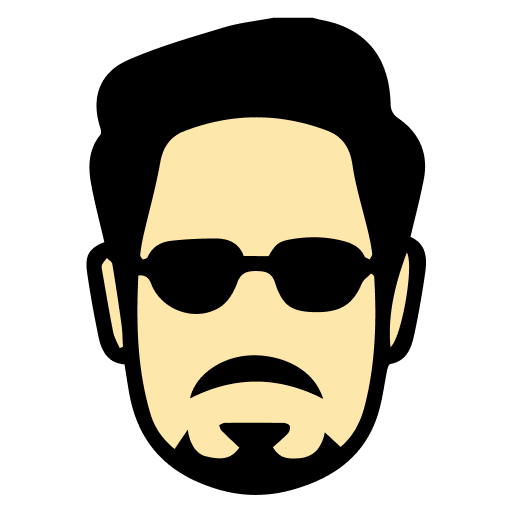
PHP powers a vast portion of web applications and remains a crucial server-side technology. Stark.ai offers a comprehensive collection of PHP interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
PHP (Hypertext Preprocessor) is a server-side scripting language designed specifically for web development....
PHP has eight primitive data types: Integer (int), Float/Double, String, Boolean, Array, Object, NULL, and Resource....
Type casting is converting one data type to another. In PHP, it can be done using either casting operators (int),...
The == operator checks only for equality of values, while === checks for both equality of values and equality of...
Superglobals are built-in variables always available in all scopes. These include: $_GET, $_POST, $_SERVER, $_FILES,...
PHP has four variable scopes: local (inside functions), global (outside functions), static (retains value between...
GET sends data through URL parameters, visible in browser history, and has length limitations. POST sends data...
Magic constants are predefined constants that change based on where they are used. Examples include __LINE__,...
The final keyword prevents child classes from overriding a method or prevents a class from being inherited. When...
Traits are mechanisms for code reuse in single inheritance languages like PHP. They allow you to reuse sets of...
Type hinting enforces specified data types for function parameters and return values. It can be used with arrays,...
Autoloading automatically loads PHP classes without explicitly using require or include statements....
Anonymous functions, also known as closures, are functions without a name that can access variables from the outside...
echo and print both output strings, but echo can take multiple parameters and is marginally faster, while print can...
yield is used to implement generators, which provide a way to iterate over a set of data without keeping the entire...
Magic methods are special methods that override PHP's default behavior when certain actions are performed on an...
self refers to the current class and is used to access static members, while $this refers to the current object...
Namespaces are a way of encapsulating related classes, interfaces, functions, and constants to avoid name...
PHP supports three types of arrays: Indexed arrays (numeric keys), Associative arrays (named keys), and...
Late static binding refers to a way of using static keyword to reference the class that was initially called at...
unset() completely removes a variable from memory, while setting a variable to null keeps the variable but sets its...
Generators provide a way to implement simple iterators without the overhead of creating a class implementing...
Abstract classes can have properties and implemented methods, while interfaces can only have method signatures. A...
Variadic functions can accept a variable number of arguments using the ... operator. func_get_args() can also be...
Constructor property promotion is a shorthand syntax that allows declaring and initializing class properties...
count() and sizeof() are identical functions and can be used interchangeably. Both return the number of elements in...
The callable type hint ensures that a function parameter can be called as a function. It accepts regular functions,...
Enums are a special type of class that represents a fixed set of possible values. They provide type safety,...
Attributes (also known as annotations in other languages) provide a way to add metadata to classes, methods,...
The four fundamental principles are: 1) Encapsulation (bundling data and methods that operate on that data within a...
public members are accessible from anywhere, private members are only accessible within the declaring class, and...
Method chaining is a technique where multiple methods are called on the same object in a single line. It's achieved...
A constructor (__construct) is automatically called when an object is created and is used to initialize object...
Dependency injection is a design pattern where objects are passed into a class through constructor or setter methods...
Static methods belong to the class itself and can be called without creating an instance of the class. They cannot...
Magic methods are special methods that override PHP's default behavior. Common ones include: __construct()...
Composition is when a class contains instances of other classes as properties, while inheritance is when a class...
Interfaces define a contract for classes by specifying which methods must be implemented. They promote loose...
Abstract classes can have both abstract and concrete methods, while interfaces can only declare method signatures. A...
Traits are mechanisms for code reuse in single inheritance languages like PHP. They allow you to define methods that...
Late static binding refers to a way of using the static keyword to reference the class that was initially called at...
Method overriding occurs when a child class provides a specific implementation for a method that is already defined...
The final keyword prevents child classes from overriding a method when used on methods, and prevents class...
Autoloading automatically loads PHP classes when they are used, eliminating the need for multiple include...
Shallow cloning (default clone keyword) creates a new object with copied scalar properties but maintains references...
Anonymous classes are classes without names, defined on-the-fly. They are useful when you need a simple, one-off...
Property type declarations allow you to specify the type of class properties. They can be scalar types, arrays,...
Constructor property promotion is a shorthand syntax that allows declaring and initializing class properties...
The Observer pattern is a behavioral design pattern where objects (observers) automatically notify their dependents...
PHP doesn't support traditional method overloading, but it can be simulated using the __call() magic method. This...
The instanceof operator is used to determine if an object is an instance of a specific class, implements an...
Singleton pattern ensures a class has only one instance. It's implemented by making the constructor private,...
Value objects are immutable objects that represent a value rather than an entity. They are used when you need to...
PHP doesn't support multiple inheritance directly, but it can be achieved through interfaces and traits. A class can...
Static binding (self::) refers to the class where the method is defined, while late static binding (static::) refers...
Named arguments allow you to pass values to a function by specifying the parameter name, regardless of their order....
The __toString() magic method allows you to define how an object should be represented as a string. It's...
PHP offers multiple ways to connect to MySQL: 1) MySQLi (object-oriented and procedural), 2) PDO (PHP Data Objects),...
PDO (PHP Data Objects) is a database abstraction layer providing consistent methods to work with multiple databases....
Prepared statements separate SQL logic from data by using placeholders for values. The database treats these values...
A transaction is a sequence of operations that must be executed as a single unit. In PHP, transactions are...
mysql_real_escape_string() escapes special characters in strings, but is deprecated and can be bypassed. Prepared...
PDO offers several fetch modes: FETCH_ASSOC (returns associative array), FETCH_NUM (returns numeric array),...
Database connection errors can be handled using try-catch blocks with PDOException for PDO, or...
LIMIT controls the maximum number of records returned, while OFFSET specifies where to start returning records....
Database connection pooling can be implemented using persistent connections (PDO::ATTR_PERSISTENT), connection...
Database migrations are version control for databases, tracking changes to database schema. They ensure consistent...
Database seeders are scripts that populate a database with initial or test data. They're useful for development...
Query optimization techniques include: using indexes properly, selecting only needed columns, using EXPLAIN to...
N+1 query problem occurs when code executes N additional queries to fetch related data for N results. It can be...
Database sharding involves distributing data across multiple databases. Implementation includes: defining sharding...
Indexes are data structures that improve the speed of data retrieval operations. They should be used on columns...
