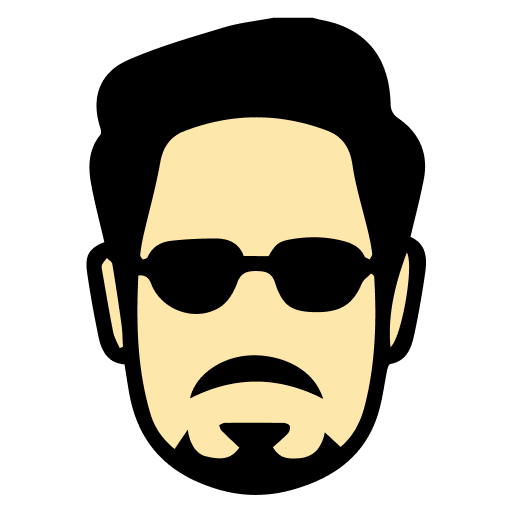
PHP powers a vast portion of web applications and remains a crucial server-side technology. Stark.ai offers a comprehensive collection of PHP interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
PHP has four variable scopes: local (inside functions), global (outside functions), static (retains value between...
Magic constants are predefined constants that change based on where they are used. Examples include __LINE__,...
The final keyword prevents child classes from overriding a method or prevents a class from being inherited. When...
Type hinting enforces specified data types for function parameters and return values. It can be used with arrays,...
Anonymous functions, also known as closures, are functions without a name that can access variables from the outside...
self refers to the current class and is used to access static members, while $this refers to the current object...
Namespaces are a way of encapsulating related classes, interfaces, functions, and constants to avoid name...
unset() completely removes a variable from memory, while setting a variable to null keeps the variable but sets its...
Abstract classes can have properties and implemented methods, while interfaces can only have method signatures. A...
The callable type hint ensures that a function parameter can be called as a function. It accepts regular functions,...
Method chaining is a technique where multiple methods are called on the same object in a single line. It's achieved...
Static methods belong to the class itself and can be called without creating an instance of the class. They cannot...
Magic methods are special methods that override PHP's default behavior. Common ones include: __construct()...
Interfaces define a contract for classes by specifying which methods must be implemented. They promote loose...
Abstract classes can have both abstract and concrete methods, while interfaces can only declare method signatures. A...
Anonymous classes are classes without names, defined on-the-fly. They are useful when you need a simple, one-off...
Property type declarations allow you to specify the type of class properties. They can be scalar types, arrays,...
PHP doesn't support multiple inheritance directly, but it can be achieved through interfaces and traits. A class can...
Static binding (self::) refers to the class where the method is defined, while late static binding (static::) refers...
Named arguments allow you to pass values to a function by specifying the parameter name, regardless of their order....
Prepared statements separate SQL logic from data by using placeholders for values. The database treats these values...
A transaction is a sequence of operations that must be executed as a single unit. In PHP, transactions are...
mysql_real_escape_string() escapes special characters in strings, but is deprecated and can be bypassed. Prepared...
PDO offers several fetch modes: FETCH_ASSOC (returns associative array), FETCH_NUM (returns numeric array),...
Database seeders are scripts that populate a database with initial or test data. They're useful for development...
Indexes are data structures that improve the speed of data retrieval operations. They should be used on columns...
Triggers are special procedures that automatically execute when certain database events occur (INSERT, UPDATE,...
Stored procedures are pre-compiled SQL statements stored in the database. In PHP, they're called using CALL...
Lazy loading delays database connection initialization until it's actually needed. This saves resources by not...
Views are virtual tables based on result sets of SQL statements. In PHP, they're queried like regular tables but...
Database backup can be implemented using PHP functions to execute system commands, dedicated backup libraries, or...
Database events are notifications of database changes that can trigger PHP code execution. They can be handled using...
Connection retry logic involves implementing exponential backoff, maximum retry attempts, proper error handling, and...
Key security considerations include: using session_regenerate_id() to prevent session fixation, setting secure and...
Session timeout can be implemented by: setting session.gc_maxlifetime in php.ini, storing last activity timestamp in...
Remember me involves: generating secure token, storing hashed token in database, setting long-lived cookie with...
