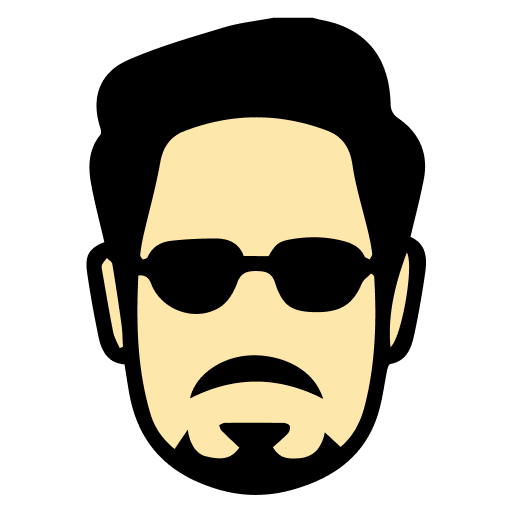
Next.js, a powerful React framework, is essential for building modern web applications with server-side rendering capabilities, making it a crucial skill for frontend and full-stack developers. Stark.ai offers a curated collection of Next.js interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Next.js is a React framework that provides features like server-side rendering, static site generation, file-based...
Create a Next.js project using 'npx create-next-app@latest' or 'yarn create next-app'. This sets up a new project...
Next.js project structure includes pages directory for routes, public for static assets, styles for CSS, components...
Core dependencies include React, React DOM, and Next.js itself. These are automatically included in package.json...
Default scripts include 'dev' for development server, 'build' for production build, 'start' for production server,...
Run development server using 'npm run dev' or 'yarn dev'. This starts the application with hot reloading at...
next.config.js is a configuration file for customizing Next.js behavior. It can configure webpack, environment...
Environment variables are handled using .env files (.env.local, .env.development, .env.production). Variables...
The pages directory uses the Pages Router, while app directory uses the newer App Router. App Router supports React...
TypeScript is supported out of the box. Create project with --typescript flag or add manually by creating...
App Router is newer, uses app directory, supports React Server Components, nested layouts, and improved routing...
In App Router, create routes by adding page.js files in app directory. In Pages Router, add files to pages...
Dynamic routes use square brackets for variable segments. Example: [id]/page.js creates dynamic route. Parameters...
Use Link component for client-side navigation. Example: <Link href='/about'>About</Link>. Provides automatic...
useRouter hook provides access to router object for programmatic navigation, route information, and parameters....
Create nested directories in app or pages folder. Each level can have its own page.js or layout.js. Supports nested...
Route groups use (groupName) syntax in app directory. Don't affect URL structure. Share layouts within groups....
Create not-found.js in app directory or 404.js in pages directory. Automatically shown for non-existent routes. Can...
Parallel routing allows simultaneous loading of multiple pages in same layout using @folder convention. Supports...
Access route parameters through params prop in page components or searchParams for query strings. Available in both...
Next.js 13+ provides async Server Components, fetch() with caching options, server actions, and client-side fetching...
Use async/await directly in Server Components. Example: async function Page() { const data = await...
Static data fetching occurs at build time using fetch with cache: 'force-cache' option. Data is cached and reused...
Use fetch with cache: 'no-store' option or revalidate: 0 for dynamic data. Data fetched on every request. Suitable...
ISR allows static pages to be updated after build time. Use fetch with revalidate option. Combines benefits of...
Use hooks like useState and useEffect in Client Components, or data fetching libraries like SWR or React Query....
Server Actions allow form handling and data mutations directly from Server Components. Use 'use server' directive....
Use fetch cache options or React cache function. Configure cache behavior in fetch requests. Support cache...
Fetch multiple data sources simultaneously using Promise.all or parallel routes. Improve performance by avoiding...
Create loading.js files for automatic loading UI. Use Suspense boundaries. Support streaming and progressive...
SSR generates HTML on the server for each request. Provides better initial page load and SEO. Next.js handles SSR...
Server Components run only on server, reduce client-side JavaScript. Support async data fetching. Cannot use...
Hydration attaches JavaScript functionality to server-rendered HTML. Happens automatically after initial page load....
Streaming SSR progressively sends HTML chunks as they're generated. Uses <Suspense> boundaries. Improves Time To...
Use async Server Components or getServerSideProps. Data available during render. Support server-only operations....
Better SEO, faster initial page load, improved performance on slow devices. Support social media previews. Handle...
Use 'use server' directive or .server.js files. Handle sensitive operations. Access server-side APIs. Support secure...
SSR cache stores rendered pages on server. Improves performance for subsequent requests. Supports cache...
Use error.js files for error boundaries. Handle server-side errors. Support fallback content. Implement error reporting.
SSR generates HTML per request, SSG at build time. SSR better for dynamic content, SSG for static. Trade-off between...
SSG generates HTML at build time instead of runtime. Pages are pre-rendered and can be served from CDN. Provides...
Use generateStaticParams for App Router or getStaticProps/getStaticPaths for Pages Router. Pages are built at build...
Define dynamic paths using generateStaticParams or getStaticPaths. Specify which paths to pre-render. Support...
Fallback controls behavior for non-generated paths. Options: false (404), true (loading), or 'blocking' (SSR)....
Fetch data during build using async components or getStaticProps. Data available at build time. Support external...
Fastest page loads, better SEO, reduced server load, improved security. Pages can be served from CDN. Support global...
Implement path validation during build. Handle invalid paths. Support custom validation. Implement error handling...
Data fetched during npm run build. Available for static page generation. Support external data sources. Handle...
Place assets in public directory. Support automatic optimization. Handle asset references. Implement asset...
API Routes are serverless endpoints built into Next.js. Created in pages/api directory (Pages Router) or app/api...
