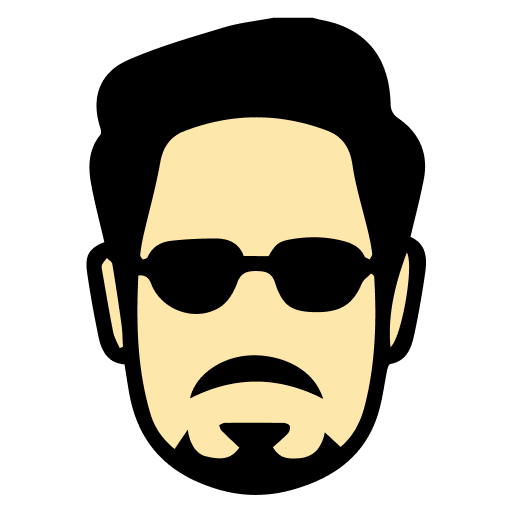
Angular's comprehensive framework and powerful features make it a leading choice for enterprise applications. Stark.ai offers a comprehensive collection of Angular interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Angular is a TypeScript-based open-source framework for building web applications. Key differences from AngularJS...
Decorators are design patterns that modify JavaScript classes. Common Angular decorators include: @Component...
NgModule is a decorator that defines a module in Angular. Essential properties include: declarations (components,...
Dependency Injection is a design pattern where dependencies are provided to classes instead of creating them....
Lifecycle hooks are methods that Angular calls on components/directives at specific moments. Major hooks: ngOnInit...
Constructor is a TypeScript feature called when class is instantiated, used for dependency injection. ngOnInit is an...
Change detection is Angular's process of synchronizing component model and view. Uses Zone.js to track async...
Data binding synchronizes component and view. Types: Interpolation {{data}}, Property binding [property], Event...
ViewChild/ViewChildren decorators provide access to child elements in component template. ViewChild returns single...
Services are singleton objects used for sharing data/functionality across components. Marked with @Injectable...
providedIn configures service scope in @Injectable decorator (root, platform, any, specific module). providers array...
Templates define component's view in HTML with Angular-specific syntax. Features: data binding, directives, pipes,...
Ahead-of-Time compilation compiles Angular application at build time. Benefits: faster rendering, smaller download...
Angular DI uses hierarchical injector tree: NullInjector (root), Platform, Module, Element (component/directive)....
Template reference variables (#var) create references to DOM elements, components, or directives in template. Used...
Zone.js provides execution context tracking for async operations. Angular uses it for automatic change detection by...
declarations list components/directives/pipes belonging to module. imports list other modules needed. providers list...
Angular elements are Angular components packaged as custom elements (Web Components). Use cases: embedding Angular...
Methods include: @Input/@Output decorators, services (state management), ViewChild/ContentChild, event emitters,...
Angular CLI uses webpack for bundling. Lazy loading implemented through RouterModule with loadChildren syntax....
Async pipe automatically subscribes/unsubscribes to observables/promises in templates. Handles subscription...
HostBinding decorates properties to bind to host element properties. HostListener decorates methods to handle host...
Error handling through: try-catch blocks, RxJS catchError operator, HTTP interceptors, ErrorHandler class, Router...
ViewEncapsulation controls component CSS encapsulation. Types: Emulated (default, scoped CSS), None (global CSS),...
Schematics are templates for generating/modifying code. Used by Angular CLI for scaffolding components, services,...
Component styles defined in styles/styleUrls properties. Supports CSS encapsulation through ViewEncapsulation. Can...
NgZone service provides access to Angular's zone for executing work inside/outside Angular's zone. Used for...
Angular i18n uses XLF/XLIFF files for translations. Supports: text extraction, pluralization, date/number...
Angular Router enables navigation between views. Core features include: path-based routing, child routes, route...
Route guards control route access/behavior. Types: CanActivate (access control), CanDeactivate (leaving control),...
Lazy loading implemented using loadChildren syntax in route configuration: path: 'admin', loadChildren: () =>...
Route parameters pass data through URL. Types: Required parameters (:id), Optional parameters (?id=), Query...
Resolvers pre-fetch data before route activation. Implement Resolve interface in service. Use cases: loading...
Child routes create hierarchical route structures. Configured using children property in route configuration....
Route events monitored using Router.events observable. Events include: NavigationStart, NavigationEnd,...
Navigation methods: router.navigate([path]), routerLink directive, router.navigateByUrl(url). navigate/navigateByUrl...
Location strategies: PathLocationStrategy (default, uses HTML5 History API, clean URLs), HashLocationStrategy (uses...
404 routes configured using wildcard path '**' as last route in configuration. Redirects to specific component/path...
RouteReuseStrategy controls component reuse during navigation. Custom implementation can define when to store/reuse...
Authentication guards implement CanActivate interface. Check authentication state, redirect unauthorized access....
Auxiliary routes (named router outlets) allow multiple independent routes. Use named router-outlet. Enable...
Query parameters preserved using queryParamsHandling option in navigation methods/routerLink. Values: 'merge'...
paramMap is newer, immutable, provides has()/get() methods. params is older, direct object access. paramMap...
Named outlets allow multiple router-outlets with unique names. Configure routes with outlet property. Enable complex...
Best practices: module-based routing, lazy loading, proper guard implementation, consistent naming, error handling,...
Route data configured using data property in route definition. Static data accessed via ActivatedRoute.data...
Router state contains current route tree information. Accessed using Router.routerState or ActivatedRoute. Provides...
Breadcrumbs implemented using router state/events. Track active route path, extract route data. Consider nested...
CanDeactivate prevents leaving route without confirmation. Common for forms, unsaved changes. Returns...
Route transitions implemented using Angular animations. Trigger on router-outlet, use route data. Consider...
Absolute paths start with '/', relative paths based on current route. Relative navigation uses '../' for parent...
Redirects configured using redirectTo property in route definition. Support path matching, parameters. Used for...
routerLinkActive adds class when route active. Configure with exact match option. Useful for navigation...
Role-based routing uses guards checking user roles/permissions. Combine with authentication guard, role service....
Matrix parameters are URL segments with key-value pairs. Format: path;key=value. Alternative to query parameters....
Route caching through RouteReuseStrategy. Store component state, reuse on navigation. Consider memory usage, state...
Path matching strategies control route matching behavior. Options: prefix (default, matches start), full (exact...
Navigation failures handled through Router.events or navigation promise rejection. Implement error handling, user...
Angular has Template-driven forms (simple, directive based, async validation) and Reactive forms (complex, explicit,...
Reactive form validation uses validators in FormControl/FormGroup construction. Built-in validators (required,...
FormControl tracks value/validation of individual form field. FormGroup groups related controls together, tracks...
Custom validators are functions returning validation errors or null. Can be synchronous (ValidatorFn) or...
FormArray manages collection of form controls/groups dynamically. Used for variable-length form fields (dynamic...
Form submission handled through ngSubmit event or form's submit method. Implement validation checks, disable submit...
Form states include: pristine/dirty, touched/untouched, valid/invalid, pending. Tracked through form control...
Cross-field validation implemented through form group validators. Access multiple control values, compare fields...
Async validators perform validation asynchronously (API calls, database checks). Return Promise/Observable. Used for...
Forms reset using reset() method on FormGroup/FormControl. Can provide initial values, reset specific controls....
FormBuilder service provides syntactic sugar for creating form controls. Simplifies complex form creation, reduces...
File uploads handled through input type='file' and FormData. Use change event to capture files, implement validation...
valueChanges and statusChanges are observables tracking form changes. valueChanges emits new values, statusChanges...
Conditional validation changes validation rules based on conditions. Implement through setValidators(),...
Form control wrappers are custom components wrapping form controls. Provide reusable validation, styling, behavior....
Form arrays validation applies at array and item levels. Validate array length, individual controls. Can implement...
updateOn controls when form control updates (change, blur, submit). Affects validation timing, value updates....
Custom error messages handled through error states in template. Access errors through control.errors property. Can...
Best practices: immediate feedback, clear error messages, consistent validation, proper error states, accessibility...
Dynamic forms built using form arrays/groups, metadata-driven approach. Generate controls from configuration/data....
ngModel provides two-way data binding in template-driven forms. Creates form controls implicitly, tracks...
Form submission errors handled through error callbacks, error states. Display user-friendly messages, highlight...
Nested form groups organize related controls hierarchically. Created using FormBuilder.group() nesting. Support...
Validation messages implemented through template logic, error states. Can use validation message service,...
Dirty checking tracks form value changes. pristine/dirty states indicate modifications. Used for save prompts, reset...
Form array validation patterns include: min/max items, unique values, dependent validations. Implement through...
Common patterns: required fields, email format, password strength, numeric ranges, custom regex. Implement through...
Form state persistence through services, local storage, state management. Consider autosave functionality, recovery...
Form control events include: valueChanges, statusChanges, focus/blur events. Handle through event bindings,...
Multi-step validation through form groups per step, wizard pattern. Validate each step, track completion status....
HttpClient is Angular's built-in HTTP client. Features: typed responses, observables for requests, interceptors...
Interceptors intercept and modify HTTP requests/responses. Use cases: authentication headers, error handling,...
Error handling through catchError operator, error interceptors. Implement global/local error handlers, retry logic....
Best practices: separate service layer, typed interfaces, proper error handling, environment configuration. Consider...
Caching implemented through interceptors, services. Use shareReplay operator, cache storage. Consider cache...
HttpParams manage URL parameters in requests. Immutable object for query parameters. Methods: set(), append(),...
File uploads using FormData, multipart/form-data content type. Support progress events, cancellation. Consider file...
Request cancellation using takeUntil operator, AbortController. Implement cleanup on component destruction....
Authentication through interceptors, auth headers. Implement token management, refresh logic. Consider session...
Headers managed through HttpHeaders class. Set content type, authorization, custom headers. Immutable object,...
Retry logic using retry/retryWhen operators. Configure retry count, delay. Consider error types, backoff strategy....
Progress events track upload/download progress. Use reportProgress option, HttpEventType. Implement progress...
Concurrent requests using forkJoin, combineLatest operators. Consider error handling, loading states. Important for...
CORS (Cross-Origin Resource Sharing) handled through server configuration, proxy settings. Configure allowed...
API versioning through URL prefixes, headers, interceptors. Configure base URLs, version management. Consider...
Timeouts configured using timeout operator, request configuration. Consider network conditions, server response...
Offline support through service workers, local storage. Implement queue system, sync logic. Consider conflict...
Response types: json, text, blob, arrayBuffer. Configure using responseType option. Consider data format,...
API mocking through interceptors, mock services. Configure development environment, test data. Consider realistic...
Security considerations: XSS prevention, CSRF protection, secure headers. Implement authentication, authorization....
Large data handling through pagination, infinite scroll. Implement virtual scrolling, data chunking. Consider...
Debouncing delays request execution until pause in triggering. Implement using debounceTime operator. Consider user...
Error mapping through interceptors, error services. Transform server errors to application format. Consider error...
WebSockets enable real-time communication. Implement using socket.io, custom services. Consider connection...
Rate limiting through interceptors, request queuing. Implement backoff strategy, request prioritization. Consider...
Transformation through interceptors, map operator. Modify request/response data format. Consider data consistency,...
Request queueing through custom services, RxJS operators. Handle sequential requests, priorities. Consider error...
Testing practices: unit tests for services, mock interceptors, integration tests. Consider error scenarios, async...
