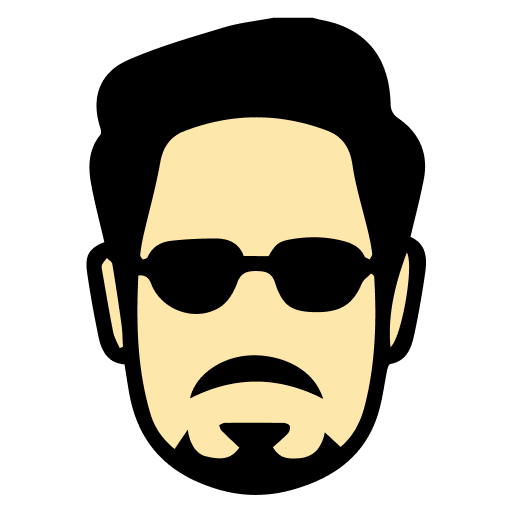
Svelte's unique compile-time approach and reactive programming model make it a powerful framework for modern web development. Stark.ai offers a comprehensive collection of Svelte interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Svelte is a compiler that creates reactive JavaScript modules. Unlike React or Vue that do most of their work in the...
A Svelte component is created in a .svelte file with three main sections: <script> for JavaScript logic, <style> for...
Reactive variables in Svelte are declared using the let keyword in the <script> section. Any variables used in the...
The $ prefix in Svelte is a special syntax that marks a statement as reactive. It automatically re-runs the code...
Svelte uses #if, :else if, and :else blocks for conditional rendering. Example: {#if condition}...{:else if...
Svelte uses the #each block for iteration. Syntax: {#each items as item, index}...{/each}. Supports destructuring,...
The export keyword in Svelte is used to declare props that a component can receive. Example: export let name; Makes...
Events in Svelte are handled using the on: directive. Example: <button on:click={handleClick}>. Supports modifiers...
Component composition in Svelte involves building larger components from smaller ones. Components can be imported...
Styles are added in the <style> block and are automatically scoped to the component. Global styles can be added...
Two-way binding is implemented using the bind: directive. Common with form elements. Example: <input...
Component initialization is handled in the <script> section. Can use onMount lifecycle function for side effects....
Derived stores are created using derived() function, combining one or more stores into a new one. Values update...
Dynamic components can be loaded using svelte:component directive. Example: <svelte:component...
Actions are reusable functions that run when an element is created. Used with use: directive. Can return destroy...
Component composition patterns include slots, context API, event forwarding. Support nested components, higher-order...
Reactive statements ($:) automatically re-run when dependencies change. Used for derived calculations and side...
Component interfaces use TypeScript with props and events. Define prop types using export let prop: Type. Support...
Lifecycle methods include onMount, onDestroy, beforeUpdate, afterUpdate. Handle component initialization, cleanup,...
State persistence uses local storage, session storage, or external stores. Implement auto-save functionality. Handle...
Advanced patterns include compound components, render props, higher-order components. Handle complex state sharing....
Optimize rendering using keyed each blocks, memoization, lazy loading. Implement virtual scrolling. Handle large...
Testing strategies include unit tests, integration tests, component tests. Use testing library/svelte. Implement...
State machines handle complex component states. Implement finite state automata. Handle state transitions. Support...
Code splitting uses dynamic imports, route-based splitting. Implement lazy loading strategies. Handle loading...
Advanced reactivity includes custom stores, derived state, reactive declarations. Handle complex dependencies....
Error boundaries catch and handle component errors. Implement error recovery. Support fallback UI. Handle error...
Accessibility implementation includes ARIA attributes, keyboard navigation, screen reader support. Handle focus...
Internationalization handles multiple languages, RTL support, number/date formatting. Implement translation loading....
Performance monitoring tracks render times, memory usage, bundle size. Implement performance metrics. Handle...
Reactivity in Svelte is the automatic updating of the DOM when data changes. It's handled through assignments to...
Writable stores are created using writable() from svelte/store. Example: const count = writable(0). Provides methods...
Reactive declarations use the $: syntax to automatically recompute values when dependencies change. Example: $:...
Arrays must be updated using assignment for reactivity. Use methods like [...array, newItem] for additions,...
Writable stores can be modified using set() and update(), while readable stores are read-only. Readable stores are...
Stores can be subscribed to using subscribe() method or automatically in templates using $ prefix. Example: $store...
Auto-subscription happens when using $ prefix with stores in components. Svelte automatically handles subscribe and...
Derived values can be created using reactive declarations ($:) or derived stores. They automatically update when...
The set function directly sets a new value for a writable store. Example: count.set(10). Triggers store updates and...
Objects must be updated through assignment for reactivity. Use spread operator or Object.assign for updates....
Derived stores are created using derived() from svelte/store. Take one or more stores as input. Transform values...
Async derived stores handle asynchronous transformations. Use derived with set callback. Handle loading states....
Custom stores implement subscribe function. Can add custom methods. Handle internal state. Support custom update...
Store initialization can be synchronous or async. Handle initial values. Support lazy initialization. Manage loading...
Store persistence uses localStorage or sessionStorage. Implement auto-save functionality. Handle serialization....
Store subscriptions cleanup happens automatically with $ prefix. Manual cleanup needs unsubscribe call. Handle...
Store error handling includes error states. Implement error recovery. Support error notifications. Handle async...
Store middleware intercepts store operations. Implement custom behaviors. Handle side effects. Support middleware...
Store optimization includes batching updates. Implement update debouncing. Handle performance bottlenecks. Support...
Store time-travel tracks state history. Implement undo/redo. Handle state snapshots. Support history compression....
Store synchronization handles multiple instances. Implement cross-tab sync. Support real-time updates. Handle...
Store migrations handle version changes. Implement data transforms. Support backward compatibility. Handle migration...
Store encryption secures sensitive data. Implement encryption/decryption. Handle key management. Support secure...
Store validation ensures data integrity. Implement validation rules. Handle validation errors. Support schema...
Store compression reduces data size. Implement compression algorithms. Handle serialization. Support decompression....
Store monitoring tracks store usage. Implement metrics collection. Handle performance tracking. Support debugging...
Store testing verifies store behavior. Implement test utilities. Handle async testing. Support mocking. Manage test state.
Store documentation describes store usage. Implement documentation generation. Handle API documentation. Support...
Props are declared using the export keyword in the script section. Example: export let propName. Optional props can...
Props are passed to child components as attributes in the markup. Example: <ChildComponent propName={value} />. Can...
Spread props allow passing multiple props using spread syntax. Example: <Component {...props} />. Useful when...
Prop validation can be handled through TypeScript types or runtime checks. Use if statements or assertions in...
Components dispatch custom events using createEventDispatcher. Parent listens using on:eventName. Example:...
Event forwarding passes events up through components using on:eventName. Component can forward DOM events or custom...
Two-way binding on props uses bind:propName. Example: <Input bind:value={name} />. Component must export the prop....
Reactive statements ($:) respond to prop changes. Can trigger side effects or derive values. Example: $:...
HTML attributes can be forwarded using $$props or $$restProps. Useful for wrapper components. Example: <div...
Props can be destructured in export statement. Example: export let { name, age } = data. Supports default values and...
Prop types can be checked using TypeScript or runtime validation. Define interfaces for props. Implement validation...
Async props can be handled using promises or async/await. Handle loading states. Use reactive statements for async...
Computed props derive values from other props using reactive declarations. Example: $: fullName = `${firstName}...
Prop watchers use reactive statements to observe changes. Can trigger side effects or validations. Handle prop...
Prop defaults are set in export statement or computed. Consider component initialization. Handle undefined values....
Event modifiers customize event behavior. Include preventDefault, stopPropagation, once, capture. Used with on:...
Prop transformation converts prop values before use. Use computed properties or methods. Handle data formatting....
Dynamic event handlers change based on props or state. Use computed values for handlers. Support conditional events....
Prop persistence saves prop values between renders. Use stores or local storage. Handle persistence lifecycle....
Compound components share state through context. Implement parent-child communication. Handle component composition....
Advanced validation uses custom validators or schemas. Handle complex validation rules. Support async validation....
Prop inheritance passes props through component hierarchy. Handle prop overrides. Support default inheritance....
Prop versioning handles breaking changes. Implement version migration. Support backwards compatibility. Handle...
Prop serialization handles complex data types. Implement custom serializers. Support bi-directional conversion....
Prop documentation uses JSDoc or TypeScript. Generate documentation automatically. Support example usage. Handle...
Prop monitoring tracks prop usage and changes. Implement monitoring tools. Handle performance tracking. Support...
Prop optimization improves performance. Handle prop memoization. Implement update batching. Support selective...
Prop security prevents injection attacks. Implement sanitization. Handle sensitive data. Support encryption. Manage...
Prop migrations handle schema changes. Implement migration strategies. Support data transformation. Handle migration...
Lifecycle methods in Svelte are functions that execute at different stages of a component's existence. Main...
onMount is a lifecycle function that runs after the component is first rendered to the DOM. It's commonly used for...
onDestroy is called when a component is unmounted from the DOM. Used for cleanup like unsubscribing from stores,...
beforeUpdate runs before the DOM is updated with new values. Useful for capturing pre-update state, like scroll...
afterUpdate runs after the DOM is updated with new values. Used for operations that require updated DOM state, like...
Async operations in onMount use async/await or promises. Handle loading states and errors appropriately. Example:...
Lifecycle methods execute in order: 1. Component creation, 2. beforeUpdate, 3. onMount, 4. afterUpdate. onDestroy...
Resource cleanup is done in onDestroy or by returning cleanup function from onMount. Clear intervals, timeouts,...
Svelte guarantees that onMount runs after DOM is ready, onDestroy runs before unmount, beforeUpdate before DOM...
During SSR, onMount and afterUpdate don't run. Other lifecycle methods run normally. Client-side hydration triggers...
Error handling uses try/catch blocks or error boundaries. Handle async errors appropriately. Implement error...
State initialization happens in component creation or onMount. Handle async initialization. Support loading states....
Store subscriptions are typically set up in onMount and cleaned in onDestroy. Handle store updates. Support...
DOM measurements use afterUpdate for accurate values. Cache measurements when needed. Handle resize events. Support...
Initialization patterns include lazy loading, conditional initialization, and dependency injection. Handle...
Prop changes trigger beforeUpdate and afterUpdate. Implement prop watchers. Handle derived values. Support prop...
Side effects management includes cleanup, ordering, and dependencies. Handle async side effects. Support...
Animations interact with beforeUpdate and afterUpdate. Handle transition states. Support animation cleanup....
Lifecycle hooks extend default lifecycle behavior. Implement custom hooks. Support hook composition. Handle hook...
Component transitions use lifecycle methods for coordination. Handle mount/unmount animations. Support transition...
Advanced initialization includes dependency graphs, async loading, and state machines. Handle complex dependencies....
Lifecycle monitoring tracks method execution and performance. Implement monitoring tools. Handle performance...
Lifecycle testing verifies method execution and timing. Implement test utilities. Handle async testing. Support...
Lifecycle optimization improves performance and efficiency. Handle method batching. Implement update optimization....
Lifecycle documentation describes method behavior and usage. Generate documentation automatically. Support example...
Complex cleanup handles nested resources and dependencies. Implement cleanup ordering. Support partial cleanup....
Error boundaries catch and handle lifecycle errors. Implement recovery strategies. Support fallback content. Handle...
State machines manage complex lifecycle states. Handle state transitions. Support parallel states. Implement state...
Lifecycle debugging tracks method execution and state. Implement debugging tools. Support breakpoints. Handle...
Lifecycle security prevents unauthorized access and manipulation. Handle sensitive operations. Support access...
DOM events in Svelte are handled using the on: directive. Example: <button on:click={handleClick}>. Supports all...
