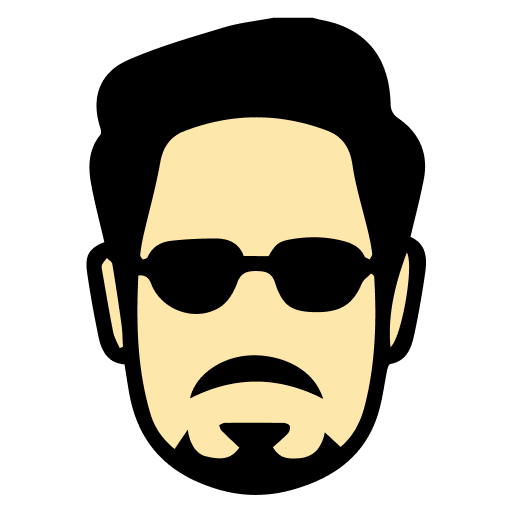
Jest is a powerful JavaScript testing framework designed for simplicity and performance. Stark.ai offers a curated collection of Jest interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Jest is a JavaScript testing framework developed by Facebook that focuses on simplicity. Key features include: 1)...
Jest setup involves: 1) Installing Jest using npm/yarn: 'npm install --save-dev jest', 2) Adding test script to...
A Jest test consists of: 1) describe blocks for grouping related tests, 2) it or test blocks for individual test...
Matchers are methods that let you test values in different ways. Common matchers include: 1) toBe() for exact...
Async testing can be handled through: 1) Returning promises: return promise.then(), 2) Async/await: async () => {...
Jest provides lifecycle hooks: 1) beforeAll() - runs once before all tests, 2) beforeEach() - runs before each test,...
Jest provides multiple mocking approaches: 1) jest.fn() for function mocking, 2) jest.mock() for module mocking, 3)...
Snapshot testing: 1) Captures component/data structure output, 2) Saves as reference file, 3) Compares against...
Test isolation involves: 1) Using beforeEach/afterEach for setup/cleanup, 2) Avoiding shared state between tests, 3)...
Code coverage measures how much code is tested. Jest configuration: 1) Add --coverage flag to test command, 2)...
Different configurations through: 1) Multiple jest.config.js files, 2) Using --config flag, 3) Environment-specific...
Custom matchers: 1) Created using expect.extend(), 2) Define matcher function with pass/message, 3) Can access...
Environment variables handled through: 1) process.env in tests, 2) .env files with jest-environment, 3) setupFiles...
Organization best practices: 1) Group related tests in describe blocks, 2) Use clear test descriptions, 3) Follow...
Timeout handling: 1) Global timeout in jest.config.js, 2) Individual test timeouts using jest.setTimeout(), 3)...
Common CLI options: 1) --watch for watch mode, 2) --coverage for coverage reports, 3) --verbose for detailed output,...
Test fixtures implementation: 1) Using beforeEach for setup, 2) Shared test data files, 3) Factory functions for...
Mock functions provide: 1) Call tracking, 2) Return value specification, 3) Implementation replacement, 4) Behavior...
Test retry handling: 1) Configure retry attempts, 2) Implement retry logic, 3) Handle async retry scenarios, 4) Log...
Test contexts provide: 1) Shared state within describe blocks, 2) Setup/teardown management, 3) Data sharing between...
Advanced mocking includes: 1) Partial module mocking, 2) Mock implementation factories, 3) Complex return value...
Complex async testing: 1) Multiple promise chains, 2) Parallel async operations, 3) Error handling scenarios, 4)...
Custom environments: 1) Extending Jest's environment, 2) Implementing global setup/teardown, 3) Custom DOM...
Advanced snapshots: 1) Custom serializers, 2) Dynamic snapshot matching, 3) Inline snapshots, 4) Snapshot...
Performance testing: 1) Execution time measurements, 2) Memory usage tracking, 3) Performance benchmarks, 4)...
jest.fn() creates a mock function that allows: 1) Tracking calls and arguments, 2) Setting return values using...
jest.spyOn() is used to: 1) Monitor method calls on objects, 2) Preserve original implementation while tracking...
Key differences: 1) jest.fn() creates new mock functions while spyOn monitors existing ones, 2) spyOn can restore...
Module mocking uses: 1) jest.mock() for automatic mocking, 2) Manual mocks in __mocks__ directory, 3)...
Mock return values can be set using: 1) mockReturnValue() for fixed values, 2) mockReturnValueOnce() for single...
Mock calls verified using: 1) toHaveBeenCalled() for call checking, 2) toHaveBeenCalledWith() for argument checking,...
mockClear(): 1) Resets call history of mock function, 2) Used between tests to ensure isolation, 3) Doesn't reset...
Async mocking involves: 1) mockResolvedValue() for successful promises, 2) mockRejectedValue() for failed promises,...
Manual mocks: 1) Created in __mocks__ directory, 2) Match module name and structure, 3) Exported as module.exports,...
ES6 class mocking: 1) Use jest.mock() for class, 2) Mock constructor and methods, 3) Implement instance methods with...
Partial mocking involves: 1) Using jest.mock() with partial implementations, 2) requireActual() for original...
Mock implementations: 1) Set using mockImplementation(), 2) Define custom behavior, 3) Can access call arguments, 4)...
Timer mocking uses: 1) jest.useFakeTimers(), 2) jest.advanceTimersByTime(), 3) jest.runAllTimers(), 4)...
HTTP request mocking: 1) Mock fetch/axios globally, 2) Use jest.mock() for HTTP clients, 3) Implement custom...
Complex sequences using: 1) mockReturnValueOnce() chain, 2) mockImplementation with state, 3) Array of return...
Limitations include: 1) Hoisting requirements, 2) ES6 module constraints, 3) Dynamic import challenges, 4) Circular...
Native object mocking: 1) Use jest.spyOn on prototype, 2) Mock specific methods, 3) Restore original behavior, 4)...
Mock contexts provide: 1) Custom this binding, 2) Shared state between calls, 3) Instance method simulation, 4)...
Mock cleanup involves: 1) Using mockReset(), 2) mockRestore() for spies, 3) Cleanup in afterEach, 4) Handling...
Mock factories: 1) Create reusable mock configurations, 2) Generate consistent test doubles, 3) Support...
Advanced patterns include: 1) Dynamic mock generation, 2) Mock inheritance chains, 3) Conditional mock behavior, 4)...
Complex async mocking: 1) Chain multiple async operations, 2) Handle race conditions, 3) Mock streaming interfaces,...
Stateful mocks require: 1) Internal state management, 2) State-dependent behavior, 3) State reset mechanisms, 4)...
Orchestration patterns: 1) Coordinate multiple mocks, 2) Manage mock interactions, 3) Synchronize mock behavior, 4)...
expect is Jest's assertion function that: 1) Takes a value to test, 2) Returns an expectation object, 3) Chains with...
Key differences: 1) toBe() uses Object.is for strict equality, 2) toEqual() performs deep equality comparison, 3)...
Truthiness matchers include: 1) toBeTruthy() - checks if value is truthy, 2) toBeFalsy() - checks if value is falsy,...
Numeric matchers include: 1) toBeGreaterThan(), 2) toBeLessThan(), 3) toBeGreaterThanOrEqual(), 4)...
String matchers include: 1) toMatch() for regex patterns, 2) toContain() for substring checks, 3) toHaveLength() for...
Array matchers include: 1) toContain() for item presence, 2) toHaveLength() for array length, 3) toEqual() for deep...
Object matchers include: 1) toEqual() for deep equality, 2) toMatchObject() for partial matching, 3)...
Exception testing uses: 1) toThrow() for any error, 2) toThrowError() with specific error, 3) expect(() =>...
The not modifier: 1) Inverts matcher expectation, 2) Used as .not before matchers, 3) Works with all matchers, 4)...
Promise testing uses: 1) resolves matcher for success, 2) rejects matcher for failures, 3) Async/await syntax, 4)...
Custom matchers created using: 1) expect.extend(), 2) Matcher function with pass/message, 3) this.isNot for...
Asymmetric matchers: 1) Allow partial matching, 2) Include expect.any(), expect.arrayContaining(), 3) Used in...
Snapshot testing uses: 1) toMatchSnapshot() matcher, 2) toMatchInlineSnapshot(), 3) Serializer customization, 4)...
Message best practices: 1) Clear failure descriptions, 2) Context-specific details, 3) Expected vs actual values, 4)...
DOM assertions use: 1) toBeInTheDocument(), 2) toHaveTextContent(), 3) toHaveAttribute(), 4) toBeVisible(), 5)...
Partial matching uses: 1) objectContaining(), 2) arrayContaining(), 3) stringContaining(), 4) stringMatching(), 5)...
Complex comparisons use: 1) Deep equality checks, 2) Custom matchers, 3) Partial matching, 4) Property path...
