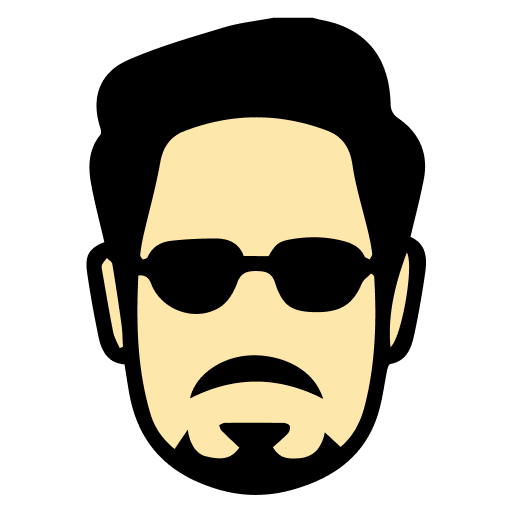
Flutter is Google's UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase. Stark.ai offers a curated collection of Flutter interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Flutter is a UI toolkit that offers: 1) Single codebase for multiple platforms (iOS, Android, Web, Desktop), 2)...
Key differences include: 1) StatelessWidget is immutable and cannot change its state after creation, 2)...
Widget Tree in Flutter: 1) Hierarchical structure of widgets that describes UI, 2) Parent widgets wrap and affect...
Hot Reload: 1) Updates code changes without app restart, 2) Preserves app state during development, 3) Injects...
Key layout widgets: 1) Container for styling and positioning, 2) Row and Column for linear layouts, 3) Stack for...
BuildContext provides: 1) Location of widget in widget tree, 2) Access to inherited widgets, 3) Theme and MediaQuery...
Keys are used for: 1) Maintaining widget state across moves in widget tree, 2) Uniquely identifying widgets, 3)...
Widget lifecycle includes: 1) Constructor creation, 2) initState for StatefulWidget initialization, 3)...
InheritedWidget provides: 1) Efficient data sharing down widget tree, 2) Automatic rebuild of dependent widgets, 3)...
Responsive design techniques: 1) Using MediaQuery for screen dimensions, 2) LayoutBuilder for constraints-based...
Key differences: 1) Container allows decoration and padding, 2) SizedBox is more lightweight and efficient, 3)...
Gesture handling includes: 1) GestureDetector widget for touch events, 2) InkWell for Material Design ripple effect,...
Button types include: 1) ElevatedButton for raised buttons, 2) TextButton for flat buttons, 3) OutlinedButton for...
Form validation involves: 1) Form widget for form state management, 2) TextFormField for input validation, 3)...
Custom widgets are used for: 1) Reusable UI components, 2) Complex widget combinations, 3) Encapsulating business...
Flutter is a UI toolkit that offers: 1) Single codebase for multiple platforms (iOS, Android, Web, Desktop), 2)...
Key differences include: 1) StatelessWidget is immutable and cannot change its state after creation, 2)...
Widget Tree in Flutter: 1) Hierarchical structure of widgets that describes UI, 2) Parent widgets wrap and affect...
Hot Reload: 1) Updates code changes without app restart, 2) Preserves app state during development, 3) Injects...
Key layout widgets: 1) Container for styling and positioning, 2) Row and Column for linear layouts, 3) Stack for...
BuildContext provides: 1) Location of widget in widget tree, 2) Access to inherited widgets, 3) Theme and MediaQuery...
Keys are used for: 1) Maintaining widget state across moves in widget tree, 2) Uniquely identifying widgets, 3)...
Widget lifecycle includes: 1) Constructor creation, 2) initState for StatefulWidget initialization, 3)...
InheritedWidget provides: 1) Efficient data sharing down widget tree, 2) Automatic rebuild of dependent widgets, 3)...
Responsive design techniques: 1) Using MediaQuery for screen dimensions, 2) LayoutBuilder for constraints-based...
Key differences: 1) Container allows decoration and padding, 2) SizedBox is more lightweight and efficient, 3)...
Gesture handling includes: 1) GestureDetector widget for touch events, 2) InkWell for Material Design ripple effect,...
Button types include: 1) ElevatedButton for raised buttons, 2) TextButton for flat buttons, 3) OutlinedButton for...
Form validation involves: 1) Form widget for form state management, 2) TextFormField for input validation, 3)...
Custom widgets are used for: 1) Reusable UI components, 2) Complex widget combinations, 3) Encapsulating business...
Key differences include: 1) const is compile-time constant, final is runtime constant, 2) const requires all values...
Differences include: 1) SafeArea adjusts for system intrusions, 2) Padding adds space around content, 3) SafeArea...
Flex and Expanded features: 1) Flex determines axis of layout, 2) Expanded fills available space, 3) flex factor for...
Slivers provide: 1) Custom scrolling effects, 2) Efficient scrollable layouts, 3) AppBar animations, 4) Lazy loading...
Asset management includes: 1) pubspec.yaml configuration, 2) Asset bundling process, 3) Resolution-aware images, 4)...
GlobalKey purposes: 1) Accessing widget state across tree, 2) Unique widget identification, 3) Form validation...
Keyboard handling includes: 1) FocusNode management, 2) TextInput configuration, 3) Keyboard type selection, 4)...
ScrollView types include: 1) ListView for linear scrolling, 2) GridView for grid layouts, 3) SingleChildScrollView...
Theming implementation: 1) ThemeData configuration, 2) Theme inheritance, 3) Custom theme creation, 4) Dark mode...
Stack and Positioned: 1) Stack allows overlapping children, 2) Positioned controls child position, 3) Stack...
Error handling includes: 1) ErrorWidget customization, 2) BuildContext error handling, 3) Error callback...
Widget composition practices: 1) Single responsibility principle, 2) Proper widget extraction, 3) State management...
Localization implementation: 1) MaterialApp localization delegates, 2) Locale resolution, 3) Asset localization, 4)...
Visibility approaches: 1) Visibility widget usage, 2) Opacity widget control, 3) Offstage widget, 4) Conditional...
Disposal and cleanup: 1) dispose() method implementation, 2) Controller cleanup, 3) Stream subscription...
Popular state management solutions include: 1) Provider for dependency injection and state management, 2) Bloc...
BLoC pattern features: 1) Separation of UI and business logic, 2) Stream-based state management, 3) Event-driven...
Provider offers: 1) Dependency injection capabilities, 2) Widget tree state management, 3) Easy data sharing between...
Key differences: 1) Ephemeral state is local to a widget, 2) App state is shared across multiple widgets, 3)...
GetX provides: 1) Simple reactive state management, 2) Dependencies injection, 3) Route management, 4) Memory...
Riverpod advantages: 1) Compile-time safety, 2) Better error handling, 3) No context requirement, 4) Multiple...
Redux implementation includes: 1) Single store for state, 2) Action creators for state changes, 3) Reducers for...
MobX features: 1) Observable state tracking, 2) Automatic updates through reactions, 3) Computed values, 4) Actions...
Form state management: 1) Form widget usage, 2) FormField implementations, 3) GlobalKey<FormState>, 4) Validation...
Global state patterns: 1) Singleton pattern, 2) Service locator pattern, 3) Provider at app root, 4) Redux store, 5)...
State persistence methods: 1) SharedPreferences for simple data, 2) SQLite for structured data, 3) Hive for NoSQL...
ChangeNotifier provides: 1) Simple state change notifications, 2) Listener management, 3) Mixin capabilities, 4)...
Testing approaches: 1) Unit testing state logic, 2) Widget testing with state, 3) Integration testing, 4) Mock state...
State organization practices: 1) Separation of concerns, 2) Single responsibility principle, 3) Immutable state...
Complex state handling: 1) State machine implementation, 2) Transition validation, 3) Side effect management, 4)...
Popular state management solutions include: 1) Provider for dependency injection and state management, 2) Bloc...
BLoC pattern features: 1) Separation of UI and business logic, 2) Stream-based state management, 3) Event-driven...
Provider offers: 1) Dependency injection capabilities, 2) Widget tree state management, 3) Easy data sharing between...
Key differences: 1) Ephemeral state is local to a widget, 2) App state is shared across multiple widgets, 3)...
GetX provides: 1) Simple reactive state management, 2) Dependencies injection, 3) Route management, 4) Memory...
Riverpod advantages: 1) Compile-time safety, 2) Better error handling, 3) No context requirement, 4) Multiple...
Redux implementation includes: 1) Single store for state, 2) Action creators for state changes, 3) Reducers for...
MobX features: 1) Observable state tracking, 2) Automatic updates through reactions, 3) Computed values, 4) Actions...
Form state management: 1) Form widget usage, 2) FormField implementations, 3) GlobalKey<FormState>, 4) Validation...
Global state patterns: 1) Singleton pattern, 2) Service locator pattern, 3) Provider at app root, 4) Redux store, 5)...
State persistence methods: 1) SharedPreferences for simple data, 2) SQLite for structured data, 3) Hive for NoSQL...
ChangeNotifier provides: 1) Simple state change notifications, 2) Listener management, 3) Mixin capabilities, 4)...
