modified. Created using URL::signedRoute(),...
Rate limiting is implemented using throttle middleware. It can be configured in RouteServiceProvider and customized...
Custom route model binding can be implemented by overriding the resolveRouteBinding() method in models or by...
Domain routing is implemented using Route::domain(). Subdomains can capture parameters:...
Custom response macros extend Response functionality using Response::macro() in a service provider. Example:...
Route caching improves performance using 'php artisan route:cache'. It requires all route closures to be converted...
Custom middleware parameters are implemented by adding additional parameters to handle() method. In routes:...
Route fallbacks are implemented using Route::fallback(). Custom 404 handling can be done by overriding the render()...
Conditional middleware can be implemented using middleware() with when() or unless() methods. Can also be done by...
API resource collections with conditional relationships use whenLoaded() method and conditional attribute inclusion....
Multiple parameter binding can be implemented using explicit route model binding in RouteServiceProvider, or by...
Soft deleted models in route binding can be included using withTrashed() scope. Custom resolution logic can be...
Blade is Laravel's templating engine that combines PHP with HTML templates. It provides convenient shortcuts for...
Variables in Blade templates are displayed using double curly braces syntax {{ $variable }}. The content within...
Blade template inheritance allows creating a base layout template (@extends) with defined sections (@section) that...
Sub-views can be included using @include('view.name') directive. You can also pass data to included views:...
Blade provides convenient directives for control structures: @if, @else, @elseif, @endif for conditionals; @foreach,...
Blade supports loops using @foreach, @for, @while directives. The $loop variable is available inside foreach loops,...
The @yield directive displays content of a specified section. It's typically used in layout templates to define...
Laravel provides the asset() helper function to generate URLs for assets. Assets are stored in the public directory...
Blade comments are written using {{-- comment --}} syntax. Unlike HTML comments, Blade comments are not included in...
Blade Components are reusable template pieces with isolated logic. Created using 'php artisan make:component', they...
Stacks in Blade allow pushing content to named locations using @push and @stack directives. Multiple @push calls can...
Blade Service Injection allows injecting services into views using @inject directive. Example: @inject('metrics',...
Form validation errors are available through $errors variable. Common patterns include @error directive for specific...
Laravel Mix is a webpack wrapper that simplifies asset compilation. It's configured in webpack.mix.js and provides...
Custom Blade directives are created in a service provider using Blade::directive(). They transform template syntax...
Slots allow passing content to components. Default slot uses <x-slot> tag, named slots use name attribute....
Blade provides @auth and @guest directives for authentication checks. Additional directives like @can, @cannot for...
View Composers bind data to views whenever they're rendered. Registered in service providers using View::composer()....
Localization uses @lang directive or __() helper for translations. Supports string replacement, pluralization, and...
Dynamic components can be rendered using <x-dynamic-component :component="$componentName">. Component name can be...
Attributes Bag ($attributes) manages additional attributes passed to components. Supports merging, filtering, and...
Anonymous components are created without class files using single Blade templates. Stored in...
Components can be organized in subdirectories and namespaced. Configure component namespaces in service provider...
Components support lazy loading using wire:init or defer loading until needed. Useful for performance optimization....
Custom if statements are added using Blade::if() in service provider. Can encapsulate complex conditional logic in...
Component methods can use dependency injection through method parameters. Laravel automatically resolves...
Components have rendering lifecycle hooks like mount(), rendering(), rendered(). Can modify component state and...
Components can be autoloaded using package discovery or manual registration in service providers. Support for...
Custom template compilation can be implemented by extending Blade compiler. Add custom compilation passes, modify...
Eloquent ORM (Object-Relational Mapping) is Laravel's built-in ORM that allows developers to interact with database...
Models can be created using Artisan command: 'php artisan make:model ModelName'. Adding -m flag creates a migration...
Migrations are like version control for databases, allowing you to define and modify database schema using PHP code....
Basic Eloquent operations include: Model::all() to retrieve all records, Model::find(id) to find by primary key,...
Relationships are defined as methods in model classes. Common types include hasOne(), hasMany(), belongsTo(),...
Seeders are used to populate database tables with sample or initial data. They are created using 'php artisan...
Eloquent provides methods like where(), orWhere(), whereBetween(), orderBy() for querying. Example:...
Mass assignment allows setting multiple model attributes at once. $fillable property in models specifies which...
Database connections are configured in config/database.php and .env file. Multiple connections can be defined for...
Model factories generate fake data for testing and seeding. Created using 'php artisan make:factory'. They use Faker...
Eager loading reduces N+1 query problems by loading relationships in advance using with() method. Example:...
Model events are triggered during various stages of a model's lifecycle (creating, created, updating, etc.)....
Soft deletes add deleted_at timestamp instead of actually deleting records. Use SoftDeletes trait in model and add...
Query scopes are reusable query constraints. Global scopes automatically apply to all queries. Local scopes are...
Polymorphic relationships allow a model to belong to multiple types of models. Uses morphTo(), morphMany(),...
Accessors and mutators modify attribute values when retrieving or setting them. Defined using...
Transactions ensure multiple database operations succeed or fail as a unit. Use DB::transaction() or...
Pivot tables implement many-to-many relationships. Created using createPivotTable migration method. Can have...
Query results can be cached using remember() or rememberForever() methods. Cache duration and key can be specified....
Subqueries can be used in selects and joins using addSelect() and joinSub(). Useful for complex queries involving...
Custom collections extend Illuminate\Database\Eloquent\Collection. Override newCollection() in model to use custom...
Models can be replicated using replicate() method. Specify which attributes to exclude. Handle relationships...
Custom model binding resolvers modify how models are resolved from route parameters. Defined in RouteServiceProvider...
Composite keys require overriding getKeyName() and getIncrementing(). Additional configuration needed for...
Custom query builders extend Illuminate\Database\Eloquent\Builder. Override newEloquentBuilder() in model. Add...
Customize toArray() and toJson() methods. Use hidden and visible properties. Implement custom casts for complex...
Sharding requires custom connection resolvers. Override getConnection() in models. Implement logic for determining...
Real-time observers can broadcast model changes using events. Implement ShouldBroadcast interface. Configure...
Recursive relationships use self-referential associations. Implement methods for traversing tree structures....
Custom connection handling requires extending Connection class. Implement custom query grammar and processor. Handle...
Laravel provides a complete authentication system out of the box using the Auth facade. It includes features for...
Basic authentication can be implemented using Auth::attempt(['email' => $email, 'password' => $password]) for login,...
Auth middleware (auth) protects routes by ensuring users are authenticated. Can be applied to routes or controllers...
Use Auth::check() to verify authentication status, Auth::user() to get current user, or @auth/@guest Blade...
Guards define how users are authenticated for each request. Laravel supports multiple authentication guards (web,...
Laravel includes password reset using Password facade. Uses notifications system to send reset links. Requires...
Remember me allows users to stay logged in across sessions using secure cookie. Implemented by passing true as...
Email verification uses MustVerifyEmail interface and VerifiesEmails trait. Sends verification email on...
Sanctum provides lightweight authentication for SPAs and mobile applications. Issues API tokens, handles SPA...
Policies organize authorization logic around models or resources. Created using make:policy command. Methods...
Role-based authorization can use Gates, Policies, or packages like Spatie permissions. Define roles and permissions...
Passport provides OAuth2 server implementation. Install using composer, run migrations, generate encryption keys....
Gates are Closures that determine if user can perform action. Registered in AuthServiceProvider using...
Custom guards extend Guard contract. Register in AuthServiceProvider using Auth::extend(). Implement user() and...
Multi-authentication uses different guards for different user types. Configure multiple providers and guards in...
Policy auto-discovery automatically registers policies based on naming conventions. Can be disabled in...
Authentication events (Login, Logout, Failed, etc.) are dispatched automatically. Can be listened to using Event...
Resource authorization combines CRUD actions with policies. Use authorizeResource() in controllers. Maps controller...
Policy filters run before other policy methods. Define before() method in policy. Can grant or deny all abilities....
Token abilities define permissions for API tokens. Specified when creating token. Check using tokenCan() method....
Policy responses can return Response objects instead of booleans. Use response() helper in policies. Support custom...
Custom user providers implement UserProvider contract. Register in AuthServiceProvider using Auth::provider()....
Rate limiting uses ThrottlesLogins trait or custom middleware. Configure attempts and lockout duration. Support...
Authorization code grant requires client registration, authorization endpoint, token endpoint. Handle redirect URI,...
Contextual authorization considers additional parameters beyond user and model. Pass context to policy methods....
Passwordless auth uses signed URLs or tokens sent via email/SMS. Implement custom guard and provider. Handle token...
Hierarchical authorization handles nested permissions and inheritance. Implement tree structure for...
