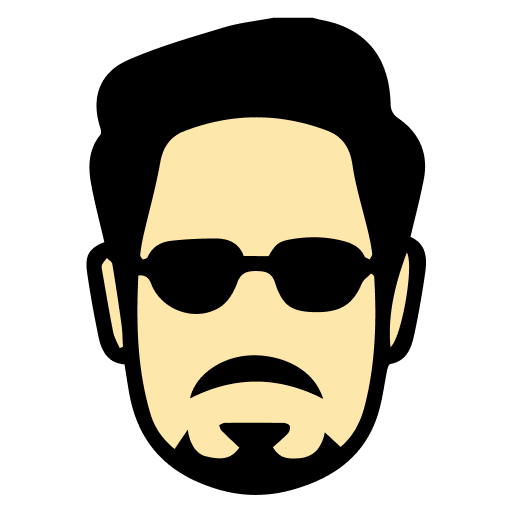
JavaScript is the backbone of modern web development, making it a must-have skill for front-end and full-stack developers. Stark.ai provides curated JavaScript interview questions, real-world scenarios, and expert tips to help you excel in your next technical interview.
JavaScript is a high-level, interpreted programming language commonly used for web development. Key features include...
`var` has function scope and can be redeclared or updated, potentially leading to issues. `let` is block-scoped and...
A closure is a function that retains access to its lexical scope, even when the function is executed outside that...
The event loop is a mechanism in JavaScript that handles asynchronous operations. It continuously checks the call...
Arrow functions are a concise syntax for defining functions in JavaScript, using `=>`. Unlike regular functions,...
The DOM is a programming interface for web documents that represents the page as a tree structure of nodes, allowing...
You can select DOM elements using methods like `document.getElementById()`, `document.getElementsByClassName()`,...
You can handle events using the `addEventListener()` method, which attaches an event handler to an element. For...
Event delegation is a technique where you attach a single event listener to a parent element rather than individual...
You can create new DOM elements using `document.createElement()`, set their attributes using methods like...
ES6 introduced several new features such as `let` and `const` for block-scoped variable declarations, arrow...
Destructuring allows you to unpack values from arrays or properties from objects into distinct variables. For...
The spread operator (`...`) is used to spread elements from an iterable (like an array) into individual elements. It...
A `Promise` in JavaScript represents a value that may be available now, in the future, or never. It allows handling...
Template literals allow for multi-line strings and embedded expressions. They are created using backticks (`` ` ``)...
var has function scope, hoisted with undefined value. let and const have block scope, hoisted but not initialized...
Type coercion automatically converts values between types during operations. Implicit coercion occurs in comparisons...
Hoisting moves declarations to top of scope during compilation. Function declarations fully hoisted with...
'this' refers to current execution context. In methods, refers to object. In global scope, refers to window/global...
Seven primitive types: string, number, boolean, null, undefined, symbol, bigint. Immutable values, passed by value....
Objects inherit properties/methods through prototype chain. Each object has internal [[Prototype]] link. Properties...
Closure allows function to access variables from outer scope even after outer function returns. Used for data...
Events propagate through DOM tree. Capturing phase (top-down), target phase, bubbling phase (bottom-up). Control...
Event loop handles asynchronous operations. Manages call stack, callback queue, microtask queue. Executes...
Use === for strict equality (type and value). == performs type coercion. Object comparison checks reference. Use...
Scope chain determines variable access. Inner scope can access outer scope variables. Created when function...
Automatic memory management through reference counting and mark-and-sweep. Objects garbage collected when...
String literals allowing embedded expressions (${expression}). Support multiline strings, tagged templates. Enable...
Object literals, constructor functions, Object.create(), classes. Each method has different prototype behavior....
Use Number.EPSILON for floating-point comparison. Handle IEEE 754 limitations. Consider BigInt for large integers....
Map allows any type as keys, maintains insertion order. Set stores unique values. Both provide efficient lookup....
Strict mode enables stricter parsing/error handling. Prevents implicit globals, this coercion. Requires variable...
Function declarations, expressions, arrow functions, generator functions. Each has different this binding, arguments...
Use Object.defineProperty(), Object.freeze(), Object.seal(). Control property behavior (writable, enumerable,...
undefined represents uninitialized value, null represents intentional absence. Different behavior in type coercion,...
Use try-catch blocks, error objects, custom errors. Handle async errors with promises. Consider error types,...
Symbols create unique identifiers. Used for private properties, special methods (Symbol.iterator). Non-enumerable by...
Use libraries (decimal.js), multiplication and division tricks. Handle IEEE 754 limitations. Consider precision...
Use get/set keywords, Object.defineProperty(). Control property access/modification. Implement validation, computed...
Use typeof, instanceof, Object.prototype.toString. Handle null/undefined cases. Consider type coercion rules....
Use meaningful names, proper casing (camelCase). Declare variables at appropriate scope. Consider hoisting, block...
Function declarations are hoisted with their implementation. Function expressions are assigned to variables, not...
Closures maintain access to their outer scope variables even after the outer function has returned. Used for data...
Arguments object contains all passed function arguments. Not available in arrow functions. Array-like but not an...
Lexical scope determines variable access based on where functions are defined, not where they're called. Affects...
Default parameters provide fallback values when arguments are undefined. Evaluated at call time. Can reference other...
IIFEs execute immediately upon definition. Create private scope, avoid global namespace pollution. Common in module...
Regular functions: 'this' determined by call context. Arrow functions: lexical 'this' from enclosing scope. Method...
Currying transforms multi-argument function into series of single-argument functions. Enables partial application,...
Rest parameters collect remaining arguments into array. Spread operator expands iterables into individual elements....
Function composition combines multiple functions into single function. Output of one function becomes input of...
Use closures to create private scope. Methods defined inside closure not accessible externally. Return public...
Generator functions create iterators using yield keyword. Enable lazy evaluation, infinite sequences. Handle...
Use tail call optimization where available. Implement iterative alternatives. Consider stack size limitations....
Pure functions always return same output for same input. No side effects, depend only on input arguments. Easier...
Cache function results based on input arguments. Improve performance for expensive calculations. Consider cache...
Function declarations hoisted with implementation. Function expressions hoisted as variables. Affects code...
Check arguments length/type for different behaviors. Use default parameters, rest parameters. Consider function...
Functions that take/return other functions. Enable function composition, callbacks, decorators. Common in functional...
Block scope limited to block (let/const). Function scope spans entire function (var). Affects variable accessibility...
bind creates new function with fixed this. call/apply execute function immediately with specified this. Arrow...
Functions that create and return other functions. Use closures for configuration, state. Handle parameter...
Functions that modify or enhance other functions. Add logging, memoization, validation. Maintain function properties...
Use arrow functions or bind for correct this context. Consider closure implications. Handle cleanup properly....
Partial application fixes some arguments, returns function expecting remainder. Currying always returns...
Throttle limits execution frequency. Debounce delays execution until pause in calls. Use closure for state. Handle...
Async functions maintain their own execution context. Handle promise chain scope. Consider error handling scope....
Modules create their own scope. Export/import controls accessibility. Consider module closure effects. Handle...
Function prototypes define shared behavior. Enable method inheritance. Consider performance implications. Handle...
Return this from methods to enable chaining. Consider immutable alternatives. Handle errors in chain. Implement...
Objects can be created using object literals, constructor functions, Object.create(), and class syntax. Object...
Prototypal inheritance uses prototype chain for property/method lookup, objects inherit directly from other objects....
Property descriptors control property behavior through attributes: writable, enumerable, configurable, get/set....
Private fields implemented using # prefix in classes, closures for privacy in constructor functions, WeakMap for...
Object.create() establishes direct prototype link without constructor call. Constructor functions create instances...
Return this from methods to enable chaining. Ensures methods return object instance. Consider immutability...
Mixins combine properties/methods from multiple sources into objects. Alternative to multiple inheritance. Implement...
Getters/setters enable computed properties, access control. Defined using get/set keywords or...
SOLID principles guide OOP design: Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation,...
Observer pattern enables event-driven communication between objects. Implement subscribe/unsubscribe methods,...
Compose objects using delegation, aggregation, or mixing. Favor composition over inheritance. Consider interface...
Classes provide cleaner syntax, enforce new usage, enable private fields. Constructor functions offer more...
Use appropriate enumeration method: Object.keys() for own enumerable properties, for...in for all enumerable...
Singleton ensures single instance existence. Implement using static property/closure. Consider lazy initialization,...
Override methods by redefining in child class/object. Access parent methods using super keyword or prototype chain....
Symbols create unique property keys, enable metaprogramming features. Used for private-like properties, special...
Implement custom equality methods, compare relevant properties. Consider deep vs shallow comparison. Handle circular...
Module pattern uses closures for encapsulation. Modern alternatives include ES modules, classes with private fields....
Use JSON.stringify/parse with custom replacer/reviver. Handle circular references, special types (Date, Map)....
Command pattern encapsulates actions as objects. Enables undo/redo, action queuing. Consider command validation,...
Use Object.freeze(), implement proper copying methods. Consider deep freezing requirements. Handle updates through...
Proxies enable custom behavior for fundamental operations. Implement property access control, validation, logging....
Strategy pattern enables interchangeable algorithms. Implement through object composition, function properties....
Decorator pattern adds behavior dynamically. Implement through object composition or class inheritance. Consider...
Implement proper initialization, cleanup methods. Handle resource management, event listeners. Consider garbage...
Value objects represent immutable values, implement value equality. Consider hash code implementation, comparison...
Pass dependencies through constructor or setter methods. Consider dependency lifecycle, initialization order. Handle...
Follow SOLID principles, use appropriate patterns. Consider JavaScript's prototype nature. Handle proper...
Array.map() creates a new array with transformed elements, returning the result of applying a function to each...
reduce() processes array elements from left to right, while reduceRight() processes from right to left. Both methods...
slice() creates a shallow copy of array portion without modifying the original array, taking start and end indices....
Array.find() returns first matching element, while filter() returns all matching elements. For sorted arrays,...
Use immutable methods (map, filter, reduce) for data transformation. Implement proper error handling for array...
Array.sort() accepts comparison function returning negative, zero, or positive values. Implement stable sorting for...
Typed arrays provide fixed-length arrays of specific numeric types. Used for binary data manipulation, WebGL, and...
