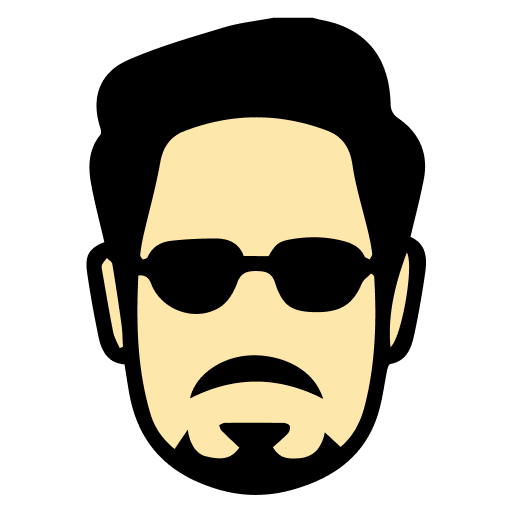
Python is one of the most sought-after skills in the tech industry, making it a popular choice for interviews. Whether you're applying for a software developer, data analyst, or machine learning role, being prepared is key. Stark.ai helps you excel in Python job interviews with curated questions, real-world scenarios, and expert answers.
Python is a high-level, interpreted programming language known for its readability, simplicity, and versatility. Key...
Python uses automatic memory management through a private heap containing all Python objects and data structures. It...
Lists are mutable, allowing modifications like adding, removing, or changing elements. Tuples are immutable, meaning...
Python's built-in data types include numeric types (int, float, complex), sequence types (list, tuple, range), text...
You can create a virtual environment using the `venv` module by running `python -m venv myenv`, where `myenv` is the...
The `__init__` method is the constructor in Python classes. It initializes the instance attributes of a class when a...
List comprehensions provide a concise way to create lists by iterating over an iterable and optionally including...
Python uses reference counting to keep track of the number of references to each object. When an object's reference...
Decorators are functions that modify or enhance other functions or methods without changing their code. They are...
Exceptions are handled using `try` and `except` blocks. Code that may raise an exception is placed inside the `try`...
The four pillars are Encapsulation (bundling data and methods), Abstraction (hiding complex implementation details),...
Inheritance is implemented by defining a class that inherits from a parent class. For example, `class...
Polymorphism allows objects of different classes to be treated as instances of a common superclass, typically using...
Encapsulation is the bundling of data and methods within a class, restricting direct access to some components. In...
Python allows a class to inherit from multiple parent classes by listing them in parentheses. For example, `class...
Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in...
Class methods are methods that receive the class itself as the first argument (conventionally `cls`) and are defined...
You can prevent a class from being subclassed by using the `final` decorator from the `typing` module (in Python...
The `super()` function returns a temporary object of the superclass, allowing access to its methods. It is commonly...
Abstraction is implemented using abstract base classes (ABCs) from the `abc` module. By defining abstract methods...
Missing data can be handled using methods like `df.dropna()` to remove missing values, `df.fillna()` to fill them...
`loc` is label-based and used for selecting rows and columns by their labels or boolean arrays. `iloc` is integer...
You can merge two DataFrames using the `pd.merge()` function, specifying the keys to join on and the type of join...
The `groupby` function is used to split a DataFrame into groups based on one or more keys, apply a function to each...
You can apply functions using the `df.apply()` method, specifying `axis=0` for columns or `axis=1` for rows....
The `pivot_table` method creates a spreadsheet-style pivot table, allowing you to summarize and aggregate data based...
Pandas provides functions like `pd.read_csv()`, `pd.read_excel()`, `pd.read_json()`, `pd.read_sql()`, and...
Data filtering and selection can be done using boolean indexing, the `query()` method, `loc` and `iloc` for label or...
Multi-indexes allow pandas DataFrames to have multiple levels of indexing on rows and/or columns. They enable more...
Performance can be optimized by using efficient data types (e.g., categorical data), avoiding unnecessary copies,...
Python is a high-level, interpreted programming language created by Guido van Rossum. It is known for its...
Python 3 is a major revision of the language that is not fully backward-compatible with Python 2. Key differences...
The Global Interpreter Lock is a mutex that prevents multiple native threads from executing Python bytecodes...
Python supports multiple programming paradigms, including object-oriented programming (OOP), functional programming,...
Python uses automatic memory management with reference counting and garbage collection. Objects are created...
Duck typing is a dynamic typing concept in Python where the type or class of an object is less important than the...
.py files are source code files containing Python script, while .pyc files are compiled bytecode files that are...
Decorators are a design pattern in Python that allows modifying or enhancing functions or classes without directly...
Method Resolution Order (MRO) is the order in which Python looks for methods in a hierarchy of classes. It uses the...
PEP 8 is the style guide for Python code that provides conventions for writing readable and consistent Python code....
Lambda functions are small, anonymous functions defined using the 'lambda' keyword. They can have any number of...
The 'with' statement is used for resource management, ensuring that a resource is properly acquired and released. It...
A shallow copy creates a new object but references the same memory addresses for nested objects, while a deep copy...
Python uses namespaces to organize and manage variable names. It follows the LEGB rule (Local, Enclosing, Global,...
Generator functions use the 'yield' keyword to return a generator iterator. They allow you to generate values...
The __init__ method is a special constructor method in Python classes that is automatically called when a new object...
Python supports multiple inheritance, allowing a class to inherit from multiple parent classes. It uses the Method...
Context managers are objects that define __enter__ and __exit__ methods, used with the 'with' statement to manage...
The '==' operator checks for value equality, comparing the values of two objects, while the 'is' operator checks for...
Metaclasses are classes of classes, allowing you to customize class creation. They define how a class behaves and...
Python uses try-except blocks to handle exceptions. You can catch specific exceptions, use multiple except blocks,...
__str__ provides a human-readable string representation of an object, typically used by print(), while __repr__...
Python uses reference counting and generational garbage collection. When an object's reference count drops to zero,...
Magic methods, identified by double underscores (e.g., __init__, __str__), allow customization of object behavior....
Python supports functional programming through features like lambda functions, map(), filter(), reduce(),...
Abstract base classes (defined using the abc module) can have both abstract and concrete methods, while Python...
Type hinting allows specifying expected types for function parameters and return values. Introduced in Python 3.5,...
*args allows a function to accept any number of positional arguments, while **kwargs allows accepting any number of...
Python uses naming conventions for encapsulation. Single underscore prefix (e.g., _variable) suggests internal use,...
Lists are mutable (can be modified after creation) and use square brackets [], while tuples are immutable (cannot be...
'==' compares the values of objects (equality), while 'is' compares the identity (memory location) of objects. For...
Python has int (integer), float (floating-point), complex (complex numbers) as numeric types. Integers have...
Python uses LEGB rule: Local, Enclosing, Global, Built-in. Variables are first searched in local scope, then...
List comprehension is a concise way to create lists based on existing lists/iterables. Syntax: [expression for item...
Slicing extracts parts of sequences using syntax sequence[start:stop:step]. Start is inclusive, stop is exclusive....
Python offers multiple string formatting methods: %-formatting (old style), str.format() method, and f-strings...
Python includes arithmetic (+, -, *, /, //, %, **), comparison (==, !=, >, <, >=, <=), logical (and, or, not),...
Type hints are added using annotations: function parameters (param: type), return types (-> type), and variable...
Generator expressions create iterators using syntax (expression for item in iterable if condition). Similar to list...
Shallow copy creates new object but references same nested objects (copy.copy()). Deep copy creates new object and...
Python offers if/elif/else statements, ternary operators (value_if_true if condition else value_if_false), and...
Walrus operator assigns values in expressions: while (n := len(a)) > 0. Benefits include more concise code, avoiding...
Python provides break (exit loop), continue (skip to next iteration), else (executes when loop completes normally),...
Python assigns references to objects, not objects themselves. Multiple names can reference same object. Assignment...
Unpacking assigns sequence elements to variables: a, b = [1, 2]. Packing collects multiple values into sequence...
Use encode() to convert string to bytes, decode() for bytes to string. Specify encoding (e.g., 'utf-8', 'ascii')....
Python 2's range creates list, xrange creates iterator. Python 3's range creates range object (iterator-like). Range...
Boolean operations (and, or, not) follow short-circuit rules. 'and' returns first false value or last value, 'or'...
F-strings (formatted string literals) allow embedding expressions: f'{var=}'. Support format specifiers, multiline...
/ performs true division (returns float), // performs floor division (returns int for ints). Floating-point follows...
Use # for single-line comments, triple quotes (''' or """) for multiline comments/docstrings. Docstrings document...
Match statements (3.10+) provide pattern matching: match value: case pattern: .... Supports patterns like literals,...
Follow PEP 8: lowercase_with_underscores for functions/variables, CapitalizedWords for classes,...
Python follows operator precedence: exponentiation highest, then multiplication/division, then addition/subtraction....
Support binary (0b), octal (0o), hexadecimal (0x) literals. Convert between bases using bin(), oct(), hex(),...
Average case time complexities for dictionary operations are: Get item O(1), Set item O(1), Delete item O(1), Search...
Lists are dynamic arrays that can store elements of different types. Arrays (from array module) store homogeneous...
heapq implements min heap, providing O(log n) push/pop operations. Used for priority queues, finding n...
sorted() creates new sorted list, leaving original unchanged. list.sort() modifies list in-place. Both use Timsort...
Implement using class with Node class (value, left, right pointers) and Tree class with insert, delete, search...
Deques (collections.deque) are double-ended queues supporting O(1) append/pop at both ends. Used for sliding...
bisect module provides binary search functionality for sorted sequences. bisect_left finds insertion point for...
Sets use hash tables with open addressing to handle collisions. When collision occurs, probing finds next empty...
Python uses Timsort, hybrid of merge sort and insertion sort. Stable sort with O(n log n) worst case. Adaptive...
Create Node class with value and next pointer. LinkedList class manages head pointer, implements insert, delete,...
OrderedDict maintains insertion order (pre-3.7), useful for LRU caches. defaultdict provides default values for...
Represent graphs using adjacency lists (dictionaries) or matrices. Implement BFS/DFS using queue/stack. Use dict/set...
Tuples are immutable, slightly more memory efficient, can be dictionary keys. Lists are mutable, support item...
Use memoization (decorator or dict) or tabulation (arrays). Handle base cases, build solution using smaller...
Python uses open addressing with random probing. Alternative methods: chaining (linked lists), linear probing,...
Stack: use append() and pop() for LIFO. Queue: use append() and pop(0)/list.pop(0) for FIFO (inefficient, use...
Counter from collections creates dictionary subclass for counting hashable objects. Supports addition, subtraction,...
Create TrieNode class with children dict and is_end flag. Implement insert, search, startswith methods. Use dict for...
bytearray is mutable version of bytes. Supports in-place modifications, useful for building binary data...
Implement iteratively or recursively on sorted sequence. Handle mid calculation, comparison, and boundary updates....
append() adds single element, extend() adds elements from iterable. append([1,2]) adds list as single element,...
Use heapq module for min heap implementation. Wrap elements in tuples with priority. Alternative:...
String concatenation with += creates new string objects. Use join() method for multiple concatenations, more...
Implement find and union operations using dictionary/array. Use path compression and union by rank optimizations....
In Python 2, range creates list, xrange creates iterator object. Python 3's range is like xrange, memory efficient...
Use decorator with cache dict, or functools.lru_cache. Store function arguments as keys. Handle mutable arguments....
Use immutable types (strings, numbers, tuples of immutables). Consider hash collisions and distribution. Custom...
Quicksort: partition around pivot, recursively sort subarrays. Mergesort: divide array, sort recursively, merge...
itertools provides efficient iteration tools: combinations(), permutations(), product(), cycle(), chain(). Memory...
A class is a blueprint for objects, defining attributes and methods. An object is an instance of a class. Classes...
Inheritance allows a class to inherit attributes and methods from another class. Implemented using class...
Instance methods (default) have self parameter, access instance data. Class methods (@classmethod) have cls...
Python uses name mangling with double underscore prefix (__var) for private attributes. Single underscore (_var) for...
Magic methods control object behavior for built-in operations. Examples: __init__ for initialization, __str__ for...
Polymorphism implemented through method overriding in inheritance and duck typing. Same method name behaves...
Properties (@property decorator) provide getter/setter functionality with attribute-like syntax. Control access to...
Multiple inheritance allows class to inherit from multiple parents: class Child(Parent1, Parent2). MRO determines...
Metaclasses are classes for classes, allow customizing class creation. Created using type or custom metaclass. Used...
Descriptors control attribute access through __get__, __set__, __delete__ methods. Used in properties, methods,...
Composition creates objects containing other objects as parts (has-a relationship), while inheritance creates is-a...
Use abc module with ABC class and @abstractmethod decorator. Abstract classes can't be instantiated, enforce...
Class variables shared among all instances (defined in class), instance variables unique to each instance (defined...
super() returns proxy object for delegating method calls to parent class. Handles multiple inheritance correctly...
__new__ creates instance, called before __init__. __init__ initializes instance after creation. __new__ rarely...
Implement using metaclass, __new__ method, or module-level instance. Control instance creation, ensure single...
__slots__ restricts instance attributes to fixed set, reduces memory usage. Faster attribute access, prevents...
Use __getattr__, __setattr__, __getattribute__, __delattr__ for custom attribute access. __getattr__ called for...
Method overriding redefines method from parent class in child class. Used to specialize behavior while maintaining...
Override special methods for operators: __add__ for +, __eq__ for ==, etc. Enable class instances to work with...
Class decorators modify or enhance class definitions. Applied using @decorator syntax above class. Can add...
Use __slots__, @property with only getter, override __setattr__/__delattr__. Store data in private tuples, implement...
__str__ for human-readable string representation, __repr__ for unambiguous representation (debugging). __repr__...
Implement __enter__ and __exit__ methods. __enter__ sets up context, __exit__ handles cleanup. Used with 'with'...
Mixins are classes providing additional functionality through multiple inheritance. Used for reusable features...
Use pickle for Python-specific serialization, implement __getstate__/__setstate__ for custom serialization. Consider...
Dataclasses (@dataclass) automatically add generated methods (__init__, __repr__, etc.). Used for classes primarily...
Implement container protocol methods: __len__, __getitem__, __setitem__, __delitem__, __iter__. Optional:...
__dict__ stores instance attributes in dictionary. Enables dynamic attribute addition. Not present when using...
Lambda functions are anonymous, single-expression functions using lambda keyword. Syntax: lambda args: expression....
List comprehensions create lists using compact syntax: [expression for item in iterable if condition]. More readable...
Decorators are higher-order functions that modify other functions. Use @decorator syntax or function_name =...
map(func, iterable) applies function to each element. filter(func, iterable) keeps elements where function returns...
Generators are functions using yield keyword to return values incrementally. Create iterator objects, maintain state...
Python uses LEGB scope (Local, Enclosing, Global, Built-in). Closures capture variable values from enclosing scope....
Annotations provide type hints: def func(x: int) -> str. Stored in __annotations__ dict. Not enforced at runtime,...
Use functools.partial to create new function with fixed arguments. Reduces function arity by pre-filling arguments....
Positional arguments (standard), keyword arguments (name=value), default arguments (def func(x=1)), variable...
Use @functools.lru_cache decorator or implement custom caching with dict. Stores function results, returns cached...
Currying transforms function with multiple arguments into series of single-argument functions. Implement using...
Recursion is function calling itself. Limited by recursion depth (default 1000). Use tail recursion optimization or...
Higher-order functions take functions as arguments or return functions. Examples: map, filter, decorators. Enable...
Pure functions always return same output for same input, have no side effects. Benefits: easier testing, debugging,...
Use docstrings (triple quotes) for function documentation. Include description, parameters, return value, examples....
Dict comprehension: {key:value for item in iterable}. Set comprehension: {expression for item in iterable}. More...
Python doesn't support traditional overloading. Use default arguments, *args, **kwargs, or @singledispatch...
Coroutines use async/await syntax, support concurrent programming. Unlike generators, can receive values with...
Nested functions have access to outer function variables (closure). Use nonlocal keyword to modify enclosed...
Function composition combines functions: f(g(x)). Implement using higher-order functions or operator module....
Implement __iter__ and __next__ methods. __iter__ returns iterator object, __next__ provides next value or raises...
functools provides tools for functional programming: reduce, partial, lru_cache, wraps, singledispatch. Enhances...
Functions are objects with attributes. Access/set via function.__dict__. Common attributes: __name__, __doc__,...
Tail recursion occurs when recursive call is last operation. Python doesn't optimize tail recursion automatically....
