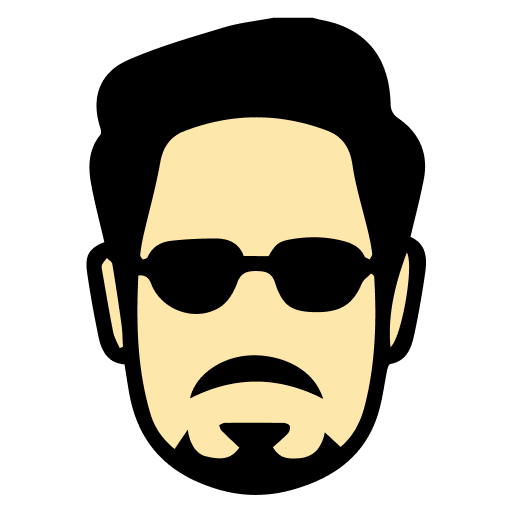
Swift is Apple's powerful and intuitive programming language used for iOS, macOS, watchOS, and tvOS development. Stark.ai offers a curated collection of Swift interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Swift differs from Objective-C in several key aspects: 1) Type safety and inference, 2) Optionals for safe handling...
Optionals in Swift represent values that may or may not exist. Unwrapping methods include: 1) Force unwrapping (!),...
Value types (struct, enum) create a new copy when assigned, while reference types (class) share the same instance....
Type Inference allows Swift to automatically deduce variable types: 1) Compiler analyzes initialization value, 2)...
Closures are self-contained blocks of functionality: 1) Can capture and store references to variables/constants, 2)...
Pattern Matching in Swift includes: 1) Switch statement patterns, 2) Case let bindings, 3) Where clauses for...
Property Wrappers add behavior to properties: 1) Encapsulate common property patterns, 2) Reduce boilerplate code,...
Swift provides five access levels: 1) open - accessible outside module, can be subclassed, 2) public - accessible...
Extensions add functionality to existing types: 1) Add computed properties, 2) Define instance/type methods, 3)...
Generics enable flexible, reusable code: 1) Type-safe abstract types, 2) Generic functions and types, 3) Type...
Swift supports various property types: 1) Stored properties (var/let), 2) Computed properties (get/set), 3) Property...
Method Dispatch determines how methods are called: 1) Static dispatch for value types, 2) Dynamic dispatch for class...
Subscripts provide shorthand access to collections: 1) Custom getter/setter syntax, 2) Multiple parameter support,...
Swift Strings are unique: 1) Unicode-correct by default, 2) Value type semantics, 3) Character-based iteration, 4)...
Tuples group multiple values: 1) Named or unnamed elements, 2) Multiple return values from functions, 3) Pattern...
Swift error handling includes: 1) Error protocol conformance, 2) throws keyword for error-prone functions, 3)...
Keypaths provide type-safe references to properties: 1) KeyPath for read-only properties, 2) WritableKeyPath for...
Static vs Class methods differ in: 1) static cannot be overridden in subclasses, 2) class allows overriding in...
Swift memory management involves: 1) Automatic Reference Counting (ARC), 2) Strong, weak, and unowned references, 3)...
Result Builders enable DSL creation: 1) Custom syntax for building complex objects, 2) SwiftUI view construction, 3)...
Swift initialization includes: 1) Designated initializers, 2) Convenience initializers, 3) Required initializers, 4)...
Lazy properties provide delayed initialization: 1) Initialized only when first accessed, 2) Must be variable (var),...
Type casting mechanisms include: 1) is operator for type checking, 2) as? for conditional downcasting, 3) as! for...
Variadic parameters accept multiple values: 1) Denoted by ... after type, 2) Treated as array inside function, 3)...
Operator overloading features: 1) Custom operator definitions, 2) Precedence group specification, 3) Infix, prefix,...
Property observers monitor changes: 1) willSet executes before change, 2) didSet executes after change, 3) Access to...
String interpolation features: 1) Basic value insertion with \(), 2) Custom interpolation definitions, 3) Formatted...
Phantom types provide compile-time safety: 1) Generic type parameters unused at runtime, 2) Type-level state...
Protocol requirements include: 1) Method signatures, 2) Property specifications, 3) Static/class requirements, 4)...
Type casting in class hierarchies involves: 1) Using 'is' for type checking, 2) 'as?' for conditional downcasting,...
Nested types in Swift: 1) Define types within other types, 2) Provide namespace scoping, 3) Support access control...
Observer pattern implementation includes: 1) Using delegation, 2) NotificationCenter usage, 3) Key-Value Observing...
Convenience initializers: 1) Provide alternative initialization patterns, 2) Must call designated initializer, 3)...
Composition vs Inheritance considerations: 1) Favor composition over inheritance, 2) Use protocols for shared...
Factory method patterns include: 1) Static factory methods, 2) Factory protocol implementation, 3) Generic factory...
Key differences include: 1) Classes are reference types while structures are value types, 2) Classes support...
Swift inheritance features include: 1) Single inheritance only (no multiple inheritance), 2) Method overriding using...
Type methods and properties belong to the type itself: 1) Declared using 'static' or 'class' keywords, 2) 'static'...
Swift supports polymorphism through: 1) Inheritance-based method overriding, 2) Protocol conformance for interface...
Initializers in Swift classes serve multiple purposes: 1) Designated initializers as primary initializers, 2)...
Deinitializers in Swift: 1) Declared using 'deinit' keyword, 2) Called automatically when object is deallocated, 3)...
Computed properties: 1) Calculate value dynamically rather than storing it, 2) Can have getter and optional setter,...
Method dispatch in Swift classes involves: 1) Dynamic dispatch by default for instance methods, 2) Static dispatch...
Access control provides: 1) Encapsulation of implementation details, 2) Interface-based programming, 3) Five access...
Reference cycle management includes: 1) Using weak references for optional references, 2) Unowned references for...
Property wrappers provide: 1) Reusable property behavior encapsulation, 2) Separation of concerns in property...
Singleton implementation includes: 1) Static shared instance property, 2) Private initializer to prevent external...
The 'final' keyword: 1) Prevents method overriding in subclasses, 2) Prevents class inheritance, 3) Enables compiler...
Method overloading allows: 1) Multiple methods with same name but different parameters, 2) Return type...
Inheritance best practices include: 1) Favoring composition over inheritance, 2) Using protocols for shared...
Decorator pattern implementation includes: 1) Protocol-based interface definition, 2) Base class implementation, 3)...
Dependency injection practices include: 1) Constructor injection, 2) Property injection, 3) Method injection, 4)...
Class cluster implementation involves: 1) Abstract factory pattern usage, 2) Private subclass creation, 3) Public...
Builder pattern implementation includes: 1) Method chaining support, 2) Default value handling, 3) Validation logic,...
Thread-safe implementation includes: 1) Serial queue usage, 2) Property wrapper implementation, 3) Atomic...
ARC automatically manages memory by: 1) Tracking strong references to class instances, 2) Deallocating memory when...
Key differences include: 1) weak references are optional and can become nil, 2) unowned references are non-optional...
Retain cycle identification and fixing involves: 1) Using Memory Graph Debugger in Xcode, 2) Implementing weak or...
Closure capture lists: 1) Define how values are captured by closures, 2) Use [weak self] or [unowned self] to...
Strong vs weak references: 1) Strong references increment reference count, weak don't, 2) Strong references keep...
ARC and deinitializers: 1) Deinit called automatically when reference count reaches zero, 2) Only available in class...
Memory management best practices: 1) Use value types when possible, 2) Implement proper weak/unowned references, 3)...
Async operation memory management: 1) Use capture lists in closures, 2) Implement proper cancellation handling, 3)...
Autoreleasepool usage: 1) Manages temporary objects memory, 2) Useful in loops processing many objects, 3) Helps...
Collection memory management: 1) Value type collections copy on write, 2) Reference type elements managed by ARC, 3)...
Reference counting: 1) Tracks number of references to objects, 2) Increments count for new references, 3) Decrements...
Memory leak debugging: 1) Use Xcode Memory Graph Debugger, 2) Implement Instruments for leak detection, 3) Monitor...
Delegate memory management: 1) Use weak references for delegates, 2) Prevent retain cycles, 3) Handle delegate...
Closure memory management: 1) Captures referenced variables strongly by default, 2) Uses capture lists for custom...
Copy-on-write impact: 1) Optimizes value type performance, 2) Shares memory until modification, 3) Creates copies...
Protocol-Oriented Programming (POP) in Swift: 1) Focuses on defining protocols and protocol extensions, 2) Favors...
Protocol Extensions enable: 1) Adding default implementations to protocols, 2) Extending functionality without...
Associated Types: 1) Define placeholder names for types used in protocols, 2) Allow protocols to be generic, 3)...
Protocol Composition includes: 1) Combining multiple protocols using & operator, 2) Creating type constraints with...
Protocol Requirements include: 1) Property requirements (get/set), 2) Method requirements with signatures, 3)...
Protocol Inheritance enables: 1) Creating protocol hierarchies, 2) Inheriting requirements from other protocols, 3)...
Type Constraints in protocol extensions: 1) Limit extension applicability to specific types, 2) Use 'where' clause...
Generic Protocols implementation: 1) Using associated types for generics, 2) Constraining associated types, 3)...
Protocol Witness Table: 1) Stores protocol conformance information, 2) Maps protocol requirements to...
Optional Protocol Requirements: 1) Marked with @objc optional, 2) Only available in Objective-C compatible...
Protocol Extension Method Dispatch: 1) Static dispatch for extension methods, 2) Dynamic dispatch for protocol...
Protocol-Oriented Design practices: 1) Start with protocols before implementations, 2) Use protocol composition for...
Conditional Conformance implementation: 1) Use where clauses for type constraints, 2) Extend generic types...
Self Requirements: 1) Use Self keyword in protocol definitions, 2) Enable type-safe method chaining, 3) Implement...
Protocol Conformance in Extensions: 1) Add conformance to existing types, 2) Implement required methods and...
Type Erasure: 1) Hides concrete types behind protocols, 2) Implements wrapper types, 3) Manages associated type...
Protocol-Based Dependency Injection: 1) Define service protocols, 2) Implement mock conformance for testing, 3) Use...
Protocol-Based Configuration: 1) Define configuration protocols, 2) Implement default configurations, 3) Support...
Protocol-Based Validation: 1) Define validation protocols, 2) Implement reusable validation logic, 3) Compose...
Protocol-Based Error Handling: 1) Define error protocols, 2) Implement error type hierarchies, 3) Handle error...
Protocol-Based State Management: 1) Define state protocols, 2) Implement state transitions, 3) Handle state...
Protocol-Based Testing: 1) Create testable interfaces, 2) Implement mock objects, 3) Support test doubles, 4) Enable...
Protocol-Based Networking: 1) Define network service protocols, 2) Implement request/response handling, 3) Manage...
Protocol-Based View Controllers: 1) Define view controller behaviors, 2) Implement reusable functionality, 3)...
Protocol-Oriented Programming (POP) in Swift: 1) Focuses on defining protocols and protocol extensions, 2) Favors...
Protocol Extensions enable: 1) Adding default implementations to protocols, 2) Extending functionality without...
Associated Types: 1) Define placeholder names for types used in protocols, 2) Allow protocols to be generic, 3)...