Use Set object for simple value deduplication. Implement custom comparison for objects. Consider memory usage with...
WeakMap and WeakSet hold weak references to objects, allowing garbage collection of keys. Used for private data...
Use Array.flat() or reduce() for nested array flattening. Consider depth of nesting and performance implications....
Pre-allocate array size when possible. Use appropriate array methods for operations. Consider memory usage and...
Array-like objects have length property and indexed elements but lack array methods. Convert to arrays using...
Consider holes in array iteration. Use appropriate methods for sparse array operations. Handle undefined values...
Use spread operator or slice() for shallow copy. Implement deep cloning for nested structures. Handle circular...
Release references to unused arrays. Handle large array cleanup properly. Consider garbage collection implications....
Use appropriate comparison methods for element types. Handle object comparison properly. Implement custom comparison...
Implement efficient chunking algorithms. Handle remainder elements properly. Consider memory usage with large...
Use appropriate locking mechanisms. Handle race conditions properly. Implement proper synchronization. Consider...
Use appropriate serialization format. Handle circular references. Implement proper type conversion. Consider...
Use Set for unique values. Implement efficient algorithms. Handle object comparison properly. Consider performance...
Use stable sorting algorithms when needed. Handle special values properly. Consider performance implications....
Use Fisher-Yates algorithm for unbiased shuffling. Implement proper randomization. Consider performance...
Implement efficient rotation algorithms. Handle different rotation directions. Consider performance implications....
Implement proper index validation. Handle negative indices appropriately. Consider performance implications....
Use Set for primitive values. Implement custom comparison for objects. Consider performance implications. Document...
Promises provide structured handling of asynchronous operations with chaining capabilities and built-in error...
async/await provides synchronous-looking code for asynchronous operations, improving readability and...
Promise.all() handles multiple concurrent Promises, resolving when all complete. Promise.race() resolves with the...
Use Promise catch() blocks or try/catch with async/await. Implement proper error propagation and recovery...
The event loop manages execution of asynchronous operations by processing the call stack, callback queue, and...
Implement proper synchronization mechanisms using Promise.race() or flags. Handle out-of-order responses...
Use Promise.race() with timeout Promise, or implement custom timeout logic. Handle cleanup for timed-out operations....
Use finally blocks or cleanup functions in Promise chains. Implement proper cancellation patterns. Handle resource...
Return values from then() handlers for proper chaining. Implement proper error handling at each step. Consider...
Implement exponential backoff strategy. Handle maximum retry attempts. Consider error types for retry decisions....
Use Promise.all() for parallel execution, implement proper rate limiting. Handle partial failures appropriately....
Use AbortController for fetch requests, implement custom cancellation tokens. Handle cleanup on cancellation....
Async iterators enable asynchronous iteration using for-await-of. Async generators combine generator and async...
Implement proper cleanup of event listeners and subscriptions. Handle reference cleanup in closures. Consider weak...
Implement proper loading states and dependencies. Handle initialization errors appropriately. Consider lazy...
Use closures to manage timing. Implement proper cleanup of timers. Consider immediate execution options. Handle edge...
Use async test frameworks. Implement proper assertions for async results. Handle timeout and error cases. Consider...
Consider event order and timing. Handle proper error propagation. Implement cleanup on handler removal. Consider...
Handle concurrent execution limits. Implement proper queue management. Consider priority handling. Handle error...
Implement proper loading and error states. Handle race conditions appropriately. Consider optimistic updates....
Implement proper fallback mechanisms. Handle partial failures appropriately. Consider retry strategies. Document...
Handle async context preservation. Implement proper error tracking. Consider performance impact. Document logging...
Implement proper dependency resolution. Handle circular dependencies appropriately. Consider initialization order....
Implement proper concurrency control. Consider caching strategies. Handle batch operations appropriately. Document...
Implement proper backpressure handling. Consider memory usage and buffering. Handle error propagation appropriately....
Handle error propagation appropriately. Implement proper recovery mechanisms. Consider error scope and isolation....
Implement proper initialization and cleanup. Handle event order appropriately. Consider dependency management....
Handle error context preservation. Implement proper error aggregation. Consider error categorization. Document...
Document async behavior and requirements clearly. Consider error cases and handling. Document cleanup requirements....
The primary methods for DOM selection include getElementById (fastest, returns single element), querySelector...
DOM updates should be batched to minimize browser reflows and repaints. Use DocumentFragment for multiple...
Event delegation involves attaching event listeners to parent elements to handle events on descendants, leveraging...
When removing DOM elements, ensure proper cleanup by removing event listeners, clearing references in JavaScript,...
innerHTML parses content as HTML, allowing element creation but presenting security risks with unsanitized input....
Efficient DOM traversal utilizes properties like parentNode, children, nextSibling, and previousSibling. Consider...
MutationObserver monitors DOM changes, useful for dynamic content updates and third-party script integration....
Handle cross-browser differences through feature detection rather than browser detection. Implement appropriate...
Minimize DOM access and updates by caching references and batching changes. Use document fragments for multiple...
Implement form validation using both HTML5 validation attributes and JavaScript validation. Handle form submission...
Data attributes (data-*) store custom data within HTML elements, accessible via dataset property. Use for...
Implement focus management considering accessibility requirements. Handle focus trapping in modals. Implement proper...
Handle view updates efficiently without full page reloads. Implement proper cleanup between view changes. Consider...
Implement efficient content loading strategies using fetch or XMLHttpRequest. Handle loading states appropriately....
Prevent XSS attacks by sanitizing input before insertion. Avoid eval() and innerHTML with untrusted content....
Use HTML5 Drag and Drop API or implement custom drag and drop logic. Handle drag events properly. Implement proper...
Use media queries and matchMedia for responsive behavior. Handle resize events efficiently. Implement proper...
Use CSS transitions when possible, falling back to JavaScript animations when needed. Implement proper animation...
Implement scroll event throttling or debouncing. Use IntersectionObserver when possible. Handle scroll position...
Render only visible elements while maintaining scroll position and size. Implement efficient item recycling. Handle...
Handle touch events for mobile devices. Implement proper gesture recognition. Consider multi-touch interactions....
Implement proper keyboard navigation. Handle keyboard shortcuts appropriately. Consider focus management. Document...
Handle positioning and visibility. Implement proper event handling. Consider accessibility requirements. Handle...
Handle DOM operation failures gracefully. Implement proper error recovery. Consider user feedback mechanisms....
Handle cross-origin communication properly. Implement proper sandbox restrictions. Consider security implications....
Synchronize DOM with application state efficiently. Handle state transitions properly. Consider optimistic updates....
Implement proper DOM virtualization. Handle memory management efficiently. Consider performance optimization....
Implement proper unit and integration tests. Handle async operations in tests. Consider test isolation. Document...
JavaScript provides several built-in error types for specific scenarios. TypeError indicates incorrect type...
Asynchronous error handling requires careful consideration of Promise chains and async/await patterns. Use catch...
Modern browsers offer comprehensive debugging tools including breakpoints, step debugging, watch expressions, and...
Custom error types should extend the built-in Error class and include additional context relevant to the application...
Production error logging requires careful consideration of security, performance, and debugging needs. Implement...
Memory leak detection requires systematic approach using Chrome DevTools Memory panel or similar tools. Profile...
Production debugging requires comprehensive error logging, source map support, and monitoring tools. Implement...
Error boundaries provide containment for runtime errors, preventing application-wide crashes. Implement hierarchical...
Third-party script errors require careful isolation and error handling strategies. Implement proper script loading...
Error recovery requires systematic approach to restore application state and functionality. Implement proper state...
Performance debugging utilizes browser performance tools, profiling, and timeline analysis. Use Performance panel...
API error handling requires comprehensive strategy for different error types and scenarios. Handle network errors,...
Event-driven code debugging requires understanding of event flow and timing. Use debugger statements strategically,...
Worker thread error handling requires proper message passing and error propagation. Implement error event listeners,...
Error messages should be clear, actionable, and provide appropriate context. Include error codes, relevant details,...
Micro-frontend error tracking requires coordinated error handling strategy. Implement proper error propagation...
Race condition debugging requires systematic analysis of timing and state changes. Implement proper logging of state...
Component lifecycle error handling requires proper error boundaries and cleanup procedures. Implement error handling...
Minified code debugging requires proper source map integration and build process configuration. Maintain source map...
Environment-specific error reporting requires appropriate configuration and handling. Implement proper error...
Memory consumption debugging requires systematic analysis using browser memory tools. Profile heap usage, analyze...
Service worker error handling requires proper event handling and recovery mechanisms. Implement error listeners,...
Recursive function debugging requires careful analysis of call stack and state changes. Implement proper logging at...
Client-side routing error monitoring requires proper route change handling and error tracking. Implement route...
Validation error handling requires comprehensive strategy for different validation scenarios. Implement proper error...
WebSocket error tracking requires proper connection state monitoring and error handling. Implement connection error...
Application state debugging requires proper state monitoring and analysis tools. Use Redux DevTools or similar state...
Browser extension error handling requires proper isolation and error reporting. Implement content script error...
Mobile browser error handling requires consideration of device-specific issues and limitations. Handle touch event...
Arrow functions provide a concise syntax for writing function expressions and maintain lexical this binding. Unlike...
Template literals provide enhanced string functionality through backtick syntax, supporting multi-line strings,...
Destructuring enables extracting values from arrays or properties from objects into distinct variables using a...
ES6 modules provide a standardized way to organize and share code between JavaScript files. They support named and...
The spread operator (...) expands elements while the rest operator collects elements into an array. Spread is useful...
ES6 classes provide a clearer syntax for object-oriented programming, supporting inheritance through extends,...
Promises represent eventual completion of asynchronous operations, providing a cleaner alternative to callbacks....
Map allows any type as keys and maintains insertion order, while Set stores unique values. Both provide efficient...
Symbols are unique and immutable primitive values, often used as property keys to avoid name collisions. They...
Generators provide a way to define iterative algorithms by writing functions that can be paused and resumed. They...
Enhanced object literals support shorthand property and method definitions, computed property names, and super...
async/await provides synchronous-looking code for asynchronous operations, building on Promises. It improves code...
Default parameters allow specifying default values for function parameters when no argument is provided. They...
