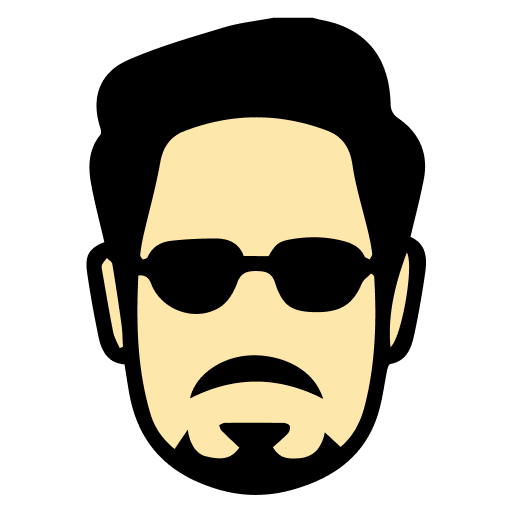
React Native is a powerful framework for building cross-platform mobile applications using JavaScript and React. Stark.ai offers a curated collection of React Native interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
The New Architecture introduces Fabric (new rendering system) and TurboModules (improved native modules). It reduces...
Fabric is a C++ rendering system that enables synchronous operations between JS and native code. It introduces a new...
TurboModules provide direct JS to native communication without serialization overhead. They use codegen to create...
React Native manages memory through automatic garbage collection in JS and ARC/GC in native code. Understanding the...
JSI enables direct communication between JavaScript and native code without going through the bridge. It provides a...
Native crashes are handled through platform-specific crash reporting tools and services. Integration requires native...
Security involves handling data encryption, secure storage, certificate pinning, code obfuscation, and protecting...
App initialization involves native bootstrap, JS engine initialization, bundle loading, and component mounting....
Custom native components require native code implementation (Java/Kotlin for Android, Objective-C/Swift for iOS) and...
Rerendering optimization involves: 1) Using React.memo for functional components, 2) Implementing...
Virtualized list challenges include: 1) Memory management for large datasets, 2) Smooth scrolling performance, 3)...
Complex gestures require the PanResponder system or gesture libraries. Implementation involves handling gesture...
Lazy loading strategies include: 1) React.lazy with Suspense, 2) Dynamic imports, 3) Route-based code splitting, 4)...
Error boundaries are components that catch JavaScript errors in their child component tree. Implementation involves...
Accessibility implementation includes: 1) Proper use of accessibility props, 2) Semantic markup, 3) Focus...
Offline-first state management requires: 1) Local state persistence, 2) Conflict resolution strategies, 3) Queue...
Large-scale state management involves: 1) Domain-driven state organization, 2) State normalization, 3) Module-based...
Multi-window state management requires: 1) State synchronization between windows, 2) Shared storage mechanisms, 3)...
Microservices state management involves: 1) Domain-based state separation, 2) Service boundary definition, 3) State...
Real-time collaboration requires: 1) Operational transformation or CRDT implementation, 2) State conflict...
Complex async patterns include: 1) Saga patterns for orchestration, 2) State machines for workflow management, 3)...
AR/VR state management involves: 1) Real-time state updates for position/orientation, 2) Scene graph state...
Complex navigation patterns involve combining multiple navigators, handling nested navigation state, implementing...
State restoration involves persisting navigation state, handling deep links, managing authentication state, and...
Custom navigators require implementing core navigation logic, gesture handling, transition animations, and proper...
Large-scale navigation involves modular navigation configuration, code splitting, proper type definitions, state...
Offline navigation requires state persistence, queue management for navigation actions, proper error handling, and...
Multi-window navigation involves managing separate navigation states, synchronizing navigation between windows,...
Navigation analytics involves tracking screen views, navigation patterns, user flows, and interaction metrics....
AR/VR navigation requires custom transition animations, gesture handling for 3D space, maintaining spatial...
Accessible navigation includes proper focus management, screen reader support, gesture alternatives, and clear...
Large-scale optimization involves: 1) Implementing proper architecture, 2) Code splitting strategies, 3) Performance...
Native module optimization includes: 1) Proper thread management, 2) Efficient data serialization, 3) Batch...
Data virtualization involves: 1) Implementing windowing techniques, 2) Managing data chunks, 3) Implementing...
Offline optimization includes: 1) Efficient data synchronization, 2) State persistence strategies, 3) Conflict...
Performance monitoring includes: 1) Metric collection implementation, 2) Analysis system setup, 3) Alert mechanism...
