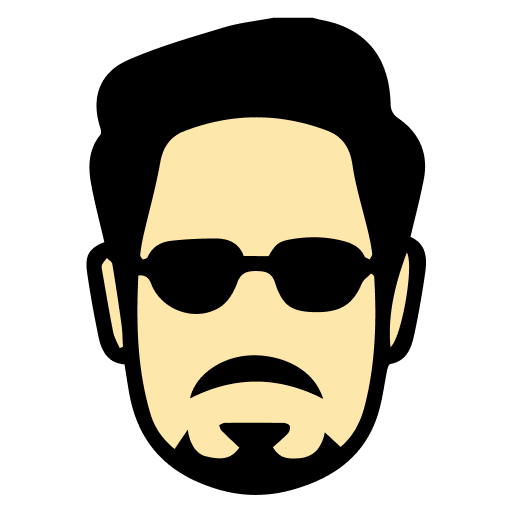
React Native is a powerful framework for building cross-platform mobile applications using JavaScript and React. Stark.ai offers a curated collection of React Native interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
React Native is a framework developed by Facebook that allows developers to build native mobile applications using...
React Native provides several core components that map directly to native UI elements: View (container), Text (text...
AppRegistry is the entry point to run a React Native application. It provides the registerComponent method used to...
Expo provides a managed workflow with pre-built components and services, making it easier to start but with limited...
Metro is React Native's JavaScript bundler that combines all JavaScript code and dependencies into a single file. It...
React Native offers multiple debugging options: Chrome Developer Tools for JavaScript debugging, React Developer...
package.json manages project dependencies, scripts, and configuration. It lists all npm packages required by the...
React Native runs on three main threads: 1) Main Thread (Native UI), 2) JavaScript Thread (JS execution), and 3)...
babel.config.js configures Babel transpilation settings for the project. It defines how modern JavaScript features...
Hot Reloading updates only the changed components while maintaining the app's state. Live Reloading refreshes the...
The bridge is the communication layer between JavaScript and native code. It serializes data and handles...
React Native provides Platform.select(), platform-specific file extensions (.ios.js/.android.js), Platform.OS...
Native modules expose platform-specific APIs to JavaScript through the bridge. They're used when requiring direct...
The Shadow Thread handles layout calculations using Yoga (Facebook's cross-platform layout engine). It processes...
iOS uses JavaScriptCore (JSC) by default, while Android can use either JSC or Hermes. Hermes is Facebook's custom JS...
Linking handles deep linking and URL scheme integration in React Native apps. It provides methods to open external...
React Native provides APIs to request and check platform permissions (camera, location, etc.). These are handled...
metro.config.js customizes the Metro bundler's behavior, including module resolution, asset handling, and...
Assets are handled through the Metro bundler, which can process images, fonts, and other resources....
The New Architecture introduces Fabric (new rendering system) and TurboModules (improved native modules). It reduces...
Fabric is a C++ rendering system that enables synchronous operations between JS and native code. It introduces a new...
TurboModules provide direct JS to native communication without serialization overhead. They use codegen to create...
React Native manages memory through automatic garbage collection in JS and ARC/GC in native code. Understanding the...
JSI enables direct communication between JavaScript and native code without going through the bridge. It provides a...
Native crashes are handled through platform-specific crash reporting tools and services. Integration requires native...
Security involves handling data encryption, secure storage, certificate pinning, code obfuscation, and protecting...
App initialization involves native bootstrap, JS engine initialization, bundle loading, and component mounting....
React Native has two types of components: 1) Class Components - ES6 classes that extend React.Component and have...
Props (properties) are read-only components that are passed from a parent component to a child component. They are...
View is a container component that works like a div in web development, used for layouting and styling. Text is...
SafeAreaView is a component that automatically adds padding to accommodate notches, status bars, and other...
Touch events are handled using components like TouchableOpacity, TouchableHighlight, TouchableWithoutFeedback, and...
FlatList is optimized for long lists of data, rendering items lazily and only what's visible on screen. ScrollView...
Data can be passed between components using props for parent-to-child communication, callback functions for...
The Image component is used to display images in React Native. It can handle both local and remote images, supports...
Style props are used to customize the appearance of components. They can be applied directly as props or through...
KeyboardAvoidingView is a component that adjusts its height or position based on the keyboard height to prevent the...
Component composition involves building larger components from smaller ones using props.children, render props, and...
Refs provide a way to access DOM nodes or React elements directly. They should be used sparingly for cases like...
FlatList performance can be optimized by: 1) Using getItemLayout to avoid measurement of items, 2) Implementing...
Controlled components have their state managed by React through props and callbacks (e.g., value and onChangeText)....
Modern React Native uses hooks like useEffect for lifecycle management. useEffect replaces componentDidMount,...
PureComponent implements shouldComponentUpdate with a shallow prop and state comparison. It's used to optimize...
Prop validation is handled using PropTypes or TypeScript. PropTypes provide runtime checking of prop types, while...
HOCs are functions that take a component and return a new component with additional props or behavior. They're used...
Modal dialogs can be implemented using the Modal component or third-party libraries. Considerations include proper...
Render props are a pattern where a component receives a function prop that returns React elements. Unlike HOCs,...
Custom native components require native code implementation (Java/Kotlin for Android, Objective-C/Swift for iOS) and...
Rerendering optimization involves: 1) Using React.memo for functional components, 2) Implementing...
Virtualized list challenges include: 1) Memory management for large datasets, 2) Smooth scrolling performance, 3)...
Complex gestures require the PanResponder system or gesture libraries. Implementation involves handling gesture...
Lazy loading strategies include: 1) React.lazy with Suspense, 2) Dynamic imports, 3) Route-based code splitting, 4)...
Error boundaries are components that catch JavaScript errors in their child component tree. Implementation involves...
Accessibility implementation includes: 1) Proper use of accessibility props, 2) Semantic markup, 3) Focus...
State is a mutable data store internal to a component that determines the component's behavior and rendering. Unlike...
useState is a React hook that adds state management to functional components. It returns an array with two elements:...
In class components, state is updated using this.setState(). It can accept either an object with new state values or...
useState is simpler and good for managing independent pieces of state. useReducer is preferred for complex state...
AsyncStorage is a simple, unencrypted, asynchronous, persistent, key-value storage system that is global to the app....
Context API provides a way to pass data through the component tree without manually passing props. It's useful for...
Form state can be handled using controlled components with useState, or through form management libraries like...
useEffect handles side effects in functional components, including state-related operations like data fetching,...
State can be shared between components through: 1) Lifting state up to a common ancestor, 2) Using Context API, 3)...
Common pitfalls include: 1) Mutating state directly instead of using setState/useState, 2) Async state updates race...
Redux implements a unidirectional data flow with a single store holding the application state. Actions describe...
Redux Toolkit is the official toolset for Redux development. It simplifies store setup, reduces boilerplate through...
State persistence involves: 1) Using AsyncStorage or similar storage solutions, 2) Implementing redux-persist for...
MobX offers simpler setup than Redux, automatic tracking of state dependencies, and more flexibility in state...
Real-time updates can be handled through: 1) WebSocket connections with state synchronization, 2) Push notifications...
Custom hooks are JavaScript functions that use React hooks to encapsulate reusable state logic. They help abstract...