Session authentication can be customized by extending guard, implementing custom user provider. Handle session...
Cross-domain authentication requires coordinating sessions across domains. Handle CORS, shared tokens. Implement...
Dynamic policy resolution determines policy class at runtime. Override getPolicyFor in AuthServiceProvider. Support...
Request handling in Laravel manages HTTP requests using the Request class. It provides methods to access input data,...
Request input data can be accessed using methods like $request->input('name'), $request->get('email'), or...
Form Request Validation is a custom request class that encapsulates validation logic. Created using 'php artisan...
Request data can be validated using validate() method, Validator facade, or Form Request classes. Basic syntax:...
Common validation rules include required, string, email, min, max, between, unique, exists, regex, date, file,...
File uploads are handled using $request->file() or $request->hasFile(). Files can be validated using file, image,...
Validation errors are automatically stored in session and can be accessed in views using $errors variable. Custom...
Old input data is accessible using old() helper function or @old() Blade directive. Data is automatically flashed to...
CSRF protection prevents cross-site request forgery attacks. Laravel includes @csrf Blade directive for forms....
JSON requests can be handled using $request->json() method. Content-Type should be application/json. Use json()...
Custom validation rules can be created using 'php artisan make:rule' command. Implement passes() method for...
Array validation uses dot notation or * wildcard. Example: 'items.*.id' => 'required|integer'. Can validate nested...
Conditional validation uses sometimes(), required_if, required_unless rules. Can be based on other field values or...
Dependent field validation checks fields based on other field values. Uses required_with, required_without rules or...
Use after database hooks in Form Requests or custom validation rules. Consider transaction rollback if validation...
Custom messages can be defined in validation rules array, language files, or Form Request classes. Support...
Validation rules can accept parameters using colon syntax: 'field' => 'size:10'. Multiple parameters use comma...
Multiple files validated using array syntax and file rules. Can validate file count, size, type for each file....
Implicit validation rules (accepted, filled) validate only when field is present. Different from required rules....
Unique validation with soft deletes requires custom rule or withoutTrashed() scope. Consider restore scenarios....
Validation rules can inherit from base Form Request classes. Use trait for shared rules. Support rule overriding and...
Dynamic rules generated based on input or conditions. Use closure rules or rule objects. Support runtime rule...
Cache validation rules for performance. Consider cache invalidation strategies. Handle dynamic rules with caching....
Validation pipelines process rules sequentially. Support dependent validations. Handle validation state between...
Cross-request validation compares data across multiple requests. Use session or cache for state. Handle race...
Compose complex rules from simple ones. Support rule chaining and grouping. Handle rule dependencies and conflicts....
Version validation rules for API compatibility. Support multiple rule versions. Handle rule deprecation and...
Test validation rules using unit and feature tests. Mock dependencies. Test edge cases and error conditions. Support...
Custom validation middleware for route-level validation. Support middleware parameters. Handle validation failure...
Dispatch events before/after validation. Handle validation lifecycle. Support event listeners and subscribers....
CSRF (Cross-Site Request Forgery) protection in Laravel automatically generates and validates tokens for each active...
Laravel provides XSS (Cross-Site Scripting) protection by automatically escaping output using {{ }} Blade syntax....
Laravel prevents SQL injection using PDO parameter binding in the query builder and Eloquent ORM. Query parameters...
Laravel automatically hashes passwords using the Hash facade and bcrypt or Argon2 algorithms. Never store plain-text...
Signed routes are URLs with a signature that ensures they haven't been modified. Created using URL::signedRoute() or...
Laravel sets HTTP-only flag on cookies by default to prevent JavaScript access. Session cookies are automatically...
Mass assignment protection prevents unintended attribute modification through $fillable and $guarded properties in...
Laravel includes security headers through middleware. Headers like X-Frame-Options, X-XSS-Protection, and...
Laravel provides encryption using the Crypt facade. Data is encrypted using OpenSSL and AES-256-CBC. Encryption key...
Laravel secures sessions using encrypted cookies, CSRF protection, and secure configuration options. Sessions can be...
Rate limiting uses the throttle middleware with configurable attempt counts and time windows. Can limit by IP, user...
Secure file uploads by validating file types, size limits, and scanning for malware. Store files outside webroot....
API authentication uses tokens, OAuth, or JWT. Laravel provides Passport and Sanctum for API auth. Supports multiple...
2FA can be implemented using packages or custom solutions. Support TOTP, SMS, or email verification. Handle backup...
Implement password policies using validation rules. Check length, complexity, history. Handle password expiration...
RBAC implements authorization using roles and permissions. Can use built-in Gates and Policies or packages like...
Audit logging tracks user actions and changes. Use model events, observers, or packages. Log authentication...
CORS (Cross-Origin Resource Sharing) is handled through middleware. Configure allowed origins, methods, headers....
Secure downloads using signed URLs or tokens. Validate user permissions. Handle file streaming and range requests....
Request validation ensures input safety. Use Form Requests, validation rules. Handle file uploads securely. Prevent...
Advanced rate limiting using multiple strategies. Support token bucket, leaky bucket algorithms. Handle distributed...
Custom security headers middleware. Configure CSP, HSTS policies. Handle subresource integrity. Implement feature...
Create custom encryption providers. Support different algorithms. Handle key rotation. Implement encryption at rest....
Implement full OAuth2 server using Passport. Handle all grant types. Support scope validation. Implement token...
Security event monitoring and alerting. Track suspicious activities. Implement IDS/IPS features. Handle security...
Custom session handlers. Implement session encryption. Handle session fixation. Support session persistence....
Secure API key generation and storage. Handle key rotation and revocation. Implement key permissions. Support...
Implement security standards compliance (GDPR, HIPAA). Handle data privacy requirements. Support security audits....
Secure WebSocket authentication and authorization. Handle connection encryption. Implement message validation....
Security testing framework implementation. Vulnerability scanning integration. Penetration testing support. Security...
Artisan is Laravel's command-line interface that provides helpful commands for development. It's accessed using 'php...
Basic Artisan commands include: 'php artisan list' to show all commands, 'php artisan help' for command details,...
Custom commands are created using 'php artisan make:command CommandName'. This generates a command class in...
Command signature defines command name and arguments/options. Format: 'name:command {argument} {--option}'. Required...
Custom commands are registered in app/Console/Kernel.php in the commands property or commands() method. They can...
Arguments are required input values, options are optional flags. Define using {argument} and {--option}. Access...
Use methods like line(), info(), comment(), question(), error() for different colored output. table() for tabular...
Command scheduling allows automated command execution at specified intervals. Defined in app/Console/Kernel.php...
Use Artisan facade: Artisan::call('command:name', ['argument' => 'value']). Can queue commands using...
Command isolation ensures each command runs independently. Use separate service providers, handle dependencies...
Interactive commands use ask(), secret(), confirm() methods. Handle user input validation, provide choices using...
Use constructor injection or method injection in handle(). Laravel container automatically resolves dependencies....
Command hooks run before/after command execution. Define in base command class. Use for setup/cleanup tasks. Support...
Use try-catch blocks, report errors using error() method. Set command exit codes. Handle graceful failures. Support...
Validate arguments/options in handle() method. Use custom validation rules. Support interactive validation. Handle...
Use Artisan::call() in tests. Assert command output and behavior. Mock dependencies. Test different argument...
Group related commands using namespaces. Define command hierarchy. Support sub-commands. Register command groups in...
Dispatch events before/after command execution. Listen for command events. Handle command lifecycle. Support...
Prevent concurrent command execution using mutexes. Implement locking mechanism. Handle timeout scenarios. Support...
Queue long-running commands using queue:work. Handle command queuing logic. Support job dispatching. Implement queue...
Create custom command middleware. Handle pre/post command execution. Implement middleware pipeline. Support...
Cache command results and configuration. Handle cache invalidation. Support cache tags. Implement cache drivers....
Create pluggable command system. Support command discovery. Handle plugin registration. Implement plugin hooks....
Version commands for backwards compatibility. Handle version negotiation. Support multiple command versions....
Monitor command execution and performance. Track command usage. Implement logging and metrics. Support alerting....
Implement rollback functionality for commands. Handle transaction-like behavior. Support partial rollbacks....
Create custom generators for scaffolding. Handle template parsing. Support stub customization. Implement file...
Generate command documentation automatically. Support markdown generation. Implement help text formatting. Handle...
Chain multiple commands in pipeline. Handle data passing between commands. Support conditional execution. Implement...
Implement command-level authorization. Handle user permissions. Support role-based access. Implement policy checks....
PHPUnit is the default testing framework in Laravel. It provides a suite of tools for writing and running automated...