Documentation through Swagger/OpenAPI, custom documentation. Generate TypeScript interfaces, API services. Consider...
Change Detection determines UI updates based on data changes. Default strategy checks all components, OnPush checks...
Lazy Loading loads modules on demand, reducing initial bundle size. Implemented through routing configuration using...
Ahead-of-Time compilation compiles templates during build. Benefits: faster rendering, smaller payload, earlier...
Memory optimization through: proper unsubscription from observables, avoiding memory leaks, cleaning up event...
trackBy provides unique identifier for items in ngFor. Helps Angular track items efficiently, reduces DOM...
Virtual scrolling renders only visible items using ScrollingModule. Improves performance with large lists. Configure...
Pure pipes execute only on input changes, impure on every change detection. Pure pipes better for performance, cache...
Bundle optimization through: tree shaking, lazy loading, proper imports, removing unused dependencies. Use built-in...
Tree Shaking removes unused code from final bundle. Enabled by default in production builds. Requires proper module...
Caching implemented through services, HTTP interceptors. Cache API responses, computed values, static assets....
Web Workers run scripts in background threads. Offload heavy computations, improve responsiveness. Implement using...
Form optimization through: proper validation timing, minimal form controls, efficient value updates. Consider...
enableProdMode disables development checks and warnings. Improves performance in production. Call before...
Preloading loads modules after initial load. Configure through PreloadAllModules or custom strategy. Balance between...
Best practices: proper change detection, lazy loading, AOT compilation, bundle optimization, caching implementation....
SSR using Angular Universal. Improves initial load, SEO. Configure through Angular CLI commands. Consider hydration,...
Differential loading serves modern/legacy bundles based on browser support. Reduces bundle size for modern browsers....
Image optimization through: lazy loading, proper sizing, format selection, CDN usage. Implement loading attribute,...
Ivy is Angular's rendering engine. Benefits: smaller bundles, faster compilation, better debugging. Default since...
Performance monitoring through: browser dev tools, Angular profiler, custom metrics. Track key indicators, implement...
Performance budgets set size limits for bundles. Configure in angular.json. Enforce during build process. Consider...
Router optimization through: preloading strategies, route guards optimization, minimal route data. Consider...
Code splitting divides code into smaller chunks. Improves initial load time, enables lazy loading. Implement through...
Library optimization through: selective imports, lazy loading, alternatives evaluation. Consider bundle impact,...
Service workers enable offline capabilities, caching. Improve load times, reduce server load. Configure through...
Efficient infinite scrolling through: virtual scrolling, data chunking, proper cleanup. Consider memory management,...
Common bottlenecks: excessive change detection, large bundles, unoptimized images, memory leaks. Identify through...
Material optimization through: selective imports, lazy loading components, proper theming. Consider bundle size...
Zone.js affects change detection performance. Consider zone-less operations, manual change detection. Optimize...
Angular supports: Unit Testing (isolated component/service testing), Integration Testing (component interactions),...
Component testing using TestBed, ComponentFixture. Test isolated logic, template bindings, component interactions....
TestBed is primary API for unit testing. Configures testing module, creates components, provides dependencies....
Service testing through TestBed injection, isolated testing. Mock dependencies, test methods/properties. Use...
Spies mock method behavior, track calls. Created using spyOn(), createSpy(). Track method calls, arguments, return...
HTTP testing using HttpTestingController. Mock requests/responses, verify request parameters. Test success/error...
Async testing handles asynchronous operations. Use async/fakeAsync/waitForAsync helpers. Test promises, observables,...
Directive testing through component creation, DOM manipulation. Test directive behavior, inputs/outputs. Create test...
E2E testing verifies full application flow. Implemented using Protractor/Cypress. Test user scenarios, navigation,...
Pipe testing through isolated tests, transformation verification. Test with different inputs, edge cases. Consider...
Test doubles include: Spies, Stubs, Mocks, Fakes, Dummies. Used for isolating components, controlling dependencies....
Route testing using RouterTestingModule, Location service. Test navigation, route parameters, guards. Mock router...
Code coverage measures tested code percentage. Generated using ng test --code-coverage. Track statements, branches,...
Form testing through ReactiveFormsModule/FormsModule. Test form validation, value changes, submissions. Simulate...
Best practices: arrange-act-assert pattern, single responsibility tests, proper isolation, meaningful descriptions....
Error testing through exception throwing, error handling verification. Test error states, recovery mechanisms....
Integration testing verifies component interactions. Test parent-child communications, service integration. Use...
Observable testing using marbles, fakeAsync. Test stream behavior, error handling. Consider unsubscription, memory...
Shallow testing renders component without children, deep includes child components. Choose based on testing scope,...
NgRx testing through TestStore, mock store. Test actions, reducers, effects separately. Consider state changes,...
Fixtures provide component testing environment. Created using TestBed.createComponent(). Access component instance,...
Lifecycle testing through hook method spies, state verification. Test initialization, destruction logic. Consider...
Snapshot testing compares component output with stored snapshot. Useful for UI regression testing. Consider update...
Custom event testing through EventEmitter simulation, output binding. Test event emission, handler execution....
Common patterns: setup/teardown, data builders, test utilities, shared configurations. Consider reusability,...
Async validator testing through fakeAsync, tick. Mock validation service, test timing. Consider error cases, loading...
Test isolation ensures tests run independently. Reset state between tests, mock dependencies. Consider test order,...
Error boundary testing through error triggering, recovery verification. Test error handling components, fallback UI....
Test harnesses provide component interaction API. Simplify component testing, abstract DOM details. Used in Angular...
XSS attacks inject malicious scripts. Angular prevents by default through automatic sanitization of HTML, style...
Angular includes built-in CSRF/XSRF protection using double-submit cookie pattern. Automatically adds XSRF-TOKEN...
CSP restricts resource loading, prevents attacks. Configure through meta tags or HTTP headers. Affects script...
Authentication through JWT tokens, session management. Implement auth guards, interceptors for token handling....
Sanitization Service prevents XSS by sanitizing values. Handles HTML, styles, URLs, resource URLs. Use...
Secure storage using encryption, HttpOnly cookies. Consider localStorage limitations, session storage. Implement...
Route security through guards, proper navigation. Validate route parameters, implement access control. Consider deep...
Security headers through server configuration, interceptors. Implement HSTS, CSP, X-Frame-Options. Consider browser...
Interceptors add security headers, handle tokens. Implement authentication, request/response transformation....
RBAC through guards, directives, services. Check user roles, permissions. Implement hierarchical roles, component...
Secure coding includes: input validation, output encoding, proper error handling. Avoid dangerous APIs, implement...
Secure transmission through HTTPS, proper encryption. Implement token-based authentication, secure headers. Consider...
DOM-based XSS occurs through client-side JavaScript. Prevent through proper sanitization, avoiding dangerous APIs....
Secure uploads through proper validation, type checking. Implement size limits, scan for malware. Consider storage...
Form security through validation, CSRF protection. Implement proper error handling, input sanitization. Consider...
OAuth implementation through authentication libraries, proper flow. Handle token management, user sessions. Consider...
Same-Origin Policy restricts resource access between origins. Affects AJAX requests, cookies, DOM access. Configure...
Service worker security through proper scope, HTTPS requirement. Implement secure caching, request handling....
WebSocket security through authentication, message validation. Implement secure connection, proper error handling....
Secure state through proper storage, access control. Implement encryption for sensitive data, clear on logout....
Security tools include: npm audit, OWASP ZAP, SonarQube. Regular dependency checking, vulnerability scanning....
Secure sessions through proper timeout, token rotation. Implement session validation, concurrent session handling....
Security testing through penetration testing, vulnerability scanning. Implement security unit tests, integration...
Secure builds through proper configuration, optimization. Enable production mode, implement source map protection....
API security through proper authentication, rate limiting. Implement input validation, error handling. Consider API...
Secure error handling through proper message sanitization, logging. Implement user-friendly messages, avoid...
Security hardening through configuration, best practices. Implement security headers, proper permissions. Consider...
Secure guards through proper authentication, authorization checks. Implement role-based access, navigation control....
PWA security through HTTPS requirement, secure manifests. Implement proper caching strategies, update mechanisms....
Angular CLI is command-line tool for Angular development. Features: project generation, development server, build...
Use 'ng new project-name' command. Configure routing, styling options. Creates project structure, installs...
Schematics are templates for code generation. Create custom generators, modify existing ones. Used by CLI for...
Configuration through angular.json file. Define build options, environments, assets. Configure multiple projects,...
ng serve options: --port (custom port), --open (auto browser), --ssl (HTTPS), --proxy-config (API proxy). Configure...
Environment config through environment.ts files. Define environment-specific variables. Use fileReplacements in...
ng generate creates application elements. Commands for components, services, pipes, etc. Options include: --flat,...
Production optimization through ng build --prod. Enables ahead-of-time compilation, minification, tree shaking....
DevTools browser extension for debugging. Features: component inspection, profiling, state management. Helps with...
CI/CD implementation through build commands, test runners. Configure deployment scripts, environment handling....
Builders customize build process, extend CLI capabilities. Create custom builders for specific needs. Configure in...
Dependency management through ng add, ng update commands. Install Angular-specific packages, update versions....
ng lint checks code quality, style guidelines. Configure rules in tslint.json/eslint.json. Enforce coding standards,...
Custom schematics through @angular-devkit/schematics. Create templates, transformation rules. Test using...
Workspace configs in angular.json define project settings. Configure build options, serve options, test options....
PWA implementation through @angular/pwa package. Use ng add @angular/pwa command. Configure service worker,...
Build configurations define different build settings. Configure in angular.json configurations section. Support...
Bundle analysis through source-map-explorer, webpack-bundle-analyzer. Use ng build --stats-json option. Identify...
Differential loading serves modern/legacy bundles. Enabled by default in new projects. Reduces bundle size for...
Library creation using ng generate library. Configure package.json, public API. Build using ng build library-name....
Asset configuration in angular.json assets array. Define source paths, output paths, glob patterns. Handle static...
i18n implementation through ng xi18n command. Extract messages, configure translations. Support multiple languages,...
CLI proxies configure backend API routing. Define in proxy.conf.json. Handle CORS, development APIs. Important for...
Custom builders extend CLI capabilities. Implement Builder interface, define schema. Test using builder testing...
Project references define project dependencies. Configure in tsconfig.json. Support monorepo setups, shared code....
ESLint integration through @angular-eslint packages. Configure rules, plugins. Replace TSLint (deprecated)....
Deployment commands build production-ready code. Use ng build --prod, configure environments. Consider deployment...
Testing utilities through ng test, ng e2e commands. Configure Karma, Protractor settings. Support unit tests, e2e...
Update strategies using ng update command. Handle dependency updates, migrations. Consider breaking changes,...
Workspace scripts in package.json. Define custom commands, build processes. Support development workflow,...
Components communicate through: @Input/@Output decorators, services, ViewChild/ContentChild, event emitters,...
@Input passes data from parent to child, @Output emits events from child to parent. @Input binds property, @Output...
ViewChild accesses child component/element in template. Provides direct reference to child instance. Used for method...
Services share data between unrelated components. Use observables/subjects for state management. Implement singleton...
EventEmitter emits custom events from child components. Used with @Output decorator. Supports event data passing,...
Sibling communication through shared service, parent component mediation. Use observables/subjects for state...
ContentChild accesses content projection elements. Used with ng-content directive. Access projected content...
Large application communication through state management (NgRx), service layers. Implement proper data flow,...