Testing approaches: 1) Unit testing state logic, 2) Widget testing with state, 3) Integration testing, 4) Mock state...
State organization practices: 1) Separation of concerns, 2) Single responsibility principle, 3) Immutable state...
Complex state handling: 1) State machine implementation, 2) Transition validation, 3) Side effect management, 4)...
State restoration includes: 1) RestorationMixin implementation, 2) RestorationProperties usage, 3) State...
Combination patterns: 1) Layer separation by responsibility, 2) Bridge pattern implementation, 3) State...
Real-time state management: 1) Stream-based updates, 2) WebSocket integration, 3) State synchronization, 4) Conflict...
Optimization strategies: 1) Selective rebuilds, 2) State granularity control, 3) Memoization techniques, 4) Batch...
Dependency injection patterns: 1) Service locator pattern, 2) Provider injection, 3) GetIt implementation, 4)...
Error state handling: 1) Error state models, 2) Error propagation, 3) Recovery mechanisms, 4) User feedback, 5)...
Undo/redo implementation: 1) Command pattern usage, 2) State history stack, 3) Memento pattern, 4) Action...
Nested state patterns: 1) Composite state objects, 2) State tree management, 3) Scoped updates, 4) Parent-child...
Offline state management: 1) Local persistence, 2) Sync strategies, 3) Conflict resolution, 4) Queue management, 5)...
Debugging practices: 1) State logging implementation, 2) DevTools integration, 3) State visualization, 4)...
State-based navigation: 1) Route state management, 2) Deep linking support, 3) Navigation state persistence, 4)...
Multi-window state patterns: 1) State synchronization, 2) Shared state storage, 3) Window communication, 4) State...
State-based theming: 1) Theme state management, 2) Dynamic theme switching, 3) Theme persistence, 4) Custom theme...
Widget testing approaches: 1) State mocking, 2) Provider testing, 3) BLoC test implementation, 4) State...
Flutter navigation approaches include: 1) Navigator 1.0 with push/pop methods, 2) Navigator 2.0 with declarative...
Key differences include: 1) Navigator 1.0 uses imperative API vs 2.0's declarative approach, 2) 2.0 provides better...
Deep linking implementation: 1) Configure AndroidManifest.xml and Info.plist, 2) Set up route parsing, 3) Handle app...
Named Routes features: 1) Define routes in MaterialApp, 2) Navigate using pushNamed, 3) Pass arguments to routes, 4)...
Nested navigation involves: 1) Multiple Navigator widgets, 2) Scope management with keys, 3) Parent-child navigation...
Route transition practices: 1) Custom PageRouteBuilder implementation, 2) Animation controller management, 3)...
Route guards implementation: 1) Navigation middleware setup, 2) Authentication checks, 3) Permission validation, 4)...
Navigation state patterns: 1) Navigation service implementation, 2) State-based routing, 3) Navigation history...
Responsive navigation: 1) Adaptive navigation patterns, 2) Screen size-based routing, 3) Navigation rail...
Navigation testing strategies: 1) Widget testing with Navigator, 2) Mock navigation service, 3) Route generation...
Tab navigation implementation: 1) BottomNavigationBar setup, 2) TabController management, 3) State preservation...
Modal handling approaches: 1) ShowDialog implementation, 2) BottomSheet presentation, 3) Modal route management, 4)...
Web routing implementation: 1) URL strategy configuration, 2) Path parameter handling, 3) Query parameter...
Back button patterns: 1) WillPopScope implementation, 2) Custom back behavior, 3) Navigation stack management, 4)...
Error handling implementation: 1) Route error catching, 2) Unknown route handling, 3) Navigation failure recovery,...
Flutter navigation approaches include: 1) Navigator 1.0 with push/pop methods, 2) Navigator 2.0 with declarative...
Key differences include: 1) Navigator 1.0 uses imperative API vs 2.0's declarative approach, 2) 2.0 provides better...
Deep linking implementation: 1) Configure AndroidManifest.xml and Info.plist, 2) Set up route parsing, 3) Handle app...
Named Routes features: 1) Define routes in MaterialApp, 2) Navigate using pushNamed, 3) Pass arguments to routes, 4)...
Nested navigation involves: 1) Multiple Navigator widgets, 2) Scope management with keys, 3) Parent-child navigation...
Route transition practices: 1) Custom PageRouteBuilder implementation, 2) Animation controller management, 3)...
Route guards implementation: 1) Navigation middleware setup, 2) Authentication checks, 3) Permission validation, 4)...
Navigation state patterns: 1) Navigation service implementation, 2) State-based routing, 3) Navigation history...
Responsive navigation: 1) Adaptive navigation patterns, 2) Screen size-based routing, 3) Navigation rail...
Navigation testing strategies: 1) Widget testing with Navigator, 2) Mock navigation service, 3) Route generation...
Tab navigation implementation: 1) BottomNavigationBar setup, 2) TabController management, 3) State preservation...
Modal handling approaches: 1) ShowDialog implementation, 2) BottomSheet presentation, 3) Modal route management, 4)...
Web routing implementation: 1) URL strategy configuration, 2) Path parameter handling, 3) Query parameter...
Back button patterns: 1) WillPopScope implementation, 2) Custom back behavior, 3) Navigation stack management, 4)...
Error handling implementation: 1) Route error catching, 2) Unknown route handling, 3) Navigation failure recovery,...
State persistence includes: 1) Route state serialization, 2) Navigation history storage, 3) State restoration...
Complex navigation strategies: 1) Flow coordinator pattern, 2) Navigation graph implementation, 3) State machine...
Auth routing implementation: 1) Route guards for authentication, 2) Login flow management, 3) Token-based...
Large-scale navigation patterns: 1) Modular routing setup, 2) Route coordination across modules, 3) Navigation...
Custom transitions include: 1) PageRouteBuilder customization, 2) Animation controller management, 3) Custom...
Offline navigation approaches: 1) Route caching mechanisms, 2) Offline state management, 3) Navigation queue...
Navigation analytics implementation: 1) Route tracking setup, 2) Navigation event logging, 3) Performance...
Multi-window patterns: 1) Window-specific navigation, 2) Navigation state sync, 3) Window communication, 4) Shared...
Navigation accessibility: 1) Semantic navigation routes, 2) Screen reader support, 3) Navigation announcements, 4)...
Performance optimization strategies: 1) Route caching implementation, 2) Lazy route loading, 3) Navigation...
Platform-specific navigation: 1) Adaptive navigation patterns, 2) Platform-specific gestures, 3) Custom back...
State restoration patterns: 1) Route state serialization, 2) Navigation stack rebuilding, 3) Deep link restoration,...
Form factor navigation: 1) Responsive navigation patterns, 2) Adaptive layouts, 3) Screen size-based routing, 4)...
Navigation testing practices: 1) Unit testing routes, 2) Widget testing navigation, 3) Integration testing flows, 4)...
Key performance factors include: 1) Widget rebuild optimization, 2) Memory management and leaks, 3) Image loading...
Widget rebuild optimization includes: 1) Using const constructors, 2) Implementing shouldRebuild method, 3) Proper...
Memory management practices: 1) Disposing controllers and streams, 2) Image caching strategies, 3) Lazy loading...
Image optimization techniques: 1) Proper image caching, 2) Resolution-aware image loading, 3) Lazy loading for...
Performance profiling tools: 1) Flutter DevTools, 2) Performance overlay, 3) Timeline view, 4) Memory profiler, 5)...
Animation optimization includes: 1) Using explicit animations, 2) Implementing repaint boundaries, 3) Hardware...
Startup optimization strategies: 1) Deferred components loading, 2) Asset preloading optimization, 3) Initialization...
List optimization techniques: 1) ListView.builder usage, 2) Caching list items, 3) Implementing pagination, 4) Using...
App size reduction: 1) Tree shaking implementation, 2) Asset optimization, 3) Code minification, 4) Split targets...
Efficient state management: 1) Proper state scoping, 2) Minimal rebuild strategy, 3) State isolation patterns, 4)...
Network optimization practices: 1) Request caching implementation, 2) Efficient data serialization, 3) Connection...
Background processing strategies: 1) Isolate implementation, 2) Compute function usage, 3) Background fetch...
Database optimization patterns: 1) Batch operations usage, 2) Index optimization, 3) Query optimization, 4)...
Efficient error handling: 1) Error boundary implementation, 2) Exception catching optimization, 3) Error logging...
Platform channel optimization: 1) Batch method calls, 2) Data serialization efficiency, 3) Channel type selection,...
Key performance factors include: 1) Widget rebuild optimization, 2) Memory management and leaks, 3) Image loading...
Widget rebuild optimization includes: 1) Using const constructors, 2) Implementing shouldRebuild method, 3) Proper...
Memory management practices: 1) Disposing controllers and streams, 2) Image caching strategies, 3) Lazy loading...
Image optimization techniques: 1) Proper image caching, 2) Resolution-aware image loading, 3) Lazy loading for...
Performance profiling tools: 1) Flutter DevTools, 2) Performance overlay, 3) Timeline view, 4) Memory profiler, 5)...
