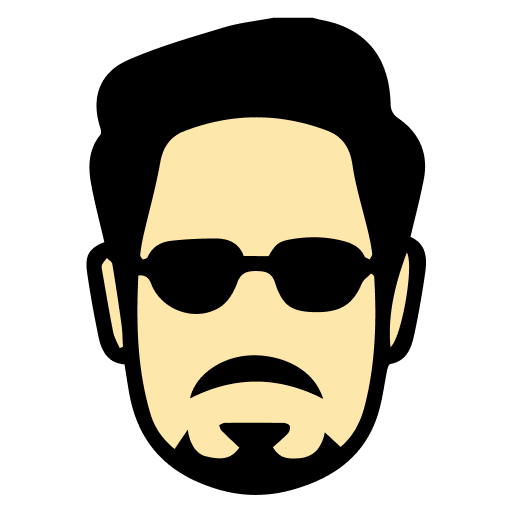
Next.js, a powerful React framework, is essential for building modern web applications with server-side rendering capabilities, making it a crucial skill for frontend and full-stack developers. Stark.ai offers a curated collection of Next.js interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Next.js is a React framework that provides features like server-side rendering, static site generation, file-based...
Create a Next.js project using 'npx create-next-app@latest' or 'yarn create next-app'. This sets up a new project...
Next.js project structure includes pages directory for routes, public for static assets, styles for CSS, components...
Core dependencies include React, React DOM, and Next.js itself. These are automatically included in package.json...
Default scripts include 'dev' for development server, 'build' for production build, 'start' for production server,...
Run development server using 'npm run dev' or 'yarn dev'. This starts the application with hot reloading at...
next.config.js is a configuration file for customizing Next.js behavior. It can configure webpack, environment...
Environment variables are handled using .env files (.env.local, .env.development, .env.production). Variables...
The pages directory uses the Pages Router, while app directory uses the newer App Router. App Router supports React...
TypeScript is supported out of the box. Create project with --typescript flag or add manually by creating...
Custom server configuration requires creating server.js file, implementing custom routing logic, and modifying...
Webpack configuration is customized in next.config.js using webpack function. Can modify loaders, plugins, and other...
CI/CD implementation includes setting up build pipelines, automated testing, deployment scripts. Configure with...
Configure path aliases in tsconfig.json or jsconfig.json using baseUrl and paths. Supports absolute imports and...
Build optimizations include code splitting, tree shaking, image optimization, and bundle analysis. Configure in...
Static files are served from public directory. Support automatic optimization for images. Configure caching headers...
Project architecture includes directory structure, code organization, module patterns. Consider scalability,...
Dependency management includes version control, package updates, security audits. Use package managers effectively....
Code quality tools include ESLint, Prettier, TypeScript. Configure linting rules. Implement pre-commit hooks. Handle...
Project documentation includes README, API docs, component documentation. Use tools like Storybook. Implement...
Advanced configuration includes custom babel config, module federation, plugin system. Handle complex build...
Monorepo setup includes workspace configuration, shared dependencies, build orchestration. Use tools like Turborepo....
Build plugins extend Next.js functionality. Create custom webpack plugins. Handle build process modifications....
Advanced optimization includes custom cache strategies, bundle optimization, resource prioritization. Handle...
Custom build processes include build scripts, asset pipeline, deployment automation. Handle build environments....
Project scalability includes architecture patterns, performance optimization, code organization. Handle growing...
Advanced testing includes test infrastructure, CI integration, test automation. Handle complex test scenarios....
Security configurations include CSP headers, security middleware, vulnerability scanning. Handle security policies....
Monitoring setup includes error tracking, performance monitoring, analytics integration. Handle monitoring tools....
Advanced deployment includes zero-downtime deployment, rollback strategies, deployment automation. Handle deployment...
App Router is newer, uses app directory, supports React Server Components, nested layouts, and improved routing...
In App Router, create routes by adding page.js files in app directory. In Pages Router, add files to pages...
Dynamic routes use square brackets for variable segments. Example: [id]/page.js creates dynamic route. Parameters...
Use Link component for client-side navigation. Example: <Link href='/about'>About</Link>. Provides automatic...
useRouter hook provides access to router object for programmatic navigation, route information, and parameters....
Create nested directories in app or pages folder. Each level can have its own page.js or layout.js. Supports nested...
Route groups use (groupName) syntax in app directory. Don't affect URL structure. Share layouts within groups....
Create not-found.js in app directory or 404.js in pages directory. Automatically shown for non-existent routes. Can...
Parallel routing allows simultaneous loading of multiple pages in same layout using @folder convention. Supports...
Access route parameters through params prop in page components or searchParams for query strings. Available in both...
Route interceptors use (.) convention in App Router. Intercept routes while preserving context. Handle overlays and...
Create loading.js files for automatic loading UI. Support Suspense boundaries. Handle streaming and progressive...
Create middleware.ts in project root. Handle route protection, redirects, headers. Support conditional execution....
Implement custom transition effects using hooks and components. Handle loading states. Support animation libraries....
Use middleware or page components for authentication checks. Handle redirects. Support role-based access. Implement...
Control prefetching with prefetch prop on Link component. Implement custom prefetch logic. Handle data preloading....
Configure rewrites in next.config.js. Handle URL transformation. Support pattern matching. Implement rewrite conditions.
Configure redirects in next.config.js or use redirect helper. Handle permanent/temporary redirects. Support redirect...
Optimize route performance through code splitting, prefetching, caching. Handle route priorities. Implement...
Implement i18n routing using middleware and configuration. Handle language detection. Support URL patterns....
Create complex dynamic routes with optional catches, multiple parameters. Handle route constraints. Support pattern...
Implement state persistence across route changes. Handle navigation state. Support history management. Implement...
Create custom caching for route data. Handle cache invalidation. Support dynamic caching. Implement cache strategies.
Implement nested and parallel layouts. Handle layout groups. Support template inheritance. Implement layout patterns.
Create error boundaries for route segments. Handle error recovery. Support fallback content. Implement error reporting.
Implement advanced code splitting strategies. Handle dynamic imports. Support chunk optimization. Implement loading...
Track route navigation and performance metrics. Handle analytics integration. Support custom events. Implement...
Implement comprehensive route testing. Handle navigation testing. Support integration tests. Implement test utilities.
Create detailed route documentation. Generate API documentation. Support example usage. Implement documentation updates.
Next.js 13+ provides async Server Components, fetch() with caching options, server actions, and client-side fetching...
Use async/await directly in Server Components. Example: async function Page() { const data = await...
Static data fetching occurs at build time using fetch with cache: 'force-cache' option. Data is cached and reused...
Use fetch with cache: 'no-store' option or revalidate: 0 for dynamic data. Data fetched on every request. Suitable...
ISR allows static pages to be updated after build time. Use fetch with revalidate option. Combines benefits of...
Use hooks like useState and useEffect in Client Components, or data fetching libraries like SWR or React Query....
Server Actions allow form handling and data mutations directly from Server Components. Use 'use server' directive....
Use fetch cache options or React cache function. Configure cache behavior in fetch requests. Support cache...
Fetch multiple data sources simultaneously using Promise.all or parallel routes. Improve performance by avoiding...
Create loading.js files for automatic loading UI. Use Suspense boundaries. Support streaming and progressive...
Handle sequential data fetching where each request depends on previous results. Manage dependencies between...
Implement on-demand revalidation using revalidatePath or revalidateTag. Handle time-based revalidation. Support...
Implement request deduplication, caching strategies, parallel fetching. Handle data preloading. Support prefetching....
Create error.js files for error handling. Implement fallback content. Support error recovery. Handle error...
Use Server Actions or API routes for data mutations. Handle optimistic updates. Support rollback mechanisms....
Implement WebSocket connections or server-sent events. Handle real-time updates. Support data synchronization....
Validate data on server and client side. Handle validation errors. Support schema validation. Implement validation...
Implement client-side storage strategies. Handle offline support. Support data synchronization. Implement...
Use prefetch methods or preload data during build. Handle route prefetching. Support data preloading. Implement...
Implement secure data fetching patterns. Handle authentication/authorization. Support data encryption. Implement...
Create custom caching logic. Handle cache invalidation patterns. Support distributed caching. Implement cache management.
Manage nested data structures. Handle circular references. Support data normalization. Implement relationship patterns.
Handle multi-client data sync. Implement conflict resolution. Support offline-first patterns. Manage sync state.
Implement data structure changes. Handle version migrations. Support data transformation. Manage migration state.
Track data fetching performance. Handle analytics integration. Support debugging tools. Implement monitoring strategies.
Implement scalable fetching patterns. Handle large datasets. Support pagination strategies. Implement performance...
Create comprehensive test suites. Handle mock data. Support integration testing. Implement test utilities.
Implement end-to-end encryption. Handle key management. Support secure transmission. Implement encryption strategies.
Handle API versioning. Support backwards compatibility. Implement version migration. Manage version state.
Create comprehensive API documentation. Generate type definitions. Support example usage. Implement documentation updates.
SSR generates HTML on the server for each request. Provides better initial page load and SEO. Next.js handles SSR...
Server Components run only on server, reduce client-side JavaScript. Support async data fetching. Cannot use...
Hydration attaches JavaScript functionality to server-rendered HTML. Happens automatically after initial page load....
Streaming SSR progressively sends HTML chunks as they're generated. Uses <Suspense> boundaries. Improves Time To...
Use async Server Components or getServerSideProps. Data available during render. Support server-only operations....
Better SEO, faster initial page load, improved performance on slow devices. Support social media previews. Handle...
Use 'use server' directive or .server.js files. Handle sensitive operations. Access server-side APIs. Support secure...
SSR cache stores rendered pages on server. Improves performance for subsequent requests. Supports cache...
Use error.js files for error boundaries. Handle server-side errors. Support fallback content. Implement error reporting.