Subjects are special observables for multicasting. Types: Subject, BehaviorSubject, ReplaySubject. Used in services...
Parent-to-child method calls through ViewChild reference, @Input properties. Consider component lifecycle, method...
Content projection (ng-content) passes content from parent to child. Supports single/multiple slots, conditional...
Event bubbling through host listeners, custom events. Control event propagation, implement handlers. Consider event...
Interaction patterns: mediator, observer, pub/sub patterns. Choose based on component relationships, data flow...
Dynamic component communication through ComponentFactoryResolver, service injection. Handle component creation,...
Change detection updates view based on data changes. Affects @Input property updates, event handling. Consider...
Two-way binding through [(ngModel)] or custom implementation. Combine @Input and @Output. Consider change detection,...
Async pipe handles observables/promises in template. Automatic subscription management, value updates. Important for...
State synchronization through services, observables. Implement proper update mechanisms, handle race conditions....
Lifecycles affect communication timing (ngOnInit, ngOnChanges). Handle initialization, updates, destruction....
Error communication through error events, error services. Implement proper error handling, user feedback. Consider...
Interfaces define component communication contracts. Specify input/output properties, methods. Important for type...
Cross-module communication through shared services, state management. Consider module boundaries, service providers....
Best practices: proper encapsulation, clear interfaces, unidirectional data flow. Consider component responsibility,...
Property changes through ngOnChanges lifecycle hook, setter methods. Implement change detection, update logic....
Dependency injection provides services, shared instances. Manages component dependencies, service scope. Important...
Query parameters through router service, ActivatedRoute. Share state through URL parameters. Consider parameter...
Decorators (@Component, @Input, @Output) configure component behavior. Define metadata, communication interfaces....
Lazy loading communication through service injection, state management. Handle module loading, component...
Anti-patterns: tight coupling, excessive prop drilling, global state abuse. Avoid direct DOM manipulation, complex...
Directives are classes that modify elements. Types: Component directives (with template), Structural directives...
Create using @Directive decorator. Implement logic in class methods. Use ElementRef/Renderer2 for DOM manipulation....
Structural directives modify DOM structure. Examples: *ngIf, *ngFor, *ngSwitch. Use microsyntax with asterisk (*)....
Pure pipes execute only on pure changes (primitive/reference). Impure pipes execute on every change detection. Pure...
Create using @Pipe decorator. Implement PipeTransform interface. Define transform method for conversion logic. Can...
Attribute directives change element appearance/behavior. Examples: ngStyle, ngClass. Can respond to user events,...
Events handled through @HostListener decorator. Respond to DOM events, custom events. Can access event data, element...
Built-in pipes: DatePipe, UpperCasePipe, LowerCasePipe, CurrencyPipe, DecimalPipe, PercentPipe, AsyncPipe, JsonPipe....
Directive composition through multiple directives on element. Handle directive interaction, priority. Consider order...
AsyncPipe unwraps observable/promise values. Automatically handles subscription/unsubscription. Updates view on new...
Custom structural directives use TemplateRef, ViewContainerRef. Create/destroy views programmatically. Handle...
Directive lifecycles: ngOnInit, ngOnDestroy, ngOnChanges, etc. Handle initialization, changes, cleanup. Consider...
Pipe parameters passed after colon in template. Multiple parameters separated by colons. Access in transform method....
Host bindings modify host element properties/attributes. Use @HostBinding decorator. Bind to element properties,...
Pipe chaining connects multiple pipes sequentially. Order matters for transformation. Consider data type...
Exported directives available for template reference. Use exportAs property. Access directive methods/properties in...
Directive dependencies injected through constructor. Access services, other directives. Consider dependency scope,...
Best practices: pure by default, handle null/undefined, proper error handling. Consider performance impact,...
Testing through TestBed configuration, component creation. Test directive behavior, pipe transformations. Consider...
Template context provides data to template. Access through let syntax. Define custom context properties. Important...
Dynamic pipes selected at runtime. Use pipe binding syntax. Consider pipe availability, performance. Important for...
Directive queries access other directives. Use ContentChild/ContentChildren, ViewChild/ViewChildren. Access child...
Pipe errors handled through error handling, default values. Consider null checks, type validation. Provide...
Selector patterns: element, attribute, class selectors. Define directive application scope. Consider naming...
i18n in pipes through locale services, formatting options. Support different formats, languages. Consider cultural...
Common patterns: decorator pattern, adapter pattern, composite pattern. Apply based on use case. Consider...
Optimization through proper change detection, minimal DOM manipulation. Use OnPush strategy when possible. Consider...
Communication through inputs/outputs, services, events. Handle parent-child interaction, sibling communication....
Conflicts resolved through priority, specific selectors. Handle multiple directives, compatibility. Consider...
Key principles include: Modularity (feature modules), Component-based architecture, Dependency Injection, Separation...
Structure using feature modules, core/shared modules, lazy loading. Implement proper folder organization, naming...
Core Module contains singleton services, one-time imported components. Houses app-wide services, guards,...
Feature modules organize related components, services, pipes. Support lazy loading, encapsulation. Improve...
Smart components handle business logic, data. Presentational components focus on UI, receive data via inputs....
Shared Module contains common components, pipes, directives. Imported by feature modules. Avoids code duplication,...
State management through services (small apps) or NgRx/other state libraries (large apps). Consider data flow, state...
Service architecture: proper separation, single responsibility, injectable services. Consider service scope,...
DDD through feature modules, domain services, entities. Organize by business domains. Consider bounded contexts,...
Communication patterns: Input/Output, Services, State Management, Event Bus. Choose based on component relationship,...
Error handling through ErrorHandler service, HTTP interceptors. Implement centralized error logging, user feedback....
API integration: service layer abstraction, typed interfaces, proper error handling. Implement caching, retry...
Micro-frontends through Module Federation, Custom Elements. Handle inter-app communication, shared dependencies....
Form patterns: Template-driven vs Reactive forms, form builders, validation services. Consider form complexity,...
Security practices: XSS prevention, CSRF protection, secure authentication. Implement proper authorization, input...
Route patterns: feature-based routing, guard protection, resolvers. Implement proper navigation, data preloading....
Performance optimization: lazy loading, change detection strategy, bundle optimization. Implement proper caching,...
Testing architecture: unit tests, integration tests, e2e tests. Implement proper test organization, coverage....
i18n architecture: translation files, language services, locale handling. Implement proper text extraction, runtime...
Auth patterns: JWT handling, guard protection, role-based access. Implement secure token storage, refresh...
Component libraries: shared styles, documentation, versioning. Implement proper encapsulation, API design. Consider...
Dependency management: proper versioning, package organization, update strategy. Implement dependency injection,...
Logging architecture: centralized logging, error tracking, monitoring. Implement proper log levels, storage...
Offline patterns: service workers, local storage, sync mechanisms. Implement proper caching, data persistence....
CI/CD practices: automated testing, build optimization, deployment strategy. Implement proper environment...
Code organization: proper folder structure, naming conventions, module organization. Implement feature-based...
Accessibility practices: ARIA labels, keyboard navigation, screen reader support. Implement proper semantic markup,...
Real-time patterns: WebSocket integration, polling strategies, state updates. Implement proper connection...
Progressive enhancement: feature detection, fallback strategies, graceful degradation. Implement proper browser...
Global error handling through ErrorHandler class. Override handleError method for custom handling. Catches all...
HTTP errors handled through interceptors, catchError operator. Implement retry logic, error transformation. Use...
Error boundaries through component error handlers, ngOnError hook. Catch and handle rendering errors. Implement...
Form errors through validators, error states. Display validation messages, highlight errors. Implement custom...
RxJS operators: catchError, retry, retryWhen, throwError. Handle stream errors, implement recovery logic. Consider...
Error logging through centralized service. Log error details, stack traces. Implement remote logging, error...
Best practices: centralized handling, proper error types, user feedback. Implement logging, monitoring. Consider...
Async errors through Promise catch, Observable error handling. Implement proper error propagation, recovery....
Error notifications through toasts, alerts, error pages. Implement user-friendly messages, action options. Consider...
Navigation errors through Router error events, guards. Implement redirection, error pages. Consider route...
Recovery patterns: retry logic, fallback options, graceful degradation. Implement automatic recovery, manual...
Error tracking through monitoring services, custom tracking. Implement error aggregation, analysis. Consider error...
Prevention strategies: input validation, type checking, defensive programming. Implement proper error boundaries,...
Memory leak prevention through proper unsubscription, cleanup. Implement resource management, leak detection....
Monitoring tools: Sentry, LogRocket, custom solutions. Implement error tracking, performance monitoring. Consider...
Library errors through error wrapping, custom handlers. Implement proper integration, error transformation. Consider...
Error testing through unit tests, integration tests. Test error scenarios, recovery logic. Consider error...
Production errors through logging, monitoring, alerts. Implement error reporting, analysis. Consider environment...
Error serialization for logging, transmission. Implement proper error format, data extraction. Consider sensitive...
Offline errors through connection checking, retry logic. Implement offline storage, sync mechanisms. Consider...
Boundary patterns: component-level, route-level, app-level boundaries. Implement proper error isolation, recovery....
State errors through error actions, error states. Implement state recovery, rollback mechanisms. Consider state...
Anti-patterns: swallowing errors, generic error messages, missing logging. Avoid broad catch blocks, silent...
Initialization errors through APP_INITIALIZER, bootstrap handling. Implement proper startup checks, fallbacks....
Reporting practices: proper error format, context inclusion, aggregation. Implement error categorization, priority...
Security errors through proper validation, authorization checks. Implement secure error messages, logging. Consider...
Documentation practices: error codes, handling procedures, recovery steps. Implement proper error guides,...
Performance errors through monitoring, optimization. Implement performance boundaries, error tracking. Consider...
Error metrics: error rates, recovery times, impact assessment. Implement metric collection, analysis. Consider...
DI errors through proper provider configuration, error catching. Implement fallback providers, error messages....
Essential tools include: Angular DevTools, Chrome DevTools, Source Maps, Augury (legacy), console methods, debugger...
Debug change detection using: enableDebugTools(), profiler, zones visualization. Check for unnecessary triggers,...
Debug memory leaks using: Chrome Memory profiler, heap snapshots, timeline recording. Check subscription cleanup,...
Debug routing through Router events logging, Router state inspection. Check guard conditions, route configurations....
Performance debugging using: Chrome Performance tools, Angular profiler, network tab. Analyze bundle sizes,...
Debug HTTP through Network tab, HttpInterceptors logging. Monitor request/response cycles, analyze headers/payloads....
Production debugging through: error logging services, source maps, monitoring tools. Implement proper error...
Debug DI through provider configuration checks, injection tree analysis. Verify service providers, scope issues....
Template debugging through: browser inspection, Angular DevTools. Check bindings, expressions, structural...
State debugging through: Redux DevTools, state logging, action tracing. Monitor state changes, action effects....
Async debugging through: async/await breakpoints, observable debugging. Monitor promise chains, subscription flows....
Form debugging through form state inspection, validation checks. Monitor form controls, error states. Analyze...
Build issues debugging through: build logs, configuration checks. Verify dependencies, version conflicts. Analyze...
Lazy loading debugging through: network monitoring, chunk loading analysis. Check module configurations, route...
Component interaction debugging through: input/output tracking, service communication monitoring. Check component...
AOT debugging through: compilation errors analysis, template checking. Verify syntax compatibility, type checking....
Security debugging through: CORS analysis, XSS checks, authentication flow verification. Monitor security headers,...