Proxy enables custom behavior for fundamental object operations, while Reflect provides methods for controlled...
let and const provide block scoping, preventing hoisting issues and temporal dead zone considerations. const...
Tagged template literals allow custom processing of template literal strings and expressions through tag functions....
New array methods include find(), findIndex(), includes(), flat(), and flatMap(). These methods enhance array...
WeakMap and WeakSet hold weak references to objects, allowing garbage collection of key objects when no other...
ES6+ adds features like named capture groups, lookbehind assertions, and the sticky flag. These enhancements improve...
Private class fields and methods (marked with #) provide true privacy in class definitions. They are enforced by the...
Improvements include rest parameters, default parameters, and destructuring in parameters. These features provide...
BigInt enables working with large integers beyond Number.MAX_SAFE_INTEGER. Numeric separators improve readability of...
Dynamic import() enables loading modules on demand, returning a Promise for module loading. This feature enables...
Destructuring patterns support default values for both object properties and array elements. Default values are used...
New string methods include startsWith(), endsWith(), includes(), padStart(), and padEnd(). These methods improve...
Optional chaining (?.) provides safe property access, while nullish coalescing (??) provides default values for...
These methods provide different levels of object immutability. freeze() prevents all modifications, seal() prevents...
Static class fields and methods belong to the class itself rather than instances. They provide utility functionality...
Spread operator and Object.assign() provide improved methods for shallow copying arrays and objects. Consider proper...
The Singleton pattern ensures a class has only one instance and provides global access to it. In modern JavaScript,...
The Observer pattern enables objects to notify multiple observers about changes in their state. Implementation...
The Factory pattern provides an interface for creating objects without specifying their exact classes. It's...
The Module pattern uses closures and immediately invoked function expressions (IIFE) to create private scope and...
The Strategy pattern enables selecting algorithms at runtime by defining a family of interchangeable algorithms....
The Decorator pattern allows adding new behaviors to objects dynamically by placing them inside wrapper objects....
The Command pattern encapsulates method invocation by treating it as an object. Implementation involves creating...
The Proxy pattern provides a surrogate object that controls access to another object. Implementation in JavaScript...
The Chain of Responsibility pattern passes requests along a chain of handlers until one processes the request....
The Mediator pattern provides a central point for object communication, reducing direct connections between objects....
The Composite pattern treats individual objects and compositions uniformly through a common interface....
The State pattern allows objects to alter behavior when internal state changes by encapsulating state-specific...
The Template Method pattern defines algorithm skeleton in base class while letting subclasses override specific...
The Adapter pattern converts interface of one class into interface expected by clients. Implementation involves...
The Iterator pattern provides way to access elements of collection sequentially without exposing underlying...
The Builder pattern constructs complex objects step by step through fluent interface. Implementation involves...
The Facade pattern provides unified interface to complex subsystem. Implementation involves creating facade class...
The Flyweight pattern shares common state between multiple objects to reduce memory usage. Implementation involves...
The Bridge pattern separates abstraction from implementation allowing them to vary independently. Implementation...
The Visitor pattern lets you add operations to objects without modifying them by placing operation logic in separate...
The Memento pattern captures and externalizes object's internal state for later restoration. Implementation involves...
The Abstract Factory pattern provides interface for creating families of related objects without specifying concrete...
The Prototype pattern creates new objects by cloning existing ones rather than through instantiation. Implementation...
The Object Pool pattern manages set of reusable objects rather than creating and destroying them. Implementation...
The Interpreter pattern defines grammar representation and interpreter for language. Implementation involves...
The Null Object pattern provides default object behavior instead of null references. Implementation involves...
The Lazy Loading pattern defers initialization of object until first use. Implementation involves creating proxy or...
The Dependency Injection pattern provides dependencies to objects instead of having them create or find...
The Repository pattern mediates between domain and data mapping layers using collection-like interface....
The Unit of Work pattern maintains list of business objects affected by transaction and coordinates changes and...
JavaScript is a high-level, interpreted programming language commonly used for web development. Key features include dynamic typing, first-class functions, event-driven programming, support for asynchronous operations (like Promises and async/await), and the ability to manipulate the DOM (Document Object Model) in web pages.
`var` has function scope and can be redeclared or updated, potentially leading to issues. `let` is block-scoped and can be updated but not redeclared within the same scope. `const` is also block-scoped and cannot be updated or redeclared, ensuring constant values.
A closure is a function that retains access to its lexical scope, even when the function is executed outside that scope. Closures allow you to create private variables and functions. For example: ```js function outer() { let count = 0; return function() { count++; return count; }; } const increment = outer(); console.log(increment()); // 1 console.log(increment()); // 2 ```
The event loop is a mechanism in JavaScript that handles asynchronous operations. It continuously checks the call stack for functions to execute. If the call stack is empty, it processes tasks from the message queue (e.g., callbacks from Promises, setTimeout, etc.). This non-blocking behavior allows JavaScript to handle tasks asynchronously.
Arrow functions are a concise syntax for defining functions in JavaScript, using `=>`. Unlike regular functions, arrow functions do not have their own `this` value; instead, they inherit `this` from their lexical context. This makes them useful for cases where you want to retain the value of `this` in a callback.
The DOM is a programming interface for web documents that represents the page as a tree structure of nodes, allowing scripts to update the content, structure, and style of a document. JavaScript can be used to manipulate the DOM by selecting elements, modifying attributes, and reacting to user events.
You can select DOM elements using methods like `document.getElementById()`, `document.getElementsByClassName()`, `document.getElementsByTagName()`, `document.querySelector()`, and `document.querySelectorAll()`. Each method offers different ways to target specific elements or groups of elements within the DOM.
You can handle events using the `addEventListener()` method, which attaches an event handler to an element. For example: `element.addEventListener('click', function() { console.log('Element clicked'); });`. Inline event handlers (e.g., `onclick`) can also be used, but `addEventListener()` is preferred as it allows for multiple handlers.
Event delegation is a technique where you attach a single event listener to a parent element rather than individual child elements. This leverages event bubbling (events moving up the DOM) to handle events on dynamically added elements. For example, a click listener on a parent container can handle clicks for its child elements.
You can create new DOM elements using `document.createElement()`, set their attributes using methods like `setAttribute()` or properties, and insert them into the document using methods like `appendChild()`, `insertBefore()`, or `innerHTML` for string-based insertion.
ES6 introduced several new features such as `let` and `const` for block-scoped variable declarations, arrow functions, template literals, destructuring assignment, default parameters, `Promise` for asynchronous code, classes, modules, and the spread/rest operators (`...`).
Destructuring allows you to unpack values from arrays or properties from objects into distinct variables. For example: ```js const [a, b] = [1, 2]; // Array destructuring const {name, age} = {name: 'John', age: 30}; // Object destructuring ```
The spread operator (`...`) is used to spread elements from an iterable (like an array) into individual elements. It can also be used to clone arrays or objects. For example: ```js const arr = [1, 2, 3]; const newArr = [...arr, 4, 5]; // [1, 2, 3, 4, 5] ```
A `Promise` in JavaScript represents a value that may be available now, in the future, or never. It allows handling of asynchronous operations by providing methods like `.then()`, `.catch()`, and `.finally()`. Promises have three states: `pending`, `fulfilled`, and `rejected`. They allow chaining and error handling in asynchronous code.
Template literals allow for multi-line strings and embedded expressions. They are created using backticks (`` ` ``) instead of quotes. For example: ```js const name = 'John'; const greeting = `Hello, ${name}!`; ```
var has function scope, hoisted with undefined value. let and const have block scope, hoisted but not initialized (temporal dead zone). const prevents reassignment but doesn't make objects immutable. Consider scope implications, use const by default, let when reassignment needed.
Type coercion automatically converts values between types during operations. Implicit coercion occurs in comparisons (== vs ===), operations (+, -, etc.). Can lead to unexpected results. Consider explicit type conversion, use strict equality (===). Example: '5' + 2 results in '52' due to string concatenation.
Hoisting moves declarations to top of scope during compilation. Function declarations fully hoisted with implementation. var declarations hoisted with undefined value. let/const hoisted without initialization. Consider declaration placement, understand scope rules.
'this' refers to current execution context. In methods, refers to object. In global scope, refers to window/global (non-strict) or undefined (strict). In event handlers, refers to event target. Use bind/arrow functions to maintain context. Consider lexical scope.
Seven primitive types: string, number, boolean, null, undefined, symbol, bigint. Immutable values, passed by value. Each has wrapper object (String, Number, etc.). Consider type checking, conversion methods. Understand differences from objects.
Objects inherit properties/methods through prototype chain. Each object has internal [[Prototype]] link. Properties looked up through chain until found or null reached. Use Object.create() or constructor functions. Consider performance, inheritance depth.
Closure allows function to access variables from outer scope even after outer function returns. Used for data privacy, module pattern, partial application. Maintains state between function calls. Consider memory implications, avoid memory leaks.
Events propagate through DOM tree. Capturing phase (top-down), target phase, bubbling phase (bottom-up). Control with addEventListener third parameter. Stop propagation with stopPropagation(). Consider event delegation, performance implications.
Event loop handles asynchronous operations. Manages call stack, callback queue, microtask queue. Executes synchronous code first, then microtasks, then macrotasks. Consider task priorities, understand single-threaded nature.
Use === for strict equality (type and value). == performs type coercion. Object comparison checks reference. Use Object.is() for NaN, +0/-0. Consider value vs reference comparison. Handle null/undefined cases.
Scope chain determines variable access. Inner scope can access outer scope variables. Created when function declared. Affects variable lookup performance. Consider lexical scope, closure implications. Handle naming conflicts.
Automatic memory management through reference counting and mark-and-sweep. Objects garbage collected when unreachable. Handle memory leaks (closures, event listeners). Consider weak references, cleanup patterns.
String literals allowing embedded expressions (${expression}). Support multiline strings, tagged templates. Enable string interpolation. Consider escaping, performance. Handle dynamic content generation.
Object literals, constructor functions, Object.create(), classes. Each method has different prototype behavior. Consider inheritance needs, performance. Handle property descriptors, initialization patterns.
Use Number.EPSILON for floating-point comparison. Handle IEEE 754 limitations. Consider BigInt for large integers. Use toFixed() for display. Implement proper rounding strategies.
