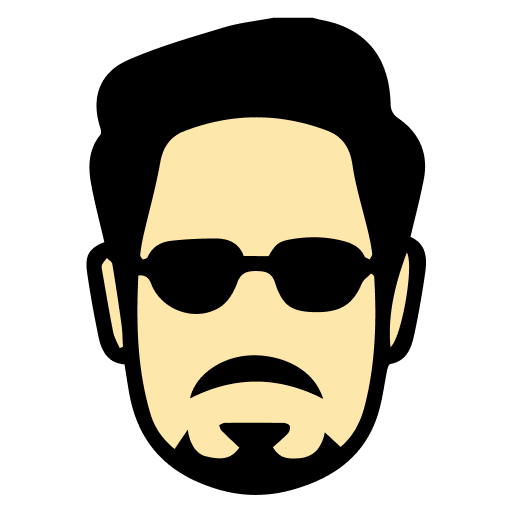
Svelte's unique compile-time approach and reactive programming model make it a powerful framework for modern web development. Stark.ai offers a comprehensive collection of Svelte interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Two-way binding is implemented using the bind: directive. Common with form elements. Example: <input...
Component initialization is handled in the <script> section. Can use onMount lifecycle function for side effects....
Derived stores are created using derived() function, combining one or more stores into a new one. Values update...
Dynamic components can be loaded using svelte:component directive. Example: <svelte:component...
Actions are reusable functions that run when an element is created. Used with use: directive. Can return destroy...
Component composition patterns include slots, context API, event forwarding. Support nested components, higher-order...
Reactive statements ($:) automatically re-run when dependencies change. Used for derived calculations and side...
Component interfaces use TypeScript with props and events. Define prop types using export let prop: Type. Support...
Lifecycle methods include onMount, onDestroy, beforeUpdate, afterUpdate. Handle component initialization, cleanup,...
State persistence uses local storage, session storage, or external stores. Implement auto-save functionality. Handle...
Derived stores are created using derived() from svelte/store. Take one or more stores as input. Transform values...
Async derived stores handle asynchronous transformations. Use derived with set callback. Handle loading states....
Custom stores implement subscribe function. Can add custom methods. Handle internal state. Support custom update...
Store initialization can be synchronous or async. Handle initial values. Support lazy initialization. Manage loading...
Store persistence uses localStorage or sessionStorage. Implement auto-save functionality. Handle serialization....
Store subscriptions cleanup happens automatically with $ prefix. Manual cleanup needs unsubscribe call. Handle...
Store error handling includes error states. Implement error recovery. Support error notifications. Handle async...
Store middleware intercepts store operations. Implement custom behaviors. Handle side effects. Support middleware...
Prop types can be checked using TypeScript or runtime validation. Define interfaces for props. Implement validation...
Async props can be handled using promises or async/await. Handle loading states. Use reactive statements for async...
Computed props derive values from other props using reactive declarations. Example: $: fullName = `${firstName}...
Prop watchers use reactive statements to observe changes. Can trigger side effects or validations. Handle prop...
Prop defaults are set in export statement or computed. Consider component initialization. Handle undefined values....
Event modifiers customize event behavior. Include preventDefault, stopPropagation, once, capture. Used with on:...
Prop transformation converts prop values before use. Use computed properties or methods. Handle data formatting....
Dynamic event handlers change based on props or state. Use computed values for handlers. Support conditional events....
Prop persistence saves prop values between renders. Use stores or local storage. Handle persistence lifecycle....
Compound components share state through context. Implement parent-child communication. Handle component composition....
Error handling uses try/catch blocks or error boundaries. Handle async errors appropriately. Implement error...
State initialization happens in component creation or onMount. Handle async initialization. Support loading states....
Store subscriptions are typically set up in onMount and cleaned in onDestroy. Handle store updates. Support...
DOM measurements use afterUpdate for accurate values. Cache measurements when needed. Handle resize events. Support...