SSR debugging through: server logs, hydration checks. Verify platform-specific code, state transfer. Analyze...
Zone.js debugging through: zone hooks monitoring, async operation tracking. Check zone contexts, patched methods....
i18n debugging through: translation key checks, locale loading verification. Monitor translation process, cultural...
Optimization debugging through: bundle analysis, performance profiling. Check optimization configurations,...
PWA debugging through: service worker inspection, cache analysis. Verify manifest configuration, offline behavior....
Test debugging through: test runner inspection, assertion analysis. Check test setup, mock configurations. Analyze...
Accessibility debugging through: ARIA validation, screen reader testing. Check semantic markup, keyboard navigation....
CSS debugging through: style inspection, layout analysis. Check style encapsulation, view encapsulation. Analyze...
WebSocket debugging through: connection state monitoring, message logging. Verify connection lifecycle, error...
Library debugging through: integration verification, API usage analysis. Check compatibility issues, version...
Environment debugging through: configuration file analysis, variable checking. Verify environment setup, build...
Browser compatibility debugging through: cross-browser testing, feature detection. Check polyfills, browser-specific...
Angular CLI build process includes: compilation, bundling, minification, tree-shaking. Uses webpack under the hood....
Environments configured through environment.ts files. Create environment-specific files, use fileReplacements in...
AOT compiles templates during build process. Benefits: faster rendering, smaller file size, better security. Default...
CI/CD implementation through pipelines (Jenkins, GitLab CI, etc.). Include build, test, deploy stages. Configure...
Optimization techniques: bundle splitting, lazy loading, tree shaking, compression. Configure production build...
Deployment configurations through environment files, runtime configuration. Handle API endpoints, feature flags,...
Webpack handles module bundling, asset management, dependency resolution. Configures loaders, plugins for build...
Versioning through semantic versioning, automated version bumping. Configure version management in package.json, git...
Deployment strategies: blue-green deployment, canary releases, rolling updates. Consider zero-downtime deployment,...
Docker implementation through multi-stage builds, optimized images. Configure Nginx for serving, environment...
Production considerations: optimization flags, environment configuration, security measures. Enable production mode,...
Asset handling through angular.json configuration, CDN integration. Optimize images, manage static files. Consider...
SSR deployment using Angular Universal, server configuration. Handle server-side execution, state transfer. Consider...
PWA deployment through service worker configuration, manifest setup. Handle offline capabilities, caching...
Automation tools: Jenkins, GitLab CI, GitHub Actions, Azure DevOps. Configure build pipelines, deployment scripts....
Dependency management through package-lock.json, npm/yarn. Handle version conflicts, security updates. Consider...
Artifact management through artifact repositories, versioning system. Handle storage, distribution of builds....
Rolling updates through deployment orchestration, version management. Handle traffic routing, health checks....
Monitoring through logging services, performance tracking. Implement error tracking, user analytics. Consider...
Configuration management through environment files, runtime config. Implement secure storage, access control....
Optimization flags control build process features. Include production mode, bundling options, optimization levels....
Multi-environment through environment-specific configurations, deployment pipelines. Handle different settings, API...
Security considerations: HTTPS setup, CSP configuration, secure headers. Implement access controls, security...
Rollbacks through version control, deployment history. Implement automated rollback procedures. Consider data...
Build performance through caching, parallel processing, incremental builds. Optimize build configuration, dependency...
Feature flags through configuration management, runtime toggles. Handle feature enabling/disabling, A/B testing....
Deployment testing through smoke tests, integration tests. Implement automated validation, environment verification....
Database migrations through versioning, rollback support. Implement migration scripts, data validation. Consider...
Documentation practices: deployment procedures, configuration guides, troubleshooting steps. Maintain version...
Cache management through versioning, cache busting strategies. Handle browser caching, CDN configuration. Consider...
Angular is a TypeScript-based open-source framework for building web applications. Key differences from AngularJS include: component-based architecture instead of MVC, better performance with improved change detection, TypeScript usage, improved dependency injection, and modular development approach.
Decorators are design patterns that modify JavaScript classes. Common Angular decorators include: @Component (defines component), @Injectable (defines service), @NgModule (defines module), @Input/@Output (property binding), @HostListener (DOM event binding), and @ViewChild (child component reference).
NgModule is a decorator that defines a module in Angular. Essential properties include: declarations (components, directives, pipes), imports (other modules), exports (shared declarations), providers (services), and bootstrap (root component). It helps organize application into cohesive blocks of functionality.
Dependency Injection is a design pattern where dependencies are provided to classes instead of creating them. Angular's DI system uses @Injectable decorator, providers array, and hierarchical injector tree. It manages object creation, lifetime, and dependencies, promoting loose coupling and testability.
Lifecycle hooks are methods that Angular calls on components/directives at specific moments. Major hooks: ngOnInit (after first ngOnChanges), ngOnChanges (when data-bound property changes), ngOnDestroy (before destruction), ngDoCheck (during change detection), ngAfterViewInit (after view initialization).
Constructor is a TypeScript feature called when class is instantiated, used for dependency injection. ngOnInit is an Angular lifecycle hook called after data-bound properties are initialized. Best practice: use constructor for DI setup, ngOnInit for initialization logic.
Change detection is Angular's process of synchronizing component model and view. Uses Zone.js to track async operations. Two strategies: Default (checks entire tree) and OnPush (only checks on reference changes). Can be optimized using immutability and proper component architecture.
Data binding synchronizes component and view. Types: Interpolation {{data}}, Property binding [property], Event binding (event), Two-way binding [(ngModel)]. Enables automatic sync between model and view, reducing manual DOM manipulation.
ViewChild/ViewChildren decorators provide access to child elements in component template. ViewChild returns single element, ViewChildren returns QueryList. Used for accessing child components, directives, or DOM elements. Important for component interaction.
Services are singleton objects used for sharing data/functionality across components. Marked with @Injectable decorator. Used for: data sharing, business logic, external interactions (HTTP calls). Promotes DRY principle and separation of concerns.
providedIn configures service scope in @Injectable decorator (root, platform, any, specific module). providers array in @NgModule registers services at module level. providedIn enables tree-shaking, preferred for service registration in modern Angular.
Templates define component's view in HTML with Angular-specific syntax. Features: data binding, directives, pipes, template expressions, template statements. Supports structural directives (*ngIf, *ngFor), event binding, and property binding.
Ahead-of-Time compilation compiles Angular application at build time. Benefits: faster rendering, smaller download size, earlier template error detection, better security. Default in production builds since Angular 9.
Angular DI uses hierarchical injector tree: NullInjector (root), Platform, Module, Element (component/directive). Child injectors inherit from parents. Enables service scope control, instance sharing, and override mechanisms.
Template reference variables (#var) create references to DOM elements, components, or directives in template. Used for direct access in template or component class via ViewChild. Useful for form handling and component interaction.
Zone.js provides execution context tracking for async operations. Angular uses it for automatic change detection by patching async operations. Enables detection of state changes from events, HTTP, timers. Critical for Angular's change detection system.
declarations list components/directives/pipes belonging to module. imports list other modules needed. providers list services for dependency injection. declarations must be unique across app, imports can be shared, providers affect dependency injection scope.
Angular elements are Angular components packaged as custom elements (Web Components). Use cases: embedding Angular components in non-Angular applications, micro-frontends, widget development. Enables framework-agnostic component reuse.
Methods include: @Input/@Output decorators, services (state management), ViewChild/ContentChild, event emitters, subject/behavior subject, parent-child interaction. Choose based on component relationship and data flow requirements.
Angular CLI uses webpack for bundling. Lazy loading implemented through RouterModule with loadChildren syntax. Reduces initial bundle size, improves load time. Modules loaded on-demand when route accessed.
Async pipe automatically subscribes/unsubscribes to observables/promises in templates. Handles subscription lifecycle, prevents memory leaks. Useful for handling async data in templates without manual subscription management.
HostBinding decorates properties to bind to host element properties. HostListener decorates methods to handle host element events. Used in directives/components for DOM interaction without direct manipulation.
Error handling through: try-catch blocks, RxJS catchError operator, HTTP interceptors, ErrorHandler class, Router error handling. No direct equivalent to React error boundaries. Global error handling configured in module providers.
ViewEncapsulation controls component CSS encapsulation. Types: Emulated (default, scoped CSS), None (global CSS), ShadowDom (true shadow DOM). Affects how component styles are applied and scoped in application.
Schematics are templates for generating/modifying code. Used by Angular CLI for scaffolding components, services, etc. Can create custom schematics for project-specific generators. Supports workspace modifications.
Component styles defined in styles/styleUrls properties. Supports CSS encapsulation through ViewEncapsulation. Can use preprocessors (SCSS/SASS), CSS variables, global styles. Styles scoped to component by default.
NgZone service provides access to Angular's zone for executing work inside/outside Angular's zone. Used for performance optimization, manual change detection control. Important for integrating with third-party libraries.
Angular i18n uses XLF/XLIFF files for translations. Supports: text extraction, pluralization, date/number formatting, runtime language switching. Built-in i18n pipes, can use ngx-translate library for dynamic translations.
Angular has Template-driven forms (simple, directive based, async validation) and Reactive forms (complex, explicit, synchronous validation). Template-driven forms use two-way binding with ngModel, while Reactive forms use FormGroup/FormControl with explicit form model.
Reactive form validation uses validators in FormControl/FormGroup construction. Built-in validators (required, minLength, pattern) or custom validators. Status and error states tracked through form control properties. Synchronous and async validation supported.
FormControl tracks value/validation of individual form field. FormGroup groups related controls together, tracks aggregate value/status. Both provide value changes observables, validation state, methods for value updates.
Custom validators are functions returning validation errors or null. Can be synchronous (ValidatorFn) or asynchronous (AsyncValidatorFn). Implement for complex validation logic. Can access external services, combine multiple validations.
FormArray manages collection of form controls/groups dynamically. Used for variable-length form fields (dynamic forms, multiple items). Provides array-like API for manipulation. Common in dynamic questionnaires, product lists.
Form submission handled through ngSubmit event or form's submit method. Implement validation checks, disable submit during processing, handle success/error responses. Consider loading states, user feedback, form reset.
Form states include: pristine/dirty, touched/untouched, valid/invalid, pending. Tracked through form control properties and CSS classes. Used for validation feedback, UI updates. Available at control and form level.
Cross-field validation implemented through form group validators. Access multiple control values, compare fields (password confirmation). Can use custom validators at group level. Important for related field validation.
Async validators perform validation asynchronously (API calls, database checks). Return Promise/Observable. Used for unique email/username validation. Handle pending state, implement debounce time. Consider error handling.
Forms reset using reset() method on FormGroup/FormControl. Can provide initial values, reset specific controls. Resets both values and validation states. Consider UI feedback, data preservation needs.
FormBuilder service provides syntactic sugar for creating form controls. Simplifies complex form creation, reduces boilerplate. Methods include control(), group(), array(). Improves code readability and maintenance.
File uploads handled through input type='file' and FormData. Use change event to capture files, implement validation (size, type). Consider progress tracking, multiple file handling, chunked uploads.
valueChanges and statusChanges are observables tracking form changes. valueChanges emits new values, statusChanges emits validation status. Used for reactive form updates, validation feedback. Support filtering, debouncing.
Conditional validation changes validation rules based on conditions. Implement through setValidators(), clearValidators(). Update validators dynamically based on form values/state. Consider validation dependencies.