Form validation state involves: 1) Tracking input values and errors, 2) Implementing validation rules and error...
Middleware intercepts actions before they reach reducers, enabling side effects, async operations, logging, and...
Context optimization includes: 1) Split contexts by functionality, 2) Memoize context values, 3) Use context...
Complex state patterns include: 1) State machines with XState, 2) Command pattern for actions, 3) Observable pattern...
Offline-first state management requires: 1) Local state persistence, 2) Conflict resolution strategies, 3) Queue...
Large-scale state management involves: 1) Domain-driven state organization, 2) State normalization, 3) Module-based...
Multi-window state management requires: 1) State synchronization between windows, 2) Shared storage mechanisms, 3)...
Microservices state management involves: 1) Domain-based state separation, 2) Service boundary definition, 3) State...
Real-time collaboration requires: 1) Operational transformation or CRDT implementation, 2) State conflict...
Complex async patterns include: 1) Saga patterns for orchestration, 2) State machines for workflow management, 3)...
AR/VR state management involves: 1) Real-time state updates for position/orientation, 2) Scene graph state...
React Navigation is a popular navigation library for React Native that provides a way to navigate between screens....
The main types are: 1) Stack Navigator for basic back/forward navigation, 2) Tab Navigator for switching between...
Navigation between screens is done using the navigation prop: navigation.navigate('ScreenName') for basic...
navigate() moves to a screen and avoids duplicating if it's already in the stack, while push() always adds a new...
Parameters can be passed between screens using: navigation.navigate('ScreenName', { paramName: value }) and accessed...
Deep linking allows apps to handle external URLs and open specific screens. It's implemented using linking...
The Android back button can be handled using the useFocusEffect or useBackHandler hooks from...
NavigationContainer is the root component that manages navigation state and integrates with the device's navigation...
Headers can be customized using screenOptions or options props on navigators or individual screens. Options include...
Navigation lifecycle events include focus/blur (when screens gain/lose focus) and beforeRemove (before screen...
Nested navigation involves placing one navigator inside another (e.g., tab navigator inside stack navigator). Each...
Navigation state can be persisted using persistNavigationState and loadNavigationState props on NavigationContainer....
Authentication flows typically use conditional navigation based on auth state, often implementing stack switching or...
Navigation events (focus, blur, beforeRemove, state) can be listened to using addListener. They're useful for...
Custom animations can be implemented using cardStyleInterpolator for stack navigator or custom transition...
Navigation middlewares intercept and modify navigation actions before they're processed. They're useful for logging,...
Deep linking with parameters requires proper linking configuration with parameter extraction from URLs, parameter...
Navigation performance optimization includes: 1) Screen preloading, 2) Lazy loading screens, 3) Minimizing...
Navigation testing involves mocking navigation props, simulating navigation actions, testing deep linking handling,...
Linking configuration defines URL pattern matching for deep links, including parameter extraction and screen...
Complex navigation patterns involve combining multiple navigators, handling nested navigation state, implementing...
State restoration involves persisting navigation state, handling deep links, managing authentication state, and...
Custom navigators require implementing core navigation logic, gesture handling, transition animations, and proper...
Large-scale navigation involves modular navigation configuration, code splitting, proper type definitions, state...
Offline navigation requires state persistence, queue management for navigation actions, proper error handling, and...
Multi-window navigation involves managing separate navigation states, synchronizing navigation between windows,...
Navigation analytics involves tracking screen views, navigation patterns, user flows, and interaction metrics....
AR/VR navigation requires custom transition animations, gesture handling for 3D space, maintaining spatial...
Accessible navigation includes proper focus management, screen reader support, gesture alternatives, and clear...
React.memo is a higher-order component that memoizes functional components to prevent unnecessary re-renders. It...
FlatList is optimized for long lists by implementing windowing, which only renders items currently visible on...
The bridge serializes data between JavaScript and native code, which can impact performance when sending large...
Hermes is a JavaScript engine optimized for React Native that improves start-up time, reduces memory usage, and...
Image optimization includes using proper image sizes, implementing lazy loading, caching images, using FastImage...
useCallback memoizes functions to prevent unnecessary recreation between renders. It's particularly useful when...
console.log statements can significantly impact performance in production by creating unnecessary bridge traffic....
useMemo memoizes computed values to prevent expensive recalculations on every render. It's useful for optimizing...
Bundle size can be reduced by removing unused dependencies, implementing code splitting, using ProGuard/R8 for...
PureComponent implements shouldComponentUpdate with a shallow prop and state comparison, preventing unnecessary...
FlatList optimization includes: 1) Using getItemLayout to avoid measurement, 2) Implementing proper key extraction,...
Bridge traffic can be reduced by: 1) Batching updates, 2) Minimizing state updates, 3) Using native modules for...
Efficient animations involve: 1) Using native driver when possible, 2) Implementing proper interpolation, 3) Using...
Memory management includes: 1) Proper cleanup in useEffect, 2) Removing event listeners, 3) Clearing timeouts and...
Network optimization includes: 1) Implementing proper caching, 2) Using request batching, 3) Implementing retry...
Dependencies impact includes: 1) Bundle size increase, 2) Startup time effects, 3) Runtime performance overhead, 4)...
Efficient state management involves: 1) Proper state structure, 2) Minimizing state updates, 3) Using appropriate...
Startup optimization includes: 1) Using Hermes engine, 2) Implementing proper code splitting, 3) Optimizing native...
Layout optimization includes: 1) Using LayoutAnimation properly, 2) Minimizing layout depth, 3) Implementing proper...
Profiling techniques include: 1) Using React DevTools, 2) Implementing performance monitoring, 3) Using native...
Large-scale optimization involves: 1) Implementing proper architecture, 2) Code splitting strategies, 3) Performance...
Native module optimization includes: 1) Proper thread management, 2) Efficient data serialization, 3) Batch...
Data virtualization involves: 1) Implementing windowing techniques, 2) Managing data chunks, 3) Implementing...
Offline optimization includes: 1) Efficient data synchronization, 2) State persistence strategies, 3) Conflict...
Performance monitoring includes: 1) Metric collection implementation, 2) Analysis system setup, 3) Alert mechanism...
AR/VR optimization includes: 1) Efficient render pipeline, 2) Asset management strategies, 3) Frame rate...
Testing automation includes: 1) Performance benchmark setup, 2) Continuous monitoring implementation, 3) Regression...
Multi-window optimization includes: 1) Resource sharing strategies, 2) State synchronization optimization, 3) Memory...
Platform.select() is a React Native utility that returns platform-specific values. It accepts an object with 'ios',...
React Native automatically picks platform-specific files using extensions like .ios.js or .android.js. For example,...
Platform.OS is a string that identifies the current operating system ('ios' or 'android'). It's used for conditional...
Platform-specific styles can be handled using Platform.select(), platform-specific style files, or conditional style...
React Native provides platform-specific components like DatePickerIOS and ProgressViewIOS for iOS, or...
Platform permissions are handled differently in iOS (Info.plist) and Android (AndroidManifest.xml). React Native...
Android's back button is hardware/software-based and requires explicit handling using BackHandler, while iOS's back...
Font handling differs between platforms in naming conventions and loading mechanisms. iOS uses the font name, while...