Map allows any type as keys, maintains insertion order. Set stores unique values. Both provide efficient lookup. Consider WeakMap/WeakSet for memory. Handle iteration, conversion methods.
Strict mode enables stricter parsing/error handling. Prevents implicit globals, this coercion. Requires variable declaration. Consider compatibility, module behavior. Handle legacy code integration.
Function declarations, expressions, arrow functions, generator functions. Each has different this binding, arguments handling. Consider hoisting behavior, performance implications. Handle scope appropriately.
Use Object.defineProperty(), Object.freeze(), Object.seal(). Control property behavior (writable, enumerable, configurable). Consider deep vs shallow immutability. Handle prototype chain.
undefined represents uninitialized value, null represents intentional absence. Different behavior in type coercion, comparisons. Consider type checking, default values. Handle both cases appropriately.
Use try-catch blocks, error objects, custom errors. Handle async errors with promises. Consider error types, propagation. Implement proper logging, recovery strategies.
Symbols create unique identifiers. Used for private properties, special methods (Symbol.iterator). Non-enumerable by default. Consider well-known symbols, registration. Handle symbol description.
Use libraries (decimal.js), multiplication and division tricks. Handle IEEE 754 limitations. Consider precision requirements. Implement proper rounding. Handle display formatting.
Use get/set keywords, Object.defineProperty(). Control property access/modification. Implement validation, computed properties. Consider performance implications. Handle inheritance.
Use typeof, instanceof, Object.prototype.toString. Handle null/undefined cases. Consider type coercion rules. Implement proper validation. Handle edge cases appropriately.
Use meaningful names, proper casing (camelCase). Declare variables at appropriate scope. Consider hoisting, block scope. Use const by default. Handle naming conflicts.
Function declarations are hoisted with their implementation. Function expressions are assigned to variables, not hoisted. Arrow functions provide lexical this binding. Each type has different scoping rules and use cases. Consider hoisting behavior, this binding, and arguments object availability.
Closures maintain access to their outer scope variables even after the outer function has returned. Used for data privacy, factory functions, and maintaining state. Common in event handlers and callbacks. Consider memory implications and potential memory leaks. Implement cleanup when necessary.
Arguments object contains all passed function arguments. Not available in arrow functions. Array-like but not an array. Use rest parameters for modern alternative. Consider performance implications. Convert to array when needed using Array.from() or spread operator.
Lexical scope determines variable access based on where functions are defined, not where they're called. Affects closure behavior and variable resolution. Inner functions have access to outer scope variables. Consider scope chain performance and variable shadowing.
Default parameters provide fallback values when arguments are undefined. Evaluated at call time. Can reference other parameters and use expressions. Consider undefined vs null behavior. Handle edge cases and parameter dependencies.
IIFEs execute immediately upon definition. Create private scope, avoid global namespace pollution. Common in module pattern implementation. Consider block scope alternatives in modern JavaScript. Handle parameter passing and return values.
Regular functions: 'this' determined by call context. Arrow functions: lexical 'this' from enclosing scope. Method shorthand: bound to object. Consider bind/call/apply usage. Handle event handlers and callback contexts correctly.
Currying transforms multi-argument function into series of single-argument functions. Enables partial application, function composition. Implement using closures or libraries. Consider performance implications. Handle argument validation and error cases.
Rest parameters collect remaining arguments into array. Spread operator expands iterables into individual elements. More flexible than arguments object. Consider performance with large arrays. Handle type checking and validation.
Function composition combines multiple functions into single function. Output of one function becomes input of another. Implement using reduce or specialized functions. Consider error handling and type consistency. Handle asynchronous composition.
Use closures to create private scope. Methods defined inside closure not accessible externally. Return public interface. Consider memory usage and encapsulation. Handle object composition and inheritance.
Generator functions create iterators using yield keyword. Enable lazy evaluation, infinite sequences. Handle memory-efficient iterations. Consider state management between yields. Implement proper error handling and cleanup.
Use tail call optimization where available. Implement iterative alternatives. Consider stack size limitations. Handle base cases properly. Implement memoization for performance. Consider trampoline pattern for deep recursion.
Pure functions always return same output for same input. No side effects, depend only on input arguments. Easier testing, debugging, and reasoning. Consider immutability and referential transparency. Handle external state appropriately.
Cache function results based on input arguments. Improve performance for expensive calculations. Consider cache size, key generation. Handle complex arguments, cleanup. Implement proper cache invalidation strategies.
Function declarations hoisted with implementation. Function expressions hoisted as variables. Affects code organization and accessibility. Consider temporal dead zone with let/const. Handle initialization order properly.
Check arguments length/type for different behaviors. Use default parameters, rest parameters. Consider function factories. Handle type checking and validation. Implement proper error handling for invalid cases.
Functions that take/return other functions. Enable function composition, callbacks, decorators. Common in functional programming patterns. Consider scope implications. Handle function context and arguments properly.
Block scope limited to block (let/const). Function scope spans entire function (var). Affects variable accessibility and lifetime. Consider hosting behavior. Handle nested scopes and shadowing properly.
bind creates new function with fixed this. call/apply execute function immediately with specified this. Arrow functions maintain lexical this. Consider performance implications. Handle partial application cases.
Functions that create and return other functions. Use closures for configuration, state. Handle parameter customization. Consider memory usage. Implement proper initialization and cleanup.
Functions that modify or enhance other functions. Add logging, memoization, validation. Maintain function properties and context. Consider chaining capabilities. Handle asynchronous decorators.
Use arrow functions or bind for correct this context. Consider closure implications. Handle cleanup properly. Implement proper error handling. Consider performance with many handlers.
Partial application fixes some arguments, returns function expecting remainder. Currying always returns single-argument function. Consider implementation differences. Handle argument order. Implement proper error checking.
Throttle limits execution frequency. Debounce delays execution until pause in calls. Use closure for state. Handle timer cleanup. Consider immediate execution option. Implement proper error handling.
Async functions maintain their own execution context. Handle promise chain scope. Consider error handling scope. Implement proper cleanup. Handle concurrent execution contexts.
Modules create their own scope. Export/import controls accessibility. Consider module closure effects. Handle circular dependencies. Implement proper encapsulation strategies.
Function prototypes define shared behavior. Enable method inheritance. Consider performance implications. Handle prototype chain properly. Implement proper initialization patterns.
Return this from methods to enable chaining. Consider immutable alternatives. Handle errors in chain. Implement proper method dependencies. Consider builder pattern implementation.
Objects can be created using object literals, constructor functions, Object.create(), and class syntax. Object literals provide simple key-value structure. Constructor functions enable prototype-based inheritance. Classes offer more familiar OOP syntax. Consider encapsulation needs and inheritance requirements when choosing creation method.
Prototypal inheritance uses prototype chain for property/method lookup, objects inherit directly from other objects. Classical inheritance uses class blueprints. Prototypal offers more flexibility but requires careful handling of prototype chain. Consider performance implications and inheritance depth.
Property descriptors control property behavior through attributes: writable, enumerable, configurable, get/set. Object.defineProperty() sets descriptors. Affects property modification, enumeration, deletion. Consider immutability needs and access control requirements.
Private fields implemented using # prefix in classes, closures for privacy in constructor functions, WeakMap for truly private data. Consider encapsulation requirements, compatibility needs. Handle inheritance of private members appropriately.
Object.create() establishes direct prototype link without constructor call. Constructor functions create instances with new keyword, initialize with constructor code. Object.create() offers more control over property descriptors. Consider initialization needs and prototype chain requirements.
Return this from methods to enable chaining. Ensures methods return object instance. Consider immutability implications, error handling in chain. Implement proper method dependencies. Document chain requirements and limitations.
Mixins combine properties/methods from multiple sources into objects. Alternative to multiple inheritance. Implement using Object.assign() or custom mixing functions. Consider property conflicts, initialization order. Handle method composition properly.
Getters/setters enable computed properties, access control. Defined using get/set keywords or Object.defineProperty(). Enable validation, computed values. Consider performance implications of computations. Handle recursive calls properly.
SOLID principles guide OOP design: Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion. Apply through proper class/object design. Consider JavaScript's dynamic nature when implementing. Ensure maintainable, extensible code.
Observer pattern enables event-driven communication between objects. Implement subscribe/unsubscribe methods, notification mechanism. Consider memory management, event handling. Handle observer lifecycle properly. Implement cleanup for removed observers.
Compose objects using delegation, aggregation, or mixing. Favor composition over inheritance. Consider interface design, dependency management. Handle lifecycle of composed objects. Implement proper cleanup procedures.
Classes provide cleaner syntax, enforce new usage, enable private fields. Constructor functions offer more flexibility, explicit prototype manipulation. Classes automatically run in strict mode. Consider compatibility requirements and feature needs.
Use appropriate enumeration method: Object.keys() for own enumerable properties, for...in for all enumerable properties including prototype chain. Consider property descriptors, inheritance. Handle non-enumerable properties when needed.
Singleton ensures single instance existence. Implement using static property/closure. Consider lazy initialization, thread safety. Handle instance access properly. Document usage restrictions and initialization requirements.
Override methods by redefining in child class/object. Access parent methods using super keyword or prototype chain. Consider composition alternatives. Handle proper method signature matching. Document overridden behavior.
Symbols create unique property keys, enable metaprogramming features. Used for private-like properties, special methods (iterators). Consider well-known symbols, property conflicts. Handle symbol description and registration appropriately.
Implement custom equality methods, compare relevant properties. Consider deep vs shallow comparison. Handle circular references, type differences. Implement proper value comparison logic. Document comparison criteria.
Module pattern uses closures for encapsulation. Modern alternatives include ES modules, classes with private fields. Consider compatibility requirements, encapsulation needs. Handle initialization and cleanup properly.
Use JSON.stringify/parse with custom replacer/reviver. Handle circular references, special types (Date, Map). Consider security implications, data validation. Implement proper error handling. Document serialization format.
Command pattern encapsulates actions as objects. Enables undo/redo, action queuing. Consider command validation, execution context. Handle command parameters properly. Implement proper error handling and rollback.
Use Object.freeze(), implement proper copying methods. Consider deep freezing requirements. Handle updates through new object creation. Implement efficient update patterns. Document immutability guarantees.