Animation optimization includes: 1) Using explicit animations, 2) Implementing repaint boundaries, 3) Hardware...
Startup optimization strategies: 1) Deferred components loading, 2) Asset preloading optimization, 3) Initialization...
List optimization techniques: 1) ListView.builder usage, 2) Caching list items, 3) Implementing pagination, 4) Using...
App size reduction: 1) Tree shaking implementation, 2) Asset optimization, 3) Code minification, 4) Split targets...
Efficient state management: 1) Proper state scoping, 2) Minimal rebuild strategy, 3) State isolation patterns, 4)...
Network optimization practices: 1) Request caching implementation, 2) Efficient data serialization, 3) Connection...
Background processing strategies: 1) Isolate implementation, 2) Compute function usage, 3) Background fetch...
Database optimization patterns: 1) Batch operations usage, 2) Index optimization, 3) Query optimization, 4)...
Efficient error handling: 1) Error boundary implementation, 2) Exception catching optimization, 3) Error logging...
Platform channel optimization: 1) Batch method calls, 2) Data serialization efficiency, 3) Channel type selection,...
Rendering optimization includes: 1) Using RepaintBoundary effectively, 2) Minimizing layout passes, 3) Implementing...
File I/O optimization: 1) Asynchronous operations usage, 2) Buffered reading/writing, 3) Stream implementation, 4)...
Caching strategies include: 1) Memory cache implementation, 2) Disk cache management, 3) Cache invalidation...
Layout optimization techniques: 1) Widget tree optimization, 2) Layout algorithm selection, 3) Custom layout...
Gesture optimization: 1) GestureDetector configuration, 2) Hit test optimization, 3) Gesture arena management, 4)...
Form optimization strategies: 1) Validation optimization, 2) Input formatting efficiency, 3) State management...
State restoration efficiency: 1) Selective state saving, 2) Serialization optimization, 3) Restoration scope...
DI optimization patterns: 1) Lazy initialization, 2) Scope management, 3) Factory optimization, 4) Service locator...
Localization optimization: 1) Asset bundling strategy, 2) Language loading optimization, 3) String lookup...
Build method optimization: 1) Minimal computation, 2) Const widget usage, 3) Method extraction, 4) State access...
Route transition optimization: 1) Custom route implementation, 2) Animation optimization, 3) Hero widget usage, 4)...
Plugin optimization strategies: 1) Lazy loading implementation, 2) Platform channel efficiency, 3) Resource...
Custom paint optimization: 1) Canvas operation efficiency, 2) Paint object reuse, 3) Clipping optimization, 4) Layer...
Stream optimization patterns: 1) Subscription management, 2) Buffer size optimization, 3) Stream controller...
Platform Channels provide: 1) Communication between Flutter and native code, 2) Method channels for function calls,...
Platform-specific implementation includes: 1) Platform.isIOS/isAndroid checks, 2) Conditional widget rendering, 3)...
Channel types include: 1) MethodChannel for method invocation, 2) EventChannel for streaming data, 3)...
Permission handling includes: 1) Permission plugin integration, 2) Runtime permission requests, 3) Permission status...
Native UI integration approaches: 1) Platform Views implementation, 2) Hybrid composition, 3) Virtual displays, 4)...
Push notification implementation: 1) Firebase Cloud Messaging setup, 2) Platform-specific configuration, 3)...
Biometric authentication includes: 1) Local authentication plugin, 2) Platform-specific APIs, 3) Fingerprint...
File system access includes: 1) Platform-specific paths, 2) Storage permission handling, 3) File operations...
Platform styling strategies: 1) Cupertino and Material widgets, 2) Platform-specific themes, 3) Adaptive widget...
Camera implementation includes: 1) Camera plugin integration, 2) Permission handling, 3) Camera preview setup, 4)...
Background processing approaches: 1) WorkManager integration, 2) Background fetch, 3) Isolate implementation, 4)...
Platform gesture handling: 1) GestureDetector configuration, 2) Platform-specific recognition, 3) Custom gesture...
Sensor handling approaches: 1) Sensor plugin integration, 2) Platform-specific APIs, 3) Data stream management, 4)...
Platform navigation includes: 1) Platform-specific gestures, 2) Navigation patterns, 3) Deep linking handling, 4)...
Storage strategies include: 1) Shared preferences, 2) Keychain/Keystore access, 3) Secure storage implementation, 4)...
Platform Channels provide: 1) Communication between Flutter and native code, 2) Method channels for function calls,...
Platform-specific implementation includes: 1) Platform.isIOS/isAndroid checks, 2) Conditional widget rendering, 3)...
Channel types include: 1) MethodChannel for method invocation, 2) EventChannel for streaming data, 3)...
Permission handling includes: 1) Permission plugin integration, 2) Runtime permission requests, 3) Permission status...
Native UI integration approaches: 1) Platform Views implementation, 2) Hybrid composition, 3) Virtual displays, 4)...
Push notification implementation: 1) Firebase Cloud Messaging setup, 2) Platform-specific configuration, 3)...
Biometric authentication includes: 1) Local authentication plugin, 2) Platform-specific APIs, 3) Fingerprint...
File system access includes: 1) Platform-specific paths, 2) Storage permission handling, 3) File operations...
Platform styling strategies: 1) Cupertino and Material widgets, 2) Platform-specific themes, 3) Adaptive widget...
Camera implementation includes: 1) Camera plugin integration, 2) Permission handling, 3) Camera preview setup, 4)...
Background processing approaches: 1) WorkManager integration, 2) Background fetch, 3) Isolate implementation, 4)...
Platform gesture handling: 1) GestureDetector configuration, 2) Platform-specific recognition, 3) Custom gesture...
Sensor handling approaches: 1) Sensor plugin integration, 2) Platform-specific APIs, 3) Data stream management, 4)...
Platform navigation includes: 1) Platform-specific gestures, 2) Navigation patterns, 3) Deep linking handling, 4)...
Storage strategies include: 1) Shared preferences, 2) Keychain/Keystore access, 3) Secure storage implementation, 4)...
Platform networking includes: 1) SSL/Certificate handling, 2) Network security configuration, 3) Platform-specific...
In-app purchase handling: 1) Platform-specific store integration, 2) Product configuration, 3) Purchase flow...
Social media integration: 1) Platform-specific SDKs, 2) Authentication flows, 3) Share functionality, 4) Deep...
Location services handling: 1) Permission management, 2) GPS integration, 3) Background location updates, 4)...
Analytics implementation: 1) Platform-specific SDKs, 2) Event tracking, 3) User properties, 4) Custom dimensions, 5)...
Audio/video handling: 1) Codec support, 2) Platform-specific players, 3) Background playback, 4) Media controls, 5)...
Deep linking implementation: 1) URL scheme configuration, 2) Universal links setup, 3) App shortcuts definition, 4)...
Platform testing approaches: 1) Integration testing, 2) Platform-specific mocks, 3) Device testing, 4) Feature...
Security feature handling: 1) Keychain/Keystore usage, 2) Biometric integration, 3) SSL pinning, 4) App signing, 5)...
Authentication approaches: 1) Biometric integration, 2) OAuth implementation, 3) SSO configuration, 4) Token...
Accessibility implementation: 1) Screen reader support, 2) Platform-specific semantics, 3) Focus handling, 4) Custom...
Error handling strategies: 1) Platform-specific error codes, 2) Error mapping, 3) Recovery mechanisms, 4) User...
Performance monitoring: 1) Platform profiling tools, 2) Metric collection, 3) Performance triggers, 4) Memory...
Debugging approaches: 1) Platform debugger integration, 2) Logging mechanisms, 3) Debug bridges, 4) Network...
Flutter is a UI toolkit that offers: 1) Single codebase for multiple platforms (iOS, Android, Web, Desktop), 2) Widget-based development with rich customizable components, 3) Hot Reload for rapid development, 4) Direct compilation to native code without bridge, 5) Built-in Material Design and Cupertino widgets, 6) High performance with Skia rendering engine, 7) Complete development and debugging tools, 8) Strong community and package ecosystem.
Key differences include: 1) StatelessWidget is immutable and cannot change its state after creation, 2) StatefulWidget maintains mutable state with a separate State object, 3) StatelessWidget rebuild only when parent widget changes, 4) StatefulWidget can rebuild when setState() is called, 5) StatelessWidget is more memory efficient, 6) StatefulWidget is used for user interaction and dynamic content, 7) StatelessWidget is used for static content, 8) State persistence across rebuilds in StatefulWidget.
Widget Tree in Flutter: 1) Hierarchical structure of widgets that describes UI, 2) Parent widgets wrap and affect child widgets, 3) Rebuilds efficiently using element tree, 4) Supports inheritance of theme and media query data, 5) Manages state propagation through tree, 6) Implements build context for widget location, 7) Handles widget lifecycle and disposal, 8) Optimizes rendering through RenderObject tree.
Hot Reload: 1) Updates code changes without app restart, 2) Preserves app state during development, 3) Injects updated source code into running Dart VM, 4) Rebuilds widget tree with new code, 5) Cannot update native code or plugin changes, 6) Requires stateful hot reload capable code, 7) May need hot restart for some changes, 8) Maintains constructor state across reloads.