Cookie limitations include: size (4KB), number per domain, browser settings blocking cookies. Workarounds include:...
session_cache_limiter() controls HTTP caching of pages with sessions. Options include: nocache, private, public,...
SameSite cookie attribute controls how cookie is sent with cross-site requests. Values: Strict, Lax, None. Helps...
Key options include: session.save_handler, session.save_path, session.gc_maxlifetime, session.cookie_lifetime,...
Garbage collection removes expired session data. Controlled by session.gc_probability, session.gc_divisor, and...
Session authentication involves: validating credentials, storing user data in session, implementing session security...
Flash data persists for only one request cycle, commonly used for temporary messages. Implementation involves...
Best practices include: using HTTPS, setting secure/httponly flags, implementing proper session timeout,...
Domain cookies are accessible across subdomains. Set using domain parameter in setcookie(). Used for maintaining...
Secure file uploads require: validating file types, checking MIME types, setting upload size limits, using...
File permissions are managed using chmod() function, umask() for default permissions. Functions like is_readable(),...
Secure file downloads require: validating file paths, checking permissions, setting proper headers (Content-Type,...
Temporary files managed using tmpfile() for automatic cleanup, tempnam() for custom temp files. Important to...
CSV operations use fgetcsv()/fputcsv() for reading/writing, handling different delimiters and enclosures. Consider...
Symbolic links managed with symlink(), readlink(), and is_link() functions. Security considerations include proper...
File compression using zlib functions (gzopen, gzwrite, gzread) or ZIP extension. Important considerations include...
File type detection using: finfo_file(), mime_content_type(), pathinfo(), checking file extensions. Important for...
Secure path manipulation using realpath(), basename(), dirname(). Prevent directory traversal, validate paths...
Memory considerations include: using streaming for large files, proper chunk sizes, clearing file handles,...
Encoding issues handled using mb_* functions, setting proper encoding in fopen(), handling BOM, implementing...
File ownership managed using chown(), chgrp() functions. Important for security and permissions management. Consider...
File cleanup includes: implementing age-based deletion, handling temporary files, proper permission checking,...
Error handling includes: try-catch blocks, checking return values, implementing logging, proper user feedback, and...
Routing maps URLs to controller actions. Features include: route parameters, middleware, route groups, named routes,...
Symfony is a framework and component library. Key features: reusable components, dependency injection, event...
Middleware processes HTTP requests/responses before reaching controllers. Used for: authentication, CSRF protection,...
Object-Relational Mappers (ORMs) like Eloquent or Doctrine map database tables to objects. Features: relationship...
Template engines (Blade, Twig) provide syntax for views. Features: template inheritance, sections, partials,...
Migrations version control database schema changes. Seeders populate databases with test/initial data. Features:...
Frameworks provide authentication systems with: user providers, guards, middleware, password hashing, remember me...
Frameworks provide validation systems with: built-in rules, custom validators, error messaging, form requests, CSRF...
Security features include: CSRF protection, XSS prevention, SQL injection protection, authentication, authorization,...
CLI tools provide commands for common tasks: generating code, running migrations, clearing cache, scheduling tasks....
Lumen is Laravel's micro-framework for microservices and APIs. Features: fast routing, basic Laravel features,...
Localization features include: language files, translation helpers, plural forms, date/number formatting. Support...
File storage abstraction supports: local storage, cloud storage (S3, etc.), FTP. Features include: file uploads,...
Collections provide fluent interface for array operations. Features: mapping, filtering, reducing, sorting,...
API authentication methods include: JWT tokens, OAuth 2.0, API keys, Basic Auth, Bearer tokens. Implementation...
Best practices include: consistent response structure, proper HTTP status codes, clear error messages, pagination...
CORS (Cross-Origin Resource Sharing) handled through proper headers: Access-Control-Allow-Origin, Allow-Methods,...
Resources/transformers format API responses: converting models to JSON/arrays, handling relationships, hiding...
File upload handling includes: multipart form data, proper validation, secure storage, progress tracking. Consider...
API documentation tools include: Swagger/OpenAPI, API Blueprint, automated documentation generation. Include:...