Deadlocks can be handled by: implementing retry logic with try-catch blocks, using proper transaction isolation...
Normalization is organizing database tables to minimize redundancy and dependency. It involves dividing large tables...
Database caching can be implemented using: memory caching (Redis, Memcached), query caching, full-page caching, or...
Triggers are special procedures that automatically execute when certain database events occur (INSERT, UPDATE,...
Database replication involves configuring master-slave setup, implementing read-write splitting in code, handling...
Stored procedures are pre-compiled SQL statements stored in the database. In PHP, they're called using CALL...
Large datasets can be handled using: cursor-based pagination, chunked processing, generator functions,...
Lazy loading delays database connection initialization until it's actually needed. This saves resources by not...
Database versioning can be implemented using migration tools, version control for schema files, semantic versioning...
Views are virtual tables based on result sets of SQL statements. In PHP, they're queried like regular tables but...
Database backup can be implemented using PHP functions to execute system commands, dedicated backup libraries, or...
Connection pooling maintains a cache of database connections for reuse, reducing the overhead of creating new...
Multi-tenancy can be implemented through separate databases, shared database with separate schemas, or shared tables...
Database events are notifications of database changes that can trigger PHP code execution. They can be handled using...
Connection retry logic involves implementing exponential backoff, maximum retry attempts, proper error handling, and...
Sessions store data on the server with a unique session ID sent to client via cookie, while cookies store data...
session_start() initiates or resumes a session. It must be called before any output is sent to browser. It generates...
Cookies are set using setcookie() function: setcookie(name, value, expire, path, domain, secure, httponly). They can...
Key security considerations include: using session_regenerate_id() to prevent session fixation, setting secure and...
Session timeout can be implemented by: setting session.gc_maxlifetime in php.ini, storing last activity timestamp in...
Session hijacking occurs when attacker steals session ID to impersonate user. Prevention includes: using HTTPS,...
Complete session destruction requires: session_unset() to clear variables, session_destroy() to destroy session...
PHP supports various session handlers: files (default), database, memcached, redis. Custom handlers can be...
Remember me involves: generating secure token, storing hashed token in database, setting long-lived cookie with...
Session fixation occurs when attacker sets victim's session ID. Prevention includes: regenerating session ID on...
Distributed sessions require: centralized storage (Redis/Memcached), consistent session handling across servers,...
Cookie limitations include: size (4KB), number per domain, browser settings blocking cookies. Workarounds include:...
Secure session validation includes: checking user agent consistency, validating IP address (with caution),...
session_cache_limiter() controls HTTP caching of pages with sessions. Options include: nocache, private, public,...
SameSite cookie attribute controls how cookie is sent with cross-site requests. Values: Strict, Lax, None. Helps...
Key options include: session.save_handler, session.save_path, session.gc_maxlifetime, session.cookie_lifetime,...
Custom session handling requires implementing SessionHandlerInterface with methods: open, close, read, write,...
Garbage collection removes expired session data. Controlled by session.gc_probability, session.gc_divisor, and...
AJAX session handling includes: implementing session checks in AJAX calls, handling session timeout responses,...
HTTP-only cookies cannot be accessed by JavaScript, protecting against XSS attacks. Set using httponly parameter in...
Session authentication involves: validating credentials, storing user data in session, implementing session security...
Flash data persists for only one request cycle, commonly used for temporary messages. Implementation involves...
Concurrent access handling includes: implementing session locking mechanisms, using database transactions for...
Secure cookies are only transmitted over HTTPS. Set using secure parameter in setcookie() or session configuration....
Cross-subdomain sessions require: setting session cookie domain to main domain, configuring session handler for...
Best practices include: using HTTPS, setting secure/httponly flags, implementing proper session timeout,...
Session migration involves: copying session data to new storage, updating session handler configuration, managing...
Domain cookies are accessible across subdomains. Set using domain parameter in setcookie(). Used for maintaining...
Multi-server synchronization requires: centralized session storage, consistent configuration across servers,...
Session adoption occurs when taking over existing session. Security implications include: potential session fixation...
PHP provides several basic file operations: fopen() for opening files, fread()/fwrite() for reading/writing,...
Secure file uploads require: validating file types, checking MIME types, setting upload size limits, using...
PHP supports several file modes: 'r' (read-only), 'w' (write, truncate), 'a' (append), 'r+' (read and write), 'w+'...
Large file handling techniques include: using fgets() for line-by-line reading, implementing chunked...
Streams provide a unified way to handle file, network, and compression operations. Used with stream_get_contents(),...
File permissions are managed using chmod() function, umask() for default permissions. Functions like is_readable(),...
Directory operations include: mkdir() for creation, rmdir() for deletion, opendir()/readdir()/closedir() for...
Secure file downloads require: validating file paths, checking permissions, setting proper headers (Content-Type,...
Common vulnerabilities include: directory traversal, file inclusion attacks, insufficient permissions, insecure file...
Temporary files managed using tmpfile() for automatic cleanup, tempnam() for custom temp files. Important to...
File locking uses flock() function with LOCK_SH (shared), LOCK_EX (exclusive), LOCK_UN (release). Important for...
CSV operations use fgetcsv()/fputcsv() for reading/writing, handling different delimiters and enclosures. Consider...
Symbolic links managed with symlink(), readlink(), and is_link() functions. Security considerations include proper...
File caching involves: implementing cache directory structure, managing cache lifetime, handling cache invalidation,...
Memory-mapped files accessed using php-io extension, providing efficient access to large files. Benefits include...
File compression using zlib functions (gzopen, gzwrite, gzread) or ZIP extension. Important considerations include...
Metadata operations include: stat(), filemtime(), filesize(), filetype(). Provide information about file properties,...
File backup implementation includes: copying files with versioning, implementing rotation system, handling large...
include produces warning on failure, require produces fatal error. require_once and include_once prevent multiple...
File type detection using: finfo_file(), mime_content_type(), pathinfo(), checking file extensions. Important for...
Atomic operations ensure file operation completeness without interruption. Use rename() for atomic writes, implement...
Secure path manipulation using realpath(), basename(), dirname(). Prevent directory traversal, validate paths...
Stream filters modify data as it's read/written. Used with stream_filter_append(), stream_filter_register(). Common...
File monitoring involves checking file changes using filemtime(), implementing polling or inotify extension,...
Memory considerations include: using streaming for large files, proper chunk sizes, clearing file handles,...
Encoding issues handled using mb_* functions, setting proper encoding in fopen(), handling BOM, implementing...
File ownership managed using chown(), chgrp() functions. Important for security and permissions management. Consider...
File cleanup includes: implementing age-based deletion, handling temporary files, proper permission checking,...
Best practices include: proper permissions, input validation, path sanitization, secure file operations,...
Error handling includes: try-catch blocks, checking return values, implementing logging, proper user feedback, and...
PHP frameworks provide: structured development patterns, built-in security features, database abstraction, code...
Laravel is a popular PHP framework featuring: Eloquent ORM, Blade templating, Artisan CLI, built-in security,...
Dependency Injection is a design pattern where dependencies are 'injected' into objects rather than created inside...