SSR generates HTML per request, SSG at build time. SSR better for dynamic content, SSG for static. Trade-off between...
Use streaming, caching, code splitting. Handle resource optimization. Support performance monitoring. Implement...
Implement server-side authentication checks. Handle protected routes. Support session management. Implement auth strategies.
Create middleware for request processing. Handle request transformation. Support middleware chain. Implement custom logic.
Handle library compatibility issues. Support SSR-specific configurations. Implement integration strategies. Manage...
Set custom headers for SSR responses. Handle caching headers. Support security headers. Implement header management.
Implement server-side state initialization. Handle state hydration. Support state serialization. Implement state strategies.
Handle server-side translations. Support locale detection. Implement translation loading. Manage language switching.
Track SSR metrics and performance. Handle monitoring integration. Support debugging tools. Implement monitoring strategies.
Create server-side rendering tests. Handle integration testing. Support unit testing. Implement test utilities.
Implement advanced caching patterns. Handle cache invalidation. Support distributed caching. Implement cache management.
Create comprehensive error handling. Support error recovery. Handle error boundaries. Implement error reporting.
Implement real-time features with SSR. Handle connection management. Support event handling. Implement WebSocket strategies.
Handle server-side security concerns. Implement XSS protection. Support CSRF prevention. Implement security strategies.
Implement SSR in microservice architecture. Handle service communication. Support service discovery. Implement...
Create detailed performance tracking. Handle metric collection. Support debugging tools. Implement monitoring solutions.
Implement server-side GraphQL operations. Handle query execution. Support data fetching. Implement GraphQL integration.
Create advanced optimization strategies. Handle resource optimization. Support performance patterns. Implement...
Implement deployment strategies for SSR. Handle environment configuration. Support scaling solutions. Implement...
Create comprehensive SSR documentation. Generate API documentation. Support example implementations. Implement...
SSG generates HTML at build time instead of runtime. Pages are pre-rendered and can be served from CDN. Provides...
Use generateStaticParams for App Router or getStaticProps/getStaticPaths for Pages Router. Pages are built at build...
Define dynamic paths using generateStaticParams or getStaticPaths. Specify which paths to pre-render. Support...
Fallback controls behavior for non-generated paths. Options: false (404), true (loading), or 'blocking' (SSR)....
Fetch data during build using async components or getStaticProps. Data available at build time. Support external...
Fastest page loads, better SEO, reduced server load, improved security. Pages can be served from CDN. Support global...
Implement path validation during build. Handle invalid paths. Support custom validation. Implement error handling...
Data fetched during npm run build. Available for static page generation. Support external data sources. Handle...
Place assets in public directory. Support automatic optimization. Handle asset references. Implement asset...
Set revalidate time in fetch options. Handle on-demand revalidation. Support revalidation triggers. Implement...
Implement pagination or chunking. Handle build performance. Support incremental builds. Implement data management strategies.
Implement build optimization strategies. Handle parallel generation. Support incremental builds. Implement caching...
Use client-side fetching for dynamic data. Implement hybrid approaches. Support progressive enhancement. Handle...
Enable preview mode for draft content. Handle authentication. Support preview routes. Implement preview strategies.
Implement error handling during build. Support fallback content. Handle partial builds. Implement error reporting.
Use build cache for faster builds. Handle cache invalidation. Support incremental builds. Implement cache strategies.
Generate static pages for multiple locales. Handle locale routing. Support translation loading. Implement i18n strategies.
Track build metrics and performance. Handle build analytics. Support debugging tools. Implement monitoring strategies.
Build only changed pages. Handle dependency tracking. Support incremental builds. Implement build optimization.
Manage nested data relationships. Handle circular dependencies. Support data validation. Implement dependency resolution.
Create custom build workflows. Handle build stages. Support parallel processing. Implement pipeline optimization.
Implement distributed build system. Handle build coordination. Support build synchronization. Implement distribution...
Implement advanced optimization techniques. Handle resource optimization. Support parallel processing. Implement...
Create build performance analysis. Handle metrics collection. Support debugging tools. Implement analysis strategies.
Implement secure build processes. Handle sensitive data. Support security scanning. Implement security measures.
Create automated build workflows. Handle CI/CD integration. Support automated testing. Implement automation strategies.
Create comprehensive build documentation. Generate build reports. Support example configurations. Implement...
API Routes are serverless endpoints built into Next.js. Created in pages/api directory (Pages Router) or app/api...
Create a file in app/api directory that exports default async function. Handle request methods (GET, POST, etc.)....
Export functions named after HTTP methods (GET, POST, PUT, DELETE). Or use conditional logic in Pages Router....
Access query params through request.nextUrl.searchParams in App Router or req.query in Pages Router. Parse and...
Access request body using await request.json() or similar methods. Validate request data. Process POST data. Return...
Use square brackets for dynamic segments [param]. Access parameters through route object. Support multiple dynamic...
Return appropriate status codes and error messages. Use try-catch blocks. Implement error handling middleware....
Configure CORS headers using middleware or within route handlers. Set Access-Control-Allow-Origin and other headers....
Middleware processes requests before reaching route handlers. Handle authentication, logging, CORS. Support...
Process multipart/form-data using appropriate middleware. Handle file storage. Validate file types and sizes....
Implement rate limiting middleware. Track request counts. Set rate limits. Handle limit exceeded responses. Support...
Implement authentication middleware. Verify tokens or credentials. Handle protected routes. Support different auth...
Cache API responses. Handle cache invalidation. Set cache headers. Implement caching strategies. Support different...
Validate request data using schemas or validation libraries. Handle validation errors. Return appropriate error...
Log API requests and responses. Track performance metrics. Handle error logging. Implement logging strategies....
Implement version control in API routes. Handle backwards compatibility. Support multiple versions. Implement...
Enable response compression middleware. Handle different compression types. Set appropriate headers. Implement...
Generate API documentation. Implement OpenAPI/Swagger. Support documentation updates. Implement documentation strategies.
Track API performance and usage. Handle monitoring integration. Support analytics. Implement monitoring strategies.
Create custom error classes. Handle different error types. Support error reporting. Implement error handling...
Implement stream handling for large responses. Handle chunked transfer. Support progressive loading. Implement...
Implement security best practices. Handle XSS/CSRF protection. Support security headers. Implement security strategies.
Integrate GraphQL server with API routes. Handle schema definition. Support resolvers. Implement GraphQL middleware.
Handle WebSocket connections in API routes. Manage socket state. Support real-time communication. Implement...
Implement transaction management in API routes. Handle rollbacks. Support nested transactions. Implement transaction...
Create comprehensive API tests. Handle integration testing. Support unit testing. Implement test strategies. Manage...
Implement performance improvements. Handle request optimization. Support response optimization. Implement...
Track API usage patterns. Handle analytics integration. Support custom metrics. Implement analytics strategies.
Implement deployment strategies for API routes. Handle environment configuration. Support scaling solutions....
Pages are special components that become routes automatically when placed in pages/ or app/ directory. Components...
Server Components are rendered on server by default in App Router. Cannot use browser APIs or React hooks. Better...
Client Components use 'use client' directive. Can use browser APIs and React hooks. Enable interactive features. Run...
Create layout.js file in app directory. Wraps child pages/components. Shares UI across routes. Supports nested...
Hydration attaches JavaScript event handlers to server-rendered HTML. Makes static content interactive. Happens...
Use metadata object or generateMetadata function in page files. Set title, description, open graph data. Support...
Create error.js files for error boundaries. Handle component errors. Support fallback content. Implement error...
Use [param] syntax for dynamic routes. Access parameters through props. Support multiple segments. Handle parameter...
Use React Context, state management libraries, or lift state up. Handle component communication. Support state...
Use dynamic imports, route-based splitting, component-based splitting. Handle lazy loading. Support chunk...
Implement performance optimizations. Use React.memo, useMemo, useCallback. Support component caching. Implement...
Create reusable component patterns. Handle component hierarchy. Support component inheritance. Implement composition...
Use CSS Modules, styled-components, or other styling solutions. Handle dynamic styles. Support theme systems....
Create unit tests, integration tests. Handle component rendering. Support interaction testing. Implement test strategies.
Implement form handling logic. Handle validation. Support form submission. Implement form state management. Handle...
Create reusable component collections. Handle component documentation. Support theming. Implement component versioning.
Implement animation libraries or CSS transitions. Handle animation states. Support transition effects. Implement...
Follow ARIA standards. Handle keyboard navigation. Support screen readers. Implement accessibility patterns.
Create compound components, render props, higher-order components. Handle complex patterns. Support pattern composition.
Track render performance. Handle performance metrics. Support profiling tools. Implement monitoring strategies.
Handle XSS prevention, input sanitization. Support content security policies. Implement security measures.
Implement i18n support. Handle translations. Support RTL layouts. Implement localization strategies.
Create error boundary components. Handle error recovery. Support fallback UI. Implement error reporting.
Implement state management solutions. Handle global state. Support state persistence. Implement state patterns.
Create component documentation. Generate API docs. Support example usage. Implement documentation strategies.
Implement semantic versioning. Handle backwards compatibility. Support version migration. Implement versioning strategies.
Design scalable component systems. Handle component organization. Support architecture patterns. Implement design systems.
Implement deployment strategies. Handle build optimization. Support continuous integration. Implement deployment patterns.
Layout components are created using layout.js files. Share UI between pages. Support nested layouts. Handle...
Use .module.css files for component-scoped CSS. Import styles as objects. Support local class naming. Automatic...
Import global CSS in app/layout.js or pages/_app.js. Apply styles across all components. Support reset styles and...
Use media queries, CSS Grid, Flexbox. Support mobile-first design. Handle breakpoints. Implement responsive...
Create multiple layout.js files in route segments. Support layout hierarchy. Share UI between related routes. Handle...