Timeout handling includes: 1) Setting timeout duration, 2) Async assertion timing, 3) Custom timeout messages, 4)...
Function call testing uses: 1) toHaveBeenCalled(), 2) toHaveBeenCalledWith(), 3) toHaveBeenCalledTimes(), 4)...
Compound assertions: 1) Chain multiple expectations, 2) Combine matchers logically, 3) Use and/or operators, 4)...
Advanced matchers include: 1) Complex matching logic, 2) Custom error formatting, 3) Assertion composition, 4) Async...
Complex async testing: 1) Multiple promise chains, 2) Race condition testing, 3) Timeout handling, 4) Error case...
State assertions include: 1) Complex state verification, 2) State transition testing, 3) Conditional assertions, 4)...
Advanced snapshots: 1) Dynamic content handling, 2) Serializer customization, 3) Snapshot transformations, 4)...
Performance assertions: 1) Execution time checks, 2) Resource usage validation, 3) Performance threshold testing, 4)...
Complex data testing: 1) Graph structure validation, 2) Tree traversal assertions, 3) Collection relationship...
Jest initialization involves: 1) Installing Jest: npm install --save-dev jest, 2) Adding test script to...
jest.config.js is the main configuration file that includes: 1) testEnvironment setting, 2) moduleFileExtensions, 3)...
Test environment configuration includes: 1) Setting testEnvironment in config (jsdom/node), 2) Creating custom...
Transform configs handle: 1) File preprocessing before tests, 2) Babel integration for modern JavaScript, 3)...
Test pattern configuration uses: 1) testMatch for glob patterns, 2) testRegex for regex patterns, 3)...
setupFilesAfterEnv: 1) Runs setup code after test framework initialization, 2) Configures global test setup, 3) Adds...
Coverage configuration includes: 1) collectCoverage flag, 2) coverageDirectory setting, 3) coverageThreshold...
Module mappings use: 1) moduleNameMapper in config, 2) Alias definitions, 3) Regular expression patterns, 4)...
Timeout configuration includes: 1) Global testTimeout setting, 2) Individual test timeouts, 3) Async operation...
Globals configuration: 1) Define global variables, 2) Set up global mocks, 3) Configure global setup/teardown, 4)...
TypeScript configuration includes: 1) Installing ts-jest, 2) Configuring transform with ts-jest, 3) Setting up...
Platform configuration involves: 1) Environment-specific configs, 2) Conditional setup logic, 3) Platform-specific...
Reporter configuration includes: 1) Custom reporter implementation, 2) Reporter options setup, 3) Multiple reporter...
Test data configuration: 1) Fixture setup locations, 2) Data loading strategies, 3) Cleanup mechanisms, 4) Data...
Snapshot configuration: 1) snapshotSerializers setup, 2) Snapshot directory location, 3) Update and retention...
Parallelization config includes: 1) maxWorkers setting, 2) Worker pool configuration, 3) Test file distribution, 4)...
Custom matcher configuration: 1) Matcher implementation, 2) Global matcher registration, 3) TypeScript definitions,...
Test filtering config: 1) testNamePattern settings, 2) Test file patterns, 3) Tag-based filtering, 4) Run group...
Retry configuration: 1) jest-retry setup, 2) Retry attempts settings, 3) Retry delay configuration, 4) Failure...
Isolation configuration: 1) Test environment isolation, 2) State reset mechanisms, 3) Module isolation, 4) Database...
Advanced environment config: 1) Custom environment implementation, 2) Environment chains, 3) Dynamic environment...
Complex suite configuration: 1) Multi-project setup, 2) Shared configurations, 3) Dynamic config generation, 4)...
Custom runner implementation: 1) Runner API implementation, 2) Test execution flow, 3) Result reporting, 4) Resource...
Monitoring configuration: 1) Metric collection setup, 2) Performance monitoring, 3) Resource tracking, 4) Alert...
Advanced coverage config: 1) Custom coverage reporters, 2) Coverage analysis tools, 3) Branch coverage requirements,...
Factory configuration: 1) Factory implementation patterns, 2) Data generation strategies, 3) Factory composition, 4)...
Scheduler implementation: 1) Test execution ordering, 2) Resource allocation, 3) Priority management, 4) Dependency...
Infrastructure configuration: 1) CI/CD integration, 2) Resource provisioning, 3) Environment management, 4) Scaling...
Advanced mocking config: 1) Custom mock factories, 2) Mock state management, 3) Mock verification systems, 4) Mock...
React Testing Library: 1) Works with Jest as test runner, 2) Provides utilities for testing React components, 3)...
Component rendering tests: 1) Use render function from Testing Library, 2) Query rendered elements, 3) Assert on...
Query types include: 1) getBy* for elements that should be present, 2) queryBy* for elements that might not exist,...
User event testing: 1) Import userEvent from @testing-library/user-event, 2) Simulate user interactions, 3) Assert...
jest-dom provides: 1) DOM-specific matchers, 2) toBeInTheDocument(), 3) toHaveClass(), 4) toBeVisible(), 5)...
Props testing includes: 1) Rendering with different prop values, 2) Testing prop type validation, 3) Verifying prop...
Form testing involves: 1) Input change simulation, 2) Form submission testing, 3) Validation testing, 4) Error...
Hook testing requires: 1) @testing-library/react-hooks, 2) renderHook utility, 3) Testing state updates, 4) Testing...
Accessibility testing includes: 1) Role-based queries, 2) ARIA attribute testing, 3) Keyboard navigation testing, 4)...
State testing involves: 1) Triggering state updates, 2) Verifying state changes, 3) Testing state-dependent...
Async testing includes: 1) Using findBy* queries, 2) Waiting for element changes, 3) Testing loading states, 4)...
Context testing involves: 1) Wrapping components in providers, 2) Testing context updates, 3) Testing consumer...
Redux integration testing: 1) Providing store wrapper, 2) Testing connected components, 3) Dispatching actions, 4)...
Router testing includes: 1) MemoryRouter setup, 2) Route rendering verification, 3) Navigation testing, 4) Route...
Dependencies testing: 1) Mocking external hooks, 2) Testing dependency interactions, 3) Testing error cases, 4)...
Error boundary testing: 1) Error simulation, 2) Fallback rendering, 3) Error recovery, 4) Props passing through...
Performance testing: 1) Render timing measurement, 2) Re-render optimization verification, 3) Memory leak detection,...
Composition testing: 1) Testing component hierarchies, 2) Props drilling verification, 3) Component interaction...
Side effect testing: 1) useEffect testing, 2) Cleanup verification, 3) External interaction testing, 4) Timing...
Lifecycle testing: 1) Mount behavior testing, 2) Update verification, 3) Unmount cleanup testing, 4) State...
Advanced patterns: 1) Component test factories, 2) Reusable test utilities, 3) Custom render methods, 4) Test...
Complex UI testing: 1) Multi-step interaction flows, 2) Conditional rendering paths, 3) State machine testing, 4)...
Microservice integration: 1) Service communication testing, 2) API interaction verification, 3) Error handling, 4)...
Scaling testing: 1) Large data set handling, 2) Performance with many components, 3) Memory optimization, 4) Render...
Visual testing: 1) Screenshot comparison, 2) Layout verification, 3) Style regression testing, 4) Component visual...
Security testing: 1) XSS prevention, 2) Data sanitization, 3) Authentication flows, 4) Authorization checks, 5)...
Advanced state testing: 1) Complex state machines, 2) State orchestration, 3) State synchronization, 4) Persistence...
Monitoring testing: 1) Performance metrics, 2) Error tracking, 3) Usage analytics, 4) Resource monitoring, 5) Health...
Stress testing: 1) Load testing components, 2) Resource limits testing, 3) Concurrent operation handling, 4) Error...
Integration testing: 1) Tests interactions between components/modules, 2) Verifies combined functionality, 3) Tests...
Setup includes: 1) Configuring test environment, 2) Setting up test databases/services, 3) Creating test fixtures,...
Common patterns: 1) Component interaction testing, 2) API integration testing, 3) Database integration testing, 4)...
Test data handling: 1) Setup test databases, 2) Create seed data, 3) Manage test data isolation, 4) Clean up after...
Mocking in integration tests: 1) Mock external services selectively, 2) Keep core integrations real, 3) Mock...
API integration testing: 1) Test real API endpoints, 2) Verify request/response handling, 3) Test error scenarios,...
Database testing practices: 1) Use test database instance, 2) Reset database state between tests, 3) Test...
Test isolation involves: 1) Independent test databases, 2) Cleaning up test data, 3) Resetting state between tests,...
Service testing strategies: 1) Test service communication, 2) Verify service contracts, 3) Test service...
Async handling: 1) Use async/await syntax, 2) Set appropriate timeouts, 3) Handle promise chains, 4) Test concurrent...
Component communication testing: 1) Test props passing, 2) Verify event handling, 3) Test context updates, 4) Check...