Initialization patterns include lazy loading, conditional initialization, and dependency injection. Handle...
Prop changes trigger beforeUpdate and afterUpdate. Implement prop watchers. Handle derived values. Support prop...
Side effects management includes cleanup, ordering, and dependencies. Handle async side effects. Support...
Animations interact with beforeUpdate and afterUpdate. Handle transition states. Support animation cleanup....
Lifecycle hooks extend default lifecycle behavior. Implement custom hooks. Support hook composition. Handle hook...
Component transitions use lifecycle methods for coordination. Handle mount/unmount animations. Support transition...
Event debouncing delays handler execution. Use timer to postpone execution. Clear previous timer on new events....
Event throttling limits execution frequency. Implement using timer and last execution time. Ensure minimum time...
Keyboard events use on:keydown, on:keyup, on:keypress. Access key information through event.key. Support key...
Drag and drop uses dragstart, dragover, drop events. Set draggable attribute. Handle data transfer. Support drag...
Form events include submit, reset, change. Prevent default submission. Handle validation. Collect form data. Support...
Custom modifiers use actions (use:). Implement modifier logic. Support parameters. Handle cleanup. Share across components.
Outside clicks check event.target relationship. Implement using actions. Handle document clicks. Support multiple...
Touch events include touchstart, touchmove, touchend. Handle gestures. Support multi-touch. Implement touch...
Scroll events track scroll position. Implement scroll handlers. Support infinite scroll. Handle scroll optimization....
Window events use svelte:window component. Listen to resize, scroll, online/offline. Handle cleanup. Support event...
Custom stores implement subscribe method. Can add custom methods. Handle internal state. Example: function...
Async stores handle asynchronous data. Support loading states. Handle errors. Example: const data = writable(null);...
Store persistence saves state to storage. Implement auto-save. Handle state rehydration. Example: Subscribe to...
Store dependencies use derived stores or reactive statements. Handle update order. Manage circular dependencies....
Store middleware intercepts store operations. Implement custom behaviors. Handle side effects. Example: Create...
Store error handling catches and processes errors. Implement error states. Support error recovery. Example:...
Store validation ensures valid state. Implement validation rules. Handle validation errors. Example: Create wrapper...
Store optimization includes batching updates, debouncing, throttling. Handle performance bottlenecks. Example:...
Store composition combines multiple stores. Create higher-order stores. Handle store relationships. Example: Combine...
Store lifecycle management handles initialization, updates, cleanup. Support store creation/destruction. Example:...
Custom transitions are functions returning animation parameters. Include css and tick functions. Handle enter/exit...
Transition events include introstart, introend, outrostart, outroend. Listen using on: directive. Example: <div...
CSS animations use standard CSS animation properties. Can be combined with transitions. Example: <div animate:flip>....
Flip animation smoothly animates layout changes. Example: <div animate:flip>. Parameters include duration, delay....
Multiple transitions can be combined using different directives. Handle timing coordination. Support transition...
Spring animations use spring() function. Handle physics-based animations. Support stiffness and damping. Example:...
Crossfade creates smooth transitions between elements. Use crossfade() function. Handle element replacement. Support...
Optimize using CSS transforms, will-change property. Handle hardware acceleration. Manage animation frame rate....
Transition groups coordinate multiple transitions. Handle group timing. Support synchronized animations. Manage...
Deferred transitions delay animation start. Handle transition timing. Support conditional transitions. Manage...
Conditional slots use if blocks around slots. Handle slot presence checks. Support dynamic slot selection. Example:...
Dynamic slot names use computed values. Support runtime slot selection. Handle dynamic content projection. Example:...
Slot validation checks content type and structure. Handle invalid content. Support content restrictions. Implement...
Slot events bubble through component hierarchy. Handle event forwarding. Support event modification. Manage event...
Slot lifecycles manage content updates. Handle content mounting/unmounting. Support cleanup operations. Manage slot state.