MVC (Model-View-Controller) separates application logic: Models handle data/business logic, Views handle...
Routing maps URLs to controller actions. Features include: route parameters, middleware, route groups, named routes,...
Symfony is a framework and component library. Key features: reusable components, dependency injection, event...
Middleware processes HTTP requests/responses before reaching controllers. Used for: authentication, CSRF protection,...
Object-Relational Mappers (ORMs) like Eloquent or Doctrine map database tables to objects. Features: relationship...
Template engines (Blade, Twig) provide syntax for views. Features: template inheritance, sections, partials,...
Service containers manage class dependencies and instantiation. Features: automatic resolution, binding interfaces...
Events allow decoupled communication between components. Features: event dispatchers, listeners, broadcasters,...
Migrations version control database schema changes. Seeders populate databases with test/initial data. Features:...
Frameworks provide authentication systems with: user providers, guards, middleware, password hashing, remember me...
CodeIgniter is lightweight framework featuring: small footprint, simple configuration, built-in security, database...
Frameworks provide caching systems supporting: file, database, memory (Redis/Memcached) caching. Features include:...
Queues handle asynchronous task processing. Features: multiple drivers (database, Redis, etc.), job retries, rate...
Service providers bootstrap application services. Handle: service registration, boot operations, package...
Frameworks provide validation systems with: built-in rules, custom validators, error messaging, form requests, CSRF...
Facades provide static interface to underlying classes. Offer convenient syntax for common services. Implementation...
Security features include: CSRF protection, XSS prevention, SQL injection protection, authentication, authorization,...
CLI tools provide commands for common tasks: generating code, running migrations, clearing cache, scheduling tasks....
Frameworks provide API tools: resource controllers, API authentication, rate limiting, response formatting,...
Frameworks include testing tools: unit testing, feature testing, browser testing, mocking, assertions. Support...
Lumen is Laravel's micro-framework for microservices and APIs. Features: fast routing, basic Laravel features,...
Localization features include: language files, translation helpers, plural forms, date/number formatting. Support...
Observers/events handle model lifecycle events (create, update, delete). Used for: maintaining related data, sending...
Authorization systems include: roles, permissions, policies, gates. Features ACL management, policy-based...
Contracts define framework component interfaces. Enable loose coupling, easy testing, component replacement. Core to...
File storage abstraction supports: local storage, cloud storage (S3, etc.), FTP. Features include: file uploads,...
Collections provide fluent interface for array operations. Features: mapping, filtering, reducing, sorting,...
REST (Representational State Transfer) is an architectural style with principles: statelessness, client-server...
API authentication methods include: JWT tokens, OAuth 2.0, API keys, Basic Auth, Bearer tokens. Implementation...
Best practices include: consistent response structure, proper HTTP status codes, clear error messages, pagination...
Versioning strategies include: URI versioning (v1/api), header versioning (Accept header), query parameter...
Rate limiting controls request frequency. Implementation includes: tracking requests per client, setting time...
CORS (Cross-Origin Resource Sharing) handled through proper headers: Access-Control-Allow-Origin, Allow-Methods,...
Resources/transformers format API responses: converting models to JSON/arrays, handling relationships, hiding...
API caching strategies include: HTTP cache headers, response caching, query result caching. Use ETags, Cache-Control...
GraphQL is query language for APIs. Features: single endpoint, client-specified data, strong typing, real-time with...
File upload handling includes: multipart form data, proper validation, secure storage, progress tracking. Consider...
Webhooks are HTTP callbacks for real-time notifications. Implementation includes: endpoint registration, payload...
API documentation tools include: Swagger/OpenAPI, API Blueprint, automated documentation generation. Include:...
Common threats include: injection attacks, unauthorized access, MITM attacks, DoS/DDoS, data exposure. Implement:...
API testing includes: unit tests, integration tests, end-to-end tests. Use tools like PHPUnit, Postman, automated...
Throttling controls API usage by limiting request rate/volume. Implementation includes: token bucket algorithm,...
Error handling includes: proper HTTP status codes, consistent error format, detailed messages, error logging....
OAuth 2.0 is authorization framework. Implementation includes: authorization flows, token management, scope...
Pagination methods include: offset/limit, cursor-based, page-based. Include metadata (total, next/prev links),...
JWTs are encoded tokens containing claims. Structure: header, payload, signature. Used for...
Logging/monitoring includes: request/response logging, error tracking, performance metrics, usage analytics....
API gateway provides single entry point for multiple services. Features: request routing, authentication, rate...
Request validation includes: input sanitization, schema validation, type checking, business rule validation....
Microservices are small, independent services. Implementation includes: service communication, data consistency,...
Optimization includes: response caching, query optimization, proper indexing, compression, connection pooling....
Composition combines multiple API calls into single endpoint. Aggregation combines data from multiple services....
Search implementation includes: query parameters, filtering, sorting, full-text search. Consider search engines...
Common patterns include: Repository, Factory, Strategy, Observer, Adapter. Used for code organization,...
Deprecation strategy includes: versioning, notification period, documentation updates, migration guides. Implement...
Orchestration coordinates multiple API calls/services. Includes: workflow management, error handling, rollback...
Analytics implementation includes: usage tracking, performance metrics, error rates, user behavior. Consider data...
PHP (Hypertext Preprocessor) is a server-side scripting language designed specifically for web development. Originally, PHP stood for Personal Home Page, but it was later renamed to PHP: Hypertext Preprocessor.
PHP has eight primitive data types: Integer (int), Float/Double, String, Boolean, Array, Object, NULL, and Resource. As of PHP 7, two additional types were added: Object and Callable.
Type casting is converting one data type to another. In PHP, it can be done using either casting operators (int), (string), (array), etc., or functions like intval(), strval(), settype(). PHP also performs automatic type conversion.
The == operator checks only for equality of values, while === checks for both equality of values and equality of types. For example, '1' == 1 returns true, but '1' === 1 returns false.
Superglobals are built-in variables always available in all scopes. These include: $_GET, $_POST, $_SERVER, $_FILES, $_COOKIE, $_SESSION, $_REQUEST, $_ENV, and $GLOBALS.
PHP has four variable scopes: local (inside functions), global (outside functions), static (retains value between function calls), and superglobal (accessible everywhere). The 'global' keyword or $GLOBALS array is used to access global variables inside functions.
GET sends data through URL parameters, visible in browser history, and has length limitations. POST sends data through HTTP request body, not visible in URL, more secure, and can handle larger data. GET is idempotent while POST is not.
Magic constants are predefined constants that change based on where they are used. Examples include __LINE__, __FILE__, __DIR__, __FUNCTION__, __CLASS__, __METHOD__, __NAMESPACE__. Their values are determined by where they are used.
The final keyword prevents child classes from overriding a method or prevents a class from being inherited. When used with methods, it prevents method overriding, and when used with classes, it prevents class inheritance.
Traits are mechanisms for code reuse in single inheritance languages like PHP. They allow you to reuse sets of methods freely in several independent classes living in different class hierarchies. They address the limitations of single inheritance.
Type hinting enforces specified data types for function parameters and return values. It can be used with arrays, objects, interfaces, and scalar types (in PHP 7+). It helps catch type-related errors early and improves code reliability.