Return self from methods to enable chaining: obj.method1().method2(). Common in builder pattern and fluent...
Use default arguments, None values, or *args/**kwargs. Consider parameter order, default mutability issues. Example:...
Function factories create and return new functions. Use closures to capture configuration. Common in parameterized...
Use Either/Maybe patterns, return tuple of (success, result), or raise exceptions. Handle errors explicitly in...
Basic structure uses try/except blocks: try to execute code that might raise exception, except to handle specific...
Create custom exceptions by inheriting from Exception class or specific exception types. Raise using raise keyword....
finally clause executes regardless of whether exception occurred or was handled. Used for cleanup operations like...
Use logging module with different levels (DEBUG, INFO, WARNING, ERROR, CRITICAL). Configure handlers, formatters,...
pdb is Python's built-in debugger, ipdb adds IPython features like tab completion, syntax highlighting. Both support...
Group exceptions in tuple: except (TypeError, ValueError) as e. Access exception info using 'as' keyword. Consider...
Context managers (with statement) ensure proper resource cleanup. Implement __enter__ and __exit__ methods or use...
Use memory_profiler, tracemalloc, gc module. Monitor object references, circular references. Tools: objgraph for...
Assertions (assert condition, message) check program correctness, disabled with -O flag. Exceptions handle runtime...
Use error tracking services (Sentry, Rollbar). Implement custom error handlers, logging middleware. Capture stack...
Create decorators for timing, logging, error catching. Example: @log_errors, @retry, @timeout. Handle function...
Use thread-specific exception handlers, threading.excepthook. Handle exceptions within thread function. Consider...
Create base exception class for application. Structure hierarchy based on error types. Include relevant error...
Use breakpoints, print debugging, sys.setrecursionlimit(). Monitor stack traces, memory usage. Tools: pdb for...
sys.excepthook handles uncaught exceptions. Customize for global error handling, logging. Access exception type,...
Use decorators or context managers for retries. Handle specific exceptions, implement backoff strategy. Example:...
IDEs provide integrated debuggers, breakpoints, variable inspection, call stack viewing. Popular: PyCharm debugger,...
Use try/except in async functions, handle event loop exceptions. asyncio.excepthook for loop exceptions. Consider...
Implement consistent error response format, HTTP status codes. Include error codes, messages, debug information....
Use profilers (cProfile, line_profiler), timing decorators. Monitor CPU usage, memory allocation. Tools: py-spy for...
Include specific error details, context information. Make messages user-friendly, actionable. Consider...
Create wrapper classes/functions for error containment. Handle subsystem failures gracefully. Consider component...
Use source-level debugging, monkey patching. Inspect library source, logging integration. Consider version...
Implement specific exception handling for DB errors. Handle connection issues, transaction rollback. Consider retry...
Use thread/process-specific logging, race condition detection. Tools: threading.VERBOSE, multiprocessing debug logs....
Create recovery procedures for critical errors. Implement state restoration, data consistency checks. Consider...
Validate configuration early, provide clear error messages. Handle missing/invalid settings gracefully. Consider...
Use memory_profiler, guppy3 for heap analysis. Monitor object lifecycle, memory patterns. Consider garbage...
Implement data masking, logging filters. Handle PII, credentials securely. Consider compliance requirements, log...
Common modes: 'r' (read), 'w' (write), 'a' (append), 'b' (binary), '+' (read/write). Can combine modes: 'rb' (read...
Use 'with' statement: 'with open(filename) as f:'. Ensures proper file closure even if exceptions occur. Best...
read() reads entire file into string, readline() reads single line, readlines() reads all lines into list. read()...
Use csv module: reader, writer, DictReader, DictWriter classes. Handle delimiters, quoting, escaping. Consider...
Use json module: json.load(file), json.dump(data, file). Handle encoding, pretty printing (indent parameter)....
Use 'b' mode flag, read/write bytes objects. Methods: read(size), write(bytes). Consider bytearray for mutable...
Buffering options: 0 (unbuffered), 1 (line buffered), >1 (size in bytes). Default is system dependent. Set with...
Specify encoding parameter in open(): UTF-8, ASCII, etc. Handle encoding/decoding errors with errors parameter. Use...
Use mmap module for memory-mapped file I/O. Treats file as memory buffer. Good for large files, shared memory....
Use fcntl module (Unix) or msvcrt (Windows). Implement advisory locking. Handle shared vs exclusive locks. Consider...
Use tempfile module: NamedTemporaryFile, TemporaryDirectory. Auto-cleanup when closed. Secure creation, unique...
Use generators for line iteration, chunk reading. Consider memory usage, buffering. Use mmap for random access....
Objects implementing file interface (read, write, etc.). Examples: StringIO, BytesIO for in-memory files. Used for...
Use os.path or pathlib for path operations. Handle permissions, existence checks. Consider race conditions, atomic...
Use configparser for INI files, yaml for YAML. Handle defaults, validation. Consider environment overrides....
Use watchdog library or platform-specific APIs. Handle file system events. Consider polling vs event-based....
Use pathlib.Path for object-oriented path operations. Handle platform differences, relative paths. Consider path...
Use openpyxl, pandas for XLSX files. xlrd/xlwt for older formats. Handle sheets, formatting, formulas. Consider...
Use gzip, zipfile, tarfile modules. Handle compression levels, passwords. Consider streaming for large files....
Use glob, fnmatch for patterns. re module for regex. Consider recursive search, filters. Handle large directories...
Use rotating file handlers, proper formatting. Handle log levels, rotation size. Consider compression, retention...
Use os.stat, Path.stat() for metadata. Handle timestamps, permissions. Consider platform differences. Implement...
Use os.replace for atomic writes. Implement write-to-temp-then-rename pattern. Handle concurrent access. Consider...
Use os.chmod, Path.chmod() for permissions. Handle umask settings. Consider security implications. Implement least...
Implement backup rotation, version naming. Handle incremental backups. Consider compression, deduplication....
Use appropriate protocols (FTP, SFTP). Handle timeouts, retries. Consider security, authentication. Implement...
Use context managers, atexit handlers. Implement proper error handling. Consider temporary files, locks. Handle...
Use mimetypes module, file signatures. Handle binary vs text files. Consider encoding detection. Implement proper...
Handle chunk size, ordering. Implement progress tracking. Consider memory efficiency. Handle partial failures....
Common import methods: 'import module', 'from module import item', 'from module import *', 'import module as alias'....
__init__.py marks directory as Python package, can initialize package attributes, execute package initialization...
Python searches modules in order: current directory, PYTHONPATH, standard library directories, site-packages....
Relative imports use dots: from . import module (current package), from .. import module (parent package). Only work...
Use venv module: python -m venv env_name. Activate using source env/bin/activate (Unix) or env\Scripts\activate...
setup.py defines package metadata, dependencies, entry points for distribution. Used with setuptools for package...
Namespace packages allow splitting package across multiple directories. No __init__.py required. Uses implicit...
pip is Python's package installer, conda is cross-platform package/environment manager. pip works with PyPI, conda...
Avoid using import statements inside functions, use dependency injection, restructure code to break cycles. Consider...
__all__ list controls what's imported with 'from module import *'. Explicitly defines public API. Good practice for...
Build distribution using setuptools/wheel, upload to PyPI using twine. Create source and wheel distributions....
Entry points define console scripts, plugin points in setup.py/pyproject.toml. Enable command-line tools, plugin...
Use requirements.txt or pyproject.toml, specify version ranges appropriately. Consider dev vs production...
Checks if module is run directly or imported. Common for script entry points, testing. Code under this block only...
Use MANIFEST.in, package_data in setup.py, include_package_data=True. Access using pkg_resources or...
Wheels are built package format, faster installation than source distributions. Contains pre-built files, metadata....
Use entry points, namespace packages, or import hooks. Define plugin interface, discovery mechanism. Consider...
importlib provides import mechanism implementation, custom importers. Used for dynamic imports, import hooks. Access...
Use virtual environments, specify version constraints carefully. Consider dependency resolution tools, container...
Modern configuration file for Python projects (PEP 518). Specifies build system requirements, project metadata....
Use packages for logical grouping, maintain clear hierarchy. Consider separation of concerns, circular dependencies....
Use docstrings, maintain README files, generate API documentation. Follow PEP 257. Include usage examples,...
Import modules when needed, use importlib.import_module(). Consider performance implications, circular dependencies....
Use __init__.py for package setup, expose public API. Handle optional dependencies, perform checks. Consider...
Use try/except for optional imports, implement fallbacks. Consider platform-specific modules. Handle missing...
Import hooks customize module importing process. Implement custom importing behavior, transformations. Used for...
Use semantic versioning, maintain changelog. Consider backwards compatibility, deprecation policy. Handle version...
Verify package sources, use package hashes. Consider supply chain attacks, dependency scanning. Implement security...
Handle platform-specific code, use conditional imports. Consider environment differences. Test on multiple...
unittest is built-in, class-based, requires test classes inheriting from TestCase. pytest is more flexible, supports...
Fixtures provide reusable test setup/teardown, defined using @pytest.fixture decorator. Support dependency...
Mocking replaces real objects with test doubles. Use unittest.mock or pytest-mock. MagicMock/Mock classes provide...
Use coverage.py or pytest-cov. Run tests with coverage collection, generate reports. Analyze uncovered lines,...
TDD cycle: write failing test, write code to pass, refactor. Tests drive design, document requirements. Write...
Use @pytest.mark.parametrize decorator to run same test with different inputs. Supports multiple parameters, custom...
Markers (@pytest.mark) categorize tests, control execution. Built-in markers: skip, skipif, xfail. Custom markers...
Use test databases, fixtures for setup/teardown. Consider transaction rollback, database isolation. Mock database...
Test doubles replace real dependencies. Mocks verify interactions, stubs provide canned responses, fakes implement...
Use pytest-asyncio for async tests. Mark tests with @pytest.mark.asyncio. Handle coroutines properly. Consider event...
Group related tests, use clear naming conventions. Separate unit/integration tests. Follow AAA pattern...
Test component interactions, external services. Use appropriate fixtures, mocking selectively. Consider test...
Use hypothesis library for property-based testing. Define properties, let framework generate test cases. Useful for...
Use fixtures, factory libraries (factory_boy). Consider data isolation, cleanup. Implement proper test data...
conftest.py provides shared fixtures across multiple test files. Defines test configuration, custom markers....
Use pytest-benchmark for performance tests. Measure execution time, resource usage. Consider baseline comparisons,...
Monkey patching modifies objects/modules at runtime for testing. Use pytest.monkeypatch fixture. Handle cleanup...
Use pytest.raises context manager or unittest.assertRaises. Test exception types, messages. Consider exception...
Plugins extend pytest functionality. Common plugins: pytest-cov, pytest-mock, pytest-django. Install via pip,...
Use requests, pytest-httpx for HTTP testing. Mock external services appropriately. Consider response validation,...
Use pytest-bdd or behave for BDD. Write tests in Gherkin syntax. Map steps to test code. Consider stakeholder...
Use environment variables, configuration files. Implement test environment management. Consider CI/CD integration....
Avoid test interdependence, slow tests, excessive mocking. Don't test implementation details. Avoid...
Use pytest-xdist for parallel testing. Consider test isolation, shared resources. Handle race conditions. Implement...
Use caplog fixture in pytest. Verify log messages, levels. Consider log handlers, formatting. Test logger...
Test input validation, authentication, authorization. Use security testing tools (bandit). Consider vulnerability...
Scopes: function (default), class, module, session. Choose based on resource costs, test isolation needs. Consider...
Integrate tests in CI/CD pipeline. Automate test execution, reporting. Consider test selection, prioritization....
Use PyTest-Qt, PyAutoGUI for GUI testing. Handle event loops properly. Consider screenshot comparisons. Test user...
Collections module provides specialized container types: defaultdict (automatic default values), Counter (counting...
datetime module handles dates and times. Use datetime.datetime with tzinfo for timezone awareness, pytz for reliable...
itertools provides functions for efficient iteration: combinations(), permutations(), product() for combinatorics;...
os module provides OS-independent interface for operating system operations. Functions: os.path for path...
json module handles JSON encoding/decoding. Methods: dumps()/loads() for strings, dump()/load() for files. Supports...
re module provides regular expression operations: compile(), match(), search(), findall(). Supports patterns,...
concurrent.futures provides high-level interface for asynchronous execution: ThreadPoolExecutor for I/O-bound tasks,...
logging provides flexible event logging: different levels (DEBUG to CRITICAL), handlers, formatters. Configure using...
pathlib provides object-oriented interface for file system paths. Path class methods: glob(), mkdir(), touch(),...
functools provides tools for functional programming: partial() for partial application, lru_cache() for caching,...
argparse handles command-line argument parsing: argument types, help messages, subcommands. Supports...
sqlite3 provides SQLite database interface: connection management, cursor operations, parameter substitution....
asyncio provides infrastructure for async/await code: event loops, coroutines, tasks. Supports async I/O operations,...
contextlib provides utilities for context managers: @contextmanager decorator, ExitStack for multiple contexts....
csv handles CSV file operations: reading, writing, different dialects. Supports DictReader/DictWriter for named...
threading provides high-level threading interface: Thread class, synchronization primitives (Lock, Event). Handle...
random provides pseudo-random number generation: randint(), choice(), shuffle(). Supports different distributions,...
pickle handles Python object serialization: dump()/load() for files, dumps()/loads() for bytes. Consider security...
sys provides system-specific parameters/functions: sys.path for module search, sys.argv for command arguments,...
shutil provides high-level file operations: copyfile(), rmtree(), make_archive(). Supports file copying, removal,...
urllib handles URL operations: request handling, parsing, encoding. Modules: request for HTTP, parse for URL...
time provides time-related functions: time() for timestamps, sleep() for delays, strftime() for formatting. Handle...
enum provides enumeration support: Enum class, auto() for automatic values. Supports unique/non-unique values,...
subprocess manages external processes: run(), Popen for process control. Handle command execution, I/O redirection,...
statistics provides statistical functions: mean(), median(), mode(), stdev(). Supports different types of averages,...
multiprocessing provides process-based parallelism: Process class, Pool for worker pools. Handle process creation,...
tempfile handles temporary files/directories: TemporaryFile, NamedTemporaryFile, TemporaryDirectory. Supports secure...
operator provides function equivalents of operators: itemgetter(), attrgetter() for data access, add(), mul() for...
platform provides system information: system(), machine(), python_version(). Access hardware, OS details, Python...
SQLAlchemy Core provides SQL abstraction layer, direct table operations. ORM provides object-relational mapping,...
Use connection pools (SQLAlchemy's Pool, psycopg2's pool). Configure pool size, overflow, timeout. Handle connection...
Use migration tools (Alembic, Django migrations). Version control migrations, test before deployment. Handle data...
Use transaction context managers, explicit commit/rollback. Handle atomic operations, savepoints. Implement proper...
Use indexing, query analysis tools. Optimize JOIN operations, limit result sets. Consider query execution plans....
Use parameterized queries, ORM query builders. Never concatenate SQL strings. Validate input data. Consider escape...
Define relationships (one-to-many, many-to-many) using relationship() in SQLAlchemy or ForeignKey in Django. Handle...
Use migration tools with version control. Implement forward/backward migrations. Track schema changes in source...
Handle specific database exceptions, implement retry logic. Log errors appropriately. Consider transaction rollback,...
Use sharding keys, implement routing logic. Handle cross-shard queries, transactions. Consider data distribution,...
Implement regular backups, verify backup integrity. Handle incremental backups, point-in-time recovery. Consider...
Implement exponential backoff, max retry attempts. Handle connection timeouts, dead connections. Consider circuit...
Use caching layers (Redis, Memcached). Implement cache invalidation strategies. Handle cache consistency. Consider...
Configure master-slave replication, handle failover. Implement read/write splitting. Consider replication lag,...
Implement batched operations, progress tracking. Handle data validation, rollback capability. Consider performance...
Monitor query performance, connection usage. Log slow queries, errors. Implement performance metrics collection....
Use proper isolation levels, row-level locking. Handle deadlock detection, prevention. Consider optimistic vs...
Implement zero-downtime migrations. Handle backward compatibility. Use temporary tables for large changes. Consider...
Use test databases, fixtures. Implement transaction rollback for tests. Handle database isolation. Consider...
Implement proper authentication, authorization. Use least privilege principle. Handle sensitive data encryption....
Use schema versioning, data versioning. Handle version conflicts. Implement upgrade/downgrade procedures. Consider...