Common threats include: injection attacks, unauthorized access, MITM attacks, DoS/DDoS, data exposure. Implement:...
Error handling includes: proper HTTP status codes, consistent error format, detailed messages, error logging....
Pagination methods include: offset/limit, cursor-based, page-based. Include metadata (total, next/prev links),...
JWTs are encoded tokens containing claims. Structure: header, payload, signature. Used for...
Request validation includes: input sanitization, schema validation, type checking, business rule validation....
Search implementation includes: query parameters, filtering, sorting, full-text search. Consider search engines...
Deprecation strategy includes: versioning, notification period, documentation updates, migration guides. Implement...
Analytics implementation includes: usage tracking, performance metrics, error rates, user behavior. Consider data...
PHP has four variable scopes: local (inside functions), global (outside functions), static (retains value between function calls), and superglobal (accessible everywhere). The 'global' keyword or $GLOBALS array is used to access global variables inside functions.
Magic constants are predefined constants that change based on where they are used. Examples include __LINE__, __FILE__, __DIR__, __FUNCTION__, __CLASS__, __METHOD__, __NAMESPACE__. Their values are determined by where they are used.
The final keyword prevents child classes from overriding a method or prevents a class from being inherited. When used with methods, it prevents method overriding, and when used with classes, it prevents class inheritance.
Type hinting enforces specified data types for function parameters and return values. It can be used with arrays, objects, interfaces, and scalar types (in PHP 7+). It helps catch type-related errors early and improves code reliability.
Anonymous functions, also known as closures, are functions without a name that can access variables from the outside scope. They are often used as callback functions and can be assigned to variables.
self refers to the current class and is used to access static members, while $this refers to the current object instance and is used to access non-static members. self is resolved at compile time, while $this is resolved at runtime.
Namespaces are a way of encapsulating related classes, interfaces, functions, and constants to avoid name collisions. They provide better organization and reusability of code. They are declared using the namespace keyword.
unset() completely removes a variable from memory, while setting a variable to null keeps the variable but sets its value to nothing. isset() returns false for both null values and unset variables.
Abstract classes can have properties and implemented methods, while interfaces can only have method signatures. A class can implement multiple interfaces but can extend only one abstract class. Abstract classes provide a partial implementation.
The callable type hint ensures that a function parameter can be called as a function. It accepts regular functions, object methods, static class methods, and closure functions. It helps enforce that passed parameters are actually callable.
Method chaining is a technique where multiple methods are called on the same object in a single line. It's achieved by returning $this from methods, allowing subsequent method calls. For example: $object->method1()->method2()->method3(). This creates more readable and fluent interfaces.
Static methods belong to the class itself and can be called without creating an instance of the class. They cannot access non-static properties/methods using $this. Non-static methods belong to class instances and can access all class members. Static methods are called using the scope resolution operator (::).
Magic methods are special methods that override PHP's default behavior. Common ones include: __construct() (constructor), __destruct() (destructor), __get() (accessing inaccessible properties), __set() (writing to inaccessible properties), __call() (calling inaccessible methods), __toString() (string representation of object).
Interfaces define a contract for classes by specifying which methods must be implemented. They promote loose coupling, enable polymorphism, and allow different classes to share a common contract. A class can implement multiple interfaces, unlike inheritance where a class can only extend one class.
Abstract classes can have both abstract and concrete methods, while interfaces can only declare method signatures. A class can implement multiple interfaces but extend only one abstract class. Abstract classes can have properties and constructor, while interfaces cannot.
Anonymous classes are classes without names, defined on-the-fly. They are useful when you need a simple, one-off object that implements an interface or extends a class. They're commonly used in testing or when you need quick object creation without formal class definition.
Property type declarations allow you to specify the type of class properties. They can be scalar types, arrays, classes, interfaces, or nullable types using ?. They ensure type safety at the property level and help catch type-related errors early.
PHP doesn't support multiple inheritance directly, but it can be achieved through interfaces and traits. A class can implement multiple interfaces and use multiple traits. Traits provide actual method implementations, while interfaces define contracts.
Static binding (self::) refers to the class where the method is defined, while late static binding (static::) refers to the class that was initially called at runtime. Late static binding allows for more flexible inheritance and method calls in static contexts.
