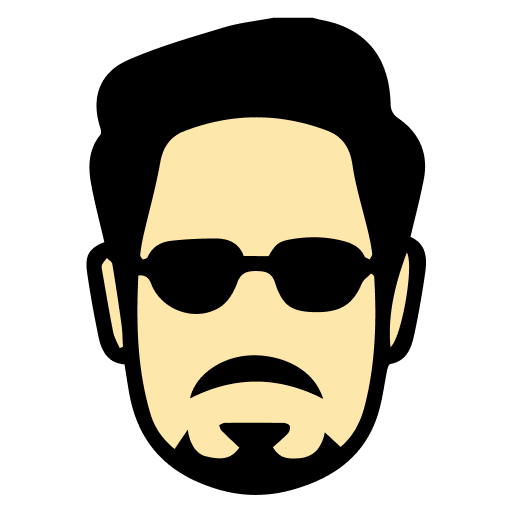
Apollo GraphQL's powerful ecosystem streamlines development of efficient and scalable GraphQL APIs. Stark.ai offers a comprehensive collection of Apollo GraphQL interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Apollo Client is a comprehensive state management library for GraphQL. Key features: declarative data fetching,...
Initialize using ApolloClient constructor, configure with cache and link options. Wrap application with...
InMemoryCache is Apollo's normalized caching system. Stores query results, handles data normalization. Features:...
Data fetching through useQuery hook (React), query components. Supports variables, polling, skip/fetchMore. Handles...
Queries for reading data (idempotent), Mutations for modifying data. Queries can run parallel, mutations execute...
Local state management through field policies, reactive variables. Can extend schema with @client directive....
Apollo Link customizes network layer behavior. Chain of middleware for request/response modification. Features:...
Error handling through GraphQL errors, network errors. Provides error information in query/mutation results....
TypePolicies configure cache behavior per type. Define key fields, field merging strategies. Features: custom merge...
Real-time updates through subscriptions, polling. WebSocket integration for live data. Features: subscription...
useQuery hook fetches and manages query data. Provides loading/error states, refetch functions. Features: automatic...
Pagination through fetchMore function, field policies. Supports offset/cursor-based pagination. Features: automatic...
Reactive Variables store local state outside cache. Update components automatically on change. Features: no query...
Cache normalization through unique identifiers, type policies. Prevents data duplication, ensures consistency....
Optimistic UI updates cache before server response. Improves perceived performance, user experience. Features:...
Authentication through HTTP headers, context. Configure ApolloLink for token management. Features: token refresh,...
Fragments define reusable field sets. Promote code reuse, maintainability. Features: colocated queries, type safety....
Query deduplication combines identical concurrent requests. Reduces network traffic, improves performance. Features:...
Cache redirects customize cache reading behavior. Define relationships between cached entities. Features: field...
File uploads through apollo-upload-client package. Supports multipart form data, progress tracking. Features:...
Error handling through error policies, custom error handling. Implement retry logic, user feedback. Features: error...
Offline support through cache persistence, local state. Implement retry strategies, queue mutations. Features: cache...
FetchPolicy controls cache/network behavior. Options: cache-first, network-only, etc. Features: customizable per...
Code splitting through dynamic imports, lazy components. Supports query splitting, fragment colocation. Features:...
Field policies customize field behavior in cache. Define read/write functions, merge strategies. Features: custom...
Data prefetching through query preloading, cache priming. Improves perceived performance, user experience. Features:...
Performance optimization through caching, query batching. Implement field limiting, pagination. Features: automatic...
Client-side operations through local state, field policies. Implement search logic, filtering functions. Features:...
Client directive marks fields for local-only operations. Enables client-side resolvers, computed fields. Features:...
SSR through getDataFromTree, cache extraction. Handle initial state, hydration. Features: automatic cache...
Dynamic queries using query variables, conditional fields. Implement skip/include directives. Features: runtime...
Loading states: loading, error, data, networkStatus. Access through useQuery hook result. Features: partial data...
Query variables through useQuery options, variable definitions. Support default values, type safety. Features:...
FetchPolicies: cache-first, network-only, cache-and-network, no-cache, standby. Control cache/network behavior....
Polling through pollInterval option in useQuery. Configure interval timing, automatic updates. Features: start/stop...
NotifyOnNetworkStatusChange tracks detailed network status. Provides component updates for network changes....
Error handling through errorPolicy option, error results. Implement error boundaries, retry logic. Features: partial...
Refetching through refetch function, network-only policy. Support manual/automatic triggers, variables update....
Skip/Include directives for conditional field inclusion. Control field selection at runtime. Features: dynamic...
Standby policy pauses query execution. Useful for inactive queries, resource management. Features: manual execution...
Partial responses through errorPolicy: 'all'. Handle missing fields, null values. Features: partial data rendering,...
Query batching combines multiple queries into single request. Implement through Apollo Link batch. Features:...
Retry logic through retry link configuration. Handle network errors, timeout scenarios. Features: exponential...
Organization through query separation, fragments. Implement colocated queries, reusable parts. Features:...
Timeouts through Apollo Link configuration. Implement timeout logic, error handling. Features: custom timeout...
Deduplication combines identical concurrent requests. Reduces network traffic, improves performance. Features:...
Prefetching through client.query, preloadQuery. Improve perceived performance, data availability. Features: cache...
Optimization through field selection, pagination. Implement proper caching, batching. Features: minimal queries,...
Nested queries through proper field selection, fragments. Implement efficient data loading, caching. Features:...
Query signatures uniquely identify operations. Used for caching, deduplication. Features: automatic generation,...
Cancellation through AbortController, cleanup functions. Handle component unmount, user actions. Features: request...
Directives modify query execution behavior. Implement @skip, @include, custom directives. Features: conditional...
TypeScript support through codegen, type definitions. Implement type safety, variable validation. Features:...
Context provides global query configuration. Share data between resolvers, links. Features: auth tokens, headers...
Persistence through cache storage, local state. Implement offline support, data retention. Features: cache...
Error recovery through retry logic, fallback data. Implement error boundaries, user feedback. Features: graceful...
Authorization through context, directives. Implement access control, role checks. Features: permission handling,...
Cost analysis evaluates query complexity, resource usage. Implement limits, optimization suggestions. Features:...
Monitoring through Apollo Studio, custom metrics. Track performance, errors, usage patterns. Features: analytics,...
Testing through mock providers, test utilities. Implement unit tests, integration tests. Features: query mocking,...
Cache normalization flattens nested data using unique identifiers. Implements automatic ID generation, reference...
Type policies define how types are stored and retrieved from cache. Configure key fields, field behaviors. Features:...
Local state through reactive variables, local-only fields. Implement client-side resolvers, type extensions....
Field policies customize individual field behavior. Define read/write functions, merge strategies. Features:...
Cache updates through update functions, refetchQueries. Implement optimistic updates, cache modifications. Features:...
Cache persistence stores cache data locally. Uses apollo3-cache-persist. Features: automatic persistence, custom...
Cache eviction through evict method, garbage collection. Implement cache cleanup, reference management. Features:...
Cache redirects customize cache data access. Define field reading behavior, data relationships. Features: custom...
Optimistic responses update UI before server response. Implement temporary cache updates, rollback handling....
Cache initialization sets up initial cache state. Configure type policies, initial data. Features: preloaded state,...