Use connection pools, proper cleanup. Handle connection lifecycle. Implement health checks. Consider connection...
Handle schema flexibility, denormalization. Implement proper indexing strategies. Consider consistency models....
Use configuration files, environment variables. Handle different environments. Implement secure credential...
Monitor query performance, implement indexing. Use query optimization tools. Consider database configuration tuning....
Track data changes, user actions. Implement audit tables. Handle audit log maintenance. Consider compliance...
Implement high availability solutions, automatic failover. Handle failover detection, recovery. Consider data...
Monitor growth trends, resource usage. Plan scaling strategies. Consider performance requirements. Implement...
Schedule maintenance windows, implement automation. Handle index maintenance, statistics updates. Consider...
Django is a full-featured framework with built-in admin, ORM, auth. Flask is a lightweight, flexible microframework....
Use Flask-RESTful or Flask API extensions. Implement resource classes, HTTP methods (GET, POST, etc.). Handle...
Middleware processes requests/responses globally. Implements security, sessions, authentication. Order matters in...
Use built-in auth in Django, Flask-Login for Flask. Implement JWT for APIs. Handle session management, password...
Use form classes (Django Forms, WTForms). Implement server-side validation, CSRF protection. Handle file uploads...
Use cache frameworks (Django's cache, Flask-Caching). Implement view caching, template fragment caching. Consider...
Use makemigrations and migrate commands. Handle schema changes, data migrations. Test migrations before deployment....
Use channels in Django, Flask-SocketIO in Flask. Handle real-time communication, event handling. Consider scaling...
Implement CSRF protection, XSS prevention, SQL injection protection. Use secure headers, HTTPS. Handle input...
Validate file types, size limits. Store files securely (filesystem/cloud storage). Handle virus scanning. Consider...
Use URL versioning, header versioning, or content negotiation. Handle backwards compatibility. Consider...
Use rate limiting middleware/decorators. Implement token bucket algorithm. Handle different rate limits per...
Use Celery, Redis Queue, or Django Q. Handle task queuing, scheduling. Implement proper error handling, retries....
Use session middleware, handle session storage (database/cache). Consider session timeout, security. Implement...
Implement proper exception handling, custom error pages. Log errors appropriately. Handle different types of errors...
Use environment variables, configuration files. Handle different environments (dev/prod). Implement secure...
Use Swagger/OpenAPI specification. Implement automatic documentation generation. Handle versioning, examples....
Use pagination classes (Django) or implement custom pagination. Handle cursor-based, offset pagination. Consider...
Implement CORS middleware, handle preflight requests. Configure allowed origins, methods, headers. Consider security...
Use unittest, pytest for testing. Implement unit tests, integration tests. Handle test data, fixtures. Consider test...
Use containerization (Docker), implement CI/CD. Handle environment configuration. Consider scaling strategies....
Use CDN, proper static file serving. Handle caching, compression. Consider performance optimization. Implement...
Use search engines (Elasticsearch), implement full-text search. Handle indexing, querying. Consider performance...
Use logging framework, handle different log levels. Implement proper log formatting. Consider log aggregation,...
Use connection pooling, handle connection lifecycle. Implement proper error handling. Consider connection timeouts....
Use role-based access control (RBAC), implement permission checks. Handle group permissions. Consider hierarchical...
Use template inheritance, handle template caching. Implement proper escaping. Consider performance optimization....
Implement rate limiting middleware, handle quota management. Consider distributed rate limiting. Implement proper...
Handle webhook registration, event delivery. Implement retry logic. Consider security implications. Handle webhook...
NumPy arrays are homogeneous (same data type), support vectorized operations, more memory efficient. Offer...
Use fillna(), dropna(), interpolate() methods. Handle different types of missing data (NaN, None). Consider...
Matplotlib for basic plots (line, scatter, bar). Seaborn for statistical visualizations (distributions,...
Use groupby(), agg(), pivot_table(). Apply different aggregation functions. Handle multi-level aggregation. Consider...
Broadcasting allows operations between arrays of different shapes. Rules: dimensions must be compatible (same, one,...
Use get_dummies() for one-hot encoding, LabelEncoder for label encoding. Handle ordinal vs nominal categories....
Use StandardScaler, MinMaxScaler, RobustScaler. Handle outliers in scaling. Consider feature distribution. Implement...
Use datetime indexing, resample(), rolling(). Handle time zones, frequencies. Implement time-based operations....
Use SMOTE for oversampling, undersampling techniques. Implement class weights. Consider ensemble methods. Handle...
Use SelectKBest, RFE, feature importance from models. Consider correlation analysis, mutual information. Implement...
Use IQR method, z-score method. Consider domain knowledge for outlier definition. Implement proper outlier treatment...
Use chunking, memory efficient methods (read_csv chunks). Consider dtype optimization. Implement proper indexing...
Use random sampling, stratified sampling, systematic sampling. Consider sample size, representation. Implement...
Use merge(), concat(), join(). Handle different join types. Consider memory implications. Implement proper key...
Use PCA, t-SNE, UMAP. Consider feature importance, correlation. Implement proper validation strategy. Handle scaling...
Use tokenization, stemming/lemmatization. Handle stop words, special characters. Implement proper text cleaning...
Use KFold, StratifiedKFold, TimeSeriesSplit. Handle validation strategy selection. Consider data characteristics....
Use Pipeline class, FeatureUnion. Handle preprocessing steps. Implement proper transformation order. Consider...
Use correlation analysis, VIF calculation. Consider feature selection strategies. Implement proper feature...
Use scipy.stats for statistical tests. Handle different test types (t-test, chi-square). Consider assumptions,...
Use DVC (Data Version Control), implement proper tracking. Handle dataset versions. Consider storage implications....
Use vectorization, proper array operations. Consider memory layout. Implement efficient algorithms. Handle large...
Create interaction features, polynomial features. Handle domain-specific transformations. Implement proper feature...
Implement data validation rules, quality metrics. Handle data integrity checks. Consider domain constraints....
Use polynomial features, spline transformations. Consider feature transformations. Implement proper validation...
Use multiprocessing, Dask for parallel operations. Handle memory management. Consider scalability issues. Implement...
Implement different augmentation strategies. Handle domain-specific augmentation. Consider data balance. Implement...
Use appropriate streaming libraries, implement proper buffering. Handle real-time updates. Consider memory...
Use SHAP values, feature importance analysis. Implement model-specific interpretation techniques. Consider global vs...
GIL is a mutex that protects access to Python objects, preventing multiple native threads from executing Python...
Threading shares memory space, affected by GIL, good for I/O-bound tasks. Multiprocessing uses separate memory...
Use async/await syntax, create event loop using asyncio.get_event_loop(). Handle coroutine execution, task...
Lock, RLock (reentrant lock), Semaphore, Event, Condition, Barrier. Each serves different synchronization needs....
Use multiprocessing.Queue, Pipe, shared memory (Value, Array). Handle synchronization properly. Consider...
gather() returns results in order, raises first exception. wait() provides more control over completion, timeout,...
Use concurrent.futures.ThreadPoolExecutor. Handle task submission, result collection. Implement proper shutdown....
Race conditions occur when multiple threads access shared data simultaneously. Prevent using locks, atomic...
Prevent using lock ordering, timeouts. Detect using deadlock detection algorithms. Implement recovery mechanisms....
async with handles asynchronous context managers. async for iterates over async iterables. Properly handle resource...
Use multiprocessing.Pool or concurrent.futures.ProcessPoolExecutor. Handle task distribution, result collection....
Implement proper exception handling in worker threads/processes. Handle task failures gracefully. Consider exception...
Use Queue from queue module, implement proper locking. Consider atomic operations. Use thread-safe collections....
CPU-bound tasks primarily use processor (calculations). I/O-bound tasks wait for external operations (network,...
Implement __aenter__ and __aexit__ methods. Handle async resource acquisition/release. Consider exception handling....
Use Queue for thread-safe communication. Implement proper synchronization. Handle termination conditions. Consider...
Use Task.cancel(), handle CancelledError. Implement cleanup handlers. Consider task dependencies. Handle...
Consider GIL implications, use thread-safe operations. Implement proper synchronization. Use atomic operations when...
Use multiprocessing pools, handle data partitioning. Consider communication overhead. Implement proper merging...
Use timeout parameters, implement timeout handling. Consider cancellation. Handle cleanup after timeout. Implement...
Use logging, debugger support for threads/processes. Implement proper monitoring. Consider race condition detection....
Thread-local storage provides thread-specific data storage. Prevents data sharing between threads. Implement using...
Use async def with yield, implement __aiter__ and __anext__. Handle async iteration properly. Consider resource...
Handle pool lifecycle, implement proper shutdown. Consider resource cleanup. Handle worker process errors. Implement...
Use appropriate IPC mechanisms (Queue, Pipe). Handle synchronization properly. Consider serialization overhead....
Use event loops, implement event handlers. Consider event propagation. Handle event ordering. Implement proper error...
Balance thread/process count, optimize resource usage. Consider overhead costs. Implement proper task granularity....
Handle resource limitations, implement proper load balancing. Consider scalability bottlenecks. Handle distributed...
Handle termination signals, implement proper cleanup. Consider ongoing operations. Handle resource release....
PEP 8 defines Python's style guide: indentation (4 spaces), line length (79 chars), naming conventions (snake_case...
Single Responsibility (one purpose per class), Open/Closed (open for extension, closed for modification), Liskov...
Follow PEP 257: describe purpose, parameters, return values, exceptions. Use consistent format (Google, NumPy,...
Catch specific exceptions, not bare except. Use context managers for resource cleanup. Implement proper error...
Extract common code into functions/classes. Use inheritance and composition appropriately. Implement utility...
Pass dependencies as parameters, use dependency containers, implement factory patterns. Consider interface-based...
Use proper package hierarchy, separate concerns, implement clear imports. Follow standard project layout (setup.py,...
Functions should be small, do one thing, have clear names. Use proper parameter design, avoid side effects. Consider...
Use appropriate log levels, implement structured logging. Consider log format, destination. Handle sensitive data...
Check style consistency, proper error handling, test coverage. Review documentation, performance implications....
Use configuration files, environment variables. Implement proper validation. Handle different environments. Consider...
Use abstract base classes (ABC), protocol classes. Implement proper method signatures. Consider duck typing. Handle...
Follow AAA pattern (Arrange-Act-Assert), use clear test names. Implement proper test isolation. Consider test...
Comment why, not what. Keep comments updated with code. Use proper inline documentation. Consider self-documenting...
Make messages clear, actionable. Include relevant context. Consider user perspective. Handle internationalization....
Follow single responsibility, maintain clear interfaces. Consider versioning strategy. Handle dependencies properly....
Use deprecation warnings, provide migration path. Document deprecation timeline. Maintain backward compatibility....
Use factory methods for object creation, implement proper initialization. Consider inheritance hierarchy. Handle...
Use descriptive names, follow conventions. Consider scope visibility. Use proper prefixes/suffixes. Maintain...
Profile before optimizing, focus on bottlenecks. Consider algorithmic efficiency. Use appropriate data structures....
Follow single responsibility, use proper inheritance. Implement encapsulation. Consider composition over...
Minimize global state, use dependency injection. Consider thread safety. Implement proper access patterns. Document...
Group related functionality, maintain clear interfaces. Consider circular dependencies. Document module purpose....
Keep interfaces simple, document clearly. Handle versioning properly. Consider backward compatibility. Implement...
Break down complex functions, use proper abstraction. Implement clear control flow. Consider cognitive complexity....
Use proper wrapper functions, maintain function metadata. Handle arguments properly. Consider chaining decorators....
Implement proper cleanup, handle partial failures. Consider system state. Document recovery procedures. Implement...
Use clear documentation style, keep documentation updated. Consider audience needs. Document assumptions. Implement...
Use meaningful commit messages, proper branching strategy. Consider merge management. Document version changes....
Metaclasses are classes for classes, allowing customization of class creation. Used for API enforcement, attribute...
Descriptors define __get__, __set__, __delete__ methods to customize attribute access. Used in properties, methods,...
Uses reference counting for basic GC, generational GC for cycles. Reference count tracks object references, frees...
ABCs define interface through @abstractmethod, can include implementation. Created using abc.ABC. Enforce interface...
Implement __enter__ and __exit__ methods, or use contextlib.contextmanager decorator. Handle resource...
send() method for two-way communication, throw() for exception injection, close() for generator cleanup. Generator...
Use __getattr__, __setattr__, __getattribute__, __delattr__. Handle attribute lookup, modification, deletion....
MRO determines method lookup order in inheritance. Uses C3 linearization algorithm. Accessible via __mro__...
Implement __iter__ and __next__ methods for iteration. Consider StopIteration handling. Implement proper cleanup....
Implement rich comparison methods (__eq__, __lt__, etc.). Use @functools.total_ordering for complete ordering....
Bound methods are instance-specific, include self parameter automatically. Unbound methods are class-level, require...
Implement __getstate__ and __setstate__ for custom serialization. Handle complex objects, circular references....
Type hints provide static typing information. Used by type checkers (mypy), IDE support. Not enforced at runtime by...
Implement container protocol methods (__len__, __getitem__, etc.). Consider sequence/mapping behavior. Handle...
Class decorators modify/enhance class definitions. Applied using @decorator syntax above class. Can modify class...
Inherit from appropriate exception base class. Implement proper initialization, custom attributes. Consider...
Protocols define interface behavior without inheritance. Use typing.Protocol for static typing. Support structural...
Use __slots__, proper data structures, generators. Consider memory profiling, garbage collection. Handle large...
Assignment expressions (:=) assign and return value in single expression. Used in while loops, if statements, list...
Implement numeric protocol methods (__add__, __mul__, etc.). Handle reverse operations (__radd__, etc.). Consider...
Generics provide type hints for collections/classes. Use typing.Generic, TypeVar. Support parametric polymorphism....
Use @property with caching, implement custom descriptors. Consider computation cost, memory usage. Handle...
@dataclass decorator provides automatic __init__, __repr__, etc. Support inheritance, frozen instances, default...
Use decorator pattern, functools.wraps for metadata preservation. Handle argument passing, return values. Consider...
__aiter__, __anext__, __aenter__, __aexit__ for async operations. Support async context managers, iterators. Handle...
Create descriptor classes with __get__, __set__, __delete__. Handle attribute access, modification. Consider...
Understand object internals, reference counting, memory allocation. Handle weak references, object lifecycle....
Python is a high-level, interpreted programming language known for its readability, simplicity, and versatility. Key features include dynamic typing, automatic memory management, an extensive standard library, support for multiple programming paradigms (procedural, object-oriented, functional), and a large ecosystem of third-party packages.
Python uses automatic memory management through a private heap containing all Python objects and data structures. It employs reference counting for garbage collection and a cyclic garbage collector to detect and collect reference cycles, ensuring efficient memory usage without manual intervention.
Lists are mutable, allowing modifications like adding, removing, or changing elements. Tuples are immutable, meaning once created, their elements cannot be altered. Lists use square brackets [ ], while tuples use parentheses ( ). Tuples can be used as keys in dictionaries due to their immutability.
Python's built-in data types include numeric types (int, float, complex), sequence types (list, tuple, range), text type (str), mapping type (dict), set types (set, frozenset), boolean type (bool), and binary types (bytes, bytearray, memoryview).
You can create a virtual environment using the `venv` module by running `python -m venv myenv`, where `myenv` is the name of the environment. Activate it with `source myenv/bin/activate` on Unix or `myenv\Scripts\activate` on Windows, isolating dependencies for your project.
The `__init__` method is the constructor in Python classes. It initializes the instance attributes of a class when a new object is created, setting up the initial state of the object.
List comprehensions provide a concise way to create lists by iterating over an iterable and optionally including conditional logic. Example: `[x**2 for x in range(10) if x % 2 == 0]` creates a list of squares of even numbers from 0 to 9.
Python uses reference counting to keep track of the number of references to each object. When an object's reference count drops to zero, it's immediately deallocated. Additionally, a cyclic garbage collector handles reference cycles by periodically identifying and collecting objects involved in cycles.
Decorators are functions that modify or enhance other functions or methods without changing their code. They are applied using the `@decorator_name` syntax above a function definition. Common uses include logging, access control, and memoization.
Exceptions are handled using `try` and `except` blocks. Code that may raise an exception is placed inside the `try` block, and the `except` block catches and handles specific exceptions. Optionally, `finally` can be used to execute code regardless of whether an exception occurred.
The four pillars are Encapsulation (bundling data and methods), Abstraction (hiding complex implementation details), Inheritance (deriving new classes from existing ones), and Polymorphism (using a unified interface for different data types).