AR/VR optimization includes: 1) Efficient render pipeline, 2) Asset management strategies, 3) Frame rate...
Testing automation includes: 1) Performance benchmark setup, 2) Continuous monitoring implementation, 3) Regression...
Multi-window optimization includes: 1) Resource sharing strategies, 2) State synchronization optimization, 3) Memory...
Complex features often require deep platform integration, custom native modules, and platform-specific...
Hardware feature access requires platform-specific implementations for cameras, sensors, Bluetooth, and other...
UI automation requires different tools and approaches (XCTest for iOS, Espresso for Android). This includes...
App extensions (widgets, share extensions, etc.) require platform-specific implementation and configuration. This...
AR features require platform-specific implementations using ARKit (iOS) and ARCore (Android). This includes...
Payment integration requires platform-specific implementations for Apple Pay and Google Pay. This includes different...
Background processing requires different implementations for iOS background modes and Android services. This...
Accessibility implementation requires platform-specific considerations for VoiceOver (iOS) and TalkBack (Android)....
Deep linking requires platform-specific configuration for Universal Links (iOS) and App Links (Android). This...
App signing and deployment differ significantly between iOS (certificates, provisioning profiles) and Android...
Complex layouts require combination of flexbox, absolute positioning, proper nesting, and possibly custom native...
Design systems implementation involves creating reusable components, centralized theme management, consistent...
Complex interactions require combination of Animated API, PanResponder, proper layout measurement, and performance...
Custom graphics require SVG implementation, canvas-like solutions, or native modules. Consider performance...
Advanced responsive layouts involve dynamic layout switching, complex grid systems, proper breakpoint management,...
Custom transitions require understanding of the Animated API, proper timing functions, layout measurement, and...
Advanced theming involves dynamic theme switching, proper context usage, style generation systems, and efficient...
Micro-interactions require fine-tuned animations, proper gesture handling, and efficient state management. Consider...
Custom layout engines require understanding of native modules, layout calculation systems, and proper integration...
GraphQL implementation involves setting up Apollo Client or similar libraries, implementing proper cache management,...
Real-time sync involves WebSockets, server-sent events, or polling strategies. Consider optimistic updates, conflict...
Complex transformations require proper data normalization, efficient algorithms, consideration of performance...
Large-scale architectures require proper service organization, API gateway implementation, microservices...
Efficient pagination involves cursor-based or offset-based strategies, proper cache management, infinite scroll...
API middleware implementation involves request/response interceptors, logging, analytics tracking, error handling,...
Advanced caching involves implementing stale-while-revalidate, cache-then-network, and proper cache invalidation...
Complex authentication involves OAuth flows, token refresh mechanisms, biometric authentication integration, and...
API monitoring involves implementing proper logging, performance tracking, error reporting, and usage analytics....
API testing involves implementing proper test coverage, mocking strategies, integration tests, and automated testing...
The New Architecture introduces Fabric (new rendering system) and TurboModules (improved native modules). It reduces bridge overhead, improves performance, and enables better native integration through codegen and static typing.
Fabric is a C++ rendering system that enables synchronous operations between JS and native code. It introduces a new threading model, improves layout performance, and enables concurrent rendering features.
TurboModules provide direct JS to native communication without serialization overhead. They use codegen to create type-safe interfaces, lazy load modules, and enable better memory management compared to traditional native modules.
React Native manages memory through automatic garbage collection in JS and ARC/GC in native code. Understanding the bridge's role in memory usage, implementing proper cleanup in components, and monitoring memory leaks are crucial.
JSI enables direct communication between JavaScript and native code without going through the bridge. It provides a way to hold native references in JavaScript and enables synchronous native method calls.
Native crashes are handled through platform-specific crash reporting tools and services. Integration requires native module setup, symbolication for JavaScript stack traces, and proper error boundary implementation.
Security involves handling data encryption, secure storage, certificate pinning, code obfuscation, and protecting against common mobile vulnerabilities. Platform-specific security features must be properly implemented.
App initialization involves native bootstrap, JS engine initialization, bundle loading, and component mounting. Understanding this process is crucial for optimizing startup time and implementing splash screens.
Custom native components require native code implementation (Java/Kotlin for Android, Objective-C/Swift for iOS) and JavaScript interface. This involves creating native view managers, implementing view properties, and handling events through the bridge.
Rerendering optimization involves: 1) Using React.memo for functional components, 2) Implementing shouldComponentUpdate for class components, 3) Proper key usage, 4) State structure optimization, 5) Callback memoization with useCallback, and 6) Value memoization with useMemo.