Key layout widgets: 1) Container for styling and positioning, 2) Row and Column for linear layouts, 3) Stack for overlapping elements, 4) Expanded and Flexible for responsive sizing, 5) GridView for grid layouts, 6) ListView for scrollable lists, 7) Wrap for flow layouts, 8) Custom SingleChildScrollView for scrollable content. Usage depends on layout requirements and content organization needs.
BuildContext provides: 1) Location of widget in widget tree, 2) Access to inherited widgets, 3) Theme and MediaQuery data access, 4) Navigation services, 5) State management access, 6) Localization data, 7) Scaffold messenger access, 8) Widget tree traversal capabilities. Essential for accessing widget tree information and services.
Keys are used for: 1) Maintaining widget state across moves in widget tree, 2) Uniquely identifying widgets, 3) Preserving scroll position in lists, 4) Managing widget lifecycle, 5) Handling dynamic widget updates, 6) Implementing list item reordering, 7) Preserving form field states, 8) Optimizing widget rebuilds. Important for widget identity and state preservation.
Widget lifecycle includes: 1) Constructor creation, 2) initState for StatefulWidget initialization, 3) didChangeDependencies for inherited widget changes, 4) build for UI rendering, 5) didUpdateWidget for widget updates, 6) setState for state changes, 7) dispose for cleanup, 8) deactivate for temporary removal. Understanding lifecycle is crucial for proper state management.
InheritedWidget provides: 1) Efficient data sharing down widget tree, 2) Automatic rebuild of dependent widgets, 3) Access to shared data through context, 4) State management capabilities, 5) Theme and MediaQuery implementation, 6) Dependency tracking, 7) Performance optimization, 8) Widget tree scoped data access. Used for efficient data propagation.
Responsive design techniques: 1) Using MediaQuery for screen dimensions, 2) LayoutBuilder for constraints-based layouts, 3) Flexible and Expanded widgets, 4) OrientationBuilder for orientation changes, 5) Responsive grid systems, 6) Adaptive widgets, 7) Custom responsive layouts, 8) Platform-specific adaptations. Essential for cross-device compatibility.
Key differences: 1) Container allows decoration and padding, 2) SizedBox is more lightweight and efficient, 3) Container can have multiple children through child property, 4) SizedBox primarily for dimensional constraints, 5) Container supports margin and alignment, 6) SizedBox for fixed dimensions, 7) Container for styling purposes, 8) SizedBox for space creation.
Gesture handling includes: 1) GestureDetector widget for touch events, 2) InkWell for Material Design ripple effect, 3) Drag and drop support, 4) Multi-touch handling, 5) Custom gesture recognition, 6) Pan and zoom gestures, 7) Tap and long press detection, 8) Gesture arena resolution.
Button types include: 1) ElevatedButton for raised buttons, 2) TextButton for flat buttons, 3) OutlinedButton for outlined style, 4) IconButton for icon-only buttons, 5) FloatingActionButton for floating actions, 6) PopupMenuButton for menus, 7) DropdownButton for selections, 8) Custom button implementations.
Form validation involves: 1) Form widget for form state management, 2) TextFormField for input validation, 3) GlobalKey<FormState> for form control, 4) Custom validators, 5) Form submission handling, 6) Error message display, 7) Real-time validation, 8) Form reset functionality.
Custom widgets are used for: 1) Reusable UI components, 2) Complex widget combinations, 3) Encapsulating business logic, 4) Improving code organization, 5) Maintaining consistent styling, 6) Reducing code duplication, 7) Better testing isolation, 8) Component abstraction.
Flutter is a UI toolkit that offers: 1) Single codebase for multiple platforms (iOS, Android, Web, Desktop), 2) Widget-based development with rich customizable components, 3) Hot Reload for rapid development, 4) Direct compilation to native code without bridge, 5) Built-in Material Design and Cupertino widgets, 6) High performance with Skia rendering engine, 7) Complete development and debugging tools, 8) Strong community and package ecosystem.
Key differences include: 1) StatelessWidget is immutable and cannot change its state after creation, 2) StatefulWidget maintains mutable state with a separate State object, 3) StatelessWidget rebuild only when parent widget changes, 4) StatefulWidget can rebuild when setState() is called, 5) StatelessWidget is more memory efficient, 6) StatefulWidget is used for user interaction and dynamic content, 7) StatelessWidget is used for static content, 8) State persistence across rebuilds in StatefulWidget.
Widget Tree in Flutter: 1) Hierarchical structure of widgets that describes UI, 2) Parent widgets wrap and affect child widgets, 3) Rebuilds efficiently using element tree, 4) Supports inheritance of theme and media query data, 5) Manages state propagation through tree, 6) Implements build context for widget location, 7) Handles widget lifecycle and disposal, 8) Optimizes rendering through RenderObject tree.
Hot Reload: 1) Updates code changes without app restart, 2) Preserves app state during development, 3) Injects updated source code into running Dart VM, 4) Rebuilds widget tree with new code, 5) Cannot update native code or plugin changes, 6) Requires stateful hot reload capable code, 7) May need hot restart for some changes, 8) Maintains constructor state across reloads.
Key layout widgets: 1) Container for styling and positioning, 2) Row and Column for linear layouts, 3) Stack for overlapping elements, 4) Expanded and Flexible for responsive sizing, 5) GridView for grid layouts, 6) ListView for scrollable lists, 7) Wrap for flow layouts, 8) Custom SingleChildScrollView for scrollable content. Usage depends on layout requirements and content organization needs.
BuildContext provides: 1) Location of widget in widget tree, 2) Access to inherited widgets, 3) Theme and MediaQuery data access, 4) Navigation services, 5) State management access, 6) Localization data, 7) Scaffold messenger access, 8) Widget tree traversal capabilities. Essential for accessing widget tree information and services.
Keys are used for: 1) Maintaining widget state across moves in widget tree, 2) Uniquely identifying widgets, 3) Preserving scroll position in lists, 4) Managing widget lifecycle, 5) Handling dynamic widget updates, 6) Implementing list item reordering, 7) Preserving form field states, 8) Optimizing widget rebuilds. Important for widget identity and state preservation.
Widget lifecycle includes: 1) Constructor creation, 2) initState for StatefulWidget initialization, 3) didChangeDependencies for inherited widget changes, 4) build for UI rendering, 5) didUpdateWidget for widget updates, 6) setState for state changes, 7) dispose for cleanup, 8) deactivate for temporary removal. Understanding lifecycle is crucial for proper state management.
InheritedWidget provides: 1) Efficient data sharing down widget tree, 2) Automatic rebuild of dependent widgets, 3) Access to shared data through context, 4) State management capabilities, 5) Theme and MediaQuery implementation, 6) Dependency tracking, 7) Performance optimization, 8) Widget tree scoped data access. Used for efficient data propagation.
Responsive design techniques: 1) Using MediaQuery for screen dimensions, 2) LayoutBuilder for constraints-based layouts, 3) Flexible and Expanded widgets, 4) OrientationBuilder for orientation changes, 5) Responsive grid systems, 6) Adaptive widgets, 7) Custom responsive layouts, 8) Platform-specific adaptations. Essential for cross-device compatibility.
Key differences: 1) Container allows decoration and padding, 2) SizedBox is more lightweight and efficient, 3) Container can have multiple children through child property, 4) SizedBox primarily for dimensional constraints, 5) Container supports margin and alignment, 6) SizedBox for fixed dimensions, 7) Container for styling purposes, 8) SizedBox for space creation.
Gesture handling includes: 1) GestureDetector widget for touch events, 2) InkWell for Material Design ripple effect, 3) Drag and drop support, 4) Multi-touch handling, 5) Custom gesture recognition, 6) Pan and zoom gestures, 7) Tap and long press detection, 8) Gesture arena resolution.
Button types include: 1) ElevatedButton for raised buttons, 2) TextButton for flat buttons, 3) OutlinedButton for outlined style, 4) IconButton for icon-only buttons, 5) FloatingActionButton for floating actions, 6) PopupMenuButton for menus, 7) DropdownButton for selections, 8) Custom button implementations.
Form validation involves: 1) Form widget for form state management, 2) TextFormField for input validation, 3) GlobalKey<FormState> for form control, 4) Custom validators, 5) Form submission handling, 6) Error message display, 7) Real-time validation, 8) Form reset functionality.
Custom widgets are used for: 1) Reusable UI components, 2) Complex widget combinations, 3) Encapsulating business logic, 4) Improving code organization, 5) Maintaining consistent styling, 6) Reducing code duplication, 7) Better testing isolation, 8) Component abstraction.
Key differences include: 1) const is compile-time constant, final is runtime constant, 2) const requires all values to be known at compile time, 3) final can be set once at runtime, 4) const objects are canonicalized, 5) const constructors create immutable objects, 6) final allows for lazy initialization, 7) const improves performance for widgets, 8) final is used for runtime-dependent values.