Autoloading automatically loads PHP classes without explicitly using require or include statements. spl_autoload_register() is commonly used to register autoloader functions. PSR-4 is the modern standard for autoloading in PHP.
Anonymous functions, also known as closures, are functions without a name that can access variables from the outside scope. They are often used as callback functions and can be assigned to variables.
echo and print both output strings, but echo can take multiple parameters and is marginally faster, while print can only take one parameter and returns a value (always 1). echo is a language construct, not a function.
yield is used to implement generators, which provide a way to iterate over a set of data without keeping the entire set in memory. It's memory-efficient for large datasets as it generates values on-the-fly.
Magic methods are special methods that override PHP's default behavior when certain actions are performed on an object. Examples include __construct(), __destruct(), __get(), __set(), __isset(), __unset(), __call(), __toString().
self refers to the current class and is used to access static members, while $this refers to the current object instance and is used to access non-static members. self is resolved at compile time, while $this is resolved at runtime.
Namespaces are a way of encapsulating related classes, interfaces, functions, and constants to avoid name collisions. They provide better organization and reusability of code. They are declared using the namespace keyword.
PHP supports three types of arrays: Indexed arrays (numeric keys), Associative arrays (named keys), and Multidimensional arrays (arrays containing other arrays). Arrays in PHP are actually ordered maps.
Late static binding refers to a way of using static keyword to reference the class that was initially called at runtime rather than the class where the method is defined. It's implemented using the static keyword instead of self.
unset() completely removes a variable from memory, while setting a variable to null keeps the variable but sets its value to nothing. isset() returns false for both null values and unset variables.
Generators provide a way to implement simple iterators without the overhead of creating a class implementing Iterator interface. They're useful when working with large datasets or infinite sequences as they save memory.
Abstract classes can have properties and implemented methods, while interfaces can only have method signatures. A class can implement multiple interfaces but can extend only one abstract class. Abstract classes provide a partial implementation.
Variadic functions can accept a variable number of arguments using the ... operator. func_get_args() can also be used to get an array of all passed arguments. They provide flexibility in function parameter handling.
Constructor property promotion is a shorthand syntax that allows declaring and initializing class properties directly in the constructor parameter list. It reduces boilerplate code by combining property declaration and constructor parameter definition.
count() and sizeof() are identical functions and can be used interchangeably. Both return the number of elements in an array or properties in an object. sizeof() is an alias of count(), created for C/C++ programmers' familiarity.
The callable type hint ensures that a function parameter can be called as a function. It accepts regular functions, object methods, static class methods, and closure functions. It helps enforce that passed parameters are actually callable.
Enums are a special type of class that represents a fixed set of possible values. They provide type safety, autocompletion, and prevent invalid values. Enums can have methods and implement interfaces, making them more powerful than constant arrays.
Attributes (also known as annotations in other languages) provide a way to add metadata to classes, methods, properties, parameters, and constants. They are defined using #[] syntax and can be used for configuration, validation, and other metadata purposes.
The four fundamental principles are: 1) Encapsulation (bundling data and methods that operate on that data within a single unit), 2) Inheritance (creating new classes that are built upon existing classes), 3) Polymorphism (ability of objects to take on multiple forms), and 4) Abstraction (hiding complex implementation details and showing only functionality).
public members are accessible from anywhere, private members are only accessible within the declaring class, and protected members are accessible within the declaring class and its child classes. This helps in implementing encapsulation and controlling access to class members.
Method chaining is a technique where multiple methods are called on the same object in a single line. It's achieved by returning $this from methods, allowing subsequent method calls. For example: $object->method1()->method2()->method3(). This creates more readable and fluent interfaces.
A constructor (__construct) is automatically called when an object is created and is used to initialize object properties. A destructor (__destruct) is called when an object is destroyed or the script ends, used for cleanup tasks. Both are magic methods in PHP.
Dependency injection is a design pattern where objects are passed into a class through constructor or setter methods rather than being created inside the class. This reduces coupling, improves testability, and makes the code more maintainable by implementing inversion of control.
Static methods belong to the class itself and can be called without creating an instance of the class. They cannot access non-static properties/methods using $this. Non-static methods belong to class instances and can access all class members. Static methods are called using the scope resolution operator (::).
Magic methods are special methods that override PHP's default behavior. Common ones include: __construct() (constructor), __destruct() (destructor), __get() (accessing inaccessible properties), __set() (writing to inaccessible properties), __call() (calling inaccessible methods), __toString() (string representation of object).
Composition is when a class contains instances of other classes as properties, while inheritance is when a class extends another class. Composition provides more flexibility and loose coupling ('has-a' relationship) compared to inheritance ('is-a' relationship). Composition is often preferred over inheritance for better maintainability.
Interfaces define a contract for classes by specifying which methods must be implemented. They promote loose coupling, enable polymorphism, and allow different classes to share a common contract. A class can implement multiple interfaces, unlike inheritance where a class can only extend one class.
Abstract classes can have both abstract and concrete methods, while interfaces can only declare method signatures. A class can implement multiple interfaces but extend only one abstract class. Abstract classes can have properties and constructor, while interfaces cannot.
Traits are mechanisms for code reuse in single inheritance languages like PHP. They allow you to define methods that can be used across multiple independent classes. Traits are useful when you need to share functionality between classes that don't share a common inheritance hierarchy.
Late static binding refers to a way of using the static keyword to reference the class that was initially called at runtime rather than the class in which the method is defined. It resolves the limitations of self:: by using static:: to refer to the runtime class.
Method overriding occurs when a child class provides a specific implementation for a method that is already defined in its parent class. The child's method must be defined with the same name and parameters. The parent method can be accessed using parent:: keyword.
The final keyword prevents child classes from overriding a method when used on methods, and prevents class inheritance when used on classes. It's used when you want to prevent further modification of classes or methods for security or design reasons.
Autoloading automatically loads PHP classes when they are used, eliminating the need for multiple include statements. It's implemented using spl_autoload_register() function. PSR-4 is the modern standard for autoloading, which maps namespace prefixes to directory structures.
Shallow cloning (default clone keyword) creates a new object with copied scalar properties but maintains references to objects. Deep cloning involves implementing __clone() method to also clone nested objects. Deep cloning ensures complete independence between original and cloned objects.
Anonymous classes are classes without names, defined on-the-fly. They are useful when you need a simple, one-off object that implements an interface or extends a class. They're commonly used in testing or when you need quick object creation without formal class definition.
Property type declarations allow you to specify the type of class properties. They can be scalar types, arrays, classes, interfaces, or nullable types using ?. They ensure type safety at the property level and help catch type-related errors early.
Constructor property promotion is a shorthand syntax that allows declaring and initializing class properties directly in the constructor parameter list. It reduces boilerplate code by combining property declaration, constructor parameter, and property assignment in one line.
The Observer pattern is a behavioral design pattern where objects (observers) automatically notify their dependents (subscribers) about state changes. PHP provides SplSubject and SplObserver interfaces for implementation. It's commonly used for event handling and maintaining consistency between related objects.