Slot composition combines multiple slots. Handle nested slots. Support slot inheritance. Implement composition patterns.
Slot optimization improves rendering efficiency. Handle content caching. Support lazy loading. Manage update frequency.
Error boundaries catch slot content errors. Handle error recovery. Support fallback content. Manage error state.
Conditional slots use if blocks around slots. Handle slot presence checks. Support dynamic slot selection. Example:...
Dynamic slot names use computed values. Support runtime slot selection. Handle dynamic content projection. Example:...
Slot validation checks content type and structure. Handle invalid content. Support content restrictions. Implement...
Slot events bubble through component hierarchy. Handle event forwarding. Support event modification. Manage event...
Slot middleware processes slot content. Handle content transformation. Support content filtering. Implement middleware chain.
Slot lifecycles manage content updates. Handle content mounting/unmounting. Support cleanup operations. Manage slot state.
Slot composition combines multiple slots. Handle nested slots. Support slot inheritance. Implement composition patterns.
Slot optimization improves rendering efficiency. Handle content caching. Support lazy loading. Manage update frequency.
Error boundaries catch slot content errors. Handle error recovery. Support fallback content. Manage error state.
Route guards protect routes using load functions. Check authentication/authorization. Return redirect for...
Nested layouts use multiple +layout.svelte files. Each level adds layout. Support layout inheritance. Handle layout...
Preloading uses data-sveltekit-preload attribute. Fetches data before navigation. Support hover preloading. Handle...
Route transitions use page transitions API. Support enter/leave animations. Handle transition lifecycle. Implement...
Route middleware uses hooks.server.js file. Handle request/response. Support authentication. Implement custom logic....
Route caching implements caching strategies. Handle data caching. Support page caching. Implement cache...
Route validation handles parameter validation. Support request validation. Implement validation rules. Handle...
Route state management uses stores or context. Handle state persistence. Support state sharing. Implement state...
Route optimization includes code splitting, preloading. Handle lazy loading. Support route prioritization. Implement...
Context patterns include provider components, dependency injection, service locator. Handle context composition....
Context updates require component reinitialization. Can combine with stores for reactive updates. Handle update...
Context dependencies manage relationships between contexts. Handle dependency order. Support circular dependencies....
Context composition combines multiple contexts. Handle context merging. Support context inheritance. Implement...
Context initialization sets up initial context state. Handle async initialization. Support initialization order....
Context middleware processes context operations. Handle context transformation. Support middleware chain. Implement...
Context error handling manages error states. Handle missing context. Support error recovery. Implement error boundaries.
Context optimization improves performance. Handle context caching. Support selective updates. Implement optimization...
Context cleanup manages resource disposal. Handle cleanup order. Support cleanup hooks. Implement cleanup strategies.
Custom actions are functions returning optional destroy method. Handle DOM node manipulation. Support parameters....
Binding validation ensures valid values. Handle input constraints. Support custom validation. Implement error...
Binding middleware processes binding operations. Handle value transformation. Support validation chain. Implement...
Binding dependencies manage related bindings. Handle dependency updates. Support dependency tracking. Implement...
Binding optimization improves update efficiency. Handle update batching. Support selective updates. Implement...
Custom directives extend element functionality. Handle directive lifecycle. Support directive parameters. Implement...
Binding error handling manages invalid states. Handle error recovery. Support error notifications. Implement error...
Binding composition combines multiple bindings. Handle binding interaction. Support binding inheritance. Implement...
Binding cleanup manages resource disposal. Handle cleanup order. Support cleanup hooks. Implement cleanup strategies.
SSR data fetching uses load functions. Handle async operations. Support caching strategies. Implement error...
SSR caching implements cache strategies. Handle cache invalidation. Support cache headers. Implement cache storage....
SSR optimization includes code splitting, caching, streaming. Handle resource optimization. Support performance...