Microservice testing: 1) Test service discovery, 2) Verify service communication, 3) Test failure scenarios, 4)...
Data flow testing: 1) Verify data passing, 2) Test state updates, 3) Check data transformations, 4) Test data...
External service testing: 1) Test API contracts, 2) Handle authentication, 3) Test error scenarios, 4) Verify data...
Error handling testing: 1) Test error propagation, 2) Verify error recovery, 3) Test error boundaries, 4) Check...
Authentication testing: 1) Test login flows, 2) Verify token handling, 3) Test permission checks, 4) Check session...
State management testing: 1) Test store interactions, 2) Verify state updates, 3) Test state synchronization, 4)...
Cache testing: 1) Test cache operations, 2) Verify cache invalidation, 3) Test cache consistency, 4) Check cache...
Queue testing: 1) Test message publishing, 2) Verify message consumption, 3) Test queue operations, 4) Check message...
File system testing: 1) Test file operations, 2) Verify file permissions, 3) Test file streams, 4) Check file...
Complex integration: 1) Test multi-step flows, 2) Verify system interactions, 3) Test state transitions, 4) Check...
Distributed testing: 1) Test network partitions, 2) Verify consensus protocols, 3) Test leader election, 4) Check...
Real-time testing: 1) Test WebSocket connections, 2) Verify event streaming, 3) Test pub/sub patterns, 4) Check...
Scalability testing: 1) Test load distribution, 2) Verify system capacity, 3) Test resource scaling, 4) Check...
Consistency testing: 1) Test eventual consistency, 2) Verify data synchronization, 3) Test conflict resolution, 4)...
Resilience testing: 1) Test failure scenarios, 2) Verify system recovery, 3) Test circuit breakers, 4) Check...
Monitoring testing: 1) Test metric collection, 2) Verify alerting systems, 3) Test logging integration, 4) Check...
Security testing: 1) Test security protocols, 2) Verify access controls, 3) Test encryption, 4) Check security...
Compliance testing: 1) Test regulatory requirements, 2) Verify audit trails, 3) Test data protection, 4) Check...
Measuring execution time: 1) Use jest.useFakeTimers(), 2) Use console.time/timeEnd, 3) Implement custom timing...
Test timeout configuration: 1) Set global timeout in config, 2) Set individual test timeouts, 3) Balance timeout vs...
Identifying slow tests: 1) Use --verbose flag, 2) Implement custom timing reporters, 3) Monitor test execution...
Basic metrics include: 1) Test execution time, 2) Setup/teardown time, 3) Memory usage, 4) Number of assertions, 5)...
Async performance testing: 1) Measure async operation duration, 2) Track concurrent operations, 3) Monitor resource...
Parallelization impacts: 1) Reduced total execution time, 2) Increased resource usage, 3) Potential test...
Memory measurement: 1) Use process.memoryUsage(), 2) Track heap snapshots, 3) Monitor garbage collection, 4) Measure...
Common bottlenecks: 1) Large test setups, 2) Excessive mocking, 3) Unnecessary test repetition, 4) Poor test...
Optimization strategies: 1) Use beforeAll when possible, 2) Minimize per-test setup, 3) Implement efficient cleanup,...
Caching impacts: 1) Test result caching, 2) Module caching, 3) Transform caching, 4) Resource caching, 5) Cache...
Benchmark implementation: 1) Define performance baselines, 2) Create benchmark suites, 3) Measure key metrics, 4)...
Rendering performance: 1) Measure render times, 2) Track re-renders, 3) Monitor DOM updates, 4) Test large datasets,...
Resource testing: 1) Monitor CPU usage, 2) Track memory consumption, 3) Measure I/O operations, 4) Test concurrent...
Network testing: 1) Measure request latency, 2) Test concurrent requests, 3) Simulate network conditions, 4) Monitor...
Test profiling: 1) Use Node.js profiler, 2) Implement custom profilers, 3) Track execution paths, 4) Measure...
Data processing: 1) Test large datasets, 2) Measure processing speed, 3) Monitor memory usage, 4) Test batch...
Memory leak testing: 1) Track object retention, 2) Monitor heap growth, 3) Test long-running operations, 4)...
Cache performance: 1) Measure hit rates, 2) Test cache efficiency, 3) Monitor cache size, 4) Test eviction policies,...
Database testing: 1) Measure query performance, 2) Test connection pooling, 3) Monitor transaction speed, 4) Test...
I/O performance: 1) Measure read/write speeds, 2) Test concurrent operations, 3) Monitor file system usage, 4) Test...
Advanced monitoring: 1) Custom metric collection, 2) Performance dashboards, 3) Trend analysis, 4) Alert systems, 5)...
Distributed testing: 1) Test network latency, 2) Measure data consistency, 3) Monitor system throughput, 4) Test...
Real-time testing: 1) Measure response times, 2) Test event processing, 3) Monitor message latency, 4) Test...
Microservice testing: 1) Test service communication, 2) Measure service latency, 3) Monitor resource usage, 4) Test...
Scalability testing: 1) Measure load handling, 2) Test resource scaling, 3) Monitor performance degradation, 4) Test...
Resilience testing: 1) Test failure recovery, 2) Measure degraded performance, 3) Test circuit breakers, 4) Monitor...
Regression testing: 1) Track performance metrics, 2) Compare against baselines, 3) Detect performance degradation,...
Optimization testing: 1) Identify bottlenecks, 2) Measure improvement impact, 3) Test optimization strategies, 4)...
Load testing: 1) Simulate user load, 2) Measure system response, 3) Monitor resource usage, 4) Test concurrent...
Capacity testing: 1) Measure maximum load, 2) Test resource limits, 3) Monitor system boundaries, 4) Test scaling...
Key principles include: 1) Test isolation - each test should be independent, 2) Clear test descriptions, 3) Single...
AAA pattern involves: 1) Arrange - setup test data and conditions, 2) Act - perform the action being tested, 3)...
Test organization best practices: 1) Keep tests close to source files, 2) Use consistent naming conventions...
Good test descriptions: 1) Clearly state what's being tested, 2) Describe expected behavior, 3) Use consistent...
Test data management: 1) Use fixtures for consistent data, 2) Implement factory functions, 3) Clean up test data...
Mock best practices: 1) Only mock what's necessary, 2) Keep mocks simple, 3) Clean up mocks after tests, 4) Use...
Assertion best practices: 1) Use specific assertions, 2) Keep assertions focused, 3) Use meaningful error messages,...
Hook guidelines: 1) Use beforeAll for one-time setup, 2) Use beforeEach for per-test setup, 3) Clean up resources in...
Error testing practices: 1) Test expected error conditions, 2) Verify error messages and types, 3) Test error...
Maintenance practices: 1) Regular test cleanup, 2) Update tests with code changes, 3) Remove obsolete tests, 4)...
Async test structure: 1) Use async/await consistently, 2) Handle promises properly, 3) Set appropriate timeouts, 4)...
Complex component patterns: 1) Break down into smaller test cases, 2) Test component integration, 3) Verify state...
Integration test organization: 1) Group related scenarios, 2) Test component interactions, 3) Verify system...
Snapshot practices: 1) Keep snapshots focused, 2) Review snapshot changes carefully, 3) Update snapshots...
Coverage management: 1) Set realistic coverage goals, 2) Focus on critical paths, 3) Don't chase 100% blindly, 4)...
Hook testing patterns: 1) Test hook behavior, 2) Verify state changes, 3) Test effect cleanup, 4) Check dependency...
Performance optimization: 1) Minimize test setup, 2) Use efficient assertions, 3) Optimize mock usage, 4) Implement...
Utility testing practices: 1) Test pure functions thoroughly, 2) Cover edge cases, 3) Test input validation, 4)...
Test double management: 1) Use appropriate double types, 2) Maintain double fidelity, 3) Clean up doubles after use,...
State management patterns: 1) Test state transitions, 2) Verify state updates, 3) Test state synchronization, 4)...
Advanced interaction testing: 1) Test complex user flows, 2) Verify component communication, 3) Test state...
Microservice testing patterns: 1) Test service boundaries, 2) Verify service communication, 3) Test service...
Async workflow testing: 1) Test workflow steps, 2) Verify state transitions, 3) Test error recovery, 4) Check...
Distributed system practices: 1) Test network partitions, 2) Verify data consistency, 3) Test system recovery, 4)...
Performance testing practices: 1) Benchmark critical operations, 2) Test resource usage, 3) Verify optimization...
Security testing patterns: 1) Test authentication flows, 2) Verify authorization rules, 3) Test data protection, 4)...
Large suite management: 1) Organize tests effectively, 2) Implement test categorization, 3) Maintain test...
Data pipeline practices: 1) Test data transformations, 2) Verify data integrity, 3) Test pipeline stages, 4) Check...
System integration structure: 1) Define integration boundaries, 2) Test system interactions, 3) Verify end-to-end...