Event modifiers customize event behavior using | symbol. Common modifiers include preventDefault, stopPropagation,...
Custom events are created using createEventDispatcher. Import from svelte, initialize in component, then dispatch...
Component events are listened to using on:eventName. Parent components can listen to dispatched events. Example:...
Event forwarding passes events up through components using on:eventName. No handler needed for forwarding. Example:...
Inline handlers can be defined directly in the on: directive. Example: <button on:click={() => count += 1}>. Good...
Event delegation handles events at a higher level using bubbling. Attach single handler to parent for multiple...
Data is passed as second argument to dispatch. Example: dispatch('custom', { detail: value }). Accessed in handler...
Event object contains event details passed to handlers. Includes properties like target, currentTarget,...
Multiple events use multiple on: directives. Example: <div on:click={handleClick} on:mouseover={handleHover}>. Each...
Event debouncing delays handler execution. Use timer to postpone execution. Clear previous timer on new events....
Event throttling limits execution frequency. Implement using timer and last execution time. Ensure minimum time...
Keyboard events use on:keydown, on:keyup, on:keypress. Access key information through event.key. Support key...
Drag and drop uses dragstart, dragover, drop events. Set draggable attribute. Handle data transfer. Support drag...
Form events include submit, reset, change. Prevent default submission. Handle validation. Collect form data. Support...
Custom modifiers use actions (use:). Implement modifier logic. Support parameters. Handle cleanup. Share across components.
Outside clicks check event.target relationship. Implement using actions. Handle document clicks. Support multiple...
Touch events include touchstart, touchmove, touchend. Handle gestures. Support multi-touch. Implement touch...
Scroll events track scroll position. Implement scroll handlers. Support infinite scroll. Handle scroll optimization....
Window events use svelte:window component. Listen to resize, scroll, online/offline. Handle cleanup. Support event...
Complex patterns combine multiple events. Handle event sequences. Implement state machines. Support event...
Event middleware intercepts and transforms events. Implement custom logic. Support middleware chain. Handle async...
Event logging tracks event occurrences. Implement logging system. Handle event details. Support filtering. Manage...
Event replay records and replays events. Store event sequence. Handle timing. Support playback control. Manage replay state.
Event tracking monitors user interactions. Implement analytics. Handle custom events. Support tracking parameters....
Cross-component events use stores or context. Handle event routing. Support event broadcasting. Implement event...
Event testing verifies event handling. Implement test utilities. Mock events. Support integration testing. Handle...
Event documentation describes event behavior. Generate documentation automatically. Support example usage. Handle...
Event error handling catches and processes errors. Implement error boundaries. Support error recovery. Handle error...
Event optimization improves handling efficiency. Implement event pooling. Handle event batching. Support event...
Stores in Svelte are reactive data containers that can be shared across components. They provide a way to manage...
Writable stores are created using writable() from svelte/store. Example: const count = writable(0). They provide...
The store contract requires an object with subscribe method that takes a callback and returns unsubscribe function....
Store changes can be subscribed to using subscribe method or $ prefix in components. Example: count.subscribe(value...
Readable stores are created using readable() and are read-only. They can only be updated through their start...
Derived stores are created using derived() and compute values based on other stores. Example: const doubled =...
Auto-subscription happens when using $ prefix with stores in components. Svelte automatically handles subscribe and...
Store values can be updated using set() or update() methods. Example: count.set(10) or count.update(n => n + 1)....
Stores are initialized with initial value in creation. Example: writable(initialValue). Can be undefined. Support...
Stores integrate with Svelte's reactivity system. Changes trigger reactive updates. Work with reactive declarations...
Custom stores implement subscribe method. Can add custom methods. Handle internal state. Example: function...
Async stores handle asynchronous data. Support loading states. Handle errors. Example: const data = writable(null);...
Store persistence saves state to storage. Implement auto-save. Handle state rehydration. Example: Subscribe to...
Store dependencies use derived stores or reactive statements. Handle update order. Manage circular dependencies....
Store middleware intercepts store operations. Implement custom behaviors. Handle side effects. Example: Create...
Store error handling catches and processes errors. Implement error states. Support error recovery. Example:...
Store validation ensures valid state. Implement validation rules. Handle validation errors. Example: Create wrapper...
Store optimization includes batching updates, debouncing, throttling. Handle performance bottlenecks. Example:...
Store composition combines multiple stores. Create higher-order stores. Handle store relationships. Example: Combine...
Store lifecycle management handles initialization, updates, cleanup. Support store creation/destruction. Example:...
Advanced patterns include state machines, event sourcing, command pattern. Handle complex state management. Example:...
Store synchronization handles multiple instances. Implement cross-tab sync. Handle conflicts. Example: Use broadcast...
Store time travel tracks state history. Implement undo/redo. Handle state snapshots. Example: Maintain history of...
Store encryption secures sensitive data. Implement encryption/decryption. Handle key management. Example: Encrypt...
Store migrations handle version changes. Implement data transforms. Support backwards compatibility. Example: Define...
Store monitoring tracks store usage. Implement metrics collection. Handle debugging. Example: Create monitoring...
Store testing verifies store behavior. Implement test utilities. Handle async testing. Example: Create test helpers,...
Store documentation describes store usage. Generate documentation automatically. Support examples. Example: Create...
Store security prevents unauthorized access. Implement access control. Handle sensitive data. Example: Create secure...
Store performance optimization includes selective updates, memoization, lazy loading. Handle large datasets....
Transitions in Svelte are built-in animations that play when elements are added to or removed from the DOM. They are...
Fade transition is used with transition:fade directive. Example: <div transition:fade>. Can accept parameters like...
in: and out: directives specify different transitions for entering and leaving. Example: <div in:fade out:fly>....
Transition parameters customize animation behavior. Example: transition:fade={{duration: 300, delay: 100}}. Include...
Fly transition animates position and opacity. Example: <div transition:fly={{y: 200}}>. Parameters include x, y...
Slide transition animates element height. Example: <div transition:slide>. Useful for collapsible content. Can...
Easing functions define animation progression. Import from svelte/easing. Example: transition:fade={{easing:...
transition:local restricts transitions to when parent component is added/removed. Prevents transitions during...
Scale transition animates size and opacity. Example: <div transition:scale>. Parameters include start, opacity,...
Draw transition animates SVG paths. Example: <path transition:draw>. Parameters include duration, delay, easing....
Custom transitions are functions returning animation parameters. Include css and tick functions. Handle enter/exit...
Transition events include introstart, introend, outrostart, outroend. Listen using on: directive. Example: <div...
CSS animations use standard CSS animation properties. Can be combined with transitions. Example: <div animate:flip>....
Flip animation smoothly animates layout changes. Example: <div animate:flip>. Parameters include duration, delay....
Multiple transitions can be combined using different directives. Handle timing coordination. Support transition...
Spring animations use spring() function. Handle physics-based animations. Support stiffness and damping. Example:...
Crossfade creates smooth transitions between elements. Use crossfade() function. Handle element replacement. Support...
Optimize using CSS transforms, will-change property. Handle hardware acceleration. Manage animation frame rate....
Transition groups coordinate multiple transitions. Handle group timing. Support synchronized animations. Manage...
Deferred transitions delay animation start. Handle transition timing. Support conditional transitions. Manage...
Complex sequences combine multiple animations. Handle timing coordination. Support sequential and parallel...
Animation middleware intercepts and modifies animations. Handle animation pipeline. Support middleware chain. Manage...
Animation state machines manage complex animation states. Handle state transitions. Support parallel states. Manage...
Animation presets define reusable animations. Handle preset parameters. Support preset composition. Manage preset library.
Animation testing verifies animation behavior. Handle timing tests. Support visual regression. Manage test assertions.
Animation debugging tracks animation state. Handle debugging tools. Support timeline inspection. Manage debug output.
Animation optimization improves performance. Handle frame rate. Support GPU acceleration. Manage rendering pipeline.
Animation documentation describes animation behavior. Generate documentation automatically. Support example...
Animation monitoring tracks animation performance. Handle metrics collection. Support performance analysis. Manage...
Slots are placeholders in components that allow parent components to pass content. Defined using <slot> element....
Default slots provide fallback content. Example: <slot>Default content</slot>. Content appears when parent doesn't...
Named slots target specific slot locations using name attribute. Example: <slot name='header'>. Content provided...
Content is passed to named slots using slot attribute. Example: <div slot='header'>Header content</div>. Must match...
Slot props pass data from child to parent through slots. Use let:propertyName directive. Example: <slot name='item'...
Use $$slots object to check slot content. Example: {#if $$slots.header}. Available in component script and template....
Fallback content appears when slot is empty. Defined between slot tags. Example: <slot>Fallback</slot>. Provides...
Slots enable flexible component composition. Support content injection at multiple points. Allow component reuse...
Slot content maintains parent component scope. Can access parent variables and functions. Cannot directly access...
Slot content can be styled in both parent and child. Child styles using :slotted() selector. Parent styles apply...
Conditional slots use if blocks around slots. Handle slot presence checks. Support dynamic slot selection. Example:...
Dynamic slot names use computed values. Support runtime slot selection. Handle dynamic content projection. Example:...
Slot validation checks content type and structure. Handle invalid content. Support content restrictions. Implement...
Slot events bubble through component hierarchy. Handle event forwarding. Support event modification. Manage event...
Slot lifecycles manage content updates. Handle content mounting/unmounting. Support cleanup operations. Manage slot state.
Slot composition combines multiple slots. Handle nested slots. Support slot inheritance. Implement composition patterns.
Slot optimization improves rendering efficiency. Handle content caching. Support lazy loading. Manage update frequency.
Error boundaries catch slot content errors. Handle error recovery. Support fallback content. Manage error state.
Slot monitoring tracks content changes. Handle performance metrics. Support debugging tools. Manage monitoring state.
Advanced patterns include render props, compound slots. Handle complex compositions. Support pattern libraries....
Slot testing verifies content projection. Handle integration testing. Support unit tests. Implement test utilities.
Slot documentation describes usage patterns. Generate documentation automatically. Support example usage. Manage...
Slot security prevents content injection. Handle content sanitization. Support content restrictions. Implement...
Slot versioning handles API changes. Implement version migration. Support backwards compatibility. Manage version state.
Slot debugging tracks content flow. Handle debugging tools. Support breakpoints. Manage debug output.
Optimization strategies improve slot performance. Handle content caching. Support virtual slots. Implement update strategies.
State management handles slot-specific state. Implement state containers. Support state sharing. Manage state updates.
Slot accessibility ensures content is accessible. Handle ARIA attributes. Support screen readers. Implement a11y patterns.
Slots are placeholders in components that allow parent components to pass content. Defined using <slot> element....
Default slots provide fallback content. Example: <slot>Default content</slot>. Content appears when parent doesn't...
Content is passed to named slots using slot attribute. Example: <div slot='header'>Header content</div>. Must match...
Slot props pass data from child to parent through slots. Use let:propertyName directive. Example: <slot name='item'...
Use $$slots object to check slot content. Example: {#if $$slots.header}. Available in component script and template....
Fallback content appears when slot is empty. Defined between slot tags. Example: <slot>Fallback</slot>. Provides...
Slots enable flexible component composition. Support content injection at multiple points. Allow component reuse...
Slot content maintains parent component scope. Can access parent variables and functions. Cannot directly access...
Slot content can be styled in both parent and child. Child styles using :slotted() selector. Parent styles apply...
Conditional slots use if blocks around slots. Handle slot presence checks. Support dynamic slot selection. Example:...
Dynamic slot names use computed values. Support runtime slot selection. Handle dynamic content projection. Example:...
Slot validation checks content type and structure. Handle invalid content. Support content restrictions. Implement...
Slot events bubble through component hierarchy. Handle event forwarding. Support event modification. Manage event...