Tests are created using 'php artisan make:test TestName'. Two types available: Feature tests (--test suffix) and...
Feature tests focus on larger portions of code and test application behavior from user perspective. Unit tests focus...
Tests are run using 'php artisan test' or './vendor/bin/phpunit'. Can filter tests using --filter flag. Support...
Assertions verify expected outcomes in tests. Common assertions include assertTrue(), assertEquals(),...
Use get(), post(), put(), patch(), delete() methods in tests. Can chain assertions like ->assertOk(),...
Database testing uses RefreshDatabase or DatabaseTransactions traits. Tests run in transactions to prevent test data...
Tinker is an REPL for Laravel. Access using 'php artisan tinker'. Test code, interact with models, execute queries...
Factories generate fake data for testing using Faker library. Created with 'php artisan make:factory'. Define model...
Use .env.testing file for test environment. Configure test database, mail settings, queues. Use config:clear before...
Use Mockery for mocking objects. Mock facades using Facade::mock(). Bind mocks in service container. Support partial...
Use actingAs() for authenticated requests. Test login, logout, registration flows. Verify middleware protection....
Use json() method for API requests. Assert response structure, status codes. Test authentication tokens. Verify API...
Use assertPushed(), assertNotPushed() for job verification. Test job execution and chain handling. Verify job delays...
Use Event::fake() to mock events. Assert event dispatch and handling. Test event listeners in isolation. Verify...
Use Mail::fake() for mail testing. Assert mail sent and contents. Test mail templates. Verify attachments and...
Use Notification::fake(). Assert notification sending and channels. Test notification content. Verify notification...
Use UploadedFile class for fake files. Test file validation and storage. Verify file processing. Support multiple...
Use Cache::fake(). Test cache storage and retrieval. Verify cache tags and expiration. Support different cache...
Use withSession() method. Test session data persistence. Verify flash messages. Support session regeneration. Test...
Use Laravel Dusk for browser testing. Test JavaScript interactions. Support multiple browsers. Handle authentication...
Create test-specific seeders. Handle complex data relationships. Support different seeding strategies. Implement...
Organize tests into suites. Configure suite-specific setup. Handle dependencies between suites. Support parallel...
Set up CI/CD pipelines. Configure test automation. Handle environment setup. Support different test stages....
Measure application performance metrics. Test response times and throughput. Profile database queries. Monitor...
Test security vulnerabilities. Implement penetration testing. Verify authentication security. Test authorization...
Generate API documentation from tests. Verify API specifications. Test API versioning. Support OpenAPI/Swagger...
Use mutation testing frameworks. Verify test coverage quality. Identify weak test cases. Support automated mutation...
Test application under heavy load. Verify system stability. Monitor resource usage. Implement crash recovery. Handle...
Maintain test suite for existing features. Automate regression checks. Handle backward compatibility. Support...
Caching in Laravel stores and retrieves data for faster access. Laravel supports multiple cache drivers (file,...
Basic cache operations include Cache::get() to retrieve, Cache::put() to store, Cache::has() to check existence,...
Route caching improves routing performance using 'php artisan route:cache'. Creates a single file of compiled...
Config caching combines all configuration files into single cached file using 'php artisan config:cache'. Improves...
View caching compiles Blade templates into PHP code. Happens automatically and stored in storage/framework/views....
Database queries can be cached using remember() method on query builder or Eloquent models. Example:...
Cache tags group related items for easy manipulation. Use Cache::tags(['tag'])->put() for storage. Can flush all...
Eager loading reduces N+1 query problems by loading relationships in advance using with() method. Example:...
Response caching stores HTTP responses using middleware. Configure using Cache-Control headers. Supports client-side...
Cache keys uniquely identify cached items. Use meaningful names and version prefixes. Handle key collisions. Support...
Cache versioning handles cache invalidation using version prefixes in keys. Increment version to invalidate all...
Distributed caching uses Redis or Memcached across multiple servers. Handle cache synchronization. Support cache...
Database optimization includes proper indexing, query optimization, using chunking for large datasets, implementing...
Fragment caching stores portions of views. Use @cache directive in Blade. Support time-based expiration. Handle...
Cache warming pre-populates cache before needed. Create warming scripts. Handle cache dependencies. Support...
Asset optimization includes minification, compression, bundling using Laravel Mix. Configure cache headers. Use CDN...
Cache policies define caching rules and strategies. Handle cache lifetime. Support conditional caching. Implement...
Queue optimization improves job processing performance. Configure worker processes. Handle job batching. Implement...
Session optimization includes choosing appropriate driver, configuring session lifetime, handling session cleanup,...
Cache locks prevent race conditions in distributed systems. Use Cache::lock() method. Handle lock timeouts....
Profile application using tools like Laravel Telescope, Clockwork. Monitor performance metrics. Track database...
Cache sharding distributes cache across multiple nodes. Implement shard selection. Handle shard rebalancing. Support...
Hierarchical caching uses multiple cache layers. Implement cache fallback. Handle cache propagation. Support cache...
Memory optimization includes monitoring allocations, implementing garbage collection, optimizing data structures,...
Cache events track cache operations. Handle cache hits/misses. Implement cache warming events. Support event...
Load balancing distributes traffic across servers. Configure load balancer. Handle session affinity. Support health...
API optimization includes implementing rate limiting, caching responses, optimizing serialization, handling...
Cache warm-up strategies include identifying critical data, implementing progressive warming, handling cache...
File system optimization includes caching file operations, implementing proper file handling, optimizing storage...
Performance monitoring includes tracking metrics, implementing logging, setting up alerts, analyzing trends,...
Queues allow deferring time-consuming tasks for background processing. Laravel supports various queue drivers...
Jobs are created using 'php artisan make:job JobName'. Jobs implement ShouldQueue interface. Define handle() method...
Queue workers are run using 'php artisan queue:work'. Can specify connection, queue name, and other options. Should...
Job dispatch sends jobs to queue for processing. Can use dispatch() helper, Job::dispatch(), or DispatchesJobs...
Failed jobs are tracked in failed_jobs table. Handle failures using failed() method in job class. Can retry failed...
Job middleware intercept job processing. Define middleware in job's middleware() method. Can rate limit, throttle,...
Job chains execute jobs in sequence using Chain::with(). Later jobs run only if previous ones succeed. Can set chain...
Queue connections are configured in config/queue.php. Define driver, connection parameters. Support multiple...
Job batching processes multiple jobs as group using Bus::batch(). Track batch progress. Handle batch completion and...
Job events track job lifecycle. Listen for job processed, failed events. Handle queue events in...
Rate limit jobs using middleware like RateLimited. Configure limits per minute/hour. Handle rate limit exceeded...
Set job timeout using timeout property or through command. Handle timeout exceptions. Implement graceful shutdown....
Unique jobs prevent duplicate processing using ShouldBeUnique interface. Define uniqueness criteria. Handle lock...
Track job progress using batch processing or custom tracking. Update progress in database. Broadcast progress...
Manage job dependencies using job chaining or custom logic. Handle dependent job failures. Support conditional job...
Lifecycle hooks handle job events like preparing, processing, failed. Implement before/after processing logic....
Monitor queue using Horizon or custom solutions. Track queue size, processing time. Set up alerts. Handle queue...
Prioritize jobs using multiple queues. Configure queue priorities. Handle high-priority job processing. Support...
Configure supervisor to manage queue workers. Set up worker processes. Handle worker failures. Monitor worker...
Scale queues using multiple workers. Handle worker balancing. Implement auto-scaling. Monitor queue performance....
Process jobs across multiple servers. Handle job distribution. Implement job coordination. Support distributed...
Version jobs for compatibility. Handle job upgrades. Support multiple versions. Implement version migration. Monitor...
Create complex scheduling patterns. Handle recurring jobs. Support conditional scheduling. Implement schedule...
Optimize job processing speed. Handle memory management. Implement queue sharding. Support batch optimization....
Manage job state across executions. Handle state persistence. Implement state recovery. Support state transitions....
Create robust error handling. Implement retry strategies. Handle permanent failures. Support error notification....
Build custom monitoring solutions. Track queue metrics. Implement alerting system. Support dashboard visualization....
Test queue jobs effectively. Mock queue operations. Verify job behavior. Support integration testing. Monitor test coverage.
Secure queue operations. Handle job authentication. Implement authorization. Support encryption. Monitor security threats.
Plan for queue failures. Implement backup strategies. Handle recovery procedures. Support failover mechanisms....
Routing in Laravel refers to defining URL paths that users can access and mapping them to specific controller actions or closure callbacks. Routes are defined in files within the routes directory, primarily in web.php and api.php.