Protocol Composition includes: 1) Combining multiple protocols using & operator, 2) Creating type constraints with...
Protocol Requirements include: 1) Property requirements (get/set), 2) Method requirements with signatures, 3)...
Protocol Inheritance enables: 1) Creating protocol hierarchies, 2) Inheriting requirements from other protocols, 3)...
Type Constraints in protocol extensions: 1) Limit extension applicability to specific types, 2) Use 'where' clause...
Generic Protocols implementation: 1) Using associated types for generics, 2) Constraining associated types, 3)...
Protocol Witness Table: 1) Stores protocol conformance information, 2) Maps protocol requirements to...
Optional Protocol Requirements: 1) Marked with @objc optional, 2) Only available in Objective-C compatible...
Protocol Extension Method Dispatch: 1) Static dispatch for extension methods, 2) Dynamic dispatch for protocol...
Protocol-Oriented Design practices: 1) Start with protocols before implementations, 2) Use protocol composition for...
Conditional Conformance implementation: 1) Use where clauses for type constraints, 2) Extend generic types...
Self Requirements: 1) Use Self keyword in protocol definitions, 2) Enable type-safe method chaining, 3) Implement...
Protocol Conformance in Extensions: 1) Add conformance to existing types, 2) Implement required methods and...
Type Erasure: 1) Hides concrete types behind protocols, 2) Implements wrapper types, 3) Manages associated type...
Protocol-Based Dependency Injection: 1) Define service protocols, 2) Implement mock conformance for testing, 3) Use...
Protocol-Based Configuration: 1) Define configuration protocols, 2) Implement default configurations, 3) Support...
Protocol-Based Validation: 1) Define validation protocols, 2) Implement reusable validation logic, 3) Compose...
Protocol-Based Error Handling: 1) Define error protocols, 2) Implement error type hierarchies, 3) Handle error...
Protocol-Based State Management: 1) Define state protocols, 2) Implement state transitions, 3) Handle state...
Protocol-Based Testing: 1) Create testable interfaces, 2) Implement mock objects, 3) Support test doubles, 4) Enable...
Protocol-Based Networking: 1) Define network service protocols, 2) Implement request/response handling, 3) Manage...
Protocol-Based View Controllers: 1) Define view controller behaviors, 2) Implement reusable functionality, 3)...
Protocol-Based Data Sources: 1) Define data source protocols, 2) Implement data fetching logic, 3) Handle data...
Protocol-Based Animation: 1) Define animation protocols, 2) Implement reusable animations, 3) Handle animation...
Protocol-Based Persistence: 1) Define storage protocols, 2) Implement various storage backends, 3) Handle data...
Protocol-Based Resource Management: 1) Define resource protocols, 2) Implement resource loading, 3) Handle resource...
Protocol-Based Event Handling: 1) Define event protocols, 2) Implement event dispatching, 3) Handle event...
Protocol-Based Logging: 1) Define logging protocols, 2) Implement different log levels, 3) Handle log formatting, 4)...
Protocol-Based Middleware: 1) Define middleware protocols, 2) Implement request/response chain, 3) Handle...
Swift Concurrency introduces: 1) async/await for asynchronous code, 2) Structured concurrency with tasks, 3) Actor...
Actors provide: 1) Data race protection through isolation, 2) Synchronized access to mutable state, 3) Serial...
async/await provides: 1) Structured approach to asynchronous code, 2) Elimination of completion handler pyramids, 3)...
Tasks represent: 1) Units of asynchronous work, 2) Structured task hierarchies, 3) Cancellation support, 4) Priority...
GCD features: 1) Queue-based task execution, 2) Serial and concurrent queues, 3) Quality of service levels, 4)...
AsyncSequence/AsyncStream provide: 1) Asynchronous iteration over values, 2) Back-pressure handling, 3) Cancellation...
Task cancellation involves: 1) Checking cancellation status, 2) Responding to cancellation, 3) Propagating...
@MainActor ensures: 1) Code runs on main thread, 2) UI updates are safe, 3) State isolation for main thread, 4)...
Concurrent data access patterns: 1) Using actors for isolation, 2) Implementing thread-safe properties, 3)...
Task Groups enable: 1) Parallel task execution, 2) Dynamic task creation, 3) Result collection, 4) Error handling,...
Async testing includes: 1) Using async test methods, 2) Implementing expectations, 3) Testing actor isolation, 4)...
Sendable protocol ensures: 1) Safe cross-actor data transfer, 2) Value type conformance, 3) Thread-safe reference...
Concurrent collections require: 1) Thread-safe access methods, 2) Atomic operations, 3) Lock-free algorithms, 4)...
AsyncThrowingStream provides: 1) Asynchronous error handling, 2) Cancellation support, 3) Back-pressure management,...
Deadlock prevention includes: 1) Using structured concurrency, 2) Implementing proper lock ordering, 3) Avoiding...
Concurrent error handling: 1) Using async throws functions, 2) Implementing error propagation, 3) Handling task...
Custom executors require: 1) Conforming to Executor protocol, 2) Managing task scheduling, 3) Implementing priority...
Task priority management: 1) Setting priority levels, 2) Priority inheritance, 3) Priority escalation, 4) QoS...
Async properties require: 1) Using async get keyword, 2) Managing property dependencies, 3) Handling cancellation,...
Background task patterns: 1) Task prioritization, 2) Resource management, 3) State preservation, 4) Background...
Concurrent networking: 1) Using async URLSession, 2) Implementing request grouping, 3) Managing timeouts, 4)...
AsyncLetBinding enables: 1) Parallel async operations, 2) Result dependency management, 3) Structured concurrency,...
Rate limiting implementation: 1) Token bucket algorithm, 2) Time-based limiting, 3) Queue-based throttling, 4)...
Concurrent memory management: 1) Weak reference usage, 2) Proper closure capture, 3) Resource cleanup, 4) Cycle...
Concurrent state machines: 1) Actor-based state management, 2) Thread-safe transitions, 3) Event handling, 4) State...
Actors provide: 1) Data race protection through isolation, 2) Synchronized access to mutable state, 3) Serial...
async/await provides: 1) Structured approach to asynchronous code, 2) Elimination of completion handler pyramids, 3)...
Tasks represent: 1) Units of asynchronous work, 2) Structured task hierarchies, 3) Cancellation support, 4) Priority...
GCD features: 1) Queue-based task execution, 2) Serial and concurrent queues, 3) Quality of service levels, 4)...
AsyncSequence/AsyncStream provide: 1) Asynchronous iteration over values, 2) Back-pressure handling, 3) Cancellation...
Task cancellation involves: 1) Checking cancellation status, 2) Responding to cancellation, 3) Propagating...
@MainActor ensures: 1) Code runs on main thread, 2) UI updates are safe, 3) State isolation for main thread, 4)...
Concurrent data access patterns: 1) Using actors for isolation, 2) Implementing thread-safe properties, 3)...
Task Groups enable: 1) Parallel task execution, 2) Dynamic task creation, 3) Result collection, 4) Error handling,...
Async testing includes: 1) Using async test methods, 2) Implementing expectations, 3) Testing actor isolation, 4)...
Sendable protocol ensures: 1) Safe cross-actor data transfer, 2) Value type conformance, 3) Thread-safe reference...
Concurrent collections require: 1) Thread-safe access methods, 2) Atomic operations, 3) Lock-free algorithms, 4)...
AsyncThrowingStream provides: 1) Asynchronous error handling, 2) Cancellation support, 3) Back-pressure management,...
Deadlock prevention includes: 1) Using structured concurrency, 2) Implementing proper lock ordering, 3) Avoiding...
Concurrent error handling: 1) Using async throws functions, 2) Implementing error propagation, 3) Handling task...
Custom executors require: 1) Conforming to Executor protocol, 2) Managing task scheduling, 3) Implementing priority...
Task priority management: 1) Setting priority levels, 2) Priority inheritance, 3) Priority escalation, 4) QoS...
Async properties require: 1) Using async get keyword, 2) Managing property dependencies, 3) Handling cancellation,...
Background task patterns: 1) Task prioritization, 2) Resource management, 3) State preservation, 4) Background...
Concurrent networking: 1) Using async URLSession, 2) Implementing request grouping, 3) Managing timeouts, 4)...
AsyncLetBinding enables: 1) Parallel async operations, 2) Result dependency management, 3) Structured concurrency,...
Rate limiting implementation: 1) Token bucket algorithm, 2) Time-based limiting, 3) Queue-based throttling, 4)...
Concurrent memory management: 1) Weak reference usage, 2) Proper closure capture, 3) Resource cleanup, 4) Cycle...
Concurrent state machines: 1) Actor-based state management, 2) Thread-safe transitions, 3) Event handling, 4) State...
Swift error handling includes: 1) try-catch blocks for throwing functions, 2) Result type for functional error...
Custom Error implementation includes: 1) Conforming to Error protocol, 2) Defining error cases with enums, 3) Adding...
Xcode debugging tools include: 1) LLDB debugger commands, 2) Breakpoints with conditions, 3) Variable inspection and...
Async error handling involves: 1) Using async throws functions, 2) Implementing Task error handling, 3) Managing...
Key differences include: 1) fatalError always terminates, used for unimplemented code, 2) assert only runs in debug...
Result type implementation: 1) Define success/failure cases, 2) Map and flatMap operations, 3) Error type...
Logging best practices: 1) Using OSLog for system integration, 2) Implementing log levels, 3) Structured logging...
Memory debugging includes: 1) Using Instruments for leaks, 2) Memory graph debugger usage, 3) Heap debugging tools,...
Error propagation patterns: 1) Using rethrows keyword, 2) Error transformation, 3) Error aggregation, 4)...
Debug-only implementation: 1) Using #if DEBUG directive, 2) Debug-only extensions, 3) Conditional compilation, 4)...
Error recovery strategies: 1) Retry mechanisms, 2) Fallback values, 3) Graceful degradation, 4) State restoration,...
Network debugging includes: 1) Charles Proxy integration, 2) URLSession debugging, 3) Network Link Conditioner, 4)...
Crash reporting techniques: 1) Symbolication process, 2) Crash log analysis, 3) Exception handling, 4) Stack trace...
Validation error handling: 1) Custom validation rules, 2) Error aggregation, 3) Field-level errors, 4) Error message...
SwiftUI debugging includes: 1) Preview debugging, 2) View hierarchy inspection, 3) State monitoring, 4) Layout...
Protocol error handling: 1) Error type constraints, 2) Protocol error requirements, 3) Default implementations, 4)...
Error localization practices: 1) LocalizedError implementation, 2) String catalog usage, 3) Error message templates,...
Performance debugging: 1) Time Profiler usage, 2) Instruments analysis, 3) Energy logging, 4) Memory allocation...
Async error patterns: 1) Task error handling, 2) Actor isolation errors, 3) Structured concurrency errors, 4)...
Error middleware: 1) Error interception, 2) Error transformation chain, 3) Logging middleware, 4) Recovery...
Database debugging: 1) SQLite debugging tools, 2) Core Data debugging, 3) Migration debugging, 4) Query...
DI error handling: 1) Resolution errors, 2) Circular dependency detection, 3) Optional dependency handling, 4)...
Memory leak debugging: 1) Instruments leaks tool, 2) Memory graph debugger, 3) Allocation tracking, 4) Retain cycle...