Inheritance is implemented by defining a class that inherits from a parent class. For example, `class ChildClass(ParentClass):` allows `ChildClass` to inherit attributes and methods from `ParentClass`, promoting code reuse and hierarchical relationships.
Polymorphism allows objects of different classes to be treated as instances of a common superclass, typically using methods with the same name but different implementations. For example, different classes like `Dog` and `Cat` can both have a `speak()` method, which behaves differently depending on the object's class.
Encapsulation is the bundling of data and methods within a class, restricting direct access to some components. In Python, it is achieved using private (prefixing with double underscores `__`) and protected (prefixing with a single underscore `_`) attributes, signaling that they should not be accessed directly outside the class.
Python allows a class to inherit from multiple parent classes by listing them in parentheses. For example, `class Child(Parent1, Parent2):` enables the child class to inherit attributes and methods from both `Parent1` and `Parent2`. Python uses the Method Resolution Order (MRO) to determine the order in which base classes are searched.
Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. This allows the subclass to modify or extend the behavior of that method.
Class methods are methods that receive the class itself as the first argument (conventionally `cls`) and are defined using the `@classmethod` decorator. They can modify class state. Static methods do not receive an implicit first argument and are defined using the `@staticmethod` decorator; they behave like regular functions but belong to the class's namespace.
You can prevent a class from being subclassed by using the `final` decorator from the `typing` module (in Python 3.8+) or by raising an exception in the `__init_subclass__` method. For example: ```python from typing import final @final class Base: pass ``` This will cause an error if someone tries to inherit from `Base`.
The `super()` function returns a temporary object of the superclass, allowing access to its methods. It is commonly used to call the superclass's `__init__` method in the subclass to ensure proper initialization.
Abstraction is implemented using abstract base classes (ABCs) from the `abc` module. By defining abstract methods using the `@abstractmethod` decorator, you can create classes that cannot be instantiated and must have their abstract methods overridden in subclasses. This enforces a common interface.
Missing data can be handled using methods like `df.dropna()` to remove missing values, `df.fillna()` to fill them with specified values or strategies (e.g., mean, median), and `df.isnull()` or `df.notnull()` to detect missing values. Additionally, interpolation methods can estimate missing data.
`loc` is label-based and used for selecting rows and columns by their labels or boolean arrays. `iloc` is integer position-based and selects by integer indices. For example, `df.loc[2, 'A']` selects the value in row labeled 2 and column 'A', while `df.iloc[2, 0]` selects the value at the third row and first column by position.
You can merge two DataFrames using the `pd.merge()` function, specifying the keys to join on and the type of join (e.g., inner, outer, left, right). Alternatively, `df1.join(df2)` can be used for joining on indexes, and `pd.concat([df1, df2])` can concatenate along a particular axis.
The `groupby` function is used to split a DataFrame into groups based on one or more keys, apply a function to each group independently, and then combine the results. It is commonly used for aggregation, transformation, and filtration operations, such as calculating group-wise statistics.
You can apply functions using the `df.apply()` method, specifying `axis=0` for columns or `axis=1` for rows. Additionally, vectorized operations or specific methods like `df.applymap()` for element-wise operations can be used for efficiency.
The `pivot_table` method creates a spreadsheet-style pivot table, allowing you to summarize and aggregate data based on specified index, columns, and values, with support for various aggregation functions. It facilitates data analysis by reorganizing data for better insights.
Pandas provides functions like `pd.read_csv()`, `pd.read_excel()`, `pd.read_json()`, `pd.read_sql()`, and `pd.read_html()` to read various file formats. Similarly, you can write DataFrames using methods like `df.to_csv()`, `df.to_excel()`, `df.to_json()`, and `df.to_sql()` to export data to different formats.
Data filtering and selection can be done using boolean indexing, the `query()` method, `loc` and `iloc` for label or position-based selection, and conditions applied to DataFrame columns. For example, `df[df['age'] > 30]` filters rows where the 'age' column is greater than 30.
Multi-indexes allow pandas DataFrames to have multiple levels of indexing on rows and/or columns. They enable more complex data structures and facilitate hierarchical data organization, making it easier to perform operations like grouping, reshaping, and selecting subsets of data based on multiple keys.
Performance can be optimized by using efficient data types (e.g., categorical data), avoiding unnecessary copies, leveraging vectorized operations instead of loops, using built-in pandas functions, applying chunk processing for large datasets, indexing appropriately, and utilizing parallel processing or libraries like Dask for handling very large DataFrames.
Python is a high-level, interpreted programming language created by Guido van Rossum. It is known for its simplicity, readability, and versatility, with key characteristics including dynamic typing, automatic memory management, and support for multiple programming paradigms.
Python 3 is a major revision of the language that is not fully backward-compatible with Python 2. Key differences include print function vs. print statement, integer division behavior, Unicode string handling, and removal of certain legacy syntax.
The Global Interpreter Lock is a mutex that prevents multiple native threads from executing Python bytecodes simultaneously. It's a mechanism in CPython that ensures thread safety but can limit the performance of multi-threaded programs, especially in CPU-bound tasks.
Python supports multiple programming paradigms, including object-oriented programming (OOP), functional programming, and procedural programming. It allows developers to use different programming styles based on their requirements.
Python uses automatic memory management with reference counting and garbage collection. Objects are created dynamically, and memory is automatically allocated and freed. The reference count of an object is incremented when it's assigned to a variable and decremented when references are removed.
Duck typing is a dynamic typing concept in Python where the type or class of an object is less important than the methods it defines. If an object has all the methods and properties required for a particular use, it can be used, regardless of its actual type.
.py files are source code files containing Python script, while .pyc files are compiled bytecode files that are created to improve performance by reducing compilation time in subsequent runs.
Decorators are a design pattern in Python that allows modifying or enhancing functions or classes without directly changing their source code. They are functions that take another function as an argument and return a modified version of that function.
Method Resolution Order (MRO) is the order in which Python looks for methods in a hierarchy of classes. It uses the C3 linearization algorithm to determine the order of method resolution in multiple inheritance scenarios, ensuring a consistent and predictable method lookup.
PEP 8 is the style guide for Python code that provides conventions for writing readable and consistent Python code. It covers aspects like indentation, naming conventions, maximum line length, and other coding standards to improve code readability and maintainability.
Lambda functions are small, anonymous functions defined using the 'lambda' keyword. They can have any number of arguments but can only have one expression. They are often used for short, one-time use functions, especially as arguments to higher-order functions.
The 'with' statement is used for resource management, ensuring that a resource is properly acquired and released. It simplifies exception handling by automatically calling close() or exit() methods, making code cleaner and reducing the risk of resource leaks.
A shallow copy creates a new object but references the same memory addresses for nested objects, while a deep copy creates a completely independent copy of an object and all its nested objects. The copy module provides methods for both types of copying.
Python uses namespaces to organize and manage variable names. It follows the LEGB rule (Local, Enclosing, Global, Built-in) for variable scope resolution. Each namespace is a mapping from names to objects, and Python searches for names in this specific order.
Generator functions use the 'yield' keyword to return a generator iterator. They allow you to generate values on-the-fly and pause the function's state, making them memory-efficient for handling large datasets or infinite sequences.
The __init__ method is a special constructor method in Python classes that is automatically called when a new object is created. It initializes the object's attributes and can take parameters to set up the initial state of the object.
Python supports multiple inheritance, allowing a class to inherit from multiple parent classes. It uses the Method Resolution Order (MRO) to determine the order of method inheritance, resolving potential conflicts using the C3 linearization algorithm.
Context managers are objects that define __enter__ and __exit__ methods, used with the 'with' statement to manage resources. They provide a clean way to set up and tear down resources, ensuring proper initialization and cleanup.
The '==' operator checks for value equality, comparing the values of two objects, while the 'is' operator checks for identity, verifying if two references point to the exact same object in memory.
Metaclasses are classes of classes, allowing you to customize class creation. They define how a class behaves and can modify the class definition at runtime. The type class is the default metaclass in Python.
Python uses try-except blocks to handle exceptions. You can catch specific exceptions, use multiple except blocks, include an else clause for code to run if no exception occurs, and use a finally clause for cleanup code that always runs.
__str__ provides a human-readable string representation of an object, typically used by print(), while __repr__ returns a more detailed, unambiguous representation often used for debugging and development.
Python uses reference counting and generational garbage collection. When an object's reference count drops to zero, it's immediately deallocated. For circular references, a cyclic garbage collector periodically runs to identify and remove unreachable objects.
Magic methods, identified by double underscores (e.g., __init__, __str__), allow customization of object behavior. They define how objects interact with built-in functions and operators, enabling operator overloading and custom object implementations.
Python supports functional programming through features like lambda functions, map(), filter(), reduce(), comprehensions, and first-class functions. These allow for writing code in a more declarative and concise manner.
Abstract base classes (defined using the abc module) can have both abstract and concrete methods, while Python doesn't have traditional interfaces. Abstract base classes provide a way to define a blueprint for other classes, ensuring certain methods are implemented.
Type hinting allows specifying expected types for function parameters and return values. Introduced in Python 3.5, it improves code readability, helps catch type-related errors early, and enables better IDE support and static type checking.
*args allows a function to accept any number of positional arguments, while **kwargs allows accepting any number of keyword arguments. They provide flexibility in function definitions and are commonly used in wrapper functions and class inheritance.
Python uses naming conventions for encapsulation. Single underscore prefix (e.g., _variable) suggests internal use, double underscore prefix (e.g., __variable) triggers name mangling to prevent naming conflicts in inheritance.
Lists are mutable (can be modified after creation) and use square brackets [], while tuples are immutable (cannot be modified after creation) and use parentheses (). Lists have methods like append(), remove(), while tuples have limited methods due to immutability.
'==' compares the values of objects (equality), while 'is' compares the identity (memory location) of objects. For example, a == b checks if a and b have the same value, while a is b checks if they are the same object in memory.
Python has int (integer), float (floating-point), complex (complex numbers) as numeric types. Integers have unlimited precision, floats are typically double-precision, and complex numbers have real and imaginary parts.
Python uses LEGB rule: Local, Enclosing, Global, Built-in. Variables are first searched in local scope, then enclosing functions, then global scope, and finally built-in scope. Use 'global' keyword to modify global variables from local scope.
List comprehension is a concise way to create lists based on existing lists/iterables. Syntax: [expression for item in iterable if condition]. Advantages include more readable and compact code, better performance than equivalent for loops, and clearer intent.
Slicing extracts parts of sequences using syntax sequence[start:stop:step]. Start is inclusive, stop is exclusive. Negative indices count from end. Extended form allows step value to control direction and skipping. Default step is 1.
Python offers multiple string formatting methods: %-formatting (old style), str.format() method, and f-strings (3.6+). F-strings are most readable: f'{variable}'. Format() uses {}, %-formatting uses %d, %s etc. Each has different use cases and syntax.
Python includes arithmetic (+, -, *, /, //, %, **), comparison (==, !=, >, <, >=, <=), logical (and, or, not), bitwise (&, |, ^, ~, <<, >>), assignment (=, +=, -=, etc.), and identity/membership (is, is not, in, not in) operators.
Type hints are added using annotations: function parameters (param: type), return types (-> type), and variable annotations (var: type). Use typing module for complex types. They're hints only, not enforced at runtime by default.
Generator expressions create iterators using syntax (expression for item in iterable if condition). Similar to list comprehension but more memory efficient for large datasets as they generate values on-the-fly instead of storing all at once.
Shallow copy creates new object but references same nested objects (copy.copy()). Deep copy creates new object and recursively copies nested objects (copy.deepcopy()). Choose based on whether nested objects need independent copies.
Python offers if/elif/else statements, ternary operators (value_if_true if condition else value_if_false), and conditional expressions. Also supports match/case (3.10+) for pattern matching and boolean short-circuit evaluation.
Walrus operator assigns values in expressions: while (n := len(a)) > 0. Benefits include more concise code, avoiding repetitive expressions, and combining assignment with conditional checks. Introduced in Python 3.8.
Python provides break (exit loop), continue (skip to next iteration), else (executes when loop completes normally), and pass (null statement) for loop control. Can be used in both for and while loops.
Python assigns references to objects, not objects themselves. Multiple names can reference same object. Assignment creates new reference, not copy. Understanding this is crucial for mutable vs immutable objects behavior.
Unpacking assigns sequence elements to variables: a, b = [1, 2]. Packing collects multiple values into sequence using *args for positional and **kwargs for keyword arguments. Supports extended unpacking with * operator.
Use encode() to convert string to bytes, decode() for bytes to string. Specify encoding (e.g., 'utf-8', 'ascii'). Handle encoding errors with error handlers like 'ignore', 'replace', 'strict'. Important for file I/O and network operations.
Python 2's range creates list, xrange creates iterator. Python 3's range creates range object (iterator-like). Range object more memory efficient as it generates values on demand rather than storing all in memory.
Boolean operations (and, or, not) follow short-circuit rules. 'and' returns first false value or last value, 'or' returns first true value or last value. Short-circuiting optimizes evaluation by skipping unnecessary checks.
F-strings (formatted string literals) allow embedding expressions: f'{var=}'. Support format specifiers, multiline strings, expressions, and = specifier for debugging. More readable and performant than older formatting methods.
/ performs true division (returns float), // performs floor division (returns int for ints). Floating-point follows IEEE 754. Be aware of floating-point precision issues and decimal module for exact decimal arithmetic.
Use # for single-line comments, triple quotes (''' or """) for multiline comments/docstrings. Docstrings document modules, classes, methods. Comments explain why, not what. Follow PEP 257 for docstring conventions.
Match statements (3.10+) provide pattern matching: match value: case pattern: .... Supports patterns like literals, sequences, mappings, classes. More powerful than switch statements in other languages.
Follow PEP 8: lowercase_with_underscores for functions/variables, CapitalizedWords for classes, _single_leading_underscore for internal use, __double_leading_underscore for name mangling, UPPERCASE for constants.
Python follows operator precedence: exponentiation highest, then multiplication/division, then addition/subtraction. Parentheses override precedence. Bitwise operators have specific precedence. Important for complex expressions.
Support binary (0b), octal (0o), hexadecimal (0x) literals. Convert between bases using bin(), oct(), hex(), int(str, base). Important for bit manipulation and low-level operations.
Average case time complexities for dictionary operations are: Get item O(1), Set item O(1), Delete item O(1), Search O(1). However, worst case can be O(n) due to hash collisions. Dictionary uses hash table implementation for efficient access.
Lists are dynamic arrays that can store elements of different types. Arrays (from array module) store homogeneous data, are more memory efficient, and support mathematical operations. Lists offer more flexibility but use more memory due to storing references.
heapq implements min heap, providing O(log n) push/pop operations. Used for priority queues, finding n smallest/largest elements, and scheduling tasks. Methods include heappush(), heappop(), heapify(). Can implement max heap by negating values.
sorted() creates new sorted list, leaving original unchanged. list.sort() modifies list in-place. Both use Timsort algorithm, accept key function and reverse parameter. sorted() works on any iterable, while sort() is list-only method.
Implement using class with Node class (value, left, right pointers) and Tree class with insert, delete, search methods. Balance tree using rotations. Time complexity O(log n) average case, O(n) worst case for unbalanced tree.
Deques (collections.deque) are double-ended queues supporting O(1) append/pop at both ends. Used for sliding windows, maintain last n items, implement queues/stacks efficiently. More efficient than lists for these operations.
bisect module provides binary search functionality for sorted sequences. bisect_left finds insertion point for element to maintain sort order, bisect_right finds rightmost position. O(log n) time complexity. Useful for maintaining sorted sequences.
Sets use hash tables with open addressing to handle collisions. When collision occurs, probing finds next empty slot. Load factor determines resize threshold. Set operations (union, intersection) are optimized using hash-based algorithms.
Python uses Timsort, hybrid of merge sort and insertion sort. Stable sort with O(n log n) worst case. Adaptive algorithm that performs well on real-world data with partially ordered sequences. Minimizes comparisons using galloping mode.
Create Node class with value and next pointer. LinkedList class manages head pointer, implements insert, delete, traverse methods. Optional: add tail pointer for O(1) append. Consider implementing iterator protocol for traversal.
OrderedDict maintains insertion order (pre-3.7), useful for LRU caches. defaultdict provides default values for missing keys, simplifying handling of nested structures and counters. Both from collections module.