Layout differences include status bar height, navigation bar presence, safe areas (iOS), and default spacing...
Gestures can differ between platforms in behavior and implementation. iOS often uses swipe gestures for navigation,...
Native modules require separate implementations in Swift/Objective-C for iOS and Java/Kotlin for Android. This...
iOS typically uses edge swipe gestures and navbar buttons, while Android uses the back button and different...
Animation implementations may need platform-specific tuning for timing, easing, and performance. Native drivers...
Debugging tools and processes differ between platforms. iOS uses Xcode debugging tools while Android uses Android...
Push notifications require different setup and handling for APNs (iOS) and FCM (Android). This includes different...
App lifecycle events and background handling differ between iOS and Android. This includes different background...
Storage mechanisms like AsyncStorage have platform-specific implementations and limitations. Secure storage, file...
Performance optimization requires platform-specific approaches for memory management, UI rendering, and native...
Network security configuration differs between platforms, including SSL pinning, network permissions, and security...
Biometric authentication requires different implementations for Touch ID/Face ID (iOS) and Fingerprint/Face Unlock...
Complex features often require deep platform integration, custom native modules, and platform-specific...
Hardware feature access requires platform-specific implementations for cameras, sensors, Bluetooth, and other...
UI automation requires different tools and approaches (XCTest for iOS, Espresso for Android). This includes...
App extensions (widgets, share extensions, etc.) require platform-specific implementation and configuration. This...
AR features require platform-specific implementations using ARKit (iOS) and ARCore (Android). This includes...
Payment integration requires platform-specific implementations for Apple Pay and Google Pay. This includes different...
Background processing requires different implementations for iOS background modes and Android services. This...
Accessibility implementation requires platform-specific considerations for VoiceOver (iOS) and TalkBack (Android)....
Deep linking requires platform-specific configuration for Universal Links (iOS) and App Links (Android). This...
App signing and deployment differ significantly between iOS (certificates, provisioning profiles) and Android...
StyleSheet is a React Native API for creating and managing styles. It provides performance optimizations by...
React Native uses Flexbox for layout but with some differences from web. The default flexDirection is 'column'...
React Native styling uses JavaScript objects with camelCase properties instead of CSS syntax. There's no inheritance...
Dimensions can be handled using fixed numbers (density-independent pixels), percentages, or the Dimensions API. The...
SafeAreaView is used to handle safe area insets (notches, status bars) on iOS devices. It automatically adds padding...
Responsive layouts are created using flexbox, percentage values, the Dimensions API, and useWindowDimensions hook....
Style arrays allow multiple styles to be applied to a component: [styles.container, styles.modified]. Later styles...
Text styling in React Native is not inherited by default. Each Text component needs its own style. Text-specific...
Width and height set fixed dimensions, while flex determines how components grow/shrink within available space. Flex...
Images can be sized using width/height properties or resizeMode prop ('contain', 'cover', 'stretch', 'center'). For...
Custom fonts require platform-specific setup: iOS needs font files added to the Xcode project and Info.plist,...
Dynamic styling can be implemented using style arrays, conditional styles in objects, or dynamic style generation...
Orientation changes require responsive layouts using flex, Dimensions API updates handling, and potentially...
Shadows require different implementations: iOS uses shadow* properties, Android uses elevation. Platform-specific...
KeyboardAvoidingView component with appropriate behavior prop ('height', 'position', 'padding') handles keyboard...
Grid layouts can be implemented using FlatList with numColumns, flexbox with flex-wrap, or custom grid components....
RTL support requires proper use of start/end instead of left/right, handling text alignment, and considering layout...
Screen density handling involves using density-independent pixels, proper image asset management (including @2x,...
Animated layouts use LayoutAnimation or Animated API. LayoutAnimation provides simple animations for layout changes....
Style performance considerations include using StyleSheet.create, avoiding inline styles, minimizing style...
Complex layouts require combination of flexbox, absolute positioning, proper nesting, and possibly custom native...
Design systems implementation involves creating reusable components, centralized theme management, consistent...
Complex interactions require combination of Animated API, PanResponder, proper layout measurement, and performance...
Custom graphics require SVG implementation, canvas-like solutions, or native modules. Consider performance...
Advanced responsive layouts involve dynamic layout switching, complex grid systems, proper breakpoint management,...
Custom transitions require understanding of the Animated API, proper timing functions, layout measurement, and...
Advanced theming involves dynamic theme switching, proper context usage, style generation systems, and efficient...
Micro-interactions require fine-tuned animations, proper gesture handling, and efficient state management. Consider...
Custom layout engines require understanding of native modules, layout calculation systems, and proper integration...
API calls in React Native can be made using the Fetch API or Axios library. The Fetch API is built into React Native...
useEffect is commonly used for making API calls when a component mounts or when dependencies change. It helps manage...
API errors should be caught using try/catch blocks or .catch() for promises. Error states should be stored in...
GET requests retrieve data and include parameters in the URL query string. POST requests send data in the request...
Loading states should be managed in component state (e.g., isLoading boolean). Show loading indicators while data is...
JSON (JavaScript Object Notation) is a data format used for API requests/responses. React Native can parse JSON...
Headers can be set using the headers object in fetch options or Axios config. Common headers include Authorization...
REST (Representational State Transfer) is an architectural style for APIs using HTTP methods (GET, POST, PUT,...
API authentication typically involves sending tokens in request headers, often using JWT (JSON Web Tokens). Tokens...
Query parameters are key-value pairs added to URLs for filtering, sorting, or pagination. They're commonly used in...
API caching can be implemented using in-memory caching, AsyncStorage, or specialized caching libraries. Consider...
Offline sync requires local storage of data, queue management for offline actions, conflict resolution strategies,...
File uploads require proper multipart/form-data handling, progress tracking, chunked uploads for large files, and...
Request cancellation can be implemented using AbortController with fetch, or cancelToken with Axios. Important for...
Rate limiting requires implementing request queuing, proper error handling for rate limit responses, exponential...
WebSockets provide real-time bidirectional communication. Implementation involves socket connection management,...
API versioning strategies include URL versioning, header versioning, or content negotiation. Apps should handle...
Security considerations include SSL/TLS, token management, certificate pinning, request/response encryption, and...
Response compression involves proper handling of Content-Encoding headers, decompression of gzipped responses, and...
Request batching involves combining multiple requests into single requests, implementing proper request queuing, and...
GraphQL implementation involves setting up Apollo Client or similar libraries, implementing proper cache management,...
Real-time sync involves WebSockets, server-sent events, or polling strategies. Consider optimistic updates, conflict...
Complex transformations require proper data normalization, efficient algorithms, consideration of performance...
Large-scale architectures require proper service organization, API gateway implementation, microservices...
Efficient pagination involves cursor-based or offset-based strategies, proper cache management, infinite scroll...
API middleware implementation involves request/response interceptors, logging, analytics tracking, error handling,...
Advanced caching involves implementing stale-while-revalidate, cache-then-network, and proper cache invalidation...
Complex authentication involves OAuth flows, token refresh mechanisms, biometric authentication integration, and...
API monitoring involves implementing proper logging, performance tracking, error reporting, and usage analytics....