Pagination through field policies, merge functions. Implement cursor-based/offset pagination. Features: automatic...
Reactive variables manage local state. Independent of GraphQL operations. Features: direct reads/writes, automatic...
Cache invalidation through evict, modify methods. Implement selective updates, refetching. Features: field-level...
Cache policies control data reading/writing. Types: cache-first, network-only, etc. Features: customizable per...
Cache conflicts through merge functions, conflict resolution. Implement custom merging logic, update priorities....
Cache extraction retrieves current cache state. Used for SSR, persistence. Features: serialization, state transfer....
Cache debugging through Apollo DevTools, logging. Inspect cache state, watch updates. Features: cache explorer,...
Custom resolvers handle field computation, data transformation. Implement local-only fields, computed values....
Real-time updates through subscriptions, cache updates. Implement automatic merging, optimistic UI. Features:...
Cache pruning removes unused data. Implements automatic cleanup, reference tracking. Features: memory optimization,...
List updates through field policies, merge functions. Implement array operations, item updates. Features: automatic...
Cache hints provide caching instructions. Configure max-age, scope. Features: field-level control, cache behavior....
Cache preloading through initial state, prefetching. Implement data warming, startup data. Features: performance...
Cache manipulation through writeQuery, writeFragment. Implement direct updates, data modifications. Features:...
Cache fragmentation through proper normalization, cleanup. Implement reference management, garbage collection....
Cache redirects customize data access paths. Implement field policies, custom resolvers. Features: data relationship...
Persistence strategies through storage options, update policies. Implement persistence plugins, recovery logic....
Nested object policies through type policies, merge functions. Implement relationship handling, nested updates....
Cache warming through prefetching, initial data. Implement strategic loading, data preparation. Features:...
Best practices include proper normalization, type policies, field policies. Implement efficient updates, proper...
Mutations implemented using useMutation hook. Configure variables, options, cache updates. Features: optimistic...
Optimistic updates provide immediate UI feedback before server response. Implement through optimisticResponse...
Cache updates through update function, refetchQueries. Implement cache modifications, field updates. Features:...
RefetchQueries updates queries after mutation. Specify queries to refetch, variables. Features: automatic query...
Error handling through onError callback, error policies. Implement error messages, recovery logic. Features: error...
Mutation variables pass dynamic data to mutations. Configure through options object, typed variables. Features: type...
Concurrent mutations through mutation queue, synchronization. Implement ordering, dependency handling. Features:...
Update function modifies cache after mutation. Access cache directly, perform updates. Features: manual cache...
Batch mutations combine multiple operations. Implement through Apollo Link batch. Features: request batching,...
Loading states track mutation progress. Access through mutation result object. Features: loading indicator, disabled...
Rollbacks through error handling, cache restoration. Implement recovery logic, state management. Features: automatic...
Mutation hooks provide declarative mutation handling. Access mutation function, result data. Features: React...
File uploads through Apollo Upload Client. Handle multipart form data, progress. Features: file handling, upload...
Mutation options configure behavior. Include variables, update function, optimistic response. Features: error...
Dependent mutations through chaining, async/await. Implement sequence control, error handling. Features: mutation...
Result types define mutation responses. Include data, loading state, error information. Features: type safety,...
Offline mutations through queue system, persistence. Implement retry logic, conflict resolution. Features: offline...
Completion handling through onCompleted callback. Implement success actions, notifications. Features: result...
Side effects through update function, callbacks. Implement additional actions, state updates. Features: cache...
Mutation policies control execution behavior. Configure error handling, cache updates. Features: retry policies,...
Mutation testing through mock providers, test utilities. Implement assertions, response mocking. Features: result...
Mutation fragments define reusable mutation parts. Share common fields, type definitions. Features: code reuse,...
Conflict handling through version checks, merge strategies. Implement conflict resolution, retry logic. Features:...
Mutation directives modify mutation behavior. Configure execution, caching. Features: custom directives, behavior...
Rate limiting through Apollo Link, request throttling. Implement request control, queue management. Features:...
Best practices include proper error handling, optimistic updates, cache management. Implement type safety, testing....
Authorization through context, headers. Implement access control, token handling. Features: permission checks,...
Composition combines multiple mutations. Implement reusable parts, shared logic. Features: code organization,...
Monitoring through Apollo Studio, custom tracking. Implement performance metrics, error logging. Features:...
Key techniques include: Query caching, field-level caching, query batching, cache normalization. Implement proper...
Query batching combines multiple queries into single request. Uses Apollo Link Batch. Reduces network requests,...
Field-level caching stores individual field values. Uses unique field identifiers, custom field policies. Enables...
Memory optimization through cache eviction, garbage collection. Implement proper cache policies, data retention....
Query deduplication eliminates duplicate network requests. Automatically combines identical queries. Reduces server...
Query prefetching loads data before needed. Use client.query() or preloadQuery(). Improves perceived performance,...
Large query optimization through pagination, field selection. Implement fragments, query splitting. Use proper fetch...
Cache normalization flattens nested data structures. Uses unique identifiers, reference handling. Reduces data...
Performance monitoring through Apollo Studio, metrics collection. Track query performance, error rates. Analyze...
Mutation optimization through optimistic updates, proper cache updates. Implement batch mutations, efficient cache...
Fetch policies control cache/network behavior. Different policies affect performance differently. Balance between...
Efficient pagination through cursor-based approach, proper cache configuration. Implement field policies, merge...
Network optimization through proper link configuration, request batching. Implement retry strategies, timeout...
Bundle optimization through proper imports, code splitting. Remove unused features, implement lazy loading. Consider...
Cache policies control data freshness, network requests. Balance between performance and data accuracy. Configure...
Real-time optimization through proper subscription handling, cache updates. Implement efficient data sync, update...
Network reduction through proper query design, field selection. Implement fragments, query composition. Consider...
Client-side optimization through cache policies, computed fields. Implement efficient data access patterns. Consider...
Field policies affect cache behavior, data access patterns. Impact on read/write performance. Consider custom field...
Efficient cache persistence through proper storage strategy, serialization. Implement selective persistence,...
Setup using @testing-library/react, MockedProvider. Configure test wrappers, mock responses. Features: mock Apollo...
MockedProvider enables Apollo Client testing. Provides mock responses, error simulation. Features: request matching,...
Query testing through mock responses, assertion utilities. Test loading states, error handling. Features: response...
Mutation testing through mock functions, result verification. Test optimistic updates, error scenarios. Features:...
Cache debugging through Apollo DevTools, cache inspection. Monitor cache updates, state changes. Features: cache...
Error testing through mock errors, response simulation. Test error handling, recovery logic. Features: network...
Integration testing through test utilities, component rendering. Test component interactions, data flow. Features:...
Debugging tools: Apollo DevTools, React DevTools, Network inspector. Monitor queries, cache state, performance....
Cache policy testing through mock operations, state verification. Test cache behavior, updates. Features: field...
Mocking practices: realistic data, proper typing. Implement consistent mocks, error cases. Features: type...
Optimistic response testing through update verification, timing checks. Test UI updates, rollbacks. Features: cache...
Snapshot testing captures component output. Compare rendered content, query results. Features: automated comparison,...
Network debugging through request inspection, response analysis. Monitor headers, payload data. Features: request...