Represent graphs using adjacency lists (dictionaries) or matrices. Implement BFS/DFS using queue/stack. Use dict/set for visited nodes. Consider weight handling for Dijkstra/shortest paths. Implement path finding and cycle detection.
Tuples are immutable, slightly more memory efficient, can be dictionary keys. Lists are mutable, support item assignment, have more methods. Tuples often used for returning multiple values, representing fixed collections.
Use memoization (decorator or dict) or tabulation (arrays). Handle base cases, build solution using smaller subproblems. Consider space optimization using rolling arrays. Common in optimization problems.
Python uses open addressing with random probing. Alternative methods: chaining (linked lists), linear probing, quadratic probing. Load factor determines rehashing. Performance depends on hash function quality and collision resolution.
Stack: use append() and pop() for LIFO. Queue: use append() and pop(0)/list.pop(0) for FIFO (inefficient, use collections.deque instead). Consider implementing size limits and empty checks.
Counter from collections creates dictionary subclass for counting hashable objects. Supports addition, subtraction, intersection, union. Methods: most_common(), elements(). Useful for frequency counting and multisets.
Create TrieNode class with children dict and is_end flag. Implement insert, search, startswith methods. Use dict for child nodes. Time complexity O(m) for operations where m is key length. Useful for autocomplete, spell check.
bytearray is mutable version of bytes. Supports in-place modifications, useful for building binary data incrementally. Methods like append(), extend(), reverse(). Consider for large binary data manipulation.
Implement iteratively or recursively on sorted sequence. Handle mid calculation, comparison, and boundary updates. Time complexity O(log n). Consider bisect module for built-in implementation. Handle edge cases and duplicates.
append() adds single element, extend() adds elements from iterable. append([1,2]) adds list as single element, extend([1,2]) adds individual elements. extend() equivalent to += operator. Consider memory and performance implications.
Use heapq module for min heap implementation. Wrap elements in tuples with priority. Alternative: queue.PriorityQueue for thread-safe version. Operations: push O(log n), pop O(log n). Handle custom comparison.
String concatenation with += creates new string objects. Use join() method for multiple concatenations, more efficient. For building strings, consider list of strings or io.StringIO. String interning affects memory usage.
Implement find and union operations using dictionary/array. Use path compression and union by rank optimizations. Time complexity nearly O(1) with optimizations. Used in Kruskal's algorithm, connected components.
In Python 2, range creates list, xrange creates iterator object. Python 3's range is like xrange, memory efficient iterator. Use for loops, sequence generation. Consider memory usage for large ranges.
Use decorator with cache dict, or functools.lru_cache. Store function arguments as keys. Handle mutable arguments. Consider cache size limits and cleanup. Used in dynamic programming, expensive computations.
Use immutable types (strings, numbers, tuples of immutables). Consider hash collisions and distribution. Custom objects need __hash__ and __eq__. Avoid mutable keys that could change hash value.
Quicksort: partition around pivot, recursively sort subarrays. Mergesort: divide array, sort recursively, merge sorted halves. Consider in-place vs new array, stability requirements, pivot selection strategies.
itertools provides efficient iteration tools: combinations(), permutations(), product(), cycle(), chain(). Memory efficient iterators for combinatorial operations. Used in generating test cases, processing sequences.
A class is a blueprint for objects, defining attributes and methods. An object is an instance of a class. Classes define structure and behavior, while objects contain actual data. Example: class Dog defines properties like breed, while a specific dog object represents an actual dog with those properties.
Inheritance allows a class to inherit attributes and methods from another class. Implemented using class Child(Parent). Supports single, multiple, and multilevel inheritance. Example: class Car(Vehicle) inherits from Vehicle class. Use super() to access parent class methods.
Instance methods (default) have self parameter, access instance data. Class methods (@classmethod) have cls parameter, access class data. Static methods (@staticmethod) have no special first parameter, don't access class/instance data. Each serves different purpose in class design.
Python uses name mangling with double underscore prefix (__var) for private attributes. Single underscore (_var) for protected attributes (convention). No true private variables, but follows 'we're all consenting adults' philosophy. Access control through properties and descriptors.
Magic methods control object behavior for built-in operations. Examples: __init__ for initialization, __str__ for string representation, __len__ for length, __getitem__ for indexing. Enable operator overloading and customize object behavior.
Polymorphism implemented through method overriding in inheritance and duck typing. Same method name behaves differently for different classes. No need for explicit interface declarations. Example: different classes implementing same method name but different behaviors.
Properties (@property decorator) provide getter/setter functionality with attribute-like syntax. Control access to attributes, add validation, make attributes read-only. Example: @property for getter, @name.setter for setter. Used for computed attributes and encapsulation.
Multiple inheritance allows class to inherit from multiple parents: class Child(Parent1, Parent2). MRO determines method lookup order using C3 linearization algorithm. Access MRO using Class.__mro__. Handle diamond problem through proper method resolution.
Metaclasses are classes for classes, allow customizing class creation. Created using type or custom metaclass. Used for API creation, attribute/method validation, class registration, abstract base classes. Example: ABCMeta for abstract classes.
Descriptors control attribute access through __get__, __set__, __delete__ methods. Used in properties, methods, class attributes. Enable reusable attribute behavior. Example: implementing validation, computed attributes, or attribute access logging.
Composition creates objects containing other objects as parts (has-a relationship), while inheritance creates is-a relationships. Composition more flexible, reduces coupling. Example: Car has-a Engine vs. ElectricCar is-a Car.
Use abc module with ABC class and @abstractmethod decorator. Abstract classes can't be instantiated, enforce interface implementation. Example: from abc import ABC, abstractmethod. Used for defining common interfaces and ensuring implementation.
Class variables shared among all instances (defined in class), instance variables unique to each instance (defined in __init__). Class variables accessed through class or instance, modified through class. Be careful with mutable class variables.
super() returns proxy object for delegating method calls to parent class. Handles multiple inheritance correctly using MRO. Used in __init__ and other overridden methods. Example: super().__init__() calls parent's __init__.
__new__ creates instance, called before __init__. __init__ initializes instance after creation. __new__ rarely overridden except for singletons, immutables. __new__ is static method, __init__ is instance method.
Implement using metaclass, __new__ method, or module-level instance. Control instance creation, ensure single instance exists. Handle thread safety if needed. Example: override __new__ to return existing instance or decorator approach.
__slots__ restricts instance attributes to fixed set, reduces memory usage. Faster attribute access, prevents dynamic attribute addition. Trade flexibility for performance. Used in classes with fixed attribute set.
Use __getattr__, __setattr__, __getattribute__, __delattr__ for custom attribute access. __getattr__ called for missing attributes, __getattribute__ for all attribute access. Be careful with infinite recursion.
Method overriding redefines method from parent class in child class. Used to specialize behavior while maintaining interface. Call parent method using super() when needed. Example: overriding __str__ for custom string representation.
Override special methods for operators: __add__ for +, __eq__ for ==, etc. Enable class instances to work with built-in operators. Example: implement __lt__ for sorting. Consider reverse operations (__radd__, etc.).
Class decorators modify or enhance class definitions. Applied using @decorator syntax above class. Can add attributes, methods, or modify class behavior. Example: dataclass decorator for automatic __init__, __repr__.
Use __slots__, @property with only getter, override __setattr__/__delattr__. Store data in private tuples, implement __new__ instead of __init__. Consider frozen dataclasses. Make all instance data immutable.
__str__ for human-readable string representation, __repr__ for unambiguous representation (debugging). __repr__ should be complete enough to recreate object if possible. Default to __repr__ if __str__ not defined.
Implement __enter__ and __exit__ methods. __enter__ sets up context, __exit__ handles cleanup. Used with 'with' statement. Alternative: @contextmanager decorator for function-based approach.
Mixins are classes providing additional functionality through multiple inheritance. Used for reusable features across different classes. Keep mixins focused, avoid state. Example: LoggerMixin for adding logging capability.
Use pickle for Python-specific serialization, implement __getstate__/__setstate__ for custom serialization. Consider JSON serialization with custom encoders/decoders. Handle security implications of deserialization.
Dataclasses (@dataclass) automatically add generated methods (__init__, __repr__, etc.). Used for classes primarily storing data. Support comparison, frozen instances, inheritance. More concise than manual implementation.
Implement container protocol methods: __len__, __getitem__, __setitem__, __delitem__, __iter__. Optional: __contains__, __reversed__. Consider collections.abc base classes. Example: custom list or dictionary implementation.
__dict__ stores instance attributes in dictionary. Enables dynamic attribute addition. Not present when using __slots__. Accessed for introspection, serialization. Consider memory implications for many instances.
Lambda functions are anonymous, single-expression functions using lambda keyword. Syntax: lambda args: expression. Best for simple operations, function arguments, and when function is used once. Example: lambda x: x*2. Limited to single expression, no statements allowed.
List comprehensions create lists using compact syntax: [expression for item in iterable if condition]. More readable and often faster than loops. Creates new list in memory. Example: [x**2 for x in range(10) if x % 2 == 0]. Good for transforming and filtering data.
Decorators are higher-order functions that modify other functions. Use @decorator syntax or function_name = decorator(function_name). Common uses: logging, timing, authentication, caching. Implement using nested functions or classes with __call__. Can take arguments using decorator factories.
map(func, iterable) applies function to each element. filter(func, iterable) keeps elements where function returns True. reduce(func, iterable) accumulates values using binary function. All return iterators (except reduce). Consider list comprehensions or generator expressions as alternatives.
Generators are functions using yield keyword to return values incrementally. Create iterator objects, maintain state between calls. Memory efficient for large sequences. Used with for loops, next(). Example: def gen(): yield from range(10). Don't store all values in memory.
Python uses LEGB scope (Local, Enclosing, Global, Built-in). Closures capture variable values from enclosing scope. Use nonlocal for enclosing scope, global for global scope. Closures common in decorators and factory functions.
Annotations provide type hints: def func(x: int) -> str. Stored in __annotations__ dict. Not enforced at runtime, used by type checkers, IDEs, documentation. PEP 484 defines type hinting standards. Help with code understanding and verification.
Use functools.partial to create new function with fixed arguments. Reduces function arity by pre-filling arguments. Example: from functools import partial; new_func = partial(original_func, arg1). Useful for callback functions and function factories.
Positional arguments (standard), keyword arguments (name=value), default arguments (def func(x=1)), variable arguments (*args for tuple, **kwargs for dict). Order matters: positional, *args, keyword, **kwargs. Consider argument flexibility vs clarity.
Use @functools.lru_cache decorator or implement custom caching with dict. Stores function results, returns cached value for same arguments. Consider cache size, argument hashability. Example: @lru_cache(maxsize=None). Good for expensive computations.
Currying transforms function with multiple arguments into series of single-argument functions. Implement using nested functions or partial application. Example: def curry(f): return lambda x: lambda y: f(x,y). Used for function composition and partial application.
Recursion is function calling itself. Limited by recursion depth (default 1000). Use tail recursion optimization or iteration for deep recursion. Example: factorial implementation. Consider stack overflow risk and performance implications.
Higher-order functions take functions as arguments or return functions. Examples: map, filter, decorators. Enable function composition and abstraction. Consider type hints for function arguments. Common in functional programming patterns.
Pure functions always return same output for same input, have no side effects. Benefits: easier testing, debugging, parallelization. Example: def add(x,y): return x+y. Avoid global state, I/O operations. Key concept in functional programming.
Use docstrings (triple quotes) for function documentation. Include description, parameters, return value, examples. Access via help() or __doc__. Follow PEP 257 conventions. Consider tools like Sphinx for documentation generation.
Dict comprehension: {key:value for item in iterable}. Set comprehension: {expression for item in iterable}. More concise than loops, creates new dict/set. Example: {x:x**2 for x in range(5)}. Consider readability vs complexity.
Python doesn't support traditional overloading. Use default arguments, *args, **kwargs, or @singledispatch decorator. Alternative: multiple dispatch based on argument types. Consider interface clarity vs flexibility.
Coroutines use async/await syntax, support concurrent programming. Unlike generators, can receive values with send(). Used with asyncio for asynchronous operations. Example: async def coro(): await operation(). Consider event loop integration.
Nested functions have access to outer function variables (closure). Use nonlocal keyword to modify enclosed variables. Common in decorators and callbacks. Consider readability and maintenance implications.
Function composition combines functions: f(g(x)). Implement using higher-order functions or operator module. Example: compose = lambda f, g: lambda x: f(g(x)). Consider readability and error handling.
Implement __iter__ and __next__ methods. __iter__ returns iterator object, __next__ provides next value or raises StopIteration. Used in for loops, generators. Example: custom range implementation. Consider memory efficiency.
functools provides tools for functional programming: reduce, partial, lru_cache, wraps, singledispatch. Enhances function manipulation and optimization. Example: @wraps preserves function metadata in decorators.
Functions are objects with attributes. Access/set via function.__dict__. Common attributes: __name__, __doc__, __module__. Use @wraps to preserve metadata in decorators. Consider documentation and debugging implications.
Tail recursion occurs when recursive call is last operation. Python doesn't optimize tail recursion automatically. Convert to iteration or use trampolining for optimization. Consider stack overflow prevention.
Return self from methods to enable chaining: obj.method1().method2(). Common in builder pattern and fluent interfaces. Consider readability and maintenance. Example: query builders, object configuration.
Use default arguments, None values, or *args/**kwargs. Consider parameter order, default mutability issues. Example: def func(required, optional=None). Document parameter optionality clearly.
Function factories create and return new functions. Use closures to capture configuration. Common in parameterized decorators, specialized functions. Example: def make_multiplier(n): return lambda x: x * n.
Use Either/Maybe patterns, return tuple of (success, result), or raise exceptions. Handle errors explicitly in function composition. Consider monadic error handling. Maintain functional purity where possible.
Basic structure uses try/except blocks: try to execute code that might raise exception, except to handle specific exceptions. Optional else clause for code when no exception occurs, finally for cleanup. Example: try: risky_operation() except TypeError: handle_error()
Create custom exceptions by inheriting from Exception class or specific exception types. Raise using raise keyword. Include meaningful error messages and attributes. Example: class CustomError(Exception): pass; raise CustomError('message')
finally clause executes regardless of whether exception occurred or was handled. Used for cleanup operations like closing files, database connections. Executes even if return, break, or continue occurs in try/except. Ensures resource cleanup.
Use logging module with different levels (DEBUG, INFO, WARNING, ERROR, CRITICAL). Configure handlers, formatters, log destinations. Example: logging.basicConfig(level=logging.INFO). Consider log rotation, timestamp formats, context information.
pdb is Python's built-in debugger, ipdb adds IPython features like tab completion, syntax highlighting. Both support stepping through code, inspecting variables, setting breakpoints. Commands: n(next), s(step), c(continue), q(quit).
Group exceptions in tuple: except (TypeError, ValueError) as e. Access exception info using 'as' keyword. Consider exception hierarchy, order from specific to general. Handle each exception type appropriately.
Context managers (with statement) ensure proper resource cleanup. Implement __enter__ and __exit__ methods or use contextlib.contextmanager. Handle exceptions in __exit__. Example: file handling, database connections.
Use memory_profiler, tracemalloc, gc module. Monitor object references, circular references. Tools: objgraph for visualization, sys.getsizeof() for object size. Consider weak references, proper cleanup in __del__.
Assertions (assert condition, message) check program correctness, disabled with -O flag. Exceptions handle runtime errors. Assertions for debugging/development, exceptions for runtime error handling. Use assertions for invariants.
Use error tracking services (Sentry, Rollbar). Implement custom error handlers, logging middleware. Capture stack traces, context information. Consider environment-specific handling, error aggregation, alert thresholds.
Create decorators for timing, logging, error catching. Example: @log_errors, @retry, @timeout. Handle function metadata using functools.wraps. Consider performance impact, logging levels.
Use thread-specific exception handlers, threading.excepthook. Handle exceptions within thread function. Consider thread safety in logging, global error handlers. Implement proper thread cleanup.
Create base exception class for application. Structure hierarchy based on error types. Include relevant error information, maintain backwards compatibility. Example: AppError -> ValidationError -> FieldValidationError.
Use breakpoints, print debugging, sys.setrecursionlimit(). Monitor stack traces, memory usage. Tools: pdb for stepping through code. Implement timeout mechanisms, recursion depth checking.
sys.excepthook handles uncaught exceptions. Customize for global error handling, logging. Access exception type, value, traceback. Consider different handling for different environments (dev/prod).
Use decorators or context managers for retries. Handle specific exceptions, implement backoff strategy. Example: @retry(times=3, exceptions=(NetworkError,)). Consider timeout, max attempts, delay between retries.