Proxies enable custom behavior for fundamental operations. Implement property access control, validation, logging. Consider performance implications. Handle trap implementation properly. Document proxy behavior and limitations.
Strategy pattern enables interchangeable algorithms. Implement through object composition, function properties. Consider strategy selection logic, default strategies. Handle strategy initialization and switching properly.
Decorator pattern adds behavior dynamically. Implement through object composition or class inheritance. Consider decoration order, interface consistency. Handle proper method delegation. Document decorator capabilities.
Implement proper initialization, cleanup methods. Handle resource management, event listeners. Consider garbage collection implications. Implement proper destruction sequence. Document lifecycle requirements.
Value objects represent immutable values, implement value equality. Consider hash code implementation, comparison methods. Handle proper immutability. Document value semantics and usage.
Pass dependencies through constructor or setter methods. Consider dependency lifecycle, initialization order. Handle optional dependencies properly. Implement proper dependency validation. Document dependency requirements.
Follow SOLID principles, use appropriate patterns. Consider JavaScript's prototype nature. Handle proper encapsulation, inheritance. Implement clear interfaces. Document design decisions and rationale.
Array.map() creates a new array with transformed elements, returning the result of applying a function to each element. In contrast, forEach() executes a function for each element but returns undefined, primarily used for side effects. Map preserves array length and maintains a one-to-one relationship with elements, making it ideal for data transformation. Consider performance implications for large arrays and memory usage when choosing between them.
reduce() processes array elements from left to right, while reduceRight() processes from right to left. Both methods take an accumulator and current value, combining elements into a single result. They're useful for sum calculations, object transformations, and array flattening. Consider initial value provision to avoid type errors and unexpected results with empty arrays. The direction matters particularly when order affects the result, such as string concatenation or mathematical operations.
slice() creates a shallow copy of array portion without modifying the original array, taking start and end indices. splice() modifies the original array by removing, replacing, or adding elements, returning removed elements. slice() is used for array copying and extraction, while splice() is for array mutation. Consider immutability requirements when choosing between them. splice() changes array length and indices, requiring careful handling in iterations.
Array.find() returns first matching element, while filter() returns all matching elements. For sorted arrays, consider binary search implementation for better performance. indexOf() and includes() check element existence. For complex objects, use custom comparison functions. Consider performance implications with large arrays and implement proper indexing strategies for frequent searches.
Use immutable methods (map, filter, reduce) for data transformation. Implement proper error handling for array operations. Consider performance with large arrays. Use appropriate array methods instead of loops when possible. Handle sparse arrays and holes appropriately. Maintain type consistency within arrays. Document array structure and element requirements.
Array.sort() accepts comparison function returning negative, zero, or positive values. Implement stable sorting for consistent results. Handle special values (null, undefined) appropriately. Consider case sensitivity and locale-specific sorting. Document comparison logic and requirements. Implement proper error handling for invalid comparisons.
Typed arrays provide fixed-length arrays of specific numeric types. Used for binary data manipulation, WebGL, and performance-critical operations. Consider memory usage and numeric precision requirements. Handle endianness appropriately. Implement proper bounds checking and type conversion. Document data format requirements.
Use Set object for simple value deduplication. Implement custom comparison for objects. Consider memory usage with large arrays. Handle reference equality vs value equality appropriately. Implement efficient algorithms for complex objects. Document deduplication criteria and requirements.
WeakMap and WeakSet hold weak references to objects, allowing garbage collection of keys. Used for private data storage, caching, and memory-sensitive operations. Consider garbage collection implications. Handle key object lifecycle properly. Implement proper cleanup strategies. Document memory management requirements.
Use Array.flat() or reduce() for nested array flattening. Consider depth of nesting and performance implications. Handle circular references appropriately. Implement custom flattening for complex structures. Document flattening depth requirements. Handle special cases and error conditions.
Pre-allocate array size when possible. Use appropriate array methods for operations. Consider memory usage and garbage collection. Implement proper caching strategies. Handle large array operations efficiently. Document performance requirements and constraints. Monitor array operation performance.
Array-like objects have length property and indexed elements but lack array methods. Convert to arrays using Array.from() or spread operator. Consider performance implications of conversion. Handle proper method delegation. Document array-like object requirements. Implement proper type checking.
Consider holes in array iteration. Use appropriate methods for sparse array operations. Handle undefined values properly. Implement proper array compaction when needed. Document sparse array handling requirements. Consider performance implications.
Use spread operator or slice() for shallow copy. Implement deep cloning for nested structures. Handle circular references appropriately. Consider performance implications of deep cloning. Document cloning requirements. Implement proper validation.
Release references to unused arrays. Handle large array cleanup properly. Consider garbage collection implications. Implement proper array reuse strategies. Document memory management requirements. Monitor memory usage patterns.
Use appropriate comparison methods for element types. Handle object comparison properly. Implement custom comparison functions when needed. Consider performance implications. Document comparison requirements. Handle special values appropriately.
Implement efficient chunking algorithms. Handle remainder elements properly. Consider memory usage with large arrays. Implement proper validation. Document chunking requirements. Handle edge cases appropriately.
Use appropriate locking mechanisms. Handle race conditions properly. Implement proper synchronization. Consider performance implications. Document concurrency requirements. Handle error conditions appropriately.
Use appropriate serialization format. Handle circular references. Implement proper type conversion. Consider performance implications. Document serialization requirements. Handle error conditions appropriately.
Use Set for unique values. Implement efficient algorithms. Handle object comparison properly. Consider performance implications. Document set operation requirements. Handle edge cases appropriately.
Use stable sorting algorithms when needed. Handle special values properly. Consider performance implications. Implement proper comparison functions. Document stability requirements. Handle edge cases appropriately.
Use Fisher-Yates algorithm for unbiased shuffling. Implement proper randomization. Consider performance implications. Document shuffling requirements. Handle edge cases appropriately. Implement validation.
Implement efficient rotation algorithms. Handle different rotation directions. Consider performance implications. Document rotation requirements. Handle edge cases appropriately. Implement proper validation.
Implement proper index validation. Handle negative indices appropriately. Consider performance implications. Document boundary requirements. Handle error conditions appropriately. Implement proper error messages.
Use Set for primitive values. Implement custom comparison for objects. Consider performance implications. Document uniqueness requirements. Handle edge cases appropriately. Implement proper validation.
Promises provide structured handling of asynchronous operations with chaining capabilities and built-in error handling through .then() and .catch(). Unlike callbacks, which can lead to callback hell, Promises enable cleaner code organization and better error propagation. Promises are preferred for modern asynchronous operations, while callbacks remain relevant for event handling and legacy code integration. Consider error handling requirements and code maintainability when choosing between them.
async/await provides synchronous-looking code for asynchronous operations, improving readability and maintainability. async functions automatically return Promises, and await pauses execution until Promises resolve. However, they can't be used in regular functions or at the top level (pre-ES modules). Error handling requires try/catch blocks, and parallel execution needs careful consideration. Consider performance implications and proper error handling strategies when using async/await.
Promise.all() handles multiple concurrent Promises, resolving when all complete. Promise.race() resolves with the first completed Promise. Promise.allSettled() waits for all Promises regardless of success/failure. Promise.any() resolves with first successful Promise. Consider error handling, timeout implementation, and performance optimization when working with multiple Promises. Implement proper cleanup for rejected Promises.
Use Promise catch() blocks or try/catch with async/await. Implement proper error propagation and recovery strategies. Handle both synchronous and asynchronous errors appropriately. Consider error types and appropriate responses. Implement logging and monitoring for asynchronous errors. Ensure proper cleanup on error conditions.
The event loop manages execution of asynchronous operations by processing the call stack, callback queue, and microtask queue. Microtasks (Promises) have priority over macrotasks (setTimeout, setInterval). Understanding the event loop is crucial for performance optimization and preventing blocking operations. Consider task prioritization and proper sequence handling.
Implement proper synchronization mechanisms using Promise.race() or flags. Handle out-of-order responses appropriately. Consider cancellation patterns and cleanup. Implement proper state management for concurrent operations. Document race condition handling strategies. Test edge cases thoroughly.
Use Promise.race() with timeout Promise, or implement custom timeout logic. Handle cleanup for timed-out operations. Consider appropriate timeout durations and retry strategies. Implement proper error messaging for timeouts. Handle partial completions appropriately. Document timeout behavior and recovery procedures.
Use finally blocks or cleanup functions in Promise chains. Implement proper cancellation patterns. Handle resource release in error cases. Consider memory management and leak prevention. Document cleanup requirements and procedures. Implement proper error handling during cleanup.
Return values from then() handlers for proper chaining. Implement proper error handling at each step. Consider Promise flattening and composition. Handle side effects appropriately. Document chain dependencies and requirements. Implement proper cleanup in chain breaks.
Implement exponential backoff strategy. Handle maximum retry attempts. Consider error types for retry decisions. Implement proper timeout handling. Document retry behavior and limitations. Handle permanent failures appropriately. Consider resource implications of retries.
Use Promise.all() for parallel execution, implement proper rate limiting. Handle partial failures appropriately. Consider dependency order and prioritization. Implement proper error aggregation. Document concurrency limitations and requirements.
Use AbortController for fetch requests, implement custom cancellation tokens. Handle cleanup on cancellation. Consider partial completion handling. Implement proper state management. Document cancellation behavior and limitations.
Async iterators enable asynchronous iteration using for-await-of. Async generators combine generator and async functionality. Handle proper error propagation. Consider memory usage and cleanup. Document iteration behavior and requirements. Implement proper cancellation.
Implement proper cleanup of event listeners and subscriptions. Handle reference cleanup in closures. Consider weak references when appropriate. Implement proper cancellation patterns. Monitor memory usage. Document cleanup requirements.
Implement proper loading states and dependencies. Handle initialization errors appropriately. Consider lazy initialization patterns. Document initialization requirements and sequence. Implement proper cleanup on failed initialization.
Use closures to manage timing. Implement proper cleanup of timers. Consider immediate execution options. Handle edge cases appropriately. Document timing behavior and requirements. Implement proper cancellation.
Use async test frameworks. Implement proper assertions for async results. Handle timeout and error cases. Consider test isolation and cleanup. Document test requirements and assumptions. Implement proper mock timing.