Error boundary patterns: 1) Error containment, 2) Fallback UI, 3) Error recovery UI, 4) State preservation, 5) Error...
Error testing practices: 1) Error case coverage, 2) Recovery testing, 3) Async error testing, 4) Mock error...
Swift error handling includes: 1) try-catch blocks for throwing functions, 2) Result type for functional error...
Custom Error implementation includes: 1) Conforming to Error protocol, 2) Defining error cases with enums, 3) Adding...
Xcode debugging tools include: 1) LLDB debugger commands, 2) Breakpoints with conditions, 3) Variable inspection and...
Async error handling involves: 1) Using async throws functions, 2) Implementing Task error handling, 3) Managing...
Key differences include: 1) fatalError always terminates, used for unimplemented code, 2) assert only runs in debug...
Result type implementation: 1) Define success/failure cases, 2) Map and flatMap operations, 3) Error type...
Logging best practices: 1) Using OSLog for system integration, 2) Implementing log levels, 3) Structured logging...
Memory debugging includes: 1) Using Instruments for leaks, 2) Memory graph debugger usage, 3) Heap debugging tools,...
Error propagation patterns: 1) Using rethrows keyword, 2) Error transformation, 3) Error aggregation, 4)...
Debug-only implementation: 1) Using #if DEBUG directive, 2) Debug-only extensions, 3) Conditional compilation, 4)...
Error recovery strategies: 1) Retry mechanisms, 2) Fallback values, 3) Graceful degradation, 4) State restoration,...
Network debugging includes: 1) Charles Proxy integration, 2) URLSession debugging, 3) Network Link Conditioner, 4)...
Crash reporting techniques: 1) Symbolication process, 2) Crash log analysis, 3) Exception handling, 4) Stack trace...
Validation error handling: 1) Custom validation rules, 2) Error aggregation, 3) Field-level errors, 4) Error message...
SwiftUI debugging includes: 1) Preview debugging, 2) View hierarchy inspection, 3) State monitoring, 4) Layout...
Protocol error handling: 1) Error type constraints, 2) Protocol error requirements, 3) Default implementations, 4)...
Error localization practices: 1) LocalizedError implementation, 2) String catalog usage, 3) Error message templates,...
Performance debugging: 1) Time Profiler usage, 2) Instruments analysis, 3) Energy logging, 4) Memory allocation...
Async error patterns: 1) Task error handling, 2) Actor isolation errors, 3) Structured concurrency errors, 4)...
Error middleware: 1) Error interception, 2) Error transformation chain, 3) Logging middleware, 4) Recovery...
Database debugging: 1) SQLite debugging tools, 2) Core Data debugging, 3) Migration debugging, 4) Query...
DI error handling: 1) Resolution errors, 2) Circular dependency detection, 3) Optional dependency handling, 4)...
Memory leak debugging: 1) Instruments leaks tool, 2) Memory graph debugger, 3) Allocation tracking, 4) Retain cycle...
Error boundary patterns: 1) Error containment, 2) Fallback UI, 3) Error recovery UI, 4) State preservation, 5) Error...
Error testing practices: 1) Error case coverage, 2) Recovery testing, 3) Async error testing, 4) Mock error...
Key optimization strategies include: 1) Using value types for better copy semantics, 2) Implementing copy-on-write...
ARC performance considerations include: 1) Minimizing strong reference cycles, 2) Using weak and unowned references...
Collection optimization includes: 1) Choosing appropriate collection types, 2) Reserving capacity upfront, 3) Using...
Memory optimization techniques: 1) Using value types appropriately, 2) Implementing proper caching strategies, 3)...
Compile time optimization includes: 1) Reducing use of generics when unnecessary, 2) Optimizing complex type...
Network optimization includes: 1) Implementing proper caching strategies, 2) Using URLSession configuration...
UI performance optimization: 1) Implementing view reuse, 2) Optimizing layout calculations, 3) Managing main thread...
String optimization includes: 1) Using string interpolation efficiently, 2) Managing string concatenation, 3)...
Protocol optimization techniques: 1) Using protocol composition efficiently, 2) Managing protocol inheritance...
Data persistence optimization: 1) Choosing appropriate storage solution, 2) Implementing efficient serialization, 3)...
Algorithm optimization includes: 1) Choosing appropriate data structures, 2) Implementing efficient sorting methods,...
Image optimization techniques: 1) Implementing proper image caching, 2) Managing image compression, 3) Using...
Closure optimization includes: 1) Managing capture lists efficiently, 2) Understanding closure overhead, 3)...
Concurrent operation optimization: 1) Using appropriate dispatch queues, 2) Managing thread safety, 3) Implementing...
Binary size optimization: 1) Managing dependency inclusion, 2) Using proper optimization flags, 3) Implementing dead...
Swift differs from Objective-C in several key aspects: 1) Type safety and inference, 2) Optionals for safe handling of nil values, 3) Modern syntax without @ symbols, 4) Tuples and multiple return values, 5) Protocol-oriented programming approach, 6) Built-in error handling, 7) Advanced pattern matching, 8) Generics support, 9) No header files needed, and 10) Better memory management with ARC.
Optionals in Swift represent values that may or may not exist. Unwrapping methods include: 1) Force unwrapping (!), 2) Optional binding (if let/guard let), 3) Optional chaining (?.), 4) Nil coalescing operator (??), 5) Implicit unwrapping (!). Best practices include avoiding force unwrapping and using optional binding for safety. Optional binding provides safe unwrapping with control flow, while optional chaining allows safe access to properties and methods.
Value types (struct, enum) create a new copy when assigned, while reference types (class) share the same instance. Key differences: 1) Value types are copied on assignment, 2) Reference types are passed by reference, 3) Value types support mutating keyword, 4) Reference types can inherit, 5) Value types are preferred for data models, 6) Reference types are better for shared resources. Understanding this difference is crucial for memory management and program design.
Type Inference allows Swift to automatically deduce variable types: 1) Compiler analyzes initialization value, 2) Determines appropriate type at compile time, 3) Reduces explicit type annotations, 4) Works with complex types and generics, 5) Maintains type safety, 6) Improves code readability. While convenient, explicit type annotation can improve code clarity and compile time.
Closures are self-contained blocks of functionality: 1) Can capture and store references to variables/constants, 2) Support trailing closure syntax, 3) Have shorthand argument names, 4) Can be used as function parameters, 5) Support multiple closure parameters, 6) Allow for escaping and non-escaping variants. They're commonly used in async operations, callbacks, and higher-order functions.
Pattern Matching in Swift includes: 1) Switch statement patterns, 2) Case let bindings, 3) Where clauses for conditions, 4) Tuple pattern matching, 5) Type casting patterns (is, as), 6) Expression pattern matching. It's powerful for control flow and data extraction, especially with enums and complex types.
Property Wrappers add behavior to properties: 1) Encapsulate common property patterns, 2) Reduce boilerplate code, 3) Support custom getter/setter logic, 4) Enable property observation, 5) Allow for computed property behavior, 6) Support dependency injection. Common examples include @State in SwiftUI and custom wrappers for persistence.
Swift provides five access levels: 1) open - accessible outside module, can be subclassed, 2) public - accessible outside module, 3) internal - default, module-level access, 4) fileprivate - file-level access, 5) private - scope-level access. Access control helps enforce encapsulation and defines module interface boundaries.
Extensions add functionality to existing types: 1) Add computed properties, 2) Define instance/type methods, 3) Provide new initializers, 4) Make types conform to protocols, 5) Add nested types, 6) Organize code by functionality. They can't override existing functionality but can add new features to any type, including system types.
Generics enable flexible, reusable code: 1) Type-safe abstract types, 2) Generic functions and types, 3) Type constraints and protocols, 4) Associated types in protocols, 5) Generic where clauses, 6) Type erasure concepts. They reduce code duplication while maintaining type safety and enabling collection types.
Swift supports various property types: 1) Stored properties (var/let), 2) Computed properties (get/set), 3) Property observers (willSet/didSet), 4) Type properties (static/class), 5) Lazy properties (lazy var), 6) Property wrappers (@propertyWrapper). Each serves different purposes in managing object state and behavior.
Method Dispatch determines how methods are called: 1) Static dispatch for value types, 2) Dynamic dispatch for class methods, 3) Table dispatch for protocol methods, 4) Direct dispatch optimizations, 5) Message dispatch in Objective-C interop, 6) Performance implications of each. Understanding dispatch affects performance and inheritance behavior.
Subscripts provide shorthand access to collections: 1) Custom getter/setter syntax, 2) Multiple parameter support, 3) Type and instance subscripts, 4) Overloading capabilities, 5) Generic subscripts, 6) Optional return types. They're commonly used in collections and custom sequence types.
Swift Strings are unique: 1) Unicode-correct by default, 2) Value type semantics, 3) Character-based iteration, 4) Complex grapheme cluster handling, 5) String interpolation features, 6) Performance optimizations. Understanding these differences is crucial for proper text handling.
Tuples group multiple values: 1) Named or unnamed elements, 2) Multiple return values from functions, 3) Pattern matching support, 4) Decomposition in assignments, 5) Type inference with tuples, 6) Limited to fixed size. They're useful for temporary grouping of related values without defining a formal structure.
Swift error handling includes: 1) Error protocol conformance, 2) throws keyword for error-prone functions, 3) do-try-catch blocks, 4) try? and try! operators, 5) Error propagation through functions, 6) Custom error types and handling, 7) Result type for functional error handling, 8) async/await error handling integration. This provides type-safe error management.
Keypaths provide type-safe references to properties: 1) KeyPath for read-only properties, 2) WritableKeyPath for mutable properties, 3) ReferenceWritableKeyPath for reference types, 4) Key path expressions with \, 5) Key path subscripting, 6) Dynamic member lookup integration. They're useful for dynamic property access and functional programming patterns.
Static vs Class methods differ in: 1) static cannot be overridden in subclasses, 2) class allows overriding in subclasses, 3) static is resolved at compile time, 4) class uses dynamic dispatch, 5) static is preferred for utility functions, 6) class is used when inheritance is needed. This affects inheritance and method dispatch behavior.
Swift memory management involves: 1) Automatic Reference Counting (ARC), 2) Strong, weak, and unowned references, 3) Reference cycles prevention, 4) Value type stack allocation, 5) Reference type heap allocation, 6) Capture lists in closures. Understanding these concepts is crucial for preventing memory leaks.
Result Builders enable DSL creation: 1) Custom syntax for building complex objects, 2) SwiftUI view construction, 3) Compile-time building of results, 4) Support for conditional and loop statements, 5) Custom builder attributes, 6) Type-safe DSL creation. They're fundamental to SwiftUI's declarative syntax.
Swift initialization includes: 1) Designated initializers, 2) Convenience initializers, 3) Required initializers, 4) Failable initializers, 5) Two-phase initialization process, 6) Initializer inheritance rules. Proper initialization ensures type safety and object validity.
Lazy properties provide delayed initialization: 1) Initialized only when first accessed, 2) Must be variable (var), 3) Not thread-safe by default, 4) Useful for expensive computations, 5) Can reference self safely, 6) Cannot have property observers. They optimize memory usage and startup time.
Type casting mechanisms include: 1) is operator for type checking, 2) as? for conditional downcasting, 3) as! for forced downcasting, 4) as for upcasting, 5) Type casting patterns in switch, 6) Any and AnyObject handling. Safe type casting is crucial for runtime type safety.