SSR session state manages user sessions. Handle session storage. Support session persistence. Implement session...
SSR middleware processes server requests. Handle request transformation. Support middleware chain. Implement...
SSR authentication manages user auth state. Handle auth flows. Support session management. Implement auth...
SSR routing handles server-side navigation. Handle route matching. Support dynamic routes. Implement routing...
SSR headers manage HTTP headers. Handle cache control. Support content types. Implement header strategies. Manage...
SSR forms handle form submissions server-side. Handle form validation. Support file uploads. Implement CSRF...
Integration testing verifies component interaction. Test multiple components. Handle data flow. Implement test...
Store testing verifies store behavior. Test store updates. Handle subscriptions. Implement store mock. Manage store state.
Test mocks simulate dependencies. Handle external services. Support mock responses. Implement mock behavior. Manage...
Route testing verifies navigation behavior. Test route parameters. Handle route changes. Implement navigation tests....
Test fixtures provide test data. Handle data setup. Support test isolation. Implement fixture management. Manage...
Animation testing verifies transition behavior. Test animation states. Handle animation timing. Implement animation...
Test hooks handle test lifecycle. Support setup/teardown. Implement test utilities. Manage test state. Handle test...
Error handling testing verifies error states. Test error boundaries. Handle error recovery. Implement error...
Test coverage tracks code coverage. Generate coverage reports. Set coverage targets. Implement coverage checks....
Test organization structures test files. Group related tests. Support test discovery. Implement naming conventions....
Caching strategies include browser cache, service workers, SSR cache. Handle cache invalidation. Support offline...
Animation optimization includes using CSS animations when possible, hardware acceleration, efficient transitions....
Large list optimization includes virtual scrolling, pagination, infinite scroll. Use keyed each blocks. Implement...
Image optimization includes lazy loading, responsive images, proper formats. Use srcset attribute. Implement...
Performance monitoring tracks metrics like load time, FCP, TTI. Use browser DevTools. Implement analytics. Handle...
SSR optimization includes caching, streaming, efficient data loading. Handle server resources. Implement response...
Resource prioritization includes critical CSS, script loading strategies, preload/prefetch. Handle resource order....
Build optimization includes proper Vite/Rollup config, production settings, environment optimization. Handle build...
Performance testing measures load time, interaction time, resource usage. Implement benchmarks. Handle performance...
Two-way binding is implemented using the bind: directive. Common with form elements. Example: <input bind:value={name}>. Supports binding to different properties like checked for checkboxes.
Component initialization is handled in the <script> section. Can use onMount lifecycle function for side effects. Top-level code runs on component creation. Support async initialization using IIFE or onMount.
Derived stores are created using derived() function, combining one or more stores into a new one. Values update automatically when source stores change. Support synchronous and asynchronous derivation.
Dynamic components can be loaded using svelte:component directive. Example: <svelte:component this={dynamicComponent} />. Support lazy loading using import(). Handle loading states.
Actions are reusable functions that run when an element is created. Used with use: directive. Can return destroy function. Example: <div use:tooltip={params}>. Support custom DOM manipulation.
Component composition patterns include slots, context API, event forwarding. Support nested components, higher-order components. Handle component communication through props and events.
Reactive statements ($:) automatically re-run when dependencies change. Used for derived calculations and side effects. Support multiple dependencies. Handle complex reactive computations.
Component interfaces use TypeScript with props and events. Define prop types using export let prop: Type. Support interface inheritance. Handle optional props and default values.
Lifecycle methods include onMount, onDestroy, beforeUpdate, afterUpdate. Handle component initialization, cleanup, updates. Support async operations. Manage side effects.
State persistence uses local storage, session storage, or external stores. Implement auto-save functionality. Handle state rehydration. Support offline state management.
Derived stores are created using derived() from svelte/store. Take one or more stores as input. Transform values using callback function. Update automatically when source stores change.