Consider event order and timing. Handle proper error propagation. Implement cleanup on handler removal. Consider memory implications. Document handler behavior and requirements. Implement proper state management.
Handle concurrent execution limits. Implement proper queue management. Consider priority handling. Handle error cases appropriately. Document queue behavior and limitations. Implement proper cleanup.
Implement proper loading and error states. Handle race conditions appropriately. Consider optimistic updates. Implement proper cleanup on state changes. Document state transitions and requirements. Handle edge cases.
Implement proper fallback mechanisms. Handle partial failures appropriately. Consider retry strategies. Document recovery procedures and limitations. Implement proper state restoration. Handle cleanup during recovery.
Handle async context preservation. Implement proper error tracking. Consider performance impact. Document logging requirements and format. Implement proper cleanup of log resources. Handle sensitive data appropriately.
Implement proper dependency resolution. Handle circular dependencies appropriately. Consider initialization order. Document dependency requirements and limitations. Implement proper cleanup. Handle dependency failures.
Implement proper concurrency control. Consider caching strategies. Handle batch operations appropriately. Document performance requirements and limitations. Implement proper monitoring. Handle resource constraints.
Implement proper backpressure handling. Consider memory usage and buffering. Handle error propagation appropriately. Document stream behavior and limitations. Implement proper cleanup. Handle partial processing.
Handle error propagation appropriately. Implement proper recovery mechanisms. Consider error scope and isolation. Document error handling requirements. Implement proper cleanup. Handle nested errors.
Implement proper initialization and cleanup. Handle event order appropriately. Consider dependency management. Document lifecycle requirements. Implement proper error handling. Handle partial completion.
Handle error context preservation. Implement proper error aggregation. Consider error categorization. Document reporting requirements. Implement proper cleanup. Handle sensitive information appropriately.
Document async behavior and requirements clearly. Consider error cases and handling. Document cleanup requirements. Implement proper examples and usage patterns. Consider versioning and compatibility. Handle API documentation appropriately.
The primary methods for DOM selection include getElementById (fastest, returns single element), querySelector (flexible, returns first match), querySelectorAll (returns static NodeList), getElementsByClassName and getElementsByTagName (return live HTMLCollections). Each method has different performance characteristics. getElementById provides the best performance for single element selection due to unique ID requirements. querySelector and querySelectorAll offer more flexibility with CSS selector syntax but may be slower for complex selectors. Consider caching DOM selections for frequently accessed elements to optimize performance.
DOM updates should be batched to minimize browser reflows and repaints. Use DocumentFragment for multiple insertions, modify elements off-DOM using cloneNode, and apply multiple style changes through CSS class toggles rather than individual style properties. Measure critical dimensions before making changes that could trigger reflow. Cache computed styles when needed for multiple reads. Consider using requestAnimationFrame for visual updates to ensure smooth animations and prevent layout thrashing. Implement proper cleanup for removed elements and event listeners.
Event delegation involves attaching event listeners to parent elements to handle events on descendants, leveraging event bubbling. This pattern improves performance by reducing the number of event listeners, handles dynamically added elements automatically, and reduces memory usage. Implement proper event target filtering using matches() or closest(). Consider event propagation and stopping mechanisms. Handle cleanup properly when removing parent elements. Document delegation patterns and requirements for maintainability.
When removing DOM elements, ensure proper cleanup by removing event listeners, clearing references in JavaScript, and handling any associated resources. Use removeEventListener for explicit event handler removal, clean up any intersection or mutation observers, and handle cleanup of third-party library integrations. Consider memory leak prevention, especially with closures and cached element references. Document cleanup requirements and implement proper error handling during cleanup processes.
innerHTML parses content as HTML, allowing element creation but presenting security risks with unsanitized input. textContent provides raw text access, ignoring styling and hidden elements, offering better performance. innerText respects CSS styling and visibility, triggering reflow. Consider security implications when using innerHTML, especially with user input. Use textContent for performance-critical text updates. Document content handling approaches and sanitization requirements.
Efficient DOM traversal utilizes properties like parentNode, children, nextSibling, and previousSibling. Consider using childNodes vs children based on needs (text nodes vs elements). Implement proper caching of frequently accessed nodes. Use appropriate iteration methods for NodeList and HTMLCollection objects. Handle cross-browser compatibility issues. Document traversal patterns and performance considerations.
MutationObserver monitors DOM changes, useful for dynamic content updates and third-party script integration. IntersectionObserver tracks element visibility, ideal for lazy loading and infinite scroll implementations. Both provide efficient alternatives to polling or scroll event listeners. Implement proper cleanup and disconnection. Consider performance implications and observation options. Document observer usage patterns and requirements.
Handle cross-browser differences through feature detection rather than browser detection. Implement appropriate fallbacks for unsupported features. Consider polyfills for broader compatibility. Test across different browsers and versions. Document browser support requirements and limitations. Implement graceful degradation strategies. Handle vendor prefix differences appropriately.
Minimize DOM access and updates by caching references and batching changes. Use document fragments for multiple insertions. Avoid forced synchronous layouts. Implement proper event delegation. Consider repaint and reflow implications. Use requestAnimationFrame for visual updates. Document performance requirements and optimization strategies. Monitor and profile DOM operations.
Implement form validation using both HTML5 validation attributes and JavaScript validation. Handle form submission events properly. Implement custom validation UI and error messages. Consider accessibility requirements. Handle form reset and clear operations. Document validation rules and requirements. Implement proper error handling and user feedback.
Data attributes (data-*) store custom data within HTML elements, accessible via dataset property. Use for configuration data, state management, and element identification. Avoid storing sensitive information. Consider naming conventions and documentation. Implement proper validation of data attribute values. Handle updates and changes appropriately.
Implement focus management considering accessibility requirements. Handle focus trapping in modals. Implement proper tabindex usage. Consider keyboard navigation patterns. Handle focus restoration after dynamic updates. Document focus management requirements. Implement proper error handling for focus operations.
Handle view updates efficiently without full page reloads. Implement proper cleanup between view changes. Consider memory management and event listener cleanup. Handle browser history and navigation. Document view lifecycle requirements. Implement proper error handling for view transitions.
Implement efficient content loading strategies using fetch or XMLHttpRequest. Handle loading states appropriately. Consider progressive enhancement. Implement proper error handling and retry logic. Document loading requirements and dependencies. Handle cleanup of loaded content when necessary.
Prevent XSS attacks by sanitizing input before insertion. Avoid eval() and innerHTML with untrusted content. Implement proper Content Security Policy. Consider iframe sandbox requirements. Document security requirements and practices. Implement proper input validation and sanitization.
Use HTML5 Drag and Drop API or implement custom drag and drop logic. Handle drag events properly. Implement proper visual feedback. Consider accessibility requirements. Handle cross-browser differences. Document drag and drop requirements. Implement proper cleanup after drag operations.
Use media queries and matchMedia for responsive behavior. Handle resize events efficiently. Implement proper breakpoint management. Consider device capabilities. Document responsive requirements. Implement proper cleanup of responsive handlers. Handle orientation changes appropriately.
Use CSS transitions when possible, falling back to JavaScript animations when needed. Implement proper animation cleanup. Consider performance implications. Handle animation completion and cancellation. Document animation requirements. Implement proper error handling for animations.
Implement scroll event throttling or debouncing. Use IntersectionObserver when possible. Handle scroll position restoration. Consider scroll performance optimization. Document scroll handling requirements. Implement proper cleanup of scroll handlers.
Render only visible elements while maintaining scroll position and size. Implement efficient item recycling. Handle dynamic content heights. Consider memory usage optimization. Document virtual scrolling requirements. Implement proper cleanup and memory management.
Handle touch events for mobile devices. Implement proper gesture recognition. Consider multi-touch interactions. Handle touch event delegation properly. Document touch interaction requirements. Implement proper cleanup of touch handlers.
Implement proper keyboard navigation. Handle keyboard shortcuts appropriately. Consider focus management. Document keyboard interaction requirements. Implement proper error handling for keyboard events. Handle modifier keys appropriately.
Handle positioning and visibility. Implement proper event handling. Consider accessibility requirements. Handle cleanup on removal. Document tooltip behavior requirements. Implement proper error handling for positioning calculations.
Handle DOM operation failures gracefully. Implement proper error recovery. Consider user feedback mechanisms. Document error handling requirements. Implement proper cleanup after errors. Handle async operation failures appropriately.
Handle cross-origin communication properly. Implement proper sandbox restrictions. Consider security implications. Handle iframe loading states. Document iframe requirements. Implement proper cleanup when removing iframes.
Synchronize DOM with application state efficiently. Handle state transitions properly. Consider optimistic updates. Implement proper error recovery. Document state management requirements. Handle cleanup during state changes.
Implement proper DOM virtualization. Handle memory management efficiently. Consider performance optimization. Document large DOM requirements. Implement proper cleanup strategies. Handle updates efficiently.
Implement proper unit and integration tests. Handle async operations in tests. Consider test isolation. Document testing requirements. Implement proper cleanup after tests. Handle test environment differences.
JavaScript provides several built-in error types for specific scenarios. TypeError indicates incorrect type operations, ReferenceError signals undefined variable access, SyntaxError represents parsing issues, RangeError indicates invalid numeric ranges, and Error serves as the base class. Custom errors should extend Error for specific application needs. When throwing errors, select the most appropriate type to facilitate debugging and error handling. Consider error message clarity and include relevant context for effective troubleshooting.
Asynchronous error handling requires careful consideration of Promise chains and async/await patterns. Use catch blocks strategically in Promise chains to handle specific error types. With async/await, implement try-catch blocks around await statements. Consider error propagation, implement proper cleanup in finally blocks, and handle uncaught rejections with window.onunhandledrejection. Ensure proper error logging and monitoring for production environments. Document error recovery strategies and implement appropriate fallback mechanisms.
Modern browsers offer comprehensive debugging tools including breakpoints, step debugging, watch expressions, and call stack analysis. Chrome DevTools and Firefox Developer Tools provide source maps support, network request monitoring, and performance profiling. Utilize console methods beyond console.log, such as console.trace for stack traces, console.table for structured data, and console.group for organized logging. Implement proper source map generation in build process for accurate debugging of minified code.