Install and configure Tailwind CSS. Use utility classes. Support JIT mode. Handle Tailwind configuration. Implement...
Use (group) folders to organize routes. Don't affect URL structure. Share layouts within groups. Support multiple groups.
Use CSS-in-JS solutions or dynamic class names. Support runtime styles. Handle style variables. Implement dynamic theming.
Support styled-components, Emotion, and other CSS-in-JS libraries. Handle server-side rendering. Support dynamic...
Implement page and layout transitions. Support animation effects. Handle transition states. Implement smooth...
Create theme providers and consumers. Handle theme switching. Support dark mode. Implement theme configuration....
Implement CSS minification, purging, and bundling. Handle critical CSS. Support CSS splitting. Implement...
Create responsive grid layouts. Handle grid areas. Support grid templates. Implement grid components. Manage grid...
Optimize runtime performance. Handle style extraction. Support server-side generation. Implement performance strategies.
Use Next.js Image component. Handle srcset and sizes. Support art direction. Implement image optimization. Manage...
Implement CSS custom properties. Handle variable scoping. Support dynamic values. Implement theme systems. Manage...
Create reusable layout components. Handle layout composition. Support layout variations. Implement common patterns.
Implement CSS transitions and keyframes. Handle animation states. Support animation libraries. Implement animation patterns.
Create visual regression tests. Handle style snapshots. Support style assertions. Implement testing strategies.
Create complex layout systems. Handle dynamic layouts. Support layout algorithms. Implement advanced patterns.
Implement CSS methodology (BEM, SMACSS). Handle style organization. Support scalable systems. Implement architecture...
Create comprehensive design systems. Handle component libraries. Support theme configuration. Implement system documentation.
Track CSS performance metrics. Handle style analysis. Support optimization tools. Implement monitoring strategies.
Create custom layout algorithms. Handle complex arrangements. Support dynamic positioning. Implement algorithm optimization.
Implement complex animation sequences. Handle animation orchestration. Support performance optimization. Implement...
Handle large-scale CSS module systems. Support composition patterns. Implement naming conventions. Handle module...
Implement CSS sanitization. Handle style injection prevention. Support content security policies. Implement security...
Create comprehensive style guides. Generate documentation. Support example usage. Implement documentation updates.
Implement layout performance improvements. Handle reflow optimization. Support layout calculation. Implement...
Next.js supports multiple state management options: React's built-in useState and useContext, external libraries...
Use useState hook for component-level state. Handle state updates. Support state initialization. Implement local...
Context API shares state between components without prop drilling. Create providers and consumers. Handle context...
Configure Redux store with Next.js. Handle server-side state hydration. Support Redux middleware. Implement Redux...
SWR is a React hooks library for data fetching. Handles caching, revalidation, and real-time updates. Support...
Use form libraries like React Hook Form or Formik. Handle form validation. Support form submission. Implement form...
Store state in localStorage or sessionStorage. Handle state recovery. Support persistence strategies. Implement...
Use query parameters and URL segments for state. Handle URL updates. Support navigation state. Implement URL-based...
Initialize state during server-side rendering. Handle state hydration. Support initial data loading. Implement SSR...
Manage loading, error, and success states. Handle async operations. Support state transitions. Implement async...
Use state machine libraries like XState. Handle state transitions. Support finite states. Implement state machine patterns.
Implement WebSocket or Server-Sent Events. Handle real-time updates. Support state synchronization. Implement...
Create middleware for state operations. Handle state transformations. Support middleware chain. Implement middleware...
Implement state version control. Handle state migrations. Support backwards compatibility. Implement versioning patterns.
Create selectors for state derivation. Handle memoization. Support selector composition. Implement selector patterns.
Implement state validation rules. Handle validation errors. Support schema validation. Implement validation patterns.
Create state change logs. Handle state debugging. Support logging middleware. Implement logging patterns.
Implement state sync between clients. Handle conflict resolution. Support offline state. Implement sync patterns.
Create state management tests. Handle state assertions. Support integration testing. Implement test patterns.
Create complex state management solutions. Handle state composition. Support state hierarchies. Implement advanced patterns.
Optimize state updates and access. Handle state normalization. Support performance monitoring. Implement...
Design scalable state systems. Handle state organization. Support architecture patterns. Implement design principles.
Implement state data encryption. Handle key management. Support secure storage. Implement security measures.
Track state changes and performance. Handle monitoring integration. Support analytics. Implement monitoring strategies.
Implement state structure changes. Handle version migrations. Support data transformation. Implement migration strategies.
Create comprehensive state documentation. Generate API docs. Support example usage. Implement documentation updates.
Implement scalable state solutions. Handle large state trees. Support performance optimization. Implement scaling strategies.
Create debugging tools and utilities. Handle state inspection. Support troubleshooting. Implement debugging strategies.
Implement state access control. Handle authentication/authorization. Support security policies. Implement security measures.
Next.js Image component is an extension of HTML <img> tag. Provides automatic image optimization. Supports lazy...
Import Image from 'next/image'. Add src, alt, width, and height props. Component optimizes and serves images...
Reduces image file size, improves page load speed, supports responsive images, optimizes for different devices,...
Images load only when they enter viewport. Reduces initial page load time. Uses loading='lazy' attribute...
Images adapt to different screen sizes. Uses sizes prop for viewport-based sizing. Supports srcset generation....
Use quality prop to set compression level. Default is 75. Balance between quality and file size. Support different...
Shows blurred version of image while loading. Use placeholder='blur' prop. Supports blurDataURL for custom blur....
Configure domains in next.config.js. Use loader prop for custom image service. Support remote image optimization....
Import images as modules. Get width and height automatically. Support webpack optimization. Handle static image assets.
Support WebP, AVIF formats. Use formats prop for specific formats. Handle browser compatibility. Implement format strategies.
Use different images for different screen sizes. Handle responsive images. Support art direction patterns. Implement...
Use priority prop for LCP images. Handle critical images. Support preload strategies. Implement performance optimization.
Create custom image loaders. Handle external image services. Support custom optimization. Implement loader strategies.
Configure cache headers. Handle browser caching. Support CDN caching. Implement caching strategies.
Handle SVG optimization. Support inline SVGs. Handle SVG sprites. Implement SVG optimization strategies.
Implement alt text. Handle role attributes. Support screen readers. Implement accessibility patterns.
Handle loading errors. Support fallback images. Implement error states. Handle error reporting.
Load images dynamically. Handle dynamic sources. Support runtime optimization. Implement dynamic patterns.
Create image component tests. Handle visual testing. Support integration testing. Implement test patterns.
Monitor loading metrics. Handle performance optimization. Support performance tracking. Implement loading strategies.
Create complex optimization strategies. Handle different use cases. Support optimization patterns. Implement...
Implement batch processing. Handle multiple images. Support processing queues. Implement scaling strategies.
Create image transformations. Handle resizing, cropping. Support filters and effects. Implement transformation pipelines.
Implement secure image handling. Handle access control. Support secure URLs. Implement security measures.
Track image performance metrics. Handle monitoring integration. Support analytics. Implement monitoring strategies.
Implement deployment strategies. Handle CDN integration. Support caching systems. Implement deployment patterns.
Create image component documentation. Generate API docs. Support example usage. Implement documentation updates.
Test optimization performance. Handle metric validation. Support automation testing. Implement test strategies.
Design scalable image systems. Handle architecture patterns. Support system organization. Implement design principles.
Next.js supports multiple authentication methods: JWT, session-based, OAuth providers, NextAuth.js library. Can...
NextAuth.js is a complete authentication solution for Next.js applications. Provides built-in support for multiple...
Store JWT in HTTP-only cookies or local storage. Implement token verification. Handle token expiration. Support...
Store session data on server. Use session cookies for client identification. Handle session expiration. Support...
Configure OAuth providers. Handle OAuth flow. Support callback URLs. Implement user profile retrieval. Manage OAuth tokens.
Implement role checking middleware. Define user roles. Handle permission checks. Support role hierarchies. Implement...
Implement authentication middleware. Verify tokens or sessions. Handle unauthorized requests. Support API security....
Implement CSRF tokens. Handle token validation. Support form submissions. Implement security headers. Prevent...
Manage user authentication state. Handle state persistence. Support state updates. Implement state management....
Use bcrypt or similar libraries. Handle password storage. Support password validation. Implement secure hashing....
Implement token refresh logic. Handle token rotation. Support silent refresh. Implement refresh strategies. Manage...
Configure social providers. Handle OAuth integration. Support user profile mapping. Implement login flow. Manage...
Implement granular permissions. Handle permission checks. Support permission groups. Implement access control lists....
Support 2FA methods. Handle verification codes. Implement backup codes. Support authentication apps. Manage 2FA setup.
Implement error handling. Support error messages. Handle recovery flows. Implement error logging. Manage error states.
Handle reset flow. Support reset tokens. Implement email notifications. Handle token expiration. Manage reset process.
Implement session storage. Handle session cleanup. Support session validation. Implement session strategies. Manage...
Create authentication tests. Handle test scenarios. Support integration testing. Implement test strategies. Manage...
Track authentication metrics. Handle monitoring integration. Support analytics. Implement monitoring strategies....
Handle authorization code flow. Support refresh tokens. Implement token exchange. Handle scopes. Manage OAuth state.
Implement secure token storage. Handle token encryption. Support token validation. Implement security measures....
Handle tenant isolation. Support tenant authentication. Implement tenant routing. Handle tenant data. Manage tenant access.
Implement distributed session management. Handle cross-domain auth. Support SSO integration. Implement auth strategies.
Track authentication events. Handle audit trail. Support compliance requirements. Implement logging strategies....
Implement security standards. Handle data privacy. Support regulatory compliance. Implement compliance measures....
Design scalable auth systems. Handle system organization. Support architecture patterns. Implement design principles.