Laravel supports common HTTP methods: GET for retrieving data, POST for creating new resources, PUT/PATCH for updating existing resources, DELETE for removing resources. These can be defined using Route::get(), Route::post(), Route::put(), Route::patch(), and Route::delete().
A controller is a PHP class that handles business logic and acts as an intermediary between Models and Views. Controllers group related request handling logic into a single class, organizing code better than closure routes.
Controllers can be created using the Artisan command: php artisan make:controller ControllerName. This creates a new controller class in app/Http/Controllers directory with basic structure and necessary imports.
Route parameters are dynamic segments in URLs that capture and pass values to controller methods. They are defined using curly braces in route definitions, like '/user/{id}' where {id} is the parameter.
Routes can be named using the name() method: Route::get('/profile', [ProfileController::class, 'show'])->name('profile'). Named routes provide a convenient way to generate URLs or redirects using route('profile').
web.php contains routes for web interface and includes session state and CSRF protection. api.php is for stateless API routes, includes rate limiting, and is prefixed with '/api'. api.php routes don't include session or CSRF middleware.
Request data can be accessed using the Request facade or by type-hinting Request in controller methods. Example: public function store(Request $request) { $name = $request->input('name'); }
Route grouping allows you to share route attributes (middleware, prefixes, namespaces) across multiple routes. Routes can be grouped using Route::group() or using the Route::prefix() method.
Redirects can be performed using redirect() helper or Route::redirect(). Examples: return redirect('/home') or Route::redirect('/here', '/there'). You can also redirect to named routes using redirect()->route('name').
Resource controllers handle CRUD operations for a resource. Created using 'php artisan make:controller ResourceController --resource', they provide methods like index(), create(), store(), show(), edit(), update(), and destroy(). They're bound to routes using Route::resource().
Route model binding automatically resolves Eloquent models from route parameters. It can be implicit (type-hint the model) or explicit (define in RouteServiceProvider). Example: Route::get('/users/{user}', function (User $user) { return $user; }).
Controller middleware filter HTTP requests before they reach controllers. They can be assigned using the middleware method in controllers or in route definitions. They're defined in app/Http/Kernel.php and can be applied to specific methods using 'only' and 'except'.
File uploads are handled using the $request->file() method. Files can be stored using Storage facade or move() method. Example: if($request->hasFile('photo')) { $path = $request->file('photo')->store('uploads'); }
Route constraints limit how route parameters may be matched using regex patterns. They can be applied using where() method or globally in RouteServiceProvider. Example: Route::get('user/{id}')->where('id', '[0-9]+').
CORS can be handled using the cors middleware and configuration in config/cors.php. Laravel provides built-in CORS support through the fruitcake/laravel-cors package. Headers and allowed origins can be configured as needed.
Dependency injection in controllers automatically resolves class dependencies in constructor or method parameters through Laravel's service container. This enables better testing and loose coupling of components.
API versioning can be implemented using route prefixes, subdomains, or headers. Common approaches include using route groups with prefixes like 'api/v1/' or namespace-based versioning in different controllers.
Signed routes are URLs with a signature hash to verify they haven't been modified. Created using URL::signedRoute(), they're useful for email verification, temporary access links, or public sharing of protected resources.
Rate limiting is implemented using throttle middleware. It can be configured in RouteServiceProvider and customized using the RateLimiter facade. Limits can be set per minute/hour and customized per user or IP.
Custom route model binding can be implemented by overriding the resolveRouteBinding() method in models or by defining custom binders in RouteServiceProvider's boot method using Route::bind().
Domain routing is implemented using Route::domain(). Subdomains can capture parameters: Route::domain('{account}.example.com')->group(function () {}). Wildcard subdomains and pattern matching are supported.
Custom response macros extend Response functionality using Response::macro() in a service provider. Example: Response::macro('caps', function ($value) { return Response::make(strtoupper($value)); });
Route caching improves performance using 'php artisan route:cache'. It requires all route closures to be converted to controller methods. Route cache must be cleared when routes change using 'route:clear'.
Custom middleware parameters are implemented by adding additional parameters to handle() method. In routes: ->middleware('role:admin,editor'). In middleware: handle($request, $next, ...$roles).
Route fallbacks are implemented using Route::fallback(). Custom 404 handling can be done by overriding the render() method in App\Exceptions\Handler or creating custom exception handlers.
Conditional middleware can be implemented using middleware() with when() or unless() methods. Can also be done by logic in middleware handle() method or by creating custom middleware classes with conditions.
API resource collections with conditional relationships use whenLoaded() method and conditional attribute inclusion. Custom collection classes can be created to handle complex transformations and relationship loading.
Multiple parameter binding can be implemented using explicit route model binding in RouteServiceProvider, or by implementing custom resolution logic in resolveRouteBinding(). Supports nested and dependent bindings.
Soft deleted models in route binding can be included using withTrashed() scope. Custom resolution logic can be implemented in resolveRouteBinding() to handle different scenarios of soft deleted models.
Blade is Laravel's templating engine that combines PHP with HTML templates. It provides convenient shortcuts for common PHP control structures, template inheritance, and component features while being compiled into plain PHP code for better performance.
Variables in Blade templates are displayed using double curly braces syntax {{ $variable }}. The content within braces is automatically escaped to prevent XSS attacks. For unescaped content, use {!! $variable !!}.
Blade template inheritance allows creating a base layout template (@extends) with defined sections (@section) that child templates can override or extend. Child templates use @extends('layout') to inherit and @section to provide content.
Sub-views can be included using @include('view.name') directive. You can also pass data to included views: @include('view.name', ['data' => $data]). For performance, use @includeIf, @includeWhen, or @includeUnless.
Blade provides convenient directives for control structures: @if, @else, @elseif, @endif for conditionals; @foreach, @while for loops; @switch, @case for switch statements. These compile to regular PHP control structures.
Blade supports loops using @foreach, @for, @while directives. The $loop variable is available inside foreach loops, providing information like index, iteration, first, last, and depth for nested loops.
The @yield directive displays content of a specified section. It's typically used in layout templates to define places where child views can inject content. It can also specify default content if section is not defined.
Laravel provides the asset() helper function to generate URLs for assets. Assets are stored in the public directory and can be referenced using asset('css/app.css'). For versioning, use mix() with Laravel Mix.
Blade comments are written using {{-- comment --}} syntax. Unlike HTML comments, Blade comments are not included in the HTML rendered to the browser, making them useful for developer notes.
Blade Components are reusable template pieces with isolated logic. Created using 'php artisan make:component', they combine a class for logic and a view for markup. Used with x- prefix: <x-alert type='error'>Message</x-alert>.
Stacks in Blade allow pushing content to named locations using @push and @stack directives. Multiple @push calls can add to same stack. Useful for scripts and styles that need to be included at specific points in layout.
Blade Service Injection allows injecting services into views using @inject directive. Example: @inject('metrics', 'App\Services\MetricsService'). The service is resolved from container and available in view.
Form validation errors are available through $errors variable. Common patterns include @error directive for specific fields and $errors->any() to check for any errors. Errors persist for one redirect via session.
Laravel Mix is a webpack wrapper that simplifies asset compilation. It's configured in webpack.mix.js and provides features for compiling, versioning, and combining CSS/JS. Used with mix() helper in templates.
Custom Blade directives are created in a service provider using Blade::directive(). They transform template syntax into PHP code. Example: Blade::directive('datetime', function ($expression) { return "<?php echo date('Y-m-d', $expression); ?>"; });
Slots allow passing content to components. Default slot uses <x-slot> tag, named slots use name attribute. Components can have multiple slots and default content. Useful for flexible component layouts.
Blade provides @auth and @guest directives for authentication checks. Additional directives like @can, @cannot for authorization. Can be combined with roles/permissions: @can('update', $post).
View Composers bind data to views whenever they're rendered. Registered in service providers using View::composer(). Useful for sharing data across multiple views without passing from controllers.
Localization uses @lang directive or __() helper for translations. Supports string replacement, pluralization, and JSON language files. Configure locale in config/app.php or change dynamically.
Dynamic components can be rendered using <x-dynamic-component :component="$componentName">. Component name can be determined at runtime. Useful for flexible UIs and plugin systems.
Attributes Bag ($attributes) manages additional attributes passed to components. Supports merging, filtering, and getting first/last. Example: <div {{ $attributes->merge(['class' => 'default']) }}>.
Anonymous components are created without class files using single Blade templates. Stored in resources/views/components. Support props through variables defined at top of template using @props directive.
Components can be organized in subdirectories and namespaced. Configure component namespaces in service provider using Blade::componentNamespace(). Allows package vendors to register component namespaces.
Components support lazy loading using wire:init or defer loading until needed. Useful for performance optimization. Can combine with placeholder loading states and transitions.