Custom error types should extend the built-in Error class and include additional context relevant to the application domain. Implement proper stack trace capture, maintain error hierarchies for specific error categories, and include helpful error messages. Consider serialization needs for error reporting, implement proper instanceof behavior, and document error type usage patterns. Ensure custom errors integrate well with existing error handling mechanisms.
Production error logging requires careful consideration of security, performance, and debugging needs. Implement structured logging formats, include relevant context without sensitive data, and ensure proper error aggregation. Use logging services with appropriate security measures, implement rate limiting for error reporting, and maintain error categorization. Consider log rotation, implement proper cleanup, and document logging requirements.
Memory leak detection requires systematic approach using Chrome DevTools Memory panel or similar tools. Profile memory usage, analyze heap snapshots, and identify retained objects. Common causes include undisposed event listeners, closure references, and detached DOM elements. Implement proper cleanup mechanisms, monitor memory usage patterns, and document memory management requirements. Regular profiling helps identify potential issues early.
Production debugging requires comprehensive error logging, source map support, and monitoring tools. Implement detailed error tracking, maintain proper logging levels, and ensure stack traces are preserved. Use feature flags for gradual rollouts, implement proper versioning, and maintain debugging endpoints. Consider performance impact of debugging features and implement appropriate security measures.
Error boundaries provide containment for runtime errors, preventing application-wide crashes. Implement hierarchical error handling, provide fallback UI components, and maintain proper error reporting. Consider component isolation, implement recovery mechanisms, and document error boundary behavior. Handle async errors appropriately and maintain proper cleanup procedures.
Third-party script errors require careful isolation and error handling strategies. Implement proper script loading error handling, use try-catch blocks around third-party code execution, and maintain error boundaries. Consider script timeout handling, implement fallback mechanisms, and document integration requirements. Monitor third-party script performance and errors separately.
Error recovery requires systematic approach to restore application state and functionality. Implement proper state backup mechanisms, handle partial failures appropriately, and provide user feedback. Consider recovery priorities, implement proper retry mechanisms, and document recovery procedures. Maintain proper cleanup during recovery process.
Performance debugging utilizes browser performance tools, profiling, and timeline analysis. Use Performance panel for runtime analysis, identify bottlenecks through flame charts, and analyze rendering performance. Consider network optimization, implement proper performance monitoring, and document performance requirements. Regular profiling helps maintain optimal performance.
API error handling requires comprehensive strategy for different error types and scenarios. Handle network errors, timeout conditions, and invalid responses appropriately. Implement retry mechanisms, maintain proper error status mapping, and provide user feedback. Consider error response formats, implement proper validation, and document API error handling requirements.
Event-driven code debugging requires understanding of event flow and timing. Use debugger statements strategically, implement proper event logging, and analyze event sequences. Consider async event handling, implement proper event cleanup, and document event debugging approaches. Maintain proper event monitoring in production.
Worker thread error handling requires proper message passing and error propagation. Implement error event listeners, handle termination conditions, and maintain proper cleanup. Consider worker lifecycle management, implement proper error reporting, and document worker error handling requirements. Handle resource cleanup appropriately.
Error messages should be clear, actionable, and provide appropriate context. Include error codes, relevant details, and recovery steps when applicable. Consider internationalization requirements, implement proper message formatting, and maintain consistent error message structure. Document error message standards and maintain proper validation.
Micro-frontend error tracking requires coordinated error handling strategy. Implement proper error propagation between components, maintain consistent error reporting, and handle cross-application errors. Consider error boundary implementation, maintain proper error isolation, and document integration requirements.
Race condition debugging requires systematic analysis of timing and state changes. Implement proper logging of state transitions, use async debugging tools, and analyze execution order. Consider concurrent operation handling, implement proper synchronization, and document race condition patterns.
Component lifecycle error handling requires proper error boundaries and cleanup procedures. Implement error handling for initialization, update, and cleanup phases. Consider state consistency, implement proper error recovery, and document lifecycle error patterns. Maintain proper error isolation between components.
Minified code debugging requires proper source map integration and build process configuration. Maintain source map generation, implement proper error stack translation, and ensure proper file organization. Consider source map security, implement proper deployment procedures, and document debugging setup requirements.
Environment-specific error reporting requires appropriate configuration and handling. Implement proper error filtering, maintain environment-specific logging levels, and handle sensitive data appropriately. Consider compliance requirements, implement proper security measures, and document environment-specific procedures.
Memory consumption debugging requires systematic analysis using browser memory tools. Profile heap usage, analyze allocation patterns, and identify memory leaks. Consider garbage collection impact, implement proper memory monitoring, and document memory optimization strategies. Regular profiling helps maintain optimal memory usage.
Service worker error handling requires proper event handling and recovery mechanisms. Implement error listeners, handle installation and activation errors, and maintain proper cleanup. Consider offline operation handling, implement proper caching strategies, and document service worker error patterns.
Recursive function debugging requires careful analysis of call stack and state changes. Implement proper logging at each recursion level, use conditional breakpoints, and analyze termination conditions. Consider stack overflow prevention, implement proper error handling, and document recursive patterns.
Client-side routing error monitoring requires proper route change handling and error tracking. Implement route transition error handling, maintain proper history state, and handle navigation errors. Consider deep linking issues, implement proper error recovery, and document routing error patterns.
Validation error handling requires comprehensive strategy for different validation scenarios. Implement proper error collection, maintain error state, and provide appropriate user feedback. Consider form validation timing, implement proper error display, and document validation requirements.
WebSocket error tracking requires proper connection state monitoring and error handling. Implement connection error handling, manage reconnection attempts, and maintain proper cleanup. Consider network conditions, implement proper timeout handling, and document connection error patterns.
Application state debugging requires proper state monitoring and analysis tools. Use Redux DevTools or similar state inspection tools, implement state logging, and analyze state transitions. Consider complex state interactions, implement proper state validation, and document state debugging procedures.
Browser extension error handling requires proper isolation and error reporting. Implement content script error handling, manage background script errors, and maintain proper communication error handling. Consider cross-origin restrictions, implement proper security measures, and document extension-specific requirements.
Mobile browser error handling requires consideration of device-specific issues and limitations. Handle touch event errors, manage memory constraints, and implement proper offline error handling. Consider mobile network conditions, implement proper performance monitoring, and document mobile-specific requirements.
Arrow functions provide a concise syntax for writing function expressions and maintain lexical this binding. Unlike traditional functions, arrow functions do not create their own this context, inheritance comes from the enclosing scope. They cannot be used as constructors, do not have their own arguments object, and are always anonymous. Consider using arrow functions for callbacks and methods that don't require their own this context.
Template literals provide enhanced string functionality through backtick syntax, supporting multi-line strings, string interpolation with ${expression}, and tagged templates for custom string processing. They eliminate the need for string concatenation and escape sequences for line breaks. Tagged templates enable powerful string manipulation through custom processing functions. Consider performance implications when using complex expressions in interpolation.
Destructuring enables extracting values from arrays or properties from objects into distinct variables using a concise syntax. It supports default values, nested destructuring, and rest parameters. This feature significantly improves code readability when working with complex data structures and function parameters. Consider using destructuring in function parameters and import statements for cleaner code organization.
ES6 modules provide a standardized way to organize and share code between JavaScript files. They support named and default exports/imports, enabling better code organization and encapsulation. Modules are always in strict mode, have their own scope, and are executed only once when imported. Consider proper module organization, circular dependency handling, and build tool configuration for module bundling.
The spread operator (...) expands elements while the rest operator collects elements into an array. Spread is useful for array manipulation, object copying, and function arguments. Rest parameters enable handling variable numbers of arguments in functions. Consider performance implications with large arrays and proper type checking when using these operators.
ES6 classes provide a clearer syntax for object-oriented programming, supporting inheritance through extends, constructor methods, static methods, and getter/setter syntax. They enforce new for instantiation and automatically enable strict mode. While classes are primarily syntactic sugar over prototypes, they improve code organization and readability. Consider proper inheritance hierarchies and encapsulation patterns.
Promises represent eventual completion of asynchronous operations, providing a cleaner alternative to callbacks. They support chaining through .then(), error handling with .catch(), and cleanup with .finally(). Promises help avoid callback hell and provide better error propagation. Consider proper error handling, Promise composition patterns, and understanding of the microtask queue.
Map allows any type as keys and maintains insertion order, while Set stores unique values. Both provide efficient lookup and iteration methods. WeakMap and WeakSet variants enable proper garbage collection of key objects. Consider use cases for each collection type, proper cleanup handling, and performance implications.
Symbols are unique and immutable primitive values, often used as property keys to avoid name collisions. They provide a way to create non-enumerable object properties and define well-known symbols for language-level behaviors. Consider Symbol usage for private-like properties, proper Symbol registration, and handling Symbol serialization.
Generators provide a way to define iterative algorithms by writing functions that can be paused and resumed. They work with the iteration protocols, enabling custom iteration behavior. Generators are useful for handling streams of data and implementing complex control flow. Consider proper error handling, memory usage, and understanding of the yield keyword.
Enhanced object literals support shorthand property and method definitions, computed property names, and super references. These features make object creation more concise and flexible. Consider proper usage of computed properties, method shorthand limitations, and super reference constraints.
async/await provides synchronous-looking code for asynchronous operations, building on Promises. It improves code readability, error handling through try/catch, and maintains proper error stack traces. Consider proper error handling, understanding of the event loop, and limitations in certain contexts.
Default parameters allow specifying default values for function parameters when no argument is provided. They support expressions and previous parameter references. This feature improves function flexibility and reduces boilerplate code. Consider evaluation timing of default values and proper parameter ordering.
Proxy enables custom behavior for fundamental object operations, while Reflect provides methods for controlled object manipulation. These features enable powerful metaprogramming capabilities, including property access interception and custom validation. Consider performance implications and proper trap implementation.
let and const provide block scoping, preventing hoisting issues and temporal dead zone considerations. const prevents reassignment but not object mutation. These declarations improve code predictability and help catch errors early. Consider using const by default and let only when reassignment is necessary.
Tagged template literals allow custom processing of template literal strings and expressions through tag functions. They enable DSL creation, sanitization, and localization features. Consider proper tag function implementation, performance implications, and security considerations.
New array methods include find(), findIndex(), includes(), flat(), and flatMap(). These methods enhance array manipulation capabilities and improve code readability. Consider proper method selection, performance implications, and polyfill requirements for older browsers.