Named arguments allow you to pass values to a function by specifying the parameter name, regardless of their order. In OOP, this improves code readability, especially with multiple optional parameters, and makes constructor calls and method invocations more explicit and maintainable.
Prepared statements separate SQL logic from data by using placeholders for values. The database treats these values as data rather than part of the SQL command, preventing injection attacks. Values are automatically escaped, and the query structure remains constant, improving security and performance.
A transaction is a sequence of operations that must be executed as a single unit. In PHP, transactions are implemented using beginTransaction(), commit(), and rollback() methods. If any operation fails, rollback() ensures all operations are undone, maintaining data integrity.
mysql_real_escape_string() escapes special characters in strings, but is deprecated and can be bypassed. Prepared statements are more secure as they separate SQL from data, handle different data types automatically, and are more efficient due to query preparation and caching.
PDO offers several fetch modes: FETCH_ASSOC (returns associative array), FETCH_NUM (returns numeric array), FETCH_BOTH (returns both), FETCH_OBJ (returns object), FETCH_CLASS (returns instance of specified class), and FETCH_LAZY (allows property access of all three).
Database seeders are scripts that populate a database with initial or test data. They're useful for development environments, testing, and providing default data. Seeders help ensure consistent data across different environments and make testing more reliable.
Indexes are data structures that improve the speed of data retrieval operations. They should be used on columns frequently used in WHERE clauses, JOIN conditions, and ORDER BY statements. However, they add overhead to write operations and consume storage space.
Triggers are special procedures that automatically execute when certain database events occur (INSERT, UPDATE, DELETE). In PHP, triggers are defined at database level but can be created and managed through PHP code. They help maintain data integrity and automate actions.
Stored procedures are pre-compiled SQL statements stored in the database. In PHP, they're called using CALL statement with PDO or MySQLi. They can improve performance, reduce network traffic, and encapsulate business logic at database level.
Lazy loading delays database connection initialization until it's actually needed. This saves resources by not establishing connections unnecessarily. It's implemented by wrapping connection logic in methods that are called only when database access is required.
Views are virtual tables based on result sets of SQL statements. In PHP, they're queried like regular tables but provide benefits like data abstraction, security through limited access, and simplified complex queries. They help maintain clean application architecture.
Database backup can be implemented using PHP functions to execute system commands, dedicated backup libraries, or framework tools. Important aspects include scheduling backups, compression, secure storage, verification, and testing recovery procedures.
Database events are notifications of database changes that can trigger PHP code execution. They can be handled using event listeners, message queues, or polling mechanisms. This enables real-time updates and maintaining data consistency across systems.
Connection retry logic involves implementing exponential backoff, maximum retry attempts, proper error handling, and logging. It helps handle temporary connection issues and improves application reliability. Implementation typically uses try-catch blocks with sleep intervals.
Key security considerations include: using session_regenerate_id() to prevent session fixation, setting secure and httponly flags, implementing session timeout, validating session data, proper session destruction, and securing session storage location.
Session timeout can be implemented by: setting session.gc_maxlifetime in php.ini, storing last activity timestamp in session, checking elapsed time on each request, and destroying session if timeout exceeded. Also consider implementing sliding expiration.
Remember me involves: generating secure token, storing hashed token in database, setting long-lived cookie with token, validating token on subsequent visits. Implementation should include token rotation, secure storage, and proper expiration handling.
Cookie limitations include: size (4KB), number per domain, browser settings blocking cookies. Workarounds include: using local storage for larger data, implementing fallback mechanisms, splitting data across multiple cookies, server-side storage alternatives.
session_cache_limiter() controls HTTP caching of pages with sessions. Options include: nocache, private, public, private_no_expire. Affects how browsers and proxies cache session pages. Important for security and proper page caching.
SameSite cookie attribute controls how cookie is sent with cross-site requests. Values: Strict, Lax, None. Helps prevent CSRF attacks and protects against cross-site request attacks. Important for modern web security compliance.
Key options include: session.save_handler, session.save_path, session.gc_maxlifetime, session.cookie_lifetime, session.cookie_secure, session.cookie_httponly. These control session behavior, storage, lifetime, and security settings.