Jest is a JavaScript testing framework developed by Facebook that focuses on simplicity. Key features include: 1) Zero config setup for most JavaScript projects, 2) Snapshot testing, 3) Built-in code coverage reports, 4) Isolated test execution, 5) Interactive mode for test development, 6) Powerful mocking system, 7) Support for async testing.
Jest setup involves: 1) Installing Jest using npm/yarn: 'npm install --save-dev jest', 2) Adding test script to package.json: { 'scripts': { 'test': 'jest' } }, 3) Creating test files with .test.js or .spec.js extensions, 4) Configuring Jest through jest.config.js if needed, 5) Setting up any required environment configurations.
A Jest test consists of: 1) describe blocks for grouping related tests, 2) it or test blocks for individual test cases, 3) expect statements for assertions. Example: describe('MyFunction', () => { it('should return correct value', () => { expect(myFunction()).toBe(true); }); });
Matchers are methods that let you test values in different ways. Common matchers include: 1) toBe() for exact equality, 2) toEqual() for deep equality, 3) toContain() for arrays/iterables, 4) toBeTruthy()/toBeFalsy() for boolean checks, 5) toMatch() for regex. Used with expect(): expect(value).matcher()
Async testing can be handled through: 1) Returning promises: return promise.then(), 2) Async/await: async () => { await result }, 3) Done callback: (done) => { process.then(done) }, 4) Resolves/rejects matchers: expect(promise).resolves.toBe(). Always ensure async operations complete before test ends.
Jest provides lifecycle hooks: 1) beforeAll() - runs once before all tests, 2) beforeEach() - runs before each test, 3) afterEach() - runs after each test, 4) afterAll() - runs once after all tests. Used for setup/cleanup operations. Example: setting up database connections, cleaning test data.
Jest provides multiple mocking approaches: 1) jest.fn() for function mocking, 2) jest.mock() for module mocking, 3) jest.spyOn() for monitoring function calls, 4) Manual mocks using __mocks__ directory, 5) Automatic mocking of node_modules. Mocks help isolate code for testing.
Snapshot testing: 1) Captures component/data structure output, 2) Saves as reference file, 3) Compares against future changes, 4) Helps detect unintended changes. Example: expect(component).toMatchSnapshot(). Useful for UI components and serializable data.
Test isolation involves: 1) Using beforeEach/afterEach for setup/cleanup, 2) Avoiding shared state between tests, 3) Mocking external dependencies, 4) Using describe blocks for context separation, 5) Resetting mocks between tests using jest.clearAllMocks().
Code coverage measures how much code is tested. Jest configuration: 1) Add --coverage flag to test command, 2) Configure coverage settings in jest.config.js, 3) Set coverage thresholds, 4) Specify files to include/exclude, 5) Generate coverage reports in different formats.
Different configurations through: 1) Multiple jest.config.js files, 2) Using --config flag, 3) Environment-specific configs, 4) Test setup files, 5) Custom test environments. Example: separating unit and integration test configs.
Custom matchers: 1) Created using expect.extend(), 2) Define matcher function with pass/message, 3) Can access expect context, 4) Support async matchers, 5) Can be shared across tests. Example: expect.extend({ toBeWithinRange(received, floor, ceiling) { ... } }).
Environment variables handled through: 1) process.env in tests, 2) .env files with jest-environment, 3) setupFiles configuration, 4) CLI arguments, 5) Custom environment setup. Consider security and isolation of test environments.
Organization best practices: 1) Group related tests in describe blocks, 2) Use clear test descriptions, 3) Follow naming conventions (.test.js/.spec.js), 4) Organize test files alongside source code, 5) Separate unit and integration tests.
Timeout handling: 1) Global timeout in jest.config.js, 2) Individual test timeouts using jest.setTimeout(), 3) Timeout option in test blocks, 4) Async test timeout handling, 5) Custom timeout messages. Default timeout is 5 seconds.
Common CLI options: 1) --watch for watch mode, 2) --coverage for coverage reports, 3) --verbose for detailed output, 4) --runInBand for sequential execution, 5) --testNamePattern for filtering tests. Can be configured in package.json scripts.
Test fixtures implementation: 1) Using beforeEach for setup, 2) Shared test data files, 3) Factory functions for test data, 4) Fixture cleanup in afterEach, 5) Module-level fixture definitions. Helps maintain consistent test data.
Mock functions provide: 1) Call tracking, 2) Return value specification, 3) Implementation replacement, 4) Behavior verification, 5) Async mock support. Example: const mock = jest.fn().mockReturnValue(value).
Test retry handling: 1) Configure retry attempts, 2) Implement retry logic, 3) Handle async retry scenarios, 4) Log retry attempts, 5) Analyze flaky test patterns. Use jest-retry for automated retries.
Test contexts provide: 1) Shared state within describe blocks, 2) Setup/teardown management, 3) Data sharing between tests, 4) Context-specific configurations, 5) Isolated test environments. Used through beforeAll/beforeEach hooks.
Advanced mocking includes: 1) Partial module mocking, 2) Mock implementation factories, 3) Complex return value sequences, 4) Mock instance methods, 5) Mock timers and events. Example: jest.spyOn() with complex implementations.
Complex async testing: 1) Multiple promise chains, 2) Parallel async operations, 3) Error handling scenarios, 4) Timeout management, 5) Race condition testing. Use async/await with proper error handling.
Custom environments: 1) Extending Jest's environment, 2) Implementing global setup/teardown, 3) Custom DOM implementation, 4) Environment-specific mocks, 5) Shared environment state. Used for specialized testing needs.
Advanced snapshots: 1) Custom serializers, 2) Dynamic snapshot matching, 3) Inline snapshots, 4) Snapshot migrations, 5) Conditional snapshot updates. Used for complex component testing.
Performance testing: 1) Execution time measurements, 2) Memory usage tracking, 3) Performance benchmarks, 4) Resource utilization tests, 5) Performance regression detection. Use custom metrics and assertions.
jest.fn() creates a mock function that allows: 1) Tracking calls and arguments, 2) Setting return values using mockReturnValue(), 3) Implementing custom behavior using mockImplementation(), 4) Clearing/resetting mock data, 5) Verifying call conditions. Example: const mock = jest.fn().mockReturnValue('result');
jest.spyOn() is used to: 1) Monitor method calls on objects, 2) Preserve original implementation while tracking calls, 3) Temporarily mock method behavior, 4) Restore original implementation using mockRestore(), 5) Access call history and mock functionality. Example: jest.spyOn(object, 'method')
Key differences: 1) jest.fn() creates new mock functions while spyOn monitors existing ones, 2) spyOn can restore original implementation, jest.fn() cannot, 3) spyOn requires an object and method, jest.fn() is standalone, 4) spyOn preserves original method by default, 5) jest.fn() starts as empty function.
Module mocking uses: 1) jest.mock() for automatic mocking, 2) Manual mocks in __mocks__ directory, 3) mockImplementation() for custom behavior, 4) requireActual() for partial mocking, 5) hoisting of jest.mock() calls. Example: jest.mock('./module', () => ({ method: jest.fn() }));
Mock return values can be set using: 1) mockReturnValue() for fixed values, 2) mockReturnValueOnce() for single calls, 3) mockImplementation() for custom logic, 4) mockResolvedValue() for promises, 5) mockRejectedValue() for promise rejections. Example: mock.mockReturnValue('result');
Mock calls verified using: 1) toHaveBeenCalled() for call checking, 2) toHaveBeenCalledWith() for argument checking, 3) toHaveBeenCalledTimes() for call count, 4) mock.calls array for detailed inspection, 5) mock.results for return values. Example: expect(mock).toHaveBeenCalledWith(arg);
mockClear(): 1) Resets call history of mock function, 2) Used between tests to ensure isolation, 3) Doesn't reset implementation or return values, 4) Often used in beforeEach, 5) Helps prevent test interdependence. Example: beforeEach(() => { mock.mockClear(); });
Async mocking involves: 1) mockResolvedValue() for successful promises, 2) mockRejectedValue() for failed promises, 3) mockImplementation() with async functions, 4) Chain .then handlers for complex cases, 5) Await mock function calls in tests. Example: mock.mockResolvedValue('result');
Manual mocks: 1) Created in __mocks__ directory, 2) Match module name and structure, 3) Exported as module.exports, 4) Can include complex implementations, 5) Automatically used when module is required. Used for consistent mock implementations across tests.