Differences include: 1) SafeArea adjusts for system intrusions, 2) Padding adds space around content, 3) SafeArea handles notches and system bars, 4) Padding is for explicit spacing control, 5) SafeArea uses MediaQuery data, 6) Padding uses EdgeInsets, 7) SafeArea for device-specific adjustments, 8) Padding for layout spacing.
Flex and Expanded features: 1) Flex determines axis of layout, 2) Expanded fills available space, 3) flex factor for space distribution, 4) Flexible vs Expanded behavior, 5) Cross-axis alignment control, 6) Main-axis size behavior, 7) Nested flex layouts, 8) Space distribution algorithms.
Slivers provide: 1) Custom scrolling effects, 2) Efficient scrollable layouts, 3) AppBar animations, 4) Lazy loading of content, 5) Custom scroll physics, 6) Persistent headers, 7) Grid and list layouts, 8) Performance optimization for scrolling. Used for complex scrolling behaviors.
Asset management includes: 1) pubspec.yaml configuration, 2) Asset bundling process, 3) Resolution-aware images, 4) Font integration, 5) Asset variants handling, 6) Package asset access, 7) Asset loading optimization, 8) Asset organization strategies.
GlobalKey purposes: 1) Accessing widget state across tree, 2) Unique widget identification, 3) Form validation control, 4) Navigator state access, 5) Scaffold manipulation, 6) Animation control, 7) Widget reference maintenance, 8) Cross-tree communication.
Keyboard handling includes: 1) FocusNode management, 2) TextInput configuration, 3) Keyboard type selection, 4) Keyboard visibility detection, 5) Input action handling, 6) Focus traversal, 7) Keyboard dismissal, 8) Custom keyboard layouts.
ScrollView types include: 1) ListView for linear scrolling, 2) GridView for grid layouts, 3) SingleChildScrollView for single child, 4) CustomScrollView for slivers, 5) PageView for swipeable pages, 6) NestedScrollView for nested scrolling, 7) ScrollController usage, 8) Scroll physics customization.
Theming implementation: 1) ThemeData configuration, 2) Theme inheritance, 3) Custom theme creation, 4) Dark mode support, 5) Dynamic theme switching, 6) Theme extension, 7) Platform-specific theming, 8) Theme data persistence.
Stack and Positioned: 1) Stack allows overlapping children, 2) Positioned controls child position, 3) Stack alignment properties, 4) Positioned absolute positioning, 5) Stack fit behavior, 6) Z-index ordering, 7) Overflow handling, 8) Size constraints management.
Error handling includes: 1) ErrorWidget customization, 2) BuildContext error handling, 3) Error callback implementation, 4) Widget error recovery, 5) Error reporting, 6) Debug error display, 7) Production error handling, 8) Error boundary widgets.
Widget composition practices: 1) Single responsibility principle, 2) Proper widget extraction, 3) State management consideration, 4) Performance optimization, 5) Code reusability, 6) Proper inheritance use, 7) Component interface design, 8) Widget testing strategy.
Localization implementation: 1) MaterialApp localization delegates, 2) Locale resolution, 3) Asset localization, 4) String internationalization, 5) RTL support, 6) Currency formatting, 7) Date/time localization, 8) Custom localizations.
Visibility approaches: 1) Visibility widget usage, 2) Opacity widget control, 3) Offstage widget, 4) Conditional rendering, 5) AnimatedOpacity transitions, 6) Layout builder conditions, 7) Stack positioning, 8) Custom visibility controllers.
Disposal and cleanup: 1) dispose() method implementation, 2) Controller cleanup, 3) Stream subscription cancellation, 4) Animation controller disposal, 5) Timer cancellation, 6) Resource release, 7) Memory leak prevention, 8) State cleanup patterns.
Popular state management solutions include: 1) Provider for dependency injection and state management, 2) Bloc pattern for reactive state management, 3) GetX for simple state management, 4) Riverpod for improved Provider pattern, 5) MobX for reactive programming, 6) Redux for unidirectional data flow, 7) InheritedWidget for built-in state propagation, 8) State management with ValueNotifier.
BLoC pattern features: 1) Separation of UI and business logic, 2) Stream-based state management, 3) Event-driven architecture, 4) Reactive programming principles, 5) State transformation through streams, 6) Easy testing and maintenance, 7) Handle complex state transitions, 8) Support for multiple event sources.
Provider offers: 1) Dependency injection capabilities, 2) Widget tree state management, 3) Easy data sharing between widgets, 4) Multiple provider types (ChangeNotifier, Stream, Value), 5) Context-based access to state, 6) Lifecycle management, 7) Efficient rebuilds, 8) Simple implementation compared to other solutions.
Key differences: 1) Ephemeral state is local to a widget, 2) App state is shared across multiple widgets, 3) Ephemeral state uses setState(), 4) App state needs state management solution, 5) Ephemeral state for UI-only state, 6) App state for business logic, 7) Ephemeral state is simpler to manage, 8) App state requires careful architecture.
GetX provides: 1) Simple reactive state management, 2) Dependencies injection, 3) Route management, 4) Memory management, 5) Utilities and extensions, 6) Performance optimization, 7) Separation of concerns, 8) Easy syntax for state updates.
Riverpod advantages: 1) Compile-time safety, 2) Better error handling, 3) No context requirement, 4) Multiple provider declarations, 5) Improved testing capabilities, 6) Auto-disposal of providers, 7) Family modifier support, 8) Override providers in tests.
Redux implementation includes: 1) Single store for state, 2) Action creators for state changes, 3) Reducers for state updates, 4) Middleware for side effects, 5) StoreProvider setup, 6) Connect widgets to store, 7) Implement pure functions, 8) Handle async actions.
MobX features: 1) Observable state tracking, 2) Automatic updates through reactions, 3) Computed values, 4) Actions for state modification, 5) Code generation for boilerplate, 6) Reactive programming model, 7) Side effects handling, 8) Integration with Flutter widgets.
Form state management: 1) Form widget usage, 2) FormField implementations, 3) GlobalKey<FormState>, 4) Validation logic, 5) Save and reset operations, 6) Error handling, 7) Custom form fields, 8) State persistence across rebuilds.
Global state patterns: 1) Singleton pattern, 2) Service locator pattern, 3) Provider at app root, 4) Redux store, 5) GetX services, 6) Static services, 7) Dependency injection, 8) Event bus pattern.
State persistence methods: 1) SharedPreferences for simple data, 2) SQLite for structured data, 3) Hive for NoSQL storage, 4) File storage for large data, 5) Secure storage for sensitive data, 6) State restoration, 7) Caching strategies, 8) Migration handling.
ChangeNotifier provides: 1) Simple state change notifications, 2) Listener management, 3) Mixin capabilities, 4) Integration with Provider, 5) Automatic cleanup, 6) Fine-grained updates, 7) Memory leak prevention, 8) Widget rebuild optimization.
Testing approaches: 1) Unit testing state logic, 2) Widget testing with state, 3) Integration testing, 4) Mock state management, 5) Test state transitions, 6) Async state testing, 7) Provider testing, 8) BLoC testing patterns.
State organization practices: 1) Separation of concerns, 2) Single responsibility principle, 3) Immutable state objects, 4) Clear state interfaces, 5) Proper error handling, 6) State restoration support, 7) Documentation, 8) Performance optimization.
Complex state handling: 1) State machine implementation, 2) Transition validation, 3) Side effect management, 4) Error state handling, 5) State history tracking, 6) Undo/redo support, 7) State snapshots, 8) Transition logging.
Popular state management solutions include: 1) Provider for dependency injection and state management, 2) Bloc pattern for reactive state management, 3) GetX for simple state management, 4) Riverpod for improved Provider pattern, 5) MobX for reactive programming, 6) Redux for unidirectional data flow, 7) InheritedWidget for built-in state propagation, 8) State management with ValueNotifier.
BLoC pattern features: 1) Separation of UI and business logic, 2) Stream-based state management, 3) Event-driven architecture, 4) Reactive programming principles, 5) State transformation through streams, 6) Easy testing and maintenance, 7) Handle complex state transitions, 8) Support for multiple event sources.
Provider offers: 1) Dependency injection capabilities, 2) Widget tree state management, 3) Easy data sharing between widgets, 4) Multiple provider types (ChangeNotifier, Stream, Value), 5) Context-based access to state, 6) Lifecycle management, 7) Efficient rebuilds, 8) Simple implementation compared to other solutions.
Key differences: 1) Ephemeral state is local to a widget, 2) App state is shared across multiple widgets, 3) Ephemeral state uses setState(), 4) App state needs state management solution, 5) Ephemeral state for UI-only state, 6) App state for business logic, 7) Ephemeral state is simpler to manage, 8) App state requires careful architecture.
GetX provides: 1) Simple reactive state management, 2) Dependencies injection, 3) Route management, 4) Memory management, 5) Utilities and extensions, 6) Performance optimization, 7) Separation of concerns, 8) Easy syntax for state updates.
Riverpod advantages: 1) Compile-time safety, 2) Better error handling, 3) No context requirement, 4) Multiple provider declarations, 5) Improved testing capabilities, 6) Auto-disposal of providers, 7) Family modifier support, 8) Override providers in tests.