Form control wrappers are custom components wrapping form controls. Provide reusable validation, styling, behavior. Implement ControlValueAccessor interface. Common for custom input components, complex controls.
Form arrays validation applies at array and item levels. Validate array length, individual controls. Can implement custom validators for array-specific rules. Consider dynamic validation updates, error aggregation.
updateOn controls when form control updates (change, blur, submit). Affects validation timing, value updates. Configurable at control/group level. Important for performance, user experience optimization.
Custom error messages handled through error states in template. Access errors through control.errors property. Can use error message service, translation support. Consider message formatting, multiple errors.
Best practices: immediate feedback, clear error messages, consistent validation, proper error states, accessibility support. Consider user experience, performance, maintainability. Implement proper error handling.
Dynamic forms built using form arrays/groups, metadata-driven approach. Generate controls from configuration/data. Consider validation rules, field dependencies. Important for configurable forms, surveys.
ngModel provides two-way data binding in template-driven forms. Creates form controls implicitly, tracks value/validation state. Requires FormsModule. Limited compared to reactive forms' explicit control.
Form submission errors handled through error callbacks, error states. Display user-friendly messages, highlight affected fields. Consider error types, recovery options. Implement proper error handling patterns.
Nested form groups organize related controls hierarchically. Created using FormBuilder.group() nesting. Support complex form structures, modular validation. Common in address forms, complex data entry.
Validation messages implemented through template logic, error states. Can use validation message service, localization support. Consider message context, multiple languages. Important for user feedback.
Dirty checking tracks form value changes. pristine/dirty states indicate modifications. Used for save prompts, reset functionality. Important for tracking user interactions, form state management.
Form array validation patterns include: min/max items, unique values, dependent validations. Implement through custom validators, array-level validation. Consider complex validation scenarios, error aggregation.
Common patterns: required fields, email format, password strength, numeric ranges, custom regex. Implement through built-in/custom validators. Consider user experience, security requirements.
Form state persistence through services, local storage, state management. Consider autosave functionality, recovery options. Important for long forms, multi-step processes. Implement proper cleanup.
Form control events include: valueChanges, statusChanges, focus/blur events. Handle through event bindings, observables. Used for validation feedback, dependent updates. Consider event ordering, performance.
Multi-step validation through form groups per step, wizard pattern. Validate each step, track completion status. Consider state management, navigation rules. Important for complex workflows.
HttpClient is Angular's built-in HTTP client. Features: typed responses, observables for requests, interceptors support, progress events, error handling. Provides methods for HTTP operations (get, post, put, delete). Supports request/response transformation.
Interceptors intercept and modify HTTP requests/responses. Use cases: authentication headers, error handling, loading indicators, caching, logging. Implement HttpInterceptor interface. Can be chained, order matters.
Error handling through catchError operator, error interceptors. Implement global/local error handlers, retry logic. Consider error types (network, server, client), user feedback. Use ErrorHandler class for global handling.
Best practices: separate service layer, typed interfaces, proper error handling, environment configuration. Consider request caching, retry strategies, cancellation. Implement proper separation of concerns.
Caching implemented through interceptors, services. Use shareReplay operator, cache storage. Consider cache invalidation, freshness checks. Important for performance optimization, offline support.
HttpParams manage URL parameters in requests. Immutable object for query parameters. Methods: set(), append(), delete(). Important for API queries, filtering. Consider parameter encoding, multiple values.
File uploads using FormData, multipart/form-data content type. Support progress events, cancellation. Consider file size limits, validation. Implement proper error handling, progress tracking.
Request cancellation using takeUntil operator, AbortController. Implement cleanup on component destruction. Important for preventing memory leaks, unnecessary requests. Consider loading states.
Authentication through interceptors, auth headers. Implement token management, refresh logic. Consider session handling, secure storage. Important for secured APIs, user sessions.
Headers managed through HttpHeaders class. Set content type, authorization, custom headers. Immutable object, chainable methods. Consider CORS, security headers. Important for API communication.
Retry logic using retry/retryWhen operators. Configure retry count, delay. Consider error types, backoff strategy. Important for handling temporary failures, network issues.
Progress events track upload/download progress. Use reportProgress option, HttpEventType. Implement progress indicators, cancellation. Important for large file operations, user feedback.
Concurrent requests using forkJoin, combineLatest operators. Consider error handling, loading states. Important for dependent data, parallel operations. Implement proper request management.
CORS (Cross-Origin Resource Sharing) handled through server configuration, proxy settings. Configure allowed origins, methods, headers. Consider security implications, browser restrictions. Important for cross-domain requests.
API versioning through URL prefixes, headers, interceptors. Configure base URLs, version management. Consider backward compatibility, migration strategy. Important for API evolution.
Timeouts configured using timeout operator, request configuration. Consider network conditions, server response time. Important for user experience, error handling. Implement proper feedback.
Offline support through service workers, local storage. Implement queue system, sync logic. Consider conflict resolution, data persistence. Important for offline-first applications.
Response types: json, text, blob, arrayBuffer. Configure using responseType option. Consider data format, transformation needs. Important for different content types, file downloads.
API mocking through interceptors, mock services. Configure development environment, test data. Consider realistic scenarios, error cases. Important for development, testing.
Security considerations: XSS prevention, CSRF protection, secure headers. Implement authentication, authorization. Consider data encryption, input validation. Important for application security.
Large data handling through pagination, infinite scroll. Implement virtual scrolling, data chunking. Consider performance impact, memory usage. Important for scalable applications.
Debouncing delays request execution until pause in triggering. Implement using debounceTime operator. Consider user input, search functionality. Important for performance optimization.
Error mapping through interceptors, error services. Transform server errors to application format. Consider error categorization, localization. Important for consistent error handling.
WebSockets enable real-time communication. Implement using socket.io, custom services. Consider connection management, reconnection logic. Important for real-time features.
Rate limiting through interceptors, request queuing. Implement backoff strategy, request prioritization. Consider server limits, user experience. Important for API consumption.
Transformation through interceptors, map operator. Modify request/response data format. Consider data consistency, type safety. Important for API integration.
Request queueing through custom services, RxJS operators. Handle sequential requests, priorities. Consider error handling, cancellation. Important for dependent operations.
Testing practices: unit tests for services, mock interceptors, integration tests. Consider error scenarios, async testing. Important for reliability, maintenance.
Documentation through Swagger/OpenAPI, custom documentation. Generate TypeScript interfaces, API services. Consider versioning, maintenance. Important for development workflow.
Change Detection determines UI updates based on data changes. Default strategy checks all components, OnPush checks only on reference changes. Improper implementation can cause performance issues. Optimize using immutable objects, pure pipes, OnPush strategy.
Lazy Loading loads modules on demand, reducing initial bundle size. Implemented through routing configuration using loadChildren. Improves initial load time, reduces memory usage. Consider module boundaries, preloading strategies.
Ahead-of-Time compilation compiles templates during build. Benefits: faster rendering, smaller payload, earlier error detection. Default in production builds. Eliminates need for template compiler in runtime.
Memory optimization through: proper unsubscription from observables, avoiding memory leaks, cleaning up event listeners, implementing trackBy for ngFor. Consider component lifecycle, large data handling.
trackBy provides unique identifier for items in ngFor. Helps Angular track items efficiently, reduces DOM operations. Improves performance with large lists, frequent updates. Customize based on data structure.
Virtual scrolling renders only visible items using ScrollingModule. Improves performance with large lists. Configure through CDK virtual scroll viewport. Consider item size, buffer size, scroll strategy.
Pure pipes execute only on input changes, impure on every change detection. Pure pipes better for performance, cache results. Use impure pipes sparingly. Consider transformation complexity, update frequency.
Bundle optimization through: tree shaking, lazy loading, proper imports, removing unused dependencies. Use built-in optimization flags, analyze bundles. Consider code splitting, dynamic imports.
Tree Shaking removes unused code from final bundle. Enabled by default in production builds. Requires proper module imports, side-effect free code. Important for reducing application size.
Caching implemented through services, HTTP interceptors. Cache API responses, computed values, static assets. Consider cache invalidation, storage limits. Important for reducing server requests.
Web Workers run scripts in background threads. Offload heavy computations, improve responsiveness. Implement using Angular CLI generation. Consider data transfer overhead, use cases.
Form optimization through: proper validation timing, minimal form controls, efficient value updates. Consider updateOn option, form structure. Important for complex forms, frequent updates.
enableProdMode disables development checks and warnings. Improves performance in production. Call before bootstrapping application. Important for production deployments.
Preloading loads modules after initial load. Configure through PreloadAllModules or custom strategy. Balance between performance and resource usage. Consider user patterns, network conditions.
Best practices: proper change detection, lazy loading, AOT compilation, bundle optimization, caching implementation. Regular performance audits, monitoring. Consider user experience, metrics.
SSR using Angular Universal. Improves initial load, SEO. Configure through Angular CLI commands. Consider hydration, state transfer. Important for SEO-critical applications.
Differential loading serves modern/legacy bundles based on browser support. Reduces bundle size for modern browsers. Enabled by default in new projects. Consider browser support requirements.
Image optimization through: lazy loading, proper sizing, format selection, CDN usage. Implement loading attribute, responsive images. Consider bandwidth usage, caching strategies.
Ivy is Angular's rendering engine. Benefits: smaller bundles, faster compilation, better debugging. Default since Angular 9. Enables tree-shakable components, improved build optimization.
Performance monitoring through: browser dev tools, Angular profiler, custom metrics. Track key indicators, implement logging. Consider user metrics, error tracking. Important for continuous optimization.
Performance budgets set size limits for bundles. Configure in angular.json. Enforce during build process. Consider different types (bundle, initial), thresholds. Important for maintaining performance.
Router optimization through: preloading strategies, route guards optimization, minimal route data. Consider navigation timing, caching. Important for smooth user experience.
Code splitting divides code into smaller chunks. Improves initial load time, enables lazy loading. Implement through route-level splitting, dynamic imports. Consider bundle size, loading strategy.
Library optimization through: selective imports, lazy loading, alternatives evaluation. Consider bundle impact, necessity. Important for maintaining lean application.
Service workers enable offline capabilities, caching. Improve load times, reduce server load. Configure through Angular PWA package. Consider cache strategies, update flow.
Efficient infinite scrolling through: virtual scrolling, data chunking, proper cleanup. Consider memory management, loading states. Important for large data sets.
Common bottlenecks: excessive change detection, large bundles, unoptimized images, memory leaks. Identify through profiling, monitoring. Consider regular performance audits.
Material optimization through: selective imports, lazy loading components, proper theming. Consider bundle size impact, usage patterns. Important for Material-based applications.
Zone.js affects change detection performance. Consider zone-less operations, manual change detection. Optimize through proper zone usage, async operations. Important for application responsiveness.
Angular supports: Unit Testing (isolated component/service testing), Integration Testing (component interactions), E2E Testing (full application flow). Uses Jasmine framework, Karma test runner, Protractor/Cypress for E2E.
Component testing using TestBed, ComponentFixture. Test isolated logic, template bindings, component interactions. Mock dependencies, simulate events. Use shallow/deep rendering based on needs.
TestBed is primary API for unit testing. Configures testing module, creates components, provides dependencies. Methods include configureTestingModule(), createComponent(). Essential for component/service testing.
Service testing through TestBed injection, isolated testing. Mock dependencies, test methods/properties. Use dependency injection, spies for external services. Test async operations using fakeAsync/tick.