Garbage collection removes expired session data. Controlled by session.gc_probability, session.gc_divisor, and session.gc_maxlifetime settings. Process runs randomly based on probability settings. Important for server resource management.
Session authentication involves: validating credentials, storing user data in session, implementing session security measures, handling remember me functionality, implementing proper logout, and managing session expiration.
Flash data persists for only one request cycle, commonly used for temporary messages. Implementation involves storing data in session, checking for data existence, displaying data, and removing after use. Often used for success/error messages.
Best practices include: using HTTPS, setting secure/httponly flags, implementing proper session timeout, regenerating session IDs, validating session data, secure storage, proper destruction, and implementing CSRF protection.
Domain cookies are accessible across subdomains. Set using domain parameter in setcookie(). Used for maintaining user state across subdomains, implementing single sign-on, sharing necessary data between related sites. Requires careful security consideration.
Secure file uploads require: validating file types, checking MIME types, setting upload size limits, using move_uploaded_file(), scanning for malware, storing files outside web root, using random filenames, setting proper permissions, and validating file contents.
File permissions are managed using chmod() function, umask() for default permissions. Functions like is_readable(), is_writable() check permissions. Important for security. Permissions should follow principle of least privilege.
Secure file downloads require: validating file paths, checking permissions, setting proper headers (Content-Type, Content-Disposition), implementing rate limiting, scanning files, and using readfile() or fpassthru() for streaming.
Temporary files managed using tmpfile() for automatic cleanup, tempnam() for custom temp files. Important to implement proper cleanup, set secure permissions, use system temp directory, and handle concurrent access properly.
CSV operations use fgetcsv()/fputcsv() for reading/writing, handling different delimiters and enclosures. Consider character encoding, handling large files, validating data, and implementing proper error handling.
Symbolic links managed with symlink(), readlink(), and is_link() functions. Security considerations include proper validation, handling recursive links, and implementing access controls. Important for file system organization.
File compression using zlib functions (gzopen, gzwrite, gzread) or ZIP extension. Important considerations include compression ratio, memory usage, handling large files, and proper error handling.
File type detection using: finfo_file(), mime_content_type(), pathinfo(), checking file extensions. Important for security in file uploads. Should not rely solely on file extensions for validation.
Secure path manipulation using realpath(), basename(), dirname(). Prevent directory traversal, validate paths against whitelist, use proper encoding, and implement access controls. Important for security.
Memory considerations include: using streaming for large files, proper chunk sizes, clearing file handles, implementing garbage collection, monitoring memory usage, and setting appropriate memory limits. Important for performance.
Encoding issues handled using mb_* functions, setting proper encoding in fopen(), handling BOM, implementing conversion functions. Important for international character support and data integrity.
File ownership managed using chown(), chgrp() functions. Important for security and permissions management. Consider system-level permissions, proper error handling, and security implications.
File cleanup includes: implementing age-based deletion, handling temporary files, proper permission checking, implementing logging, and error handling. Consider automated scheduling and resource management.
Error handling includes: try-catch blocks, checking return values, implementing logging, proper user feedback, and recovery mechanisms. Consider different error types and appropriate response strategies.
Routing maps URLs to controller actions. Features include: route parameters, middleware, route groups, named routes, resource routing. Frameworks handle request parsing, parameter binding, and response generation. Supports RESTful routing patterns.
Symfony is a framework and component library. Key features: reusable components, dependency injection, event dispatcher, console tools, security system. Components can be used independently in other projects. Follows SOLID principles.
Middleware processes HTTP requests/responses before reaching controllers. Used for: authentication, CSRF protection, logging, request modification. Can be global or route-specific. Implements pipeline pattern for request handling.
Object-Relational Mappers (ORMs) like Eloquent or Doctrine map database tables to objects. Features: relationship handling, query building, migrations, model events. Simplifies database operations and provides abstraction layer.
Template engines (Blade, Twig) provide syntax for views. Features: template inheritance, sections, partials, escaping, custom directives. Compiles templates to plain PHP for performance. Separates logic from presentation.