Redux implementation includes: 1) Single store for state, 2) Action creators for state changes, 3) Reducers for state updates, 4) Middleware for side effects, 5) StoreProvider setup, 6) Connect widgets to store, 7) Implement pure functions, 8) Handle async actions.
MobX features: 1) Observable state tracking, 2) Automatic updates through reactions, 3) Computed values, 4) Actions for state modification, 5) Code generation for boilerplate, 6) Reactive programming model, 7) Side effects handling, 8) Integration with Flutter widgets.
Form state management: 1) Form widget usage, 2) FormField implementations, 3) GlobalKey<FormState>, 4) Validation logic, 5) Save and reset operations, 6) Error handling, 7) Custom form fields, 8) State persistence across rebuilds.
Global state patterns: 1) Singleton pattern, 2) Service locator pattern, 3) Provider at app root, 4) Redux store, 5) GetX services, 6) Static services, 7) Dependency injection, 8) Event bus pattern.
State persistence methods: 1) SharedPreferences for simple data, 2) SQLite for structured data, 3) Hive for NoSQL storage, 4) File storage for large data, 5) Secure storage for sensitive data, 6) State restoration, 7) Caching strategies, 8) Migration handling.
ChangeNotifier provides: 1) Simple state change notifications, 2) Listener management, 3) Mixin capabilities, 4) Integration with Provider, 5) Automatic cleanup, 6) Fine-grained updates, 7) Memory leak prevention, 8) Widget rebuild optimization.
Testing approaches: 1) Unit testing state logic, 2) Widget testing with state, 3) Integration testing, 4) Mock state management, 5) Test state transitions, 6) Async state testing, 7) Provider testing, 8) BLoC testing patterns.
State organization practices: 1) Separation of concerns, 2) Single responsibility principle, 3) Immutable state objects, 4) Clear state interfaces, 5) Proper error handling, 6) State restoration support, 7) Documentation, 8) Performance optimization.
Complex state handling: 1) State machine implementation, 2) Transition validation, 3) Side effect management, 4) Error state handling, 5) State history tracking, 6) Undo/redo support, 7) State snapshots, 8) Transition logging.
State restoration includes: 1) RestorationMixin implementation, 2) RestorationProperties usage, 3) State serialization, 4) Restoration ID management, 5) Widget state preservation, 6) Platform integration, 7) Complex object restoration, 8) Testing restoration logic.
Combination patterns: 1) Layer separation by responsibility, 2) Bridge pattern implementation, 3) State transformation adapters, 4) Event forwarding, 5) State synchronization, 6) Compatibility layers, 7) Performance optimization, 8) Testing strategy.
Real-time state management: 1) Stream-based updates, 2) WebSocket integration, 3) State synchronization, 4) Conflict resolution, 5) Offline state handling, 6) Event ordering, 7) State reconciliation, 8) Background updates.
Optimization strategies: 1) Selective rebuilds, 2) State granularity control, 3) Memoization techniques, 4) Batch updates, 5) Async state updates, 6) Cache management, 7) Event debouncing, 8) Update prioritization.
Dependency injection patterns: 1) Service locator pattern, 2) Provider injection, 3) GetIt implementation, 4) Factory patterns, 5) Scope management, 6) Lazy initialization, 7) Testing support, 8) Lifecycle management.
Error state handling: 1) Error state models, 2) Error propagation, 3) Recovery mechanisms, 4) User feedback, 5) Error logging, 6) State rollback, 7) Retry mechanisms, 8) Error boundary implementation.
Undo/redo implementation: 1) Command pattern usage, 2) State history stack, 3) Memento pattern, 4) Action serialization, 5) State snapshots, 6) Memory optimization, 7) Composite commands, 8) History limitation.
Nested state patterns: 1) Composite state objects, 2) State tree management, 3) Scoped updates, 4) Parent-child state sync, 5) State inheritance, 6) Nested providers, 7) State isolation, 8) Update propagation.
Offline state management: 1) Local persistence, 2) Sync strategies, 3) Conflict resolution, 4) Queue management, 5) State merging, 6) Version control, 7) Background sync, 8) Error recovery.
Debugging practices: 1) State logging implementation, 2) DevTools integration, 3) State visualization, 4) Time-travel debugging, 5) State assertions, 6) Error tracking, 7) Performance monitoring, 8) Test scenario reproduction.
State-based navigation: 1) Route state management, 2) Deep linking support, 3) Navigation state persistence, 4) State-based redirects, 5) Navigation guards, 6) Route parameters, 7) Navigation history, 8) State restoration.
Multi-window state patterns: 1) State synchronization, 2) Shared state storage, 3) Window communication, 4) State isolation, 5) Update propagation, 6) Conflict resolution, 7) State persistence, 8) Window lifecycle management.
State-based theming: 1) Theme state management, 2) Dynamic theme switching, 3) Theme persistence, 4) Custom theme data, 5) Platform-specific themes, 6) Theme inheritance, 7) Theme animation, 8) Theme state restoration.
Widget testing approaches: 1) State mocking, 2) Provider testing, 3) BLoC test implementation, 4) State initialization, 5) Async state testing, 6) State changes verification, 7) Integration testing, 8) Error state testing.
Key performance factors include: 1) Widget rebuild optimization, 2) Memory management and leaks, 3) Image loading and caching, 4) State management efficiency, 5) Navigation and routing overhead, 6) Animation performance, 7) Network request handling, 8) Platform-specific optimizations. Understanding these factors is crucial for optimizing Flutter applications.
Widget rebuild optimization includes: 1) Using const constructors, 2) Implementing shouldRebuild method, 3) Proper widget tree structuring, 4) State management optimization, 5) Using ValueNotifier for specific updates, 6) Implementing RepaintBoundary, 7) Minimizing setState scope, 8) Using BuildContext efficiently.
Memory management practices: 1) Disposing controllers and streams, 2) Image caching strategies, 3) Lazy loading implementation, 4) Proper state cleanup, 5) Memory leak detection, 6) Resource pooling, 7) Background task management, 8) Large list optimization.
Image optimization techniques: 1) Proper image caching, 2) Resolution-aware image loading, 3) Lazy loading for lists, 4) Image compression strategies, 5) Memory-efficient image formats, 6) Placeholder implementations, 7) Network image optimization, 8) Asset size management.
Performance profiling tools: 1) Flutter DevTools, 2) Performance overlay, 3) Timeline view, 4) Memory profiler, 5) CPU profiler, 6) Layout explorer, 7) Widget inspector, 8) Platform-specific profiling tools. These tools help identify and resolve performance bottlenecks.
Animation optimization includes: 1) Using explicit animations, 2) Implementing repaint boundaries, 3) Hardware acceleration usage, 4) Frame callback optimization, 5) Compositor-friendly animations, 6) Reducing animation complexity, 7) Using AnimationController efficiently, 8) Performance monitoring.
Startup optimization strategies: 1) Deferred components loading, 2) Asset preloading optimization, 3) Initialization order management, 4) Plugin loading optimization, 5) Reducing app size, 6) Code optimization, 7) Platform-specific optimizations, 8) Init-time compilation.
List optimization techniques: 1) ListView.builder usage, 2) Caching list items, 3) Implementing pagination, 4) Using const widgets, 5) Image loading optimization, 6) Item recycling, 7) Lazy loading implementation, 8) Memory management strategies.
App size reduction: 1) Tree shaking implementation, 2) Asset optimization, 3) Code minification, 4) Split targets configuration, 5) Plugin optimization, 6) Platform-specific code separation, 7) Dependency management, 8) Resource compression.
Efficient state management: 1) Proper state scoping, 2) Minimal rebuild strategy, 3) State isolation patterns, 4) Change notification optimization, 5) Memory-efficient state, 6) State disposal handling, 7) Batch updates implementation, 8) State persistence optimization.
Network optimization practices: 1) Request caching implementation, 2) Efficient data serialization, 3) Connection pooling, 4) Response compression, 5) Request batching, 6) Error handling optimization, 7) Background download management, 8) Network state handling.
Background processing strategies: 1) Isolate implementation, 2) Compute function usage, 3) Background fetch optimization, 4) Platform channels efficiency, 5) Work Manager integration, 6) Resource management, 7) Background state handling, 8) Memory constraints management.
Database optimization patterns: 1) Batch operations usage, 2) Index optimization, 3) Query optimization, 4) Connection pooling, 5) Caching strategies, 6) Async operation management, 7) Transaction optimization, 8) Memory-efficient queries.
Efficient error handling: 1) Error boundary implementation, 2) Exception catching optimization, 3) Error logging efficiency, 4) Recovery strategy optimization, 5) Memory leak prevention, 6) Stack trace handling, 7) Error reporting optimization, 8) Debug mode performance.
Platform channel optimization: 1) Batch method calls, 2) Data serialization efficiency, 3) Channel type selection, 4) Event channel optimization, 5) Background thread usage, 6) Error handling efficiency, 7) Resource cleanup, 8) Memory management.