Slot middleware processes slot content. Handle content transformation. Support content filtering. Implement middleware chain.
Slot lifecycles manage content updates. Handle content mounting/unmounting. Support cleanup operations. Manage slot state.
Slot composition combines multiple slots. Handle nested slots. Support slot inheritance. Implement composition patterns.
Slot optimization improves rendering efficiency. Handle content caching. Support lazy loading. Manage update frequency.
Error boundaries catch slot content errors. Handle error recovery. Support fallback content. Manage error state.
Slot monitoring tracks content changes. Handle performance metrics. Support debugging tools. Manage monitoring state.
Advanced patterns include render props, compound slots. Handle complex compositions. Support pattern libraries....
Slot testing verifies content projection. Handle integration testing. Support unit tests. Implement test utilities.
Slot documentation describes usage patterns. Generate documentation automatically. Support example usage. Manage...
Slot security prevents content injection. Handle content sanitization. Support content restrictions. Implement...
Slot versioning handles API changes. Implement version migration. Support backwards compatibility. Manage version state.
Slot debugging tracks content flow. Handle debugging tools. Support breakpoints. Manage debug output.
Optimization strategies improve slot performance. Handle content caching. Support virtual slots. Implement update strategies.
State management handles slot-specific state. Implement state containers. Support state sharing. Manage state updates.
Slot accessibility ensures content is accessible. Handle ARIA attributes. Support screen readers. Implement a11y patterns.
SvelteKit provides file-based routing where files in the routes directory automatically become pages. URLs...
Create a route by adding a +page.svelte file in the routes directory. Example: src/routes/about/+page.svelte becomes...
Dynamic routes use square brackets in file names. Example: [slug]/+page.svelte creates dynamic segment. Parameters...
Route parameters accessed through page.params. Example: export let data; const { slug } = data. Available in...
Layout files (+layout.svelte) provide shared UI for multiple routes. Define common elements like navigation, footer....
Navigation uses <a> tags or programmatic goto function. SvelteKit handles client-side navigation. Supports...
Route groups organize routes using (group) syntax. Don't affect URL structure. Share layouts within groups. Support...
Data loading uses +page.js or +page.server.js files. Export load function for data fetching. Returns props for page...
Error page (+error.svelte) handles route errors. Displays when errors occur. Access error details through error...
Redirects use redirect function from @sveltejs/kit. Can redirect in load functions or actions. Support...
Route guards protect routes using load functions. Check authentication/authorization. Return redirect for...
Nested layouts use multiple +layout.svelte files. Each level adds layout. Support layout inheritance. Handle layout...
Preloading uses data-sveltekit-preload attribute. Fetches data before navigation. Support hover preloading. Handle...
Route transitions use page transitions API. Support enter/leave animations. Handle transition lifecycle. Implement...
Route middleware uses hooks.server.js file. Handle request/response. Support authentication. Implement custom logic....
Route caching implements caching strategies. Handle data caching. Support page caching. Implement cache...
Route validation handles parameter validation. Support request validation. Implement validation rules. Handle...
Route state management uses stores or context. Handle state persistence. Support state sharing. Implement state...
Route optimization includes code splitting, preloading. Handle lazy loading. Support route prioritization. Implement...
Advanced patterns include nested routes, parallel routes. Handle complex navigation. Support route composition....
Route i18n supports multiple languages. Handle URL localization. Support language switching. Implement i18n...
Route testing verifies routing behavior. Handle navigation testing. Support integration testing. Implement test...
Route monitoring tracks navigation patterns. Handle analytics integration. Support performance tracking. Implement...
Route security prevents unauthorized access. Handle authentication flows. Support authorization rules. Implement...
Route documentation describes routing structure. Generate documentation automatically. Support example routes....
Route debugging tracks routing issues. Handle debugging tools. Support state inspection. Implement debug logging....
Route accessibility ensures accessible navigation. Handle focus management. Support screen readers. Implement a11y...
Error handling manages routing errors. Handle error boundaries. Support error recovery. Implement error logging....
Code splitting optimizes route loading. Handle chunk generation. Support dynamic imports. Implement splitting...
The Context API allows passing data through the component tree without prop drilling. Uses setContext and getContext...
Context is set using setContext function from svelte. Example: setContext('key', value). Must be called during...
Context is retrieved using getContext function. Example: const value = getContext('key'). Must use same key as...
Context keys must be unique within component tree. Often use symbols for guaranteed uniqueness. Example: const key =...
Context exists throughout component lifecycle. Created during initialization. Available until component destruction....
Common uses include theme data, localization, authentication state, shared functionality. Useful for cross-cutting...
Functions can be shared through context. Example: setContext('api', { method: () => {} }). Allows child components...
Context is scoped to component and descendants. Not available to parent or sibling components. Multiple instances...
getContext returns undefined if context not found. Should handle undefined case. Can provide default values....
Context is static, set during initialization. Stores are reactive, can change over time. Context good for static...
Context patterns include provider components, dependency injection, service locator. Handle context composition....
Context updates require component reinitialization. Can combine with stores for reactive updates. Handle update...
Context dependencies manage relationships between contexts. Handle dependency order. Support circular dependencies....
Context composition combines multiple contexts. Handle context merging. Support context inheritance. Implement...
Context initialization sets up initial context state. Handle async initialization. Support initialization order....
Context middleware processes context operations. Handle context transformation. Support middleware chain. Implement...
Context error handling manages error states. Handle missing context. Support error recovery. Implement error boundaries.
Context optimization improves performance. Handle context caching. Support selective updates. Implement optimization...
Context cleanup manages resource disposal. Handle cleanup order. Support cleanup hooks. Implement cleanup strategies.
Advanced patterns include context injection, service location, dependency trees. Handle complex dependencies....
Context testing verifies context behavior. Handle test isolation. Support integration testing. Implement test utilities.
Context monitoring tracks context usage. Handle performance tracking. Support debugging tools. Implement monitoring...
Context documentation describes context usage. Generate documentation automatically. Support example usage. Manage...
Context versioning handles API changes. Implement version migration. Support backwards compatibility. Manage version state.
Context security prevents unauthorized access. Handle access control. Support security policies. Implement security measures.
Context debugging tracks context issues. Handle debugging tools. Support state inspection. Implement debug logging.
Performance monitoring tracks context efficiency. Handle metrics collection. Support performance analysis. Implement...
Dependency injection manages component dependencies. Handle injection patterns. Support service location. Implement...
Type safety ensures correct context usage. Handle TypeScript integration. Support type checking. Implement type definitions.
Bindings create two-way data flow between DOM elements and variables using bind: directive. Example: <input...
Basic form bindings include value for text inputs, checked for checkboxes, group for radio/checkbox groups. Example:...
use: directive attaches actions (reusable DOM node functionality) to elements. Example: <div use:action>. Actions...
class: directive conditionally applies CSS classes. Example: <div class:active={isActive}>. Shorthand available when...
Custom component binding uses bind: on exported props. Component must export the variable. Example: <CustomInput...
style: directive sets inline styles conditionally. Example: <div style:color={textColor}>. Can use shorthand when...
Select elements bind using value or selectedIndex. Example: <select bind:value={selected}>. Supports multiple...
this binding references DOM element or component instance. Example: <div bind:this={element}>. Useful for direct DOM...
bind:group groups radio/checkbox inputs. Binds multiple inputs to single value/array. Example: <input type='radio'...
Contenteditable elements bind using textContent or innerHTML. Example: <div contenteditable...
Custom actions are functions returning optional destroy method. Handle DOM node manipulation. Support parameters....
Binding validation ensures valid values. Handle input constraints. Support custom validation. Implement error...
Binding middleware processes binding operations. Handle value transformation. Support validation chain. Implement...
Binding dependencies manage related bindings. Handle dependency updates. Support dependency tracking. Implement...
Binding optimization improves update efficiency. Handle update batching. Support selective updates. Implement...
Custom directives extend element functionality. Handle directive lifecycle. Support directive parameters. Implement...
Binding error handling manages invalid states. Handle error recovery. Support error notifications. Implement error...
Binding composition combines multiple bindings. Handle binding interaction. Support binding inheritance. Implement...
Binding cleanup manages resource disposal. Handle cleanup order. Support cleanup hooks. Implement cleanup strategies.
Advanced patterns include computed bindings, conditional bindings. Handle complex scenarios. Support pattern composition.
Binding testing verifies binding behavior. Handle test isolation. Support integration testing. Implement test utilities.
Binding monitoring tracks binding usage. Handle performance tracking. Support debugging tools. Implement monitoring...
Binding documentation describes binding usage. Generate documentation automatically. Support example usage. Manage...
Binding security prevents unauthorized access. Handle input sanitization. Support security policies. Implement...
Binding debuggers track binding issues. Handle debugging tools. Support state inspection. Implement debug logging.
Type safety ensures correct binding usage. Handle TypeScript integration. Support type checking. Implement type definitions.
Optimization strategies improve binding performance. Handle update batching. Support selective updates. Implement...
State management handles binding state. Handle state updates. Support state sharing. Implement state patterns.
Binding accessibility ensures accessible usage. Handle ARIA attributes. Support screen readers. Implement a11y patterns.
Server-Side Rendering (SSR) generates HTML on the server instead of client. Provides better initial page load, SEO...
Hydration is the process where client-side JavaScript takes over server-rendered HTML. Makes static content...
Server-side data loading uses load functions in +page.server.js. Returns data for page rendering. Supports async...
Client-Side Rendering (CSR) fallback handles cases where SSR fails or is disabled. Uses +page.js instead of...
Disable SSR using export const ssr = false in +page.js. Page renders only on client. Useful for browser-specific...
Server-only modules run exclusively on server. Use .server.js extension. Cannot be imported by client code. Useful...
SSR errors handled by error.svelte pages. Support error boundaries. Can provide fallback content. Error details...
Streaming SSR sends HTML in chunks as it's generated. Improves Time To First Byte (TTFB). Supports progressive...
Environment variables accessed through $env/static/private or $env/dynamic/private. Only available server-side. Must...
Prerendering generates static HTML at build time. Uses export const prerender = true. Improves performance. Suitable...
SSR data fetching uses load functions. Handle async operations. Support caching strategies. Implement error...
SSR caching implements cache strategies. Handle cache invalidation. Support cache headers. Implement cache storage....
SSR optimization includes code splitting, caching, streaming. Handle resource optimization. Support performance...
SSR session state manages user sessions. Handle session storage. Support session persistence. Implement session...
SSR middleware processes server requests. Handle request transformation. Support middleware chain. Implement...
SSR authentication manages user auth state. Handle auth flows. Support session management. Implement auth...
SSR routing handles server-side navigation. Handle route matching. Support dynamic routes. Implement routing...
SSR headers manage HTTP headers. Handle cache control. Support content types. Implement header strategies. Manage...
SSR forms handle form submissions server-side. Handle form validation. Support file uploads. Implement CSRF...
Advanced patterns include streaming, progressive enhancement. Handle complex scenarios. Support pattern composition....
SSR testing verifies server rendering. Handle test isolation. Support integration testing. Implement test utilities....
SSR monitoring tracks server performance. Handle metrics collection. Support debugging tools. Implement monitoring...
SSR security prevents vulnerabilities. Handle input sanitization. Support security headers. Implement security...
SSR error handling manages server errors. Handle error recovery. Support error boundaries. Implement error logging....
Optimization strategies improve SSR performance. Handle resource optimization. Support caching strategies. Implement...
State management handles server state. Handle state serialization. Support state hydration. Implement state patterns.
SSR documentation describes server features. Generate documentation automatically. Support example usage. Manage...
SSR debugging tracks server issues. Handle debugging tools. Support state inspection. Implement debug logging.
SSR internationalization handles multiple languages. Support content translation. Implement i18n patterns. Manage...