Subscription testing through mock WebSocket, event simulation. Test real-time updates, connection handling....
Performance testing through metrics collection, timing analysis. Test query execution, cache efficiency. Features:...
Cache debugging through state inspection, update tracking. Identify inconsistencies, normalization issues. Features:...
Local state testing through resolver verification, cache inspection. Test field policies, local mutations. Features:...
Error boundary testing through error simulation, recovery verification. Test fallback UI, error handling. Features:...
Variable debugging through request inspection, value tracking. Monitor variable usage, type validation. Features:...
Testing utilities include MockedProvider, test renderers. Provide mock data, assertion helpers. Features: component...
E2E testing through Cypress, TestCafe integration. Test full application flow, user scenarios. Features: full stack...
Mutation debugging through update tracking, result verification. Monitor cache changes, optimistic updates....
Pagination testing through data loading verification, cache updates. Test fetch more functionality, cursor handling....
Common pitfalls: improper mocking, async handling issues. Address timing problems, cache management. Features: test...
Fragment debugging through composition analysis, reuse verification. Monitor fragment spreading, type conditions....
Error handling testing through scenario simulation, recovery verification. Test error states, user feedback....
Mock schema through type definitions, resolvers. Implement test data, response patterns. Features: schema...
Cache policy debugging through policy inspection, behavior analysis. Monitor field policies, type policies....
Reactive variable testing through value tracking, update verification. Test reactivity, dependency updates....
Component testing practices: isolation, proper mocking. Implement realistic scenarios, error cases. Features:...
Authentication through HTTP headers, context setup. Use Apollo Link for token management. Features: JWT handling,...
Token management through secure storage, automatic refresh. Implement token rotation, expiration handling. Features:...
Authorization through directives, field-level checks. Implement role-based access, permission validation. Features:...
Apollo Link manages request/response pipeline. Add authentication headers, handle errors. Features: token injection,...
Secure uploads through proper validation, token verification. Implement file type checks, size limits. Features:...
Cache security through proper data handling, sensitive info protection. Implement cache policies, access control....
OAuth integration through proper flow implementation, token management. Handle authorization code, access tokens....
CORS handling through proper server configuration, client setup. Implement request headers, preflight handling....
RBAC through directives, context checks. Implement permission system, role validation. Features: hierarchical roles,...
Mutation security through proper validation, authorization checks. Implement input sanitization, access control....
Session management through proper storage, expiration handling. Implement session tokens, refresh mechanism....
Persisted queries security through proper whitelisting, validation. Implement query registry, access control....
Secure websockets through proper authentication, token validation. Implement connection params, protocol security....
Error security through proper message handling, stack trace protection. Implement error masking, logging strategy....
Rate limiting through proper configuration, request tracking. Implement throttling logic, error responses. Features:...
Local state security through proper encryption, access control. Implement secure storage, data handling. Features:...
Secure persistence through proper encryption, storage strategy. Implement secure cache, local storage. Features:...
Fragment security through proper access control, type checking. Implement fragment masking, permission validation....
Secure downloads through proper authentication, access validation. Implement token verification, stream handling....
Directive security through proper validation, execution control. Implement directive restrictions, permission...
SDL is language for defining GraphQL schemas. Define types, queries, mutations. Features: type system, field...
Custom scalars through scalar type definition, serialization methods. Implement parsing, validation logic. Features:...
Unions combine multiple types, interfaces define shared fields. Implement type resolution, conditional fields....
Schema evolution through careful changes, deprecation notices. Implement backwards compatibility, gradual updates....
Input types define mutation arguments. Structure complex inputs, validate data. Features: type safety, nested...
Schema directives modify schema behavior. Implement custom directives, execution logic. Features: field...
Schema stitching/federation combines multiple schemas. Implement service composition, type merging. Features:...
Schema validation through tools, linting rules. Implement type checking, consistency rules. Features: schema...
Type design through proper naming, field organization. Implement clear interfaces, proper relationships. Features:...
Code generation through GraphQL tools, type generators. Generate TypeScript/Flow types. Features: type safety,...
Type nullability through exclamation mark (!). Define required fields, optional fields. Features: type safety, error...
Schema documentation through descriptions, comments. Implement proper documentation, examples. Features:...
Enum types define fixed set of values. Implement type restrictions, validation. Features: type safety, clear...
Type extensions through extend keyword, additional fields. Modify existing types, add functionality. Features:...
List types through square brackets notation. Handle array fields, collection types. Features: array validation, null...
Type resolvers through resolver functions, field definitions. Implement data fetching, field computation. Features:...
Abstract types through interfaces, unions. Implement polymorphic schemas, type conditions. Features: type...
Schema deprecation through @deprecated directive, documentation. Implement migration paths, client communication....
Validation directives through custom implementations. Implement field validation, input constraints. Features:...
Recursive types through self-referential definitions. Implement tree structures, nested data. Features: circular...
Subscriptions enable real-time data updates through WebSocket connection. Maintain live connection for event-based...
WebSocket setup through split network interface, protocol configuration. Configure connection parameters,...
Subscription client manages WebSocket connections, subscription lifecycle. Handle connection states, message...
Subscription authentication through connection params, token validation. Implement secure WebSocket connection,...
Subscription events through PubSub system, event filtering. Implement event triggers, data transformation. Features:...
Subscription cleanup through proper unsubscription, connection termination. Handle component unmount, cleanup...
Subscription variables through dynamic parameters, event filtering. Configure subscription behavior, data filtering....
Subscription errors through error callbacks, connection recovery. Implement error handling, retry logic. Features:...
Subscription link options through configuration parameters, connection settings. Configure timeout, reconnect...
Subscription filtering through withFilter function, filter conditions. Implement data filtering, event selection....
Payload transformation through resolvers, data mapping. Implement data formatting, structure conversion. Features:...
Reconnection handling through retry policies, connection monitoring. Implement backoff strategy, state recovery....
Subscription contexts through context providers, data sharing. Share data across subscription resolvers. Features:...
Subscription testing through mock WebSocket, event simulation. Test subscription behavior, event handling. Features:...
Best practices include proper cleanup, error handling, connection management. Implement efficient filtering, payload...
Error types include: Network errors, GraphQL errors, Runtime errors. Network errors for connection issues, GraphQL...
Global error handling through error link, onError callback. Implement centralized error processing, logging....
Error policies control how errors affect result data. Options: none, all, ignore. Determine partial data handling,...
Network error handling through retry logic, fallback strategies. Implement timeout handling, offline detection....
Error tracking through logging services, monitoring tools. Implement error reporting, analytics collection....
Mutation error handling through try-catch blocks, error callbacks. Handle operation failures, optimistic updates....
Error links provide custom error handling middleware. Intercept and process errors, modify error behavior. Features:...
Partial error handling through error policies, data extraction. Process partial responses, handle missing data....
Error UI through error boundaries, component feedback. Display error messages, recovery options. Features: user...
Retry logic through retry link, attempt configuration. Implement exponential backoff, max attempts. Features: retry...
Error transformations through custom error processing, formatting. Modify error structure, add context. Features:...
Subscription error handling through connection monitoring, error callbacks. Handle WebSocket errors, connection...
Error boundaries catch rendering errors, provide fallback UI. Implement component-level error handling. Features:...
Error logging through centralized service, structured formats. Implement error categorization, context capture....