PHP doesn't support traditional method overloading, but it can be simulated using the __call() magic method. This method handles calls to inaccessible or undefined methods, allowing you to implement different behaviors based on the number or types of arguments passed.
The instanceof operator is used to determine if an object is an instance of a specific class, implements an interface, or is an instance of a class that extends another class. It returns true if the object is of the specified type, false otherwise.
Singleton pattern ensures a class has only one instance. It's implemented by making the constructor private, creating a private static instance property, and a public static method to get the instance. The method creates the instance if it doesn't exist or returns the existing one.
Value objects are immutable objects that represent a value rather than an entity. They are used when you need to encapsulate values that have their own business rules and validation. Common examples include Date, Money, or Email value objects.
PHP doesn't support multiple inheritance directly, but it can be achieved through interfaces and traits. A class can implement multiple interfaces and use multiple traits. Traits provide actual method implementations, while interfaces define contracts.
Static binding (self::) refers to the class where the method is defined, while late static binding (static::) refers to the class that was initially called at runtime. Late static binding allows for more flexible inheritance and method calls in static contexts.
Named arguments allow you to pass values to a function by specifying the parameter name, regardless of their order. In OOP, this improves code readability, especially with multiple optional parameters, and makes constructor calls and method invocations more explicit and maintainable.
The __toString() magic method allows you to define how an object should be represented as a string. It's automatically called when an object is used in a string context. It must return a string and can be useful for debugging or displaying object information.
PHP offers multiple ways to connect to MySQL: 1) MySQLi (object-oriented and procedural), 2) PDO (PHP Data Objects), and 3) mysql_* functions (deprecated, shouldn't be used). PDO is preferred as it supports multiple database types and offers better security features.
PDO (PHP Data Objects) is a database abstraction layer providing consistent methods to work with multiple databases. Advantages include: database portability, prepared statements support, consistent error handling, and support for transactions. It provides a secure and flexible approach to database operations.
Prepared statements separate SQL logic from data by using placeholders for values. The database treats these values as data rather than part of the SQL command, preventing injection attacks. Values are automatically escaped, and the query structure remains constant, improving security and performance.
A transaction is a sequence of operations that must be executed as a single unit. In PHP, transactions are implemented using beginTransaction(), commit(), and rollback() methods. If any operation fails, rollback() ensures all operations are undone, maintaining data integrity.
mysql_real_escape_string() escapes special characters in strings, but is deprecated and can be bypassed. Prepared statements are more secure as they separate SQL from data, handle different data types automatically, and are more efficient due to query preparation and caching.
PDO offers several fetch modes: FETCH_ASSOC (returns associative array), FETCH_NUM (returns numeric array), FETCH_BOTH (returns both), FETCH_OBJ (returns object), FETCH_CLASS (returns instance of specified class), and FETCH_LAZY (allows property access of all three).
Database connection errors can be handled using try-catch blocks with PDOException for PDO, or mysqli_connect_errno() and mysqli_connect_error() for MySQLi. Good practice includes logging errors, displaying user-friendly messages, and implementing connection retry logic.
LIMIT controls the maximum number of records returned, while OFFSET specifies where to start returning records. These are commonly used for pagination. In PHP, they're often used with prepared statements: 'SELECT * FROM table LIMIT ? OFFSET ?'
Database connection pooling can be implemented using persistent connections (PDO::ATTR_PERSISTENT), connection management libraries like PHP-CP, or external connection poolers like PgBouncer. This helps reduce connection overhead and improve application performance.
Database migrations are version control for databases, tracking changes to database schema. They ensure consistent database structure across different environments, enable rollback of changes, and facilitate team collaboration. Tools like Phinx or Laravel Migrations help manage this process.
Database seeders are scripts that populate a database with initial or test data. They're useful for development environments, testing, and providing default data. Seeders help ensure consistent data across different environments and make testing more reliable.
Query optimization techniques include: using indexes properly, selecting only needed columns, using EXPLAIN to analyze queries, implementing caching, using prepared statements, limiting result sets, optimizing JOIN operations, and avoiding N+1 query problems.
N+1 query problem occurs when code executes N additional queries to fetch related data for N results. It can be solved using eager loading (JOIN queries), implementing proper indexing, using subqueries, or utilizing ORM features like with() in Laravel.
Database sharding involves distributing data across multiple databases. Implementation includes: defining sharding key, creating routing logic, managing cross-shard queries, and handling transactions. PHP frameworks or custom implementations can manage connection routing and data distribution.
Indexes are data structures that improve the speed of data retrieval operations. They should be used on columns frequently used in WHERE clauses, JOIN conditions, and ORDER BY statements. However, they add overhead to write operations and consume storage space.
Deadlocks can be handled by: implementing retry logic with try-catch blocks, using proper transaction isolation levels, ordering operations consistently, minimizing transaction duration, implementing timeouts, and logging deadlock incidents for analysis.
Normalization is organizing database tables to minimize redundancy and dependency. It involves dividing large tables into smaller ones and defining relationships. This improves data integrity, reduces anomalies, and makes the database more efficient and maintainable.
Database caching can be implemented using: memory caching (Redis, Memcached), query caching, full-page caching, or ORM caching. Proper cache invalidation strategies, TTL settings, and cache tags help maintain data consistency while improving performance.
Triggers are special procedures that automatically execute when certain database events occur (INSERT, UPDATE, DELETE). In PHP, triggers are defined at database level but can be created and managed through PHP code. They help maintain data integrity and automate actions.
Database replication involves configuring master-slave setup, implementing read-write splitting in code, handling replication lag, and managing failover. PHP applications can use different connections for read/write operations and implement logic to handle replication issues.
Stored procedures are pre-compiled SQL statements stored in the database. In PHP, they're called using CALL statement with PDO or MySQLi. They can improve performance, reduce network traffic, and encapsulate business logic at database level.
Large datasets can be handled using: cursor-based pagination, chunked processing, generator functions, memory-efficient algorithms, background job processing, and streaming results. Proper indexing and query optimization are also crucial.
Lazy loading delays database connection initialization until it's actually needed. This saves resources by not establishing connections unnecessarily. It's implemented by wrapping connection logic in methods that are called only when database access is required.
Database versioning can be implemented using migration tools, version control for schema files, semantic versioning for database changes, and proper documentation. This ensures consistent database state across environments and facilitates deployment.
Views are virtual tables based on result sets of SQL statements. In PHP, they're queried like regular tables but provide benefits like data abstraction, security through limited access, and simplified complex queries. They help maintain clean application architecture.
Database backup can be implemented using PHP functions to execute system commands, dedicated backup libraries, or framework tools. Important aspects include scheduling backups, compression, secure storage, verification, and testing recovery procedures.
Connection pooling maintains a cache of database connections for reuse, reducing the overhead of creating new connections. Benefits include improved performance, better resource utilization, and connection management. It's especially useful in high-traffic applications.
Multi-tenancy can be implemented through separate databases, shared database with separate schemas, or shared tables with tenant IDs. PHP code manages tenant identification, data isolation, and connection routing. Proper security measures ensure data separation.