SSR accessibility ensures server-rendered content is accessible. Handle ARIA attributes. Support screen readers....
Vitest is the recommended testing framework for Svelte applications. It's fast, provides good integration with...
Component tests use @testing-library/svelte. Mount components using render method. Test component behavior and DOM...
Svelte Inspector is a development tool enabled with 'ctrl + shift + i'. Shows component hierarchy, props, state....
Async tests use async/await syntax. Test async operations using act function. Handle promises and timeouts. Example:...
Testing matchers verify component state/behavior. Include toBeInTheDocument, toHaveTextContent, toBeVisible....
Store state can be debugged using $store syntax. Subscribe to store changes. Log state updates. Use Svelte devtools....
Testing hierarchy includes unit tests, integration tests, end-to-end tests. Focus on component isolation. Test...
Event handlers tested using fireEvent. Simulate user interactions. Verify event outcomes. Example:...
Snapshot testing captures component output. Compares against stored snapshots. Detects UI changes. Example:...
Props testing verifies component behavior with different props. Test prop types and values. Handle prop updates....
Integration testing verifies component interaction. Test multiple components. Handle data flow. Implement test...
Store testing verifies store behavior. Test store updates. Handle subscriptions. Implement store mock. Manage store state.
Test mocks simulate dependencies. Handle external services. Support mock responses. Implement mock behavior. Manage...
Route testing verifies navigation behavior. Test route parameters. Handle route changes. Implement navigation tests....
Test fixtures provide test data. Handle data setup. Support test isolation. Implement fixture management. Manage...
Animation testing verifies transition behavior. Test animation states. Handle animation timing. Implement animation...
Test hooks handle test lifecycle. Support setup/teardown. Implement test utilities. Manage test state. Handle test...
Error handling testing verifies error states. Test error boundaries. Handle error recovery. Implement error...
Test coverage tracks code coverage. Generate coverage reports. Set coverage targets. Implement coverage checks....
Test organization structures test files. Group related tests. Support test discovery. Implement naming conventions....
Advanced patterns include test factories, test builders. Handle complex scenarios. Support pattern composition....
Test automation handles continuous testing. Set up CI/CD pipelines. Support automated runs. Implement test...
Performance testing measures component efficiency. Test rendering speed. Handle resource usage. Implement...
Accessibility testing verifies a11y compliance. Test screen readers. Handle keyboard navigation. Implement ARIA...
Visual regression detects UI changes. Compare screenshots. Handle visual diffs. Implement image comparison. Manage...
Test monitoring tracks test execution. Handle test metrics. Support test analytics. Implement monitoring tools....
Test documentation describes test cases. Generate documentation automatically. Support example tests. Manage...
Security testing verifies application security. Test vulnerability prevention. Handle security scenarios. Implement...
Load testing verifies application performance. Test under heavy load. Handle stress scenarios. Implement load...
Environment management handles test environments. Set up configurations. Support multiple environments. Implement...
Svelte achieves performance through compile-time optimization. Generates vanilla JavaScript with minimal runtime...
Code splitting automatically breaks application into smaller chunks. Routes loaded on demand. Reduces initial bundle...
Svelte compiles reactive statements into efficient JavaScript. Updates only affected DOM elements. No diffing...
Asset optimization includes minification, bundling, compression. Uses Vite for build process. Optimizes images and...
Component optimization includes proper key usage, avoiding unnecessary updates, using tick() function. Implement...
Lazy loading defers loading of components until needed. Uses dynamic imports. Supports route-level code splitting....
Memory leak prevention includes proper cleanup in onDestroy, unsubscribing from stores, removing event listeners....
Prefetching loads route data before navigation. Uses sveltekit:prefetch directive. Improves perceived performance....
Store optimization includes batching updates, using derived stores efficiently, implementing selective updates....
Tree-shaking removes unused code during build. Reduces bundle size. Supported by default. Works with ES modules....
Caching strategies include browser cache, service workers, SSR cache. Handle cache invalidation. Support offline...
Animation optimization includes using CSS animations when possible, hardware acceleration, efficient transitions....
Large list optimization includes virtual scrolling, pagination, infinite scroll. Use keyed each blocks. Implement...
Image optimization includes lazy loading, responsive images, proper formats. Use srcset attribute. Implement...
Performance monitoring tracks metrics like load time, FCP, TTI. Use browser DevTools. Implement analytics. Handle...
SSR optimization includes caching, streaming, efficient data loading. Handle server resources. Implement response...
Resource prioritization includes critical CSS, script loading strategies, preload/prefetch. Handle resource order....
Build optimization includes proper Vite/Rollup config, production settings, environment optimization. Handle build...
Performance testing measures load time, interaction time, resource usage. Implement benchmarks. Handle performance...
Advanced strategies include worker threads, memory pooling, render optimization. Handle complex scenarios. Support...
Bundle analysis examines build output size, dependencies, chunks. Use bundle analyzers. Handle size optimization....
Network optimization includes request batching, protocol optimization, CDN usage. Handle network caching. Implement...
Runtime optimization includes memory management, event delegation, efficient algorithms. Handle runtime performance....
Rendering optimization includes layout thrashing prevention, paint optimization, composite layers. Handle render...
State optimization includes efficient updates, state normalization, update batching. Handle state management....
Resource optimization includes asset management, resource loading, dependency optimization. Handle resource usage....
Security optimization includes CSP, secure headers, vulnerability prevention. Handle security measures. Implement...
Monitoring tools track performance metrics, resource usage, errors. Handle monitoring systems. Implement tracking strategies.
Optimization documentation describes performance strategies. Generate performance reports. Support optimization...
Svelte is a compiler that creates reactive JavaScript modules. Unlike React or Vue that do most of their work in the browser, Svelte shifts that work into a compile step that happens when you build your app, resulting in highly optimized vanilla JavaScript with minimal runtime overhead.
A Svelte component is created in a .svelte file with three main sections: <script> for JavaScript logic, <style> for component-scoped CSS, and the template section for HTML markup. Styles are automatically scoped to the component.
Reactive variables in Svelte are declared using the let keyword in the <script> section. Any variables used in the template are automatically reactive. Example: let count = 0. When count changes, the UI automatically updates.
The $ prefix in Svelte is a special syntax that marks a statement as reactive. It automatically re-runs the code whenever any referenced values change. It's commonly used with derived values and store subscriptions.
Svelte uses #if, :else if, and :else blocks for conditional rendering. Example: {#if condition}...{:else if otherCondition}...{:else}...{/if}. These blocks can contain any valid HTML or component markup.
Svelte uses the #each block for iteration. Syntax: {#each items as item, index}...{/each}. Supports destructuring, key specification, and else blocks for empty arrays. Example: {#each users as {id, name} (id)}.
The export keyword in Svelte is used to declare props that a component can receive. Example: export let name; Makes the variable available as a prop when using the component: <Component name='value' />.
Events in Svelte are handled using the on: directive. Example: <button on:click={handleClick}>. Supports modifiers like preventDefault using |. Example: <form on:submit|preventDefault={handleSubmit}>.
Component composition in Svelte involves building larger components from smaller ones. Components can be imported and used like HTML elements. Example: import Child from './Child.svelte'; then use <Child /> in template.
Styles are added in the <style> block and are automatically scoped to the component. Global styles can be added using :global() modifier. Supports regular CSS with automatic vendor prefixing.
Two-way binding is implemented using the bind: directive. Common with form elements. Example: <input bind:value={name}>. Supports binding to different properties like checked for checkboxes.
Component initialization is handled in the <script> section. Can use onMount lifecycle function for side effects. Top-level code runs on component creation. Support async initialization using IIFE or onMount.
Derived stores are created using derived() function, combining one or more stores into a new one. Values update automatically when source stores change. Support synchronous and asynchronous derivation.
Dynamic components can be loaded using svelte:component directive. Example: <svelte:component this={dynamicComponent} />. Support lazy loading using import(). Handle loading states.
Actions are reusable functions that run when an element is created. Used with use: directive. Can return destroy function. Example: <div use:tooltip={params}>. Support custom DOM manipulation.
Component composition patterns include slots, context API, event forwarding. Support nested components, higher-order components. Handle component communication through props and events.
Reactive statements ($:) automatically re-run when dependencies change. Used for derived calculations and side effects. Support multiple dependencies. Handle complex reactive computations.
Component interfaces use TypeScript with props and events. Define prop types using export let prop: Type. Support interface inheritance. Handle optional props and default values.
Lifecycle methods include onMount, onDestroy, beforeUpdate, afterUpdate. Handle component initialization, cleanup, updates. Support async operations. Manage side effects.
State persistence uses local storage, session storage, or external stores. Implement auto-save functionality. Handle state rehydration. Support offline state management.
Advanced patterns include compound components, render props, higher-order components. Handle complex state sharing. Support flexible component composition. Implement reusable logic.
Optimize rendering using keyed each blocks, memoization, lazy loading. Implement virtual scrolling. Handle large lists. Optimize reactive updates. Monitor performance metrics.
Testing strategies include unit tests, integration tests, component tests. Use testing library/svelte. Implement test utilities. Handle async testing. Support snapshot testing.
State machines handle complex component states. Implement finite state automata. Handle state transitions. Support parallel states. Manage side effects in state changes.
Code splitting uses dynamic imports, route-based splitting. Implement lazy loading strategies. Handle loading states. Optimize bundle size. Support prefetching.
Advanced reactivity includes custom stores, derived state, reactive declarations. Handle complex dependencies. Implement reactive cleanup. Support async reactivity.
Error boundaries catch and handle component errors. Implement error recovery. Support fallback UI. Handle error reporting. Manage error state.
Accessibility implementation includes ARIA attributes, keyboard navigation, screen reader support. Handle focus management. Implement semantic HTML. Support a11y testing.
Internationalization handles multiple languages, RTL support, number/date formatting. Implement translation loading. Support locale switching. Handle dynamic content.
Performance monitoring tracks render times, memory usage, bundle size. Implement performance metrics. Handle performance optimization. Support monitoring tools.
Reactivity in Svelte is the automatic updating of the DOM when data changes. It's handled through assignments to declared variables and the $: syntax. Svelte's compiler creates the necessary code to update the DOM when dependencies change.
Writable stores are created using writable() from svelte/store. Example: const count = writable(0). Provides methods like set() and update() to modify the store value. Subscribe to changes using subscribe() method.
Reactive declarations use the $: syntax to automatically recompute values when dependencies change. Example: $: doubled = count * 2. They run whenever any referenced values change.
Arrays must be updated using assignment for reactivity. Use methods like [...array, newItem] for additions, array.filter() for removals, or array.map() for updates. Assignment triggers reactivity.
Writable stores can be modified using set() and update(), while readable stores are read-only. Readable stores are created using readable() and only change through their start function.
Stores can be subscribed to using subscribe() method or automatically in templates using $ prefix. Example: $store in template or store.subscribe(value => {}) in script.
Auto-subscription happens when using $ prefix with stores in components. Svelte automatically handles subscribe and unsubscribe. No manual cleanup needed. Works in template and reactive statements.
Derived values can be created using reactive declarations ($:) or derived stores. They automatically update when dependencies change. Used for computed values that depend on other state.
The set function directly sets a new value for a writable store. Example: count.set(10). Triggers store updates and notifies all subscribers. Used for direct value updates.
Objects must be updated through assignment for reactivity. Use spread operator or Object.assign for updates. Example: obj = {...obj, newProp: value}. Assignment triggers reactivity.
Derived stores are created using derived() from svelte/store. Take one or more stores as input. Transform values using callback function. Update automatically when source stores change.
Async derived stores handle asynchronous transformations. Use derived with set callback. Handle loading states. Support cancellation. Manage async dependencies.
Custom stores implement subscribe function. Can add custom methods. Handle internal state. Support custom update logic. Implement cleanup on unsubscribe.