Recovery strategies through fallback options, state reset. Implement data recovery, cache updates. Features:...
Initialize through ApolloClient constructor, configuration options. Set up cache, link configuration. Features:...
Essential options include: uri for GraphQL endpoint, cache implementation, default options. Configure link chain,...
Cache configuration through InMemoryCache options, type policies. Set up field policies, cache behavior. Features:...
Apollo Link configures request pipeline, middleware chain. Set up network handling, error processing. Features:...
Authentication setup through context, headers configuration. Implement token management, auth middleware. Features:...
Local state through cache configuration, local resolvers. Set up client-side fields, local mutations. Features:...
Error handling through onError link, global handlers. Set up error policies, retry logic. Features: error...
Fetch policies control cache/network behavior. Configure default policies, per-query settings. Features: cache...
Subscription setup through WebSocket link, protocol configuration. Configure connection parameters, client options....
Persisted queries through automatic persisting, cache configuration. Set up query registry, automatic extraction....
Development tools through Apollo DevTools integration, debugging options. Set up logging, inspection tools....
Type policies through cache configuration, field definitions. Set up custom field behavior, merge functions....
Testing setup through MockedProvider, test utilities. Configure mock responses, network behavior. Features: query...
Fragment matching through IntrospectionFragmentMatcher, type configuration. Set up union types, interface handling....
Batch operations through Apollo Link Batch, queue configuration. Set up request batching, timing options. Features:...
Apollo Client is a comprehensive state management library for GraphQL. Key features: declarative data fetching, zero-config caching, predictable mutations, automatic query deduplication. Integrates with any GraphQL API and UI framework.
Initialize using ApolloClient constructor, configure with cache and link options. Wrap application with ApolloProvider. Example: new ApolloClient({ cache: new InMemoryCache(), uri: 'graphql-endpoint' }). Set default options for queries/mutations.
InMemoryCache is Apollo's normalized caching system. Stores query results, handles data normalization. Features: type policies, field policies, custom resolvers. Essential for performance, offline capabilities, data consistency.
Data fetching through useQuery hook (React), query components. Supports variables, polling, skip/fetchMore. Handles loading/error states automatically. Features automatic caching, real-time updates.
Queries for reading data (idempotent), Mutations for modifying data. Queries can run parallel, mutations execute sequentially. Queries automatically cached, mutations require cache updates. Different hooks: useQuery vs useMutation.
Local state management through field policies, reactive variables. Can extend schema with @client directive. Features: local resolvers, cache manipulation. Integrates with global cache management.
Apollo Link customizes network layer behavior. Chain of middleware for request/response modification. Features: error handling, authentication, logging. Enables advanced network customization.
Error handling through GraphQL errors, network errors. Provides error information in query/mutation results. Features: error policies, retry mechanisms. Supports custom error handling logic.
TypePolicies configure cache behavior per type. Define key fields, field merging strategies. Features: custom merge functions, field policies. Essential for cache normalization.
Real-time updates through subscriptions, polling. WebSocket integration for live data. Features: subscription components/hooks, automatic updates. Supports optimistic UI updates.
useQuery hook fetches and manages query data. Provides loading/error states, refetch functions. Features: automatic caching, skip/pollInterval options. Essential for React component data fetching.
Pagination through fetchMore function, field policies. Supports offset/cursor-based pagination. Features: automatic cache merging, custom merge functions. Handles loading states automatically.
Reactive Variables store local state outside cache. Update components automatically on change. Features: no query requirement, direct reads/writes. Useful for global UI state.
Cache normalization through unique identifiers, type policies. Prevents data duplication, ensures consistency. Features: custom key fields, merge functions. Essential for efficient caching.
Optimistic UI updates cache before server response. Improves perceived performance, user experience. Features: optimistic response object, error rollback. Used with mutations for instant feedback.
Authentication through HTTP headers, context. Configure ApolloLink for token management. Features: token refresh, error handling. Supports different auth strategies.
Fragments define reusable field sets. Promote code reuse, maintainability. Features: colocated queries, type safety. Used for component-specific data requirements.
Query deduplication combines identical concurrent requests. Reduces network traffic, improves performance. Features: automatic caching, request batching. Configurable through client options.
Cache redirects customize cache reading behavior. Define relationships between cached entities. Features: field policies, custom resolvers. Useful for complex data relationships.
File uploads through apollo-upload-client package. Supports multipart form data, progress tracking. Features: multiple file uploads, abort capability. Requires server-side configuration.
Error handling through error policies, custom error handling. Implement retry logic, user feedback. Features: error components, global error handling. Consider different error types.
Offline support through cache persistence, local state. Implement retry strategies, queue mutations. Features: cache storage, conflict resolution. Consider offline-first architecture.
FetchPolicy controls cache/network behavior. Options: cache-first, network-only, etc. Features: customizable per query, default policies. Balances performance and data freshness.
Code splitting through dynamic imports, lazy components. Supports query splitting, fragment colocation. Features: automatic chunk loading, performance optimization. Important for large applications.
Field policies customize field behavior in cache. Define read/write functions, merge strategies. Features: custom field handling, computed fields. Essential for complex cache management.
Data prefetching through query preloading, cache priming. Improves perceived performance, user experience. Features: manual prefetch, automatic preloading. Consider resource utilization.
Performance optimization through caching, query batching. Implement field limiting, pagination. Features: automatic garbage collection, cache policies. Consider bundle size, network usage.
Client-side operations through local state, field policies. Implement search logic, filtering functions. Features: reactive variables, local resolvers. Consider performance implications.
Client directive marks fields for local-only operations. Enables client-side resolvers, computed fields. Features: local state management, schema extension. Used for UI state, computed data.
SSR through getDataFromTree, cache extraction. Handle initial state, hydration. Features: automatic cache population, state transfer. Consider SEO, performance requirements.
Dynamic queries using query variables, conditional fields. Implement skip/include directives. Features: runtime variables, dynamic field selection. Consider query complexity, caching implications.
Loading states: loading, error, data, networkStatus. Access through useQuery hook result. Features: partial data handling, refetch status. Important for user feedback, error handling.
Query variables through useQuery options, variable definitions. Support default values, type safety. Features: variable validation, dynamic updates. Consider variable scoping, reusability.
FetchPolicies: cache-first, network-only, cache-and-network, no-cache, standby. Control cache/network behavior. Features: per-query configuration, default policies. Balance performance and data freshness.
Polling through pollInterval option in useQuery. Configure interval timing, automatic updates. Features: start/stop polling, skip conditions. Consider server load, real-time requirements.
NotifyOnNetworkStatusChange tracks detailed network status. Provides component updates for network changes. Features: refetch status, error states. Important for loading indicators, error handling.
Error handling through errorPolicy option, error results. Implement error boundaries, retry logic. Features: partial data handling, error recovery. Consider user experience, error reporting.
Refetching through refetch function, network-only policy. Support manual/automatic triggers, variables update. Features: selective refetching, loading states. Consider cache invalidation, performance.
Skip/Include directives for conditional field inclusion. Control field selection at runtime. Features: dynamic queries, conditional data. Consider query complexity, performance impact.
Standby policy pauses query execution. Useful for inactive queries, resource management. Features: manual execution control, cache preservation. Consider memory usage, query prioritization.