IDEs provide integrated debuggers, breakpoints, variable inspection, call stack viewing. Popular: PyCharm debugger, VS Code debugger. Features: conditional breakpoints, expression evaluation, watch variables.
Use try/except in async functions, handle event loop exceptions. asyncio.excepthook for loop exceptions. Consider concurrent error handling, task cancellation. Example: async with AsyncExitStack().
Implement consistent error response format, HTTP status codes. Include error codes, messages, debug information. Consider API versioning, client error handling. Example: {error: {code: 'VAL_001', message: 'Invalid input'}}.
Use profilers (cProfile, line_profiler), timing decorators. Monitor CPU usage, memory allocation. Tools: py-spy for sampling profiler. Consider bottlenecks, optimization opportunities.
Include specific error details, context information. Make messages user-friendly, actionable. Consider internationalization, security implications. Example: 'Failed to connect to database at host:port: timeout after 30s'.
Create wrapper classes/functions for error containment. Handle subsystem failures gracefully. Consider component isolation, fallback behavior. Example: class ErrorBoundary context manager.
Use source-level debugging, monkey patching. Inspect library source, logging integration. Consider version compatibility, issue tracking. Tools: debugger step-into, logging interception.
Implement specific exception handling for DB errors. Handle connection issues, transaction rollback. Consider retry logic, connection pooling. Example: handle SQLAlchemy exceptions specifically.
Use thread/process-specific logging, race condition detection. Tools: threading.VERBOSE, multiprocessing debug logs. Consider deadlock detection, thread sanitizers.
Create recovery procedures for critical errors. Implement state restoration, data consistency checks. Consider transaction boundaries, cleanup operations. Example: backup/restore mechanisms.
Validate configuration early, provide clear error messages. Handle missing/invalid settings gracefully. Consider environment-specific defaults, configuration versioning. Example: config validation decorators.
Use memory_profiler, guppy3 for heap analysis. Monitor object lifecycle, memory patterns. Consider garbage collection timing, object pooling. Tools: objgraph for reference visualization.
Implement data masking, logging filters. Handle PII, credentials securely. Consider compliance requirements, log retention policies. Example: custom log formatters for sensitive data.
Common modes: 'r' (read), 'w' (write), 'a' (append), 'b' (binary), '+' (read/write). Can combine modes: 'rb' (read binary), 'w+' (read/write). Default is text mode, 'b' for binary. Consider encoding parameter for text files.
Use 'with' statement: 'with open(filename) as f:'. Ensures proper file closure even if exceptions occur. Best practice over manual open/close. Can handle multiple files: 'with open(f1) as a, open(f2) as b:'.
read() reads entire file into string, readline() reads single line, readlines() reads all lines into list. read() can specify size in bytes. Consider memory usage for large files. Use iteration for efficient line reading.
Use csv module: reader, writer, DictReader, DictWriter classes. Handle delimiters, quoting, escaping. Consider newline='' parameter when opening. Example: csv.DictReader for named columns. Handle different dialects.
Use json module: json.load(file), json.dump(data, file). Handle encoding, pretty printing (indent parameter). Consider custom serialization (default parameter). Handle JSON parsing errors, large files.
Use 'b' mode flag, read/write bytes objects. Methods: read(size), write(bytes). Consider bytearray for mutable binary data. Handle endianness, struct module for binary structures. Buffer protocols.
Buffering options: 0 (unbuffered), 1 (line buffered), >1 (size in bytes). Default is system dependent. Set with buffering parameter. Consider performance implications, memory usage. Flush buffer manually with flush().
Specify encoding parameter in open(): UTF-8, ASCII, etc. Handle encoding/decoding errors with errors parameter. Use codecs module for advanced encoding. Consider BOM, default system encoding.
Use mmap module for memory-mapped file I/O. Treats file as memory buffer. Good for large files, shared memory. Consider platform differences, file size limitations. Handle proper cleanup.
Use fcntl module (Unix) or msvcrt (Windows). Implement advisory locking. Handle shared vs exclusive locks. Consider timeout, deadlock prevention. Example: with FileLock(filename):.
Use tempfile module: NamedTemporaryFile, TemporaryDirectory. Auto-cleanup when closed. Secure creation, unique names. Consider cleanup on program exit. Handle permissions properly.
Use generators for line iteration, chunk reading. Consider memory usage, buffering. Use mmap for random access. Implement progress tracking. Handle cleanup properly. Consider parallel processing.
Objects implementing file interface (read, write, etc.). Examples: StringIO, BytesIO for in-memory files. Used for compatibility with file operations. Consider context manager implementation.
Use os.path or pathlib for path operations. Handle permissions, existence checks. Consider race conditions, atomic operations. Implement proper error handling. Use shutil for high-level operations.
Use configparser for INI files, yaml for YAML. Handle defaults, validation. Consider environment overrides. Implement config reloading. Handle sensitive data properly.
Use watchdog library or platform-specific APIs. Handle file system events. Consider polling vs event-based. Implement proper cleanup. Handle recursive monitoring.
Use pathlib.Path for object-oriented path operations. Handle platform differences, relative paths. Consider path normalization, validation. Handle special characters, spaces.
Use openpyxl, pandas for XLSX files. xlrd/xlwt for older formats. Handle sheets, formatting, formulas. Consider memory usage for large files. Implement proper cleanup.
Use gzip, zipfile, tarfile modules. Handle compression levels, passwords. Consider streaming for large files. Implement progress tracking. Handle multiple file archives.
Use glob, fnmatch for patterns. re module for regex. Consider recursive search, filters. Handle large directories efficiently. Implement proper error handling.
Use rotating file handlers, proper formatting. Handle log levels, rotation size. Consider compression, retention policy. Implement proper cleanup. Handle concurrent access.
Use os.stat, Path.stat() for metadata. Handle timestamps, permissions. Consider platform differences. Implement proper error handling. Handle symbolic links.
Use os.replace for atomic writes. Implement write-to-temp-then-rename pattern. Handle concurrent access. Consider file system limitations. Implement proper error recovery.
Use os.chmod, Path.chmod() for permissions. Handle umask settings. Consider security implications. Implement least privilege principle. Handle permission inheritance.
Implement backup rotation, version naming. Handle incremental backups. Consider compression, deduplication. Implement proper cleanup. Handle backup verification.
Use appropriate protocols (FTP, SFTP). Handle timeouts, retries. Consider security, authentication. Implement progress tracking. Handle network errors properly.
Use context managers, atexit handlers. Implement proper error handling. Consider temporary files, locks. Handle program crashes. Implement cleanup verification.
Use mimetypes module, file signatures. Handle binary vs text files. Consider encoding detection. Implement proper validation. Handle unknown file types.
Handle chunk size, ordering. Implement progress tracking. Consider memory efficiency. Handle partial failures. Implement verification steps.
Common import methods: 'import module', 'from module import item', 'from module import *', 'import module as alias'. Each has different namespace effects. 'import *' not recommended due to namespace pollution. Consider relative vs absolute imports.
__init__.py marks directory as Python package, can initialize package attributes, execute package initialization code. Can be empty. Controls what's exported with __all__. Essential for Python 2, optional but recommended for Python 3.
Python searches modules in order: current directory, PYTHONPATH, standard library directories, site-packages. Accessible via sys.path. Can modify runtime using sys.path.append(). Consider virtual environment impact on search path.
Relative imports use dots: from . import module (current package), from .. import module (parent package). Only work within packages. Use explicit relative imports for clarity. Consider package restructuring implications.
Use venv module: python -m venv env_name. Activate using source env/bin/activate (Unix) or env\Scripts\activate (Windows). Manages project-specific dependencies. Consider requirements.txt for dependency tracking.
setup.py defines package metadata, dependencies, entry points for distribution. Used with setuptools for package building and installation. Includes version, requirements, package data. Consider modern alternatives like pyproject.toml.
Namespace packages allow splitting package across multiple directories. No __init__.py required. Uses implicit namespace package mechanism. Useful for plugin systems, large frameworks. Consider version compatibility issues.
pip is Python's package installer, conda is cross-platform package/environment manager. pip works with PyPI, conda has own repositories. conda handles non-Python dependencies. Consider project requirements for choosing.
Avoid using import statements inside functions, use dependency injection, restructure code to break cycles. Consider lazy imports, import inside functions. Signals possible design issues. Review module dependencies.
__all__ list controls what's imported with 'from module import *'. Explicitly defines public API. Good practice for large modules. Example: __all__ = ['func1', 'func2']. Consider documentation and maintenance.
Build distribution using setuptools/wheel, upload to PyPI using twine. Create source and wheel distributions. Consider versioning, documentation, licenses. Handle package data, dependencies correctly.
Entry points define console scripts, plugin points in setup.py/pyproject.toml. Enable command-line tools, plugin systems. Format: name=module:function. Consider cross-platform compatibility, documentation.
Use requirements.txt or pyproject.toml, specify version ranges appropriately. Consider dev vs production dependencies. Use pip-tools or poetry for dependency management. Handle transitive dependencies.
Checks if module is run directly or imported. Common for script entry points, testing. Code under this block only runs when module executed directly. Consider module reusability, testing implications.
Use MANIFEST.in, package_data in setup.py, include_package_data=True. Access using pkg_resources or importlib.resources. Consider data file locations, installation requirements. Handle path resolution.
Wheels are built package format, faster installation than source distributions. Contains pre-built files, metadata. Reduces installation time, ensures consistency. Consider pure Python vs platform-specific wheels.
Use entry points, namespace packages, or import hooks. Define plugin interface, discovery mechanism. Consider version compatibility, security. Handle plugin loading/unloading, configuration.
importlib provides import mechanism implementation, custom importers. Used for dynamic imports, import hooks. Access to import internals. Consider performance, security implications. Handle import errors.
Use virtual environments, specify version constraints carefully. Consider dependency resolution tools, container isolation. Handle conflicts through requirement specifications. Review dependency tree.
Modern configuration file for Python projects (PEP 518). Specifies build system requirements, project metadata. Replaces setup.py, setup.cfg. Used by poetry, flit. Consider migration from setuptools.
Use packages for logical grouping, maintain clear hierarchy. Consider separation of concerns, circular dependencies. Implement proper initialization. Handle configuration, plugin systems appropriately.
Use docstrings, maintain README files, generate API documentation. Follow PEP 257. Include usage examples, installation instructions. Consider documentation generation tools (Sphinx). Maintain changelog.
Import modules when needed, use importlib.import_module(). Consider performance implications, circular dependencies. Handle import errors appropriately. Implement proper cleanup.
Use __init__.py for package setup, expose public API. Handle optional dependencies, perform checks. Consider backwards compatibility. Implement proper error handling.
Use try/except for optional imports, implement fallbacks. Consider platform-specific modules. Handle missing dependencies gracefully. Document requirements clearly.
Import hooks customize module importing process. Implement custom importing behavior, transformations. Used for special loading requirements. Consider performance impact, maintenance complexity.
Use semantic versioning, maintain changelog. Consider backwards compatibility, deprecation policy. Handle version bumping, release process. Document version requirements clearly.
Verify package sources, use package hashes. Consider supply chain attacks, dependency scanning. Implement security updates policy. Review dependencies regularly.
Handle platform-specific code, use conditional imports. Consider environment differences. Test on multiple platforms. Handle path separators, line endings. Document platform requirements.
unittest is built-in, class-based, requires test classes inheriting from TestCase. pytest is more flexible, supports function-based tests, better fixtures, parametrization, and plugins. pytest has more powerful assertions and better error reporting. Consider project needs for framework choice.
Fixtures provide reusable test setup/teardown, defined using @pytest.fixture decorator. Support dependency injection, different scopes (function, class, module, session). Enable clean test organization, resource sharing. Example: database connections, test data setup.
Mocking replaces real objects with test doubles. Use unittest.mock or pytest-mock. MagicMock/Mock classes provide automatic attribute creation. Common uses: external services, databases, file operations. Consider patch decorator/context manager.
Use coverage.py or pytest-cov. Run tests with coverage collection, generate reports. Analyze uncovered lines, branches. Set minimum coverage requirements. Consider meaningful vs. superficial coverage. Focus on critical paths.
TDD cycle: write failing test, write code to pass, refactor. Tests drive design, document requirements. Write minimal code to pass tests. Benefits: better design, regression protection, documentation. Consider Red-Green-Refactor cycle.
Use @pytest.mark.parametrize decorator to run same test with different inputs. Supports multiple parameters, custom IDs. Reduces test code duplication. Example: @pytest.mark.parametrize('input,expected', [(1,2), (2,4)]). Consider data organization.
Markers (@pytest.mark) categorize tests, control execution. Built-in markers: skip, skipif, xfail. Custom markers for test organization, selection. Register markers in pytest.ini. Consider marker documentation, organization.
Use test databases, fixtures for setup/teardown. Consider transaction rollback, database isolation. Mock database when appropriate. Implement proper cleanup. Use tools like pytest-django for framework-specific support.
Test doubles replace real dependencies. Mocks verify interactions, stubs provide canned responses, fakes implement lightweight alternatives. Choose based on test needs. Consider interaction vs. state testing.
Use pytest-asyncio for async tests. Mark tests with @pytest.mark.asyncio. Handle coroutines properly. Consider event loop management. Test async contexts, timeouts. Handle async cleanup properly.
Group related tests, use clear naming conventions. Separate unit/integration tests. Follow AAA pattern (Arrange-Act-Assert). Maintain test independence. Consider test discoverability, maintenance.
Test component interactions, external services. Use appropriate fixtures, mocking selectively. Consider test environment setup. Handle cleanup properly. Balance coverage vs. execution time.
Use hypothesis library for property-based testing. Define properties, let framework generate test cases. Useful for finding edge cases. Consider strategy definition, test case generation. Handle test case reduction.
Use fixtures, factory libraries (factory_boy). Consider data isolation, cleanup. Implement proper test data generation. Handle complex data relationships. Consider data versioning, maintenance.
conftest.py provides shared fixtures across multiple test files. Defines test configuration, custom markers. Supports fixture overriding, plugin hooks. Consider scope organization, reusability.
Use pytest-benchmark for performance tests. Measure execution time, resource usage. Consider baseline comparisons, statistical analysis. Handle environment variations. Document performance requirements.
Monkey patching modifies objects/modules at runtime for testing. Use pytest.monkeypatch fixture. Handle cleanup properly. Consider implications on test isolation. Use sparingly, prefer dependency injection.
Use pytest.raises context manager or unittest.assertRaises. Test exception types, messages. Consider exception inheritance, multiple exceptions. Test cleanup handling. Verify exception context.
Plugins extend pytest functionality. Common plugins: pytest-cov, pytest-mock, pytest-django. Install via pip, configure in pytest.ini. Consider plugin interactions, maintenance. Document plugin requirements.
Use requests, pytest-httpx for HTTP testing. Mock external services appropriately. Consider response validation, error cases. Handle authentication, rate limiting. Test different HTTP methods.
Use pytest-bdd or behave for BDD. Write tests in Gherkin syntax. Map steps to test code. Consider stakeholder communication. Balance readability vs. maintenance. Document behavior specifications.
Use environment variables, configuration files. Implement test environment management. Consider CI/CD integration. Handle sensitive data properly. Document environment requirements.
Avoid test interdependence, slow tests, excessive mocking. Don't test implementation details. Avoid non-deterministic tests. Consider maintenance cost. Document test assumptions clearly.
Use pytest-xdist for parallel testing. Consider test isolation, shared resources. Handle race conditions. Implement proper cleanup. Balance parallelism vs. resource usage.
Use caplog fixture in pytest. Verify log messages, levels. Consider log handlers, formatting. Test logger configuration. Handle temporary logger modifications.
Test input validation, authentication, authorization. Use security testing tools (bandit). Consider vulnerability scanning. Test security configurations. Document security requirements.
Scopes: function (default), class, module, session. Choose based on resource costs, test isolation needs. Consider cleanup timing. Handle dependencies between fixtures. Document scope requirements.
Integrate tests in CI/CD pipeline. Automate test execution, reporting. Consider test selection, prioritization. Handle test failures appropriately. Document test requirements.
Use PyTest-Qt, PyAutoGUI for GUI testing. Handle event loops properly. Consider screenshot comparisons. Test user interactions. Handle window management. Document visual requirements.