Key performance factors include: 1) Widget rebuild optimization, 2) Memory management and leaks, 3) Image loading and caching, 4) State management efficiency, 5) Navigation and routing overhead, 6) Animation performance, 7) Network request handling, 8) Platform-specific optimizations. Understanding these factors is crucial for optimizing Flutter applications.
Widget rebuild optimization includes: 1) Using const constructors, 2) Implementing shouldRebuild method, 3) Proper widget tree structuring, 4) State management optimization, 5) Using ValueNotifier for specific updates, 6) Implementing RepaintBoundary, 7) Minimizing setState scope, 8) Using BuildContext efficiently.
Memory management practices: 1) Disposing controllers and streams, 2) Image caching strategies, 3) Lazy loading implementation, 4) Proper state cleanup, 5) Memory leak detection, 6) Resource pooling, 7) Background task management, 8) Large list optimization.
Image optimization techniques: 1) Proper image caching, 2) Resolution-aware image loading, 3) Lazy loading for lists, 4) Image compression strategies, 5) Memory-efficient image formats, 6) Placeholder implementations, 7) Network image optimization, 8) Asset size management.
Performance profiling tools: 1) Flutter DevTools, 2) Performance overlay, 3) Timeline view, 4) Memory profiler, 5) CPU profiler, 6) Layout explorer, 7) Widget inspector, 8) Platform-specific profiling tools. These tools help identify and resolve performance bottlenecks.
Animation optimization includes: 1) Using explicit animations, 2) Implementing repaint boundaries, 3) Hardware acceleration usage, 4) Frame callback optimization, 5) Compositor-friendly animations, 6) Reducing animation complexity, 7) Using AnimationController efficiently, 8) Performance monitoring.
Startup optimization strategies: 1) Deferred components loading, 2) Asset preloading optimization, 3) Initialization order management, 4) Plugin loading optimization, 5) Reducing app size, 6) Code optimization, 7) Platform-specific optimizations, 8) Init-time compilation.
List optimization techniques: 1) ListView.builder usage, 2) Caching list items, 3) Implementing pagination, 4) Using const widgets, 5) Image loading optimization, 6) Item recycling, 7) Lazy loading implementation, 8) Memory management strategies.
App size reduction: 1) Tree shaking implementation, 2) Asset optimization, 3) Code minification, 4) Split targets configuration, 5) Plugin optimization, 6) Platform-specific code separation, 7) Dependency management, 8) Resource compression.
Efficient state management: 1) Proper state scoping, 2) Minimal rebuild strategy, 3) State isolation patterns, 4) Change notification optimization, 5) Memory-efficient state, 6) State disposal handling, 7) Batch updates implementation, 8) State persistence optimization.
Network optimization practices: 1) Request caching implementation, 2) Efficient data serialization, 3) Connection pooling, 4) Response compression, 5) Request batching, 6) Error handling optimization, 7) Background download management, 8) Network state handling.
Background processing strategies: 1) Isolate implementation, 2) Compute function usage, 3) Background fetch optimization, 4) Platform channels efficiency, 5) Work Manager integration, 6) Resource management, 7) Background state handling, 8) Memory constraints management.
Database optimization patterns: 1) Batch operations usage, 2) Index optimization, 3) Query optimization, 4) Connection pooling, 5) Caching strategies, 6) Async operation management, 7) Transaction optimization, 8) Memory-efficient queries.
Efficient error handling: 1) Error boundary implementation, 2) Exception catching optimization, 3) Error logging efficiency, 4) Recovery strategy optimization, 5) Memory leak prevention, 6) Stack trace handling, 7) Error reporting optimization, 8) Debug mode performance.
Platform channel optimization: 1) Batch method calls, 2) Data serialization efficiency, 3) Channel type selection, 4) Event channel optimization, 5) Background thread usage, 6) Error handling efficiency, 7) Resource cleanup, 8) Memory management.
Rendering optimization includes: 1) Using RepaintBoundary effectively, 2) Minimizing layout passes, 3) Implementing custom render objects, 4) Optimizing shader compilation, 5) Frame pipeline optimization, 6) GPU thread management, 7) Vsync implementation, 8) Render tree optimization.
File I/O optimization: 1) Asynchronous operations usage, 2) Buffered reading/writing, 3) Stream implementation, 4) Cache management, 5) Batch operations, 6) Memory mapping for large files, 7) File compression strategies, 8) Resource cleanup patterns.
Caching strategies include: 1) Memory cache implementation, 2) Disk cache management, 3) Cache invalidation policies, 4) LRU cache implementation, 5) Cache size optimization, 6) Cache warming strategies, 7) Network cache handling, 8) Cache synchronization.
Layout optimization techniques: 1) Widget tree optimization, 2) Layout algorithm selection, 3) Custom layout implementation, 4) Constraint optimization, 5) Layout caching, 6) Lazy widget building, 7) Size calculation optimization, 8) Layout rebuild minimization.
Gesture optimization: 1) GestureDetector configuration, 2) Hit test optimization, 3) Gesture arena management, 4) Touch slop customization, 5) Gesture recognition efficiency, 6) Event propagation control, 7) Memory usage optimization, 8) Concurrent gesture handling.
Form optimization strategies: 1) Validation optimization, 2) Input formatting efficiency, 3) State management optimization, 4) Form field caching, 5) Memory leak prevention, 6) Form submission optimization, 7) Error handling efficiency, 8) Form reset optimization.
State restoration efficiency: 1) Selective state saving, 2) Serialization optimization, 3) Restoration scope management, 4) Memory usage optimization, 5) Async restoration handling, 6) State versioning, 7) Restoration prioritization, 8) Error recovery optimization.
DI optimization patterns: 1) Lazy initialization, 2) Scope management, 3) Factory optimization, 4) Service locator efficiency, 5) Dependency tree optimization, 6) Memory management, 7) Initialization order, 8) Cleanup strategies.
Localization optimization: 1) Asset bundling strategy, 2) Language loading optimization, 3) String lookup efficiency, 4) Memory usage optimization, 5) Locale switching performance, 6) Resource management, 7) Fallback handling, 8) Cache implementation.
Build method optimization: 1) Minimal computation, 2) Const widget usage, 3) Method extraction, 4) State access efficiency, 5) Context usage optimization, 6) Key implementation, 7) Widget factoring, 8) Conditional rendering optimization.
Route transition optimization: 1) Custom route implementation, 2) Animation optimization, 3) Hero widget usage, 4) Page route caching, 5) Navigator optimization, 6) Memory management, 7) Gesture handling, 8) Transition state management.
Plugin optimization strategies: 1) Lazy loading implementation, 2) Platform channel efficiency, 3) Resource management, 4) Memory leak prevention, 5) Background operation handling, 6) Error handling optimization, 7) Plugin lifecycle management, 8) Version compatibility handling.
Custom paint optimization: 1) Canvas operation efficiency, 2) Paint object reuse, 3) Clipping optimization, 4) Layer management, 5) Render object caching, 6) Drawing command batching, 7) Memory usage optimization, 8) Repaint boundary usage.
Stream optimization patterns: 1) Subscription management, 2) Buffer size optimization, 3) Stream controller efficiency, 4) Memory leak prevention, 5) Event processing optimization, 6) Error handling efficiency, 7) Stream transformation optimization, 8) Cleanup implementation.
Platform Channels provide: 1) Communication between Flutter and native code, 2) Method channels for function calls, 3) Event channels for continuous data streams, 4) Basic value channels for simple data, 5) Binary messenger for raw data, 6) Async/await support, 7) Error handling mechanisms, 8) Type-safe message passing.
Platform-specific implementation includes: 1) Platform.isIOS/isAndroid checks, 2) Conditional widget rendering, 3) Platform-specific plugins, 4) Custom channel implementation, 5) Platform-specific UI components, 6) Feature detection, 7) Fallback mechanisms, 8) Platform-specific configuration.
Channel types include: 1) MethodChannel for method invocation, 2) EventChannel for streaming data, 3) BasicMessageChannel for custom data, 4) BinaryMessenger for raw data, 5) JSONMessageCodec support, 6) StandardMessageCodec usage, 7) Custom codec implementation, 8) Platform-specific channel variations.
Permission handling includes: 1) Permission plugin integration, 2) Runtime permission requests, 3) Permission status checking, 4) Permission denial handling, 5) Platform-specific permission logic, 6) Permission group management, 7) Permission callback handling, 8) Permission persistence.
Native UI integration approaches: 1) Platform Views implementation, 2) Hybrid composition, 3) Virtual displays, 4) AndroidView and UiKitView, 5) Native widget embedding, 6) Performance optimization, 7) Lifecycle management, 8) Platform-specific styling.
Push notification implementation: 1) Firebase Cloud Messaging setup, 2) Platform-specific configuration, 3) Notification handling, 4) Background message processing, 5) Notification permissions, 6) Custom notification UI, 7) Deep link handling, 8) Token management.
Biometric authentication includes: 1) Local authentication plugin, 2) Platform-specific APIs, 3) Fingerprint authentication, 4) Face ID integration, 5) Error handling, 6) Fallback mechanisms, 7) Security level configuration, 8) Authentication state management.