Custom if statements are added using Blade::if() in service provider. Can encapsulate complex conditional logic in reusable directives. Example: Blade::if('env', function ($environment) { return app()->environment($environment); });
Component methods can use dependency injection through method parameters. Laravel automatically resolves dependencies from container. Useful for accessing services within component methods.
Components have rendering lifecycle hooks like mount(), rendering(), rendered(). Can modify component state and attributes during render cycle. Useful for complex component behavior.
Components can be autoloaded using package discovery or manual registration in service providers. Support for component aliases, custom paths, and conditional loading based on environment.
Custom template compilation can be implemented by extending Blade compiler. Add custom compilation passes, modify existing directives, or add preprocessing steps. Requires understanding of Laravel's compilation process.
Eloquent ORM (Object-Relational Mapping) is Laravel's built-in ORM that allows developers to interact with database tables using elegant object-oriented models. Each database table has a corresponding Model that is used for interacting with that table.
Models can be created using Artisan command: 'php artisan make:model ModelName'. Adding -m flag creates a migration file too. Models are placed in app/Models directory and extend Illuminate\Database\Eloquent\Model class.
Migrations are like version control for databases, allowing you to define and modify database schema using PHP code. They enable team collaboration by maintaining consistent database structure across different development environments.
Basic Eloquent operations include: Model::all() to retrieve all records, Model::find(id) to find by primary key, Model::create([]) to create new records, save() to update, and delete() to remove records.
Relationships are defined as methods in model classes. Common types include hasOne(), hasMany(), belongsTo(), belongsToMany(). These methods specify how models are related to each other in the database.
Seeders are used to populate database tables with sample or initial data. They are created using 'php artisan make:seeder' and can be run using 'php artisan db:seed'. Useful for testing and initial application setup.
Eloquent provides methods like where(), orWhere(), whereBetween(), orderBy() for querying. Example: User::where('active', 1)->orderBy('name')->get(). Queries return collections of model instances.
Mass assignment allows setting multiple model attributes at once. $fillable property in models specifies which attributes can be mass assigned, while $guarded specifies which cannot. This prevents unintended attribute modifications.
Database connections are configured in config/database.php and .env file. Multiple connections can be defined for different databases. The default connection is specified in .env using DB_CONNECTION.
Model factories generate fake data for testing and seeding. Created using 'php artisan make:factory'. They use Faker library to generate realistic test data. Factories can define states for different scenarios.
Eager loading reduces N+1 query problems by loading relationships in advance using with() method. Example: User::with('posts')->get(). Can eager load multiple or nested relationships: with(['posts', 'profile']).
Model events are triggered during various stages of a model's lifecycle (creating, created, updating, etc.). Observers group event listeners for a model in a single class. Created using 'php artisan make:observer'.
Soft deletes add deleted_at timestamp instead of actually deleting records. Use SoftDeletes trait in model and add deleted_at column in migration. Provides withTrashed(), onlyTrashed() scopes for querying.
Query scopes are reusable query constraints. Global scopes automatically apply to all queries. Local scopes are methods prefixed with 'scope' and can be chained. Example: public function scopeActive($query) { return $query->where('active', 1); }
Polymorphic relationships allow a model to belong to multiple types of models. Uses morphTo(), morphMany(), morphToMany() methods. Requires type and ID columns in database. Common for comments, likes, tags scenarios.
Accessors and mutators modify attribute values when retrieving or setting them. Defined using get{Attribute}Attribute and set{Attribute}Attribute methods. Useful for formatting dates, calculating values, or encoding data.
Transactions ensure multiple database operations succeed or fail as a unit. Use DB::transaction() or beginTransaction(), commit(), rollback(). Can be nested. Important for maintaining data integrity.
Pivot tables implement many-to-many relationships. Created using createPivotTable migration method. Can have additional columns accessed via withPivot(). Pivot model can be customized using using() method.
Query results can be cached using remember() or rememberForever() methods. Cache duration and key can be specified. Useful for expensive queries. Example: User::remember(60)->get();
Subqueries can be used in selects and joins using addSelect() and joinSub(). Useful for complex queries involving aggregates or related data. Can improve performance over multiple queries.
Custom collections extend Illuminate\Database\Eloquent\Collection. Override newCollection() in model to use custom collection. Add methods for specialized collection operations specific to model type.
Models can be replicated using replicate() method. Specify which attributes to exclude. Handle relationships manually. Useful for creating similar records with slight modifications.
Custom model binding resolvers modify how models are resolved from route parameters. Defined in RouteServiceProvider using Route::bind() or by overriding resolveRouteBinding() in model.
Composite keys require overriding getKeyName() and getIncrementing(). Additional configuration needed for relationships. Consider performance implications. May need custom query scopes.
Custom query builders extend Illuminate\Database\Eloquent\Builder. Override newEloquentBuilder() in model. Add methods for specialized query operations. Useful for complex, reusable queries.
Customize toArray() and toJson() methods. Use hidden and visible properties. Implement custom casts for complex attributes. Handle relationship serialization. Consider API resource classes.
Sharding requires custom connection resolvers. Override getConnection() in models. Implement logic for determining shard. Consider transaction and relationship implications across shards.
Real-time observers can broadcast model changes using events. Implement ShouldBroadcast interface. Configure broadcast driver. Handle authentication and authorization for broadcasts.
Recursive relationships use self-referential associations. Implement methods for traversing tree structures. Consider performance with nested eager loading. Use closure table pattern for complex hierarchies.
Custom connection handling requires extending Connection class. Implement custom query grammar and processor. Handle transaction management. Consider read/write splitting scenarios.
Laravel provides a complete authentication system out of the box using the Auth facade. It includes features for user registration, login, password reset, and remember me functionality. Can be scaffolded using laravel/ui or breeze/jetstream packages.
Basic authentication can be implemented using Auth::attempt(['email' => $email, 'password' => $password]) for login, Auth::login($user) for manual login, and Auth::logout() for logging out. Session-based authentication is default.
Auth middleware (auth) protects routes by ensuring users are authenticated. Can be applied to routes or controllers using middleware('auth'). Redirects unauthenticated users to login page or returns 401 for API routes.
Use Auth::check() to verify authentication status, Auth::user() to get current user, or @auth/@guest Blade directives in views. Request object also provides auth()->user() helper.
Guards define how users are authenticated for each request. Laravel supports multiple authentication guards (web, api) configured in config/auth.php. Each guard specifies provider and driver for authentication.
Laravel includes password reset using Password facade. Uses notifications system to send reset links. Requires password_resets table. Can customize views, expiration time, and throttling.
Remember me allows users to stay logged in across sessions using secure cookie. Implemented by passing true as second parameter to Auth::attempt() or using remember() method. Requires remember_token column.
Email verification uses MustVerifyEmail interface and VerifiesEmails trait. Sends verification email on registration. Can protect routes with verified middleware. Customizable verification notice and email.
Sanctum provides lightweight authentication for SPAs and mobile applications. Issues API tokens, handles SPA authentication through cookies. Supports multiple tokens per user with different abilities.
Policies organize authorization logic around models or resources. Created using make:policy command. Methods correspond to actions (view, create, update, delete). Used with Gate facade or @can directive.
Role-based authorization can use Gates, Policies, or packages like Spatie permissions. Define roles and permissions in database. Check using can() method or middleware. Support multiple roles per user.
Passport provides OAuth2 server implementation. Install using composer, run migrations, generate encryption keys. Supports password grant, authorization code grant, and personal access tokens.
Gates are Closures that determine if user can perform action. Registered in AuthServiceProvider using Gate::define(). Can use Gate::allows() or $user->can() to check authorization. Support custom parameters.
Custom guards extend Guard contract. Register in AuthServiceProvider using Auth::extend(). Implement user() and validate() methods. Configure in auth.php. Useful for specialized authentication needs.
Multi-authentication uses different guards for different user types. Configure multiple providers and guards in auth.php. Use guard() method to specify guard. Support separate sessions and authentication logic.
Policy auto-discovery automatically registers policies based on naming conventions. Can be disabled in AuthServiceProvider. Override getPolicyFor() for custom mapping. Supports policy discovery in packages.
Authentication events (Login, Logout, Failed, etc.) are dispatched automatically. Can be listened to using Event facade or subscribers. Useful for logging, notifications, or additional security measures.
Resource authorization combines CRUD actions with policies. Use authorizeResource() in controllers. Maps controller methods to policy methods. Supports automatic authorization using middleware.
Policy filters run before other policy methods. Define before() method in policy. Can grant or deny all abilities. Useful for super-admin scenarios or global authorization rules.
Token abilities define permissions for API tokens. Specified when creating token. Check using tokenCan() method. Support multiple abilities per token. Can be combined with other authorization methods.