Optimize authentication flow. Handle caching strategies. Support performance monitoring. Implement optimization techniques.
Create security test suites. Handle penetration testing. Support vulnerability scanning. Implement security measures.
Implement deployment strategies. Handle environment configuration. Support scaling solutions. Implement deployment patterns.
Core Web Vitals are key metrics measuring user experience: LCP (Largest Contentful Paint), FID (First Input Delay),...
Code splitting automatically splits JavaScript bundles by route. Reduces initial bundle size. Supports dynamic...
Next.js Image component automatically optimizes images. Supports lazy loading, responsive sizes, modern formats....
Next.js automatically determines which pages can be statically generated. Improves page load performance. Supports...
Next.js Script component optimizes third-party script loading. Supports loading strategies (defer, lazy). Prevents...
Next.js automatically prefetches links in viewport. Reduces page load time. Supports custom prefetch behavior. Uses...
Next.js automatically optimizes font loading. Reduces layout shift. Supports CSS size-adjust. Implements font...
Next.js supports multiple caching strategies: build-time cache, server-side cache, client-side cache. Improves...
Use dynamic imports, React.lazy(), and Suspense. Handle component loading states. Support code splitting. Implement...
Use @next/bundle-analyzer, analyze bundle size, identify large dependencies. Support code splitting analysis....
Implement memo, useMemo, useCallback hooks. Handle component optimization. Support render optimization. Implement...
Use Web Vitals API, implement analytics, track performance metrics. Support performance tracking. Implement...
Use CSS Modules, implement critical CSS, handle CSS-in-JS optimization. Support style optimization. Implement CSS strategies.
Implement resource hints, handle preload/prefetch, optimize loading order. Support priority strategies. Implement...
Configure server push, handle multiplexing, optimize request prioritization. Support HTTP/2 features. Implement...
Configure CDN caching, handle asset distribution, optimize edge caching. Support CDN strategies. Implement delivery...
Implement load testing, measure performance metrics, use Lighthouse scores. Support performance benchmarking....
Implement mobile-first optimization, handle responsive optimization, optimize touch interactions. Support mobile...
Set performance targets, monitor metrics, implement budget tracking. Support performance goals. Implement budget strategies.
Implement WebAssembly modules, handle integration, optimize performance. Support WASM strategies. Implement...
Configure service workers, handle offline support, implement caching strategies. Support PWA features. Implement...
Implement memory management, handle memory leaks, optimize resource usage. Support memory monitoring. Implement...
Handle 3D rendering optimization, implement WebGL best practices, optimize graphics performance. Support graphics...
Create custom monitoring solutions, handle metric collection, implement analytics integration. Support monitoring...
Create performance guidelines, document optimization strategies, implement documentation updates. Support best...
Implement build optimization, handle CI/CD performance, optimize deployment process. Support pipeline strategies....
Middleware runs before request is completed. Enables custom code execution between request and response. Can modify...
next.config.js is used for custom Next.js configuration. Supports various options like rewrites, redirects,...
Environment variables configured in .env files. Support different environments (.env.local, .env.production). Access...
Configure redirects in next.config.js using redirects array. Support permanent/temporary redirects. Handle path...
Rewrites allow URL mapping without path change. Configured in next.config.js. Support external rewrites. Handle path...
Add custom headers using headers in next.config.js. Support security headers, CORS headers. Handle header...
Matcher defines paths where middleware runs. Uses path matching patterns. Support multiple matchers. Handle path...
Customize webpack config in next.config.js. Modify loaders, plugins, optimization settings. Support module...
basePath sets base URL path for application. Useful for sub-path deployments. Handle path prefixing. Support path...
Configure allowed image domains in next.config.js. Support external image optimization. Handle domain whitelist....
Create middleware functions. Handle request processing. Support middleware chain. Implement custom logic. Manage...
Implement error handling in middleware. Support error recovery. Handle error responses. Implement logging. Manage...
Optimize build settings in next.config.js. Handle bundling options. Support optimization flags. Implement build strategies.
Compose multiple middleware functions. Handle execution order. Support middleware chaining. Implement composition patterns.
Validate configuration settings. Handle validation errors. Support schema validation. Implement validation strategies.
Create middleware tests. Handle test scenarios. Support integration testing. Implement test strategies.
Handle module aliases. Support path mapping. Configure module imports. Implement resolution strategies.
Manage middleware state. Handle state persistence. Support state sharing. Implement state patterns.
Handle response compression. Support compression options. Configure compression settings. Implement optimization strategies.
Configure CORS settings. Handle cross-origin requests. Support CORS headers. Implement security policies.
Create complex middleware solutions. Handle advanced scenarios. Support pattern composition. Implement advanced strategies.
Manage multiple configurations. Handle environment-specific settings. Support configuration versioning. Implement...
Create security-focused middleware. Handle security measures. Support security policies. Implement protection strategies.
Create logging solutions. Handle log management. Support log analysis. Implement logging strategies.
Scale configuration solutions. Handle large-scale settings. Support scaling strategies. Implement scaling patterns.
Create middleware documentation. Generate config docs. Support example usage. Implement documentation updates.
Configure deployment settings. Handle environment setup. Support deployment strategies. Implement deployment patterns.
Create comprehensive test suites. Handle test scenarios. Support testing patterns. Implement test strategies.
Jest and React Testing Library are recommended for unit and integration testing. Cypress or Playwright for...
Configure jest.config.js for Next.js. Set up environment and transforms. Handle module mocking. Support TypeScript...
Use React Testing Library to render pages. Test page components. Handle data fetching. Support routing tests. Test...
Use Chrome DevTools or VS Code debugger. Set breakpoints. Inspect component state. Handle error tracing. Support source maps.
Mock HTTP requests. Test API endpoints. Handle response validation. Support API testing. Implement test scenarios.
Captures component output. Compares against stored snapshots. Detects UI changes. Support snapshot updates. Handle...
Use Node.js debugger. Handle server breakpoints. Inspect server state. Support server-side debugging. Implement logging.
Test individual components/functions. Handle isolated testing. Support test coverage. Implement unit test cases....
Create test fixtures. Handle mock data. Support test databases. Implement data generation. Handle test state.
Mock fetch requests. Handle async testing. Support data mocking. Implement fetch testing. Handle response simulation.
Test component interactions. Handle feature testing. Support workflow testing. Implement integration scenarios....
Use React DevTools Profiler. Handle performance monitoring. Support optimization. Implement performance debugging....
Mock authentication state. Handle protected routes. Support auth testing. Implement auth scenarios. Handle user sessions.
Configure coverage reporting. Handle coverage goals. Support coverage analysis. Implement coverage tracking. Handle...
Create middleware tests. Handle request processing. Support middleware testing. Implement test scenarios. Handle...
Use Cypress or Playwright. Handle user flows. Support browser testing. Implement test scenarios. Handle end-to-end workflows.
Handle route debugging. Support navigation testing. Implement route testing. Handle path resolution. Debug routing logic.
Test error scenarios. Handle error boundaries. Support error testing. Implement error cases. Handle error recovery.
Test state changes. Handle state updates. Support state testing. Implement state scenarios. Handle state logic.
Create complex test scenarios. Handle advanced cases. Support pattern testing. Implement testing strategies. Handle...
Implement CI/CD testing. Handle automated tests. Support test pipelines. Implement automation strategies. Handle...
Test performance metrics. Handle load testing. Support stress testing. Implement performance scenarios. Handle...
Implement security tests. Handle vulnerability testing. Support penetration testing. Implement security scenarios....
Track test execution. Handle test analytics. Support monitoring tools. Implement monitoring strategies. Handle test metrics.
Create test documentation. Handle documentation updates. Support example tests. Implement documentation strategies....
Use visual regression testing. Handle UI testing. Support screenshot testing. Implement visual validation. Handle...
Configure test environments. Handle environment setup. Support environment isolation. Implement environment...
Set up continuous testing. Handle automated runs. Support test pipelines. Implement continuous strategies. Handle...
Maintain test suites. Handle test updates. Support test refactoring. Implement maintenance strategies. Handle test debt.
Next.js is a React framework that provides features like server-side rendering, static site generation, file-based routing, API routes, and built-in optimizations. It simplifies building production-ready React applications with improved performance and SEO capabilities.
Create a Next.js project using 'npx create-next-app@latest' or 'yarn create next-app'. This sets up a new project with default configuration including TypeScript support, ESLint, and basic project structure.
Next.js project structure includes pages directory for routes, public for static assets, styles for CSS, components for reusable UI components, and next.config.js for configuration. The app directory is used for the App Router in newer versions.
Core dependencies include React, React DOM, and Next.js itself. These are automatically included in package.json when creating a new project. Additional dependencies can be added based on project requirements.
Default scripts include 'dev' for development server, 'build' for production build, 'start' for production server, and 'lint' for linting. These are defined in package.json and can be run using npm or yarn.
Run development server using 'npm run dev' or 'yarn dev'. This starts the application with hot reloading at localhost:3000 by default. Changes are automatically reflected without manual refresh.
next.config.js is a configuration file for customizing Next.js behavior. It can configure webpack, environment variables, redirects, rewrites, image optimization, and other build-time features.
Environment variables are handled using .env files (.env.local, .env.development, .env.production). Variables prefixed with NEXT_PUBLIC_ are exposed to the browser. Others are only available server-side.
The pages directory uses the Pages Router, while app directory uses the newer App Router. App Router supports React Server Components, nested layouts, and improved routing patterns by default.
TypeScript is supported out of the box. Create project with --typescript flag or add manually by creating tsconfig.json and installing typescript and @types/react. Next.js automatically detects and configures TypeScript.
Custom server configuration requires creating server.js file, implementing custom routing logic, and modifying package.json scripts. Supports Express or other Node.js server frameworks. Affects features like automatic static optimization.