Migrations version control database schema changes. Seeders populate databases with test/initial data. Features: rollback capability, timestamps, factory patterns. Essential for database version control and testing.
Frameworks provide authentication systems with: user providers, guards, middleware, password hashing, remember me functionality, OAuth support. Includes session management, token authentication, and multiple authentication schemes.
Frameworks provide validation systems with: built-in rules, custom validators, error messaging, form requests, CSRF protection. Supports client and server-side validation, file validation, and complex validation scenarios.
Security features include: CSRF protection, XSS prevention, SQL injection protection, authentication, authorization, encryption, password hashing. Frameworks provide middleware and helpers for common security needs.
CLI tools provide commands for common tasks: generating code, running migrations, clearing cache, scheduling tasks. Support custom commands, interactive mode. Essential for development and deployment workflows.
Lumen is Laravel's micro-framework for microservices and APIs. Features: fast routing, basic Laravel features, minimal overhead. Best for simple applications, APIs where full framework unnecessary.
Localization features include: language files, translation helpers, plural forms, date/number formatting. Support multiple languages, language switching, fallback locales. Important for international applications.
File storage abstraction supports: local storage, cloud storage (S3, etc.), FTP. Features include: file uploads, storage drivers, file operations, URL generation. Provides consistent interface across storage systems.
Collections provide fluent interface for array operations. Features: mapping, filtering, reducing, sorting, grouping. Extends array functionality with object-oriented interface. Important for data manipulation.
API authentication methods include: JWT tokens, OAuth 2.0, API keys, Basic Auth, Bearer tokens. Implementation involves token generation, validation, middleware for protection, and proper error handling. Consider security best practices like token expiration.
Best practices include: consistent response structure, proper HTTP status codes, clear error messages, pagination metadata, proper content type headers. Use envelope pattern when needed, handle nested resources, implement proper serialization.
CORS (Cross-Origin Resource Sharing) handled through proper headers: Access-Control-Allow-Origin, Allow-Methods, Allow-Headers. Implementation includes preflight requests handling, proper middleware configuration, security considerations.
Resources/transformers format API responses: converting models to JSON/arrays, handling relationships, hiding sensitive data. Features include conditional attributes, nested resources, custom transformations. Important for consistent response formatting.
File upload handling includes: multipart form data, proper validation, secure storage, progress tracking. Consider chunked uploads for large files, implement proper error handling, use secure file operations.
API documentation tools include: Swagger/OpenAPI, API Blueprint, automated documentation generation. Include: endpoints, parameters, responses, examples. Keep documentation updated, consider interactive documentation.
Common threats include: injection attacks, unauthorized access, MITM attacks, DoS/DDoS, data exposure. Implement: authentication, rate limiting, input validation, proper encryption, secure headers.
Error handling includes: proper HTTP status codes, consistent error format, detailed messages, error logging. Implement global exception handler, format exceptions properly, consider security in error messages.
Pagination methods include: offset/limit, cursor-based, page-based. Include metadata (total, next/prev links), handle large datasets, implement proper caching. Consider performance and use case requirements.
JWTs are encoded tokens containing claims. Structure: header, payload, signature. Used for authentication/authorization. Implementation includes token generation, validation, refresh mechanisms. Consider security implications.
Request validation includes: input sanitization, schema validation, type checking, business rule validation. Implement validation middleware, proper error responses, custom validation rules. Consider performance impact.
Search implementation includes: query parameters, filtering, sorting, full-text search. Consider search engines integration, performance optimization, proper indexing. Implement relevance scoring.
Deprecation strategy includes: versioning, notification period, documentation updates, migration guides. Implement warning headers, monitoring deprecated usage, proper communication. Consider client impact.
Analytics implementation includes: usage tracking, performance metrics, error rates, user behavior. Consider data collection, storage, visualization tools. Implement proper privacy measures.
Understand concepts like OOP, functions, arrays, and error handling.
Work on database integration, API development, and security implementation.
Dive into frameworks, design patterns, and performance optimization.
Expect hands-on challenges to build, debug, and optimize PHP applications.
Join thousands of successful candidates preparing with Stark.ai. Start practicing PHP questions, mock interviews, and more to secure your dream role.
Start Preparing now