Partial responses through errorPolicy: 'all'. Handle missing fields, null values. Features: partial data rendering, error boundaries. Consider user experience, data completeness.
Query batching combines multiple queries into single request. Implement through Apollo Link batch. Features: automatic batching, timeout configuration. Consider network optimization, request timing.
Retry logic through retry link configuration. Handle network errors, timeout scenarios. Features: exponential backoff, max attempts. Consider error types, retry conditions.
Organization through query separation, fragments. Implement colocated queries, reusable parts. Features: maintainable structure, type safety. Consider code reuse, maintainability.
Timeouts through Apollo Link configuration. Implement timeout logic, error handling. Features: custom timeout values, cancel operations. Consider network conditions, user experience.
Deduplication combines identical concurrent requests. Reduces network traffic, improves performance. Features: automatic caching, request optimization. Consider cache policies, timing.
Prefetching through client.query, preloadQuery. Improve perceived performance, data availability. Features: cache warming, anticipatory loading. Consider resource usage, timing.
Optimization through field selection, pagination. Implement proper caching, batching. Features: minimal queries, efficient loading. Consider data requirements, network usage.
Nested queries through proper field selection, fragments. Implement efficient data loading, caching. Features: normalized data, relationship handling. Consider query complexity, performance.
Query signatures uniquely identify operations. Used for caching, deduplication. Features: automatic generation, query identification. Consider cache keys, operation tracking.
Cancellation through AbortController, cleanup functions. Handle component unmount, user actions. Features: request termination, resource cleanup. Consider in-flight requests, state management.
Directives modify query execution behavior. Implement @skip, @include, custom directives. Features: conditional fields, runtime control. Consider schema support, client capabilities.
TypeScript support through codegen, type definitions. Implement type safety, variable validation. Features: automatic types, compile-time checks. Consider development experience, maintenance.
Context provides global query configuration. Share data between resolvers, links. Features: auth tokens, headers management. Consider request lifecycle, security implications.
Persistence through cache storage, local state. Implement offline support, data retention. Features: cache serialization, state recovery. Consider storage limits, data privacy.
Error recovery through retry logic, fallback data. Implement error boundaries, user feedback. Features: graceful degradation, recovery options. Consider user experience, data integrity.
Authorization through context, directives. Implement access control, role checks. Features: permission handling, secure queries. Consider security requirements, user roles.
Cost analysis evaluates query complexity, resource usage. Implement limits, optimization suggestions. Features: query validation, performance metrics. Consider server resources, scalability.
Monitoring through Apollo Studio, custom metrics. Track performance, errors, usage patterns. Features: analytics, debugging tools. Consider monitoring strategy, performance impact.
Testing through mock providers, test utilities. Implement unit tests, integration tests. Features: query mocking, response simulation. Consider test coverage, maintenance.
Cache normalization flattens nested data using unique identifiers. Implements automatic ID generation, reference handling. Features: customizable key fields, type policies. Essential for consistent data representation and updates.
Type policies define how types are stored and retrieved from cache. Configure key fields, field behaviors. Features: custom merge functions, field read/write functions. Controls cache behavior per GraphQL type.
Local state through reactive variables, local-only fields. Implement client-side resolvers, type extensions. Features: @client directive, local mutations. Manages UI state alongside server data.
Field policies customize individual field behavior. Define read/write functions, merge strategies. Features: computed fields, custom caching logic. Handles complex field requirements.
Cache updates through update functions, refetchQueries. Implement optimistic updates, cache modifications. Features: automatic updates, manual cache writes. Ensures cache consistency after mutations.
Cache persistence stores cache data locally. Uses apollo3-cache-persist. Features: automatic persistence, custom storage. Enables offline support, faster initial loads.
Cache eviction through evict method, garbage collection. Implement cache cleanup, reference management. Features: automatic garbage collection, manual eviction. Manages cache size and memory usage.
Cache redirects customize cache data access. Define field reading behavior, data relationships. Features: custom resolvers, field policies. Handles complex data relationships and queries.
Optimistic responses update UI before server response. Implement temporary cache updates, rollback handling. Features: immediate feedback, error recovery. Improves perceived performance.
Cache initialization sets up initial cache state. Configure type policies, initial data. Features: preloaded state, type configuration. Essential for proper cache setup and behavior.
Pagination through field policies, merge functions. Implement cursor-based/offset pagination. Features: automatic merging, custom merge strategies. Manages paginated data in cache.
Reactive variables manage local state. Independent of GraphQL operations. Features: direct reads/writes, automatic updates. Simplifies local state management.
Cache invalidation through evict, modify methods. Implement selective updates, refetching. Features: field-level invalidation, type-level eviction. Ensures data freshness.
Cache policies control data reading/writing. Types: cache-first, network-only, etc. Features: customizable per query, default settings. Balances performance and data freshness.
Cache conflicts through merge functions, conflict resolution. Implement custom merging logic, update priorities. Features: deterministic merging, conflict detection. Maintains cache consistency.
Cache extraction retrieves current cache state. Used for SSR, persistence. Features: serialization, state transfer. Important for server rendering, cache backup.
Cache debugging through Apollo DevTools, logging. Inspect cache state, watch updates. Features: cache explorer, update tracking. Essential for development and troubleshooting.
Custom resolvers handle field computation, data transformation. Implement local-only fields, computed values. Features: field-level resolution, cache integration. Extends cache capabilities.
Real-time updates through subscriptions, cache updates. Implement automatic merging, optimistic UI. Features: subscription integration, cache consistency. Maintains real-time data state.
Cache pruning removes unused data. Implements automatic cleanup, reference tracking. Features: memory optimization, cache size management. Prevents cache bloat.
List updates through field policies, merge functions. Implement array operations, item updates. Features: automatic merging, custom ordering. Manages list-based data.
Cache hints provide caching instructions. Configure max-age, scope. Features: field-level control, cache behavior. Optimizes cache performance and freshness.
Cache preloading through initial state, prefetching. Implement data warming, startup data. Features: performance optimization, initial load. Improves application startup.
Cache manipulation through writeQuery, writeFragment. Implement direct updates, data modifications. Features: programmatic updates, cache control. Enables manual cache management.
Cache fragmentation through proper normalization, cleanup. Implement reference management, garbage collection. Features: performance optimization, memory management. Maintains cache efficiency.
Cache redirects customize data access paths. Implement field policies, custom resolvers. Features: data relationship handling, query optimization. Manages complex data access patterns.
Persistence strategies through storage options, update policies. Implement persistence plugins, recovery logic. Features: offline support, state preservation. Ensures data availability.
Nested object policies through type policies, merge functions. Implement relationship handling, nested updates. Features: complex data structures, reference management. Handles nested data effectively.
Cache warming through prefetching, initial data. Implement strategic loading, data preparation. Features: performance optimization, user experience. Improves application responsiveness.
Best practices include proper normalization, type policies, field policies. Implement efficient updates, proper cleanup. Features: performance optimization, maintainability. Ensures robust cache implementation.
Mutations implemented using useMutation hook. Configure variables, options, cache updates. Features: optimistic updates, error handling. Common pattern: mutation function returns promise with result.
Optimistic updates provide immediate UI feedback before server response. Implement through optimisticResponse option. Features: temporary cache updates, automatic rollback on error. Improves perceived performance.