Webpack configuration is customized in next.config.js using webpack function. Can modify loaders, plugins, and other webpack options. Supports extending default configuration while maintaining Next.js optimizations.
CI/CD implementation includes setting up build pipelines, automated testing, deployment scripts. Configure with services like GitHub Actions, Jenkins, or CircleCI. Handle environment variables and build caching.
Configure path aliases in tsconfig.json or jsconfig.json using baseUrl and paths. Supports absolute imports and custom module resolution. Improves code organization and maintainability.
Build optimizations include code splitting, tree shaking, image optimization, and bundle analysis. Configure in next.config.js. Use build plugins and optimization features. Monitor build performance.
Static files are served from public directory. Support automatic optimization for images. Configure caching headers and CDN options. Handle different file types appropriately.
Project architecture includes directory structure, code organization, module patterns. Consider scalability, maintainability. Implement feature-based or domain-driven design. Handle shared code.
Dependency management includes version control, package updates, security audits. Use package managers effectively. Handle peer dependencies. Implement dependency optimization.
Code quality tools include ESLint, Prettier, TypeScript. Configure linting rules. Implement pre-commit hooks. Handle code formatting. Support team coding standards.
Project documentation includes README, API docs, component documentation. Use tools like Storybook. Implement documentation generation. Maintain documentation updates.
Advanced configuration includes custom babel config, module federation, plugin system. Handle complex build scenarios. Implement configuration management. Support extensibility.
Monorepo setup includes workspace configuration, shared dependencies, build orchestration. Use tools like Turborepo. Handle package management. Implement shared configurations.
Build plugins extend Next.js functionality. Create custom webpack plugins. Handle build process modifications. Implement plugin architecture. Support plugin configuration.
Advanced optimization includes custom cache strategies, bundle optimization, resource prioritization. Handle performance bottlenecks. Implement optimization pipelines.
Custom build processes include build scripts, asset pipeline, deployment automation. Handle build environments. Implement build orchestration. Support custom builds.
Project scalability includes architecture patterns, performance optimization, code organization. Handle growing codebases. Implement scalable patterns. Support team collaboration.
Advanced testing includes test infrastructure, CI integration, test automation. Handle complex test scenarios. Implement test strategies. Support comprehensive testing.
Security configurations include CSP headers, security middleware, vulnerability scanning. Handle security policies. Implement security measures. Support secure deployment.
Monitoring setup includes error tracking, performance monitoring, analytics integration. Handle monitoring tools. Implement logging strategies. Support monitoring dashboards.
Advanced deployment includes zero-downtime deployment, rollback strategies, deployment automation. Handle deployment environments. Implement deployment pipelines. Support deployment monitoring.
Next.js 13+ provides async Server Components, fetch() with caching options, server actions, and client-side fetching methods. Supports static and dynamic data fetching with options for revalidation.
Use async/await directly in Server Components. Example: async function Page() { const data = await fetch('api/data'); return <Component data={data} />}. Supports automatic caching and revalidation.
Static data fetching occurs at build time using fetch with cache: 'force-cache' option. Data is cached and reused across requests. Suitable for content that doesn't change frequently.
Use fetch with cache: 'no-store' option or revalidate: 0 for dynamic data. Data fetched on every request. Suitable for real-time or frequently changing data.
ISR allows static pages to be updated after build time. Use fetch with revalidate option. Combines benefits of static and dynamic rendering. Pages regenerated based on time interval.
Use hooks like useState and useEffect in Client Components, or data fetching libraries like SWR or React Query. Handle loading states and errors. Support real-time updates.
Server Actions allow form handling and data mutations directly from Server Components. Use 'use server' directive. Support progressive enhancement. Handle form submissions securely.
Use fetch cache options or React cache function. Configure cache behavior in fetch requests. Support cache revalidation. Handle cache invalidation.
Fetch multiple data sources simultaneously using Promise.all or parallel routes. Improve performance by avoiding waterfall requests. Handle loading states independently.
Create loading.js files for automatic loading UI. Use Suspense boundaries. Support streaming and progressive rendering. Implement loading skeletons.
Handle sequential data fetching where each request depends on previous results. Manage dependencies between requests. Implement efficient loading patterns.
Implement on-demand revalidation using revalidatePath or revalidateTag. Handle time-based revalidation. Support cache invalidation. Manage revalidation triggers.
Implement request deduplication, caching strategies, parallel fetching. Handle data preloading. Support prefetching. Implement performance optimizations.
Create error.js files for error handling. Implement fallback content. Support error recovery. Handle error reporting. Manage error states.
Use Server Actions or API routes for data mutations. Handle optimistic updates. Support rollback mechanisms. Implement mutation strategies.
Implement WebSocket connections or server-sent events. Handle real-time updates. Support data synchronization. Implement real-time strategies.
Validate data on server and client side. Handle validation errors. Support schema validation. Implement validation strategies.
Implement client-side storage strategies. Handle offline support. Support data synchronization. Implement persistence patterns.
Use prefetch methods or preload data during build. Handle route prefetching. Support data preloading. Implement prefetch strategies.
Implement secure data fetching patterns. Handle authentication/authorization. Support data encryption. Implement security measures.
Create custom caching logic. Handle cache invalidation patterns. Support distributed caching. Implement cache management.
Manage nested data structures. Handle circular references. Support data normalization. Implement relationship patterns.
Handle multi-client data sync. Implement conflict resolution. Support offline-first patterns. Manage sync state.
Implement data structure changes. Handle version migrations. Support data transformation. Manage migration state.
Track data fetching performance. Handle analytics integration. Support debugging tools. Implement monitoring strategies.
Implement scalable fetching patterns. Handle large datasets. Support pagination strategies. Implement performance optimization.
Create comprehensive test suites. Handle mock data. Support integration testing. Implement test utilities.
Implement end-to-end encryption. Handle key management. Support secure transmission. Implement encryption strategies.
Handle API versioning. Support backwards compatibility. Implement version migration. Manage version state.
Create comprehensive API documentation. Generate type definitions. Support example usage. Implement documentation updates.
SSR generates HTML on the server for each request. Provides better initial page load and SEO. Next.js handles SSR automatically. Combines with client-side hydration for interactivity.
Server Components run only on server, reduce client-side JavaScript. Support async data fetching. Cannot use client-side hooks or browser APIs. Improve performance and bundle size.
Hydration attaches JavaScript functionality to server-rendered HTML. Happens automatically after initial page load. Makes static content interactive. Preserves server-rendered state.
Streaming SSR progressively sends HTML chunks as they're generated. Uses <Suspense> boundaries. Improves Time To First Byte (TTFB). Supports progressive rendering.
Use async Server Components or getServerSideProps. Data available during render. Support server-only operations. Handle loading states.
Better SEO, faster initial page load, improved performance on slow devices. Support social media previews. Handle browser without JavaScript. Improve accessibility.
Use 'use server' directive or .server.js files. Handle sensitive operations. Access server-side APIs. Support secure data handling.
SSR cache stores rendered pages on server. Improves performance for subsequent requests. Supports cache invalidation. Handle cache strategies.
Use error.js files for error boundaries. Handle server-side errors. Support fallback content. Implement error reporting.
SSR generates HTML per request, SSG at build time. SSR better for dynamic content, SSG for static. Trade-off between freshness and performance.
Use streaming, caching, code splitting. Handle resource optimization. Support performance monitoring. Implement optimization strategies.
Implement server-side authentication checks. Handle protected routes. Support session management. Implement auth strategies.
Create middleware for request processing. Handle request transformation. Support middleware chain. Implement custom logic.
Handle library compatibility issues. Support SSR-specific configurations. Implement integration strategies. Manage dependencies.
Set custom headers for SSR responses. Handle caching headers. Support security headers. Implement header management.
Implement server-side state initialization. Handle state hydration. Support state serialization. Implement state strategies.
Handle server-side translations. Support locale detection. Implement translation loading. Manage language switching.
Track SSR metrics and performance. Handle monitoring integration. Support debugging tools. Implement monitoring strategies.
Create server-side rendering tests. Handle integration testing. Support unit testing. Implement test utilities.
Implement advanced caching patterns. Handle cache invalidation. Support distributed caching. Implement cache management.
Create comprehensive error handling. Support error recovery. Handle error boundaries. Implement error reporting.
Implement real-time features with SSR. Handle connection management. Support event handling. Implement WebSocket strategies.
Handle server-side security concerns. Implement XSS protection. Support CSRF prevention. Implement security strategies.
Implement SSR in microservice architecture. Handle service communication. Support service discovery. Implement integration patterns.
Create detailed performance tracking. Handle metric collection. Support debugging tools. Implement monitoring solutions.
Implement server-side GraphQL operations. Handle query execution. Support data fetching. Implement GraphQL integration.
Create advanced optimization strategies. Handle resource optimization. Support performance patterns. Implement optimization techniques.
Implement deployment strategies for SSR. Handle environment configuration. Support scaling solutions. Implement deployment patterns.
Create comprehensive SSR documentation. Generate API documentation. Support example implementations. Implement documentation updates.
SSG generates HTML at build time instead of runtime. Pages are pre-rendered and can be served from CDN. Provides fastest possible performance. Ideal for content that doesn't change frequently.
Use generateStaticParams for App Router or getStaticProps/getStaticPaths for Pages Router. Pages are built at build time. Support static data fetching. Content cached and reused.
Define dynamic paths using generateStaticParams or getStaticPaths. Specify which paths to pre-render. Support fallback behavior. Handle path generation.
Fallback controls behavior for non-generated paths. Options: false (404), true (loading), or 'blocking' (SSR). Affects user experience and build time.
Fetch data during build using async components or getStaticProps. Data available at build time. Support external APIs. Handle build-time data requirements.
Fastest page loads, better SEO, reduced server load, improved security. Pages can be served from CDN. Support global deployment. Lower hosting costs.