Async derived stores handle asynchronous transformations. Use derived with set callback. Handle loading states. Support cancellation. Manage async dependencies.
Custom stores implement subscribe function. Can add custom methods. Handle internal state. Support custom update logic. Implement cleanup on unsubscribe.
Store initialization can be synchronous or async. Handle initial values. Support lazy initialization. Manage loading states. Handle initialization errors.
Store persistence uses localStorage or sessionStorage. Implement auto-save functionality. Handle serialization. Support state rehydration. Manage persistence errors.
Store subscriptions cleanup happens automatically with $ prefix. Manual cleanup needs unsubscribe call. Handle cleanup in onDestroy. Prevent memory leaks.
Store error handling includes error states. Implement error recovery. Support error notifications. Handle async errors. Manage error boundaries.
Store middleware intercepts store operations. Implement custom behaviors. Handle side effects. Support middleware chain. Manage middleware order.
Prop types can be checked using TypeScript or runtime validation. Define interfaces for props. Implement validation in onMount or initialization. Handle type errors appropriately.
Async props can be handled using promises or async/await. Handle loading states. Use reactive statements for async updates. Manage error states for async props.
Computed props derive values from other props using reactive declarations. Example: $: fullName = `${firstName} ${lastName}`. Update automatically when dependencies change.
Prop watchers use reactive statements to observe changes. Can trigger side effects or validations. Handle prop updates asynchronously. Support complex watch conditions.
Prop defaults are set in export statement or computed. Consider component initialization. Handle undefined values. Support dynamic defaults. Validate default values.
Event modifiers customize event behavior. Include preventDefault, stopPropagation, once, capture. Used with on: directive. Example: on:click|preventDefault. Support multiple modifiers.
Prop transformation converts prop values before use. Use computed properties or methods. Handle data formatting. Support bi-directional transformation. Validate transformed values.
Dynamic event handlers change based on props or state. Use computed values for handlers. Support conditional events. Handle dynamic binding. Manage handler lifecycle.
Prop persistence saves prop values between renders. Use stores or local storage. Handle persistence lifecycle. Support value restoration. Manage persistence errors.
Compound components share state through context. Implement parent-child communication. Handle component composition. Support flexible APIs. Manage shared state.
Error handling uses try/catch blocks or error boundaries. Handle async errors appropriately. Implement error recovery strategies. Support error reporting.
State initialization happens in component creation or onMount. Handle async initialization. Support loading states. Implement initialization strategies.
Store subscriptions are typically set up in onMount and cleaned in onDestroy. Handle store updates. Support auto-subscription. Manage subscription lifecycle.
DOM measurements use afterUpdate for accurate values. Cache measurements when needed. Handle resize events. Support responsive measurements.
Initialization patterns include lazy loading, conditional initialization, and dependency injection. Handle initialization order. Support async initialization.
Prop changes trigger beforeUpdate and afterUpdate. Implement prop watchers. Handle derived values. Support prop validation in lifecycle.
Side effects management includes cleanup, ordering, and dependencies. Handle async side effects. Support cancellation. Implement side effect tracking.
Animations interact with beforeUpdate and afterUpdate. Handle transition states. Support animation cleanup. Implement animation queuing.
Lifecycle hooks extend default lifecycle behavior. Implement custom hooks. Support hook composition. Handle hook dependencies.
Component transitions use lifecycle methods for coordination. Handle mount/unmount animations. Support transition states. Implement transition hooks.
Event debouncing delays handler execution. Use timer to postpone execution. Clear previous timer on new events. Example: implement custom action or store for debouncing.
Event throttling limits execution frequency. Implement using timer and last execution time. Ensure minimum time between executions. Support custom throttle intervals.
Keyboard events use on:keydown, on:keyup, on:keypress. Access key information through event.key. Support key combinations. Handle keyboard navigation.