Variadic parameters accept multiple values: 1) Denoted by ... after type, 2) Treated as array inside function, 3) Only one per function signature, 4) Can be combined with regular parameters, 5) Support type inference, 6) Useful for flexible APIs. They enable flexible function parameter counts.
Operator overloading features: 1) Custom operator definitions, 2) Precedence group specification, 3) Infix, prefix, and postfix operators, 4) Protocol conformance for operators, 5) Type-safe operator implementation, 6) Standard library operator patterns. This enables custom operations for user-defined types.
Property observers monitor changes: 1) willSet executes before change, 2) didSet executes after change, 3) Access to old/new values, 4) Cannot be used with computed properties, 5) Support for inheritance, 6) Useful for UI updates and validation. They enable reactive property behavior.
String interpolation features: 1) Basic value insertion with \(), 2) Custom interpolation definitions, 3) Formatted value presentation, 4) Expression evaluation, 5) Multi-line string support, 6) Localization integration. It provides flexible string formatting and composition.
Phantom types provide compile-time safety: 1) Generic type parameters unused at runtime, 2) Type-level state encoding, 3) Compile-time validation, 4) Unit type safety, 5) State machine implementation, 6) API design constraints. They enable advanced type-safety patterns.
Protocol requirements include: 1) Method signatures, 2) Property specifications, 3) Static/class requirements, 4) Optional requirements with @objc, 5) Associated type constraints, 6) Default implementations via extensions. This enables flexible protocol-oriented design.
Type casting in class hierarchies involves: 1) Using 'is' for type checking, 2) 'as?' for conditional downcasting, 3) 'as!' for forced downcasting, 4) 'as' for upcasting, 5) Type casting patterns in switch statements, 6) Handling inheritance relationships, 7) Protocol conformance checking, 8) Runtime type determination.
Nested types in Swift: 1) Define types within other types, 2) Provide namespace scoping, 3) Support access control relationships, 4) Enable related type grouping, 5) Support generic type constraints, 6) Allow internal implementation hiding, 7) Improve code organization, 8) Support builder pattern implementation.
Observer pattern implementation includes: 1) Using delegation, 2) NotificationCenter usage, 3) Key-Value Observing (KVO), 4) Custom observer protocols, 5) Combine framework integration, 6) Weak reference handling, 7) Event broadcasting mechanisms, 8) Memory management considerations.
Convenience initializers: 1) Provide alternative initialization patterns, 2) Must call designated initializer, 3) Support initialization abstraction, 4) Reduce code duplication, 5) Enable default parameter values, 6) Support initialization delegation, 7) Improve initialization readability, 8) Maintain initialization safety.
Composition vs Inheritance considerations: 1) Favor composition over inheritance, 2) Use protocols for shared behavior, 3) Implement delegation patterns, 4) Consider value type composition, 5) Use generic constraints, 6) Implement dependency injection, 7) Handle state sharing, 8) Manage object lifecycle.
Factory method patterns include: 1) Static factory methods, 2) Factory protocol implementation, 3) Generic factory methods, 4) Abstract factory pattern, 5) Factory method inheritance, 6) Dependency injection support, 7) Error handling in factories, 8) Configuration-based creation.
Key differences include: 1) Classes are reference types while structures are value types, 2) Classes support inheritance while structures don't, 3) Classes have deinitializers, structures don't, 4) Classes allow reference counting with ARC, 5) Structures automatically get a memberwise initializer, 6) Classes can participate in type casting, 7) Structures are preferred for data models in Swift for better performance and thread safety, 8) Classes are better for shared resources and when identity is important.
Swift inheritance features include: 1) Single inheritance only (no multiple inheritance), 2) Method overriding using 'override' keyword, 3) Preventing overrides with 'final' keyword, 4) Super class initialization requirements, 5) Property override rules, 6) Access control in inheritance hierarchy, 7) Protocol inheritance is allowed and can be multiple, 8) Required initializers in subclasses. Limitations include no multiple class inheritance and strict initialization rules.
Type methods and properties belong to the type itself: 1) Declared using 'static' or 'class' keywords, 2) 'static' prevents override in subclasses, 3) 'class' allows override in subclasses, 4) Can access other type properties and methods, 5) Cannot access instance methods or properties directly, 6) Useful for utility functions and shared resources, 7) Support computed and stored properties, 8) Thread-safe by default.
Swift supports polymorphism through: 1) Inheritance-based method overriding, 2) Protocol conformance for interface polymorphism, 3) Generic type parameters, 4) Type casting and runtime checks, 5) Dynamic dispatch for class methods, 6) Static dispatch optimization when possible, 7) Protocol extensions for default implementations, 8) Associated types in protocols for type relationships.
Initializers in Swift classes serve multiple purposes: 1) Designated initializers as primary initializers, 2) Convenience initializers for initialization shortcuts, 3) Required initializers that must be implemented by subclasses, 4) Failable initializers that might return nil, 5) Two-phase initialization process, 6) Initializer inheritance rules, 7) Super class initialization requirements, 8) Automatic initializer inheritance conditions.
Deinitializers in Swift: 1) Declared using 'deinit' keyword, 2) Called automatically when object is deallocated, 3) Only available in classes, not structures, 4) Cannot be called directly, 5) No parameters or parentheses, 6) Used for cleanup operations, 7) Important for resource management, 8) Called in reverse order of initialization for inheritance hierarchies.
Computed properties: 1) Calculate value dynamically rather than storing it, 2) Can have getter and optional setter, 3) Useful for derived values, 4) Cannot use property observers, 5) Can be overridden in subclasses, 6) Support access control, 7) Can depend on other properties, 8) Useful for encapsulation and maintaining consistency. They're ideal when a property's value depends on other properties.
Method dispatch in Swift classes involves: 1) Dynamic dispatch by default for instance methods, 2) Static dispatch for final methods, 3) Table dispatch for protocol methods, 4) Message dispatch for @objc methods, 5) Dispatch optimization by compiler, 6) Override table maintenance, 7) Performance implications of different dispatch types, 8) Direct dispatch for private methods.
Access control provides: 1) Encapsulation of implementation details, 2) Interface-based programming, 3) Five access levels (open, public, internal, fileprivate, private), 4) Module-level boundaries, 5) Subclass and override control, 6) Property getter/setter control, 7) Protocol conformance requirements, 8) Framework API design control. It's crucial for maintaining proper encapsulation and API design.
Reference cycle management includes: 1) Using weak references for optional references, 2) Unowned references for non-optional references, 3) Closure capture lists, 4) Parent-child relationship considerations, 5) Delegate pattern implementation, 6) Property observer cleanup, 7) Deinitializer usage, 8) ARC (Automatic Reference Counting) understanding.
Property wrappers provide: 1) Reusable property behavior encapsulation, 2) Separation of concerns in property implementation, 3) Custom getter/setter logic, 4) State management patterns, 5) Validation and transformation logic, 6) Thread safety implementation, 7) Dependency injection patterns, 8) Observable property patterns.
Singleton implementation includes: 1) Static shared instance property, 2) Private initializer to prevent external creation, 3) Thread-safety considerations, 4) Lazy initialization support, 5) Property wrapper usage for singletons, 6) Testing considerations, 7) Dependency injection alternatives, 8) Access control implementation.
The 'final' keyword: 1) Prevents method overriding in subclasses, 2) Prevents class inheritance, 3) Enables compiler optimizations, 4) Improves method dispatch performance, 5) Helps maintain API contract, 6) Supports design by contract, 7) Reduces runtime overhead, 8) Important for framework design.
Method overloading allows: 1) Multiple methods with same name but different parameters, 2) Return type differentiation, 3) Generic type constraints, 4) Parameter label variations, 5) Default parameter values, 6) Type-specific implementations, 7) Operator overloading, 8) Protocol requirement satisfaction.
Inheritance best practices include: 1) Favoring composition over inheritance, 2) Using protocols for shared behavior, 3) Keeping inheritance hierarchies shallow, 4) Documenting inheritance requirements, 5) Proper use of override keyword, 6) Access control consideration, 7) Initialization pattern implementation, 8) Memory management awareness.
Decorator pattern implementation includes: 1) Protocol-based interface definition, 2) Base class implementation, 3) Decorator class hierarchy, 4) Composition over inheritance, 5) Dynamic behavior addition, 6) Property forwarding, 7) Method delegation, 8) Stack-based decoration.
Dependency injection practices include: 1) Constructor injection, 2) Property injection, 3) Method injection, 4) Protocol-based dependencies, 5) Container management, 6) Scope management, 7) Testing considerations, 8) Circular dependency prevention.
Class cluster implementation involves: 1) Abstract factory pattern usage, 2) Private subclass creation, 3) Public interface definition, 4) Factory method implementation, 5) Type-specific optimization, 6) Initialization pattern design, 7) Inheritance hierarchy management, 8) API simplification.
Builder pattern implementation includes: 1) Method chaining support, 2) Default value handling, 3) Validation logic, 4) Immutable object creation, 5) Complex object construction, 6) Optional parameter management, 7) Fluent interface design, 8) Result builder integration.
Thread-safe implementation includes: 1) Serial queue usage, 2) Property wrapper implementation, 3) Atomic operations, 4) Lock mechanisms, 5) Barrier flags, 6) Read-write synchronization, 7) Thread-safe property access, 8) Concurrent queue management.
ARC automatically manages memory by: 1) Tracking strong references to class instances, 2) Deallocating memory when reference count reaches zero, 3) Managing reference cycles through weak and unowned references, 4) Handling retain/release operations at compile time, 5) Working only with reference types (classes), not value types (structs, enums). ARC eliminates manual memory management while ensuring deterministic cleanup.
Key differences include: 1) weak references are optional and can become nil, 2) unowned references are non-optional and assume always valid, 3) weak is used when reference might become nil during lifetime, 4) unowned is used when reference never becomes nil while instance exists, 5) weak requires explicit unwrapping, 6) unowned assumes permanent valid reference. Choose weak for optional references and unowned for guaranteed valid references.
Retain cycle identification and fixing involves: 1) Using Memory Graph Debugger in Xcode, 2) Implementing weak or unowned references appropriately, 3) Using capture lists in closures [weak self], 4) Proper delegate pattern implementation, 5) Breaking parent-child cyclic references, 6) Memory leak instruments usage. Regular testing and monitoring help prevent memory leaks.
Closure capture lists: 1) Define how values are captured by closures, 2) Use [weak self] or [unowned self] to prevent retain cycles, 3) Allow multiple captured variables, 4) Support value type capturing, 5) Enable explicit capture rules, 6) Help manage reference cycles in asynchronous operations. Proper use prevents memory leaks in closure-heavy code.
Strong vs weak references: 1) Strong references increment reference count, weak don't, 2) Strong references keep objects alive, weak allow deallocation, 3) Strong is the default reference type, 4) Weak must be optional variables, 5) Strong creates ownership relationship, 6) Weak used for delegate patterns and breaking retain cycles. Understanding this difference is crucial for proper memory management.
ARC and deinitializers: 1) Deinit called automatically when reference count reaches zero, 2) Only available in class types, 3) Can't be called directly, 4) Used for cleanup operations, 5) Called in reverse order of inheritance chain, 6) Helps verify proper memory management. Deinitializers are crucial for resource cleanup and debugging memory issues.