Policy responses can return Response objects instead of booleans. Use response() helper in policies. Support custom messages and status codes. Useful for detailed authorization feedback.
Custom user providers implement UserProvider contract. Register in AuthServiceProvider using Auth::provider(). Implement retrieveById, retrieveByToken, updateRememberToken methods. Support non-database authentication.
Rate limiting uses ThrottlesLogins trait or custom middleware. Configure attempts and lockout duration. Support IP-based and user-based throttling. Can customize decay time and storage.
Authorization code grant requires client registration, authorization endpoint, token endpoint. Handle redirect URI, state parameter, PKCE. Support refresh tokens and token revocation. Implement scope validation.
Contextual authorization considers additional parameters beyond user and model. Pass context to policy methods. Support complex authorization rules. Can use additional services or external APIs.
Passwordless auth uses signed URLs or tokens sent via email/SMS. Implement custom guard and provider. Handle token generation and verification. Support expiration and single-use tokens.
Hierarchical authorization handles nested permissions and inheritance. Implement tree structure for roles/permissions. Support permission propagation. Handle circular dependencies and performance.
Session authentication can be customized by extending guard, implementing custom user provider. Handle session storage, regeneration. Support custom session drivers and authentication logic.
Cross-domain authentication requires coordinating sessions across domains. Handle CORS, shared tokens. Implement single sign-on. Support token forwarding and validation across domains.
Dynamic policy resolution determines policy class at runtime. Override getPolicyFor in AuthServiceProvider. Support multiple policy implementations. Handle policy resolution cache.
Request handling in Laravel manages HTTP requests using the Request class. It provides methods to access input data, files, headers, and server variables. Request handling is the foundation of processing user input in Laravel applications.
Request input data can be accessed using methods like $request->input('name'), $request->get('email'), or $request->all(). For specific input types, use $request->query() for GET parameters and $request->post() for POST data.
Form Request Validation is a custom request class that encapsulates validation logic. Created using 'php artisan make:request'. Contains rules() method for validation rules and authorize() method for authorization checks.
Request data can be validated using validate() method, Validator facade, or Form Request classes. Basic syntax: $validated = $request->validate(['field' => 'rule|rule2']). Failed validation redirects back with errors.
Common validation rules include required, string, email, min, max, between, unique, exists, regex, date, file, image, and numeric. Rules can be combined using pipe (|) or array syntax.
File uploads are handled using $request->file() or $request->hasFile(). Files can be validated using file, image, mimes rules. Use store() or storeAs() methods to save uploaded files.
Validation errors are automatically stored in session and can be accessed in views using $errors variable. Custom error messages can be defined in validation rules or language files.
Old input data is accessible using old() helper function or @old() Blade directive. Data is automatically flashed to session on validation failure. Useful for repopulating forms.
CSRF protection prevents cross-site request forgery attacks. Laravel includes @csrf Blade directive for forms. VerifyCsrfToken middleware validates token. Can be disabled for specific routes.
JSON requests can be handled using $request->json() method. Content-Type should be application/json. Use json() method for responses. APIs typically return JSON responses by default.
Custom validation rules can be created using 'php artisan make:rule' command. Implement passes() method for validation logic. Can include custom error message. Register in service provider if needed.
Array validation uses dot notation or * wildcard. Example: 'items.*.id' => 'required|integer'. Can validate nested arrays and specific array keys. Supports array size validation.
Conditional validation uses sometimes(), required_if, required_unless rules. Can be based on other field values or custom conditions. Supports complex validation scenarios based on input data.
Dependent field validation checks fields based on other field values. Uses required_with, required_without rules or custom validation logic. Can implement complex dependencies between fields.
Use after database hooks in Form Requests or custom validation rules. Consider transaction rollback if validation fails. Handle unique rule with database transactions carefully.
Custom messages can be defined in validation rules array, language files, or Form Request classes. Support placeholders for field names and values. Can be localized for different languages.
Validation rules can accept parameters using colon syntax: 'field' => 'size:10'. Multiple parameters use comma separation. Can reference other field values or custom parameters.
Multiple files validated using array syntax and file rules. Can validate file count, size, type for each file. Support batch processing and individual file validation.
Implicit validation rules (accepted, filled) validate only when field is present. Different from required rules. Useful for optional fields that need specific format when present.
Unique validation with soft deletes requires custom rule or withoutTrashed() scope. Consider restore scenarios. Handle unique constraints across active and deleted records.
Validation rules can inherit from base Form Request classes. Use trait for shared rules. Support rule overriding and extension. Handle rule conflicts and dependencies.
Dynamic rules generated based on input or conditions. Use closure rules or rule objects. Support runtime rule modification. Handle complex validation scenarios.
Cache validation rules for performance. Consider cache invalidation strategies. Handle dynamic rules with caching. Support cache tags and versioning.
Validation pipelines process rules sequentially. Support dependent validations. Handle validation state between steps. Implement rollback mechanisms.
Cross-request validation compares data across multiple requests. Use session or cache for state. Handle race conditions. Support sequential validation steps.
Compose complex rules from simple ones. Support rule chaining and grouping. Handle rule dependencies and conflicts. Implement custom rule factories.
Version validation rules for API compatibility. Support multiple rule versions. Handle rule deprecation and migration. Implement version negotiation.
Test validation rules using unit and feature tests. Mock dependencies. Test edge cases and error conditions. Support test data providers.
Custom validation middleware for route-level validation. Support middleware parameters. Handle validation failure responses. Implement middleware groups.
Dispatch events before/after validation. Handle validation lifecycle. Support event listeners and subscribers. Implement custom validation events.
CSRF (Cross-Site Request Forgery) protection in Laravel automatically generates and validates tokens for each active user session. It's implemented through the VerifyCsrfToken middleware and @csrf Blade directive in forms.
Laravel provides XSS (Cross-Site Scripting) protection by automatically escaping output using {{ }} Blade syntax. HTML entities are converted to prevent script injection. Use {!! !!} for trusted content that needs to render HTML.
Laravel prevents SQL injection using PDO parameter binding in the query builder and Eloquent ORM. Query parameters are automatically escaped. Never concatenate strings directly into queries.
Laravel automatically hashes passwords using the Hash facade and bcrypt or Argon2 algorithms. Never store plain-text passwords. Password hashing is handled by the HashedAttributes trait in the User model.
Signed routes are URLs with a signature that ensures they haven't been modified. Created using URL::signedRoute() or URL::temporarySignedRoute(). Useful for email verification or temporary access links.
Laravel sets HTTP-only flag on cookies by default to prevent JavaScript access. Session cookies are automatically HTTP-only. Config can be modified in config/session.php.
Mass assignment protection prevents unintended attribute modification through $fillable and $guarded properties in models. Attributes must be explicitly marked as fillable to allow mass assignment.
Laravel includes security headers through middleware. Headers like X-Frame-Options, X-XSS-Protection, and X-Content-Type-Options are set by default. Additional headers can be added via middleware.
Laravel provides encryption using the Crypt facade. Data is encrypted using OpenSSL and AES-256-CBC. Encryption key is stored in .env file. All encrypted values are signed to prevent tampering.
Laravel secures sessions using encrypted cookies, CSRF protection, and secure configuration options. Sessions can be stored in various drivers (file, database, Redis). Session IDs are regularly rotated.
Rate limiting uses the throttle middleware with configurable attempt counts and time windows. Can limit by IP, user ID, or custom parameters. Supports Redis for distributed applications.
Secure file uploads by validating file types, size limits, and scanning for malware. Store files outside webroot. Use Storage facade for safe file operations. Implement proper permissions.
API authentication uses tokens, OAuth, or JWT. Laravel provides Passport and Sanctum for API auth. Supports multiple authentication guards and token abilities.
2FA can be implemented using packages or custom solutions. Support TOTP, SMS, or email verification. Handle backup codes and device remembering. Integrate with authentication flow.
Implement password policies using validation rules. Check length, complexity, history. Handle password expiration and rotation. Support password strength indicators.
RBAC implements authorization using roles and permissions. Can use built-in Gates and Policies or packages like Spatie Permissions. Support hierarchical roles and permission inheritance.
Audit logging tracks user actions and changes. Use model events, observers, or packages. Log authentication attempts, data modifications. Support audit trail review and reporting.
CORS (Cross-Origin Resource Sharing) is handled through middleware. Configure allowed origins, methods, headers. Support preflight requests. Handle credentials and caching.
Secure downloads using signed URLs or tokens. Validate user permissions. Handle file streaming and range requests. Implement download tracking and rate limiting.
Request validation ensures input safety. Use Form Requests, validation rules. Handle file uploads securely. Prevent mass assignment vulnerabilities. Sanitize input data.