ES6 class mocking: 1) Use jest.mock() for class, 2) Mock constructor and methods, 3) Implement instance methods with mockImplementation, 4) Mock static methods directly, 5) Handle inheritance if needed. Example: jest.mock('./MyClass', () => { return jest.fn().mockImplementation(() => { return {method: jest.fn()}; }); });
Partial mocking involves: 1) Using jest.mock() with partial implementations, 2) requireActual() for original methods, 3) Mixing real and mocked exports, 4) Careful management of module state, 5) Clear documentation of mixed implementation. Example: jest.mock('./module', () => ({ ...jest.requireActual('./module'), specificMethod: jest.fn() }));
Mock implementations: 1) Set using mockImplementation(), 2) Define custom behavior, 3) Can access call arguments, 4) Support async operations, 5) Can be changed between tests. Example: mock.mockImplementation((arg) => arg * 2);
Timer mocking uses: 1) jest.useFakeTimers(), 2) jest.advanceTimersByTime(), 3) jest.runAllTimers(), 4) jest.runOnlyPendingTimers(), 5) jest.clearAllTimers(). Helps test setTimeout, setInterval, etc. Example: jest.useFakeTimers(); jest.advanceTimersByTime(1000);
HTTP request mocking: 1) Mock fetch/axios globally, 2) Use jest.mock() for HTTP clients, 3) Implement custom response handling, 4) Mock different response scenarios, 5) Handle async behavior properly. Consider using libraries like jest-fetch-mock.
Complex sequences using: 1) mockReturnValueOnce() chain, 2) mockImplementation with state, 3) Array of return values, 4) Conditional return logic, 5) Mock result queues. Example: mock.mockReturnValueOnce(1).mockReturnValueOnce(2).mockReturnValue(3);
Limitations include: 1) Hoisting requirements, 2) ES6 module constraints, 3) Dynamic import challenges, 4) Circular dependency issues, 5) Reset complexities. Understanding helps avoid common pitfalls and design better tests.
Native object mocking: 1) Use jest.spyOn on prototype, 2) Mock specific methods, 3) Restore original behavior, 4) Handle inheritance chain, 5) Consider side effects. Example: jest.spyOn(console, 'log').mockImplementation();
Mock contexts provide: 1) Custom this binding, 2) Shared state between calls, 3) Instance method simulation, 4) Constructor behavior, 5) Method chaining support. Used for complex object mocking scenarios.
Mock cleanup involves: 1) Using mockReset(), 2) mockRestore() for spies, 3) Cleanup in afterEach, 4) Handling partial restorations, 5) Managing global mocks. Ensures test isolation and prevents side effects.
Mock factories: 1) Create reusable mock configurations, 2) Generate consistent test doubles, 3) Support parameterization, 4) Enable mock composition, 5) Facilitate maintenance. Example: const createMock = (config) => jest.fn().mockImplementation(config);
Advanced patterns include: 1) Dynamic mock generation, 2) Mock inheritance chains, 3) Conditional mock behavior, 4) Mock composition, 5) State-dependent mocking. Used for complex module interactions.
Complex async mocking: 1) Chain multiple async operations, 2) Handle race conditions, 3) Mock streaming interfaces, 4) Simulate timeouts, 5) Test error scenarios. Example: mock.mockImplementation(async () => { /* complex async logic */ });
Stateful mocks require: 1) Internal state management, 2) State-dependent behavior, 3) State reset mechanisms, 4) State verification, 5) Thread-safe state handling. Used for complex behavioral simulation.
Orchestration patterns: 1) Coordinate multiple mocks, 2) Manage mock interactions, 3) Synchronize mock behavior, 4) Handle mock dependencies, 5) Implement mock workflows. Used for system-level testing.
expect is Jest's assertion function that: 1) Takes a value to test, 2) Returns an expectation object, 3) Chains with matchers (toBe, toEqual, etc.), 4) Supports async assertions, 5) Can be extended with custom matchers. Example: expect(value).toBe(expected);
Key differences: 1) toBe() uses Object.is for strict equality, 2) toEqual() performs deep equality comparison, 3) toBe() is for primitives and object references, 4) toEqual() is for object/array content comparison, 5) toEqual() recursively checks nested structures.
Truthiness matchers include: 1) toBeTruthy() - checks if value is truthy, 2) toBeFalsy() - checks if value is falsy, 3) toBeNull() - checks for null, 4) toBeUndefined() - checks for undefined, 5) toBeDefined() - checks if value is not undefined.
Numeric matchers include: 1) toBeGreaterThan(), 2) toBeLessThan(), 3) toBeGreaterThanOrEqual(), 4) toBeLessThanOrEqual(), 5) toBeCloseTo() for floating point. Example: expect(value).toBeGreaterThan(3);
String matchers include: 1) toMatch() for regex patterns, 2) toContain() for substring checks, 3) toHaveLength() for string length, 4) toEqual() for exact matches, 5) toString() for string conversion checks. Example: expect(string).toMatch(/pattern/);
Array matchers include: 1) toContain() for item presence, 2) toHaveLength() for array length, 3) toEqual() for deep equality, 4) arrayContaining() for partial matches, 5) toContainEqual() for object matching in arrays.
Object matchers include: 1) toEqual() for deep equality, 2) toMatchObject() for partial matching, 3) toHaveProperty() for property checks, 4) objectContaining() for subset matching, 5) toBeInstanceOf() for type checking.
Exception testing uses: 1) toThrow() for any error, 2) toThrowError() with specific error, 3) expect(() => {}).toThrow() syntax, 4) Error message matching, 5) Error type checking. Example: expect(() => fn()).toThrow('error message');
The not modifier: 1) Inverts matcher expectation, 2) Used as .not before matchers, 3) Works with all matchers, 4) Maintains proper error messages, 5) Useful for negative assertions. Example: expect(value).not.toBe(3);
Promise testing uses: 1) resolves matcher for success, 2) rejects matcher for failures, 3) Async/await syntax, 4) Return promises in tests, 5) Chain additional matchers. Example: expect(promise).resolves.toBe(value);
Custom matchers created using: 1) expect.extend(), 2) Matcher function with pass/message, 3) this.isNot for negation, 4) Async matcher support, 5) Custom error messages. Example: expect.extend({ customMatcher(received, expected) { return { pass: condition, message: () => message }; } });
Asymmetric matchers: 1) Allow partial matching, 2) Include expect.any(), expect.arrayContaining(), 3) Used in object/array comparisons, 4) Support custom implementations, 5) Useful for flexible assertions. Example: expect({ prop: 'value' }).toEqual(expect.objectContaining({ prop: expect.any(String) }));
Snapshot testing uses: 1) toMatchSnapshot() matcher, 2) toMatchInlineSnapshot(), 3) Serializer customization, 4) Dynamic snapshot content, 5) Snapshot update flow. Example: expect(component).toMatchSnapshot();
Message best practices: 1) Clear failure descriptions, 2) Context-specific details, 3) Expected vs actual values, 4) Custom matcher messages, 5) Error hint inclusion. Helps with test debugging and maintenance.
DOM assertions use: 1) toBeInTheDocument(), 2) toHaveTextContent(), 3) toHaveAttribute(), 4) toBeVisible(), 5) toBeDisabled(). Often used with @testing-library/jest-dom matchers.
Partial matching uses: 1) objectContaining(), 2) arrayContaining(), 3) stringContaining(), 4) stringMatching(), 5) Custom matchers for specific cases. Example: expect(object).toEqual(expect.objectContaining({ key: value }));
Complex comparisons use: 1) Deep equality checks, 2) Custom matchers, 3) Partial matching, 4) Property path assertions, 5) Nested object validation. Consider performance and maintainability.
Timeout handling includes: 1) Setting timeout duration, 2) Async assertion timing, 3) Custom timeout messages, 4) Retry mechanisms, 5) Polling assertions. Important for async testing scenarios.
Function call testing uses: 1) toHaveBeenCalled(), 2) toHaveBeenCalledWith(), 3) toHaveBeenCalledTimes(), 4) toHaveBeenLastCalledWith(), 5) Call argument inspection. Used with mock functions and spies.
Compound assertions: 1) Chain multiple expectations, 2) Combine matchers logically, 3) Use and/or operators, 4) Group related assertions, 5) Complex condition testing. Example: expect(value).toBeDefined().and.toBeTruthy();
Advanced matchers include: 1) Complex matching logic, 2) Custom error formatting, 3) Assertion composition, 4) Async matcher support, 5) Context-aware matching. Used for specialized testing needs.
Complex async testing: 1) Multiple promise chains, 2) Race condition testing, 3) Timeout handling, 4) Error case coverage, 5) State verification. Requires careful timing management.
State assertions include: 1) Complex state verification, 2) State transition testing, 3) Conditional assertions, 4) State history validation, 5) Side effect checking. Used for stateful component testing.
Advanced snapshots: 1) Dynamic content handling, 2) Serializer customization, 3) Snapshot transformations, 4) Conditional snapshots, 5) Snapshot maintenance strategies. Used for complex component testing.
Performance assertions: 1) Execution time checks, 2) Resource usage validation, 3) Performance threshold testing, 4) Benchmark comparisons, 5) Performance regression detection. Used for performance testing.
Complex data testing: 1) Graph structure validation, 2) Tree traversal assertions, 3) Collection relationship testing, 4) Data integrity checks, 5) Structure transformation verification. Used for complex data scenarios.