Virtualized list challenges include: 1) Memory management for large datasets, 2) Smooth scrolling performance, 3) Handling variable height items, 4) Implementing pull-to-refresh and infinite scroll, 5) Managing scroll position restoration, and 6) Platform-specific optimizations.
Complex gestures require the PanResponder system or gesture libraries. Implementation involves handling gesture recognition, touch responder negotiation, animation integration, and proper cleanup. Consider interaction with scrolling views and native gesture handlers.
Lazy loading strategies include: 1) React.lazy with Suspense, 2) Dynamic imports, 3) Route-based code splitting, 4) Component prefetching, and 5) Loading state management. Consider bundle size impact and user experience during loading.
Error boundaries are components that catch JavaScript errors in their child component tree. Implementation involves getDerivedStateFromError and componentDidCatch lifecycle methods, fallback UI rendering, and error reporting integration.
Accessibility implementation includes: 1) Proper use of accessibility props, 2) Semantic markup, 3) Focus management, 4) Screen reader support, 5) Color contrast, 6) Touch target sizing, and 7) Platform-specific accessibility guidelines.
Offline-first state management requires: 1) Local state persistence, 2) Conflict resolution strategies, 3) Queue management for offline actions, 4) State synchronization upon reconnection, 5) Optimistic UI updates with proper error handling.
Large-scale state management involves: 1) Domain-driven state organization, 2) State normalization, 3) Module-based state splitting, 4) Lazy loading state modules, 5) State hydration strategies, 6) Performance optimization patterns.
Multi-window state management requires: 1) State synchronization between windows, 2) Shared storage mechanisms, 3) Event-based communication, 4) Consistent state updates across windows, 5) Handle window lifecycle events.
Microservices state management involves: 1) Domain-based state separation, 2) Service boundary definition, 3) State consistency patterns, 4) Event-driven state updates, 5) Distributed state management strategies.
Real-time collaboration requires: 1) Operational transformation or CRDT implementation, 2) State conflict resolution, 3) Presence management, 4) State versioning, 5) Optimistic updates with proper conflict handling.
Complex async patterns include: 1) Saga patterns for orchestration, 2) State machines for workflow management, 3) Queue-based state updates, 4) Retry and recovery strategies, 5) Transaction-like state updates.
AR/VR state management involves: 1) Real-time state updates for position/orientation, 2) Scene graph state management, 3) Object interaction state, 4) Performance optimization for state updates, 5) Platform-specific state handling.
Large-scale optimization involves: 1) Implementing proper architecture, 2) Code splitting strategies, 3) Performance monitoring systems, 4) Efficient state management, 5) Proper resource management, 6) Optimization automation.
Native module optimization includes: 1) Proper thread management, 2) Efficient data serialization, 3) Batch processing implementation, 4) Memory management strategies, 5) Proper error handling, 6) Performance benchmarking.
Data virtualization involves: 1) Implementing windowing techniques, 2) Managing data chunks, 3) Implementing efficient scrolling, 4) Memory management strategies, 5) Optimizing render performance, 6) Handling large datasets.
Offline optimization includes: 1) Efficient data synchronization, 2) State persistence strategies, 3) Conflict resolution handling, 4) Resource management, 5) Background processing optimization, 6) Storage optimization.
Performance monitoring includes: 1) Metric collection implementation, 2) Analysis system setup, 3) Alert mechanism creation, 4) Performance tracking automation, 5) Reporting system implementation, 6) Optimization feedback loops.
AR/VR optimization includes: 1) Efficient render pipeline, 2) Asset management strategies, 3) Frame rate optimization, 4) Memory usage management, 5) Thread management, 6) Native integration optimization.
Testing automation includes: 1) Performance benchmark setup, 2) Continuous monitoring implementation, 3) Regression testing strategies, 4) Automated optimization verification, 5) Performance metric tracking, 6) Test environment management.
Multi-window optimization includes: 1) Resource sharing strategies, 2) State synchronization optimization, 3) Memory management approaches, 4) Process communication efficiency, 5) UI thread management, 6) Context switching optimization.
Complex features often require deep platform integration, custom native modules, and platform-specific optimizations. This includes handling different APIs, lifecycle management, and maintaining consistent behavior across platforms.
Hardware feature access requires platform-specific implementations for cameras, sensors, Bluetooth, and other hardware components. This includes different APIs, permissions, and optimization strategies.