Advanced rate limiting using multiple strategies. Support token bucket, leaky bucket algorithms. Handle distributed rate limiting. Implement custom response headers.
Custom security headers middleware. Configure CSP, HSTS policies. Handle subresource integrity. Implement feature policies. Support header reporting.
Create custom encryption providers. Support different algorithms. Handle key rotation. Implement encryption at rest. Support HSM integration.
Implement full OAuth2 server using Passport. Handle all grant types. Support scope validation. Implement token management. Handle client credentials.
Security event monitoring and alerting. Track suspicious activities. Implement IDS/IPS features. Handle security incident response. Support forensics.
Custom session handlers. Implement session encryption. Handle session fixation. Support session persistence. Implement session cleanup.
Secure API key generation and storage. Handle key rotation and revocation. Implement key permissions. Support multiple key types. Handle key distribution.
Implement security standards compliance (GDPR, HIPAA). Handle data privacy requirements. Support security audits. Implement compliance reporting.
Secure WebSocket authentication and authorization. Handle connection encryption. Implement message validation. Support secure broadcasting.
Security testing framework implementation. Vulnerability scanning integration. Penetration testing support. Security test automation. Compliance testing.
Artisan is Laravel's command-line interface that provides helpful commands for development. It's accessed using 'php artisan' and includes commands for database migrations, cache clearing, job processing, and other common tasks.
Basic Artisan commands include: 'php artisan list' to show all commands, 'php artisan help' for command details, 'php artisan serve' to start development server, 'php artisan tinker' for REPL, and 'php artisan make' for generating files.
Custom commands are created using 'php artisan make:command CommandName'. This generates a command class in app/Console/Commands. Define command signature and description, implement handle() method for command logic.
Command signature defines command name and arguments/options. Format: 'name:command {argument} {--option}'. Required arguments in curly braces, optional in square brackets. Options prefixed with --.
Custom commands are registered in app/Console/Kernel.php in the commands property or commands() method. They can also be registered using $this->load() method to auto-register all commands in a directory.
Arguments are required input values, options are optional flags. Define using {argument} and {--option}. Access using $this->argument() and $this->option() in handle() method. Can have default values.
Use methods like line(), info(), comment(), question(), error() for different colored output. table() for tabular data, progressBar() for progress indicators. All methods available through Command class.
Command scheduling allows automated command execution at specified intervals. Defined in app/Console/Kernel.php schedule() method. Uses cron expressions or fluent interface. Requires cron entry for schedule:run.
Use Artisan facade: Artisan::call('command:name', ['argument' => 'value']). Can queue commands using Artisan::queue(). Get command output using Artisan::output().
Command isolation ensures each command runs independently. Use separate service providers, handle dependencies properly. Important for testing and avoiding side effects between commands.
Interactive commands use ask(), secret(), confirm() methods. Handle user input validation, provide choices using choice() method. Support default values and validation callbacks.
Use constructor injection or method injection in handle(). Laravel container automatically resolves dependencies. Can bind interfaces to implementations in service providers.
Command hooks run before/after command execution. Define in base command class. Use for setup/cleanup tasks. Support global hooks for all commands. Handle error cases.
Use try-catch blocks, report errors using error() method. Set command exit codes. Handle graceful failures. Support retry logic. Implement proper cleanup on failure.
Validate arguments/options in handle() method. Use custom validation rules. Support interactive validation. Handle validation failures gracefully. Provide helpful error messages.
Use Artisan::call() in tests. Assert command output and behavior. Mock dependencies. Test different argument combinations. Verify side effects.
Group related commands using namespaces. Define command hierarchy. Support sub-commands. Register command groups in console kernel. Handle group-level options.
Dispatch events before/after command execution. Listen for command events. Handle command lifecycle. Support event-driven command execution. Implement event listeners.
Prevent concurrent command execution using mutexes. Implement locking mechanism. Handle timeout scenarios. Support distributed locks. Release locks properly.
Queue long-running commands using queue:work. Handle command queuing logic. Support job dispatching. Implement queue priorities. Handle failed queued commands.
Create custom command middleware. Handle pre/post command execution. Implement middleware pipeline. Support middleware parameters. Register middleware globally or per command.
Cache command results and configuration. Handle cache invalidation. Support cache tags. Implement cache drivers. Handle distributed caching scenarios.
Create pluggable command system. Support command discovery. Handle plugin registration. Implement plugin hooks. Support plugin configuration.
Version commands for backwards compatibility. Handle version negotiation. Support multiple command versions. Implement version deprecation. Handle version migration.
Monitor command execution and performance. Track command usage. Implement logging and metrics. Support alerting. Handle monitoring in distributed systems.
Implement rollback functionality for commands. Handle transaction-like behavior. Support partial rollbacks. Implement cleanup on failure. Handle distributed rollbacks.
Create custom generators for scaffolding. Handle template parsing. Support stub customization. Implement file generation logic. Handle naming conventions.
Generate command documentation automatically. Support markdown generation. Implement help text formatting. Handle multilingual documentation. Support interactive help.
Chain multiple commands in pipeline. Handle data passing between commands. Support conditional execution. Implement pipeline recovery. Handle pipeline monitoring.
Implement command-level authorization. Handle user permissions. Support role-based access. Implement policy checks. Handle authorization failures.
PHPUnit is the default testing framework in Laravel. It provides a suite of tools for writing and running automated tests. Laravel extends PHPUnit with additional assertions and helper methods for testing applications.
Tests are created using 'php artisan make:test TestName'. Two types available: Feature tests (--test suffix) and Unit tests (--unit flag). Tests extend TestCase class and are stored in tests directory.
Feature tests focus on larger portions of code and test application behavior from user perspective. Unit tests focus on individual classes or methods in isolation. Feature tests typically test HTTP requests, while unit tests verify specific functionality.
Tests are run using 'php artisan test' or './vendor/bin/phpunit'. Can filter tests using --filter flag. Support parallel testing with --parallel option. Generate coverage reports with --coverage flag.
Assertions verify expected outcomes in tests. Common assertions include assertTrue(), assertEquals(), assertDatabaseHas(). Laravel adds web-specific assertions like assertStatus(), assertViewIs(), assertJson().
Use get(), post(), put(), patch(), delete() methods in tests. Can chain assertions like ->assertOk(), ->assertRedirect(). Submit forms using call() method with request data.
Database testing uses RefreshDatabase or DatabaseTransactions traits. Tests run in transactions to prevent test data persistence. Use factories to generate test data. Assert database state using assertDatabaseHas().
Tinker is an REPL for Laravel. Access using 'php artisan tinker'. Test code, interact with models, execute queries interactively. Useful for debugging and exploring application state.
Factories generate fake data for testing using Faker library. Created with 'php artisan make:factory'. Define model attributes and relationships. Support states for different scenarios.
Use .env.testing file for test environment. Configure test database, mail settings, queues. Use config:clear before testing. Support different configurations per test suite.
Use Mockery for mocking objects. Mock facades using Facade::mock(). Bind mocks in service container. Support partial mocks and spy objects. Verify mock expectations.
Use actingAs() for authenticated requests. Test login, logout, registration flows. Verify middleware protection. Test password reset functionality. Support multiple guards in tests.
Use json() method for API requests. Assert response structure, status codes. Test authentication tokens. Verify API rate limiting. Support API versioning in tests.
Use assertPushed(), assertNotPushed() for job verification. Test job execution and chain handling. Verify job delays and retries. Support queue-specific assertions.
Use Event::fake() to mock events. Assert event dispatch and handling. Test event listeners in isolation. Verify event payload. Support conditional event assertions.
Use Mail::fake() for mail testing. Assert mail sent and contents. Test mail templates. Verify attachments and recipients. Support markdown mail testing.
Use Notification::fake(). Assert notification sending and channels. Test notification content. Verify notification queuing. Support custom channel testing.
Use UploadedFile class for fake files. Test file validation and storage. Verify file processing. Support multiple file uploads. Test file deletion.
Use Cache::fake(). Test cache storage and retrieval. Verify cache tags and expiration. Support different cache drivers. Test cache clearing.
Use withSession() method. Test session data persistence. Verify flash messages. Support session regeneration. Test session expiration.
Use Laravel Dusk for browser testing. Test JavaScript interactions. Support multiple browsers. Handle authentication in browser tests. Test file downloads.
Create test-specific seeders. Handle complex data relationships. Support different seeding strategies. Implement seeder factories. Handle large dataset seeding.
Organize tests into suites. Configure suite-specific setup. Handle dependencies between suites. Support parallel suite execution. Implement suite-level fixtures.
Set up CI/CD pipelines. Configure test automation. Handle environment setup. Support different test stages. Implement test reporting.
Measure application performance metrics. Test response times and throughput. Profile database queries. Monitor memory usage. Implement load testing.