Jest initialization involves: 1) Installing Jest: npm install --save-dev jest, 2) Adding test script to package.json: { 'scripts': { 'test': 'jest' } }, 3) Creating jest.config.js (optional), 4) Setting up test directories, 5) Configuring environment if needed.
jest.config.js is the main configuration file that includes: 1) testEnvironment setting, 2) moduleFileExtensions, 3) setupFilesAfterEnv for setup files, 4) testMatch for test file patterns, 5) coverageThreshold settings, 6) transform configurations for preprocessors.
Test environment configuration includes: 1) Setting testEnvironment in config (jsdom/node), 2) Creating custom environments, 3) Configuring environment variables, 4) Setting up global mocks, 5) Handling environment-specific setup.
Transform configs handle: 1) File preprocessing before tests, 2) Babel integration for modern JavaScript, 3) TypeScript transformation, 4) Custom transformers for specific files, 5) Cache configuration for transforms.
Test pattern configuration uses: 1) testMatch for glob patterns, 2) testRegex for regex patterns, 3) testPathIgnorePatterns for exclusions, 4) File naming conventions (.test.js, .spec.js), 5) Directory organization patterns.
setupFilesAfterEnv: 1) Runs setup code after test framework initialization, 2) Configures global test setup, 3) Adds custom matchers, 4) Sets up test environment, 5) Configures global mocks. Example: ['./jest.setup.js']
Coverage configuration includes: 1) collectCoverage flag, 2) coverageDirectory setting, 3) coverageThreshold requirements, 4) collectCoverageFrom patterns, 5) coverageReporters options for report formats.
Module mappings use: 1) moduleNameMapper in config, 2) Alias definitions, 3) Regular expression patterns, 4) Identity-obj-proxy for style imports, 5) Custom module resolvers. Helps with import resolution and mocking.
Timeout configuration includes: 1) Global testTimeout setting, 2) Individual test timeouts, 3) Async operation timeouts, 4) Setup/teardown timeouts, 5) Custom timeout messages. Default is usually 5000ms.
Globals configuration: 1) Define global variables, 2) Set up global mocks, 3) Configure global setup/teardown, 4) Define global matchers, 5) Set up global test utilities. Available throughout test suite.
TypeScript configuration includes: 1) Installing ts-jest, 2) Configuring transform with ts-jest, 3) Setting up tsconfig.json, 4) Configuring type checking, 5) Handling TypeScript paths and aliases.
Platform configuration involves: 1) Environment-specific configs, 2) Conditional setup logic, 3) Platform-specific mocks, 4) Environment detection, 5) Platform-specific test runners.
Reporter configuration includes: 1) Custom reporter implementation, 2) Reporter options setup, 3) Multiple reporter configuration, 4) Output formatting, 5) Integration with CI/CD systems.
Test data configuration: 1) Fixture setup locations, 2) Data loading strategies, 3) Cleanup mechanisms, 4) Data isolation approaches, 5) Shared test data management.
Snapshot configuration: 1) snapshotSerializers setup, 2) Snapshot directory location, 3) Update and retention policies, 4) Custom serializers, 5) Snapshot format options.
Parallelization config includes: 1) maxWorkers setting, 2) Worker pool configuration, 3) Test file distribution, 4) Resource allocation, 5) Parallel run coordination.
Custom matcher configuration: 1) Matcher implementation, 2) Global matcher registration, 3) TypeScript definitions, 4) Matcher error messages, 5) Matcher documentation.
Test filtering config: 1) testNamePattern settings, 2) Test file patterns, 3) Tag-based filtering, 4) Run group configuration, 5) Conditional test execution.
Retry configuration: 1) jest-retry setup, 2) Retry attempts settings, 3) Retry delay configuration, 4) Failure conditions, 5) Retry reporting options.
Isolation configuration: 1) Test environment isolation, 2) State reset mechanisms, 3) Module isolation, 4) Database isolation, 5) Resource cleanup strategies.
Advanced environment config: 1) Custom environment implementation, 2) Environment chains, 3) Dynamic environment setup, 4) Environment state management, 5) Environment cleanup strategies.
Complex suite configuration: 1) Multi-project setup, 2) Shared configurations, 3) Dynamic config generation, 4) Configuration inheritance, 5) Project-specific overrides.
Custom runner implementation: 1) Runner API implementation, 2) Test execution flow, 3) Result reporting, 4) Resource management, 5) Integration with Jest infrastructure.
Monitoring configuration: 1) Metric collection setup, 2) Performance monitoring, 3) Resource tracking, 4) Alert configuration, 5) Monitoring integration points.
Advanced coverage config: 1) Custom coverage reporters, 2) Coverage analysis tools, 3) Branch coverage requirements, 4) Coverage enforcement rules, 5) Coverage trend analysis.
Factory configuration: 1) Factory implementation patterns, 2) Data generation strategies, 3) Factory composition, 4) State management, 5) Factory cleanup mechanisms.
Scheduler implementation: 1) Test execution ordering, 2) Resource allocation, 3) Priority management, 4) Dependency resolution, 5) Schedule optimization.
Infrastructure configuration: 1) CI/CD integration, 2) Resource provisioning, 3) Environment management, 4) Scaling strategies, 5) Infrastructure monitoring.
Advanced mocking config: 1) Custom mock factories, 2) Mock state management, 3) Mock verification systems, 4) Mock cleanup strategies, 5) Mock interaction recording.
React Testing Library: 1) Works with Jest as test runner, 2) Provides utilities for testing React components, 3) Focuses on testing behavior over implementation, 4) Encourages accessibility-first testing, 5) Uses queries to find elements. Example: render(<Component />); expect(screen.getByText('text')).toBeInTheDocument();
Component rendering tests: 1) Use render function from Testing Library, 2) Query rendered elements, 3) Assert on element presence, 4) Check component content, 5) Verify proper props rendering. Example: const { getByText } = render(<Component prop={value} />);
Query types include: 1) getBy* for elements that should be present, 2) queryBy* for elements that might not exist, 3) findBy* for async elements, 4) getAllBy*, queryAllBy*, findAllBy* for multiple elements, 5) Role-based, text-based, and label-based queries.
User event testing: 1) Import userEvent from @testing-library/user-event, 2) Simulate user interactions, 3) Assert on resulting changes, 4) Handle async events, 5) Test form interactions. Example: await userEvent.click(button); expect(result).toBeVisible();
jest-dom provides: 1) DOM-specific matchers, 2) toBeInTheDocument(), 3) toHaveClass(), 4) toBeVisible(), 5) toHaveValue() and other UI-specific assertions. Enhances Jest for DOM testing.
Props testing includes: 1) Rendering with different prop values, 2) Testing prop type validation, 3) Verifying prop updates, 4) Testing default props, 5) Testing required props. Example: render(<Component testProp='value' />);
Form testing involves: 1) Input change simulation, 2) Form submission testing, 3) Validation testing, 4) Error message verification, 5) Form state testing. Use userEvent for input interactions.
Hook testing requires: 1) @testing-library/react-hooks, 2) renderHook utility, 3) Testing state updates, 4) Testing hook effects, 5) Testing custom hook logic. Example: const { result } = renderHook(() => useCustomHook());
Accessibility testing includes: 1) Role-based queries, 2) ARIA attribute testing, 3) Keyboard navigation testing, 4) Screen reader compatibility, 5) Color contrast verification. Ensures inclusive user experience.
State testing involves: 1) Triggering state updates, 2) Verifying state changes, 3) Testing state-dependent rendering, 4) Testing state transitions, 5) Async state updates. Focus on behavior over implementation.
Async testing includes: 1) Using findBy* queries, 2) Waiting for element changes, 3) Testing loading states, 4) Error state testing, 5) Data fetching verification. Example: await findByText('loaded content');
Context testing involves: 1) Wrapping components in providers, 2) Testing context updates, 3) Testing consumer behavior, 4) Multiple context interaction, 5) Context state changes.
Redux integration testing: 1) Providing store wrapper, 2) Testing connected components, 3) Dispatching actions, 4) Testing state updates, 5) Testing selectors. Use proper store setup.
Router testing includes: 1) MemoryRouter setup, 2) Route rendering verification, 3) Navigation testing, 4) Route parameter testing, 5) Route protection testing. Consider router context.
Dependencies testing: 1) Mocking external hooks, 2) Testing dependency interactions, 3) Testing error cases, 4) Testing cleanup, 5) Testing hook composition. Use proper isolation.
Error boundary testing: 1) Error simulation, 2) Fallback rendering, 3) Error recovery, 4) Props passing through boundaries, 5) Nested error boundaries. Test error handling.
Performance testing: 1) Render timing measurement, 2) Re-render optimization verification, 3) Memory leak detection, 4) React profiler integration, 5) Performance regression testing.