Database events are notifications of database changes that can trigger PHP code execution. They can be handled using event listeners, message queues, or polling mechanisms. This enables real-time updates and maintaining data consistency across systems.
Connection retry logic involves implementing exponential backoff, maximum retry attempts, proper error handling, and logging. It helps handle temporary connection issues and improves application reliability. Implementation typically uses try-catch blocks with sleep intervals.
Sessions store data on the server with a unique session ID sent to client via cookie, while cookies store data directly on the client's browser. Sessions are more secure for sensitive data and expire when browser closes by default, while cookies can persist for specified durations.
session_start() initiates or resumes a session. It must be called before any output is sent to browser. It generates a unique session ID, creates/loads the session file on server, and sets a session cookie. It also loads existing session data into $_SESSION superglobal.
Cookies are set using setcookie() function: setcookie(name, value, expire, path, domain, secure, httponly). They can be retrieved using $_COOKIE superglobal array. Parameters control cookie lifetime, visibility, and security settings.
Key security considerations include: using session_regenerate_id() to prevent session fixation, setting secure and httponly flags, implementing session timeout, validating session data, proper session destruction, and securing session storage location.
Session timeout can be implemented by: setting session.gc_maxlifetime in php.ini, storing last activity timestamp in session, checking elapsed time on each request, and destroying session if timeout exceeded. Also consider implementing sliding expiration.
Session hijacking occurs when attacker steals session ID to impersonate user. Prevention includes: using HTTPS, setting secure/httponly flags, regenerating session IDs, implementing IP validation, using token-based authentication, and proper session timeout.
Complete session destruction requires: session_unset() to clear variables, session_destroy() to destroy session data, clearing session cookie using setcookie(), and unset($_SESSION) array. Best practice includes clearing all related cookies and session data.
PHP supports various session handlers: files (default), database, memcached, redis. Custom handlers can be implemented using SessionHandler interface. Each has advantages for different scenarios (scalability, persistence, performance).
Remember me involves: generating secure token, storing hashed token in database, setting long-lived cookie with token, validating token on subsequent visits. Implementation should include token rotation, secure storage, and proper expiration handling.
Session fixation occurs when attacker sets victim's session ID. Prevention includes: regenerating session ID on login/privilege change using session_regenerate_id(true), validating session data, and implementing proper session security measures.
Distributed sessions require: centralized storage (Redis/Memcached), consistent session handling across servers, proper load balancing configuration, implementing sticky sessions or session replication, and handling failover scenarios.
Cookie limitations include: size (4KB), number per domain, browser settings blocking cookies. Workarounds include: using local storage for larger data, implementing fallback mechanisms, splitting data across multiple cookies, server-side storage alternatives.
Secure session validation includes: checking user agent consistency, validating IP address (with caution), implementing CSRF tokens, validating session age, checking session data integrity, and implementing proper authentication checks.
session_cache_limiter() controls HTTP caching of pages with sessions. Options include: nocache, private, public, private_no_expire. Affects how browsers and proxies cache session pages. Important for security and proper page caching.
SameSite cookie attribute controls how cookie is sent with cross-site requests. Values: Strict, Lax, None. Helps prevent CSRF attacks and protects against cross-site request attacks. Important for modern web security compliance.
Key options include: session.save_handler, session.save_path, session.gc_maxlifetime, session.cookie_lifetime, session.cookie_secure, session.cookie_httponly. These control session behavior, storage, lifetime, and security settings.
Custom session handling requires implementing SessionHandlerInterface with methods: open, close, read, write, destroy, gc. Used for custom storage solutions or specific session management requirements. Must handle all session operations properly.
Garbage collection removes expired session data. Controlled by session.gc_probability, session.gc_divisor, and session.gc_maxlifetime settings. Process runs randomly based on probability settings. Important for server resource management.
AJAX session handling includes: implementing session checks in AJAX calls, handling session timeout responses, providing user feedback, implementing automatic logout, and managing session refresh mechanisms. Consider implementing keepalive requests.
HTTP-only cookies cannot be accessed by JavaScript, protecting against XSS attacks. Set using httponly parameter in setcookie() or session configuration. Important security measure for sensitive cookies like session IDs.
Session authentication involves: validating credentials, storing user data in session, implementing session security measures, handling remember me functionality, implementing proper logout, and managing session expiration.
Flash data persists for only one request cycle, commonly used for temporary messages. Implementation involves storing data in session, checking for data existence, displaying data, and removing after use. Often used for success/error messages.
Concurrent access handling includes: implementing session locking mechanisms, using database transactions for session storage, handling race conditions, implementing proper session state management, and considering distributed session storage.
Secure cookies are only transmitted over HTTPS. Set using secure parameter in setcookie() or session configuration. Should be used for all sensitive data and session cookies when site uses HTTPS. Essential for maintaining transport security.
Cross-subdomain sessions require: setting session cookie domain to main domain, configuring session handler for shared access, managing session security across subdomains, and handling domain-specific session data appropriately.
Best practices include: using HTTPS, setting secure/httponly flags, implementing proper session timeout, regenerating session IDs, validating session data, secure storage, proper destruction, and implementing CSRF protection.
Session migration involves: copying session data to new storage, updating session handler configuration, managing transition period, handling failover scenarios, and ensuring data consistency. Important for system upgrades or architecture changes.
Domain cookies are accessible across subdomains. Set using domain parameter in setcookie(). Used for maintaining user state across subdomains, implementing single sign-on, sharing necessary data between related sites. Requires careful security consideration.
Multi-server synchronization requires: centralized session storage, consistent configuration across servers, handling race conditions, implementing proper locking mechanisms, and managing session replication or shared storage.
Session adoption occurs when taking over existing session. Security implications include: potential session fixation attacks, need for proper validation, importance of session regeneration, and implementing proper authentication checks. Requires careful implementation.
PHP provides several basic file operations: fopen() for opening files, fread()/fwrite() for reading/writing, file_get_contents()/file_put_contents() for simple operations, fclose() for closing files, and unlink() for deletion. Additional functions include copy(), rename(), and file_exists().
Secure file uploads require: validating file types, checking MIME types, setting upload size limits, using move_uploaded_file(), scanning for malware, storing files outside web root, using random filenames, setting proper permissions, and validating file contents.
PHP supports several file modes: 'r' (read-only), 'w' (write, truncate), 'a' (append), 'r+' (read and write), 'w+' (read and write, truncate), 'a+' (read and append). Adding 'b' for binary mode. Each mode affects file pointer position and file handling behavior.
Large file handling techniques include: using fgets() for line-by-line reading, implementing chunked reading/writing, using generators, setting proper memory limits, implementing progress tracking, and using stream functions for memory efficiency.
Streams provide a unified way to handle file, network, and compression operations. Used with stream_get_contents(), fopen() with stream wrappers (file://, http://, ftp://, etc.). Support filters and contexts for advanced operations.
File permissions are managed using chmod() function, umask() for default permissions. Functions like is_readable(), is_writable() check permissions. Important for security. Permissions should follow principle of least privilege.
Directory operations include: mkdir() for creation, rmdir() for deletion, opendir()/readdir()/closedir() for reading, scandir() for listing contents, dirname()/basename() for path manipulation, and is_dir() for checking directory existence.