Drag and drop uses dragstart, dragover, drop events. Set draggable attribute. Handle data transfer. Support drag visualization. Implement drop zones.
Form events include submit, reset, change. Prevent default submission. Handle validation. Collect form data. Support dynamic forms.
Custom modifiers use actions (use:). Implement modifier logic. Support parameters. Handle cleanup. Share across components.
Outside clicks check event.target relationship. Implement using actions. Handle document clicks. Support multiple elements. Clean up listeners.
Touch events include touchstart, touchmove, touchend. Handle gestures. Support multi-touch. Implement touch feedback. Handle touch coordinates.
Scroll events track scroll position. Implement scroll handlers. Support infinite scroll. Handle scroll optimization. Manage scroll restoration.
Window events use svelte:window component. Listen to resize, scroll, online/offline. Handle cleanup. Support event parameters.
Custom stores implement subscribe method. Can add custom methods. Handle internal state. Example: function createCustomStore() { const { subscribe, set } = writable(0); return { subscribe, increment: () => update(n => n + 1) }; }
Async stores handle asynchronous data. Support loading states. Handle errors. Example: const data = writable(null); async function fetchData() { const response = await fetch(url); data.set(await response.json()); }
Store persistence saves state to storage. Implement auto-save. Handle state rehydration. Example: Subscribe to changes and save to localStorage. Load initial state from storage.
Store dependencies use derived stores or reactive statements. Handle update order. Manage circular dependencies. Support dependency tracking.
Store middleware intercepts store operations. Implement custom behaviors. Handle side effects. Example: Create wrapper store with logging or validation.
Store error handling catches and processes errors. Implement error states. Support error recovery. Example: Try-catch in store operations, maintain error state.
Store validation ensures valid state. Implement validation rules. Handle validation errors. Example: Create wrapper store that validates updates.
Store optimization includes batching updates, debouncing, throttling. Handle performance bottlenecks. Example: Implement update batching or debounced updates.
Store composition combines multiple stores. Create higher-order stores. Handle store relationships. Example: Combine multiple stores into single interface.
Store lifecycle management handles initialization, updates, cleanup. Support store creation/destruction. Example: Implement cleanup in onDestroy, handle store initialization.
Custom transitions are functions returning animation parameters. Include css and tick functions. Handle enter/exit animations. Support custom parameters.
Transition events include introstart, introend, outrostart, outroend. Listen using on: directive. Example: <div transition:fade on:introend={handleEnd}>.
CSS animations use standard CSS animation properties. Can be combined with transitions. Example: <div animate:flip>. Support keyframes and animation properties.
Flip animation smoothly animates layout changes. Example: <div animate:flip>. Parameters include duration, delay. Useful for list reordering.
Multiple transitions can be combined using different directives. Handle timing coordination. Support transition groups. Manage transition order.
Spring animations use spring() function. Handle physics-based animations. Support stiffness and damping. Example: spring(value, {stiffness: 0.3}).
Crossfade creates smooth transitions between elements. Use crossfade() function. Handle element replacement. Support key-based transitions.
Optimize using CSS transforms, will-change property. Handle hardware acceleration. Manage animation frame rate. Monitor performance metrics.
Transition groups coordinate multiple transitions. Handle group timing. Support synchronized animations. Manage group lifecycle.
Deferred transitions delay animation start. Handle transition timing. Support conditional transitions. Manage transition state.
Conditional slots use if blocks around slots. Handle slot presence checks. Support dynamic slot selection. Example: {#if condition}<slot></slot>{/if}
Dynamic slot names use computed values. Support runtime slot selection. Handle dynamic content projection. Example: <slot name={dynamicName}>
Slot validation checks content type and structure. Handle invalid content. Support content restrictions. Implement validation logic.
Slot events bubble through component hierarchy. Handle event forwarding. Support event modification. Manage event propagation.
Slot lifecycles manage content updates. Handle content mounting/unmounting. Support cleanup operations. Manage slot state.