File system access includes: 1) Platform-specific paths, 2) Storage permission handling, 3) File operations implementation, 4) Directory management, 5) External storage access, 6) File metadata handling, 7) Platform restrictions handling, 8) Error management.
Platform styling strategies: 1) Cupertino and Material widgets, 2) Platform-specific themes, 3) Adaptive widget usage, 4) Custom platform detection, 5) Platform-specific assets, 6) Dynamic styling, 7) Platform-specific layouts, 8) Theme inheritance.
Camera implementation includes: 1) Camera plugin integration, 2) Permission handling, 3) Camera preview setup, 4) Image capture handling, 5) Video recording, 6) Camera controls, 7) Platform-specific features, 8) Error handling.
Background processing approaches: 1) WorkManager integration, 2) Background fetch, 3) Isolate implementation, 4) Platform-specific services, 5) Background tasks scheduling, 6) Battery optimization, 7) State persistence, 8) Error recovery.
Platform gesture handling: 1) GestureDetector configuration, 2) Platform-specific recognition, 3) Custom gesture implementation, 4) Touch feedback, 5) Gesture conflict resolution, 6) Platform-specific animations, 7) Accessibility support, 8) Error handling.
Sensor handling approaches: 1) Sensor plugin integration, 2) Platform-specific APIs, 3) Data stream management, 4) Sensor calibration, 5) Battery optimization, 6) Error handling, 7) Permission management, 8) Platform compatibility.
Platform navigation includes: 1) Platform-specific gestures, 2) Navigation patterns, 3) Deep linking handling, 4) Custom transitions, 5) Back button behavior, 6) Navigation state management, 7) Platform-specific routes, 8) Error handling.
Storage strategies include: 1) Shared preferences, 2) Keychain/Keystore access, 3) Secure storage implementation, 4) Platform-specific APIs, 5) Data encryption, 6) Storage permissions, 7) Migration handling, 8) Error management.
Platform Channels provide: 1) Communication between Flutter and native code, 2) Method channels for function calls, 3) Event channels for continuous data streams, 4) Basic value channels for simple data, 5) Binary messenger for raw data, 6) Async/await support, 7) Error handling mechanisms, 8) Type-safe message passing.
Platform-specific implementation includes: 1) Platform.isIOS/isAndroid checks, 2) Conditional widget rendering, 3) Platform-specific plugins, 4) Custom channel implementation, 5) Platform-specific UI components, 6) Feature detection, 7) Fallback mechanisms, 8) Platform-specific configuration.
Channel types include: 1) MethodChannel for method invocation, 2) EventChannel for streaming data, 3) BasicMessageChannel for custom data, 4) BinaryMessenger for raw data, 5) JSONMessageCodec support, 6) StandardMessageCodec usage, 7) Custom codec implementation, 8) Platform-specific channel variations.
Permission handling includes: 1) Permission plugin integration, 2) Runtime permission requests, 3) Permission status checking, 4) Permission denial handling, 5) Platform-specific permission logic, 6) Permission group management, 7) Permission callback handling, 8) Permission persistence.
Native UI integration approaches: 1) Platform Views implementation, 2) Hybrid composition, 3) Virtual displays, 4) AndroidView and UiKitView, 5) Native widget embedding, 6) Performance optimization, 7) Lifecycle management, 8) Platform-specific styling.
Push notification implementation: 1) Firebase Cloud Messaging setup, 2) Platform-specific configuration, 3) Notification handling, 4) Background message processing, 5) Notification permissions, 6) Custom notification UI, 7) Deep link handling, 8) Token management.
Biometric authentication includes: 1) Local authentication plugin, 2) Platform-specific APIs, 3) Fingerprint authentication, 4) Face ID integration, 5) Error handling, 6) Fallback mechanisms, 7) Security level configuration, 8) Authentication state management.
File system access includes: 1) Platform-specific paths, 2) Storage permission handling, 3) File operations implementation, 4) Directory management, 5) External storage access, 6) File metadata handling, 7) Platform restrictions handling, 8) Error management.
Platform styling strategies: 1) Cupertino and Material widgets, 2) Platform-specific themes, 3) Adaptive widget usage, 4) Custom platform detection, 5) Platform-specific assets, 6) Dynamic styling, 7) Platform-specific layouts, 8) Theme inheritance.
Camera implementation includes: 1) Camera plugin integration, 2) Permission handling, 3) Camera preview setup, 4) Image capture handling, 5) Video recording, 6) Camera controls, 7) Platform-specific features, 8) Error handling.
Background processing approaches: 1) WorkManager integration, 2) Background fetch, 3) Isolate implementation, 4) Platform-specific services, 5) Background tasks scheduling, 6) Battery optimization, 7) State persistence, 8) Error recovery.
Platform gesture handling: 1) GestureDetector configuration, 2) Platform-specific recognition, 3) Custom gesture implementation, 4) Touch feedback, 5) Gesture conflict resolution, 6) Platform-specific animations, 7) Accessibility support, 8) Error handling.
Sensor handling approaches: 1) Sensor plugin integration, 2) Platform-specific APIs, 3) Data stream management, 4) Sensor calibration, 5) Battery optimization, 6) Error handling, 7) Permission management, 8) Platform compatibility.
Platform navigation includes: 1) Platform-specific gestures, 2) Navigation patterns, 3) Deep linking handling, 4) Custom transitions, 5) Back button behavior, 6) Navigation state management, 7) Platform-specific routes, 8) Error handling.
Storage strategies include: 1) Shared preferences, 2) Keychain/Keystore access, 3) Secure storage implementation, 4) Platform-specific APIs, 5) Data encryption, 6) Storage permissions, 7) Migration handling, 8) Error management.
Platform networking includes: 1) SSL/Certificate handling, 2) Network security configuration, 3) Platform-specific headers, 4) Proxy settings, 5) Network type detection, 6) Background transfer, 7) Download manager integration, 8) Platform-specific optimizations.
In-app purchase handling: 1) Platform-specific store integration, 2) Product configuration, 3) Purchase flow implementation, 4) Receipt verification, 5) Subscription management, 6) Restore purchases, 7) Error handling, 8) Transaction persistence.
Social media integration: 1) Platform-specific SDKs, 2) Authentication flows, 3) Share functionality, 4) Deep linking, 5) Profile data access, 6) Permission handling, 7) Platform compliance, 8) Error management.
Location services handling: 1) Permission management, 2) GPS integration, 3) Background location updates, 4) Geofencing implementation, 5) Battery optimization, 6) Location accuracy, 7) Platform-specific features, 8) Error handling.
Analytics implementation: 1) Platform-specific SDKs, 2) Event tracking, 3) User properties, 4) Custom dimensions, 5) Crash reporting, 6) Performance monitoring, 7) Offline data collection, 8) Privacy compliance.
Audio/video handling: 1) Codec support, 2) Platform-specific players, 3) Background playback, 4) Media controls, 5) DRM implementation, 6) Streaming optimization, 7) Platform restrictions, 8) Error handling.
Deep linking implementation: 1) URL scheme configuration, 2) Universal links setup, 3) App shortcuts definition, 4) Route handling, 5) State restoration, 6) Parameter parsing, 7) Platform-specific features, 8) Error management.
Platform testing approaches: 1) Integration testing, 2) Platform-specific mocks, 3) Device testing, 4) Feature verification, 5) Performance testing, 6) UI testing, 7) Security testing, 8) Automation setup.
Security feature handling: 1) Keychain/Keystore usage, 2) Biometric integration, 3) SSL pinning, 4) App signing, 5) Data encryption, 6) Secure storage, 7) Root/jailbreak detection, 8) Platform compliance.
Authentication approaches: 1) Biometric integration, 2) OAuth implementation, 3) SSO configuration, 4) Token management, 5) Secure storage, 6) Session handling, 7) Platform-specific flows, 8) Error handling.
Accessibility implementation: 1) Screen reader support, 2) Platform-specific semantics, 3) Focus handling, 4) Custom actions, 5) Navigation adaptation, 6) Color contrast, 7) Dynamic type support, 8) Testing tools.
Error handling strategies: 1) Platform-specific error codes, 2) Error mapping, 3) Recovery mechanisms, 4) User feedback, 5) Logging implementation, 6) Crash reporting, 7) Debug information, 8) Error analytics.
Performance monitoring: 1) Platform profiling tools, 2) Metric collection, 3) Performance triggers, 4) Memory monitoring, 5) Frame rate tracking, 6) Network monitoring, 7) Battery impact, 8) Report generation.
Debugging approaches: 1) Platform debugger integration, 2) Logging mechanisms, 3) Debug bridges, 4) Network inspection, 5) Memory analysis, 6) Performance profiling, 7) Crash analysis, 8) Debug builds configuration.
Learn about widgets, state management, and the Dart programming language.
Get hands-on experience with Provider, Riverpod, Bloc, and Redux.
Learn techniques to improve rendering, reduce jank, and handle large data efficiently.
Work on projects that include Firebase integration, animations, and responsive UI.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Flutter questions, mock interviews, and more to secure your dream role.
Start Preparing now