Store initialization can be synchronous or async. Handle initial values. Support lazy initialization. Manage loading states. Handle initialization errors.
Store persistence uses localStorage or sessionStorage. Implement auto-save functionality. Handle serialization. Support state rehydration. Manage persistence errors.
Store subscriptions cleanup happens automatically with $ prefix. Manual cleanup needs unsubscribe call. Handle cleanup in onDestroy. Prevent memory leaks.
Store error handling includes error states. Implement error recovery. Support error notifications. Handle async errors. Manage error boundaries.
Store middleware intercepts store operations. Implement custom behaviors. Handle side effects. Support middleware chain. Manage middleware order.
Store optimization includes batching updates. Implement update debouncing. Handle performance bottlenecks. Support selective updates. Monitor update frequency.
Store time-travel tracks state history. Implement undo/redo. Handle state snapshots. Support history compression. Manage memory usage.
Store synchronization handles multiple instances. Implement cross-tab sync. Support real-time updates. Handle conflicts. Manage sync state.
Store migrations handle version changes. Implement data transforms. Support backward compatibility. Handle migration errors. Manage migration state.
Store encryption secures sensitive data. Implement encryption/decryption. Handle key management. Support secure storage. Manage encrypted state.
Store validation ensures data integrity. Implement validation rules. Handle validation errors. Support schema validation. Manage validation state.
Store compression reduces data size. Implement compression algorithms. Handle serialization. Support decompression. Manage compressed state.
Store monitoring tracks store usage. Implement metrics collection. Handle performance tracking. Support debugging tools. Manage monitoring state.
Store testing verifies store behavior. Implement test utilities. Handle async testing. Support mocking. Manage test state.
Store documentation describes store usage. Implement documentation generation. Handle API documentation. Support examples. Manage documentation state.
Props are declared using the export keyword in the script section. Example: export let propName. Optional props can have default values: export let propName = defaultValue. Props are passed to components as attributes.
Props are passed to child components as attributes in the markup. Example: <ChildComponent propName={value} />. Can use shorthand when prop name matches variable: <ChildComponent {value} />.
Spread props allow passing multiple props using spread syntax. Example: <Component {...props} />. Useful when forwarding many props. All properties of the object become individual props.
Prop validation can be handled through TypeScript types or runtime checks. Use if statements or assertions in component initialization. Can throw errors for invalid props.
Components dispatch custom events using createEventDispatcher. Parent listens using on:eventName. Example: dispatch('message', data). Events bubble by default.
Event forwarding passes events up through components using on:eventName. Component can forward DOM events or custom events. Use on:message|stopPropagation to prevent bubbling.
Two-way binding on props uses bind:propName. Example: <Input bind:value={name} />. Component must export the prop. Updates flow both ways between parent and child.
Reactive statements ($:) respond to prop changes. Can trigger side effects or derive values. Example: $: console.log(propName). Runs whenever referenced props update.
HTML attributes can be forwarded using $$props or $$restProps. Useful for wrapper components. Example: <div {...$$restProps}>. Passes any unhandled props to element.
Props can be destructured in export statement. Example: export let { name, age } = data. Supports default values and renaming. Makes prop handling more concise.
Prop types can be checked using TypeScript or runtime validation. Define interfaces for props. Implement validation in onMount or initialization. Handle type errors appropriately.
Async props can be handled using promises or async/await. Handle loading states. Use reactive statements for async updates. Manage error states for async props.
Computed props derive values from other props using reactive declarations. Example: $: fullName = `${firstName} ${lastName}`. Update automatically when dependencies change.
Prop watchers use reactive statements to observe changes. Can trigger side effects or validations. Handle prop updates asynchronously. Support complex watch conditions.
Prop defaults are set in export statement or computed. Consider component initialization. Handle undefined values. Support dynamic defaults. Validate default values.
Event modifiers customize event behavior. Include preventDefault, stopPropagation, once, capture. Used with on: directive. Example: on:click|preventDefault. Support multiple modifiers.
Prop transformation converts prop values before use. Use computed properties or methods. Handle data formatting. Support bi-directional transformation. Validate transformed values.
Dynamic event handlers change based on props or state. Use computed values for handlers. Support conditional events. Handle dynamic binding. Manage handler lifecycle.
Prop persistence saves prop values between renders. Use stores or local storage. Handle persistence lifecycle. Support value restoration. Manage persistence errors.
Compound components share state through context. Implement parent-child communication. Handle component composition. Support flexible APIs. Manage shared state.
Advanced validation uses custom validators or schemas. Handle complex validation rules. Support async validation. Implement validation pipelines. Manage validation state.
Prop inheritance passes props through component hierarchy. Handle prop overrides. Support default inheritance. Implement inheritance rules. Manage inheritance chain.
Prop versioning handles breaking changes. Implement version migration. Support backwards compatibility. Handle version conflicts. Manage version state.
Prop serialization handles complex data types. Implement custom serializers. Support bi-directional conversion. Handle serialization errors. Manage serialized state.
Prop documentation uses JSDoc or TypeScript. Generate documentation automatically. Support example usage. Handle deprecated props. Manage documentation updates.
Prop monitoring tracks prop usage and changes. Implement monitoring tools. Handle performance tracking. Support debugging. Manage monitoring state.
Prop optimization improves performance. Handle prop memoization. Implement update batching. Support selective updates. Manage update frequency.
Prop security prevents injection attacks. Implement sanitization. Handle sensitive data. Support encryption. Manage security policies.
Prop migrations handle schema changes. Implement migration strategies. Support data transformation. Handle migration errors. Manage migration state.
Lifecycle methods in Svelte are functions that execute at different stages of a component's existence. Main lifecycle methods include onMount, onDestroy, beforeUpdate, and afterUpdate. They help manage side effects and component behavior.
onMount is a lifecycle function that runs after the component is first rendered to the DOM. It's commonly used for initialization, data fetching, and setting up subscriptions. Returns a cleanup function optionally.
onDestroy is called when a component is unmounted from the DOM. Used for cleanup like unsubscribing from stores, clearing intervals, or removing event listeners. Prevents memory leaks.
beforeUpdate runs before the DOM is updated with new values. Useful for capturing pre-update state, like scroll position. Can be used multiple times in a component.
afterUpdate runs after the DOM is updated with new values. Used for operations that require updated DOM state, like updating scroll position or third-party libraries.
Async operations in onMount use async/await or promises. Handle loading states and errors appropriately. Example: onMount(async () => { const data = await fetchData(); })
Lifecycle methods execute in order: 1. Component creation, 2. beforeUpdate, 3. onMount, 4. afterUpdate. onDestroy runs when component is unmounted. Updates trigger beforeUpdate and afterUpdate.
Resource cleanup is done in onDestroy or by returning cleanup function from onMount. Clear intervals, timeouts, event listeners, and subscriptions to prevent memory leaks.
Svelte guarantees that onMount runs after DOM is ready, onDestroy runs before unmount, beforeUpdate before DOM updates, and afterUpdate after DOM updates. Component initialization always runs.
During SSR, onMount and afterUpdate don't run. Other lifecycle methods run normally. Client-side hydration triggers lifecycle methods appropriately. Handle SSR-specific logic.
Error handling uses try/catch blocks or error boundaries. Handle async errors appropriately. Implement error recovery strategies. Support error reporting.
State initialization happens in component creation or onMount. Handle async initialization. Support loading states. Implement initialization strategies.
Store subscriptions are typically set up in onMount and cleaned in onDestroy. Handle store updates. Support auto-subscription. Manage subscription lifecycle.
DOM measurements use afterUpdate for accurate values. Cache measurements when needed. Handle resize events. Support responsive measurements.
Initialization patterns include lazy loading, conditional initialization, and dependency injection. Handle initialization order. Support async initialization.
Prop changes trigger beforeUpdate and afterUpdate. Implement prop watchers. Handle derived values. Support prop validation in lifecycle.
Side effects management includes cleanup, ordering, and dependencies. Handle async side effects. Support cancellation. Implement side effect tracking.
Animations interact with beforeUpdate and afterUpdate. Handle transition states. Support animation cleanup. Implement animation queuing.
Lifecycle hooks extend default lifecycle behavior. Implement custom hooks. Support hook composition. Handle hook dependencies.
Component transitions use lifecycle methods for coordination. Handle mount/unmount animations. Support transition states. Implement transition hooks.
Advanced initialization includes dependency graphs, async loading, and state machines. Handle complex dependencies. Support initialization rollback.
Lifecycle monitoring tracks method execution and performance. Implement monitoring tools. Handle performance tracking. Support debugging.
Lifecycle testing verifies method execution and timing. Implement test utilities. Handle async testing. Support integration testing.
Lifecycle optimization improves performance and efficiency. Handle method batching. Implement update optimization. Support selective updates.
Lifecycle documentation describes method behavior and usage. Generate documentation automatically. Support example usage. Handle versioning.
Complex cleanup handles nested resources and dependencies. Implement cleanup ordering. Support partial cleanup. Handle cleanup failures.
Error boundaries catch and handle lifecycle errors. Implement recovery strategies. Support fallback content. Handle error reporting.
State machines manage complex lifecycle states. Handle state transitions. Support parallel states. Implement state persistence.
Lifecycle debugging tracks method execution and state. Implement debugging tools. Support breakpoints. Handle debugging output.
Lifecycle security prevents unauthorized access and manipulation. Handle sensitive operations. Support access control. Implement security policies.
DOM events in Svelte are handled using the on: directive. Example: <button on:click={handleClick}>. Supports all standard DOM events like click, submit, input, etc. Event handler receives the event object as parameter.
Event modifiers customize event behavior using | symbol. Common modifiers include preventDefault, stopPropagation, once, capture. Example: <form on:submit|preventDefault={handleSubmit}>.
Custom events are created using createEventDispatcher. Import from svelte, initialize in component, then dispatch events. Example: const dispatch = createEventDispatcher(); dispatch('custom', data);
Component events are listened to using on:eventName. Parent components can listen to dispatched events. Example: <Child on:custom={handleCustom}>. Events bubble by default.
Event forwarding passes events up through components using on:eventName. No handler needed for forwarding. Example: <button on:click>. Useful for bubbling events through component hierarchy.
Inline handlers can be defined directly in the on: directive. Example: <button on:click={() => count += 1}>. Good for simple operations but avoid complex logic.
Event delegation handles events at a higher level using bubbling. Attach single handler to parent for multiple children. Use event.target to identify source. Improves performance for many elements.
Data is passed as second argument to dispatch. Example: dispatch('custom', { detail: value }). Accessed in handler through event.detail. Supports any serializable data.
Event object contains event details passed to handlers. Includes properties like target, currentTarget, preventDefault(). Available as first parameter in event handlers.
Multiple events use multiple on: directives. Example: <div on:click={handleClick} on:mouseover={handleHover}>. Each event can have its own modifiers and handlers.
Event debouncing delays handler execution. Use timer to postpone execution. Clear previous timer on new events. Example: implement custom action or store for debouncing.
Event throttling limits execution frequency. Implement using timer and last execution time. Ensure minimum time between executions. Support custom throttle intervals.
Keyboard events use on:keydown, on:keyup, on:keypress. Access key information through event.key. Support key combinations. Handle keyboard navigation.
Drag and drop uses dragstart, dragover, drop events. Set draggable attribute. Handle data transfer. Support drag visualization. Implement drop zones.
Form events include submit, reset, change. Prevent default submission. Handle validation. Collect form data. Support dynamic forms.
Custom modifiers use actions (use:). Implement modifier logic. Support parameters. Handle cleanup. Share across components.
Outside clicks check event.target relationship. Implement using actions. Handle document clicks. Support multiple elements. Clean up listeners.
Touch events include touchstart, touchmove, touchend. Handle gestures. Support multi-touch. Implement touch feedback. Handle touch coordinates.