Slot composition combines multiple slots. Handle nested slots. Support slot inheritance. Implement composition patterns.
Slot optimization improves rendering efficiency. Handle content caching. Support lazy loading. Manage update frequency.
Error boundaries catch slot content errors. Handle error recovery. Support fallback content. Manage error state.
Conditional slots use if blocks around slots. Handle slot presence checks. Support dynamic slot selection. Example: {#if condition}<slot></slot>{/if}
Dynamic slot names use computed values. Support runtime slot selection. Handle dynamic content projection. Example: <slot name={dynamicName}>
Slot validation checks content type and structure. Handle invalid content. Support content restrictions. Implement validation logic.
Slot events bubble through component hierarchy. Handle event forwarding. Support event modification. Manage event propagation.
Slot middleware processes slot content. Handle content transformation. Support content filtering. Implement middleware chain.
Slot lifecycles manage content updates. Handle content mounting/unmounting. Support cleanup operations. Manage slot state.
Slot composition combines multiple slots. Handle nested slots. Support slot inheritance. Implement composition patterns.
Slot optimization improves rendering efficiency. Handle content caching. Support lazy loading. Manage update frequency.
Error boundaries catch slot content errors. Handle error recovery. Support fallback content. Manage error state.
Route guards protect routes using load functions. Check authentication/authorization. Return redirect for unauthorized access. Support async checks.
Nested layouts use multiple +layout.svelte files. Each level adds layout. Support layout inheritance. Handle layout data. Share layout between routes.
Preloading uses data-sveltekit-preload attribute. Fetches data before navigation. Support hover preloading. Handle preload strategies. Optimize performance.
Route transitions use page transitions API. Support enter/leave animations. Handle transition lifecycle. Implement custom transitions. Manage transition state.
Route middleware uses hooks.server.js file. Handle request/response. Support authentication. Implement custom logic. Manage middleware chain.
Route caching implements caching strategies. Handle data caching. Support page caching. Implement cache invalidation. Manage cache lifecycle.
Route validation handles parameter validation. Support request validation. Implement validation rules. Handle validation errors. Manage validation state.
Route state management uses stores or context. Handle state persistence. Support state sharing. Implement state updates. Manage state lifecycle.
Route optimization includes code splitting, preloading. Handle lazy loading. Support route prioritization. Implement performance monitoring.
Context patterns include provider components, dependency injection, service locator. Handle context composition. Support context inheritance. Implement context strategies.
Context updates require component reinitialization. Can combine with stores for reactive updates. Handle update propagation. Manage update lifecycle.
Context dependencies manage relationships between contexts. Handle dependency order. Support circular dependencies. Implement dependency resolution.
Context composition combines multiple contexts. Handle context merging. Support context inheritance. Implement composition patterns.
Context initialization sets up initial context state. Handle async initialization. Support initialization order. Implement initialization strategies.
Context middleware processes context operations. Handle context transformation. Support middleware chain. Implement middleware patterns.
Context error handling manages error states. Handle missing context. Support error recovery. Implement error boundaries.
Context optimization improves performance. Handle context caching. Support selective updates. Implement optimization strategies.
Context cleanup manages resource disposal. Handle cleanup order. Support cleanup hooks. Implement cleanup strategies.
Custom actions are functions returning optional destroy method. Handle DOM node manipulation. Support parameters. Example: use:customAction={params}.
Binding validation ensures valid values. Handle input constraints. Support custom validation. Implement error handling. Manage validation state.
Binding middleware processes binding operations. Handle value transformation. Support validation chain. Implement middleware pattern.
Binding dependencies manage related bindings. Handle dependency updates. Support dependency tracking. Implement dependency resolution.
Binding optimization improves update efficiency. Handle update batching. Support selective updates. Implement performance monitoring.
Custom directives extend element functionality. Handle directive lifecycle. Support directive parameters. Implement cleanup methods.