UI automation requires different tools and approaches (XCTest for iOS, Espresso for Android). This includes different test writing strategies, CI/CD integration, and handling platform-specific UI elements.
App extensions (widgets, share extensions, etc.) require platform-specific implementation and configuration. This includes different development approaches, data sharing mechanisms, and lifecycle management.
AR features require platform-specific implementations using ARKit (iOS) and ARCore (Android). This includes different initialization, tracking, and rendering approaches while maintaining consistent AR experiences.
Payment integration requires platform-specific implementations for Apple Pay and Google Pay. This includes different APIs, security requirements, and user experience considerations while maintaining consistent payment flows.
Background processing requires different implementations for iOS background modes and Android services. This includes handling different lifecycle events, scheduling mechanisms, and power management considerations.
Accessibility implementation requires platform-specific considerations for VoiceOver (iOS) and TalkBack (Android). This includes different markup, focus management, and gesture handling while maintaining consistent accessibility.
Deep linking requires platform-specific configuration for Universal Links (iOS) and App Links (Android). This includes different setup processes, validation requirements, and handling mechanisms.
App signing and deployment differ significantly between iOS (certificates, provisioning profiles) and Android (keystore). This includes different build processes, signing mechanisms, and deployment procedures.
Complex layouts require combination of flexbox, absolute positioning, proper nesting, and possibly custom native components. Consider performance implications, maintainability, and cross-platform consistency.
Design systems implementation involves creating reusable components, centralized theme management, consistent spacing/typography systems, and proper component composition. Consider maintenance, scalability, and team collaboration.
Complex interactions require combination of Animated API, PanResponder, proper layout measurement, and performance optimization. Consider native driver usage, gesture handling, and proper cleanup of animations.
Custom graphics require SVG implementation, canvas-like solutions, or native modules. Consider performance implications, platform differences, and proper integration with React Native's layout system.
Advanced responsive layouts involve dynamic layout switching, complex grid systems, proper breakpoint management, and orientation handling. Consider performance, maintainability, and testing across different devices.
Custom transitions require understanding of the Animated API, proper timing functions, layout measurement, and platform-specific considerations. Consider performance, gesture integration, and proper cleanup.
Advanced theming involves dynamic theme switching, proper context usage, style generation systems, and efficient updates. Consider performance implications, maintainability, and proper typing support.
Micro-interactions require fine-tuned animations, proper gesture handling, and efficient state management. Consider performance, user feedback, and proper integration with the overall UX.
Custom layout engines require understanding of native modules, layout calculation systems, and proper integration with React Native. Consider performance implications, maintenance complexity, and cross-platform support.
GraphQL implementation involves setting up Apollo Client or similar libraries, implementing proper cache management, handling queries/mutations, and managing local state. Consider code generation and type safety.
Real-time sync involves WebSockets, server-sent events, or polling strategies. Consider optimistic updates, conflict resolution, proper error handling, and state management for real-time data.
Complex transformations require proper data normalization, efficient algorithms, consideration of performance impact, and possibly using web workers. Consider implementing caching for expensive transformations.
Large-scale architectures require proper service organization, API gateway implementation, microservices integration, and efficient data flow management. Consider implementing proper error boundaries and fallback strategies.
Efficient pagination involves cursor-based or offset-based strategies, proper cache management, infinite scroll implementation, and optimized data fetching. Consider implementing virtual scrolling for large datasets.
API middleware implementation involves request/response interceptors, logging, analytics tracking, error handling, and authentication management. Consider implementing proper middleware composition and order.
Advanced caching involves implementing stale-while-revalidate, cache-then-network, and proper cache invalidation strategies. Consider implementing proper cache hierarchies and optimization techniques.
Complex authentication involves OAuth flows, token refresh mechanisms, biometric authentication integration, and proper security measures. Consider implementing proper state management and error handling.
API monitoring involves implementing proper logging, performance tracking, error reporting, and usage analytics. Consider implementing proper monitoring infrastructure and alerting systems.
API testing involves implementing proper test coverage, mocking strategies, integration tests, and automated testing pipelines. Consider implementing proper test data management and CI/CD integration.
Solve React Native development challenges tailored for interviews.
Explore MoreUnderstand core components, props, and state management techniques.
Learn best practices for handling animations, rendering optimizations, and memory management.
Understand how to integrate native code for platform-specific functionalities.
Familiarize yourself with React Native debugging tools and common issue resolution techniques.
Join thousands of successful candidates preparing with Stark.ai. Start practicing React Native questions, mock interviews, and more to secure your dream role.
Start Preparing now