Memory management best practices: 1) Use value types when possible, 2) Implement proper weak/unowned references, 3) Break retain cycles in closures, 4) Use proper delegate patterns, 5) Monitor memory usage with instruments, 6) Implement deinitializers for cleanup. Regular testing and monitoring ensure optimal memory usage.
Async operation memory management: 1) Use capture lists in closures, 2) Implement proper cancellation handling, 3) Break retain cycles in completion handlers, 4) Handle self references carefully, 5) Clean up resources on cancellation, 6) Monitor async operation lifecycle. Careful management prevents leaks in async code.
Autoreleasepool usage: 1) Manages temporary objects memory, 2) Useful in loops processing many objects, 3) Helps reduce peak memory usage, 4) Important for command-line tools, 5) Handles bridged Objective-C objects, 6) Provides explicit memory release points. Used less in Swift than Objective-C but still important for specific scenarios.
Collection memory management: 1) Value type collections copy on write, 2) Reference type elements managed by ARC, 3) Proper cleanup of collection elements, 4) Memory efficient array slicing, 5) Capacity management for arrays, 6) Collection lifecycle management. Understanding collection behavior ensures efficient memory usage.
Reference counting: 1) Tracks number of references to objects, 2) Increments count for new references, 3) Decrements count when references removed, 4) Deallocates when count reaches zero, 5) Handles in compile time by ARC, 6) Only applies to class instances. Forms the basis of Swift's memory management system.
Memory leak debugging: 1) Use Xcode Memory Graph Debugger, 2) Implement Instruments for leak detection, 3) Monitor object deallocation, 4) Check retain cycles, 5) Analyze memory usage patterns, 6) Use runtime memory debugging tools. Regular debugging prevents memory issues in production.
Delegate memory management: 1) Use weak references for delegates, 2) Prevent retain cycles, 3) Handle delegate lifecycle properly, 4) Clean up delegate references, 5) Consider multiple delegate patterns, 6) Implement proper delegate cleanup. Proper delegate management prevents common memory leaks.
Closure memory management: 1) Captures referenced variables strongly by default, 2) Uses capture lists for custom capturing, 3) Manages closure lifecycle, 4) Handles async closure memory, 5) Prevents retain cycles with weak self, 6) Cleans up captured references. Understanding closure capture rules is crucial.
Copy-on-write impact: 1) Optimizes value type performance, 2) Shares memory until modification, 3) Creates copies only when needed, 4) Reduces memory usage, 5) Applies to standard library collections, 6) Balances safety and efficiency. Important optimization technique for value types.
Protocol-Oriented Programming (POP) in Swift: 1) Focuses on defining protocols and protocol extensions, 2) Favors composition over inheritance, 3) Enables multiple protocol inheritance, 4) Works with both value and reference types, 5) Provides default implementations through protocol extensions, 6) Promotes better code reuse and modularity. POP offers more flexibility than traditional OOP by avoiding deep inheritance hierarchies.
Protocol Extensions enable: 1) Adding default implementations to protocols, 2) Extending functionality without subclassing, 3) Providing computed properties and methods, 4) Implementing protocol requirements, 5) Constraining extensions to specific types, 6) Adding functionality to existing types. They're useful for sharing implementation across multiple types without inheritance.
Associated Types: 1) Define placeholder names for types used in protocols, 2) Allow protocols to be generic, 3) Specified using 'associatedtype' keyword, 4) Can have constraints and default types, 5) Resolved at compile time, 6) Enable type-safe collections and algorithms. They provide flexibility while maintaining type safety.
Protocol Composition includes: 1) Combining multiple protocols using & operator, 2) Creating type constraints with multiple requirements, 3) Using in function parameters and variables, 4) Implementing multiple protocol conformance, 5) Handling protocol conflicts, 6) Managing protocol hierarchy. Enables types to conform to multiple protocols simultaneously.
Protocol Requirements include: 1) Property requirements (get/set), 2) Method requirements with signatures, 3) Initializer requirements, 4) Static/class requirements, 5) Associated type requirements, 6) Optional requirements with @objc. They define the contract that conforming types must fulfill.
Protocol Inheritance enables: 1) Creating protocol hierarchies, 2) Inheriting requirements from other protocols, 3) Refining protocol requirements, 4) Combining related protocols, 5) Organizing protocol-based APIs, 6) Supporting protocol composition. Useful for building modular and extensible APIs.
Type Constraints in protocol extensions: 1) Limit extension applicability to specific types, 2) Use 'where' clause for constraints, 3) Constrain by conformance to other protocols, 4) Add type-specific functionality, 5) Override default implementations, 6) Enable specialized behavior. Provides fine-grained control over protocol extensions.
Generic Protocols implementation: 1) Using associated types for generics, 2) Constraining associated types, 3) Creating generic protocol extensions, 4) Handling type inference, 5) Managing protocol composition with generics, 6) Implementing generic requirements. Enables creation of flexible, reusable protocol definitions.
Protocol Witness Table: 1) Stores protocol conformance information, 2) Maps protocol requirements to implementations, 3) Created at compile time, 4) Handles dynamic dispatch for protocols, 5) Manages associated type resolution, 6) Optimizes protocol method calls. Critical for protocol performance and functionality.
Optional Protocol Requirements: 1) Marked with @objc optional, 2) Only available in Objective-C compatible protocols, 3) Require runtime checking, 4) Handle unimplemented requirements safely, 5) Provide fallback behavior, 6) Support backward compatibility. Useful for creating flexible protocol interfaces.
Protocol Extension Method Dispatch: 1) Static dispatch for extension methods, 2) Dynamic dispatch for protocol requirements, 3) Resolution rules for conflicts, 4) Extension method overriding behavior, 5) Interaction with class inheritance, 6) Performance implications. Understanding dispatch behavior is crucial for correct implementation.
Protocol-Oriented Design practices: 1) Start with protocols before implementations, 2) Use protocol composition for modularity, 3) Leverage protocol extensions for default behavior, 4) Keep protocols focused and single-purpose, 5) Use associated types for flexibility, 6) Consider value types first. Promotes maintainable and flexible code design.
Conditional Conformance implementation: 1) Use where clauses for type constraints, 2) Extend generic types conditionally, 3) Implement requirements based on conditions, 4) Handle nested type conformance, 5) Manage multiple conditional conformances, 6) Consider performance implications. Enables type-safe conditional behavior.
Self Requirements: 1) Use Self keyword in protocol definitions, 2) Enable type-safe method chaining, 3) Implement comparison protocols, 4) Handle type constraints with Self, 5) Support builder patterns, 6) Enable fluent interfaces. Important for type-safe protocol design.
Protocol Conformance in Extensions: 1) Add conformance to existing types, 2) Implement required methods and properties, 3) Handle associated type requirements, 4) Manage conditional conformance, 5) Deal with retroactive modeling, 6) Consider scope and access control. Enables adding protocol support to types you don't own.
Type Erasure: 1) Hides concrete types behind protocols, 2) Implements wrapper types, 3) Manages associated type requirements, 4) Enables protocol use in collections, 5) Handles protocol composition, 6) Maintains type safety. Used when concrete types need to be abstracted away.
Protocol-Based Dependency Injection: 1) Define service protocols, 2) Implement mock conformance for testing, 3) Use protocol composition for dependencies, 4) Handle optional dependencies, 5) Manage dependency lifecycle, 6) Support dependency configuration. Enables flexible and testable architecture.
Protocol-Based Configuration: 1) Define configuration protocols, 2) Implement default configurations, 3) Support configuration composition, 4) Handle environment-specific configs, 5) Manage configuration inheritance, 6) Enable runtime configuration changes. Useful for flexible system configuration.
Protocol-Based Validation: 1) Define validation protocols, 2) Implement reusable validation logic, 3) Compose validation rules, 4) Handle validation errors, 5) Support custom validation rules, 6) Enable validation chaining. Creates flexible and reusable validation systems.
Protocol-Based Error Handling: 1) Define error protocols, 2) Implement error type hierarchies, 3) Handle error propagation, 4) Support error recovery, 5) Manage error context, 6) Enable error transformation. Creates systematic error handling approaches.
Protocol-Based State Management: 1) Define state protocols, 2) Implement state transitions, 3) Handle state validation, 4) Manage state persistence, 5) Support state observation, 6) Enable state restoration. Creates flexible state management systems.
Protocol-Based Testing: 1) Create testable interfaces, 2) Implement mock objects, 3) Support test doubles, 4) Enable behavior verification, 5) Handle test isolation, 6) Manage test dependencies. Improves code testability and maintainability.
Protocol-Based Networking: 1) Define network service protocols, 2) Implement request/response handling, 3) Manage authentication, 4) Handle error scenarios, 5) Support response parsing, 6) Enable request configuration. Creates modular networking layers.
Protocol-Based View Controllers: 1) Define view controller behaviors, 2) Implement reusable functionality, 3) Support composition of features, 4) Handle view lifecycle, 5) Manage navigation flow, 6) Enable view controller testing. Improves view controller maintainability.
Protocol-Oriented Programming (POP) in Swift: 1) Focuses on defining protocols and protocol extensions, 2) Favors composition over inheritance, 3) Enables multiple protocol inheritance, 4) Works with both value and reference types, 5) Provides default implementations through protocol extensions, 6) Promotes better code reuse and modularity. POP offers more flexibility than traditional OOP by avoiding deep inheritance hierarchies.
Protocol Extensions enable: 1) Adding default implementations to protocols, 2) Extending functionality without subclassing, 3) Providing computed properties and methods, 4) Implementing protocol requirements, 5) Constraining extensions to specific types, 6) Adding functionality to existing types. They're useful for sharing implementation across multiple types without inheritance.
Associated Types: 1) Define placeholder names for types used in protocols, 2) Allow protocols to be generic, 3) Specified using 'associatedtype' keyword, 4) Can have constraints and default types, 5) Resolved at compile time, 6) Enable type-safe collections and algorithms. They provide flexibility while maintaining type safety.
Protocol Composition includes: 1) Combining multiple protocols using & operator, 2) Creating type constraints with multiple requirements, 3) Using in function parameters and variables, 4) Implementing multiple protocol conformance, 5) Handling protocol conflicts, 6) Managing protocol hierarchy. Enables types to conform to multiple protocols simultaneously.
Protocol Requirements include: 1) Property requirements (get/set), 2) Method requirements with signatures, 3) Initializer requirements, 4) Static/class requirements, 5) Associated type requirements, 6) Optional requirements with @objc. They define the contract that conforming types must fulfill.
Protocol Inheritance enables: 1) Creating protocol hierarchies, 2) Inheriting requirements from other protocols, 3) Refining protocol requirements, 4) Combining related protocols, 5) Organizing protocol-based APIs, 6) Supporting protocol composition. Useful for building modular and extensible APIs.
Type Constraints in protocol extensions: 1) Limit extension applicability to specific types, 2) Use 'where' clause for constraints, 3) Constrain by conformance to other protocols, 4) Add type-specific functionality, 5) Override default implementations, 6) Enable specialized behavior. Provides fine-grained control over protocol extensions.
Generic Protocols implementation: 1) Using associated types for generics, 2) Constraining associated types, 3) Creating generic protocol extensions, 4) Handling type inference, 5) Managing protocol composition with generics, 6) Implementing generic requirements. Enables creation of flexible, reusable protocol definitions.