WeakMap and WeakSet hold weak references to objects, allowing garbage collection of key objects when no other references exist. They are useful for storing metadata about objects without preventing their cleanup. Consider proper use cases, limitations, and garbage collection behavior.
ES6+ adds features like named capture groups, lookbehind assertions, and the sticky flag. These enhancements improve regular expression capabilities and maintainability. Consider compatibility requirements, proper pattern construction, and performance implications.
Private class fields and methods (marked with #) provide true privacy in class definitions. They are enforced by the JavaScript engine and not accessible outside the class. Consider proper encapsulation patterns, inheritance implications, and compatibility requirements.
Improvements include rest parameters, default parameters, and destructuring in parameters. These features provide more flexible and expressive function definitions. Consider proper parameter ordering, destructuring patterns, and compatibility requirements.
BigInt enables working with large integers beyond Number.MAX_SAFE_INTEGER. Numeric separators improve readability of large numbers. Consider type coercion rules, performance implications, and proper numeric literal formatting.
Dynamic import() enables loading modules on demand, returning a Promise for module loading. This feature enables code splitting and conditional module loading. Consider proper error handling, bundling configuration, and performance optimization.
Destructuring patterns support default values for both object properties and array elements. Default values are used when the extracted value is undefined. Consider proper default value expressions, nested destructuring, and undefined handling.
New string methods include startsWith(), endsWith(), includes(), padStart(), and padEnd(). These methods improve string manipulation capabilities and reduce the need for regular expressions. Consider proper method selection and compatibility requirements.
Optional chaining (?.) provides safe property access, while nullish coalescing (??) provides default values for null/undefined. These operators improve code safety and reduce boilerplate. Consider proper operator usage and compatibility requirements.
These methods provide different levels of object immutability. freeze() prevents all modifications, seal() prevents property addition/deletion, and preventExtensions() prevents property addition. Consider proper immutability requirements and performance implications.
Static class fields and methods belong to the class itself rather than instances. They provide utility functionality and shared state at the class level. Consider proper usage patterns, inheritance behavior, and initialization timing.
Spread operator and Object.assign() provide improved methods for shallow copying arrays and objects. Consider proper deep copying requirements, performance implications, and prototype handling.
The Singleton pattern ensures a class has only one instance and provides global access to it. In modern JavaScript, it's implemented using a combination of class definition and module system. The implementation should include private instance storage, lazy initialization, and proper export mechanics. Consider thread safety in concurrent environments, implement proper instance checking, and ensure proper cleanup in testing environments. Handle proper initialization timing and instance access synchronization.
The Observer pattern enables objects to notify multiple observers about changes in their state. Implementation involves maintaining a list of observers, methods for adding/removing observers, and notification mechanics. This pattern is fundamental for event-driven programming and reactive systems. Consider memory management for observer references, implement proper error handling in notification chains, and ensure proper cleanup of observer relationships.
The Factory pattern provides an interface for creating objects without specifying their exact classes. It's particularly useful when object creation involves complex logic or configuration. The pattern encapsulates instantiation logic, enables runtime object creation decisions, and promotes loose coupling. Consider inheritance hierarchies, implement proper error handling for invalid configurations, and document factory interface requirements.
The Module pattern uses closures and immediately invoked function expressions (IIFE) to create private scope and expose public API. Modern JavaScript implements this through ES modules with import/export statements. The pattern enables information hiding, state encapsulation, and dependency management. Consider proper exposure of public interface, implement proper initialization, and handle cleanup requirements.
The Strategy pattern enables selecting algorithms at runtime by defining a family of interchangeable algorithms. Implementation involves creating a strategy interface and concrete strategy implementations. This pattern promotes code reuse and maintainability by isolating algorithm implementations. Consider proper strategy selection mechanism, implement error handling for invalid strategies, and document strategy requirements.
The Decorator pattern allows adding new behaviors to objects dynamically by placing them inside wrapper objects. Implementation involves creating a common interface and series of decorator classes. This pattern provides flexible alternative to subclassing for extending functionality. Consider proper composition of decorators, implement proper method delegation, and maintain proper object state.
The Command pattern encapsulates method invocation by treating it as an object. Implementation involves creating command objects with execute methods and optional undo functionality. This pattern enables operation queuing, logging, and undo mechanisms. Consider proper command state management, implement error handling, and handle command lifecycle properly.
The Proxy pattern provides a surrogate object that controls access to another object. Implementation in JavaScript often uses the Proxy object for intercepting operations. This pattern enables access control, lazy initialization, and logging. Consider performance implications, implement proper validation, and handle method delegation appropriately.
The Chain of Responsibility pattern passes requests along a chain of handlers until one processes the request. Implementation involves creating handler classes with next references. This pattern decouples senders and receivers and enables dynamic handler chains. Consider proper chain construction, implement error handling, and handle chain modification properly.
The Mediator pattern provides a central point for object communication, reducing direct connections between objects. Implementation involves creating a mediator object that coordinates interactions. This pattern reduces coupling and simplifies object relationships. Consider proper event handling, implement error management, and maintain proper object references.
The Composite pattern treats individual objects and compositions uniformly through a common interface. Implementation involves creating component interface and composite/leaf classes. This pattern simplifies client code when working with tree structures. Consider proper child management, implement traversal methods, and handle proper cleanup.
The State pattern allows objects to alter behavior when internal state changes by encapsulating state-specific behavior in separate classes. Implementation involves creating state classes and context class. This pattern localizes state-specific behavior and makes state transitions explicit. Consider proper state transition management, implement proper cleanup, and handle invalid states.
The Template Method pattern defines algorithm skeleton in base class while letting subclasses override specific steps. Implementation involves creating abstract base class with template method. This pattern enables code reuse and ensures consistent algorithm structure. Consider proper abstraction level, implement hooks for customization, and document override requirements.
The Adapter pattern converts interface of one class into interface expected by clients. Implementation involves creating adapter class that implements target interface. This pattern enables integration of incompatible components. Consider proper interface mapping, implement error handling, and maintain proper object lifecycle.
The Iterator pattern provides way to access elements of collection sequentially without exposing underlying representation. Implementation involves creating iterator interface with next/hasNext methods. This pattern simplifies collection traversal and enables different iteration strategies. Consider proper iteration state management, implement proper cleanup, and handle concurrent modification.
The Builder pattern constructs complex objects step by step through fluent interface. Implementation involves creating builder class with configuration methods. This pattern separates construction from representation and enables fine-grained object creation control. Consider proper validation, implement error handling, and maintain proper object state.
The Facade pattern provides unified interface to complex subsystem. Implementation involves creating facade class that wraps subsystem functionality. This pattern reduces coupling between clients and subsystem components. Consider proper abstraction level, implement error handling, and maintain proper documentation.
The Flyweight pattern shares common state between multiple objects to reduce memory usage. Implementation involves separating intrinsic and extrinsic state. This pattern is useful when dealing with large numbers of similar objects. Consider proper state separation, implement proper caching, and handle object creation efficiently.
The Bridge pattern separates abstraction from implementation allowing them to vary independently. Implementation involves creating abstraction and implementor hierarchies. This pattern is useful when dealing with platform-specific implementations. Consider proper interface design, implement proper delegation, and maintain proper documentation.
The Visitor pattern lets you add operations to objects without modifying them by placing operation logic in separate visitor objects. Implementation involves creating visitor interface and concrete visitors. This pattern enables separation of concerns and open-closed principle. Consider proper double dispatch, implement error handling, and maintain proper documentation.
The Memento pattern captures and externalizes object's internal state for later restoration. Implementation involves creating memento class and caretaker for managing mementos. This pattern enables undo/redo functionality and state preservation. Consider proper state encapsulation, implement proper cleanup, and handle memory management.
The Abstract Factory pattern provides interface for creating families of related objects without specifying concrete classes. Implementation involves creating abstract factory interface and concrete factories. This pattern ensures object compatibility within product families. Consider proper factory selection, implement error handling, and maintain proper documentation.
The Prototype pattern creates new objects by cloning existing ones rather than through instantiation. Implementation involves defining clone method in prototype objects. This pattern enables flexible object creation without coupling to specific classes. Consider deep vs shallow cloning, implement proper state copying, and handle circular references.
The Object Pool pattern manages set of reusable objects rather than creating and destroying them. Implementation involves creating pool manager for object lifecycle. This pattern reduces overhead of frequent object creation. Consider pool size management, implement proper cleanup, and handle concurrent access.
The Interpreter pattern defines grammar representation and interpreter for language. Implementation involves creating abstract syntax tree and interpreter methods. This pattern is useful for parsing and evaluating expressions. Consider proper grammar definition, implement error handling, and maintain proper documentation.
The Null Object pattern provides default object behavior instead of null references. Implementation involves creating null object class implementing same interface. This pattern simplifies client code by eliminating null checks. Consider proper interface compliance, implement meaningful default behavior, and document null object usage.
The Lazy Loading pattern defers initialization of object until first use. Implementation involves creating proxy or wrapper for delayed initialization. This pattern improves application startup time and resource usage. Consider proper initialization timing, implement error handling, and handle cleanup properly.
The Dependency Injection pattern provides dependencies to objects instead of having them create or find dependencies. Implementation involves creating injector/container for managing dependencies. This pattern improves modularity and testability. Consider proper dependency resolution, implement lifecycle management, and handle circular dependencies.
The Repository pattern mediates between domain and data mapping layers using collection-like interface. Implementation involves creating repository interface and concrete implementations. This pattern centralizes data access logic and enables substitution of data sources. Consider proper abstraction level, implement error handling, and maintain proper documentation.
The Unit of Work pattern maintains list of business objects affected by transaction and coordinates changes and resolution of problems. Implementation involves creating unit of work class managing object states. This pattern ensures data consistency and transaction management. Consider proper state tracking, implement rollback mechanism, and handle concurrent modifications.
Be fluent in JavaScript basics like closures, this, and promises.
Solve JavaScript problems to build confidence.
Share projects showcasing your JavaScript expertise.
Be ready to explain how you solved challenges in previous projects.
Join thousands of successful candidates preparing with Stark.ai. Start practicing JavaScript questions, mock interviews, and more to secure your dream role.
Start Preparing now