API testing involves implementing proper test coverage, mocking strategies, integration tests, and automated testing...
React Native is a framework developed by Facebook that allows developers to build native mobile applications using JavaScript and React. Unlike React which creates a virtual DOM for browser rendering, React Native creates actual native UI components that are rendered on mobile devices. This means React Native apps have native performance while still allowing cross-platform development using a single codebase.
React Native provides several core components that map directly to native UI elements: View (container), Text (text display), Image (image display), ScrollView (scrollable container), TextInput (text input field), TouchableOpacity/TouchableHighlight (touchable elements), and FlatList/SectionList (optimized scrollable lists).
AppRegistry is the entry point to run a React Native application. It provides the registerComponent method used to register the root component of the application. This registration tells React Native which component to render when the application starts, similar to ReactDOM.render in web applications.
Expo provides a managed workflow with pre-built components and services, making it easier to start but with limited native module access. React Native CLI offers a bare workflow with full native code access but requires more setup and native development knowledge.
Metro is React Native's JavaScript bundler that combines all JavaScript code and dependencies into a single file. It handles transpilation, asset management, and provides hot reloading during development. It's optimized specifically for React Native's mobile environment needs.
React Native offers multiple debugging options: Chrome Developer Tools for JavaScript debugging, React Developer Tools for component inspection, built-in Debug menu on devices, and console logging. It also supports remote debugging and various third-party debugging tools.
package.json manages project dependencies, scripts, and configuration. It lists all npm packages required by the project, defines scripts for development and building, and contains metadata about the project including name, version, and license information.
React Native runs on three main threads: 1) Main Thread (Native UI), 2) JavaScript Thread (JS execution), and 3) Shadow Thread (layout calculations). These threads work together through the bridge to create the native application experience.
babel.config.js configures Babel transpilation settings for the project. It defines how modern JavaScript features are converted to compatible code, handles JSX transformation, and can include various plugins and presets for additional functionality.
Hot Reloading updates only the changed components while maintaining the app's state. Live Reloading refreshes the entire app and resets state when code changes. Hot Reloading is more efficient during development as it preserves the development flow.
The bridge is the communication layer between JavaScript and native code. It serializes data and handles asynchronous communication between JS and native threads. All native module calls and UI updates pass through the bridge, which can impact performance with heavy data transfers.
React Native provides Platform.select(), platform-specific file extensions (.ios.js/.android.js), Platform.OS checks, and platform-specific components. These methods allow writing platform-specific logic while maintaining a shared codebase.
Native modules expose platform-specific APIs to JavaScript through the bridge. They're used when requiring direct access to platform APIs, implementing performance-critical features, or integrating third-party SDKs that don't have React Native implementations.
The Shadow Thread handles layout calculations using Yoga (Facebook's cross-platform layout engine). It processes flex layouts and converts them to native layouts, running separately from the main thread to ensure smooth UI performance.
iOS uses JavaScriptCore (JSC) by default, while Android can use either JSC or Hermes. Hermes is Facebook's custom JS engine optimized for React Native, offering better performance, reduced memory usage, and faster startup times.
Linking handles deep linking and URL scheme integration in React Native apps. It provides methods to open external links and handle incoming deep links, enabling integration with other apps and web content.
React Native provides APIs to request and check platform permissions (camera, location, etc.). These are handled through native modules and require configuration in platform-specific files (AndroidManifest.xml, Info.plist).
metro.config.js customizes the Metro bundler's behavior, including module resolution, asset handling, and transformation settings. It can be modified to support different file types, customize bundling, and optimize build performance.
Assets are handled through the Metro bundler, which can process images, fonts, and other resources. Platform-specific asset selection is supported through asset suffixes, and the require syntax is used for static asset references.
The New Architecture introduces Fabric (new rendering system) and TurboModules (improved native modules). It reduces bridge overhead, improves performance, and enables better native integration through codegen and static typing.
Fabric is a C++ rendering system that enables synchronous operations between JS and native code. It introduces a new threading model, improves layout performance, and enables concurrent rendering features.
TurboModules provide direct JS to native communication without serialization overhead. They use codegen to create type-safe interfaces, lazy load modules, and enable better memory management compared to traditional native modules.
React Native manages memory through automatic garbage collection in JS and ARC/GC in native code. Understanding the bridge's role in memory usage, implementing proper cleanup in components, and monitoring memory leaks are crucial.
JSI enables direct communication between JavaScript and native code without going through the bridge. It provides a way to hold native references in JavaScript and enables synchronous native method calls.
Native crashes are handled through platform-specific crash reporting tools and services. Integration requires native module setup, symbolication for JavaScript stack traces, and proper error boundary implementation.
Security involves handling data encryption, secure storage, certificate pinning, code obfuscation, and protecting against common mobile vulnerabilities. Platform-specific security features must be properly implemented.
App initialization involves native bootstrap, JS engine initialization, bundle loading, and component mounting. Understanding this process is crucial for optimizing startup time and implementing splash screens.
React Native has two types of components: 1) Class Components - ES6 classes that extend React.Component and have lifecycle methods, state management, and render method. 2) Functional Components - JavaScript functions that accept props and return React elements, commonly used with hooks in modern React Native development.
Props (properties) are read-only components that are passed from a parent component to a child component. They are used to customize and configure components, allowing for component reusability and maintaining the one-way data flow architecture in React Native applications.
View is a container component that works like a div in web development, used for layouting and styling. Text is specifically designed for displaying text content and text-specific styling. Text components must be used to render any string content in React Native.
SafeAreaView is a component that automatically adds padding to accommodate notches, status bars, and other device-specific screen elements. It ensures content is displayed within the visible area of the device, particularly important for iOS devices with notches.
Touch events are handled using components like TouchableOpacity, TouchableHighlight, TouchableWithoutFeedback, and Pressable. These components provide visual feedback and handle various touch interactions like taps, long presses, and press in/out events.
FlatList is optimized for long lists of data, rendering items lazily and only what's visible on screen. ScrollView renders all its child components at once, making it suitable for a small number of items. FlatList provides better performance for long lists but requires more setup.
Data can be passed between components using props for parent-to-child communication, callback functions for child-to-parent communication, and context or state management solutions for components that aren't directly related.
The Image component is used to display images in React Native. It can handle both local and remote images, supports various image formats, provides loading and error states, and includes properties for resizing and styling images.
Style props are used to customize the appearance of components. They can be applied directly as props or through StyleSheet.create(). React Native uses a subset of CSS properties with camelCase naming convention and some platform-specific properties.
KeyboardAvoidingView is a component that adjusts its height or position based on the keyboard height to prevent the keyboard from overlapping with input fields. It's particularly useful for forms and input-heavy screens on iOS devices.
Component composition involves building larger components from smaller ones using props.children, render props, and higher-order components. This promotes code reuse, maintainability, and separation of concerns in the application architecture.
Refs provide a way to access DOM nodes or React elements directly. They should be used sparingly for cases like managing focus, text selection, animations, or integrating with third-party libraries. Refs should not be used for tasks that can be handled through props and state.
FlatList performance can be optimized by: 1) Using getItemLayout to avoid measurement of items, 2) Implementing windowSize and maxToRenderPerBatch, 3) Using removeClippedSubviews, 4) Implementing proper key extraction, and 5) Optimizing renderItem function with memo or PureComponent.