Protocol Witness Table: 1) Stores protocol conformance information, 2) Maps protocol requirements to implementations, 3) Created at compile time, 4) Handles dynamic dispatch for protocols, 5) Manages associated type resolution, 6) Optimizes protocol method calls. Critical for protocol performance and functionality.
Optional Protocol Requirements: 1) Marked with @objc optional, 2) Only available in Objective-C compatible protocols, 3) Require runtime checking, 4) Handle unimplemented requirements safely, 5) Provide fallback behavior, 6) Support backward compatibility. Useful for creating flexible protocol interfaces.
Protocol Extension Method Dispatch: 1) Static dispatch for extension methods, 2) Dynamic dispatch for protocol requirements, 3) Resolution rules for conflicts, 4) Extension method overriding behavior, 5) Interaction with class inheritance, 6) Performance implications. Understanding dispatch behavior is crucial for correct implementation.
Protocol-Oriented Design practices: 1) Start with protocols before implementations, 2) Use protocol composition for modularity, 3) Leverage protocol extensions for default behavior, 4) Keep protocols focused and single-purpose, 5) Use associated types for flexibility, 6) Consider value types first. Promotes maintainable and flexible code design.
Conditional Conformance implementation: 1) Use where clauses for type constraints, 2) Extend generic types conditionally, 3) Implement requirements based on conditions, 4) Handle nested type conformance, 5) Manage multiple conditional conformances, 6) Consider performance implications. Enables type-safe conditional behavior.
Self Requirements: 1) Use Self keyword in protocol definitions, 2) Enable type-safe method chaining, 3) Implement comparison protocols, 4) Handle type constraints with Self, 5) Support builder patterns, 6) Enable fluent interfaces. Important for type-safe protocol design.
Protocol Conformance in Extensions: 1) Add conformance to existing types, 2) Implement required methods and properties, 3) Handle associated type requirements, 4) Manage conditional conformance, 5) Deal with retroactive modeling, 6) Consider scope and access control. Enables adding protocol support to types you don't own.
Type Erasure: 1) Hides concrete types behind protocols, 2) Implements wrapper types, 3) Manages associated type requirements, 4) Enables protocol use in collections, 5) Handles protocol composition, 6) Maintains type safety. Used when concrete types need to be abstracted away.
Protocol-Based Dependency Injection: 1) Define service protocols, 2) Implement mock conformance for testing, 3) Use protocol composition for dependencies, 4) Handle optional dependencies, 5) Manage dependency lifecycle, 6) Support dependency configuration. Enables flexible and testable architecture.
Protocol-Based Configuration: 1) Define configuration protocols, 2) Implement default configurations, 3) Support configuration composition, 4) Handle environment-specific configs, 5) Manage configuration inheritance, 6) Enable runtime configuration changes. Useful for flexible system configuration.
Protocol-Based Validation: 1) Define validation protocols, 2) Implement reusable validation logic, 3) Compose validation rules, 4) Handle validation errors, 5) Support custom validation rules, 6) Enable validation chaining. Creates flexible and reusable validation systems.
Protocol-Based Error Handling: 1) Define error protocols, 2) Implement error type hierarchies, 3) Handle error propagation, 4) Support error recovery, 5) Manage error context, 6) Enable error transformation. Creates systematic error handling approaches.
Protocol-Based State Management: 1) Define state protocols, 2) Implement state transitions, 3) Handle state validation, 4) Manage state persistence, 5) Support state observation, 6) Enable state restoration. Creates flexible state management systems.
Protocol-Based Testing: 1) Create testable interfaces, 2) Implement mock objects, 3) Support test doubles, 4) Enable behavior verification, 5) Handle test isolation, 6) Manage test dependencies. Improves code testability and maintainability.
Protocol-Based Networking: 1) Define network service protocols, 2) Implement request/response handling, 3) Manage authentication, 4) Handle error scenarios, 5) Support response parsing, 6) Enable request configuration. Creates modular networking layers.
Protocol-Based View Controllers: 1) Define view controller behaviors, 2) Implement reusable functionality, 3) Support composition of features, 4) Handle view lifecycle, 5) Manage navigation flow, 6) Enable view controller testing. Improves view controller maintainability.
Protocol-Based Data Sources: 1) Define data source protocols, 2) Implement data fetching logic, 3) Handle data updates, 4) Manage caching strategies, 5) Support pagination, 6) Enable data transformation. Creates flexible data management systems.
Protocol-Based Animation: 1) Define animation protocols, 2) Implement reusable animations, 3) Handle animation chaining, 4) Support timing functions, 5) Manage animation states, 6) Enable custom animations. Creates modular animation systems.
Protocol-Based Persistence: 1) Define storage protocols, 2) Implement various storage backends, 3) Handle data migration, 4) Manage data versioning, 5) Support data encryption, 6) Enable storage configuration. Creates flexible storage solutions.
Protocol-Based Resource Management: 1) Define resource protocols, 2) Implement resource loading, 3) Handle resource cleanup, 4) Manage resource lifecycle, 5) Support resource caching, 6) Enable resource monitoring. Creates efficient resource handling systems.
Protocol-Based Event Handling: 1) Define event protocols, 2) Implement event dispatching, 3) Handle event subscription, 4) Manage event priorities, 5) Support event filtering, 6) Enable event transformation. Creates flexible event systems.
Protocol-Based Logging: 1) Define logging protocols, 2) Implement different log levels, 3) Handle log formatting, 4) Manage log destinations, 5) Support log filtering, 6) Enable log persistence. Creates configurable logging systems.
Protocol-Based Middleware: 1) Define middleware protocols, 2) Implement request/response chain, 3) Handle cross-cutting concerns, 4) Manage middleware order, 5) Support middleware configuration, 6) Enable middleware composition. Creates flexible processing pipelines.
Swift Concurrency introduces: 1) async/await for asynchronous code, 2) Structured concurrency with tasks, 3) Actor model for state isolation, 4) Improved error handling, 5) Better code readability compared to completion handlers, 6) Built-in deadlock prevention. Unlike GCD, it provides compile-time checking and safer concurrency patterns.
Actors provide: 1) Data race protection through isolation, 2) Synchronized access to mutable state, 3) Serial execution of methods, 4) Async interface for external access, 5) Safe state management across tasks, 6) Reference type semantics. Use actors when shared mutable state needs thread-safe access.
async/await provides: 1) Structured approach to asynchronous code, 2) Elimination of completion handler pyramids, 3) Linear code flow for async operations, 4) Automatic error propagation, 5) Integration with throwing functions, 6) Better stack traces. Solves callback hell and improves code readability.
Tasks represent: 1) Units of asynchronous work, 2) Structured task hierarchies, 3) Cancellation support, 4) Priority management, 5) Task-local storage, 6) Task groups for parallel execution. Tasks provide structured approach to managing concurrent operations.
GCD features: 1) Queue-based task execution, 2) Serial and concurrent queues, 3) Quality of service levels, 4) Dispatch groups for synchronization, 5) Barrier flags for synchronization, 6) Semaphores for resource management. Provides low-level concurrency primitives.
AsyncSequence/AsyncStream provide: 1) Asynchronous iteration over values, 2) Back-pressure handling, 3) Cancellation support, 4) Integration with for-await-in loops, 5) Buffer control, 6) Continuation handling. Used for handling streams of asynchronous values.
Task cancellation involves: 1) Checking cancellation status, 2) Responding to cancellation, 3) Propagating cancellation to child tasks, 4) Implementing cleanup code, 5) Handling cancellation errors, 6) Setting up cancellation handlers. Ensures graceful task termination.
@MainActor ensures: 1) Code runs on main thread, 2) UI updates are safe, 3) State isolation for main thread, 4) Automatic thread switching, 5) Compile-time checking, 6) Integration with async/await. Use for UI-related code and main thread operations.
Concurrent data access patterns: 1) Using actors for isolation, 2) Implementing thread-safe properties, 3) Queue-based synchronization, 4) Read-write patterns, 5) Lock mechanisms, 6) Copy-on-write for value types. Ensures thread-safe data access.
Task Groups enable: 1) Parallel task execution, 2) Dynamic task creation, 3) Result collection, 4) Error handling, 5) Cancellation propagation, 6) Resource limiting. Used for managing multiple concurrent tasks with similar purpose.
Async testing includes: 1) Using async test methods, 2) Implementing expectations, 3) Testing actor isolation, 4) Simulating delays, 5) Testing cancellation, 6) Verifying async sequences. Ensures proper testing of concurrent code.
Sendable protocol ensures: 1) Safe cross-actor data transfer, 2) Value type conformance, 3) Thread-safe reference types, 4) Compile-time checking, 5) Actor isolation preservation, 6) Concurrent data safety. Used for safe data sharing between concurrent contexts.
Concurrent collections require: 1) Thread-safe access methods, 2) Atomic operations, 3) Lock-free algorithms, 4) Copy-on-write optimization, 5) Consistency guarantees, 6) Performance considerations. Ensures safe concurrent access to collection data.
AsyncThrowingStream provides: 1) Asynchronous error handling, 2) Cancellation support, 3) Back-pressure management, 4) Buffer control, 5) Continuation handling, 6) Integration with async/await. Used for error-throwing asynchronous sequences.
Deadlock prevention includes: 1) Using structured concurrency, 2) Implementing proper lock ordering, 3) Avoiding nested locks, 4) Using actors for isolation, 5) Implementing timeouts, 6) Proper resource release. Prevents concurrent access issues.
Concurrent error handling: 1) Using async throws functions, 2) Implementing error propagation, 3) Handling task cancellation, 4) Managing timeouts, 5) Implementing retry logic, 6) Proper cleanup on errors. Ensures robust error management.
Custom executors require: 1) Conforming to Executor protocol, 2) Managing task scheduling, 3) Implementing priority handling, 4) Resource management, 5) Queue management, 6) Performance optimization. Used for specialized execution contexts.
Task priority management: 1) Setting priority levels, 2) Priority inheritance, 3) Priority escalation, 4) QoS integration, 5) Task scheduling impact, 6) Priority propagation. Ensures proper resource allocation for tasks.
Async properties require: 1) Using async get keyword, 2) Managing property dependencies, 3) Handling cancellation, 4) Implementing caching, 5) Error handling, 6) Actor isolation consideration. Used for properties requiring async computation.
Background task patterns: 1) Task prioritization, 2) Resource management, 3) State preservation, 4) Background execution limits, 5) Task completion handling, 6) System integration. Ensures efficient background processing.
Concurrent networking: 1) Using async URLSession, 2) Implementing request grouping, 3) Managing timeouts, 4) Handling cancellation, 5) Error handling, 6) Response processing. Ensures efficient network operations.
AsyncLetBinding enables: 1) Parallel async operations, 2) Result dependency management, 3) Structured concurrency, 4) Error propagation, 5) Cancellation handling, 6) Resource optimization. Used for concurrent independent operations.
Rate limiting implementation: 1) Token bucket algorithm, 2) Time-based limiting, 3) Queue-based throttling, 4) Semaphore usage, 5) BackPressure handling, 6) Overflow management. Prevents resource exhaustion.
Concurrent memory management: 1) Weak reference usage, 2) Proper closure capture, 3) Resource cleanup, 4) Cycle prevention, 5) Buffer management, 6) Leak detection. Ensures proper resource handling.