Implement path validation during build. Handle invalid paths. Support custom validation. Implement error handling for path generation.
Data fetched during npm run build. Available for static page generation. Support external data sources. Handle build-time operations.
Place assets in public directory. Support automatic optimization. Handle asset references. Implement asset management strategies.
Set revalidate time in fetch options. Handle on-demand revalidation. Support revalidation triggers. Implement revalidation strategies.
Implement pagination or chunking. Handle build performance. Support incremental builds. Implement data management strategies.
Implement build optimization strategies. Handle parallel generation. Support incremental builds. Implement caching strategies.
Use client-side fetching for dynamic data. Implement hybrid approaches. Support progressive enhancement. Handle content updates.
Enable preview mode for draft content. Handle authentication. Support preview routes. Implement preview strategies.
Implement error handling during build. Support fallback content. Handle partial builds. Implement error reporting.
Use build cache for faster builds. Handle cache invalidation. Support incremental builds. Implement cache strategies.
Generate static pages for multiple locales. Handle locale routing. Support translation loading. Implement i18n strategies.
Track build metrics and performance. Handle build analytics. Support debugging tools. Implement monitoring strategies.
Build only changed pages. Handle dependency tracking. Support incremental builds. Implement build optimization.
Manage nested data relationships. Handle circular dependencies. Support data validation. Implement dependency resolution.
Create custom build workflows. Handle build stages. Support parallel processing. Implement pipeline optimization.
Implement distributed build system. Handle build coordination. Support build synchronization. Implement distribution strategies.
Implement advanced optimization techniques. Handle resource optimization. Support parallel processing. Implement optimization strategies.
Create build performance analysis. Handle metrics collection. Support debugging tools. Implement analysis strategies.
Implement secure build processes. Handle sensitive data. Support security scanning. Implement security measures.
Create automated build workflows. Handle CI/CD integration. Support automated testing. Implement automation strategies.
Create comprehensive build documentation. Generate build reports. Support example configurations. Implement documentation updates.
API Routes are serverless endpoints built into Next.js. Created in pages/api directory (Pages Router) or app/api directory (App Router). Handle HTTP requests and provide backend functionality.
Create a file in app/api directory that exports default async function. Handle request methods (GET, POST, etc.). Return Response object. Example: export async function GET() { return Response.json({ data: 'hello' }) }
Export functions named after HTTP methods (GET, POST, PUT, DELETE). Or use conditional logic in Pages Router. Support method-specific logic. Handle unsupported methods.
Access query params through request.nextUrl.searchParams in App Router or req.query in Pages Router. Parse and validate parameters. Handle missing parameters.
Access request body using await request.json() or similar methods. Validate request data. Process POST data. Return appropriate response.
Use square brackets for dynamic segments [param]. Access parameters through route object. Support multiple dynamic segments. Handle parameter validation.
Return appropriate status codes and error messages. Use try-catch blocks. Implement error handling middleware. Support error logging.
Configure CORS headers using middleware or within route handlers. Set Access-Control-Allow-Origin and other headers. Handle preflight requests.
Middleware processes requests before reaching route handlers. Handle authentication, logging, CORS. Support middleware chains. Implement custom middleware.
Process multipart/form-data using appropriate middleware. Handle file storage. Validate file types and sizes. Implement upload progress.
Implement rate limiting middleware. Track request counts. Set rate limits. Handle limit exceeded responses. Support different limit strategies.
Implement authentication middleware. Verify tokens or credentials. Handle protected routes. Support different auth strategies.
Cache API responses. Handle cache invalidation. Set cache headers. Implement caching strategies. Support different cache stores.
Validate request data using schemas or validation libraries. Handle validation errors. Return appropriate error responses. Implement validation strategies.
Log API requests and responses. Track performance metrics. Handle error logging. Implement logging strategies. Support different log formats.
Implement version control in API routes. Handle backwards compatibility. Support multiple versions. Implement versioning strategies.
Enable response compression middleware. Handle different compression types. Set appropriate headers. Implement compression strategies.
Generate API documentation. Implement OpenAPI/Swagger. Support documentation updates. Implement documentation strategies.
Track API performance and usage. Handle monitoring integration. Support analytics. Implement monitoring strategies.
Create custom error classes. Handle different error types. Support error reporting. Implement error handling strategies. Manage error states.
Implement stream handling for large responses. Handle chunked transfer. Support progressive loading. Implement streaming strategies.
Implement security best practices. Handle XSS/CSRF protection. Support security headers. Implement security strategies.
Integrate GraphQL server with API routes. Handle schema definition. Support resolvers. Implement GraphQL middleware.
Handle WebSocket connections in API routes. Manage socket state. Support real-time communication. Implement WebSocket strategies.
Implement transaction management in API routes. Handle rollbacks. Support nested transactions. Implement transaction strategies.
Create comprehensive API tests. Handle integration testing. Support unit testing. Implement test strategies. Manage test coverage.
Implement performance improvements. Handle request optimization. Support response optimization. Implement optimization strategies.
Track API usage patterns. Handle analytics integration. Support custom metrics. Implement analytics strategies.
Implement deployment strategies for API routes. Handle environment configuration. Support scaling solutions. Implement deployment patterns.
Pages are special components that become routes automatically when placed in pages/ or app/ directory. Components are reusable UI pieces that don't create routes. Pages can use getStaticProps/getServerSideProps while components cannot.
Server Components are rendered on server by default in App Router. Cannot use browser APIs or React hooks. Better performance and bundle size. Support async operations directly.
Client Components use 'use client' directive. Can use browser APIs and React hooks. Enable interactive features. Run on client side after hydration.
Create layout.js file in app directory. Wraps child pages/components. Shares UI across routes. Supports nested layouts. Uses children prop for content injection.
Hydration attaches JavaScript event handlers to server-rendered HTML. Makes static content interactive. Happens automatically after initial load. Preserves server-rendered state.
Use metadata object or generateMetadata function in page files. Set title, description, open graph data. Support dynamic metadata. Handle SEO requirements.
Create error.js files for error boundaries. Handle component errors. Support fallback content. Implement error reporting. Manage error states.
Use [param] syntax for dynamic routes. Access parameters through props. Support multiple segments. Handle parameter validation. Implement dynamic routing.
Use React Context, state management libraries, or lift state up. Handle component communication. Support state updates. Implement state management patterns.
Use dynamic imports, route-based splitting, component-based splitting. Handle lazy loading. Support chunk optimization. Implement loading strategies.
Implement performance optimizations. Use React.memo, useMemo, useCallback. Support component caching. Implement optimization strategies.
Create reusable component patterns. Handle component hierarchy. Support component inheritance. Implement composition strategies.
Use CSS Modules, styled-components, or other styling solutions. Handle dynamic styles. Support theme systems. Implement styling strategies.
Create unit tests, integration tests. Handle component rendering. Support interaction testing. Implement test strategies.
Implement form handling logic. Handle validation. Support form submission. Implement form state management. Handle form errors.
Create reusable component collections. Handle component documentation. Support theming. Implement component versioning.
Implement animation libraries or CSS transitions. Handle animation states. Support transition effects. Implement animation strategies.
Follow ARIA standards. Handle keyboard navigation. Support screen readers. Implement accessibility patterns.
Create compound components, render props, higher-order components. Handle complex patterns. Support pattern composition.
Track render performance. Handle performance metrics. Support profiling tools. Implement monitoring strategies.
Handle XSS prevention, input sanitization. Support content security policies. Implement security measures.
Implement i18n support. Handle translations. Support RTL layouts. Implement localization strategies.
Create error boundary components. Handle error recovery. Support fallback UI. Implement error reporting.
Implement state management solutions. Handle global state. Support state persistence. Implement state patterns.
Create component documentation. Generate API docs. Support example usage. Implement documentation strategies.
Implement semantic versioning. Handle backwards compatibility. Support version migration. Implement versioning strategies.
Design scalable component systems. Handle component organization. Support architecture patterns. Implement design systems.
Implement deployment strategies. Handle build optimization. Support continuous integration. Implement deployment patterns.
Layout components are created using layout.js files. Share UI between pages. Support nested layouts. Handle persistent navigation and UI elements. Available in App Router.
Use .module.css files for component-scoped CSS. Import styles as objects. Support local class naming. Automatic unique class generation. Built-in support in Next.js.
Import global CSS in app/layout.js or pages/_app.js. Apply styles across all components. Support reset styles and base themes. Handle global styling patterns.
Use media queries, CSS Grid, Flexbox. Support mobile-first design. Handle breakpoints. Implement responsive patterns. Support different screen sizes.
Create multiple layout.js files in route segments. Support layout hierarchy. Share UI between related routes. Handle layout composition.
Install and configure Tailwind CSS. Use utility classes. Support JIT mode. Handle Tailwind configuration. Implement responsive design.
Use (group) folders to organize routes. Don't affect URL structure. Share layouts within groups. Support multiple groups.
Use CSS-in-JS solutions or dynamic class names. Support runtime styles. Handle style variables. Implement dynamic theming.
Support styled-components, Emotion, and other CSS-in-JS libraries. Handle server-side rendering. Support dynamic styles. Implement styling patterns.
Implement page and layout transitions. Support animation effects. Handle transition states. Implement smooth navigation experiences.
Create theme providers and consumers. Handle theme switching. Support dark mode. Implement theme configuration. Manage theme variables.
Implement CSS minification, purging, and bundling. Handle critical CSS. Support CSS splitting. Implement optimization strategies.
Create responsive grid layouts. Handle grid areas. Support grid templates. Implement grid components. Manage grid responsiveness.
Optimize runtime performance. Handle style extraction. Support server-side generation. Implement performance strategies.
Use Next.js Image component. Handle srcset and sizes. Support art direction. Implement image optimization. Manage responsive breakpoints.