Controlled components have their state managed by React through props and callbacks (e.g., value and onChangeText). Uncontrolled components maintain their own internal state. Controlled components provide more predictable behavior but require more setup.
Modern React Native uses hooks like useEffect for lifecycle management. useEffect replaces componentDidMount, componentDidUpdate, and componentWillUnmount. The dependency array controls when effects run, and cleanup functions handle teardown.
PureComponent implements shouldComponentUpdate with a shallow prop and state comparison. It's used to optimize performance by preventing unnecessary renders when props or state haven't changed. Should be used for components with simple props/state structures.
Prop validation is handled using PropTypes or TypeScript. PropTypes provide runtime checking of prop types, while TypeScript offers compile-time type checking. Both help catch bugs early and improve code documentation.
HOCs are functions that take a component and return a new component with additional props or behavior. They're used for code reuse, adding functionality like authentication, logging, or data fetching. HOCs follow the principle of composition over inheritance.
Modal dialogs can be implemented using the Modal component or third-party libraries. Considerations include proper animation handling, backdrop press handling, accessibility, and platform-specific behaviors like hardware back button on Android.
Render props are a pattern where a component receives a function prop that returns React elements. Unlike HOCs, render props provide more flexibility in composing behavior and avoid naming collisions. They're useful for sharing stateful logic between components.
Custom native components require native code implementation (Java/Kotlin for Android, Objective-C/Swift for iOS) and JavaScript interface. This involves creating native view managers, implementing view properties, and handling events through the bridge.
Rerendering optimization involves: 1) Using React.memo for functional components, 2) Implementing shouldComponentUpdate for class components, 3) Proper key usage, 4) State structure optimization, 5) Callback memoization with useCallback, and 6) Value memoization with useMemo.
Virtualized list challenges include: 1) Memory management for large datasets, 2) Smooth scrolling performance, 3) Handling variable height items, 4) Implementing pull-to-refresh and infinite scroll, 5) Managing scroll position restoration, and 6) Platform-specific optimizations.
Complex gestures require the PanResponder system or gesture libraries. Implementation involves handling gesture recognition, touch responder negotiation, animation integration, and proper cleanup. Consider interaction with scrolling views and native gesture handlers.
Lazy loading strategies include: 1) React.lazy with Suspense, 2) Dynamic imports, 3) Route-based code splitting, 4) Component prefetching, and 5) Loading state management. Consider bundle size impact and user experience during loading.
Error boundaries are components that catch JavaScript errors in their child component tree. Implementation involves getDerivedStateFromError and componentDidCatch lifecycle methods, fallback UI rendering, and error reporting integration.
Accessibility implementation includes: 1) Proper use of accessibility props, 2) Semantic markup, 3) Focus management, 4) Screen reader support, 5) Color contrast, 6) Touch target sizing, and 7) Platform-specific accessibility guidelines.
State is a mutable data store internal to a component that determines the component's behavior and rendering. Unlike props which are read-only and passed from parent to child, state can be modified using setState() in class components or state updater functions in hooks, triggering re-renders when changed.
useState is a React hook that adds state management to functional components. It returns an array with two elements: the current state value and a function to update it. The hook accepts an initial state value and can be used multiple times in a single component for different state variables.
In class components, state is updated using this.setState(). It can accept either an object with new state values or a function that receives previous state as an argument. setState() performs shallow merging and updates are asynchronous for performance optimization.
useState is simpler and good for managing independent pieces of state. useReducer is preferred for complex state logic, especially when state updates depend on multiple factors or when one action should update multiple state values. useReducer follows a Redux-like pattern with actions and reducers.
AsyncStorage is a simple, unencrypted, asynchronous, persistent, key-value storage system that is global to the app. It's commonly used for storing user preferences, tokens, and other data that needs to persist across app restarts.
Context API provides a way to pass data through the component tree without manually passing props. It's useful for sharing global data like themes, user authentication, or language preferences. Should be used when data needs to be accessible by many components at different nesting levels.
Form state can be handled using controlled components with useState, or through form management libraries like Formik or React Hook Form. State typically includes input values, validation errors, and form submission status.
useEffect handles side effects in functional components, including state-related operations like data fetching, subscriptions, or manual DOM manipulations. It runs after render and can clean up effects by returning a function.
State can be shared between components through: 1) Lifting state up to a common ancestor, 2) Using Context API, 3) Implementing state management libraries like Redux or MobX, 4) Using custom hooks for shared state logic.
Common pitfalls include: 1) Mutating state directly instead of using setState/useState, 2) Async state updates race conditions, 3) Over-using global state, 4) Not considering component re-renders, 5) Improper state structure leading to performance issues.
Redux implements a unidirectional data flow with a single store holding the application state. Actions describe state changes, reducers specify how actions transform state, and components connect to the store using hooks or HOCs. Middleware handles side effects.
Redux Toolkit is the official toolset for Redux development. It simplifies store setup, reduces boilerplate through createSlice, handles immutable updates with Immer, and includes RTK Query for data fetching. It provides utilities for common Redux patterns.
State persistence involves: 1) Using AsyncStorage or similar storage solutions, 2) Implementing redux-persist for Redux state, 3) Handling storage serialization/deserialization, 4) Managing storage versioning, 5) Implementing proper error handling and fallbacks.
MobX offers simpler setup than Redux, automatic tracking of state dependencies, and more flexibility in state structure. Drawbacks include potential overuse of observables, harder debugging due to automatic updates, and less predictable state changes.
Real-time updates can be handled through: 1) WebSocket connections with state synchronization, 2) Push notifications with state updates, 3) Polling with proper state merging, 4) Optimistic updates with rollback handling.
Custom hooks are JavaScript functions that use React hooks to encapsulate reusable state logic. They help abstract complex state management, share stateful logic between components, and improve code organization and reusability.
Form validation state involves: 1) Tracking input values and errors, 2) Implementing validation rules and error messages, 3) Managing submission state, 4) Handling async validation, 5) Coordinating multiple form fields' validation state.
Middleware intercepts actions before they reach reducers, enabling side effects, async operations, logging, and routing. Common middleware includes redux-thunk for async actions, redux-saga for complex workflows, and custom middleware for specific needs.
Context optimization includes: 1) Split contexts by functionality, 2) Memoize context values, 3) Use context selectors to prevent unnecessary rerenders, 4) Implement context providers at appropriate levels, 5) Consider alternative solutions for frequently changing values.
Complex state patterns include: 1) State machines with XState, 2) Command pattern for actions, 3) Observable pattern for state streams, 4) Reducer composition for modular state logic, 5) Event sourcing for state history.
Offline-first state management requires: 1) Local state persistence, 2) Conflict resolution strategies, 3) Queue management for offline actions, 4) State synchronization upon reconnection, 5) Optimistic UI updates with proper error handling.
Large-scale state management involves: 1) Domain-driven state organization, 2) State normalization, 3) Module-based state splitting, 4) Lazy loading state modules, 5) State hydration strategies, 6) Performance optimization patterns.
Multi-window state management requires: 1) State synchronization between windows, 2) Shared storage mechanisms, 3) Event-based communication, 4) Consistent state updates across windows, 5) Handle window lifecycle events.