Secure file downloads require: validating file paths, checking permissions, setting proper headers (Content-Type, Content-Disposition), implementing rate limiting, scanning files, and using readfile() or fpassthru() for streaming.
Common vulnerabilities include: directory traversal, file inclusion attacks, insufficient permissions, insecure file uploads, path manipulation, and race conditions. Prevention requires proper validation, sanitization, and secure coding practices.
Temporary files managed using tmpfile() for automatic cleanup, tempnam() for custom temp files. Important to implement proper cleanup, set secure permissions, use system temp directory, and handle concurrent access properly.
File locking uses flock() function with LOCK_SH (shared), LOCK_EX (exclusive), LOCK_UN (release). Important for preventing race conditions in concurrent access. Should implement proper error handling and timeout mechanisms.
CSV operations use fgetcsv()/fputcsv() for reading/writing, handling different delimiters and enclosures. Consider character encoding, handling large files, validating data, and implementing proper error handling.
Symbolic links managed with symlink(), readlink(), and is_link() functions. Security considerations include proper validation, handling recursive links, and implementing access controls. Important for file system organization.
File caching involves: implementing cache directory structure, managing cache lifetime, handling cache invalidation, implementing file locking for concurrent access, and proper error handling. Consider using existing caching libraries.
Memory-mapped files accessed using php-io extension, providing efficient access to large files. Benefits include faster access and shared memory capabilities. Consider memory limitations and proper resource management.
File compression using zlib functions (gzopen, gzwrite, gzread) or ZIP extension. Important considerations include compression ratio, memory usage, handling large files, and proper error handling.
Metadata operations include: stat(), filemtime(), filesize(), filetype(). Provide information about file properties, modification times, and attributes. Important for file management and caching implementations.
File backup implementation includes: copying files with versioning, implementing rotation system, handling large files, compression, secure storage, verification, and proper error handling. Consider automated backup scheduling.
include produces warning on failure, require produces fatal error. require_once and include_once prevent multiple inclusions. Choose based on dependency criticality. Important for modular code organization.
File type detection using: finfo_file(), mime_content_type(), pathinfo(), checking file extensions. Important for security in file uploads. Should not rely solely on file extensions for validation.
Atomic operations ensure file operation completeness without interruption. Use rename() for atomic writes, implement proper locking, handle temporary files correctly. Important for data integrity and concurrent access.
Secure path manipulation using realpath(), basename(), dirname(). Prevent directory traversal, validate paths against whitelist, use proper encoding, and implement access controls. Important for security.
Stream filters modify data as it's read/written. Used with stream_filter_append(), stream_filter_register(). Common for encryption, compression, character encoding. Consider performance impact and proper error handling.
File monitoring involves checking file changes using filemtime(), implementing polling or inotify extension, handling multiple files, logging changes, and proper resource management. Consider performance impact.
Memory considerations include: using streaming for large files, proper chunk sizes, clearing file handles, implementing garbage collection, monitoring memory usage, and setting appropriate memory limits. Important for performance.
Encoding issues handled using mb_* functions, setting proper encoding in fopen(), handling BOM, implementing conversion functions. Important for international character support and data integrity.
File ownership managed using chown(), chgrp() functions. Important for security and permissions management. Consider system-level permissions, proper error handling, and security implications.
File cleanup includes: implementing age-based deletion, handling temporary files, proper permission checking, implementing logging, and error handling. Consider automated scheduling and resource management.
Best practices include: proper permissions, input validation, path sanitization, secure file operations, implementing access controls, proper error handling, and following principle of least privilege. Regular security audits recommended.
Error handling includes: try-catch blocks, checking return values, implementing logging, proper user feedback, and recovery mechanisms. Consider different error types and appropriate response strategies.
PHP frameworks provide: structured development patterns, built-in security features, database abstraction, code reusability, MVC architecture, robust routing systems, template engines, built-in tools for testing, and faster development cycles. They also enforce consistent coding standards.
Laravel is a popular PHP framework featuring: Eloquent ORM, Blade templating, Artisan CLI, built-in security, database migrations, authentication system, task scheduling, queue management, real-time events, and extensive package ecosystem via Composer.
Dependency Injection is a design pattern where dependencies are 'injected' into objects rather than created inside them. Frameworks use DI containers to manage object creation and lifecycle. This promotes loose coupling, testability, and maintainable code.
MVC (Model-View-Controller) separates application logic: Models handle data/business logic, Views handle presentation, Controllers handle request flow. Frameworks provide structure and base classes for each component, promoting organized and maintainable code.
Routing maps URLs to controller actions. Features include: route parameters, middleware, route groups, named routes, resource routing. Frameworks handle request parsing, parameter binding, and response generation. Supports RESTful routing patterns.
Symfony is a framework and component library. Key features: reusable components, dependency injection, event dispatcher, console tools, security system. Components can be used independently in other projects. Follows SOLID principles.
Middleware processes HTTP requests/responses before reaching controllers. Used for: authentication, CSRF protection, logging, request modification. Can be global or route-specific. Implements pipeline pattern for request handling.
Object-Relational Mappers (ORMs) like Eloquent or Doctrine map database tables to objects. Features: relationship handling, query building, migrations, model events. Simplifies database operations and provides abstraction layer.
Template engines (Blade, Twig) provide syntax for views. Features: template inheritance, sections, partials, escaping, custom directives. Compiles templates to plain PHP for performance. Separates logic from presentation.
Service containers manage class dependencies and instantiation. Features: automatic resolution, binding interfaces to implementations, singleton patterns, contextual binding. Core to dependency injection implementation.
Events allow decoupled communication between components. Features: event dispatchers, listeners, broadcasters, queued events. Used for logging, notifications, cache clearing, etc. Supports synchronous and asynchronous processing.
Migrations version control database schema changes. Seeders populate databases with test/initial data. Features: rollback capability, timestamps, factory patterns. Essential for database version control and testing.
Frameworks provide authentication systems with: user providers, guards, middleware, password hashing, remember me functionality, OAuth support. Includes session management, token authentication, and multiple authentication schemes.
CodeIgniter is lightweight framework featuring: small footprint, simple configuration, built-in security, database abstraction, form/data validation, session handling. Known for simplicity, performance, and minimal configuration.
Frameworks provide caching systems supporting: file, database, memory (Redis/Memcached) caching. Features include: cache tags, atomic locks, cache drivers, automatic cache clearing. Important for performance optimization.
Queues handle asynchronous task processing. Features: multiple drivers (database, Redis, etc.), job retries, rate limiting, scheduled jobs. Used for emails, notifications, and resource-intensive tasks.
Service providers bootstrap application services. Handle: service registration, boot operations, package configuration, event registration. Core to framework initialization and package integration.
Frameworks provide validation systems with: built-in rules, custom validators, error messaging, form requests, CSRF protection. Supports client and server-side validation, file validation, and complex validation scenarios.
Facades provide static interface to underlying classes. Offer convenient syntax for common services. Implementation varies: Laravel uses facade pattern, others use static proxies or service locators.