Composition testing: 1) Testing component hierarchies, 2) Props drilling verification, 3) Component interaction testing, 4) Render prop testing, 5) HOC testing. Test component relationships.
Side effect testing: 1) useEffect testing, 2) Cleanup verification, 3) External interaction testing, 4) Timing dependencies, 5) Side effect ordering. Consider effect dependencies.
Lifecycle testing: 1) Mount behavior testing, 2) Update verification, 3) Unmount cleanup testing, 4) State persistence, 5) Effect timing. Test component phases.
Advanced patterns: 1) Component test factories, 2) Reusable test utilities, 3) Custom render methods, 4) Test composition, 5) Shared test behaviors. Improve test maintainability.
Complex UI testing: 1) Multi-step interaction flows, 2) Conditional rendering paths, 3) State machine testing, 4) Animation testing, 5) Gesture handling. Test user scenarios.
Microservice integration: 1) Service communication testing, 2) API interaction verification, 3) Error handling, 4) State synchronization, 5) Service dependency management.
Scaling testing: 1) Large data set handling, 2) Performance with many components, 3) Memory optimization, 4) Render optimization, 5) Resource management. Test scalability limits.
Visual testing: 1) Screenshot comparison, 2) Layout verification, 3) Style regression testing, 4) Component visual states, 5) Responsive design testing. Use visual testing tools.
Security testing: 1) XSS prevention, 2) Data sanitization, 3) Authentication flows, 4) Authorization checks, 5) Secure props handling. Test security measures.
Advanced state testing: 1) Complex state machines, 2) State orchestration, 3) State synchronization, 4) Persistence testing, 5) State recovery scenarios. Test state complexity.
Monitoring testing: 1) Performance metrics, 2) Error tracking, 3) Usage analytics, 4) Resource monitoring, 5) Health checks. Test monitoring integration.
Stress testing: 1) Load testing components, 2) Resource limits testing, 3) Concurrent operation handling, 4) Error recovery under stress, 5) Performance degradation testing.
Integration testing: 1) Tests interactions between components/modules, 2) Verifies combined functionality, 3) Tests real dependencies (vs mocked in unit tests), 4) Focuses on component communication, 5) Tests larger parts of the application together. More comprehensive but slower than unit tests.
Setup includes: 1) Configuring test environment, 2) Setting up test databases/services, 3) Creating test fixtures, 4) Configuring global setup/teardown, 5) Setting appropriate timeouts. Example: setupFilesAfterEnv for global setup.
Common patterns: 1) Component interaction testing, 2) API integration testing, 3) Database integration testing, 4) Service integration testing, 5) End-to-end flows. Focus on testing interfaces between parts.
Test data handling: 1) Setup test databases, 2) Create seed data, 3) Manage test data isolation, 4) Clean up after tests, 5) Use realistic test data. Important for meaningful integration tests.
Mocking in integration tests: 1) Mock external services selectively, 2) Keep core integrations real, 3) Mock non-essential dependencies, 4) Use realistic mock data, 5) Balance between isolation and realism.
API integration testing: 1) Test real API endpoints, 2) Verify request/response handling, 3) Test error scenarios, 4) Check authentication/authorization, 5) Test API versioning. Use actual HTTP calls.
Database testing practices: 1) Use test database instance, 2) Reset database state between tests, 3) Test transactions and rollbacks, 4) Verify data integrity, 5) Test database migrations.
Test isolation involves: 1) Independent test databases, 2) Cleaning up test data, 3) Resetting state between tests, 4) Isolating external services, 5) Managing shared resources. Prevents test interference.
Service testing strategies: 1) Test service communication, 2) Verify service contracts, 3) Test service dependencies, 4) Check error handling, 5) Test service state management.
Async handling: 1) Use async/await syntax, 2) Set appropriate timeouts, 3) Handle promise chains, 4) Test concurrent operations, 5) Verify async state changes. Important for realistic testing.
Component communication testing: 1) Test props passing, 2) Verify event handling, 3) Test context updates, 4) Check state propagation, 5) Test component lifecycles. Focus on interaction points.
Microservice testing: 1) Test service discovery, 2) Verify service communication, 3) Test failure scenarios, 4) Check service dependencies, 5) Test service scaling. Consider distributed nature.
Data flow testing: 1) Verify data passing, 2) Test state updates, 3) Check data transformations, 4) Test data validation, 5) Verify data consistency. Focus on data integrity.
External service testing: 1) Test API contracts, 2) Handle authentication, 3) Test error scenarios, 4) Verify data formats, 5) Test service availability. Consider service stability.
Error handling testing: 1) Test error propagation, 2) Verify error recovery, 3) Test error boundaries, 4) Check error logging, 5) Test error state management. Important for reliability.
Authentication testing: 1) Test login flows, 2) Verify token handling, 3) Test permission checks, 4) Check session management, 5) Test authentication errors. Critical for security.
State management testing: 1) Test store interactions, 2) Verify state updates, 3) Test state synchronization, 4) Check state persistence, 5) Test state cleanup. Focus on data flow.
Cache testing: 1) Test cache operations, 2) Verify cache invalidation, 3) Test cache consistency, 4) Check cache performance, 5) Test cache failures. Important for performance.
Queue testing: 1) Test message publishing, 2) Verify message consumption, 3) Test queue operations, 4) Check message ordering, 5) Test queue errors. Focus on reliability.
File system testing: 1) Test file operations, 2) Verify file permissions, 3) Test file streams, 4) Check file cleanup, 5) Test file locks. Consider system interactions.
Complex integration: 1) Test multi-step flows, 2) Verify system interactions, 3) Test state transitions, 4) Check data consistency, 5) Test recovery scenarios. Focus on system behavior.
Distributed testing: 1) Test network partitions, 2) Verify consensus protocols, 3) Test leader election, 4) Check data replication, 5) Test system recovery. Consider CAP theorem.
Real-time testing: 1) Test WebSocket connections, 2) Verify event streaming, 3) Test pub/sub patterns, 4) Check message ordering, 5) Test connection recovery. Focus on timing.
Scalability testing: 1) Test load distribution, 2) Verify system capacity, 3) Test resource scaling, 4) Check performance degradation, 5) Test bottlenecks. Important for growth.
Consistency testing: 1) Test eventual consistency, 2) Verify data synchronization, 3) Test conflict resolution, 4) Check consistency models, 5) Test partition tolerance. Critical for reliability.
Resilience testing: 1) Test failure scenarios, 2) Verify system recovery, 3) Test circuit breakers, 4) Check fallback mechanisms, 5) Test degraded operations. Focus on reliability.
Monitoring testing: 1) Test metric collection, 2) Verify alerting systems, 3) Test logging integration, 4) Check monitoring accuracy, 5) Test monitoring scalability. Important for operations.
Security testing: 1) Test security protocols, 2) Verify access controls, 3) Test encryption, 4) Check security boundaries, 5) Test security monitoring. Critical for protection.
Compliance testing: 1) Test regulatory requirements, 2) Verify audit trails, 3) Test data protection, 4) Check policy enforcement, 5) Test compliance reporting. Important for regulations.
Measuring execution time: 1) Use jest.useFakeTimers(), 2) Use console.time/timeEnd, 3) Implement custom timing decorators, 4) Use performance.now(), 5) Configure Jest's verbose timing option. Example: const start = performance.now(); /* test */ const duration = performance.now() - start;
Test timeout configuration: 1) Set global timeout in config, 2) Set individual test timeouts, 3) Balance timeout vs expected performance, 4) Handle slow tests appropriately, 5) Monitor timeout failures for performance issues.
Identifying slow tests: 1) Use --verbose flag, 2) Implement custom timing reporters, 3) Monitor test execution times, 4) Set timing thresholds, 5) Use test profiling tools. Helps optimize test suite performance.
Basic metrics include: 1) Test execution time, 2) Setup/teardown time, 3) Memory usage, 4) Number of assertions, 5) Test suite duration. Important for establishing baselines.
Async performance testing: 1) Measure async operation duration, 2) Track concurrent operations, 3) Monitor resource usage, 4) Test throughput capabilities, 5) Handle timeouts appropriately.
Parallelization impacts: 1) Reduced total execution time, 2) Increased resource usage, 3) Potential test interference, 4) Worker process management, 5) Load balancing considerations.
Memory measurement: 1) Use process.memoryUsage(), 2) Track heap snapshots, 3) Monitor garbage collection, 4) Measure memory leaks, 5) Implement memory thresholds.
Common bottlenecks: 1) Large test setups, 2) Excessive mocking, 3) Unnecessary test repetition, 4) Poor test isolation, 5) Inefficient assertions. Identify and optimize these areas.
Optimization strategies: 1) Use beforeAll when possible, 2) Minimize per-test setup, 3) Implement efficient cleanup, 4) Share setup where appropriate, 5) Cache test resources.