Microservices state management involves: 1) Domain-based state separation, 2) Service boundary definition, 3) State consistency patterns, 4) Event-driven state updates, 5) Distributed state management strategies.
Real-time collaboration requires: 1) Operational transformation or CRDT implementation, 2) State conflict resolution, 3) Presence management, 4) State versioning, 5) Optimistic updates with proper conflict handling.
Complex async patterns include: 1) Saga patterns for orchestration, 2) State machines for workflow management, 3) Queue-based state updates, 4) Retry and recovery strategies, 5) Transaction-like state updates.
AR/VR state management involves: 1) Real-time state updates for position/orientation, 2) Scene graph state management, 3) Object interaction state, 4) Performance optimization for state updates, 5) Platform-specific state handling.
React.memo is a higher-order component that memoizes functional components to prevent unnecessary re-renders. It performs a shallow comparison of props and only re-renders if props have changed, improving performance by reducing render cycles for components with stable props.
FlatList is optimized for long lists by implementing windowing, which only renders items currently visible on screen. It provides better performance than ScrollView for long lists by recycling DOM elements and managing memory efficiently.
The bridge serializes data between JavaScript and native code, which can impact performance when sending large amounts of data. Minimizing bridge traffic by batching updates and reducing unnecessary communication improves app performance.
Hermes is a JavaScript engine optimized for React Native that improves start-up time, reduces memory usage, and decreases app size. It provides better performance than traditional JavaScript engines through ahead-of-time compilation and optimized bytecode.
Image optimization includes using proper image sizes, implementing lazy loading, caching images, using FastImage component for better performance, and implementing progressive loading for large images. Also consider using image compression and proper formats.
useCallback memoizes functions to prevent unnecessary recreation between renders. It's particularly useful when passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders.
console.log statements can significantly impact performance in production by creating unnecessary bridge traffic. They should be removed or disabled in production builds using babel plugins or proper configuration.
useMemo memoizes computed values to prevent expensive recalculations on every render. It's useful for optimizing performance when dealing with complex calculations or data transformations that don't need to be recomputed unless dependencies change.
Bundle size can be reduced by removing unused dependencies, implementing code splitting, using ProGuard/R8 for Android, enabling Hermes, implementing tree shaking, and properly configuring the Metro bundler.
PureComponent implements shouldComponentUpdate with a shallow prop and state comparison, preventing unnecessary renders. It's useful for optimizing class components when you know that shallow comparison is sufficient for determining updates.
FlatList optimization includes: 1) Using getItemLayout to avoid measurement, 2) Implementing proper key extraction, 3) Using removeClippedSubviews, 4) Optimizing renderItem with memo, 5) Adjusting windowSize and maxToRenderPerBatch, 6) Implementing proper item height calculations.
Bridge traffic can be reduced by: 1) Batching updates, 2) Minimizing state updates, 3) Using native modules for heavy computations, 4) Implementing proper data serialization, 5) Using Hermes engine, 6) Optimizing image loading and processing.
Efficient animations involve: 1) Using native driver when possible, 2) Implementing proper interpolation, 3) Using layoutAnimation for simple layouts, 4) Optimizing gesture handling, 5) Managing animation memory usage, 6) Using proper timing functions.
Memory management includes: 1) Proper cleanup in useEffect, 2) Removing event listeners, 3) Clearing timeouts and intervals, 4) Implementing proper image caching, 5) Managing large data structures, 6) Monitoring memory leaks.
Network optimization includes: 1) Implementing proper caching, 2) Using request batching, 3) Implementing retry logic, 4) Optimizing payload size, 5) Using proper compression, 6) Implementing offline support, 7) Managing concurrent requests.
Dependencies impact includes: 1) Bundle size increase, 2) Startup time effects, 3) Runtime performance overhead, 4) Memory usage implications, 5) Bridge traffic increase. Proper dependency management and auditing is crucial.
Efficient state management involves: 1) Proper state structure, 2) Minimizing state updates, 3) Using appropriate state management tools, 4) Implementing proper memoization, 5) Managing state persistence efficiently.
Startup optimization includes: 1) Using Hermes engine, 2) Implementing proper code splitting, 3) Optimizing native module initialization, 4) Managing asset loading, 5) Implementing proper splash screen, 6) Reducing initial bundle size.
Layout optimization includes: 1) Using LayoutAnimation properly, 2) Minimizing layout depth, 3) Implementing proper flex layouts, 4) Managing dynamic layouts efficiently, 5) Using proper measurement techniques.
Profiling techniques include: 1) Using React DevTools, 2) Implementing performance monitoring, 3) Using native profiling tools, 4) Analyzing bridge traffic, 5) Monitoring memory usage, 6) Implementing proper logging.
Large-scale optimization involves: 1) Implementing proper architecture, 2) Code splitting strategies, 3) Performance monitoring systems, 4) Efficient state management, 5) Proper resource management, 6) Optimization automation.
Native module optimization includes: 1) Proper thread management, 2) Efficient data serialization, 3) Batch processing implementation, 4) Memory management strategies, 5) Proper error handling, 6) Performance benchmarking.
Data virtualization involves: 1) Implementing windowing techniques, 2) Managing data chunks, 3) Implementing efficient scrolling, 4) Memory management strategies, 5) Optimizing render performance, 6) Handling large datasets.
Offline optimization includes: 1) Efficient data synchronization, 2) State persistence strategies, 3) Conflict resolution handling, 4) Resource management, 5) Background processing optimization, 6) Storage optimization.
Performance monitoring includes: 1) Metric collection implementation, 2) Analysis system setup, 3) Alert mechanism creation, 4) Performance tracking automation, 5) Reporting system implementation, 6) Optimization feedback loops.
AR/VR optimization includes: 1) Efficient render pipeline, 2) Asset management strategies, 3) Frame rate optimization, 4) Memory usage management, 5) Thread management, 6) Native integration optimization.
Testing automation includes: 1) Performance benchmark setup, 2) Continuous monitoring implementation, 3) Regression testing strategies, 4) Automated optimization verification, 5) Performance metric tracking, 6) Test environment management.
Multi-window optimization includes: 1) Resource sharing strategies, 2) State synchronization optimization, 3) Memory management approaches, 4) Process communication efficiency, 5) UI thread management, 6) Context switching optimization.
Platform.select() is a React Native utility that returns platform-specific values. It accepts an object with 'ios', 'android', and 'default' keys, returning the value that matches the current platform. It's commonly used for platform-specific styles, components, or behavior.
React Native automatically picks platform-specific files using extensions like .ios.js or .android.js. For example, Component.ios.js for iOS and Component.android.js for Android. This allows maintaining separate implementations while keeping a common interface.
Platform.OS is a string that identifies the current operating system ('ios' or 'android'). It's used for conditional rendering and logic based on the platform, allowing developers to handle platform-specific differences in code.
Platform-specific styles can be handled using Platform.select(), platform-specific style files, or conditional style objects. This includes handling different shadow implementations, native components styling, and platform-specific measurements.
React Native provides platform-specific components like DatePickerIOS and ProgressViewIOS for iOS, or DatePickerAndroid and ProgressBarAndroid for Android. These components are designed to match native platform UI guidelines and behavior.