Concurrent state machines: 1) Actor-based state management, 2) Thread-safe transitions, 3) Event handling, 4) State validation, 5) Error handling, 6) State observation. Ensures safe state management.
Actors provide: 1) Data race protection through isolation, 2) Synchronized access to mutable state, 3) Serial execution of methods, 4) Async interface for external access, 5) Safe state management across tasks, 6) Reference type semantics. Use actors when shared mutable state needs thread-safe access.
async/await provides: 1) Structured approach to asynchronous code, 2) Elimination of completion handler pyramids, 3) Linear code flow for async operations, 4) Automatic error propagation, 5) Integration with throwing functions, 6) Better stack traces. Solves callback hell and improves code readability.
Tasks represent: 1) Units of asynchronous work, 2) Structured task hierarchies, 3) Cancellation support, 4) Priority management, 5) Task-local storage, 6) Task groups for parallel execution. Tasks provide structured approach to managing concurrent operations.
GCD features: 1) Queue-based task execution, 2) Serial and concurrent queues, 3) Quality of service levels, 4) Dispatch groups for synchronization, 5) Barrier flags for synchronization, 6) Semaphores for resource management. Provides low-level concurrency primitives.
AsyncSequence/AsyncStream provide: 1) Asynchronous iteration over values, 2) Back-pressure handling, 3) Cancellation support, 4) Integration with for-await-in loops, 5) Buffer control, 6) Continuation handling. Used for handling streams of asynchronous values.
Task cancellation involves: 1) Checking cancellation status, 2) Responding to cancellation, 3) Propagating cancellation to child tasks, 4) Implementing cleanup code, 5) Handling cancellation errors, 6) Setting up cancellation handlers. Ensures graceful task termination.
@MainActor ensures: 1) Code runs on main thread, 2) UI updates are safe, 3) State isolation for main thread, 4) Automatic thread switching, 5) Compile-time checking, 6) Integration with async/await. Use for UI-related code and main thread operations.
Concurrent data access patterns: 1) Using actors for isolation, 2) Implementing thread-safe properties, 3) Queue-based synchronization, 4) Read-write patterns, 5) Lock mechanisms, 6) Copy-on-write for value types. Ensures thread-safe data access.
Task Groups enable: 1) Parallel task execution, 2) Dynamic task creation, 3) Result collection, 4) Error handling, 5) Cancellation propagation, 6) Resource limiting. Used for managing multiple concurrent tasks with similar purpose.
Async testing includes: 1) Using async test methods, 2) Implementing expectations, 3) Testing actor isolation, 4) Simulating delays, 5) Testing cancellation, 6) Verifying async sequences. Ensures proper testing of concurrent code.
Sendable protocol ensures: 1) Safe cross-actor data transfer, 2) Value type conformance, 3) Thread-safe reference types, 4) Compile-time checking, 5) Actor isolation preservation, 6) Concurrent data safety. Used for safe data sharing between concurrent contexts.
Concurrent collections require: 1) Thread-safe access methods, 2) Atomic operations, 3) Lock-free algorithms, 4) Copy-on-write optimization, 5) Consistency guarantees, 6) Performance considerations. Ensures safe concurrent access to collection data.
AsyncThrowingStream provides: 1) Asynchronous error handling, 2) Cancellation support, 3) Back-pressure management, 4) Buffer control, 5) Continuation handling, 6) Integration with async/await. Used for error-throwing asynchronous sequences.
Deadlock prevention includes: 1) Using structured concurrency, 2) Implementing proper lock ordering, 3) Avoiding nested locks, 4) Using actors for isolation, 5) Implementing timeouts, 6) Proper resource release. Prevents concurrent access issues.
Concurrent error handling: 1) Using async throws functions, 2) Implementing error propagation, 3) Handling task cancellation, 4) Managing timeouts, 5) Implementing retry logic, 6) Proper cleanup on errors. Ensures robust error management.
Custom executors require: 1) Conforming to Executor protocol, 2) Managing task scheduling, 3) Implementing priority handling, 4) Resource management, 5) Queue management, 6) Performance optimization. Used for specialized execution contexts.
Task priority management: 1) Setting priority levels, 2) Priority inheritance, 3) Priority escalation, 4) QoS integration, 5) Task scheduling impact, 6) Priority propagation. Ensures proper resource allocation for tasks.
Async properties require: 1) Using async get keyword, 2) Managing property dependencies, 3) Handling cancellation, 4) Implementing caching, 5) Error handling, 6) Actor isolation consideration. Used for properties requiring async computation.
Background task patterns: 1) Task prioritization, 2) Resource management, 3) State preservation, 4) Background execution limits, 5) Task completion handling, 6) System integration. Ensures efficient background processing.
Concurrent networking: 1) Using async URLSession, 2) Implementing request grouping, 3) Managing timeouts, 4) Handling cancellation, 5) Error handling, 6) Response processing. Ensures efficient network operations.
AsyncLetBinding enables: 1) Parallel async operations, 2) Result dependency management, 3) Structured concurrency, 4) Error propagation, 5) Cancellation handling, 6) Resource optimization. Used for concurrent independent operations.
Rate limiting implementation: 1) Token bucket algorithm, 2) Time-based limiting, 3) Queue-based throttling, 4) Semaphore usage, 5) BackPressure handling, 6) Overflow management. Prevents resource exhaustion.
Concurrent memory management: 1) Weak reference usage, 2) Proper closure capture, 3) Resource cleanup, 4) Cycle prevention, 5) Buffer management, 6) Leak detection. Ensures proper resource handling.
Concurrent state machines: 1) Actor-based state management, 2) Thread-safe transitions, 3) Event handling, 4) State validation, 5) Error handling, 6) State observation. Ensures safe state management.
Swift error handling includes: 1) try-catch blocks for throwing functions, 2) Result type for functional error handling, 3) Optional chaining for nil handling, 4) Guard statements for early exits, 5) Assertions for debugging, 6) Error protocol for custom errors, 7) Fatal errors for unrecoverable situations, 8) Optional try (try?) for converting errors to nil.
Custom Error implementation includes: 1) Conforming to Error protocol, 2) Defining error cases with enums, 3) Adding associated values for context, 4) Implementing LocalizedError for messages, 5) Adding custom properties for details, 6) Creating error hierarchies, 7) Handling different error cases, 8) Providing recovery suggestions.
Xcode debugging tools include: 1) LLDB debugger commands, 2) Breakpoints with conditions, 3) Variable inspection and watchpoints, 4) Memory graph debugger, 5) Thread navigator, 6) Console logging, 7) View debugger for UI, 8) Network request debugger.
Async error handling involves: 1) Using async throws functions, 2) Implementing Task error handling, 3) Managing actor isolation errors, 4) Handling concurrent errors, 5) Propagating errors in async sequences, 6) Using async variants of try, 7) Implementing error recovery, 8) Proper cancellation handling.
Key differences include: 1) fatalError always terminates, used for unimplemented code, 2) assert only runs in debug builds, 3) precondition runs in debug and -O builds, 4) assert can be disabled, 5) Different message handling, 6) Performance implications, 7) Usage in production code, 8) Integration with debugger.
Result type implementation: 1) Define success/failure cases, 2) Map and flatMap operations, 3) Error type constraints, 4) Converting to throws, 5) Handling async results, 6) Chaining operations, 7) Pattern matching on results, 8) Implementing custom transforms.
Logging best practices: 1) Using OSLog for system integration, 2) Implementing log levels, 3) Structured logging format, 4) Performance considerations, 5) Sensitive data handling, 6) Log rotation and storage, 7) Remote logging setup, 8) Debug vs release logging.
Memory debugging includes: 1) Using Instruments for leaks, 2) Memory graph debugger usage, 3) Heap debugging tools, 4) Reference cycle detection, 5) Allocation tracking, 6) Virtual memory analysis, 7) Memory pressure testing, 8) Debugging retain cycles.
Error propagation patterns: 1) Using rethrows keyword, 2) Error transformation, 3) Error aggregation, 4) Hierarchical error handling, 5) Context preservation, 6) Chain of responsibility, 7) Middleware error handling, 8) Error recovery strategies.
Debug-only implementation: 1) Using #if DEBUG directive, 2) Debug-only extensions, 3) Conditional compilation, 4) Debug logging setup, 5) Development-time features, 6) Performance impact handling, 7) Debug-only assertions, 8) Testing integrations.
Error recovery strategies: 1) Retry mechanisms, 2) Fallback values, 3) Graceful degradation, 4) State restoration, 5) User feedback, 6) Automatic recovery, 7) Recovery suggestions, 8) Cleanup operations.
Network debugging includes: 1) Charles Proxy integration, 2) URLSession debugging, 3) Network Link Conditioner, 4) Request/response logging, 5) Error pattern analysis, 6) Timeout handling, 7) Connection monitoring, 8) SSL/TLS debugging.
Crash reporting techniques: 1) Symbolication process, 2) Crash log analysis, 3) Exception handling, 4) Stack trace collection, 5) Debug information format, 6) Third-party integration, 7) User data collection, 8) Privacy considerations.
Validation error handling: 1) Custom validation rules, 2) Error aggregation, 3) Field-level errors, 4) Error message localization, 5) UI feedback integration, 6) Chain validation, 7) Cross-field validation, 8) Async validation.
SwiftUI debugging includes: 1) Preview debugging, 2) View hierarchy inspection, 3) State monitoring, 4) Layout debugging, 5) Performance profiling, 6) Animation debugging, 7) Environment value tracking, 8) View modifier debugging.
Protocol error handling: 1) Error type constraints, 2) Protocol error requirements, 3) Default implementations, 4) Generic error handling, 5) Error protocol composition, 6) Associated error types, 7) Error transformation, 8) Protocol extension error handling.
Error localization practices: 1) LocalizedError implementation, 2) String catalog usage, 3) Error message templates, 4) Dynamic variable substitution, 5) Pluralization handling, 6) Region-specific messages, 7) Fallback messages, 8) Format specifiers.
Performance debugging: 1) Time Profiler usage, 2) Instruments analysis, 3) Energy logging, 4) Memory allocation tracking, 5) Thread performance, 6) Core Animation debugging, 7) CPU usage analysis, 8) I/O monitoring.
Async error patterns: 1) Task error handling, 2) Actor isolation errors, 3) Structured concurrency errors, 4) Continuation errors, 5) AsyncSequence errors, 6) Task cancellation, 7) Error propagation, 8) Recovery strategies.
Error middleware: 1) Error interception, 2) Error transformation chain, 3) Logging middleware, 4) Recovery middleware, 5) Analytics integration, 6) Error filtering, 7) Context enrichment, 8) Error aggregation.
Database debugging: 1) SQLite debugging tools, 2) Core Data debugging, 3) Migration debugging, 4) Query optimization, 5) Schema validation, 6) Persistence error handling, 7) Data consistency checks, 8) Transaction debugging.
DI error handling: 1) Resolution errors, 2) Circular dependency detection, 3) Optional dependency handling, 4) Lifecycle errors, 5) Configuration validation, 6) Scope errors, 7) Factory errors, 8) Registration errors.
Memory leak debugging: 1) Instruments leaks tool, 2) Memory graph debugger, 3) Allocation tracking, 4) Retain cycle detection, 5) Heap analysis, 6) Object lifecycle tracking, 7) Autorelease pool debugging, 8) Reference counting analysis.