Collections module provides specialized container types: defaultdict (automatic default values), Counter (counting hashable objects), deque (double-ended queue), namedtuple (tuple with named fields), OrderedDict (ordered dictionary pre-3.7). Each optimized for specific use cases and performance requirements.
datetime module handles dates and times. Use datetime.datetime with tzinfo for timezone awareness, pytz for reliable timezone handling. Methods: astimezone(), replace(tzinfo=), timezone conversions. Consider DST transitions, UTC conversions.
itertools provides functions for efficient iteration: combinations(), permutations(), product() for combinatorics; cycle(), repeat() for infinite iterators; chain(), islice() for iterator manipulation. Memory efficient for large datasets.
os module provides OS-independent interface for operating system operations. Functions: os.path for path manipulation, os.environ for environment variables, os.walk for directory traversal. Consider platform differences, security implications.
json module handles JSON encoding/decoding. Methods: dumps()/loads() for strings, dump()/load() for files. Supports custom serialization with default/object_hook. Handle encoding issues, pretty printing, security considerations.
re module provides regular expression operations: compile(), match(), search(), findall(). Supports patterns, groups, flags. Consider using raw strings (r'pattern'), pre-compilation for performance. Handle different regex patterns and flags.
concurrent.futures provides high-level interface for asynchronous execution: ThreadPoolExecutor for I/O-bound tasks, ProcessPoolExecutor for CPU-bound tasks. Supports map(), submit(), as_completed(). Handle thread/process management, exceptions.
logging provides flexible event logging: different levels (DEBUG to CRITICAL), handlers, formatters. Configure using basicConfig() or dictConfig(). Consider log rotation, formatting, handling in different environments.
pathlib provides object-oriented interface for file system paths. Path class methods: glob(), mkdir(), touch(), resolve(). More readable than os.path, handles platform differences. Consider migration from os.path.
functools provides tools for functional programming: partial() for partial application, lru_cache() for caching, reduce() for reduction operations. Includes wraps() for preserving function metadata. Consider performance implications.
argparse handles command-line argument parsing: argument types, help messages, subcommands. Supports required/optional arguments, default values, custom actions. Consider user interface design, error handling.
sqlite3 provides SQLite database interface: connection management, cursor operations, parameter substitution. Supports transactions, custom row factories. Consider connection lifecycle, error handling, SQL injection prevention.
asyncio provides infrastructure for async/await code: event loops, coroutines, tasks. Supports async I/O operations, concurrency. Handle task scheduling, synchronization primitives, error handling.
contextlib provides utilities for context managers: @contextmanager decorator, ExitStack for multiple contexts. Supports resource management, cleanup operations. Consider error handling, nested contexts.
csv handles CSV file operations: reading, writing, different dialects. Supports DictReader/DictWriter for named columns. Handle different formats, encoding issues, custom dialects. Consider large file handling.
threading provides high-level threading interface: Thread class, synchronization primitives (Lock, Event). Handle thread creation, synchronization, communication. Consider thread safety, deadlock prevention.
random provides pseudo-random number generation: randint(), choice(), shuffle(). Supports different distributions, seeding. Consider cryptographic needs (use secrets instead), reproducibility requirements.
pickle handles Python object serialization: dump()/load() for files, dumps()/loads() for bytes. Consider security implications, version compatibility. Handle custom object serialization, protocol versions.
sys provides system-specific parameters/functions: sys.path for module search, sys.argv for command arguments, sys.stdin/stdout/stderr for I/O. Handle interpreter interaction, system limitations.
shutil provides high-level file operations: copyfile(), rmtree(), make_archive(). Supports file copying, removal, archiving. Handle permissions, recursive operations, platform differences.
urllib handles URL operations: request handling, parsing, encoding. Modules: request for HTTP, parse for URL parsing, error for exceptions. Consider error handling, security, timeout configuration.
time provides time-related functions: time() for timestamps, sleep() for delays, strftime() for formatting. Handle different time representations, platform differences. Consider timezone implications.
enum provides enumeration support: Enum class, auto() for automatic values. Supports unique/non-unique values, custom behavior. Consider type safety, value comparison, serialization needs.
subprocess manages external processes: run(), Popen for process control. Handle command execution, I/O redirection, process communication. Consider security, platform differences, error handling.
statistics provides statistical functions: mean(), median(), mode(), stdev(). Supports different types of averages, variance calculations. Consider numerical stability, data types, population vs sample.
multiprocessing provides process-based parallelism: Process class, Pool for worker pools. Handle process creation, communication, synchronization. Consider GIL bypass, resource sharing, cleanup.
tempfile handles temporary files/directories: TemporaryFile, NamedTemporaryFile, TemporaryDirectory. Supports secure creation, automatic cleanup. Consider platform differences, cleanup guarantees.
operator provides function equivalents of operators: itemgetter(), attrgetter() for data access, add(), mul() for arithmetic. Useful in functional programming, sorting. Consider performance implications.
platform provides system information: system(), machine(), python_version(). Access hardware, OS details, Python environment. Consider cross-platform compatibility, information security.
SQLAlchemy Core provides SQL abstraction layer, direct table operations. ORM provides object-relational mapping, domain model abstraction. Core offers better performance, more control. ORM provides higher-level abstractions, easier object manipulation. Consider use case requirements for choosing.
Use connection pools (SQLAlchemy's Pool, psycopg2's pool). Configure pool size, overflow, timeout. Handle connection recycling, cleanup. Consider concurrent access patterns, resource limits. Implement proper error handling and monitoring.
Use migration tools (Alembic, Django migrations). Version control migrations, test before deployment. Handle data migrations separately. Consider rollback strategies, large table modifications. Document migration steps and dependencies.
Use transaction context managers, explicit commit/rollback. Handle atomic operations, savepoints. Implement proper error handling. Consider isolation levels, deadlock prevention. Manage transaction scope and nesting.
Use indexing, query analysis tools. Optimize JOIN operations, limit result sets. Consider query execution plans. Implement caching strategies. Monitor query performance. Use database-specific optimization features.
Use parameterized queries, ORM query builders. Never concatenate SQL strings. Validate input data. Consider escape sequences, prepared statements. Implement proper access controls and input sanitization.
Define relationships (one-to-many, many-to-many) using relationship() in SQLAlchemy or ForeignKey in Django. Handle lazy loading, eager loading. Consider cascade operations, backref relationships. Manage relationship lifecycle.
Use migration tools with version control. Implement forward/backward migrations. Track schema changes in source control. Consider database branching strategies. Handle schema conflicts and dependencies.
Handle specific database exceptions, implement retry logic. Log errors appropriately. Consider transaction rollback, connection recovery. Implement proper cleanup. Provide meaningful error messages.
Use sharding keys, implement routing logic. Handle cross-shard queries, transactions. Consider data distribution, rebalancing. Implement proper shard management. Handle shard failures and recovery.
Implement regular backups, verify backup integrity. Handle incremental backups, point-in-time recovery. Consider backup automation, retention policies. Test recovery procedures. Document backup/restore processes.
Implement exponential backoff, max retry attempts. Handle connection timeouts, dead connections. Consider circuit breaker pattern. Implement proper logging and monitoring. Handle cleanup properly.
Use caching layers (Redis, Memcached). Implement cache invalidation strategies. Handle cache consistency. Consider cache warming, eviction policies. Implement proper error handling for cache failures.
Configure master-slave replication, handle failover. Implement read/write splitting. Consider replication lag, consistency requirements. Handle replication errors and recovery. Monitor replication status.
Implement batched operations, progress tracking. Handle data validation, rollback capability. Consider performance impact, downtime requirements. Test migration procedures. Document migration steps.
Monitor query performance, connection usage. Log slow queries, errors. Implement performance metrics collection. Consider monitoring tools integration. Handle alert threshold configuration.
Use proper isolation levels, row-level locking. Handle deadlock detection, prevention. Consider optimistic vs pessimistic locking. Implement proper transaction boundaries. Handle concurrent access patterns.
Implement zero-downtime migrations. Handle backward compatibility. Use temporary tables for large changes. Consider rollback procedures. Test migration scripts. Document change procedures.
Use test databases, fixtures. Implement transaction rollback for tests. Handle database isolation. Consider performance testing. Test migration scripts. Implement proper cleanup procedures.
Implement proper authentication, authorization. Use least privilege principle. Handle sensitive data encryption. Consider audit logging. Implement access control policies. Handle security patches.
Use schema versioning, data versioning. Handle version conflicts. Implement upgrade/downgrade procedures. Consider backward compatibility. Document version dependencies.
Use connection pools, proper cleanup. Handle connection lifecycle. Implement health checks. Consider connection timeouts. Handle connection leaks. Monitor connection usage.
Handle schema flexibility, denormalization. Implement proper indexing strategies. Consider consistency models. Handle scaling operations. Implement proper error handling. Monitor performance.
Use configuration files, environment variables. Handle different environments. Implement secure credential management. Consider configuration versioning. Document configuration requirements.
Monitor query performance, implement indexing. Use query optimization tools. Consider database configuration tuning. Handle connection pooling optimization. Monitor resource usage.
Track data changes, user actions. Implement audit tables. Handle audit log maintenance. Consider compliance requirements. Implement proper retention policies. Handle audit log security.
Implement high availability solutions, automatic failover. Handle failover detection, recovery. Consider data consistency. Implement proper monitoring. Document failover procedures.
Monitor growth trends, resource usage. Plan scaling strategies. Consider performance requirements. Implement monitoring tools. Document capacity requirements. Handle growth projections.
Schedule maintenance windows, implement automation. Handle index maintenance, statistics updates. Consider performance impact. Implement proper monitoring. Document maintenance procedures.
Django is a full-featured framework with built-in admin, ORM, auth. Flask is a lightweight, flexible microframework. Django follows 'batteries included' philosophy, while Flask follows minimalist approach. Consider project size, requirements for choice. Django better for large applications, Flask for microservices.
Use Flask-RESTful or Flask API extensions. Implement resource classes, HTTP methods (GET, POST, etc.). Handle serialization, authentication. Consider API versioning, documentation (Swagger/OpenAPI). Implement proper error handling and status codes.
Middleware processes requests/responses globally. Implements security, sessions, authentication. Order matters in MIDDLEWARE setting. Can modify request/response objects. Consider performance impact, execution order. Implement custom middleware for cross-cutting concerns.
Use built-in auth in Django, Flask-Login for Flask. Implement JWT for APIs. Handle session management, password hashing. Consider OAuth integration, multi-factor authentication. Implement proper security measures and token management.
Use form classes (Django Forms, WTForms). Implement server-side validation, CSRF protection. Handle file uploads safely. Consider client-side validation, error messages. Implement proper sanitization and validation rules.
Use cache frameworks (Django's cache, Flask-Caching). Implement view caching, template fragment caching. Consider cache invalidation strategies. Handle cache backends (Redis, Memcached). Implement proper cache key management.
Use makemigrations and migrate commands. Handle schema changes, data migrations. Test migrations before deployment. Consider backwards compatibility. Implement proper rollback procedures. Document migration dependencies.
Use channels in Django, Flask-SocketIO in Flask. Handle real-time communication, event handling. Consider scaling considerations, connection management. Implement proper error handling and reconnection strategies.
Implement CSRF protection, XSS prevention, SQL injection protection. Use secure headers, HTTPS. Handle input validation, output encoding. Consider security headers, cookie security. Implement proper access controls.
Validate file types, size limits. Store files securely (filesystem/cloud storage). Handle virus scanning. Consider performance implications. Implement proper cleanup procedures. Handle concurrent uploads.
Use URL versioning, header versioning, or content negotiation. Handle backwards compatibility. Consider documentation updates. Implement proper version management. Handle deprecated versions gracefully.
Use rate limiting middleware/decorators. Implement token bucket algorithm. Handle different rate limits per user/API. Consider distributed rate limiting. Implement proper error responses and headers.
Use Celery, Redis Queue, or Django Q. Handle task queuing, scheduling. Implement proper error handling, retries. Consider monitoring, scaling. Handle task priorities and dependencies.
Use session middleware, handle session storage (database/cache). Consider session timeout, security. Implement proper cleanup. Handle session invalidation. Consider distributed session management.
Implement proper exception handling, custom error pages. Log errors appropriately. Handle different types of errors (404, 500). Consider user experience. Implement proper error reporting and monitoring.
Use environment variables, configuration files. Handle different environments (dev/prod). Implement secure credential management. Consider configuration versioning. Document configuration requirements.
Use Swagger/OpenAPI specification. Implement automatic documentation generation. Handle versioning, examples. Consider interactive documentation. Implement proper testing of documentation.
Use pagination classes (Django) or implement custom pagination. Handle cursor-based, offset pagination. Consider performance implications. Implement proper link headers. Handle edge cases.
Implement CORS middleware, handle preflight requests. Configure allowed origins, methods, headers. Consider security implications. Handle credentials properly. Implement proper error responses.
Use unittest, pytest for testing. Implement unit tests, integration tests. Handle test data, fixtures. Consider test coverage. Implement proper test organization and documentation.
Use containerization (Docker), implement CI/CD. Handle environment configuration. Consider scaling strategies. Implement proper monitoring. Document deployment procedures.
Use CDN, proper static file serving. Handle caching, compression. Consider performance optimization. Implement proper cache invalidation. Handle versioning of static files.
Use search engines (Elasticsearch), implement full-text search. Handle indexing, querying. Consider performance optimization. Implement proper result ranking. Handle search suggestions.
Use logging framework, handle different log levels. Implement proper log formatting. Consider log aggregation, analysis. Implement proper log rotation. Handle sensitive data in logs.
Use connection pooling, handle connection lifecycle. Implement proper error handling. Consider connection timeouts. Handle connection leaks. Monitor connection usage.
Use role-based access control (RBAC), implement permission checks. Handle group permissions. Consider hierarchical roles. Implement proper access control lists (ACL).
Use template inheritance, handle template caching. Implement proper escaping. Consider performance optimization. Handle template organization. Implement proper error handling.
Implement rate limiting middleware, handle quota management. Consider distributed rate limiting. Implement proper headers. Handle burst traffic. Document rate limit policies.
Handle webhook registration, event delivery. Implement retry logic. Consider security implications. Handle webhook validation. Implement proper error handling.
NumPy arrays are homogeneous (same data type), support vectorized operations, more memory efficient. Offer broadcasting, advanced indexing, mathematical operations. Better performance for numerical computations. Fixed size vs dynamic size of lists.
Use fillna(), dropna(), interpolate() methods. Handle different types of missing data (NaN, None). Consider imputation strategies (mean, median, forward/backward fill). Check missing patterns. Handle missing data in calculations.
Matplotlib for basic plots (line, scatter, bar). Seaborn for statistical visualizations (distributions, regressions). Handle customization, styling. Consider plot types for different data. Implement interactive features.
Use groupby(), agg(), pivot_table(). Apply different aggregation functions. Handle multi-level aggregation. Consider performance implications. Implement custom aggregation functions. Handle grouping with different criteria.
Broadcasting allows operations between arrays of different shapes. Rules: dimensions must be compatible (same, one, or missing). Automatically expands arrays to match shapes. Consider memory implications. Handle dimension compatibility.
Use get_dummies() for one-hot encoding, LabelEncoder for label encoding. Handle ordinal vs nominal categories. Consider feature hashing for high cardinality. Implement proper encoding strategy for ML models.
Use StandardScaler, MinMaxScaler, RobustScaler. Handle outliers in scaling. Consider feature distribution. Implement proper scaling strategy. Handle scaling in train/test split.
Use datetime indexing, resample(), rolling(). Handle time zones, frequencies. Implement time-based operations. Consider seasonal decomposition. Handle missing timestamps. Implement proper date parsing.
Use SMOTE for oversampling, undersampling techniques. Implement class weights. Consider ensemble methods. Handle evaluation metrics properly. Implement cross-validation strategy for imbalanced data.
Use SelectKBest, RFE, feature importance from models. Consider correlation analysis, mutual information. Implement proper validation strategy. Handle feature selection in pipeline.
Use IQR method, z-score method. Consider domain knowledge for outlier definition. Implement proper outlier treatment strategy. Handle outliers in different features. Consider impact on model performance.
Use chunking, memory efficient methods (read_csv chunks). Consider dtype optimization. Implement proper indexing strategy. Use efficient operations (vectorization). Handle memory constraints.
Use random sampling, stratified sampling, systematic sampling. Consider sample size, representation. Implement proper sampling strategy. Handle sampling in time series. Consider sampling bias.
Use merge(), concat(), join(). Handle different join types. Consider memory implications. Implement proper key matching strategy. Handle duplicates in merging.
Use PCA, t-SNE, UMAP. Consider feature importance, correlation. Implement proper validation strategy. Handle scaling before reduction. Consider interpretation of reduced dimensions.