Platform permissions are handled differently in iOS (Info.plist) and Android (AndroidManifest.xml). React Native provides APIs to request permissions at runtime, but setup and configuration must be done separately for each platform.
Android's back button is hardware/software-based and requires explicit handling using BackHandler, while iOS's back gesture is part of navigation and handled automatically by navigation libraries. Different approaches are needed for consistent behavior.
Font handling differs between platforms in naming conventions and loading mechanisms. iOS uses the font name, while Android uses the file name. Custom fonts require different setup in Xcode and Android assets folder.
Layout differences include status bar height, navigation bar presence, safe areas (iOS), and default spacing behaviors. These need to be handled using platform-specific components like SafeAreaView and appropriate styling.
Gestures can differ between platforms in behavior and implementation. iOS often uses swipe gestures for navigation, while Android relies more on the back button. PanResponder and gesture handlers need platform-specific configuration.
Native modules require separate implementations in Swift/Objective-C for iOS and Java/Kotlin for Android. This includes proper method mapping, event handling, and data type conversion while maintaining a consistent JavaScript interface.
iOS typically uses edge swipe gestures and navbar buttons, while Android uses the back button and different navigation patterns. Navigation libraries need platform-specific configuration for proper behavior and animations.
Animation implementations may need platform-specific tuning for timing, easing, and performance. Native drivers might work differently, and some animations may need platform-specific implementations for optimal performance.
Debugging tools and processes differ between platforms. iOS uses Xcode debugging tools while Android uses Android Studio. Chrome Developer Tools work differently, and native debugging requires platform-specific approaches.
Push notifications require different setup and handling for APNs (iOS) and FCM (Android). This includes different configuration, payload structures, and handling mechanisms while maintaining a consistent app experience.
App lifecycle events and background handling differ between iOS and Android. This includes different background modes, state restoration, and process lifecycle management requiring platform-specific implementations.
Storage mechanisms like AsyncStorage have platform-specific implementations and limitations. Secure storage, file system access, and data persistence require different approaches and configurations per platform.
Performance optimization requires platform-specific approaches for memory management, UI rendering, and native module optimization. Different profiling tools and optimization techniques are needed for each platform.
Network security configuration differs between platforms, including SSL pinning, network permissions, and security policies. Different configuration files and implementation approaches are needed for each platform.
Biometric authentication requires different implementations for Touch ID/Face ID (iOS) and Fingerprint/Face Unlock (Android). This includes different APIs, security models, and user experience considerations.
Complex features often require deep platform integration, custom native modules, and platform-specific optimizations. This includes handling different APIs, lifecycle management, and maintaining consistent behavior across platforms.
Hardware feature access requires platform-specific implementations for cameras, sensors, Bluetooth, and other hardware components. This includes different APIs, permissions, and optimization strategies.
UI automation requires different tools and approaches (XCTest for iOS, Espresso for Android). This includes different test writing strategies, CI/CD integration, and handling platform-specific UI elements.
App extensions (widgets, share extensions, etc.) require platform-specific implementation and configuration. This includes different development approaches, data sharing mechanisms, and lifecycle management.
AR features require platform-specific implementations using ARKit (iOS) and ARCore (Android). This includes different initialization, tracking, and rendering approaches while maintaining consistent AR experiences.
Payment integration requires platform-specific implementations for Apple Pay and Google Pay. This includes different APIs, security requirements, and user experience considerations while maintaining consistent payment flows.
Background processing requires different implementations for iOS background modes and Android services. This includes handling different lifecycle events, scheduling mechanisms, and power management considerations.
Accessibility implementation requires platform-specific considerations for VoiceOver (iOS) and TalkBack (Android). This includes different markup, focus management, and gesture handling while maintaining consistent accessibility.
Deep linking requires platform-specific configuration for Universal Links (iOS) and App Links (Android). This includes different setup processes, validation requirements, and handling mechanisms.
App signing and deployment differ significantly between iOS (certificates, provisioning profiles) and Android (keystore). This includes different build processes, signing mechanisms, and deployment procedures.
StyleSheet is a React Native API for creating and managing styles. It provides performance optimizations by validating styles at compile time, converting them to atomic IDs, and preventing new object creation on rerenders. It also provides better error checking and auto-completion support.
React Native uses Flexbox for layout but with some differences from web. The default flexDirection is 'column' instead of 'row'. Flexbox properties like flex, flexDirection, justifyContent, and alignItems are used to create flexible layouts that work across different screen sizes.
React Native styling uses JavaScript objects with camelCase properties instead of CSS syntax. There's no inheritance of styles like CSS, no cascading, and no selectors. Units are unitless numbers (no px, em, etc.), and not all CSS properties are supported.
Dimensions can be handled using fixed numbers (density-independent pixels), percentages, or the Dimensions API. The useWindowDimensions hook provides responsive dimensions. flex properties are commonly used for dynamic sizing.
SafeAreaView is used to handle safe area insets (notches, status bars) on iOS devices. It automatically adds padding to avoid overlay with system UI elements. It's essential for proper layout on modern iOS devices with notches or home indicators.
Responsive layouts are created using flexbox, percentage values, the Dimensions API, and useWindowDimensions hook. Platform.select can be used for platform-specific layouts, and dynamic styling based on screen size helps ensure proper display across devices.
Style arrays allow multiple styles to be applied to a component: [styles.container, styles.modified]. Later styles override earlier ones. This is useful for conditional styling and style composition. Arrays can include both StyleSheet styles and inline style objects.
Text styling in React Native is not inherited by default. Each Text component needs its own style. Text-specific properties like fontSize, fontWeight, lineHeight are available. Custom fonts require platform-specific setup.
Width and height set fixed dimensions, while flex determines how components grow/shrink within available space. Flex is preferred for responsive layouts as it adapts to different screen sizes. Fixed dimensions should be used sparingly.
Images can be sized using width/height properties or resizeMode prop ('contain', 'cover', 'stretch', 'center'). For network images, dimensions must be specified explicitly. Image sizing should consider aspect ratio and responsive layout needs.
Custom fonts require platform-specific setup: iOS needs font files added to the Xcode project and Info.plist, Android needs fonts in android/app/src/main/assets/fonts. React Native config needs updating, and linking may be required. Font names might differ between platforms.
Dynamic styling can be implemented using style arrays, conditional styles in objects, or dynamic style generation functions. StyleSheet.create should be used outside component render for performance. Consider memoization for complex style calculations.
Orientation changes require responsive layouts using flex, Dimensions API updates handling, and potentially different layouts for portrait/landscape. useWindowDimensions hook helps track dimensions, and specific orientation styles may be needed.
Shadows require different implementations: iOS uses shadow* properties, Android uses elevation. Platform-specific shadow styles are needed for consistent appearance. Consider using libraries for cross-platform shadow implementation.
KeyboardAvoidingView component with appropriate behavior prop ('height', 'position', 'padding') handles keyboard overlap. Platform-specific adjustments may be needed. Consider scroll view integration and input accessibility.
Grid layouts can be implemented using FlatList with numColumns, flexbox with flex-wrap, or custom grid components. Consider performance for large grids, responsive behavior, and proper item sizing calculations.
RTL support requires proper use of start/end instead of left/right, handling text alignment, and considering layout direction. Use I18nManager for RTL control, and test layouts in both directions.