Spies mock method behavior, track calls. Created using spyOn(), createSpy(). Track method calls, arguments, return values. Essential for mocking dependencies, verifying interactions.
HTTP testing using HttpTestingController. Mock requests/responses, verify request parameters. Test success/error scenarios, multiple requests. Important for API integration testing.
Async testing handles asynchronous operations. Use async/fakeAsync/waitForAsync helpers. Test promises, observables, timers. Important for testing real-world scenarios.
Directive testing through component creation, DOM manipulation. Test directive behavior, inputs/outputs. Create test host components. Consider element interactions, lifecycle hooks.
E2E testing verifies full application flow. Implemented using Protractor/Cypress. Test user scenarios, navigation, interactions. Important for regression testing, feature verification.
Pipe testing through isolated tests, transformation verification. Test with different inputs, edge cases. Consider pure/impure pipes, parameter handling. Simple to test due to pure function nature.
Test doubles include: Spies, Stubs, Mocks, Fakes, Dummies. Used for isolating components, controlling dependencies. Choose based on testing needs, complexity. Important for unit testing.
Route testing using RouterTestingModule, Location service. Test navigation, route parameters, guards. Mock router service, verify navigation calls. Important for application flow testing.
Code coverage measures tested code percentage. Generated using ng test --code-coverage. Track statements, branches, functions coverage. Important for quality assurance, identifying untested code.
Form testing through ReactiveFormsModule/FormsModule. Test form validation, value changes, submissions. Simulate user input, verify form state. Consider complex validation scenarios.
Best practices: arrange-act-assert pattern, single responsibility tests, proper isolation, meaningful descriptions. Consider test maintainability, readability. Follow testing pyramid principles.
Error testing through exception throwing, error handling verification. Test error states, recovery mechanisms. Consider different error types, user feedback. Important for robustness.
Integration testing verifies component interactions. Test parent-child communications, service integration. Use TestBed for configuration, fixture for interaction. Consider component relationships.
Observable testing using marbles, fakeAsync. Test stream behavior, error handling. Consider unsubscription, memory leaks. Important for reactive programming testing.
Shallow testing renders component without children, deep includes child components. Choose based on testing scope, complexity. Consider component dependencies, testing goals.
NgRx testing through TestStore, mock store. Test actions, reducers, effects separately. Consider state changes, async operations. Important for state management testing.
Fixtures provide component testing environment. Created using TestBed.createComponent(). Access component instance, debug element. Essential for component testing, DOM interaction.
Lifecycle testing through hook method spies, state verification. Test initialization, destruction logic. Consider timing, dependencies. Important for component behavior testing.
Snapshot testing compares component output with stored snapshot. Useful for UI regression testing. Consider update process, maintenance. Important for template stability.
Custom event testing through EventEmitter simulation, output binding. Test event emission, handler execution. Consider event payload, timing. Important for component interaction.
Common patterns: setup/teardown, data builders, test utilities, shared configurations. Consider reusability, maintenance. Important for testing efficiency, consistency.
Async validator testing through fakeAsync, tick. Mock validation service, test timing. Consider error cases, loading states. Important for form validation testing.
Test isolation ensures tests run independently. Reset state between tests, mock dependencies. Consider test order, shared resources. Important for reliable testing.
Error boundary testing through error triggering, recovery verification. Test error handling components, fallback UI. Consider different error scenarios, user experience.
Test harnesses provide component interaction API. Simplify component testing, abstract DOM details. Used in Angular Material testing. Important for complex component testing.
XSS attacks inject malicious scripts. Angular prevents by default through automatic sanitization of HTML, style bindings. Use DomSanitizer for trusted content, avoid bypass methods. Implement Content Security Policy (CSP).
Angular includes built-in CSRF/XSRF protection using double-submit cookie pattern. Automatically adds XSRF-TOKEN cookie to requests. Configure through HttpClientXsrfModule. Server must support token validation.
CSP restricts resource loading, prevents attacks. Configure through meta tags or HTTP headers. Affects script execution, style loading, image sources. Consider inline styles/scripts restrictions.
Authentication through JWT tokens, session management. Implement auth guards, interceptors for token handling. Secure token storage, implement refresh mechanism. Consider OAuth integration.
Sanitization Service prevents XSS by sanitizing values. Handles HTML, styles, URLs, resource URLs. Use bypassSecurityTrustHtml for trusted content. Important for dynamic content rendering.
Secure storage using encryption, HttpOnly cookies. Consider localStorage limitations, session storage. Implement secure token management. Important for sensitive data protection.
Route security through guards, proper navigation. Validate route parameters, implement access control. Consider deep linking security, route resolvers. Important for navigation security.
Security headers through server configuration, interceptors. Implement HSTS, CSP, X-Frame-Options. Consider browser compatibility, header requirements. Important for transport security.
Interceptors add security headers, handle tokens. Implement authentication, request/response transformation. Consider error handling, retry logic. Important for API security.
RBAC through guards, directives, services. Check user roles, permissions. Implement hierarchical roles, component visibility. Important for access management.
Secure coding includes: input validation, output encoding, proper error handling. Avoid dangerous APIs, implement security controls. Consider secure defaults, code review.
Secure transmission through HTTPS, proper encryption. Implement token-based authentication, secure headers. Consider data minimization, transport security. Important for data protection.
DOM-based XSS occurs through client-side JavaScript. Prevent through proper sanitization, avoiding dangerous APIs. Use Angular's built-in protections, validate user input. Consider template security.
Secure uploads through proper validation, type checking. Implement size limits, scan for malware. Consider storage location, access control. Important for upload security.
Form security through validation, CSRF protection. Implement proper error handling, input sanitization. Consider client/server validation, secure submission. Important for user input.
OAuth implementation through authentication libraries, proper flow. Handle token management, user sessions. Consider security best practices, implementation standards.
Same-Origin Policy restricts resource access between origins. Affects AJAX requests, cookies, DOM access. Configure CORS for cross-origin requests. Important for application security.
Service worker security through proper scope, HTTPS requirement. Implement secure caching, request handling. Consider update mechanism, cache poisoning prevention.
WebSocket security through authentication, message validation. Implement secure connection, proper error handling. Consider connection timeout, protocol security.
Secure state through proper storage, access control. Implement encryption for sensitive data, clear on logout. Consider state persistence, security implications.
Security tools include: npm audit, OWASP ZAP, SonarQube. Regular dependency checking, vulnerability scanning. Consider automation, continuous monitoring.
Secure sessions through proper timeout, token rotation. Implement session validation, concurrent session handling. Consider session fixation prevention.
Security testing through penetration testing, vulnerability scanning. Implement security unit tests, integration tests. Consider OWASP guidelines, security requirements.
Secure builds through proper configuration, optimization. Enable production mode, implement source map protection. Consider build optimization, security flags.
API security through proper authentication, rate limiting. Implement input validation, error handling. Consider API versioning, documentation security.
Secure error handling through proper message sanitization, logging. Implement user-friendly messages, avoid sensitive information. Consider error tracking, monitoring.
Security hardening through configuration, best practices. Implement security headers, proper permissions. Consider environment security, deployment practices.
Secure guards through proper authentication, authorization checks. Implement role-based access, navigation control. Consider guard composition, reusability.
PWA security through HTTPS requirement, secure manifests. Implement proper caching strategies, update mechanisms. Consider offline security, service worker security.
Angular CLI is command-line tool for Angular development. Features: project generation, development server, build optimization, code generation, testing utilities. Provides commands for common development tasks, project scaffolding.
Use 'ng new project-name' command. Configure routing, styling options. Creates project structure, installs dependencies. Options include: --routing, --style, --skip-tests, --strict. Consider project requirements.
Schematics are templates for code generation. Create custom generators, modify existing ones. Used by CLI for component/service generation. Support project-specific templates.
Configuration through angular.json file. Define build options, environments, assets. Configure multiple projects, shared settings. Important for project customization.
ng serve options: --port (custom port), --open (auto browser), --ssl (HTTPS), --proxy-config (API proxy). Configure development server behavior. Important for local development.
Environment config through environment.ts files. Define environment-specific variables. Use fileReplacements in angular.json. Important for different deployment scenarios.
ng generate creates application elements. Commands for components, services, pipes, etc. Options include: --flat, --skip-tests, --module. Supports custom schematics.
Production optimization through ng build --prod. Enables ahead-of-time compilation, minification, tree shaking. Configure build options in angular.json. Consider performance budgets.
DevTools browser extension for debugging. Features: component inspection, profiling, state management. Helps with performance analysis, debugging. Important for development workflow.
CI/CD implementation through build commands, test runners. Configure deployment scripts, environment handling. Consider build optimization, testing automation.
Builders customize build process, extend CLI capabilities. Create custom builders for specific needs. Configure in angular.json. Important for build customization.
Dependency management through ng add, ng update commands. Install Angular-specific packages, update versions. Consider compatibility, migration requirements.
ng lint checks code quality, style guidelines. Configure rules in tslint.json/eslint.json. Enforce coding standards, catch errors. Important for code quality.
Custom schematics through @angular-devkit/schematics. Create templates, transformation rules. Test using schematics-cli. Important for project-specific generators.
Workspace configs in angular.json define project settings. Configure build options, serve options, test options. Support multiple projects, shared configurations.
PWA implementation through @angular/pwa package. Use ng add @angular/pwa command. Configure service worker, manifest. Consider offline capabilities.
Build configurations define different build settings. Configure in angular.json configurations section. Support multiple environments, build options. Important for deployment.
Bundle analysis through source-map-explorer, webpack-bundle-analyzer. Use ng build --stats-json option. Identify large modules, optimize size. Important for performance.
Differential loading serves modern/legacy bundles. Enabled by default in new projects. Reduces bundle size for modern browsers. Consider browser support requirements.
Library creation using ng generate library. Configure package.json, public API. Build using ng build library-name. Important for code sharing.
Asset configuration in angular.json assets array. Define source paths, output paths, glob patterns. Handle static files, images. Important for resource management.
i18n implementation through ng xi18n command. Extract messages, configure translations. Support multiple languages, build configurations. Important for localization.
CLI proxies configure backend API routing. Define in proxy.conf.json. Handle CORS, development APIs. Important for local development.
Custom builders extend CLI capabilities. Implement Builder interface, define schema. Test using builder testing utilities. Important for custom build processes.
Project references define project dependencies. Configure in tsconfig.json. Support monorepo setups, shared code. Important for multi-project workspaces.
ESLint integration through @angular-eslint packages. Configure rules, plugins. Replace TSLint (deprecated). Important for code quality.
Deployment commands build production-ready code. Use ng build --prod, configure environments. Consider deployment platform requirements. Important for release process.
Testing utilities through ng test, ng e2e commands. Configure Karma, Protractor settings. Support unit tests, e2e tests. Important for quality assurance.
Update strategies using ng update command. Handle dependency updates, migrations. Consider breaking changes, testing. Important for maintenance.
Workspace scripts in package.json. Define custom commands, build processes. Support development workflow, automation. Important for project management.
Components communicate through: @Input/@Output decorators, services, ViewChild/ContentChild, event emitters, observables/subjects, NgRx store. Each method suitable for different scenarios based on component relationships.
@Input passes data from parent to child, @Output emits events from child to parent. @Input binds property, @Output uses EventEmitter. Important for parent-child communication. Consider property changes detection.
ViewChild accesses child component/element in template. Provides direct reference to child instance. Used for method calls, property access. Consider lifecycle hooks timing.