Caching impacts: 1) Test result caching, 2) Module caching, 3) Transform caching, 4) Resource caching, 5) Cache invalidation strategies. Proper caching improves performance.
Benchmark implementation: 1) Define performance baselines, 2) Create benchmark suites, 3) Measure key metrics, 4) Compare against standards, 5) Track performance trends.
Rendering performance: 1) Measure render times, 2) Track re-renders, 3) Monitor DOM updates, 4) Test large datasets, 5) Profile component lifecycle.
Resource testing: 1) Monitor CPU usage, 2) Track memory consumption, 3) Measure I/O operations, 4) Test concurrent processing, 5) Implement resource limits.
Network testing: 1) Measure request latency, 2) Test concurrent requests, 3) Simulate network conditions, 4) Monitor bandwidth usage, 5) Test connection pooling.
Test profiling: 1) Use Node.js profiler, 2) Implement custom profilers, 3) Track execution paths, 4) Measure function calls, 5) Analyze bottlenecks.
Data processing: 1) Test large datasets, 2) Measure processing speed, 3) Monitor memory usage, 4) Test batch processing, 5) Benchmark algorithms.
Memory leak testing: 1) Track object retention, 2) Monitor heap growth, 3) Test long-running operations, 4) Implement cleanup verification, 5) Use memory profiling tools.
Cache performance: 1) Measure hit rates, 2) Test cache efficiency, 3) Monitor cache size, 4) Test eviction policies, 5) Benchmark cache operations.
Database testing: 1) Measure query performance, 2) Test connection pooling, 3) Monitor transaction speed, 4) Test concurrent access, 5) Benchmark CRUD operations.
I/O performance: 1) Measure read/write speeds, 2) Test concurrent operations, 3) Monitor file system usage, 4) Test streaming performance, 5) Benchmark file operations.
Advanced monitoring: 1) Custom metric collection, 2) Performance dashboards, 3) Trend analysis, 4) Alert systems, 5) Performance regression detection.
Distributed testing: 1) Test network latency, 2) Measure data consistency, 3) Monitor system throughput, 4) Test scalability, 5) Benchmark distributed operations.
Real-time testing: 1) Measure response times, 2) Test event processing, 3) Monitor message latency, 4) Test concurrent users, 5) Benchmark real-time operations.
Microservice testing: 1) Test service communication, 2) Measure service latency, 3) Monitor resource usage, 4) Test service scaling, 5) Benchmark service operations.
Scalability testing: 1) Measure load handling, 2) Test resource scaling, 3) Monitor performance degradation, 4) Test concurrent operations, 5) Benchmark scaling operations.
Resilience testing: 1) Test failure recovery, 2) Measure degraded performance, 3) Test circuit breakers, 4) Monitor system stability, 5) Benchmark recovery operations.
Regression testing: 1) Track performance metrics, 2) Compare against baselines, 3) Detect performance degradation, 4) Monitor trends, 5) Implement automated checks.
Optimization testing: 1) Identify bottlenecks, 2) Measure improvement impact, 3) Test optimization strategies, 4) Monitor resource efficiency, 5) Benchmark optimizations.
Load testing: 1) Simulate user load, 2) Measure system response, 3) Monitor resource usage, 4) Test concurrent users, 5) Benchmark load handling.
Capacity testing: 1) Measure maximum load, 2) Test resource limits, 3) Monitor system boundaries, 4) Test scaling limits, 5) Benchmark capacity handling.
Key principles include: 1) Test isolation - each test should be independent, 2) Clear test descriptions, 3) Single assertion per test when possible, 4) Proper setup and cleanup, 5) Following the Arrange-Act-Assert pattern, 6) Testing behavior not implementation, 7) Maintaining test readability.
AAA pattern involves: 1) Arrange - setup test data and conditions, 2) Act - perform the action being tested, 3) Assert - verify the expected outcome. Important for: test clarity, maintainability, and proper organization of test logic.
Test organization best practices: 1) Keep tests close to source files, 2) Use consistent naming conventions (.test.js, .spec.js), 3) Group related tests in describe blocks, 4) Organize by feature or component, 5) Maintain clear file structure.
Good test descriptions: 1) Clearly state what's being tested, 2) Describe expected behavior, 3) Use consistent terminology, 4) Follow 'it should...' pattern, 5) Are specific and unambiguous. Helps with test documentation and maintenance.
Test data management: 1) Use fixtures for consistent data, 2) Implement factory functions, 3) Clean up test data after use, 4) Keep test data minimal and focused, 5) Avoid sharing mutable test data between tests.
Mock best practices: 1) Only mock what's necessary, 2) Keep mocks simple, 3) Clean up mocks after tests, 4) Use meaningful mock implementations, 5) Avoid mocking everything. Mocks should be minimal and focused.
Assertion best practices: 1) Use specific assertions, 2) Keep assertions focused, 3) Use meaningful error messages, 4) Avoid multiple assertions when possible, 5) Test one concept per test case.
Hook guidelines: 1) Use beforeAll for one-time setup, 2) Use beforeEach for per-test setup, 3) Clean up resources in afterEach/afterAll, 4) Keep hooks focused and minimal, 5) Avoid complex logic in hooks.
Error testing practices: 1) Test expected error conditions, 2) Verify error messages and types, 3) Test error handling behavior, 4) Use proper error assertions, 5) Consider edge cases and invalid inputs.
Maintenance practices: 1) Regular test cleanup, 2) Update tests with code changes, 3) Remove obsolete tests, 4) Maintain test documentation, 5) Refactor tests when needed. Keep tests current and valuable.
Async test structure: 1) Use async/await consistently, 2) Handle promises properly, 3) Set appropriate timeouts, 4) Test both success and failure cases, 5) Verify async state changes.
Complex component patterns: 1) Break down into smaller test cases, 2) Test component integration, 3) Verify state management, 4) Test edge cases, 5) Maintain test isolation.
Integration test organization: 1) Group related scenarios, 2) Test component interactions, 3) Verify system integration, 4) Maintain clear test boundaries, 5) Focus on integration points.
Snapshot practices: 1) Keep snapshots focused, 2) Review snapshot changes carefully, 3) Update snapshots intentionally, 4) Maintain snapshot readability, 5) Use inline snapshots when appropriate.
Coverage management: 1) Set realistic coverage goals, 2) Focus on critical paths, 3) Don't chase 100% blindly, 4) Monitor coverage trends, 5) Use coverage reports effectively.
Hook testing patterns: 1) Test hook behavior, 2) Verify state changes, 3) Test effect cleanup, 4) Check dependency updates, 5) Test error cases. Use @testing-library/react-hooks.
Performance optimization: 1) Minimize test setup, 2) Use efficient assertions, 3) Optimize mock usage, 4) Implement proper test isolation, 5) Use test parallelization effectively.
Utility testing practices: 1) Test pure functions thoroughly, 2) Cover edge cases, 3) Test input validation, 4) Verify error handling, 5) Maintain function independence.
Test double management: 1) Use appropriate double types, 2) Maintain double fidelity, 3) Clean up doubles after use, 4) Document double behavior, 5) Verify double interactions.
State management patterns: 1) Test state transitions, 2) Verify state updates, 3) Test state synchronization, 4) Check state persistence, 5) Test state cleanup.
Advanced interaction testing: 1) Test complex user flows, 2) Verify component communication, 3) Test state propagation, 4) Check event handling, 5) Test integration points.
Microservice testing patterns: 1) Test service boundaries, 2) Verify service communication, 3) Test service isolation, 4) Check service resilience, 5) Test service scaling.
Async workflow testing: 1) Test workflow steps, 2) Verify state transitions, 3) Test error recovery, 4) Check workflow completion, 5) Test concurrent workflows.
Distributed system practices: 1) Test network partitions, 2) Verify data consistency, 3) Test system recovery, 4) Check system coordination, 5) Test scaling behavior.
Performance testing practices: 1) Benchmark critical operations, 2) Test resource usage, 3) Verify optimization effectiveness, 4) Test scaling limits, 5) Monitor performance regression.
Security testing patterns: 1) Test authentication flows, 2) Verify authorization rules, 3) Test data protection, 4) Check security boundaries, 5) Test security policies.
Large suite management: 1) Organize tests effectively, 2) Implement test categorization, 3) Maintain test independence, 4) Optimize test execution, 5) Monitor test health.
Data pipeline practices: 1) Test data transformations, 2) Verify data integrity, 3) Test pipeline stages, 4) Check error handling, 5) Test pipeline recovery.
System integration structure: 1) Define integration boundaries, 2) Test system interactions, 3) Verify end-to-end flows, 4) Test system resilience, 5) Check system recovery.
Understand how Jest works and its key features.
Learn how to use spies, mocks, and fake timers effectively.
Explore best practices for fast and reliable testing.
Learn how to use Jest’s snapshot feature for UI testing.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Jest questions, mock interviews, and more to secure your dream role.
Start Preparing now