Screen density handling involves using density-independent pixels, proper image asset management (including @2x, @3x), and responsive design practices. Consider platform-specific density differences and testing on various devices.
Animated layouts use LayoutAnimation or Animated API. LayoutAnimation provides simple animations for layout changes. Complex animations might require Animated API with proper measurement and layout calculation.
Style performance considerations include using StyleSheet.create, avoiding inline styles, minimizing style calculations in render, proper use of flex for layouts, and implementing efficient dynamic styling strategies.
Complex layouts require combination of flexbox, absolute positioning, proper nesting, and possibly custom native components. Consider performance implications, maintainability, and cross-platform consistency.
Design systems implementation involves creating reusable components, centralized theme management, consistent spacing/typography systems, and proper component composition. Consider maintenance, scalability, and team collaboration.
Complex interactions require combination of Animated API, PanResponder, proper layout measurement, and performance optimization. Consider native driver usage, gesture handling, and proper cleanup of animations.
Custom graphics require SVG implementation, canvas-like solutions, or native modules. Consider performance implications, platform differences, and proper integration with React Native's layout system.
Advanced responsive layouts involve dynamic layout switching, complex grid systems, proper breakpoint management, and orientation handling. Consider performance, maintainability, and testing across different devices.
Custom transitions require understanding of the Animated API, proper timing functions, layout measurement, and platform-specific considerations. Consider performance, gesture integration, and proper cleanup.
Advanced theming involves dynamic theme switching, proper context usage, style generation systems, and efficient updates. Consider performance implications, maintainability, and proper typing support.
Micro-interactions require fine-tuned animations, proper gesture handling, and efficient state management. Consider performance, user feedback, and proper integration with the overall UX.
Custom layout engines require understanding of native modules, layout calculation systems, and proper integration with React Native. Consider performance implications, maintenance complexity, and cross-platform support.
API calls in React Native can be made using the Fetch API or Axios library. The Fetch API is built into React Native and supports promises, while Axios provides additional features like request/response interceptors, automatic JSON transformation, and better error handling.
useEffect is commonly used for making API calls when a component mounts or when dependencies change. It helps manage side effects, cleanup functions for canceling requests, and proper data fetching lifecycle. The dependency array controls when the effect reruns.
API errors should be caught using try/catch blocks or .catch() for promises. Error states should be stored in component state, displayed to users appropriately, and logged for debugging. Consider implementing retry logic and proper error boundaries.
GET requests retrieve data and include parameters in the URL query string. POST requests send data in the request body and are used for creating/updating resources. GET requests should be idempotent, while POST requests can modify server state.
Loading states should be managed in component state (e.g., isLoading boolean). Show loading indicators while data is being fetched, handle errors appropriately, and ensure good UX during loading. Consider skeleton screens or placeholder content.
JSON (JavaScript Object Notation) is a data format used for API requests/responses. React Native can parse JSON using JSON.parse() and stringify using JSON.stringify(). Most modern APIs use JSON for data exchange.
Headers can be set using the headers object in fetch options or Axios config. Common headers include Authorization for authentication tokens, Content-Type for specifying data format, and Accept for specifying expected response format.
REST (Representational State Transfer) is an architectural style for APIs using HTTP methods (GET, POST, PUT, DELETE). It provides standardized ways to interact with resources, making APIs predictable and easier to understand.
API authentication typically involves sending tokens in request headers, often using JWT (JSON Web Tokens). Tokens should be stored securely (e.g., AsyncStorage), refreshed when needed, and included in requests via interceptors.
Query parameters are key-value pairs added to URLs for filtering, sorting, or pagination. They're commonly used in GET requests to modify the response. Parameters should be properly encoded using encodeURIComponent() when necessary.
API caching can be implemented using in-memory caching, AsyncStorage, or specialized caching libraries. Consider cache invalidation strategies, TTL (Time To Live), and proper cache updates when data changes.
Offline sync requires local storage of data, queue management for offline actions, conflict resolution strategies, and proper sync when online. Consider using libraries like WatermelonDB or implementing custom sync logic.
File uploads require proper multipart/form-data handling, progress tracking, chunked uploads for large files, and proper error handling. Consider platform-specific file access APIs and compression before upload.
Request cancellation can be implemented using AbortController with fetch, or cancelToken with Axios. Important for preventing memory leaks and unnecessary updates when components unmount or dependencies change.
Rate limiting requires implementing request queuing, proper error handling for rate limit responses, exponential backoff for retries, and tracking request counts. Consider using libraries for request throttling.
WebSockets provide real-time bidirectional communication. Implementation involves socket connection management, proper event handling, reconnection strategies, and state management for real-time data.
API versioning strategies include URL versioning, header versioning, or content negotiation. Apps should handle different API versions gracefully, possibly supporting multiple versions during transition periods.
Security considerations include SSL/TLS, token management, certificate pinning, request/response encryption, and proper error handling. Avoid storing sensitive data in plain text and implement proper authentication flows.
Response compression involves proper handling of Content-Encoding headers, decompression of gzipped responses, and optimization of data transfer. Consider implementing compression for requests when appropriate.
Request batching involves combining multiple requests into single requests, implementing proper request queuing, and handling responses efficiently. Consider trade-offs between reduced network calls and increased complexity.
GraphQL implementation involves setting up Apollo Client or similar libraries, implementing proper cache management, handling queries/mutations, and managing local state. Consider code generation and type safety.
Real-time sync involves WebSockets, server-sent events, or polling strategies. Consider optimistic updates, conflict resolution, proper error handling, and state management for real-time data.
Complex transformations require proper data normalization, efficient algorithms, consideration of performance impact, and possibly using web workers. Consider implementing caching for expensive transformations.
Large-scale architectures require proper service organization, API gateway implementation, microservices integration, and efficient data flow management. Consider implementing proper error boundaries and fallback strategies.
Efficient pagination involves cursor-based or offset-based strategies, proper cache management, infinite scroll implementation, and optimized data fetching. Consider implementing virtual scrolling for large datasets.
API middleware implementation involves request/response interceptors, logging, analytics tracking, error handling, and authentication management. Consider implementing proper middleware composition and order.
Advanced caching involves implementing stale-while-revalidate, cache-then-network, and proper cache invalidation strategies. Consider implementing proper cache hierarchies and optimization techniques.
Complex authentication involves OAuth flows, token refresh mechanisms, biometric authentication integration, and proper security measures. Consider implementing proper state management and error handling.
API monitoring involves implementing proper logging, performance tracking, error reporting, and usage analytics. Consider implementing proper monitoring infrastructure and alerting systems.
API testing involves implementing proper test coverage, mocking strategies, integration tests, and automated testing pipelines. Consider implementing proper test data management and CI/CD integration.
Solve React Native development challenges tailored for interviews.
Explore MoreUnderstand core components, props, and state management techniques.
Learn best practices for handling animations, rendering optimizations, and memory management.
Understand how to integrate native code for platform-specific functionalities.
Familiarize yourself with React Native debugging tools and common issue resolution techniques.
Join thousands of successful candidates preparing with Stark.ai. Start practicing React Native questions, mock interviews, and more to secure your dream role.
Start Preparing now