Services share data between unrelated components. Use observables/subjects for state management. Implement singleton services for global state. Consider dependency injection scope.
EventEmitter emits custom events from child components. Used with @Output decorator. Supports event data passing, multiple subscribers. Consider unsubscription, memory management.
Sibling communication through shared service, parent component mediation. Use observables/subjects for state sharing. Consider component hierarchy, data flow direction.
ContentChild accesses content projection elements. Used with ng-content directive. Access projected content references. Consider content initialization timing.
Large application communication through state management (NgRx), service layers. Implement proper data flow, component organization. Consider scalability, maintainability.
Subjects are special observables for multicasting. Types: Subject, BehaviorSubject, ReplaySubject. Used in services for state sharing. Consider subscription management.
Parent-to-child method calls through ViewChild reference, @Input properties. Consider component lifecycle, method availability. Important for component interaction.
Content projection (ng-content) passes content from parent to child. Supports single/multiple slots, conditional projection. Important for component reusability.
Event bubbling through host listeners, custom events. Control event propagation, implement handlers. Consider event capture, delegation patterns.
Interaction patterns: mediator, observer, pub/sub patterns. Choose based on component relationships, data flow needs. Consider maintainability, testability.
Dynamic component communication through ComponentFactoryResolver, service injection. Handle component creation, destruction. Consider lifecycle management.
Change detection updates view based on data changes. Affects @Input property updates, event handling. Consider OnPush strategy, performance implications.
Two-way binding through [(ngModel)] or custom implementation. Combine @Input and @Output. Consider change detection, event handling. Important for form controls.
Async pipe handles observables/promises in template. Automatic subscription management, value updates. Important for reactive programming patterns.
State synchronization through services, observables. Implement proper update mechanisms, handle race conditions. Consider state consistency, updates timing.
Lifecycles affect communication timing (ngOnInit, ngOnChanges). Handle initialization, updates, destruction. Consider parent-child timing, change detection.
Error communication through error events, error services. Implement proper error handling, user feedback. Consider error boundaries, recovery strategies.
Interfaces define component communication contracts. Specify input/output properties, methods. Important for type safety, documentation. Consider interface segregation.
Cross-module communication through shared services, state management. Consider module boundaries, service providers. Important for modular applications.
Best practices: proper encapsulation, clear interfaces, unidirectional data flow. Consider component responsibility, communication patterns. Important for maintainability.
Property changes through ngOnChanges lifecycle hook, setter methods. Implement change detection, update logic. Consider simple/complex properties.
Dependency injection provides services, shared instances. Manages component dependencies, service scope. Important for loosely coupled components.
Query parameters through router service, ActivatedRoute. Share state through URL parameters. Consider parameter persistence, navigation handling.
Decorators (@Component, @Input, @Output) configure component behavior. Define metadata, communication interfaces. Important for component definition.
Lazy loading communication through service injection, state management. Handle module loading, component initialization. Consider communication timing.
Anti-patterns: tight coupling, excessive prop drilling, global state abuse. Avoid direct DOM manipulation, complex parent-child chains. Consider code maintainability.
Directives are classes that modify elements. Types: Component directives (with template), Structural directives (modify DOM structure like *ngIf), Attribute directives (modify element behavior like [ngStyle]). Core building blocks of Angular.
Create using @Directive decorator. Implement logic in class methods. Use ElementRef/Renderer2 for DOM manipulation. Can have inputs/outputs, host listeners. Consider selector naming, encapsulation.
Structural directives modify DOM structure. Examples: *ngIf, *ngFor, *ngSwitch. Use microsyntax with asterisk (*). Create template views, handle context. Important for dynamic content.
Pure pipes execute only on pure changes (primitive/reference). Impure pipes execute on every change detection. Pure pipes better for performance. Impure needed for dynamic transformations.
Create using @Pipe decorator. Implement PipeTransform interface. Define transform method for conversion logic. Can accept multiple arguments. Consider pure/impure setting.
Attribute directives change element appearance/behavior. Examples: ngStyle, ngClass. Can respond to user events, modify element properties. Used for dynamic styling, behavior modification.
Events handled through @HostListener decorator. Respond to DOM events, custom events. Can access event data, element properties. Consider event propagation, prevention.
Built-in pipes: DatePipe, UpperCasePipe, LowerCasePipe, CurrencyPipe, DecimalPipe, PercentPipe, AsyncPipe, JsonPipe. Each serves specific transformation need. Consider localization support.
Directive composition through multiple directives on element. Handle directive interaction, priority. Consider order of execution, conflicts. Important for complex behaviors.
AsyncPipe unwraps observable/promise values. Automatically handles subscription/unsubscription. Updates view on new values. Important for reactive programming, memory management.
Custom structural directives use TemplateRef, ViewContainerRef. Create/destroy views programmatically. Handle context binding, view manipulation. Consider microsyntax support.
Directive lifecycles: ngOnInit, ngOnDestroy, ngOnChanges, etc. Handle initialization, changes, cleanup. Consider timing, dependency availability. Important for proper resource management.
Pipe parameters passed after colon in template. Multiple parameters separated by colons. Access in transform method. Consider optional parameters, default values.
Host bindings modify host element properties/attributes. Use @HostBinding decorator. Bind to element properties, classes, styles. Consider property naming, updates.
Pipe chaining connects multiple pipes sequentially. Order matters for transformation. Consider data type compatibility, performance. Use for complex transformations.
Exported directives available for template reference. Use exportAs property. Access directive methods/properties in template. Important for directive interaction.
Directive dependencies injected through constructor. Access services, other directives. Consider dependency scope, availability. Important for directive functionality.
Best practices: pure by default, handle null/undefined, proper error handling. Consider performance impact, reusability. Document parameters, behavior.
Testing through TestBed configuration, component creation. Test directive behavior, pipe transformations. Consider different scenarios, edge cases. Important for reliability.
Template context provides data to template. Access through let syntax. Define custom context properties. Important for dynamic content, loops.
Dynamic pipes selected at runtime. Use pipe binding syntax. Consider pipe availability, performance. Important for flexible transformations.
Directive queries access other directives. Use ContentChild/ContentChildren, ViewChild/ViewChildren. Access child directives, elements. Consider timing, availability.
Pipe errors handled through error handling, default values. Consider null checks, type validation. Provide meaningful error messages. Important for robust applications.
Selector patterns: element, attribute, class selectors. Define directive application scope. Consider naming conventions, specificity. Important for proper directive usage.
i18n in pipes through locale services, formatting options. Support different formats, languages. Consider cultural differences, formats. Important for global applications.
Common patterns: decorator pattern, adapter pattern, composite pattern. Apply based on use case. Consider reusability, maintainability. Important for scalable applications.
Optimization through proper change detection, minimal DOM manipulation. Use OnPush strategy when possible. Consider event binding, updates frequency. Important for application performance.
Communication through inputs/outputs, services, events. Handle parent-child interaction, sibling communication. Consider component hierarchy, data flow. Important for complex directives.
Conflicts resolved through priority, specific selectors. Handle multiple directives, compatibility. Consider directive composition, interaction. Important for complex applications.
Key principles include: Modularity (feature modules), Component-based architecture, Dependency Injection, Separation of Concerns, Single Responsibility. Focus on maintainability, scalability, and reusability. Consider proper layer separation.
Structure using feature modules, core/shared modules, lazy loading. Implement proper folder organization, naming conventions. Consider scalability, maintainability. Follow LIFT principle (Locatable, Isolated, Focused, Testable).
Core Module contains singleton services, one-time imported components. Houses app-wide services, guards, interceptors. Should be imported only in AppModule. Ensures single instance of services.
Feature modules organize related components, services, pipes. Support lazy loading, encapsulation. Improve maintainability, testing. Consider domain boundaries, functionality grouping.
Smart components handle business logic, data. Presentational components focus on UI, receive data via inputs. Promotes reusability, separation of concerns. Consider component responsibility division.
Shared Module contains common components, pipes, directives. Imported by feature modules. Avoids code duplication, promotes reuse. Consider module size, import frequency.
State management through services (small apps) or NgRx/other state libraries (large apps). Consider data flow, state access patterns. Implement proper immutability, change detection strategies.
Service architecture: proper separation, single responsibility, injectable services. Consider service scope, dependency injection. Implement proper error handling, logging.
DDD through feature modules, domain services, entities. Organize by business domains. Consider bounded contexts, domain logic separation. Important for complex business applications.
Communication patterns: Input/Output, Services, State Management, Event Bus. Choose based on component relationship, data flow needs. Consider component coupling, maintainability.
Error handling through ErrorHandler service, HTTP interceptors. Implement centralized error logging, user feedback. Consider different error types, recovery strategies.
API integration: service layer abstraction, typed interfaces, proper error handling. Implement caching, retry strategies. Consider API versioning, security.
Micro-frontends through Module Federation, Custom Elements. Handle inter-app communication, shared dependencies. Consider deployment strategy, team organization.
Form patterns: Template-driven vs Reactive forms, form builders, validation services. Consider form complexity, validation requirements. Implement proper error handling, user feedback.
Security practices: XSS prevention, CSRF protection, secure authentication. Implement proper authorization, input validation. Consider security headers, secure configuration.
Route patterns: feature-based routing, guard protection, resolvers. Implement proper navigation, data preloading. Consider lazy loading, route parameters.
Performance optimization: lazy loading, change detection strategy, bundle optimization. Implement proper caching, virtual scrolling. Consider loading strategies, performance metrics.
Testing architecture: unit tests, integration tests, e2e tests. Implement proper test organization, coverage. Consider test pyramid, testing strategies.
i18n architecture: translation files, language services, locale handling. Implement proper text extraction, runtime translation. Consider cultural differences, formatting.
Auth patterns: JWT handling, guard protection, role-based access. Implement secure token storage, refresh mechanisms. Consider session management, security.
Component libraries: shared styles, documentation, versioning. Implement proper encapsulation, API design. Consider reusability, maintainability.
Dependency management: proper versioning, package organization, update strategy. Implement dependency injection, module organization. Consider bundle size, compatibility.
Logging architecture: centralized logging, error tracking, monitoring. Implement proper log levels, storage strategy. Consider privacy, performance impact.
Offline patterns: service workers, local storage, sync mechanisms. Implement proper caching, data persistence. Consider offline-first approach, sync conflicts.
CI/CD practices: automated testing, build optimization, deployment strategy. Implement proper environment configuration, versioning. Consider deployment automation, monitoring.
Code organization: proper folder structure, naming conventions, module organization. Implement feature-based structure, shared code. Consider scalability, maintainability.
Accessibility practices: ARIA labels, keyboard navigation, screen reader support. Implement proper semantic markup, focus management. Consider WCAG guidelines, testing.
Real-time patterns: WebSocket integration, polling strategies, state updates. Implement proper connection management, error handling. Consider scalability, performance.
Progressive enhancement: feature detection, fallback strategies, graceful degradation. Implement proper browser support, feature flags. Consider user experience, compatibility.
Global error handling through ErrorHandler class. Override handleError method for custom handling. Catches all unhandled errors. Implement logging, user notification, error reporting.
HTTP errors handled through interceptors, catchError operator. Implement retry logic, error transformation. Use error handling services. Consider different error types, user feedback.
Error boundaries through component error handlers, ngOnError hook. Catch and handle rendering errors. Implement fallback UI, error recovery. Consider component hierarchy.
Form errors through validators, error states. Display validation messages, highlight errors. Implement custom validators, async validation. Consider user experience, feedback timing.