Security features include: CSRF protection, XSS prevention, SQL injection protection, authentication, authorization, encryption, password hashing. Frameworks provide middleware and helpers for common security needs.
CLI tools provide commands for common tasks: generating code, running migrations, clearing cache, scheduling tasks. Support custom commands, interactive mode. Essential for development and deployment workflows.
Frameworks provide API tools: resource controllers, API authentication, rate limiting, response formatting, versioning support. Include features for RESTful APIs, API documentation, and testing.
Frameworks include testing tools: unit testing, feature testing, browser testing, mocking, assertions. Support PHPUnit integration, database testing, HTTP testing. Essential for maintaining code quality.
Lumen is Laravel's micro-framework for microservices and APIs. Features: fast routing, basic Laravel features, minimal overhead. Best for simple applications, APIs where full framework unnecessary.
Localization features include: language files, translation helpers, plural forms, date/number formatting. Support multiple languages, language switching, fallback locales. Important for international applications.
Observers/events handle model lifecycle events (create, update, delete). Used for: maintaining related data, sending notifications, logging changes. Helps separate concerns and maintain clean models.
Authorization systems include: roles, permissions, policies, gates. Features ACL management, policy-based authorization, role hierarchies. Integrates with authentication and middleware systems.
Contracts define framework component interfaces. Enable loose coupling, easy testing, component replacement. Core to framework extensibility and maintainability. Follow interface segregation principle.
File storage abstraction supports: local storage, cloud storage (S3, etc.), FTP. Features include: file uploads, storage drivers, file operations, URL generation. Provides consistent interface across storage systems.
Collections provide fluent interface for array operations. Features: mapping, filtering, reducing, sorting, grouping. Extends array functionality with object-oriented interface. Important for data manipulation.
REST (Representational State Transfer) is an architectural style with principles: statelessness, client-server separation, cacheable resources, uniform interface, layered system. Uses HTTP methods (GET, POST, PUT, DELETE) for CRUD operations.
API authentication methods include: JWT tokens, OAuth 2.0, API keys, Basic Auth, Bearer tokens. Implementation involves token generation, validation, middleware for protection, and proper error handling. Consider security best practices like token expiration.
Best practices include: consistent response structure, proper HTTP status codes, clear error messages, pagination metadata, proper content type headers. Use envelope pattern when needed, handle nested resources, implement proper serialization.
Versioning strategies include: URI versioning (v1/api), header versioning (Accept header), query parameter versioning. Consider backward compatibility, deprecation policies, documentation. Choose strategy based on client needs.
Rate limiting controls request frequency. Implementation includes: tracking requests per client, setting time windows, using tokens/keys, implementing cooldown periods. Use Redis/cache for distributed systems. Return proper headers for limit status.
CORS (Cross-Origin Resource Sharing) handled through proper headers: Access-Control-Allow-Origin, Allow-Methods, Allow-Headers. Implementation includes preflight requests handling, proper middleware configuration, security considerations.
Resources/transformers format API responses: converting models to JSON/arrays, handling relationships, hiding sensitive data. Features include conditional attributes, nested resources, custom transformations. Important for consistent response formatting.
API caching strategies include: HTTP cache headers, response caching, query result caching. Use ETags, Cache-Control headers, implement cache invalidation. Consider cache layers (client, CDN, server) and cache lifetime.
GraphQL is query language for APIs. Features: single endpoint, client-specified data, strong typing, real-time with subscriptions. Differs from REST in data fetching, versioning approach, and response structure. Consider use case for implementation.
File upload handling includes: multipart form data, proper validation, secure storage, progress tracking. Consider chunked uploads for large files, implement proper error handling, use secure file operations.
Webhooks are HTTP callbacks for real-time notifications. Implementation includes: endpoint registration, payload signing, retry logic, event queuing. Consider security, validation, and proper error handling.
API documentation tools include: Swagger/OpenAPI, API Blueprint, automated documentation generation. Include: endpoints, parameters, responses, examples. Keep documentation updated, consider interactive documentation.
Common threats include: injection attacks, unauthorized access, MITM attacks, DoS/DDoS, data exposure. Implement: authentication, rate limiting, input validation, proper encryption, secure headers.
API testing includes: unit tests, integration tests, end-to-end tests. Use tools like PHPUnit, Postman, automated testing. Test authentication, validation, error cases, performance. Implement CI/CD pipeline.
Throttling controls API usage by limiting request rate/volume. Implementation includes: token bucket algorithm, sliding window, concurrent request limiting. Consider user tiers, custom limits, proper error responses.
Error handling includes: proper HTTP status codes, consistent error format, detailed messages, error logging. Implement global exception handler, format exceptions properly, consider security in error messages.
OAuth 2.0 is authorization framework. Implementation includes: authorization flows, token management, scope handling, refresh tokens. Consider security best practices, proper flow selection, token storage.
Pagination methods include: offset/limit, cursor-based, page-based. Include metadata (total, next/prev links), handle large datasets, implement proper caching. Consider performance and use case requirements.
JWTs are encoded tokens containing claims. Structure: header, payload, signature. Used for authentication/authorization. Implementation includes token generation, validation, refresh mechanisms. Consider security implications.
Logging/monitoring includes: request/response logging, error tracking, performance metrics, usage analytics. Implement proper log levels, monitoring tools integration, alerting systems. Consider privacy and security.
API gateway provides single entry point for multiple services. Features: request routing, authentication, rate limiting, caching, monitoring. Benefits include centralized control, security, scalability.
Request validation includes: input sanitization, schema validation, type checking, business rule validation. Implement validation middleware, proper error responses, custom validation rules. Consider performance impact.
Microservices are small, independent services. Implementation includes: service communication, data consistency, deployment strategies, service discovery. Consider scalability, monitoring, error handling.
Optimization includes: response caching, query optimization, proper indexing, compression, connection pooling. Consider bottlenecks, monitoring, scaling strategies. Implement proper profiling.
Composition combines multiple API calls into single endpoint. Aggregation combines data from multiple services. Consider performance, error handling, data consistency. Implement proper caching strategies.
Search implementation includes: query parameters, filtering, sorting, full-text search. Consider search engines integration, performance optimization, proper indexing. Implement relevance scoring.
Common patterns include: Repository, Factory, Strategy, Observer, Adapter. Used for code organization, maintainability, scalability. Consider use case requirements, team familiarity, implementation complexity.
Deprecation strategy includes: versioning, notification period, documentation updates, migration guides. Implement warning headers, monitoring deprecated usage, proper communication. Consider client impact.
Orchestration coordinates multiple API calls/services. Includes: workflow management, error handling, rollback mechanisms. Consider transaction management, service dependencies, monitoring. Implement proper logging.
Analytics implementation includes: usage tracking, performance metrics, error rates, user behavior. Consider data collection, storage, visualization tools. Implement proper privacy measures.
Understand concepts like OOP, functions, arrays, and error handling.
Work on database integration, API development, and security implementation.
Dive into frameworks, design patterns, and performance optimization.
Expect hands-on challenges to build, debug, and optimize PHP applications.
Join thousands of successful candidates preparing with Stark.ai. Start practicing PHP questions, mock interviews, and more to secure your dream role.
Start Preparing now