Cache updates through update function, refetchQueries. Implement cache modifications, field updates. Features: automatic cache normalization, manual cache writes. Ensures cache consistency.
RefetchQueries updates queries after mutation. Specify queries to refetch, variables. Features: automatic query updates, cache refresh. Ensures data consistency across components.
Error handling through onError callback, error policies. Implement error messages, recovery logic. Features: error tracking, user feedback. Handles network errors, GraphQL errors.
Mutation variables pass dynamic data to mutations. Configure through options object, typed variables. Features: type safety, dynamic values. Handles user input, dynamic data.
Concurrent mutations through mutation queue, synchronization. Implement ordering, dependency handling. Features: race condition prevention, sequence control. Maintains data consistency.
Update function modifies cache after mutation. Access cache directly, perform updates. Features: manual cache writes, complex updates. Handles custom cache modifications.
Batch mutations combine multiple operations. Implement through Apollo Link batch. Features: request batching, optimization. Reduces network requests, improves performance.
Loading states track mutation progress. Access through mutation result object. Features: loading indicator, disabled states. Handles user feedback, UI updates.
Rollbacks through error handling, cache restoration. Implement recovery logic, state management. Features: automatic rollback, manual recovery. Handles failed mutations.
Mutation hooks provide declarative mutation handling. Access mutation function, result data. Features: React integration, type safety. Simplifies mutation implementation.
File uploads through Apollo Upload Client. Handle multipart form data, progress. Features: file handling, upload tracking. Supports single/multiple file uploads.
Mutation options configure behavior. Include variables, update function, optimistic response. Features: error policies, refetch queries. Controls mutation execution.
Dependent mutations through chaining, async/await. Implement sequence control, error handling. Features: mutation ordering, data dependencies. Manages related mutations.
Result types define mutation responses. Include data, loading state, error information. Features: type safety, result handling. Structures mutation responses.
Offline mutations through queue system, persistence. Implement retry logic, conflict resolution. Features: offline support, sync handling. Handles network interruptions.
Completion handling through onCompleted callback. Implement success actions, notifications. Features: result processing, UI updates. Handles successful mutations.
Side effects through update function, callbacks. Implement additional actions, state updates. Features: cache modifications, external updates. Manages mutation impacts.
Mutation policies control execution behavior. Configure error handling, cache updates. Features: retry policies, update strategies. Defines mutation behavior.
Mutation testing through mock providers, test utilities. Implement assertions, response mocking. Features: result verification, error testing. Ensures mutation reliability.
Mutation fragments define reusable mutation parts. Share common fields, type definitions. Features: code reuse, maintainability. Reduces duplicate mutation code.
Conflict handling through version checks, merge strategies. Implement conflict resolution, retry logic. Features: data consistency, user feedback. Manages concurrent updates.
Mutation directives modify mutation behavior. Configure execution, caching. Features: custom directives, behavior control. Extends mutation capabilities.
Rate limiting through Apollo Link, request throttling. Implement request control, queue management. Features: request limits, timing control. Prevents server overload.
Best practices include proper error handling, optimistic updates, cache management. Implement type safety, testing. Features: performance optimization, reliability. Ensures robust mutations.
Authorization through context, headers. Implement access control, token handling. Features: permission checks, secure mutations. Ensures authorized access.
Composition combines multiple mutations. Implement reusable parts, shared logic. Features: code organization, maintainability. Structures complex mutations.
Monitoring through Apollo Studio, custom tracking. Implement performance metrics, error logging. Features: analytics, debugging. Tracks mutation behavior.
Key techniques include: Query caching, field-level caching, query batching, cache normalization. Implement proper fetch policies, query deduplication. Features automatic garbage collection and cache persistence.
Query batching combines multiple queries into single request. Uses Apollo Link Batch. Reduces network requests, improves performance. Best for multiple simultaneous queries. Consider timeout configuration and request ordering.
Field-level caching stores individual field values. Uses unique field identifiers, custom field policies. Enables granular cache control, reduces redundant requests. Improves performance for frequently accessed data.
Memory optimization through cache eviction, garbage collection. Implement proper cache policies, data retention. Monitor memory usage, clean unused data. Consider cache size limits and cleanup strategies.
Query deduplication eliminates duplicate network requests. Automatically combines identical queries. Reduces server load, improves client performance. Enable/disable through client configuration.
Query prefetching loads data before needed. Use client.query() or preloadQuery(). Improves perceived performance, reduces loading time. Consider resource usage and timing strategies.
Large query optimization through pagination, field selection. Implement fragments, query splitting. Use proper fetch policies, cache strategies. Consider data requirements and network impact.
Cache normalization flattens nested data structures. Uses unique identifiers, reference handling. Reduces data duplication, improves cache efficiency. Enables consistent data updates.
Performance monitoring through Apollo Studio, metrics collection. Track query performance, error rates. Analyze cache effectiveness, network usage. Consider client-side and server-side metrics.
Mutation optimization through optimistic updates, proper cache updates. Implement batch mutations, efficient cache writes. Consider update strategies and rollback mechanisms.
Fetch policies control cache/network behavior. Different policies affect performance differently. Balance between data freshness and performance. Consider use case requirements and network conditions.
Efficient pagination through cursor-based approach, proper cache configuration. Implement field policies, merge functions. Consider data loading strategies and UX requirements.
Network optimization through proper link configuration, request batching. Implement retry strategies, timeout handling. Consider error handling and network conditions.
Bundle optimization through proper imports, code splitting. Remove unused features, implement lazy loading. Consider build configuration and dependency management.
Cache policies control data freshness, network requests. Balance between performance and data accuracy. Configure based on data requirements and user experience.
Real-time optimization through proper subscription handling, cache updates. Implement efficient data sync, update strategies. Consider WebSocket configuration and connection management.
Network reduction through proper query design, field selection. Implement fragments, query composition. Consider data requirements and bandwidth usage.
Client-side optimization through cache policies, computed fields. Implement efficient data access patterns. Consider data volume and computation cost.
Field policies affect cache behavior, data access patterns. Impact on read/write performance. Consider custom field handling and cache efficiency.
Efficient cache persistence through proper storage strategy, serialization. Implement selective persistence, cleanup. Consider storage limits and performance impact.
Setup using @testing-library/react, MockedProvider. Configure test wrappers, mock responses. Features: mock Apollo Client, query mocking. Essential for unit and integration testing.
MockedProvider enables Apollo Client testing. Provides mock responses, error simulation. Features: request matching, response timing control. Essential for component testing.
Query testing through mock responses, assertion utilities. Test loading states, error handling. Features: response timing, cache behavior. Verify data fetching logic.
Mutation testing through mock functions, result verification. Test optimistic updates, error scenarios. Features: cache updates, response handling. Verify data modification logic.
Cache debugging through Apollo DevTools, cache inspection. Monitor cache updates, state changes. Features: cache explorer, query watcher. Essential for troubleshooting.
Error testing through mock errors, response simulation. Test error handling, recovery logic. Features: network errors, GraphQL errors. Verify error handling implementation.
Integration testing through test utilities, component rendering. Test component interactions, data flow. Features: full rendering, async testing. Verify component integration.
Debugging tools: Apollo DevTools, React DevTools, Network inspector. Monitor queries, cache state, performance. Features: request tracking, state inspection. Essential for development.