Scroll events track scroll position. Implement scroll handlers. Support infinite scroll. Handle scroll optimization. Manage scroll restoration.
Window events use svelte:window component. Listen to resize, scroll, online/offline. Handle cleanup. Support event parameters.
Complex patterns combine multiple events. Handle event sequences. Implement state machines. Support event composition. Manage event flow.
Event middleware intercepts and transforms events. Implement custom logic. Support middleware chain. Handle async middleware. Manage middleware order.
Event logging tracks event occurrences. Implement logging system. Handle event details. Support filtering. Manage log storage.
Event replay records and replays events. Store event sequence. Handle timing. Support playback control. Manage replay state.
Event tracking monitors user interactions. Implement analytics. Handle custom events. Support tracking parameters. Manage tracking data.
Cross-component events use stores or context. Handle event routing. Support event broadcasting. Implement event hierarchy. Manage event scope.
Event testing verifies event handling. Implement test utilities. Mock events. Support integration testing. Handle async events.
Event documentation describes event behavior. Generate documentation automatically. Support example usage. Handle event versioning.
Event error handling catches and processes errors. Implement error boundaries. Support error recovery. Handle error reporting.
Event optimization improves handling efficiency. Implement event pooling. Handle event batching. Support event prioritization.
Stores in Svelte are reactive data containers that can be shared across components. They provide a way to manage global state and notify subscribers when data changes. Created using writable(), readable(), or derived().
Writable stores are created using writable() from svelte/store. Example: const count = writable(0). They provide set() and update() methods to modify values, and subscribe() to react to changes.
The store contract requires an object with subscribe method that takes a callback and returns unsubscribe function. Any object meeting this contract can be used as a store with $ prefix.
Store changes can be subscribed to using subscribe method or $ prefix in components. Example: count.subscribe(value => console.log(value)) or use $count directly in template.
Readable stores are created using readable() and are read-only. They can only be updated through their start function. Useful for external data sources that components shouldn't modify.
Derived stores are created using derived() and compute values based on other stores. Example: const doubled = derived(count, $count => $count * 2). Update automatically when source stores change.
Auto-subscription happens when using $ prefix with stores in components. Svelte automatically handles subscribe and unsubscribe. No manual cleanup needed. Works in template and script.
Store values can be updated using set() or update() methods. Example: count.set(10) or count.update(n => n + 1). Updates notify all subscribers automatically.
Stores are initialized with initial value in creation. Example: writable(initialValue). Can be undefined. Support synchronous and asynchronous initialization.
Stores integrate with Svelte's reactivity system. Changes trigger reactive updates. Work with reactive declarations ($:). Support reactive dependencies tracking.
Custom stores implement subscribe method. Can add custom methods. Handle internal state. Example: function createCustomStore() { const { subscribe, set } = writable(0); return { subscribe, increment: () => update(n => n + 1) }; }
Async stores handle asynchronous data. Support loading states. Handle errors. Example: const data = writable(null); async function fetchData() { const response = await fetch(url); data.set(await response.json()); }
Store persistence saves state to storage. Implement auto-save. Handle state rehydration. Example: Subscribe to changes and save to localStorage. Load initial state from storage.
Store dependencies use derived stores or reactive statements. Handle update order. Manage circular dependencies. Support dependency tracking.
Store middleware intercepts store operations. Implement custom behaviors. Handle side effects. Example: Create wrapper store with logging or validation.
Store error handling catches and processes errors. Implement error states. Support error recovery. Example: Try-catch in store operations, maintain error state.
Store validation ensures valid state. Implement validation rules. Handle validation errors. Example: Create wrapper store that validates updates.
Store optimization includes batching updates, debouncing, throttling. Handle performance bottlenecks. Example: Implement update batching or debounced updates.
Store composition combines multiple stores. Create higher-order stores. Handle store relationships. Example: Combine multiple stores into single interface.
Store lifecycle management handles initialization, updates, cleanup. Support store creation/destruction. Example: Implement cleanup in onDestroy, handle store initialization.
Advanced patterns include state machines, event sourcing, command pattern. Handle complex state management. Example: Implement store as state machine.
Store synchronization handles multiple instances. Implement cross-tab sync. Handle conflicts. Example: Use broadcast channel for cross-tab communication.
Store time travel tracks state history. Implement undo/redo. Handle state snapshots. Example: Maintain history of states, implement restore functionality.
Store encryption secures sensitive data. Implement encryption/decryption. Handle key management. Example: Encrypt data before storage, decrypt on retrieval.
Store migrations handle version changes. Implement data transforms. Support backwards compatibility. Example: Define migration strategies between versions.
Store monitoring tracks store usage. Implement metrics collection. Handle debugging. Example: Create monitoring wrapper for stores, track operations.
Store testing verifies store behavior. Implement test utilities. Handle async testing. Example: Create test helpers, mock store functionality.
Store documentation describes store usage. Generate documentation automatically. Support examples. Example: Create documentation generator for stores.
Store security prevents unauthorized access. Implement access control. Handle sensitive data. Example: Create secure store wrapper with access checks.
Store performance optimization includes selective updates, memoization, lazy loading. Handle large datasets. Example: Implement selective update mechanism.
Transitions in Svelte are built-in animations that play when elements are added to or removed from the DOM. They are added using the transition: directive, with built-in functions like fade, fly, slide, etc.
Fade transition is used with transition:fade directive. Example: <div transition:fade>. Can accept parameters like duration and delay. Animates opacity from 0 to 1 or vice versa.
in: and out: directives specify different transitions for entering and leaving. Example: <div in:fade out:fly>. Allows different animations for element addition and removal.
Transition parameters customize animation behavior. Example: transition:fade={{duration: 300, delay: 100}}. Include properties like duration, delay, easing function.
Fly transition animates position and opacity. Example: <div transition:fly={{y: 200}}>. Parameters include x, y coordinates, duration, opacity settings.
Slide transition animates element height. Example: <div transition:slide>. Useful for collapsible content. Can customize duration and easing.
Easing functions define animation progression. Import from svelte/easing. Example: transition:fade={{easing: quintOut}}. Affects animation timing and feel.
transition:local restricts transitions to when parent component is added/removed. Prevents transitions during internal state changes. Example: <div transition:fade|local>.
Scale transition animates size and opacity. Example: <div transition:scale>. Parameters include start, opacity, duration. Useful for pop-in effects.
Draw transition animates SVG paths. Example: <path transition:draw>. Parameters include duration, delay, easing. Useful for path animations.
Custom transitions are functions returning animation parameters. Include css and tick functions. Handle enter/exit animations. Support custom parameters.
Transition events include introstart, introend, outrostart, outroend. Listen using on: directive. Example: <div transition:fade on:introend={handleEnd}>.
CSS animations use standard CSS animation properties. Can be combined with transitions. Example: <div animate:flip>. Support keyframes and animation properties.
Flip animation smoothly animates layout changes. Example: <div animate:flip>. Parameters include duration, delay. Useful for list reordering.
Multiple transitions can be combined using different directives. Handle timing coordination. Support transition groups. Manage transition order.
Spring animations use spring() function. Handle physics-based animations. Support stiffness and damping. Example: spring(value, {stiffness: 0.3}).
Crossfade creates smooth transitions between elements. Use crossfade() function. Handle element replacement. Support key-based transitions.
Optimize using CSS transforms, will-change property. Handle hardware acceleration. Manage animation frame rate. Monitor performance metrics.
Transition groups coordinate multiple transitions. Handle group timing. Support synchronized animations. Manage group lifecycle.
Deferred transitions delay animation start. Handle transition timing. Support conditional transitions. Manage transition state.
Complex sequences combine multiple animations. Handle timing coordination. Support sequential and parallel animations. Manage animation state.
Animation middleware intercepts and modifies animations. Handle animation pipeline. Support middleware chain. Manage animation flow.
Animation state machines manage complex animation states. Handle state transitions. Support parallel states. Manage animation logic.
Animation presets define reusable animations. Handle preset parameters. Support preset composition. Manage preset library.
Animation testing verifies animation behavior. Handle timing tests. Support visual regression. Manage test assertions.
Animation debugging tracks animation state. Handle debugging tools. Support timeline inspection. Manage debug output.
Animation optimization improves performance. Handle frame rate. Support GPU acceleration. Manage rendering pipeline.
Animation documentation describes animation behavior. Generate documentation automatically. Support example animations. Manage documentation updates.
Animation monitoring tracks animation performance. Handle metrics collection. Support performance analysis. Manage monitoring data.
Slots are placeholders in components that allow parent components to pass content. Defined using <slot> element. Enable component composition and content projection. Basic slots receive any content passed between component tags.
Default slots provide fallback content. Example: <slot>Default content</slot>. Content appears when parent doesn't provide slot content. Useful for optional content.
Named slots target specific slot locations using name attribute. Example: <slot name='header'>. Content provided using slot='header' attribute. Allows multiple distinct content areas.
Content is passed to named slots using slot attribute. Example: <div slot='header'>Header content</div>. Must match slot name in component. Can pass any valid HTML or components.
Slot props pass data from child to parent through slots. Use let:propertyName directive. Example: <slot name='item' let:item>. Enables parent to access child component data.
Use $$slots object to check slot content. Example: {#if $$slots.header}. Available in component script and template. Useful for conditional rendering.
Fallback content appears when slot is empty. Defined between slot tags. Example: <slot>Fallback</slot>. Provides default UI when parent doesn't provide content.
Slots enable flexible component composition. Support content injection at multiple points. Allow component reuse with different content. Enable layout component patterns.
Slot content maintains parent component scope. Can access parent variables and functions. Cannot directly access child component state. Uses parent component context.
Slot content can be styled in both parent and child. Child styles using :slotted() selector. Parent styles apply normally. Support style encapsulation.
Conditional slots use if blocks around slots. Handle slot presence checks. Support dynamic slot selection. Example: {#if condition}<slot></slot>{/if}
Dynamic slot names use computed values. Support runtime slot selection. Handle dynamic content projection. Example: <slot name={dynamicName}>
Slot validation checks content type and structure. Handle invalid content. Support content restrictions. Implement validation logic.
Slot events bubble through component hierarchy. Handle event forwarding. Support event modification. Manage event propagation.
Slot lifecycles manage content updates. Handle content mounting/unmounting. Support cleanup operations. Manage slot state.
Slot composition combines multiple slots. Handle nested slots. Support slot inheritance. Implement composition patterns.
Slot optimization improves rendering efficiency. Handle content caching. Support lazy loading. Manage update frequency.
Error boundaries catch slot content errors. Handle error recovery. Support fallback content. Manage error state.
Slot monitoring tracks content changes. Handle performance metrics. Support debugging tools. Manage monitoring state.
Advanced patterns include render props, compound slots. Handle complex compositions. Support pattern libraries. Implement reusable patterns.
Slot testing verifies content projection. Handle integration testing. Support unit tests. Implement test utilities.
Slot documentation describes usage patterns. Generate documentation automatically. Support example usage. Manage documentation updates.
Slot security prevents content injection. Handle content sanitization. Support content restrictions. Implement security policies.
Slot versioning handles API changes. Implement version migration. Support backwards compatibility. Manage version state.
Slot debugging tracks content flow. Handle debugging tools. Support breakpoints. Manage debug output.
Optimization strategies improve slot performance. Handle content caching. Support virtual slots. Implement update strategies.
State management handles slot-specific state. Implement state containers. Support state sharing. Manage state updates.
Slot accessibility ensures content is accessible. Handle ARIA attributes. Support screen readers. Implement a11y patterns.
Slots are placeholders in components that allow parent components to pass content. Defined using <slot> element. Enable component composition and content projection. Basic slots receive any content passed between component tags.
Default slots provide fallback content. Example: <slot>Default content</slot>. Content appears when parent doesn't provide slot content. Useful for optional content.