RxJS operators: catchError, retry, retryWhen, throwError. Handle stream errors, implement recovery logic. Consider error transformation, retry strategies.
Error logging through centralized service. Log error details, stack traces. Implement remote logging, error tracking. Consider privacy, performance impact.
Best practices: centralized handling, proper error types, user feedback. Implement logging, monitoring. Consider error recovery, graceful degradation.
Async errors through Promise catch, Observable error handling. Implement proper error propagation, recovery. Consider loading states, timeout handling.
Error notifications through toasts, alerts, error pages. Implement user-friendly messages, action options. Consider error severity, context-appropriate feedback.
Navigation errors through Router error events, guards. Implement redirection, error pages. Consider route parameters, navigation history.
Recovery patterns: retry logic, fallback options, graceful degradation. Implement automatic recovery, manual intervention. Consider user experience, data integrity.
Error tracking through monitoring services, custom tracking. Implement error aggregation, analysis. Consider error patterns, impact assessment.
Prevention strategies: input validation, type checking, defensive programming. Implement proper error boundaries, testing. Consider edge cases, error scenarios.
Memory leak prevention through proper unsubscription, cleanup. Implement resource management, leak detection. Consider component lifecycle, subscription handling.
Monitoring tools: Sentry, LogRocket, custom solutions. Implement error tracking, performance monitoring. Consider tool integration, configuration.
Library errors through error wrapping, custom handlers. Implement proper integration, error transformation. Consider library updates, compatibility issues.
Error testing through unit tests, integration tests. Test error scenarios, recovery logic. Consider error simulation, boundary testing.
Production errors through logging, monitoring, alerts. Implement error reporting, analysis. Consider environment differences, security implications.
Error serialization for logging, transmission. Implement proper error format, data extraction. Consider sensitive information, error context.
Offline errors through connection checking, retry logic. Implement offline storage, sync mechanisms. Consider network conditions, data persistence.
Boundary patterns: component-level, route-level, app-level boundaries. Implement proper error isolation, recovery. Consider error propagation, UI feedback.
State errors through error actions, error states. Implement state recovery, rollback mechanisms. Consider state consistency, error propagation.
Anti-patterns: swallowing errors, generic error messages, missing logging. Avoid broad catch blocks, silent failures. Consider proper error handling practices.
Initialization errors through APP_INITIALIZER, bootstrap handling. Implement proper startup checks, fallbacks. Consider application requirements, dependencies.
Reporting practices: proper error format, context inclusion, aggregation. Implement error categorization, priority handling. Consider privacy, compliance requirements.
Security errors through proper validation, authorization checks. Implement secure error messages, logging. Consider sensitive information, attack vectors.
Documentation practices: error codes, handling procedures, recovery steps. Implement proper error guides, troubleshooting docs. Consider user/developer documentation.
Performance errors through monitoring, optimization. Implement performance boundaries, error tracking. Consider resource usage, user experience impact.
Error metrics: error rates, recovery times, impact assessment. Implement metric collection, analysis. Consider monitoring, alerting thresholds.
DI errors through proper provider configuration, error catching. Implement fallback providers, error messages. Consider circular dependencies, missing providers.
Essential tools include: Angular DevTools, Chrome DevTools, Source Maps, Augury (legacy), console methods, debugger statement. VS Code debugging extensions. Browser developer tools for network, performance analysis.
Debug change detection using: enableDebugTools(), profiler, zones visualization. Check for unnecessary triggers, performance impacts. Analyze component update cycles, implement proper change detection strategy.
Debug memory leaks using: Chrome Memory profiler, heap snapshots, timeline recording. Check subscription cleanup, component destruction. Monitor memory usage patterns, implement proper unsubscribe patterns.
Debug routing through Router events logging, Router state inspection. Check guard conditions, route configurations. Analyze navigation lifecycle, implement proper route tracing.
Performance debugging using: Chrome Performance tools, Angular profiler, network tab. Analyze bundle sizes, rendering times, bottlenecks. Monitor CPU usage, implement performance optimizations.
Debug HTTP through Network tab, HttpInterceptors logging. Monitor request/response cycles, analyze headers/payloads. Check error responses, implement proper request tracking.
Production debugging through: error logging services, source maps, monitoring tools. Implement proper error tracking, user feedback collection. Analyze production logs, error patterns.
Debug DI through provider configuration checks, injection tree analysis. Verify service providers, scope issues. Check circular dependencies, implement proper provider debugging.
Template debugging through: browser inspection, Angular DevTools. Check bindings, expressions, structural directives. Analyze template syntax, implement proper debugging statements.
State debugging through: Redux DevTools, state logging, action tracing. Monitor state changes, action effects. Analyze state mutations, implement proper state tracking.
Async debugging through: async/await breakpoints, observable debugging. Monitor promise chains, subscription flows. Analyze async timing issues, implement proper async tracking.
Form debugging through form state inspection, validation checks. Monitor form controls, error states. Analyze validation rules, implement proper form debugging.
Build issues debugging through: build logs, configuration checks. Verify dependencies, version conflicts. Analyze build errors, implement proper build debugging.
Lazy loading debugging through: network monitoring, chunk loading analysis. Check module configurations, route setup. Analyze loading patterns, implement proper chunk tracking.
Component interaction debugging through: input/output tracking, service communication monitoring. Check component hierarchy, event propagation. Analyze component communication patterns.
AOT debugging through: compilation errors analysis, template checking. Verify syntax compatibility, type checking. Analyze compilation process, implement proper AOT debugging.
Security debugging through: CORS analysis, XSS checks, authentication flow verification. Monitor security headers, implement proper security debugging.
SSR debugging through: server logs, hydration checks. Verify platform-specific code, state transfer. Analyze rendering process, implement proper SSR debugging.
Zone.js debugging through: zone hooks monitoring, async operation tracking. Check zone contexts, patched methods. Analyze zone behavior, implement proper zone debugging.
i18n debugging through: translation key checks, locale loading verification. Monitor translation process, cultural formatting. Analyze localization issues, implement proper i18n debugging.
Optimization debugging through: bundle analysis, performance profiling. Check optimization configurations, tree-shaking. Analyze build optimization, implement proper performance debugging.
PWA debugging through: service worker inspection, cache analysis. Verify manifest configuration, offline behavior. Analyze PWA features, implement proper PWA debugging.
Test debugging through: test runner inspection, assertion analysis. Check test setup, mock configurations. Analyze test environment, implement proper test debugging.
Accessibility debugging through: ARIA validation, screen reader testing. Check semantic markup, keyboard navigation. Analyze accessibility requirements, implement proper a11y debugging.
CSS debugging through: style inspection, layout analysis. Check style encapsulation, view encapsulation. Analyze styling issues, implement proper CSS debugging.
WebSocket debugging through: connection state monitoring, message logging. Verify connection lifecycle, error handling. Analyze real-time communication, implement proper WebSocket debugging.
Library debugging through: integration verification, API usage analysis. Check compatibility issues, version conflicts. Analyze library behavior, implement proper integration debugging.
Environment debugging through: configuration file analysis, variable checking. Verify environment setup, build configurations. Analyze environment-specific issues, implement proper config debugging.
Browser compatibility debugging through: cross-browser testing, feature detection. Check polyfills, browser-specific issues. Analyze compatibility requirements, implement proper browser debugging.
Angular CLI build process includes: compilation, bundling, minification, tree-shaking. Uses webpack under the hood. Key features: production optimization, source maps generation, differential loading. Configured through angular.json.
Environments configured through environment.ts files. Create environment-specific files, use fileReplacements in angular.json. Configure variables per environment. Important for different deployment scenarios.
AOT compiles templates during build process. Benefits: faster rendering, smaller file size, better security. Default in production builds. Catches template errors during build time.
CI/CD implementation through pipelines (Jenkins, GitLab CI, etc.). Include build, test, deploy stages. Configure environment variables, deployment targets. Consider automated testing, versioning.
Optimization techniques: bundle splitting, lazy loading, tree shaking, compression. Configure production build flags, performance budgets. Consider source map generation, cache busting.
Deployment configurations through environment files, runtime configuration. Handle API endpoints, feature flags, third-party keys. Consider security, configuration management.
Webpack handles module bundling, asset management, dependency resolution. Configures loaders, plugins for build process. Customizable through custom webpack configuration. Important for build optimization.
Versioning through semantic versioning, automated version bumping. Configure version management in package.json, git tags. Consider changelog generation, release notes.
Deployment strategies: blue-green deployment, canary releases, rolling updates. Consider zero-downtime deployment, rollback capabilities. Implement proper monitoring, health checks.
Docker implementation through multi-stage builds, optimized images. Configure Nginx for serving, environment variables. Consider container orchestration, scaling strategies.
Production considerations: optimization flags, environment configuration, security measures. Enable production mode, implement proper error handling. Consider performance, monitoring setup.
Asset handling through angular.json configuration, CDN integration. Optimize images, manage static files. Consider caching strategies, asset versioning.
SSR deployment using Angular Universal, server configuration. Handle server-side execution, state transfer. Consider SEO requirements, performance implications.
PWA deployment through service worker configuration, manifest setup. Handle offline capabilities, caching strategies. Consider update flow, notification handling.
Automation tools: Jenkins, GitLab CI, GitHub Actions, Azure DevOps. Configure build pipelines, deployment scripts. Consider integration testing, automated releases.
Dependency management through package-lock.json, npm/yarn. Handle version conflicts, security updates. Consider dependency auditing, update strategies.
Artifact management through artifact repositories, versioning system. Handle storage, distribution of builds. Consider cleanup policies, retention rules.
Rolling updates through deployment orchestration, version management. Handle traffic routing, health checks. Consider zero-downtime deployment, rollback procedures.
Monitoring through logging services, performance tracking. Implement error tracking, user analytics. Consider alerting systems, incident response.
Configuration management through environment files, runtime config. Implement secure storage, access control. Consider configuration versioning, audit trail.
Optimization flags control build process features. Include production mode, bundling options, optimization levels. Consider performance impact, debugging capabilities.
Multi-environment through environment-specific configurations, deployment pipelines. Handle different settings, API endpoints. Consider environment isolation, access control.
Security considerations: HTTPS setup, CSP configuration, secure headers. Implement access controls, security scanning. Consider vulnerability assessment, compliance requirements.
Rollbacks through version control, deployment history. Implement automated rollback procedures. Consider data consistency, dependency compatibility.
Build performance through caching, parallel processing, incremental builds. Optimize build configuration, dependency management. Consider CI/CD pipeline efficiency.
Feature flags through configuration management, runtime toggles. Handle feature enabling/disabling, A/B testing. Consider flag cleanup, documentation.
Deployment testing through smoke tests, integration tests. Implement automated validation, environment verification. Consider test coverage, failure scenarios.
Database migrations through versioning, rollback support. Implement migration scripts, data validation. Consider zero-downtime updates, data integrity.
Documentation practices: deployment procedures, configuration guides, troubleshooting steps. Maintain version history, change logs. Consider knowledge transfer, maintenance.
Cache management through versioning, cache busting strategies. Handle browser caching, CDN configuration. Consider cache invalidation, performance impact.
Understand concepts like components, modules, services, and dependency injection.
Work on state management, routing, and component communications.
Dive into RxJS, change detection, and performance optimization.
Expect hands-on challenges to build, test, and optimize Angular applications.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Angular questions, mock interviews, and more to secure your dream role.
Start Preparing now