Test security vulnerabilities. Implement penetration testing. Verify authentication security. Test authorization rules. Check input validation.
Generate API documentation from tests. Verify API specifications. Test API versioning. Support OpenAPI/Swagger integration. Implement documentation automation.
Use mutation testing frameworks. Verify test coverage quality. Identify weak test cases. Support automated mutation analysis. Handle false positives.
Test application under heavy load. Verify system stability. Monitor resource usage. Implement crash recovery. Handle concurrent requests.
Maintain test suite for existing features. Automate regression checks. Handle backward compatibility. Support feature flags in tests. Implement version testing.
Caching in Laravel stores and retrieves data for faster access. Laravel supports multiple cache drivers (file, database, Redis, Memcached) configured in config/cache.php. Caching improves application performance by reducing database queries and computation.
Basic cache operations include Cache::get() to retrieve, Cache::put() to store, Cache::has() to check existence, Cache::forget() to remove items. Also supports Cache::remember() for compute-and-store operations.
Route caching improves routing performance using 'php artisan route:cache'. Creates a single file of compiled routes. Should be used in production. Must be cleared when routes change using route:clear.
Config caching combines all configuration files into single cached file using 'php artisan config:cache'. Improves performance by reducing file loading. Must be cleared when configs change using config:clear.
View caching compiles Blade templates into PHP code. Happens automatically and stored in storage/framework/views. Can be cleared using view:clear. Improves rendering performance.
Database queries can be cached using remember() method on query builder or Eloquent models. Example: User::remember(60)->get(). Also supports tags and cache invalidation strategies.
Cache tags group related items for easy manipulation. Use Cache::tags(['tag'])->put() for storage. Can flush all tagged cache using Cache::tags(['tag'])->flush(). Not all drivers support tagging.
Eager loading reduces N+1 query problems by loading relationships in advance using with() method. Example: User::with('posts')->get(). Improves performance by reducing database queries.
Response caching stores HTTP responses using middleware. Configure using Cache-Control headers. Supports client-side and server-side caching. Improves response times for static content.
Cache keys uniquely identify cached items. Use meaningful names and version prefixes. Handle key collisions. Support cache namespacing. Consider key length limits.
Cache versioning handles cache invalidation using version prefixes in keys. Increment version to invalidate all related cache. Support rolling updates. Handle version migrations.
Distributed caching uses Redis or Memcached across multiple servers. Handle cache synchronization. Support cache replication. Implement fallback strategies. Monitor cache health.
Database optimization includes proper indexing, query optimization, using chunking for large datasets, implementing pagination, optimizing relationships, and monitoring query performance.
Fragment caching stores portions of views. Use @cache directive in Blade. Support time-based expiration. Handle dynamic content. Implement cache tags for fragments.
Cache warming pre-populates cache before needed. Create warming scripts. Handle cache dependencies. Support progressive warming. Implement warming strategies.
Asset optimization includes minification, compression, bundling using Laravel Mix. Configure cache headers. Use CDN integration. Implement lazy loading. Support asset versioning.
Cache policies define caching rules and strategies. Handle cache lifetime. Support conditional caching. Implement cache priorities. Configure cache headers.
Queue optimization improves job processing performance. Configure worker processes. Handle job batching. Implement queue priorities. Monitor queue performance.
Session optimization includes choosing appropriate driver, configuring session lifetime, handling session cleanup, implementing session locking, monitoring session size.
Cache locks prevent race conditions in distributed systems. Use Cache::lock() method. Handle lock timeouts. Implement automatic release. Support lock retries.
Profile application using tools like Laravel Telescope, Clockwork. Monitor performance metrics. Track database queries. Analyze memory usage. Identify bottlenecks.
Cache sharding distributes cache across multiple nodes. Implement shard selection. Handle shard rebalancing. Support shard failover. Monitor shard health.
Hierarchical caching uses multiple cache layers. Implement cache fallback. Handle cache propagation. Support cache invalidation hierarchy. Monitor cache hit rates.
Memory optimization includes monitoring allocations, implementing garbage collection, optimizing data structures, handling memory leaks, configuring PHP memory limits.
Cache events track cache operations. Handle cache hits/misses. Implement cache warming events. Support event listeners. Monitor cache performance.
Load balancing distributes traffic across servers. Configure load balancer. Handle session affinity. Support health checks. Monitor server performance.
API optimization includes implementing rate limiting, caching responses, optimizing serialization, handling pagination, monitoring API metrics.
Cache warm-up strategies include identifying critical data, implementing progressive warming, handling cache dependencies, monitoring warm-up performance.
File system optimization includes caching file operations, implementing proper file handling, optimizing storage operations, monitoring disk usage.
Performance monitoring includes tracking metrics, implementing logging, setting up alerts, analyzing trends, identifying performance issues.
Queues allow deferring time-consuming tasks for background processing. Laravel supports various queue drivers (database, Redis, SQS, etc.). Queues improve application response time by handling heavy tasks asynchronously.
Jobs are created using 'php artisan make:job JobName'. Jobs implement ShouldQueue interface. Define handle() method for job logic. Jobs can be dispatched using dispatch() helper or Job::dispatch().
Queue workers are run using 'php artisan queue:work'. Can specify connection, queue name, and other options. Should be monitored using supervisor or similar process manager in production.
Job dispatch sends jobs to queue for processing. Can use dispatch() helper, Job::dispatch(), or DispatchesJobs trait. Supports delayed dispatch and customizing queue/connection.
Failed jobs are tracked in failed_jobs table. Handle failures using failed() method in job class. Can retry failed jobs using queue:retry command. Support custom failure handling.
Job middleware intercept job processing. Define middleware in job's middleware() method. Can rate limit, throttle, or modify job behavior. Support global and per-job middleware.
Job chains execute jobs in sequence using Chain::with(). Later jobs run only if previous ones succeed. Can set chain catch callback for failure handling.
Queue connections are configured in config/queue.php. Define driver, connection parameters. Support multiple connections. Can set default connection. Handle queue priorities.
Job batching processes multiple jobs as group using Bus::batch(). Track batch progress. Handle batch completion and failures. Support adding jobs to existing batch.
Job events track job lifecycle. Listen for job processed, failed events. Handle queue events in EventServiceProvider. Support custom event listeners.
Rate limit jobs using middleware like RateLimited. Configure limits per minute/hour. Handle rate limit exceeded scenarios. Support custom rate limiting strategies.
Set job timeout using timeout property or through command. Handle timeout exceptions. Implement graceful shutdown. Support retry after timeout.
Unique jobs prevent duplicate processing using ShouldBeUnique interface. Define uniqueness criteria. Handle lock timeout. Support unique job queuing strategies.
Track job progress using batch processing or custom tracking. Update progress in database. Broadcast progress updates. Support progress monitoring interface.
Manage job dependencies using job chaining or custom logic. Handle dependent job failures. Support conditional job execution. Implement dependency resolution.
Lifecycle hooks handle job events like preparing, processing, failed. Implement before/after processing logic. Support cleanup operations. Handle job cancellation.
Monitor queue using Horizon or custom solutions. Track queue size, processing time. Set up alerts. Handle queue bottlenecks. Support queue metrics.
Prioritize jobs using multiple queues. Configure queue priorities. Handle high-priority job processing. Support dynamic prioritization. Monitor queue priorities.
Configure supervisor to manage queue workers. Set up worker processes. Handle worker failures. Monitor worker status. Support automatic restart.
Scale queues using multiple workers. Handle worker balancing. Implement auto-scaling. Monitor queue performance. Support horizontal scaling.
Process jobs across multiple servers. Handle job distribution. Implement job coordination. Support distributed locks. Monitor distributed processing.
Version jobs for compatibility. Handle job upgrades. Support multiple versions. Implement version migration. Monitor version conflicts.
Create complex scheduling patterns. Handle recurring jobs. Support conditional scheduling. Implement schedule dependencies. Monitor schedule execution.
Optimize job processing speed. Handle memory management. Implement queue sharding. Support batch optimization. Monitor performance metrics.
Manage job state across executions. Handle state persistence. Implement state recovery. Support state transitions. Monitor state changes.
Create robust error handling. Implement retry strategies. Handle permanent failures. Support error notification. Monitor error patterns.
Build custom monitoring solutions. Track queue metrics. Implement alerting system. Support dashboard visualization. Monitor queue health.
Test queue jobs effectively. Mock queue operations. Verify job behavior. Support integration testing. Monitor test coverage.
Secure queue operations. Handle job authentication. Implement authorization. Support encryption. Monitor security threats.
Plan for queue failures. Implement backup strategies. Handle recovery procedures. Support failover mechanisms. Monitor recovery process.