Content is passed to named slots using slot attribute. Example: <div slot='header'>Header content</div>. Must match slot name in component. Can pass any valid HTML or components.
Slot props pass data from child to parent through slots. Use let:propertyName directive. Example: <slot name='item' let:item>. Enables parent to access child component data.
Use $$slots object to check slot content. Example: {#if $$slots.header}. Available in component script and template. Useful for conditional rendering.
Fallback content appears when slot is empty. Defined between slot tags. Example: <slot>Fallback</slot>. Provides default UI when parent doesn't provide content.
Slots enable flexible component composition. Support content injection at multiple points. Allow component reuse with different content. Enable layout component patterns.
Slot content maintains parent component scope. Can access parent variables and functions. Cannot directly access child component state. Uses parent component context.
Slot content can be styled in both parent and child. Child styles using :slotted() selector. Parent styles apply normally. Support style encapsulation.
Conditional slots use if blocks around slots. Handle slot presence checks. Support dynamic slot selection. Example: {#if condition}<slot></slot>{/if}
Dynamic slot names use computed values. Support runtime slot selection. Handle dynamic content projection. Example: <slot name={dynamicName}>
Slot validation checks content type and structure. Handle invalid content. Support content restrictions. Implement validation logic.
Slot events bubble through component hierarchy. Handle event forwarding. Support event modification. Manage event propagation.
Slot middleware processes slot content. Handle content transformation. Support content filtering. Implement middleware chain.
Slot lifecycles manage content updates. Handle content mounting/unmounting. Support cleanup operations. Manage slot state.
Slot composition combines multiple slots. Handle nested slots. Support slot inheritance. Implement composition patterns.
Slot optimization improves rendering efficiency. Handle content caching. Support lazy loading. Manage update frequency.
Error boundaries catch slot content errors. Handle error recovery. Support fallback content. Manage error state.
Slot monitoring tracks content changes. Handle performance metrics. Support debugging tools. Manage monitoring state.
Advanced patterns include render props, compound slots. Handle complex compositions. Support pattern libraries. Implement reusable patterns.
Slot testing verifies content projection. Handle integration testing. Support unit tests. Implement test utilities.
Slot documentation describes usage patterns. Generate documentation automatically. Support example usage. Manage documentation updates.
Slot security prevents content injection. Handle content sanitization. Support content restrictions. Implement security policies.
Slot versioning handles API changes. Implement version migration. Support backwards compatibility. Manage version state.
Slot debugging tracks content flow. Handle debugging tools. Support breakpoints. Manage debug output.
Optimization strategies improve slot performance. Handle content caching. Support virtual slots. Implement update strategies.
State management handles slot-specific state. Implement state containers. Support state sharing. Manage state updates.
Slot accessibility ensures content is accessible. Handle ARIA attributes. Support screen readers. Implement a11y patterns.
SvelteKit provides file-based routing where files in the routes directory automatically become pages. URLs correspond to file paths. Supports dynamic routes, nested layouts, and route parameters.
Create a route by adding a +page.svelte file in the routes directory. Example: src/routes/about/+page.svelte becomes '/about'. File structure mirrors URL structure.
Dynamic routes use square brackets in file names. Example: [slug]/+page.svelte creates dynamic segment. Parameters available through page store. Support multiple dynamic segments.
Route parameters accessed through page.params. Example: export let data; const { slug } = data. Available in +page.svelte and +page.server.js files.
Layout files (+layout.svelte) provide shared UI for multiple routes. Define common elements like navigation, footer. Support nested layouts. Content injected via <slot>.
Navigation uses <a> tags or programmatic goto function. SvelteKit handles client-side navigation. Supports prefetching with data attribute. Maintains scroll position.
Route groups organize routes using (group) syntax. Don't affect URL structure. Share layouts within groups. Support multiple groups. Help organize large applications.
Data loading uses +page.js or +page.server.js files. Export load function for data fetching. Returns props for page component. Supports server-side loading.
Error page (+error.svelte) handles route errors. Displays when errors occur. Access error details through error prop. Support custom error handling.
Redirects use redirect function from @sveltejs/kit. Can redirect in load functions or actions. Support temporary/permanent redirects. Handle authentication redirects.
Route guards protect routes using load functions. Check authentication/authorization. Return redirect for unauthorized access. Support async checks.
Nested layouts use multiple +layout.svelte files. Each level adds layout. Support layout inheritance. Handle layout data. Share layout between routes.
Preloading uses data-sveltekit-preload attribute. Fetches data before navigation. Support hover preloading. Handle preload strategies. Optimize performance.
Route transitions use page transitions API. Support enter/leave animations. Handle transition lifecycle. Implement custom transitions. Manage transition state.
Route middleware uses hooks.server.js file. Handle request/response. Support authentication. Implement custom logic. Manage middleware chain.
Route caching implements caching strategies. Handle data caching. Support page caching. Implement cache invalidation. Manage cache lifecycle.
Route validation handles parameter validation. Support request validation. Implement validation rules. Handle validation errors. Manage validation state.
Route state management uses stores or context. Handle state persistence. Support state sharing. Implement state updates. Manage state lifecycle.
Route optimization includes code splitting, preloading. Handle lazy loading. Support route prioritization. Implement performance monitoring.
Advanced patterns include nested routes, parallel routes. Handle complex navigation. Support route composition. Implement routing strategies.
Route i18n supports multiple languages. Handle URL localization. Support language switching. Implement i18n patterns. Manage translations.
Route testing verifies routing behavior. Handle navigation testing. Support integration testing. Implement test utilities. Manage test coverage.
Route monitoring tracks navigation patterns. Handle analytics integration. Support performance tracking. Implement monitoring tools. Manage monitoring data.
Route security prevents unauthorized access. Handle authentication flows. Support authorization rules. Implement security measures. Manage security policies.
Route documentation describes routing structure. Generate documentation automatically. Support example routes. Manage documentation updates. Handle versioning.
Route debugging tracks routing issues. Handle debugging tools. Support state inspection. Implement debug logging. Manage debug output.
Route accessibility ensures accessible navigation. Handle focus management. Support screen readers. Implement a11y patterns. Manage announcements.
Error handling manages routing errors. Handle error boundaries. Support error recovery. Implement error logging. Manage error state.
Code splitting optimizes route loading. Handle chunk generation. Support dynamic imports. Implement splitting strategies. Manage bundle size.
The Context API allows passing data through the component tree without prop drilling. Uses setContext and getContext functions. Context is available to component and its descendants. Useful for sharing data/functionality.
Context is set using setContext function from svelte. Example: setContext('key', value). Must be called during component initialization. Value can be any type including functions.
Context is retrieved using getContext function. Example: const value = getContext('key'). Must use same key as setContext. Available in component and child components.
Context keys must be unique within component tree. Often use symbols for guaranteed uniqueness. Example: const key = Symbol(). Prevents key collisions between different contexts.
Context exists throughout component lifecycle. Created during initialization. Available until component destruction. Cannot be changed after initialization. New values require component reinitialization.
Common uses include theme data, localization, authentication state, shared functionality. Useful for cross-cutting concerns. Avoids prop drilling. Supports component composition.
Functions can be shared through context. Example: setContext('api', { method: () => {} }). Allows child components to access shared methods. Supports dependency injection pattern.
Context is scoped to component and descendants. Not available to parent or sibling components. Multiple instances create separate contexts. Follows component hierarchy.
getContext returns undefined if context not found. Should handle undefined case. Can provide default values. Consider error handling for required context.
Context is static, set during initialization. Stores are reactive, can change over time. Context good for static values/dependencies. Stores better for changing state.
Context patterns include provider components, dependency injection, service locator. Handle context composition. Support context inheritance. Implement context strategies.
Context updates require component reinitialization. Can combine with stores for reactive updates. Handle update propagation. Manage update lifecycle.
Context dependencies manage relationships between contexts. Handle dependency order. Support circular dependencies. Implement dependency resolution.
Context composition combines multiple contexts. Handle context merging. Support context inheritance. Implement composition patterns.
Context initialization sets up initial context state. Handle async initialization. Support initialization order. Implement initialization strategies.
Context middleware processes context operations. Handle context transformation. Support middleware chain. Implement middleware patterns.
Context error handling manages error states. Handle missing context. Support error recovery. Implement error boundaries.
Context optimization improves performance. Handle context caching. Support selective updates. Implement optimization strategies.
Context cleanup manages resource disposal. Handle cleanup order. Support cleanup hooks. Implement cleanup strategies.
Advanced patterns include context injection, service location, dependency trees. Handle complex dependencies. Support pattern composition.
Context testing verifies context behavior. Handle test isolation. Support integration testing. Implement test utilities.
Context monitoring tracks context usage. Handle performance tracking. Support debugging tools. Implement monitoring strategies.
Context documentation describes context usage. Generate documentation automatically. Support example usage. Manage documentation updates.
Context versioning handles API changes. Implement version migration. Support backwards compatibility. Manage version state.
Context security prevents unauthorized access. Handle access control. Support security policies. Implement security measures.
Context debugging tracks context issues. Handle debugging tools. Support state inspection. Implement debug logging.
Performance monitoring tracks context efficiency. Handle metrics collection. Support performance analysis. Implement optimization strategies.
Dependency injection manages component dependencies. Handle injection patterns. Support service location. Implement injection strategies.
Type safety ensures correct context usage. Handle TypeScript integration. Support type checking. Implement type definitions.
Bindings create two-way data flow between DOM elements and variables using bind: directive. Example: <input bind:value={text}>. Updates flow both ways, DOM to variable and variable to DOM.
Basic form bindings include value for text inputs, checked for checkboxes, group for radio/checkbox groups. Example: <input bind:value>, <input type='checkbox' bind:checked>.
use: directive attaches actions (reusable DOM node functionality) to elements. Example: <div use:action>. Actions can have parameters. Support cleanup through returned function.
class: directive conditionally applies CSS classes. Example: <div class:active={isActive}>. Shorthand available when variable name matches class name.
Custom component binding uses bind: on exported props. Component must export the variable. Example: <CustomInput bind:value>. Supports two-way binding.
style: directive sets inline styles conditionally. Example: <div style:color={textColor}>. Can use shorthand when variable name matches style property.
Select elements bind using value or selectedIndex. Example: <select bind:value={selected}>. Supports multiple selection with array binding.
this binding references DOM element or component instance. Example: <div bind:this={element}>. Useful for direct DOM manipulation or component method access.
bind:group groups radio/checkbox inputs. Binds multiple inputs to single value/array. Example: <input type='radio' bind:group={selected} value='option'>.
Contenteditable elements bind using textContent or innerHTML. Example: <div contenteditable bind:textContent={text}>. Supports rich text editing.
Custom actions are functions returning optional destroy method. Handle DOM node manipulation. Support parameters. Example: use:customAction={params}.
Binding validation ensures valid values. Handle input constraints. Support custom validation. Implement error handling. Manage validation state.
Binding middleware processes binding operations. Handle value transformation. Support validation chain. Implement middleware pattern.
Binding dependencies manage related bindings. Handle dependency updates. Support dependency tracking. Implement dependency resolution.
Binding optimization improves update efficiency. Handle update batching. Support selective updates. Implement performance monitoring.
Custom directives extend element functionality. Handle directive lifecycle. Support directive parameters. Implement cleanup methods.
Binding error handling manages invalid states. Handle error recovery. Support error notifications. Implement error boundaries.
Binding composition combines multiple bindings. Handle binding interaction. Support binding inheritance. Implement composition patterns.
Binding cleanup manages resource disposal. Handle cleanup order. Support cleanup hooks. Implement cleanup strategies.
Advanced patterns include computed bindings, conditional bindings. Handle complex scenarios. Support pattern composition.
Binding testing verifies binding behavior. Handle test isolation. Support integration testing. Implement test utilities.