Implement CSS custom properties. Handle variable scoping. Support dynamic values. Implement theme systems. Manage variable inheritance.
Create reusable layout components. Handle layout composition. Support layout variations. Implement common patterns.
Implement CSS transitions and keyframes. Handle animation states. Support animation libraries. Implement animation patterns.
Create visual regression tests. Handle style snapshots. Support style assertions. Implement testing strategies.
Create complex layout systems. Handle dynamic layouts. Support layout algorithms. Implement advanced patterns.
Implement CSS methodology (BEM, SMACSS). Handle style organization. Support scalable systems. Implement architecture patterns.
Create comprehensive design systems. Handle component libraries. Support theme configuration. Implement system documentation.
Track CSS performance metrics. Handle style analysis. Support optimization tools. Implement monitoring strategies.
Create custom layout algorithms. Handle complex arrangements. Support dynamic positioning. Implement algorithm optimization.
Implement complex animation sequences. Handle animation orchestration. Support performance optimization. Implement animation systems.
Handle large-scale CSS module systems. Support composition patterns. Implement naming conventions. Handle module organization.
Implement CSS sanitization. Handle style injection prevention. Support content security policies. Implement security measures.
Create comprehensive style guides. Generate documentation. Support example usage. Implement documentation updates.
Implement layout performance improvements. Handle reflow optimization. Support layout calculation. Implement optimization strategies.
Next.js supports multiple state management options: React's built-in useState and useContext, external libraries like Redux and Zustand, server state with React Query/SWR. Choose based on application needs.
Use useState hook for component-level state. Handle state updates. Support state initialization. Implement local state patterns. Manage state lifecycle.
Context API shares state between components without prop drilling. Create providers and consumers. Handle context updates. Support global state patterns.
Configure Redux store with Next.js. Handle server-side state hydration. Support Redux middleware. Implement Redux patterns. Manage store configuration.
SWR is a React hooks library for data fetching. Handles caching, revalidation, and real-time updates. Support optimistic updates. Implement data fetching patterns.
Use form libraries like React Hook Form or Formik. Handle form validation. Support form submission. Implement form state patterns. Manage form data.
Store state in localStorage or sessionStorage. Handle state recovery. Support persistence strategies. Implement state serialization. Manage persistent data.
Use query parameters and URL segments for state. Handle URL updates. Support navigation state. Implement URL-based patterns. Manage route state.
Initialize state during server-side rendering. Handle state hydration. Support initial data loading. Implement SSR state patterns.
Manage loading, error, and success states. Handle async operations. Support state transitions. Implement async patterns. Manage async flow.
Use state machine libraries like XState. Handle state transitions. Support finite states. Implement state machine patterns.
Implement WebSocket or Server-Sent Events. Handle real-time updates. Support state synchronization. Implement real-time patterns.
Create middleware for state operations. Handle state transformations. Support middleware chain. Implement middleware patterns.
Implement state version control. Handle state migrations. Support backwards compatibility. Implement versioning patterns.
Create selectors for state derivation. Handle memoization. Support selector composition. Implement selector patterns.
Implement state validation rules. Handle validation errors. Support schema validation. Implement validation patterns.
Create state change logs. Handle state debugging. Support logging middleware. Implement logging patterns.
Implement state sync between clients. Handle conflict resolution. Support offline state. Implement sync patterns.
Create state management tests. Handle state assertions. Support integration testing. Implement test patterns.
Create complex state management solutions. Handle state composition. Support state hierarchies. Implement advanced patterns.
Optimize state updates and access. Handle state normalization. Support performance monitoring. Implement optimization strategies.
Design scalable state systems. Handle state organization. Support architecture patterns. Implement design principles.
Implement state data encryption. Handle key management. Support secure storage. Implement security measures.
Track state changes and performance. Handle monitoring integration. Support analytics. Implement monitoring strategies.
Implement state structure changes. Handle version migrations. Support data transformation. Implement migration strategies.
Create comprehensive state documentation. Generate API docs. Support example usage. Implement documentation updates.
Implement scalable state solutions. Handle large state trees. Support performance optimization. Implement scaling strategies.
Create debugging tools and utilities. Handle state inspection. Support troubleshooting. Implement debugging strategies.
Implement state access control. Handle authentication/authorization. Support security policies. Implement security measures.
Next.js Image component is an extension of HTML <img> tag. Provides automatic image optimization. Supports lazy loading, responsive sizing, and modern image formats. Handles image optimization on-demand.
Import Image from 'next/image'. Add src, alt, width, and height props. Component optimizes and serves images automatically. Example: <Image src='/img.png' alt='image' width={500} height={300} />
Reduces image file size, improves page load speed, supports responsive images, optimizes for different devices, reduces bandwidth usage, improves Core Web Vitals scores.
Images load only when they enter viewport. Reduces initial page load time. Uses loading='lazy' attribute automatically. Supports intersection observer API.
Images adapt to different screen sizes. Uses sizes prop for viewport-based sizing. Supports srcset generation. Implements responsive design patterns.
Use quality prop to set compression level. Default is 75. Balance between quality and file size. Support different quality levels for different images.
Shows blurred version of image while loading. Use placeholder='blur' prop. Supports blurDataURL for custom blur. Improves perceived performance.
Configure domains in next.config.js. Use loader prop for custom image service. Support remote image optimization. Handle remote image domains.
Import images as modules. Get width and height automatically. Support webpack optimization. Handle static image assets.
Support WebP, AVIF formats. Use formats prop for specific formats. Handle browser compatibility. Implement format strategies.
Use different images for different screen sizes. Handle responsive images. Support art direction patterns. Implement device-specific images.
Use priority prop for LCP images. Handle critical images. Support preload strategies. Implement performance optimization.
Create custom image loaders. Handle external image services. Support custom optimization. Implement loader strategies.
Configure cache headers. Handle browser caching. Support CDN caching. Implement caching strategies.
Handle SVG optimization. Support inline SVGs. Handle SVG sprites. Implement SVG optimization strategies.
Implement alt text. Handle role attributes. Support screen readers. Implement accessibility patterns.
Handle loading errors. Support fallback images. Implement error states. Handle error reporting.
Load images dynamically. Handle dynamic sources. Support runtime optimization. Implement dynamic patterns.
Create image component tests. Handle visual testing. Support integration testing. Implement test patterns.
Monitor loading metrics. Handle performance optimization. Support performance tracking. Implement loading strategies.
Create complex optimization strategies. Handle different use cases. Support optimization patterns. Implement advanced techniques.
Implement batch processing. Handle multiple images. Support processing queues. Implement scaling strategies.
Create image transformations. Handle resizing, cropping. Support filters and effects. Implement transformation pipelines.
Implement secure image handling. Handle access control. Support secure URLs. Implement security measures.
Track image performance metrics. Handle monitoring integration. Support analytics. Implement monitoring strategies.
Implement deployment strategies. Handle CDN integration. Support caching systems. Implement deployment patterns.
Create image component documentation. Generate API docs. Support example usage. Implement documentation updates.
Test optimization performance. Handle metric validation. Support automation testing. Implement test strategies.
Design scalable image systems. Handle architecture patterns. Support system organization. Implement design principles.
Next.js supports multiple authentication methods: JWT, session-based, OAuth providers, NextAuth.js library. Can implement custom authentication or use third-party solutions. Supports both client and server-side authentication.
NextAuth.js is a complete authentication solution for Next.js applications. Provides built-in support for multiple providers (OAuth, email, credentials). Handles sessions, JWT, and database integration.
Store JWT in HTTP-only cookies or local storage. Implement token verification. Handle token expiration. Support refresh tokens. Manage token lifecycle.
Store session data on server. Use session cookies for client identification. Handle session expiration. Support session persistence. Implement session management.
Configure OAuth providers. Handle OAuth flow. Support callback URLs. Implement user profile retrieval. Manage OAuth tokens.
Implement role checking middleware. Define user roles. Handle permission checks. Support role hierarchies. Implement access control.
Implement authentication middleware. Verify tokens or sessions. Handle unauthorized requests. Support API security. Implement rate limiting.
Implement CSRF tokens. Handle token validation. Support form submissions. Implement security headers. Prevent cross-site request forgery.
Manage user authentication state. Handle state persistence. Support state updates. Implement state management. Handle state synchronization.
Use bcrypt or similar libraries. Handle password storage. Support password validation. Implement secure hashing. Manage salt generation.
Implement token refresh logic. Handle token rotation. Support silent refresh. Implement refresh strategies. Manage token storage.
Configure social providers. Handle OAuth integration. Support user profile mapping. Implement login flow. Manage provider tokens.
Implement granular permissions. Handle permission checks. Support permission groups. Implement access control lists. Manage permission hierarchy.
Support 2FA methods. Handle verification codes. Implement backup codes. Support authentication apps. Manage 2FA setup.
Implement error handling. Support error messages. Handle recovery flows. Implement error logging. Manage error states.
Handle reset flow. Support reset tokens. Implement email notifications. Handle token expiration. Manage reset process.
Implement session storage. Handle session cleanup. Support session validation. Implement session strategies. Manage session state.
Create authentication tests. Handle test scenarios. Support integration testing. Implement test strategies. Manage test coverage.
Track authentication metrics. Handle monitoring integration. Support analytics. Implement monitoring strategies. Manage monitoring data.
Handle authorization code flow. Support refresh tokens. Implement token exchange. Handle scopes. Manage OAuth state.
Implement secure token storage. Handle token encryption. Support token validation. Implement security measures. Manage token lifecycle.
Handle tenant isolation. Support tenant authentication. Implement tenant routing. Handle tenant data. Manage tenant access.
Implement distributed session management. Handle cross-domain auth. Support SSO integration. Implement auth strategies.
Track authentication events. Handle audit trail. Support compliance requirements. Implement logging strategies. Manage audit data.