Cache policy testing through mock operations, state verification. Test cache behavior, updates. Features: field policies, type policies. Verify cache configuration.
Mocking practices: realistic data, proper typing. Implement consistent mocks, error cases. Features: type generation, schema validation. Ensure reliable test data.
Optimistic response testing through update verification, timing checks. Test UI updates, rollbacks. Features: cache inspection, state tracking. Verify optimistic behavior.
Snapshot testing captures component output. Compare rendered content, query results. Features: automated comparison, update mechanism. Verify rendering consistency.
Network debugging through request inspection, response analysis. Monitor headers, payload data. Features: request tracking, error detection. Verify API communication.
Subscription testing through mock WebSocket, event simulation. Test real-time updates, connection handling. Features: subscription mocking, event verification. Verify real-time behavior.
Performance testing through metrics collection, timing analysis. Test query execution, cache efficiency. Features: performance tracking, optimization verification. Verify system performance.
Cache debugging through state inspection, update tracking. Identify inconsistencies, normalization issues. Features: cache comparison, update verification. Resolve cache problems.
Local state testing through resolver verification, cache inspection. Test field policies, local mutations. Features: state management, update validation. Verify local operations.
Error boundary testing through error simulation, recovery verification. Test fallback UI, error handling. Features: error capture, component recovery. Verify error management.
Variable debugging through request inspection, value tracking. Monitor variable usage, type validation. Features: variable inspection, value verification. Resolve query issues.
Testing utilities include MockedProvider, test renderers. Provide mock data, assertion helpers. Features: component testing, query simulation. Essential for test implementation.
E2E testing through Cypress, TestCafe integration. Test full application flow, user scenarios. Features: full stack testing, integration verification. Verify system functionality.
Mutation debugging through update tracking, result verification. Monitor cache changes, optimistic updates. Features: mutation tracking, state verification. Resolve update issues.
Pagination testing through data loading verification, cache updates. Test fetch more functionality, cursor handling. Features: load testing, cache behavior. Verify pagination logic.
Common pitfalls: improper mocking, async handling issues. Address timing problems, cache management. Features: test isolation, proper setup. Avoid testing antipatterns.
Fragment debugging through composition analysis, reuse verification. Monitor fragment spreading, type conditions. Features: fragment inspection, usage tracking. Resolve fragment issues.
Error handling testing through scenario simulation, recovery verification. Test error states, user feedback. Features: error testing, handling validation. Verify error management.
Mock schema through type definitions, resolvers. Implement test data, response patterns. Features: schema simulation, type checking. Essential for isolated testing.
Cache policy debugging through policy inspection, behavior analysis. Monitor field policies, type policies. Features: policy tracking, update verification. Resolve policy issues.
Reactive variable testing through value tracking, update verification. Test reactivity, dependency updates. Features: state management, update validation. Verify reactive behavior.
Component testing practices: isolation, proper mocking. Implement realistic scenarios, error cases. Features: component rendering, interaction testing. Ensure reliable tests.
Authentication through HTTP headers, context setup. Use Apollo Link for token management. Features: JWT handling, session management. Implement token refresh, secure storage.
Token management through secure storage, automatic refresh. Implement token rotation, expiration handling. Features: JWT validation, secure transmission. Consider security implications.
Authorization through directives, field-level checks. Implement role-based access, permission validation. Features: context-based auth, error handling. Ensure proper access control.
Apollo Link manages request/response pipeline. Add authentication headers, handle errors. Features: token injection, request modification. Essential for security implementation.
Secure uploads through proper validation, token verification. Implement file type checks, size limits. Features: multipart requests, progress tracking. Consider security measures.
Cache security through proper data handling, sensitive info protection. Implement cache policies, access control. Features: data encryption, secure storage. Prevent data leaks.
OAuth integration through proper flow implementation, token management. Handle authorization code, access tokens. Features: refresh flow, state management. Ensure secure authentication.
CORS handling through proper server configuration, client setup. Implement request headers, preflight handling. Features: origin validation, credential handling. Ensure secure cross-origin requests.
RBAC through directives, context checks. Implement permission system, role validation. Features: hierarchical roles, permission inheritance. Ensure proper authorization.
Mutation security through proper validation, authorization checks. Implement input sanitization, access control. Features: data validation, error handling. Prevent unauthorized modifications.
Session management through proper storage, expiration handling. Implement session tokens, refresh mechanism. Features: session validation, secure storage. Ensure secure user sessions.
Persisted queries security through proper whitelisting, validation. Implement query registry, access control. Features: query verification, cache security. Prevent query injection.
Secure websockets through proper authentication, token validation. Implement connection params, protocol security. Features: connection lifecycle, error handling. Ensure secure real-time communication.
Error security through proper message handling, stack trace protection. Implement error masking, logging strategy. Features: error sanitization, security logging. Prevent sensitive information exposure.
Rate limiting through proper configuration, request tracking. Implement throttling logic, error responses. Features: limit enforcement, user identification. Prevent abuse and DOS attacks.
Local state security through proper encryption, access control. Implement secure storage, data handling. Features: sensitive data protection, secure operations. Prevent client-side vulnerabilities.
Secure persistence through proper encryption, storage strategy. Implement secure cache, local storage. Features: data protection, access control. Ensure secure client-side storage.
Fragment security through proper access control, type checking. Implement fragment masking, permission validation. Features: field-level security, type restrictions. Prevent unauthorized data access.
Secure downloads through proper authentication, access validation. Implement token verification, stream handling. Features: file access control, secure transfer. Ensure secure file delivery.
Directive security through proper validation, execution control. Implement directive restrictions, permission checks. Features: directive scope, security boundaries. Prevent directive misuse.
SDL is language for defining GraphQL schemas. Define types, queries, mutations. Features: type system, field definitions, relationships. Essential for API structure definition.
Custom scalars through scalar type definition, serialization methods. Implement parsing, validation logic. Features: type safety, custom validation. Handle specialized data types.
Unions combine multiple types, interfaces define shared fields. Implement type resolution, conditional fields. Features: polymorphic queries, type flexibility. Enable complex type relationships.
Schema evolution through careful changes, deprecation notices. Implement backwards compatibility, gradual updates. Features: version management, client compatibility. Maintain API stability.
Input types define mutation arguments. Structure complex inputs, validate data. Features: type safety, nested objects. Essential for mutation parameters.
Schema directives modify schema behavior. Implement custom directives, execution logic. Features: field transformation, validation rules. Extend schema capabilities.
Schema stitching/federation combines multiple schemas. Implement service composition, type merging. Features: distributed schemas, service integration. Enable microservices architecture.
Schema validation through tools, linting rules. Implement type checking, consistency rules. Features: schema testing, error detection. Ensure schema quality.
Type design through proper naming, field organization. Implement clear interfaces, proper relationships. Features: schema clarity, maintainability. Follow GraphQL conventions.
Code generation through GraphQL tools, type generators. Generate TypeScript/Flow types. Features: type safety, development efficiency. Automate type definitions.
Type nullability through exclamation mark (!). Define required fields, optional fields. Features: type safety, error prevention. Control field requirements.
Schema documentation through descriptions, comments. Implement proper documentation, examples. Features: self-documenting API, tooling support. Improve developer experience.
Enum types define fixed set of values. Implement type restrictions, validation. Features: type safety, clear constraints. Limit possible field values.