Use tokenization, stemming/lemmatization. Handle stop words, special characters. Implement proper text cleaning strategy. Consider language specifics. Handle text encoding issues.
Use KFold, StratifiedKFold, TimeSeriesSplit. Handle validation strategy selection. Consider data characteristics. Implement proper scoring metrics. Handle cross-validation with parameter tuning.
Use Pipeline class, FeatureUnion. Handle preprocessing steps. Implement proper transformation order. Consider parameter tuning in pipeline. Handle custom transformers.
Use correlation analysis, VIF calculation. Consider feature selection strategies. Implement proper feature elimination. Handle correlation in model building. Consider impact on model interpretation.
Use scipy.stats for statistical tests. Handle different test types (t-test, chi-square). Consider assumptions, sample size. Implement proper test selection. Handle multiple testing.
Use DVC (Data Version Control), implement proper tracking. Handle dataset versions. Consider storage implications. Implement proper documentation. Handle version dependencies.
Use vectorization, proper array operations. Consider memory layout. Implement efficient algorithms. Handle large arrays properly. Consider parallel processing options.
Create interaction features, polynomial features. Handle domain-specific transformations. Implement proper feature validation. Consider feature importance. Handle feature scaling.
Implement data validation rules, quality metrics. Handle data integrity checks. Consider domain constraints. Implement proper error handling. Document validation procedures.
Use polynomial features, spline transformations. Consider feature transformations. Implement proper validation strategy. Handle overfitting risks. Consider model selection.
Use multiprocessing, Dask for parallel operations. Handle memory management. Consider scalability issues. Implement proper error handling. Consider overhead vs benefits.
Implement different augmentation strategies. Handle domain-specific augmentation. Consider data balance. Implement proper validation strategy. Handle augmentation in pipeline.
Use appropriate streaming libraries, implement proper buffering. Handle real-time updates. Consider memory management. Implement proper error handling. Handle data consistency.
Use SHAP values, feature importance analysis. Implement model-specific interpretation techniques. Consider global vs local interpretation. Handle complex model interpretation.
GIL is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecode simultaneously. Limits CPU-bound threads to run sequentially. Affects only CPython implementation. Consider multiprocessing for CPU-bound tasks.
Threading shares memory space, affected by GIL, good for I/O-bound tasks. Multiprocessing uses separate memory spaces, bypasses GIL, good for CPU-bound tasks. Consider overhead, data sharing needs, and system resources when choosing.
Use async/await syntax, create event loop using asyncio.get_event_loop(). Handle coroutine execution, task scheduling. Consider error handling, cancellation. Implement proper cleanup. Handle event loop lifecycle.
Lock, RLock (reentrant lock), Semaphore, Event, Condition, Barrier. Each serves different synchronization needs. Handle proper acquisition/release. Consider deadlock prevention. Implement proper error handling.
Use multiprocessing.Queue, Pipe, shared memory (Value, Array). Handle synchronization properly. Consider serialization overhead. Implement proper locking mechanisms. Handle process termination cleanup.
gather() returns results in order, raises first exception. wait() provides more control over completion, timeout, returns done/pending sets. Consider exception handling, cancellation behavior. Handle task dependencies.
Use concurrent.futures.ThreadPoolExecutor. Handle task submission, result collection. Implement proper shutdown. Consider pool size optimization. Handle exceptions in worker threads. Manage resource usage.
Race conditions occur when multiple threads access shared data simultaneously. Prevent using locks, atomic operations. Implement proper synchronization. Consider thread safety in design. Use thread-safe data structures.
Prevent using lock ordering, timeouts. Detect using deadlock detection algorithms. Implement recovery mechanisms. Consider lock hierarchy. Handle nested locks carefully. Implement proper error recovery.
async with handles asynchronous context managers. async for iterates over async iterables. Properly handle resource cleanup, iteration. Consider exception handling. Implement proper asynchronous patterns.
Use multiprocessing.Pool or concurrent.futures.ProcessPoolExecutor. Handle task distribution, result collection. Consider process communication overhead. Implement proper cleanup. Handle process failures.
Implement proper exception handling in worker threads/processes. Handle task failures gracefully. Consider exception propagation. Implement logging and monitoring. Handle cleanup in error cases.
Use Queue from queue module, implement proper locking. Consider atomic operations. Use thread-safe collections. Implement proper synchronization mechanisms. Handle concurrent access patterns.
CPU-bound tasks primarily use processor (calculations). I/O-bound tasks wait for external operations (network, disk). Choose appropriate concurrency model (multiprocessing vs threading). Consider resource utilization.
Implement __aenter__ and __aexit__ methods. Handle async resource acquisition/release. Consider exception handling. Implement proper cleanup. Handle async initialization.
Use Queue for thread-safe communication. Implement proper synchronization. Handle termination conditions. Consider buffer size. Implement proper error handling. Handle backpressure.
Use Task.cancel(), handle CancelledError. Implement cleanup handlers. Consider task dependencies. Handle cancellation propagation. Implement proper state cleanup.
Consider GIL implications, use thread-safe operations. Implement proper synchronization. Use atomic operations when possible. Consider thread-local storage. Handle shared resource access.
Use multiprocessing pools, handle data partitioning. Consider communication overhead. Implement proper merging strategy. Handle process synchronization. Consider memory usage.
Use timeout parameters, implement timeout handling. Consider cancellation. Handle cleanup after timeout. Implement proper error reporting. Consider partial results handling.
Use logging, debugger support for threads/processes. Implement proper monitoring. Consider race condition detection. Handle debugging synchronization issues. Implement proper error tracking.
Thread-local storage provides thread-specific data storage. Prevents data sharing between threads. Implement using threading.local(). Consider cleanup requirements. Handle initialization properly.
Use async def with yield, implement __aiter__ and __anext__. Handle async iteration properly. Consider resource management. Implement proper cleanup. Handle cancellation.
Handle pool lifecycle, implement proper shutdown. Consider resource cleanup. Handle worker process errors. Implement proper task distribution. Consider pool size optimization.
Use appropriate IPC mechanisms (Queue, Pipe). Handle synchronization properly. Consider serialization overhead. Implement proper error handling. Handle process termination.
Use event loops, implement event handlers. Consider event propagation. Handle event ordering. Implement proper error handling. Consider event queue management.
Balance thread/process count, optimize resource usage. Consider overhead costs. Implement proper task granularity. Handle load balancing. Consider system resources.
Handle resource limitations, implement proper load balancing. Consider scalability bottlenecks. Handle distributed processing. Implement proper monitoring. Consider performance metrics.
Handle termination signals, implement proper cleanup. Consider ongoing operations. Handle resource release. Implement proper state saving. Consider recovery procedures.
PEP 8 defines Python's style guide: indentation (4 spaces), line length (79 chars), naming conventions (snake_case for functions/variables, PascalCase for classes), import organization. Ensures code readability and consistency across Python community. Key for maintainability and collaboration.
Single Responsibility (one purpose per class), Open/Closed (open for extension, closed for modification), Liskov Substitution (subtypes must be substitutable), Interface Segregation (specific interfaces), Dependency Inversion (depend on abstractions). Implement using proper class design, inheritance, and abstractions.
Follow PEP 257: describe purpose, parameters, return values, exceptions. Use consistent format (Google, NumPy, reStructuredText). Include examples when appropriate. Consider doctest integration. Keep updated with code changes. Essential for code understanding and maintenance.
Catch specific exceptions, not bare except. Use context managers for resource cleanup. Implement proper error hierarchy. Log exceptions appropriately. Consider error recovery strategies. Don't suppress exceptions without good reason. Document exceptional cases.
Extract common code into functions/classes. Use inheritance and composition appropriately. Implement utility modules. Consider code reusability. Balance DRY with readability. Use proper abstraction levels. Consider maintenance implications.
Pass dependencies as parameters, use dependency containers, implement factory patterns. Consider interface-based design. Handle dependency lifecycle. Use proper abstraction. Consider testing implications. Implement proper configuration management.
Use proper package hierarchy, separate concerns, implement clear imports. Follow standard project layout (setup.py, requirements.txt, docs/, tests/). Consider module organization. Implement proper configuration management. Document project structure.
Functions should be small, do one thing, have clear names. Use proper parameter design, avoid side effects. Consider return value clarity. Implement proper validation. Document function behavior. Consider error cases.
Use appropriate log levels, implement structured logging. Consider log format, destination. Handle sensitive data appropriately. Implement proper error reporting. Consider log rotation, retention. Document logging conventions.
Check style consistency, proper error handling, test coverage. Review documentation, performance implications. Consider security aspects. Look for code smells. Provide constructive feedback. Document review process.
Use configuration files, environment variables. Implement proper validation. Handle different environments. Consider security implications. Document configuration options. Implement proper defaults.
Use abstract base classes (ABC), protocol classes. Implement proper method signatures. Consider duck typing. Handle interface evolution. Document interface contracts. Consider backward compatibility.
Follow AAA pattern (Arrange-Act-Assert), use clear test names. Implement proper test isolation. Consider test readability. Handle test data properly. Document test cases. Maintain test suite.
Comment why, not what. Keep comments updated with code. Use proper inline documentation. Consider self-documenting code. Document complex algorithms. Avoid redundant comments. Keep comments concise.
Make messages clear, actionable. Include relevant context. Consider user perspective. Handle internationalization. Document error conditions. Implement proper error categorization. Consider error recovery.
Follow single responsibility, maintain clear interfaces. Consider versioning strategy. Handle dependencies properly. Document package usage. Implement proper testing. Consider distribution aspects.
Use deprecation warnings, provide migration path. Document deprecation timeline. Maintain backward compatibility. Consider impact on users. Implement proper versioning. Handle removal process.
Use factory methods for object creation, implement proper initialization. Consider inheritance hierarchy. Handle configuration. Document factory behavior. Consider error cases.
Use descriptive names, follow conventions. Consider scope visibility. Use proper prefixes/suffixes. Maintain consistency. Document naming patterns. Consider international aspects.
Profile before optimizing, focus on bottlenecks. Consider algorithmic efficiency. Use appropriate data structures. Handle memory usage. Document optimization decisions. Consider maintenance implications.
Follow single responsibility, use proper inheritance. Implement encapsulation. Consider composition over inheritance. Document class behavior. Handle initialization properly. Consider class evolution.
Minimize global state, use dependency injection. Consider thread safety. Implement proper access patterns. Document global dependencies. Consider testing implications. Handle state initialization.
Group related functionality, maintain clear interfaces. Consider circular dependencies. Document module purpose. Implement proper importing. Handle module initialization. Consider module evolution.
Keep interfaces simple, document clearly. Handle versioning properly. Consider backward compatibility. Implement proper validation. Document API changes. Consider security aspects.
Break down complex functions, use proper abstraction. Implement clear control flow. Consider cognitive complexity. Document complex logic. Use appropriate design patterns. Consider maintenance aspects.
Use proper wrapper functions, maintain function metadata. Handle arguments properly. Consider chaining decorators. Document decorator behavior. Consider performance implications.
Implement proper cleanup, handle partial failures. Consider system state. Document recovery procedures. Implement proper logging. Handle cascading failures. Consider user experience.
Use clear documentation style, keep documentation updated. Consider audience needs. Document assumptions. Implement proper examples. Consider automation tools. Maintain documentation quality.
Use meaningful commit messages, proper branching strategy. Consider merge management. Document version changes. Implement proper tagging. Handle release process. Consider collaboration aspects.
Metaclasses are classes for classes, allowing customization of class creation. Used for API enforcement, attribute validation, class registration, abstract base classes. Created using type or custom metaclass. Example: class MyMetaclass(type): def __new__(cls, name, bases, attrs). Consider complexity trade-offs.
Descriptors define __get__, __set__, __delete__ methods to customize attribute access. Used in properties, methods, class attributes. Enable managed attributes, validation, computed values. Example: property implementation using descriptors. Consider performance implications.
Uses reference counting for basic GC, generational GC for cycles. Reference count tracks object references, frees when zero. Cycle detector identifies and collects unreachable reference cycles. Consider memory management, performance implications.
ABCs define interface through @abstractmethod, can include implementation. Created using abc.ABC. Enforce interface implementation, provide default methods. Unlike Java interfaces, can have implementation. Consider multiple inheritance implications.
Implement __enter__ and __exit__ methods, or use contextlib.contextmanager decorator. Handle resource acquisition/release, exception handling. Consider cleanup guarantees. Example: file handling, database connections.
send() method for two-way communication, throw() for exception injection, close() for generator cleanup. Generator delegation using yield from. Consider coroutine behavior, exception handling. Implement proper cleanup.
Use __getattr__, __setattr__, __getattribute__, __delattr__. Handle attribute lookup, modification, deletion. Consider infinite recursion, performance. Implement proper attribute management. Handle special cases.
MRO determines method lookup order in inheritance. Uses C3 linearization algorithm. Accessible via __mro__ attribute. Handles multiple inheritance, method overriding. Consider inheritance complexity, diamond problem.
Implement __iter__ and __next__ methods for iteration. Consider StopIteration handling. Implement proper cleanup. Handle resource management. Consider memory efficiency. Example: custom sequence types.
Implement rich comparison methods (__eq__, __lt__, etc.). Use @functools.total_ordering for complete ordering. Consider type checking, reflexivity, transitivity. Handle edge cases. Implement proper comparison logic.
Bound methods are instance-specific, include self parameter automatically. Unbound methods are class-level, require explicit instance. Consider method types, descriptor protocol. Handle method binding properly.
Implement __getstate__ and __setstate__ for custom serialization. Handle complex objects, circular references. Consider security implications. Implement proper state management. Handle version compatibility.
Type hints provide static typing information. Used by type checkers (mypy), IDE support. Not enforced at runtime by default. Enhance code readability, maintainability. Consider performance impact, compatibility.
Implement container protocol methods (__len__, __getitem__, etc.). Consider sequence/mapping behavior. Handle iteration, containment checks. Implement proper indexing. Consider memory management.
Class decorators modify/enhance class definitions. Applied using @decorator syntax above class. Can modify class attributes, methods, add functionality. Consider inheritance implications. Handle class modification properly.
Inherit from appropriate exception base class. Implement proper initialization, custom attributes. Consider exception hierarchy. Handle error messages, context. Implement proper cleanup. Document exception conditions.
Protocols define interface behavior without inheritance. Use typing.Protocol for static typing. Support structural subtyping. Consider duck typing implications. Implement protocol compatibility.
Use __slots__, proper data structures, generators. Consider memory profiling, garbage collection. Handle large datasets efficiently. Implement proper cleanup. Monitor memory usage. Consider caching strategies.
Assignment expressions (:=) assign and return value in single expression. Used in while loops, if statements, list comprehensions. Enhances code readability, reduces redundancy. Consider proper usage contexts.
Implement numeric protocol methods (__add__, __mul__, etc.). Handle reverse operations (__radd__, etc.). Consider type coercion, precision. Implement proper arithmetic behavior. Handle special cases.
Generics provide type hints for collections/classes. Use typing.Generic, TypeVar. Support parametric polymorphism. Consider type checking implications. Implement proper type constraints. Handle type variables.
Use @property with caching, implement custom descriptors. Consider computation cost, memory usage. Handle invalidation properly. Implement thread safety. Consider cache management.
@dataclass decorator provides automatic __init__, __repr__, etc. Support inheritance, frozen instances, default values. Consider field options, ordering. Implement custom initialization. Handle field dependencies.
Use decorator pattern, functools.wraps for metadata preservation. Handle argument passing, return values. Consider function attributes. Implement proper wrapping. Handle special methods.
__aiter__, __anext__, __aenter__, __aexit__ for async operations. Support async context managers, iterators. Handle coroutine behavior. Implement proper cleanup. Consider async patterns.
Create descriptor classes with __get__, __set__, __delete__. Handle attribute access, modification. Consider validation, computation. Implement proper state management. Handle inheritance.
Understand object internals, reference counting, memory allocation. Handle weak references, object lifecycle. Consider memory sharing, copy semantics. Implement proper memory management. Handle circular references.
Solve Python coding challenges designed to mirror interview questions.
Explore MoreEnsure your Python expertise is highlighted with ATS-friendly resumes.
Explore MoreMaster Python syntax, data types, and core libraries.
Regularly solve Python problems on platforms like Stark.ai.
Discuss your hands-on experience with Python.
Highlight how Python helped you solve specific problems.
Join thousands of successful candidates who prepared with Stark.ai. Practice Python questions, mock interviews, and more to secure your dream job.
Start Preparing now