Implement security standards. Handle data privacy. Support regulatory compliance. Implement compliance measures. Manage compliance reporting.
Design scalable auth systems. Handle system organization. Support architecture patterns. Implement design principles.
Optimize authentication flow. Handle caching strategies. Support performance monitoring. Implement optimization techniques.
Create security test suites. Handle penetration testing. Support vulnerability scanning. Implement security measures.
Implement deployment strategies. Handle environment configuration. Support scaling solutions. Implement deployment patterns.
Core Web Vitals are key metrics measuring user experience: LCP (Largest Contentful Paint), FID (First Input Delay), CLS (Cumulative Layout Shift). Next.js provides built-in optimizations for these metrics.
Code splitting automatically splits JavaScript bundles by route. Reduces initial bundle size. Supports dynamic imports. Improves page load performance. Built into Next.js by default.
Next.js Image component automatically optimizes images. Supports lazy loading, responsive sizes, modern formats. Reduces image file size. Improves loading performance.
Next.js automatically determines which pages can be statically generated. Improves page load performance. Supports hybrid static and dynamic pages. No configuration required.
Next.js Script component optimizes third-party script loading. Supports loading strategies (defer, lazy). Prevents render blocking. Improves page performance.
Next.js automatically prefetches links in viewport. Reduces page load time. Supports custom prefetch behavior. Uses intersection observer API.
Next.js automatically optimizes font loading. Reduces layout shift. Supports CSS size-adjust. Implements font display strategies.
Next.js supports multiple caching strategies: build-time cache, server-side cache, client-side cache. Improves response times. Supports cache invalidation.
Use dynamic imports, React.lazy(), and Suspense. Handle component loading states. Support code splitting. Implement loading strategies.
Use @next/bundle-analyzer, analyze bundle size, identify large dependencies. Support code splitting analysis. Implement size optimization.
Implement memo, useMemo, useCallback hooks. Handle component optimization. Support render optimization. Implement rendering strategies.
Use Web Vitals API, implement analytics, track performance metrics. Support performance tracking. Implement monitoring strategies.
Use CSS Modules, implement critical CSS, handle CSS-in-JS optimization. Support style optimization. Implement CSS strategies.
Implement resource hints, handle preload/prefetch, optimize loading order. Support priority strategies. Implement resource optimization.
Configure server push, handle multiplexing, optimize request prioritization. Support HTTP/2 features. Implement protocol optimization.
Configure CDN caching, handle asset distribution, optimize edge caching. Support CDN strategies. Implement delivery optimization.
Implement load testing, measure performance metrics, use Lighthouse scores. Support performance benchmarking. Implement testing strategies.
Implement mobile-first optimization, handle responsive optimization, optimize touch interactions. Support mobile strategies. Implement device optimization.
Set performance targets, monitor metrics, implement budget tracking. Support performance goals. Implement budget strategies.
Implement WebAssembly modules, handle integration, optimize performance. Support WASM strategies. Implement optimization techniques.
Configure service workers, handle offline support, implement caching strategies. Support PWA features. Implement worker optimization.
Implement memory management, handle memory leaks, optimize resource usage. Support memory monitoring. Implement optimization strategies.
Handle 3D rendering optimization, implement WebGL best practices, optimize graphics performance. Support graphics optimization. Implement rendering strategies.
Create custom monitoring solutions, handle metric collection, implement analytics integration. Support monitoring strategies. Implement tool development.
Create performance guidelines, document optimization strategies, implement documentation updates. Support best practices. Implement documentation management.
Implement build optimization, handle CI/CD performance, optimize deployment process. Support pipeline strategies. Implement optimization techniques.
Middleware runs before request is completed. Enables custom code execution between request and response. Can modify response, redirect requests, add headers. Defined in middleware.ts file.
next.config.js is used for custom Next.js configuration. Supports various options like rewrites, redirects, environment variables. Exports configuration object or function.
Environment variables configured in .env files. Support different environments (.env.local, .env.production). Access via process.env. NEXT_PUBLIC_ prefix for client-side access.
Configure redirects in next.config.js using redirects array. Support permanent/temporary redirects. Handle path matching. Implement redirect conditions.
Rewrites allow URL mapping without path change. Configured in next.config.js. Support external rewrites. Handle path transformation. Maintain URL appearance.
Add custom headers using headers in next.config.js. Support security headers, CORS headers. Handle header conditions. Implement header policies.
Matcher defines paths where middleware runs. Uses path matching patterns. Support multiple matchers. Handle path exclusions. Configure middleware scope.
Customize webpack config in next.config.js. Modify loaders, plugins, optimization settings. Support module customization. Handle build process.
basePath sets base URL path for application. Useful for sub-path deployments. Handle path prefixing. Support path configuration.
Configure allowed image domains in next.config.js. Support external image optimization. Handle domain whitelist. Implement image security.
Create middleware functions. Handle request processing. Support middleware chain. Implement custom logic. Manage middleware flow.
Implement error handling in middleware. Support error recovery. Handle error responses. Implement logging. Manage error states.
Optimize build settings in next.config.js. Handle bundling options. Support optimization flags. Implement build strategies.
Compose multiple middleware functions. Handle execution order. Support middleware chaining. Implement composition patterns.
Validate configuration settings. Handle validation errors. Support schema validation. Implement validation strategies.
Create middleware tests. Handle test scenarios. Support integration testing. Implement test strategies.
Handle module aliases. Support path mapping. Configure module imports. Implement resolution strategies.
Manage middleware state. Handle state persistence. Support state sharing. Implement state patterns.
Handle response compression. Support compression options. Configure compression settings. Implement optimization strategies.
Configure CORS settings. Handle cross-origin requests. Support CORS headers. Implement security policies.
Create complex middleware solutions. Handle advanced scenarios. Support pattern composition. Implement advanced strategies.
Manage multiple configurations. Handle environment-specific settings. Support configuration versioning. Implement management strategies.
Create security-focused middleware. Handle security measures. Support security policies. Implement protection strategies.
Create logging solutions. Handle log management. Support log analysis. Implement logging strategies.
Scale configuration solutions. Handle large-scale settings. Support scaling strategies. Implement scaling patterns.
Create middleware documentation. Generate config docs. Support example usage. Implement documentation updates.
Configure deployment settings. Handle environment setup. Support deployment strategies. Implement deployment patterns.
Create comprehensive test suites. Handle test scenarios. Support testing patterns. Implement test strategies.
Jest and React Testing Library are recommended for unit and integration testing. Cypress or Playwright for end-to-end testing. Vitest gaining popularity for faster test execution. Built-in Next.js testing support.
Configure jest.config.js for Next.js. Set up environment and transforms. Handle module mocking. Support TypeScript testing. Default configuration available with next/jest.
Use React Testing Library to render pages. Test page components. Handle data fetching. Support routing tests. Test page lifecycle.
Use Chrome DevTools or VS Code debugger. Set breakpoints. Inspect component state. Handle error tracing. Support source maps.
Mock HTTP requests. Test API endpoints. Handle response validation. Support API testing. Implement test scenarios.
Captures component output. Compares against stored snapshots. Detects UI changes. Support snapshot updates. Handle snapshot maintenance.
Use Node.js debugger. Handle server breakpoints. Inspect server state. Support server-side debugging. Implement logging.
Test individual components/functions. Handle isolated testing. Support test coverage. Implement unit test cases. Handle component logic.
Create test fixtures. Handle mock data. Support test databases. Implement data generation. Handle test state.
Mock fetch requests. Handle async testing. Support data mocking. Implement fetch testing. Handle response simulation.
Test component interactions. Handle feature testing. Support workflow testing. Implement integration scenarios. Handle component communication.
Use React DevTools Profiler. Handle performance monitoring. Support optimization. Implement performance debugging. Handle bottlenecks.
Mock authentication state. Handle protected routes. Support auth testing. Implement auth scenarios. Handle user sessions.
Configure coverage reporting. Handle coverage goals. Support coverage analysis. Implement coverage tracking. Handle code coverage.
Create middleware tests. Handle request processing. Support middleware testing. Implement test scenarios. Handle middleware chain.
Use Cypress or Playwright. Handle user flows. Support browser testing. Implement test scenarios. Handle end-to-end workflows.
Handle route debugging. Support navigation testing. Implement route testing. Handle path resolution. Debug routing logic.
Test error scenarios. Handle error boundaries. Support error testing. Implement error cases. Handle error recovery.
Test state changes. Handle state updates. Support state testing. Implement state scenarios. Handle state logic.
Create complex test scenarios. Handle advanced cases. Support pattern testing. Implement testing strategies. Handle edge cases.
Implement CI/CD testing. Handle automated tests. Support test pipelines. Implement automation strategies. Handle continuous testing.
Test performance metrics. Handle load testing. Support stress testing. Implement performance scenarios. Handle optimization testing.
Implement security tests. Handle vulnerability testing. Support penetration testing. Implement security scenarios. Handle security validation.
Track test execution. Handle test analytics. Support monitoring tools. Implement monitoring strategies. Handle test metrics.
Create test documentation. Handle documentation updates. Support example tests. Implement documentation strategies. Handle maintenance.
Use visual regression testing. Handle UI testing. Support screenshot testing. Implement visual validation. Handle visual comparison.
Configure test environments. Handle environment setup. Support environment isolation. Implement environment management. Handle configurations.
Set up continuous testing. Handle automated runs. Support test pipelines. Implement continuous strategies. Handle test automation.
Maintain test suites. Handle test updates. Support test refactoring. Implement maintenance strategies. Handle test debt.
Understand SSR, SSG, and ISR strategies.
Work with getStaticProps, getServerSideProps, and SWR.
Explore file-based routing and app directory structure.
Expect discussions about optimization and caching strategies.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Next.js questions, mock interviews, and more to secure your dream role.
Start Preparing now