Type extensions through extend keyword, additional fields. Modify existing types, add functionality. Features: schema flexibility, modular design. Enable schema evolution.
List types through square brackets notation. Handle array fields, collection types. Features: array validation, null handling. Manage collections of values.
Type resolvers through resolver functions, field definitions. Implement data fetching, field computation. Features: data resolution, business logic. Handle field values.
Abstract types through interfaces, unions. Implement polymorphic schemas, type conditions. Features: type flexibility, shared fields. Enable complex type hierarchies.
Schema deprecation through @deprecated directive, documentation. Implement migration paths, client communication. Features: version management, backward compatibility. Handle API evolution.
Validation directives through custom implementations. Implement field validation, input constraints. Features: runtime validation, error handling. Ensure data integrity.
Recursive types through self-referential definitions. Implement tree structures, nested data. Features: circular references, depth control. Handle hierarchical data.
Subscriptions enable real-time data updates through WebSocket connection. Maintain live connection for event-based updates. Features: real-time data, event handling. Essential for live updates.
WebSocket setup through split network interface, protocol configuration. Configure connection parameters, authentication. Features: persistent connection, protocol handling. Enable real-time communication.
Subscription client manages WebSocket connections, subscription lifecycle. Handle connection states, message processing. Features: message queuing, reconnection logic. Manage subscription operations.
Subscription authentication through connection params, token validation. Implement secure WebSocket connection, session management. Features: token handling, connection security. Ensure secure subscriptions.
Subscription events through PubSub system, event filtering. Implement event triggers, data transformation. Features: event publishing, subscription filtering. Handle real-time updates.
Subscription cleanup through proper unsubscription, connection termination. Handle component unmount, cleanup effects. Features: resource management, memory cleanup. Prevent memory leaks.
Subscription variables through dynamic parameters, event filtering. Configure subscription behavior, data filtering. Features: dynamic subscriptions, parameter handling. Customize subscription data.
Subscription errors through error callbacks, connection recovery. Implement error handling, retry logic. Features: error reporting, connection management. Handle subscription failures.
Subscription link options through configuration parameters, connection settings. Configure timeout, reconnect attempts. Features: link customization, behavior control. Optimize subscription handling.
Subscription filtering through withFilter function, filter conditions. Implement data filtering, event selection. Features: selective updates, data relevance. Control update flow.
Payload transformation through resolvers, data mapping. Implement data formatting, structure conversion. Features: data shaping, response formatting. Customize subscription data.
Reconnection handling through retry policies, connection monitoring. Implement backoff strategy, state recovery. Features: connection resilience, session management. Maintain subscription stability.
Subscription contexts through context providers, data sharing. Share data across subscription resolvers. Features: context access, data availability. Enable shared subscription state.
Subscription testing through mock WebSocket, event simulation. Test subscription behavior, event handling. Features: connection testing, event verification. Ensure subscription reliability.
Best practices include proper cleanup, error handling, connection management. Implement efficient filtering, payload optimization. Features: performance consideration, resource management. Optimize subscription usage.
Error types include: Network errors, GraphQL errors, Runtime errors. Network errors for connection issues, GraphQL errors for operation failures, Runtime errors for execution problems. Each requires specific handling strategies.
Global error handling through error link, onError callback. Implement centralized error processing, logging. Features: error transformation, retry logic. Handle application-wide errors.
Error policies control how errors affect result data. Options: none, all, ignore. Determine partial data handling, error inclusion. Configure per-operation behavior.
Network error handling through retry logic, fallback strategies. Implement timeout handling, offline detection. Features: connection recovery, user feedback. Manage connectivity issues.
Error tracking through logging services, monitoring tools. Implement error reporting, analytics collection. Features: error patterns, performance impact. Monitor application health.
Mutation error handling through try-catch blocks, error callbacks. Handle operation failures, optimistic updates. Features: rollback logic, error recovery. Manage mutation failures.
Error links provide custom error handling middleware. Intercept and process errors, modify error behavior. Features: error transformation, retry logic. Extend error handling capabilities.
Partial error handling through error policies, data extraction. Process partial responses, handle missing data. Features: graceful degradation, fallback content. Manage incomplete results.
Error UI through error boundaries, component feedback. Display error messages, recovery options. Features: user communication, action guidance. Improve error experience.
Retry logic through retry link, attempt configuration. Implement exponential backoff, max attempts. Features: retry conditions, timeout handling. Manage operation retries.
Error transformations through custom error processing, formatting. Modify error structure, add context. Features: error normalization, message formatting. Standardize error handling.
Subscription error handling through connection monitoring, error callbacks. Handle WebSocket errors, connection failures. Features: reconnection logic, state recovery. Manage subscription issues.
Error boundaries catch rendering errors, provide fallback UI. Implement component-level error handling. Features: error isolation, recovery options. Prevent application crashes.
Error logging through centralized service, structured formats. Implement error categorization, context capture. Features: log aggregation, analysis tools. Track application errors.
Recovery strategies through fallback options, state reset. Implement data recovery, cache updates. Features: graceful degradation, user feedback. Handle error recovery.
Initialize through ApolloClient constructor, configuration options. Set up cache, link configuration. Features: network interface, cache setup. Essential for client creation.
Essential options include: uri for GraphQL endpoint, cache implementation, default options. Configure link chain, error handling. Features: request policies, connection settings.
Cache configuration through InMemoryCache options, type policies. Set up field policies, cache behavior. Features: normalization settings, custom identifiers.
Apollo Link configures request pipeline, middleware chain. Set up network handling, error processing. Features: request modification, response handling.
Authentication setup through context, headers configuration. Implement token management, auth middleware. Features: request authorization, session handling.
Local state through cache configuration, local resolvers. Set up client-side fields, local mutations. Features: local data management, cache integration.
Error handling through onError link, global handlers. Set up error policies, retry logic. Features: error transformation, recovery strategies.
Fetch policies control cache/network behavior. Configure default policies, per-query settings. Features: cache usage, network requests.
Subscription setup through WebSocket link, protocol configuration. Configure connection parameters, client options. Features: real-time updates, connection management.
Persisted queries through automatic persisting, cache configuration. Set up query registry, automatic extraction. Features: query optimization, network efficiency.
Development tools through Apollo DevTools integration, debugging options. Set up logging, inspection tools. Features: cache inspection, query debugging.
Type policies through cache configuration, field definitions. Set up custom field behavior, merge functions. Features: cache normalization, field computing.
Testing setup through MockedProvider, test utilities. Configure mock responses, network behavior. Features: query testing, mutation verification.
Fragment matching through IntrospectionFragmentMatcher, type configuration. Set up union types, interface handling. Features: type resolution, fragment support.
Batch operations through Apollo Link Batch, queue configuration. Set up request batching, timing options. Features: request optimization, network efficiency.
Showcase your Apollo GraphQL expertise with an ATS-friendly resume.
Explore MoreUnderstand concepts like queries, mutations, subscriptions, and schema design.
Work on implementing Apollo Client, Server, and state management solutions.
Dive into caching strategies, error handling, and performance optimization.
Expect hands-on challenges to build, test, and optimize GraphQL APIs.
Join thousands of successful candidates preparing with Stark.ai. Start practicing GraphQL questions, mock interviews, and more to secure your dream role.
Start Preparing now