Binding error handling manages invalid states. Handle error recovery. Support error notifications. Implement error boundaries.
Binding composition combines multiple bindings. Handle binding interaction. Support binding inheritance. Implement composition patterns.
Binding cleanup manages resource disposal. Handle cleanup order. Support cleanup hooks. Implement cleanup strategies.
SSR data fetching uses load functions. Handle async operations. Support caching strategies. Implement error handling. Manage data dependencies.
SSR caching implements cache strategies. Handle cache invalidation. Support cache headers. Implement cache storage. Manage cache lifecycle.
SSR optimization includes code splitting, caching, streaming. Handle resource optimization. Support performance monitoring. Implement optimization strategies.
SSR session state manages user sessions. Handle session storage. Support session persistence. Implement session security. Manage session lifecycle.
SSR middleware processes server requests. Handle request transformation. Support middleware chain. Implement middleware patterns. Manage middleware order.
SSR authentication manages user auth state. Handle auth flows. Support session management. Implement auth strategies. Manage auth security.
SSR routing handles server-side navigation. Handle route matching. Support dynamic routes. Implement routing strategies. Manage route state.
SSR headers manage HTTP headers. Handle cache control. Support content types. Implement header strategies. Manage header security.
SSR forms handle form submissions server-side. Handle form validation. Support file uploads. Implement CSRF protection. Manage form state.
Integration testing verifies component interaction. Test multiple components. Handle data flow. Implement test scenarios. Manage test environment.
Store testing verifies store behavior. Test store updates. Handle subscriptions. Implement store mock. Manage store state.
Test mocks simulate dependencies. Handle external services. Support mock responses. Implement mock behavior. Manage mock state.
Route testing verifies navigation behavior. Test route parameters. Handle route changes. Implement navigation tests. Manage route state.
Test fixtures provide test data. Handle data setup. Support test isolation. Implement fixture management. Manage fixture cleanup.
Animation testing verifies transition behavior. Test animation states. Handle animation timing. Implement animation checks. Manage animation events.
Test hooks handle test lifecycle. Support setup/teardown. Implement test utilities. Manage test state. Handle test dependencies.
Error handling testing verifies error states. Test error boundaries. Handle error recovery. Implement error scenarios. Manage error logging.
Test coverage tracks code coverage. Generate coverage reports. Set coverage targets. Implement coverage checks. Manage coverage gaps.
Test organization structures test files. Group related tests. Support test discovery. Implement naming conventions. Manage test hierarchy.
Caching strategies include browser cache, service workers, SSR cache. Handle cache invalidation. Support offline functionality. Implement cache policies.
Animation optimization includes using CSS animations when possible, hardware acceleration, efficient transitions. Handle animation timing. Minimize reflows.
Large list optimization includes virtual scrolling, pagination, infinite scroll. Use keyed each blocks. Implement list chunking. Handle DOM recycling.
Image optimization includes lazy loading, responsive images, proper formats. Use srcset attribute. Implement placeholder images. Handle image compression.
Performance monitoring tracks metrics like load time, FCP, TTI. Use browser DevTools. Implement analytics. Handle performance reporting.
SSR optimization includes caching, streaming, efficient data loading. Handle server resources. Implement response compression. Optimize render time.
Resource prioritization includes critical CSS, script loading strategies, preload/prefetch. Handle resource order. Implement loading priorities.
Build optimization includes proper Vite/Rollup config, production settings, environment optimization. Handle build process. Implement build strategies.
Performance testing measures load time, interaction time, resource usage. Implement benchmarks. Handle performance metrics. Support performance monitoring.
Understand concepts like reactivity, components, stores, and lifecycle methods.
Work on creating components and implementing state management solutions.
Dive into animations, transitions, and advanced compiler features.
Expect hands-on challenges to build, optimize, and debug Svelte applications.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Svelte questions, mock interviews, and more to secure your dream role.
Start Preparing now