Binding monitoring tracks binding usage. Handle performance tracking. Support debugging tools. Implement monitoring strategies.
Binding documentation describes binding usage. Generate documentation automatically. Support example usage. Manage documentation updates.
Binding security prevents unauthorized access. Handle input sanitization. Support security policies. Implement security measures.
Binding debuggers track binding issues. Handle debugging tools. Support state inspection. Implement debug logging.
Type safety ensures correct binding usage. Handle TypeScript integration. Support type checking. Implement type definitions.
Optimization strategies improve binding performance. Handle update batching. Support selective updates. Implement performance metrics.
State management handles binding state. Handle state updates. Support state sharing. Implement state patterns.
Binding accessibility ensures accessible usage. Handle ARIA attributes. Support screen readers. Implement a11y patterns.
Server-Side Rendering (SSR) generates HTML on the server instead of client. Provides better initial page load, SEO benefits. SvelteKit handles SSR automatically with hydration on client-side.
Hydration is the process where client-side JavaScript takes over server-rendered HTML. Makes static content interactive. Preserves server-rendered state. Happens automatically in SvelteKit.
Server-side data loading uses load functions in +page.server.js. Returns data for page rendering. Supports async operations. Data available during SSR and hydration.
Client-Side Rendering (CSR) fallback handles cases where SSR fails or is disabled. Uses +page.js instead of +page.server.js. Provides graceful degradation.
Disable SSR using export const ssr = false in +page.js. Page renders only on client. Useful for browser-specific functionality. Impacts initial page load.
Server-only modules run exclusively on server. Use .server.js extension. Cannot be imported by client code. Useful for sensitive operations like database access.
SSR errors handled by error.svelte pages. Support error boundaries. Can provide fallback content. Error details available through error prop.
Streaming SSR sends HTML in chunks as it's generated. Improves Time To First Byte (TTFB). Supports progressive rendering. Available through Response.body.
Environment variables accessed through $env/static/private or $env/dynamic/private. Only available server-side. Must prefix with VITE_ for client access.
Prerendering generates static HTML at build time. Uses export const prerender = true. Improves performance. Suitable for static content.
SSR data fetching uses load functions. Handle async operations. Support caching strategies. Implement error handling. Manage data dependencies.
SSR caching implements cache strategies. Handle cache invalidation. Support cache headers. Implement cache storage. Manage cache lifecycle.
SSR optimization includes code splitting, caching, streaming. Handle resource optimization. Support performance monitoring. Implement optimization strategies.
SSR session state manages user sessions. Handle session storage. Support session persistence. Implement session security. Manage session lifecycle.
SSR middleware processes server requests. Handle request transformation. Support middleware chain. Implement middleware patterns. Manage middleware order.
SSR authentication manages user auth state. Handle auth flows. Support session management. Implement auth strategies. Manage auth security.
SSR routing handles server-side navigation. Handle route matching. Support dynamic routes. Implement routing strategies. Manage route state.
SSR headers manage HTTP headers. Handle cache control. Support content types. Implement header strategies. Manage header security.
SSR forms handle form submissions server-side. Handle form validation. Support file uploads. Implement CSRF protection. Manage form state.
Advanced patterns include streaming, progressive enhancement. Handle complex scenarios. Support pattern composition. Implement advanced strategies.
SSR testing verifies server rendering. Handle test isolation. Support integration testing. Implement test utilities. Manage test coverage.
SSR monitoring tracks server performance. Handle metrics collection. Support debugging tools. Implement monitoring strategies.
SSR security prevents vulnerabilities. Handle input sanitization. Support security headers. Implement security measures. Manage security policies.
SSR error handling manages server errors. Handle error recovery. Support error boundaries. Implement error logging. Manage error state.
Optimization strategies improve SSR performance. Handle resource optimization. Support caching strategies. Implement performance metrics.
State management handles server state. Handle state serialization. Support state hydration. Implement state patterns.
SSR documentation describes server features. Generate documentation automatically. Support example usage. Manage documentation updates.
SSR debugging tracks server issues. Handle debugging tools. Support state inspection. Implement debug logging.
SSR internationalization handles multiple languages. Support content translation. Implement i18n patterns. Manage translations.
SSR accessibility ensures server-rendered content is accessible. Handle ARIA attributes. Support screen readers. Implement a11y patterns.
Vitest is the recommended testing framework for Svelte applications. It's fast, provides good integration with SvelteKit, and supports component testing. Jest can also be used but requires additional configuration.
Component tests use @testing-library/svelte. Mount components using render method. Test component behavior and DOM updates. Example: import { render } from '@testing-library/svelte'; const { getByText } = render(Component);
Svelte Inspector is a development tool enabled with 'ctrl + shift + i'. Shows component hierarchy, props, state. Helps debug component structure and data flow. Available in development mode.
Async tests use async/await syntax. Test async operations using act function. Handle promises and timeouts. Example: await fireEvent.click(button); await findByText('result');
Testing matchers verify component state/behavior. Include toBeInTheDocument, toHaveTextContent, toBeVisible. Provided by @testing-library/jest-dom. Support custom matchers.
Store state can be debugged using $store syntax. Subscribe to store changes. Log state updates. Use Svelte devtools. Monitor store mutations.
Testing hierarchy includes unit tests, integration tests, end-to-end tests. Focus on component isolation. Test component interaction. Verify application flow.
Event handlers tested using fireEvent. Simulate user interactions. Verify event outcomes. Example: fireEvent.click(button); expect(result).toBe(expected);
Snapshot testing captures component output. Compares against stored snapshots. Detects UI changes. Example: expect(container).toMatchSnapshot();
Props testing verifies component behavior with different props. Test prop types and values. Handle prop updates. Verify component rendering.
Integration testing verifies component interaction. Test multiple components. Handle data flow. Implement test scenarios. Manage test environment.
Store testing verifies store behavior. Test store updates. Handle subscriptions. Implement store mock. Manage store state.
Test mocks simulate dependencies. Handle external services. Support mock responses. Implement mock behavior. Manage mock state.
Route testing verifies navigation behavior. Test route parameters. Handle route changes. Implement navigation tests. Manage route state.
Test fixtures provide test data. Handle data setup. Support test isolation. Implement fixture management. Manage fixture cleanup.
Animation testing verifies transition behavior. Test animation states. Handle animation timing. Implement animation checks. Manage animation events.
Test hooks handle test lifecycle. Support setup/teardown. Implement test utilities. Manage test state. Handle test dependencies.
Error handling testing verifies error states. Test error boundaries. Handle error recovery. Implement error scenarios. Manage error logging.
Test coverage tracks code coverage. Generate coverage reports. Set coverage targets. Implement coverage checks. Manage coverage gaps.
Test organization structures test files. Group related tests. Support test discovery. Implement naming conventions. Manage test hierarchy.
Advanced patterns include test factories, test builders. Handle complex scenarios. Support pattern composition. Implement testing strategies.
Test automation handles continuous testing. Set up CI/CD pipelines. Support automated runs. Implement test reporting. Manage test environments.
Performance testing measures component efficiency. Test rendering speed. Handle resource usage. Implement benchmarks. Manage performance metrics.
Accessibility testing verifies a11y compliance. Test screen readers. Handle keyboard navigation. Implement ARIA testing. Manage compliance reports.
Visual regression detects UI changes. Compare screenshots. Handle visual diffs. Implement image comparison. Manage baseline images.
Test monitoring tracks test execution. Handle test metrics. Support test analytics. Implement monitoring tools. Manage monitoring data.
Test documentation describes test cases. Generate documentation automatically. Support example tests. Manage documentation updates. Handle versioning.
Security testing verifies application security. Test vulnerability prevention. Handle security scenarios. Implement security checks. Manage security reports.
Load testing verifies application performance. Test under heavy load. Handle stress scenarios. Implement load simulation. Manage performance data.
Environment management handles test environments. Set up configurations. Support multiple environments. Implement environment isolation. Manage dependencies.
Svelte achieves performance through compile-time optimization. Generates vanilla JavaScript with minimal runtime overhead. Uses surgical DOM updates. Eliminates virtual DOM overhead.
Code splitting automatically breaks application into smaller chunks. Routes loaded on demand. Reduces initial bundle size. Uses dynamic imports. Improves load time.
Svelte compiles reactive statements into efficient JavaScript. Updates only affected DOM elements. No diffing required. Uses fine-grained reactivity system. Minimizes overhead.
Asset optimization includes minification, bundling, compression. Uses Vite for build process. Optimizes images and styles. Supports cache headers. Improves load performance.
Component optimization includes proper key usage, avoiding unnecessary updates, using tick() function. Implement efficient loops. Minimize state changes. Use proper event handling.
Lazy loading defers loading of components until needed. Uses dynamic imports. Supports route-level code splitting. Improves initial load time. Reduces bundle size.
Memory leak prevention includes proper cleanup in onDestroy, unsubscribing from stores, removing event listeners. Clear intervals/timeouts. Handle component disposal.
Prefetching loads route data before navigation. Uses sveltekit:prefetch directive. Improves perceived performance. Supports hover-based prefetching. Reduces loading time.
Store optimization includes batching updates, using derived stores efficiently, implementing selective updates. Minimize store subscribers. Handle update frequency.
Tree-shaking removes unused code during build. Reduces bundle size. Supported by default. Works with ES modules. Eliminates dead code.
Caching strategies include browser cache, service workers, SSR cache. Handle cache invalidation. Support offline functionality. Implement cache policies.
Animation optimization includes using CSS animations when possible, hardware acceleration, efficient transitions. Handle animation timing. Minimize reflows.
Large list optimization includes virtual scrolling, pagination, infinite scroll. Use keyed each blocks. Implement list chunking. Handle DOM recycling.
Image optimization includes lazy loading, responsive images, proper formats. Use srcset attribute. Implement placeholder images. Handle image compression.
Performance monitoring tracks metrics like load time, FCP, TTI. Use browser DevTools. Implement analytics. Handle performance reporting.
SSR optimization includes caching, streaming, efficient data loading. Handle server resources. Implement response compression. Optimize render time.
Resource prioritization includes critical CSS, script loading strategies, preload/prefetch. Handle resource order. Implement loading priorities.
Build optimization includes proper Vite/Rollup config, production settings, environment optimization. Handle build process. Implement build strategies.
Performance testing measures load time, interaction time, resource usage. Implement benchmarks. Handle performance metrics. Support performance monitoring.
Advanced strategies include worker threads, memory pooling, render optimization. Handle complex scenarios. Support optimization patterns.
Bundle analysis examines build output size, dependencies, chunks. Use bundle analyzers. Handle size optimization. Implement analysis tools.
Network optimization includes request batching, protocol optimization, CDN usage. Handle network caching. Implement request strategies.
Runtime optimization includes memory management, event delegation, efficient algorithms. Handle runtime performance. Implement optimization techniques.
Rendering optimization includes layout thrashing prevention, paint optimization, composite layers. Handle render performance. Implement render strategies.
State optimization includes efficient updates, state normalization, update batching. Handle state management. Implement state strategies.
Resource optimization includes asset management, resource loading, dependency optimization. Handle resource usage. Implement optimization policies.
Security optimization includes CSP, secure headers, vulnerability prevention. Handle security measures. Implement security strategies.
Monitoring tools track performance metrics, resource usage, errors. Handle monitoring systems. Implement tracking strategies.
Optimization documentation describes performance strategies. Generate performance reports. Support optimization guidelines. Implement documentation updates.
Understand concepts like reactivity, components, stores, and lifecycle methods.
Work on creating components and implementing state management solutions.
Dive into animations, transitions, and advanced compiler features.
Expect hands-on challenges to build, optimize, and debug Svelte applications.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Svelte questions, mock interviews, and more to secure your dream role.
Start Preparing now