Error boundary patterns: 1) Error containment, 2) Fallback UI, 3) Error recovery UI, 4) State preservation, 5) Error isolation, 6) Component reset, 7) Error reporting, 8) User feedback mechanisms.
Error testing practices: 1) Error case coverage, 2) Recovery testing, 3) Async error testing, 4) Mock error injection, 5) Error propagation tests, 6) UI error testing, 7) Performance impact testing, 8) Integration testing.
Swift error handling includes: 1) try-catch blocks for throwing functions, 2) Result type for functional error handling, 3) Optional chaining for nil handling, 4) Guard statements for early exits, 5) Assertions for debugging, 6) Error protocol for custom errors, 7) Fatal errors for unrecoverable situations, 8) Optional try (try?) for converting errors to nil.
Custom Error implementation includes: 1) Conforming to Error protocol, 2) Defining error cases with enums, 3) Adding associated values for context, 4) Implementing LocalizedError for messages, 5) Adding custom properties for details, 6) Creating error hierarchies, 7) Handling different error cases, 8) Providing recovery suggestions.
Xcode debugging tools include: 1) LLDB debugger commands, 2) Breakpoints with conditions, 3) Variable inspection and watchpoints, 4) Memory graph debugger, 5) Thread navigator, 6) Console logging, 7) View debugger for UI, 8) Network request debugger.
Async error handling involves: 1) Using async throws functions, 2) Implementing Task error handling, 3) Managing actor isolation errors, 4) Handling concurrent errors, 5) Propagating errors in async sequences, 6) Using async variants of try, 7) Implementing error recovery, 8) Proper cancellation handling.
Key differences include: 1) fatalError always terminates, used for unimplemented code, 2) assert only runs in debug builds, 3) precondition runs in debug and -O builds, 4) assert can be disabled, 5) Different message handling, 6) Performance implications, 7) Usage in production code, 8) Integration with debugger.
Result type implementation: 1) Define success/failure cases, 2) Map and flatMap operations, 3) Error type constraints, 4) Converting to throws, 5) Handling async results, 6) Chaining operations, 7) Pattern matching on results, 8) Implementing custom transforms.
Logging best practices: 1) Using OSLog for system integration, 2) Implementing log levels, 3) Structured logging format, 4) Performance considerations, 5) Sensitive data handling, 6) Log rotation and storage, 7) Remote logging setup, 8) Debug vs release logging.
Memory debugging includes: 1) Using Instruments for leaks, 2) Memory graph debugger usage, 3) Heap debugging tools, 4) Reference cycle detection, 5) Allocation tracking, 6) Virtual memory analysis, 7) Memory pressure testing, 8) Debugging retain cycles.
Error propagation patterns: 1) Using rethrows keyword, 2) Error transformation, 3) Error aggregation, 4) Hierarchical error handling, 5) Context preservation, 6) Chain of responsibility, 7) Middleware error handling, 8) Error recovery strategies.
Debug-only implementation: 1) Using #if DEBUG directive, 2) Debug-only extensions, 3) Conditional compilation, 4) Debug logging setup, 5) Development-time features, 6) Performance impact handling, 7) Debug-only assertions, 8) Testing integrations.
Error recovery strategies: 1) Retry mechanisms, 2) Fallback values, 3) Graceful degradation, 4) State restoration, 5) User feedback, 6) Automatic recovery, 7) Recovery suggestions, 8) Cleanup operations.
Network debugging includes: 1) Charles Proxy integration, 2) URLSession debugging, 3) Network Link Conditioner, 4) Request/response logging, 5) Error pattern analysis, 6) Timeout handling, 7) Connection monitoring, 8) SSL/TLS debugging.
Crash reporting techniques: 1) Symbolication process, 2) Crash log analysis, 3) Exception handling, 4) Stack trace collection, 5) Debug information format, 6) Third-party integration, 7) User data collection, 8) Privacy considerations.
Validation error handling: 1) Custom validation rules, 2) Error aggregation, 3) Field-level errors, 4) Error message localization, 5) UI feedback integration, 6) Chain validation, 7) Cross-field validation, 8) Async validation.
SwiftUI debugging includes: 1) Preview debugging, 2) View hierarchy inspection, 3) State monitoring, 4) Layout debugging, 5) Performance profiling, 6) Animation debugging, 7) Environment value tracking, 8) View modifier debugging.
Protocol error handling: 1) Error type constraints, 2) Protocol error requirements, 3) Default implementations, 4) Generic error handling, 5) Error protocol composition, 6) Associated error types, 7) Error transformation, 8) Protocol extension error handling.
Error localization practices: 1) LocalizedError implementation, 2) String catalog usage, 3) Error message templates, 4) Dynamic variable substitution, 5) Pluralization handling, 6) Region-specific messages, 7) Fallback messages, 8) Format specifiers.
Performance debugging: 1) Time Profiler usage, 2) Instruments analysis, 3) Energy logging, 4) Memory allocation tracking, 5) Thread performance, 6) Core Animation debugging, 7) CPU usage analysis, 8) I/O monitoring.
Async error patterns: 1) Task error handling, 2) Actor isolation errors, 3) Structured concurrency errors, 4) Continuation errors, 5) AsyncSequence errors, 6) Task cancellation, 7) Error propagation, 8) Recovery strategies.
Error middleware: 1) Error interception, 2) Error transformation chain, 3) Logging middleware, 4) Recovery middleware, 5) Analytics integration, 6) Error filtering, 7) Context enrichment, 8) Error aggregation.
Database debugging: 1) SQLite debugging tools, 2) Core Data debugging, 3) Migration debugging, 4) Query optimization, 5) Schema validation, 6) Persistence error handling, 7) Data consistency checks, 8) Transaction debugging.
DI error handling: 1) Resolution errors, 2) Circular dependency detection, 3) Optional dependency handling, 4) Lifecycle errors, 5) Configuration validation, 6) Scope errors, 7) Factory errors, 8) Registration errors.
Memory leak debugging: 1) Instruments leaks tool, 2) Memory graph debugger, 3) Allocation tracking, 4) Retain cycle detection, 5) Heap analysis, 6) Object lifecycle tracking, 7) Autorelease pool debugging, 8) Reference counting analysis.
Error boundary patterns: 1) Error containment, 2) Fallback UI, 3) Error recovery UI, 4) State preservation, 5) Error isolation, 6) Component reset, 7) Error reporting, 8) User feedback mechanisms.
Error testing practices: 1) Error case coverage, 2) Recovery testing, 3) Async error testing, 4) Mock error injection, 5) Error propagation tests, 6) UI error testing, 7) Performance impact testing, 8) Integration testing.
Key optimization strategies include: 1) Using value types for better copy semantics, 2) Implementing copy-on-write for large value types, 3) Avoiding unnecessary object creation, 4) Optimizing collection operations, 5) Using lazy properties for expensive computations, 6) Implementing proper memory management, 7) Utilizing compiler optimizations, 8) Profiling and measuring performance metrics.
ARC performance considerations include: 1) Minimizing strong reference cycles, 2) Using weak and unowned references appropriately, 3) Implementing proper object lifecycle management, 4) Optimizing closure capture lists, 5) Managing collection strong references, 6) Understanding retain/release overhead, 7) Proper deinitialization handling, 8) Memory graph optimization.
Collection optimization includes: 1) Choosing appropriate collection types, 2) Reserving capacity upfront, 3) Using lazy collections for large datasets, 4) Implementing efficient sorting algorithms, 5) Optimizing iteration patterns, 6) Using contiguous arrays when possible, 7) Managing memory allocation, 8) Implementing custom collection types when needed.
Memory optimization techniques: 1) Using value types appropriately, 2) Implementing proper caching strategies, 3) Managing object lifecycles, 4) Optimizing image memory usage, 5) Using autorelease pools when needed, 6) Implementing memory warnings handling, 7) Proper resource cleanup, 8) Memory footprint monitoring.
Compile time optimization includes: 1) Reducing use of generics when unnecessary, 2) Optimizing complex type inference, 3) Managing module dependencies, 4) Using whole module optimization, 5) Implementing proper access control, 6) Reducing preprocessor directive usage, 7) Optimizing build settings, 8) Managing source file organization.
Network optimization includes: 1) Implementing proper caching strategies, 2) Using URLSession configuration optimization, 3) Managing request batching, 4) Implementing proper error handling, 5) Optimizing data serialization, 6) Managing background tasks, 7) Implementing request prioritization, 8) Network reachability handling.
UI performance optimization: 1) Implementing view reuse, 2) Optimizing layout calculations, 3) Managing main thread operations, 4) Implementing proper layer optimization, 5) Using drawRect efficiently, 6) Managing image loading and caching, 7) Implementing scrolling optimization, 8) Reducing view hierarchy complexity.
String optimization includes: 1) Using string interpolation efficiently, 2) Managing string concatenation, 3) Implementing proper unicode handling, 4) Using string indexes properly, 5) Managing string storage, 6) Optimizing string comparison, 7) Using string subranges efficiently, 8) Implementing proper string caching.
Protocol optimization techniques: 1) Using protocol composition efficiently, 2) Managing protocol inheritance hierarchy, 3) Implementing static dispatch when possible, 4) Optimizing protocol witness tables, 5) Using associated types properly, 6) Managing protocol conformance, 7) Implementing protocol extensions efficiently, 8) Understanding protocol overhead.
Data persistence optimization: 1) Choosing appropriate storage solution, 2) Implementing efficient serialization, 3) Managing batch operations, 4) Optimizing query performance, 5) Implementing proper caching, 6) Managing data migration, 7) Optimizing data models, 8) Implementing proper error handling.
Algorithm optimization includes: 1) Choosing appropriate data structures, 2) Implementing efficient sorting methods, 3) Managing time complexity, 4) Optimizing space usage, 5) Using dynamic programming when applicable, 6) Implementing proper memoization, 7) Managing recursive algorithms, 8) Understanding Big O notation.
Image optimization techniques: 1) Implementing proper image caching, 2) Managing image compression, 3) Using appropriate image formats, 4) Optimizing image loading, 5) Managing memory usage, 6) Implementing lazy loading, 7) Using image resizing efficiently, 8) Managing image metadata.
Closure optimization includes: 1) Managing capture lists efficiently, 2) Understanding closure overhead, 3) Implementing proper memory management, 4) Using @escaping appropriately, 5) Optimizing closure syntax, 6) Managing closure context, 7) Understanding closure lifetime, 8) Implementing proper retain cycles prevention.
Concurrent operation optimization: 1) Using appropriate dispatch queues, 2) Managing thread safety, 3) Implementing proper synchronization, 4) Optimizing task scheduling, 5) Managing resource contention, 6) Implementing proper cancellation, 7) Using operation dependencies, 8) Managing queue priorities.
Binary size optimization: 1) Managing dependency inclusion, 2) Using proper optimization flags, 3) Implementing dead code elimination, 4) Managing asset optimization, 5) Using proper linking options, 6) Managing framework integration, 7) Implementing proper code stripping, 8) Understanding symbol table optimization.
Understand Swift syntax, optionals, generics, and type safety.
Understand ARC, retain cycles, and memory optimization techniques.
Practice creating UI layouts and animations.
Prepare for algorithm-based problem-solving using Swift.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Swift questions, mock interviews, and more to secure your dream role.
Start Preparing now