Create a file in app/api directory that exports default async function. Handle request methods (GET, POST, etc.)....
Export functions named after HTTP methods (GET, POST, PUT, DELETE). Or use conditional logic in Pages Router....
Access query params through request.nextUrl.searchParams in App Router or req.query in Pages Router. Parse and...
Access request body using await request.json() or similar methods. Validate request data. Process POST data. Return...
Use square brackets for dynamic segments [param]. Access parameters through route object. Support multiple dynamic...
Return appropriate status codes and error messages. Use try-catch blocks. Implement error handling middleware....
Configure CORS headers using middleware or within route handlers. Set Access-Control-Allow-Origin and other headers....
Middleware processes requests before reaching route handlers. Handle authentication, logging, CORS. Support...
Process multipart/form-data using appropriate middleware. Handle file storage. Validate file types and sizes....
Pages are special components that become routes automatically when placed in pages/ or app/ directory. Components...
Server Components are rendered on server by default in App Router. Cannot use browser APIs or React hooks. Better...
Client Components use 'use client' directive. Can use browser APIs and React hooks. Enable interactive features. Run...
Create layout.js file in app directory. Wraps child pages/components. Shares UI across routes. Supports nested...
Hydration attaches JavaScript event handlers to server-rendered HTML. Makes static content interactive. Happens...
Use metadata object or generateMetadata function in page files. Set title, description, open graph data. Support...
Create error.js files for error boundaries. Handle component errors. Support fallback content. Implement error...
Use [param] syntax for dynamic routes. Access parameters through props. Support multiple segments. Handle parameter...
Use React Context, state management libraries, or lift state up. Handle component communication. Support state...
Layout components are created using layout.js files. Share UI between pages. Support nested layouts. Handle...
Use .module.css files for component-scoped CSS. Import styles as objects. Support local class naming. Automatic...
Import global CSS in app/layout.js or pages/_app.js. Apply styles across all components. Support reset styles and...
Use media queries, CSS Grid, Flexbox. Support mobile-first design. Handle breakpoints. Implement responsive...
Create multiple layout.js files in route segments. Support layout hierarchy. Share UI between related routes. Handle...
Install and configure Tailwind CSS. Use utility classes. Support JIT mode. Handle Tailwind configuration. Implement...
Use (group) folders to organize routes. Don't affect URL structure. Share layouts within groups. Support multiple groups.
Use CSS-in-JS solutions or dynamic class names. Support runtime styles. Handle style variables. Implement dynamic theming.
Support styled-components, Emotion, and other CSS-in-JS libraries. Handle server-side rendering. Support dynamic...
Implement page and layout transitions. Support animation effects. Handle transition states. Implement smooth...
Next.js supports multiple state management options: React's built-in useState and useContext, external libraries...
Use useState hook for component-level state. Handle state updates. Support state initialization. Implement local...
Context API shares state between components without prop drilling. Create providers and consumers. Handle context...
Configure Redux store with Next.js. Handle server-side state hydration. Support Redux middleware. Implement Redux...
SWR is a React hooks library for data fetching. Handles caching, revalidation, and real-time updates. Support...
Use form libraries like React Hook Form or Formik. Handle form validation. Support form submission. Implement form...
Store state in localStorage or sessionStorage. Handle state recovery. Support persistence strategies. Implement...
Use query parameters and URL segments for state. Handle URL updates. Support navigation state. Implement URL-based...
Initialize state during server-side rendering. Handle state hydration. Support initial data loading. Implement SSR...
Manage loading, error, and success states. Handle async operations. Support state transitions. Implement async...
Next.js Image component is an extension of HTML <img> tag. Provides automatic image optimization. Supports lazy...
Import Image from 'next/image'. Add src, alt, width, and height props. Component optimizes and serves images...
Reduces image file size, improves page load speed, supports responsive images, optimizes for different devices,...
Images load only when they enter viewport. Reduces initial page load time. Uses loading='lazy' attribute...
Images adapt to different screen sizes. Uses sizes prop for viewport-based sizing. Supports srcset generation....
Use quality prop to set compression level. Default is 75. Balance between quality and file size. Support different...
Shows blurred version of image while loading. Use placeholder='blur' prop. Supports blurDataURL for custom blur....
Configure domains in next.config.js. Use loader prop for custom image service. Support remote image optimization....
Import images as modules. Get width and height automatically. Support webpack optimization. Handle static image assets.
Support WebP, AVIF formats. Use formats prop for specific formats. Handle browser compatibility. Implement format strategies.
Next.js supports multiple authentication methods: JWT, session-based, OAuth providers, NextAuth.js library. Can...
NextAuth.js is a complete authentication solution for Next.js applications. Provides built-in support for multiple...
Store JWT in HTTP-only cookies or local storage. Implement token verification. Handle token expiration. Support...
Store session data on server. Use session cookies for client identification. Handle session expiration. Support...
Configure OAuth providers. Handle OAuth flow. Support callback URLs. Implement user profile retrieval. Manage OAuth tokens.
Implement role checking middleware. Define user roles. Handle permission checks. Support role hierarchies. Implement...
Implement authentication middleware. Verify tokens or sessions. Handle unauthorized requests. Support API security....
Implement CSRF tokens. Handle token validation. Support form submissions. Implement security headers. Prevent...
Manage user authentication state. Handle state persistence. Support state updates. Implement state management....
Core Web Vitals are key metrics measuring user experience: LCP (Largest Contentful Paint), FID (First Input Delay),...
Code splitting automatically splits JavaScript bundles by route. Reduces initial bundle size. Supports dynamic...
Next.js Image component automatically optimizes images. Supports lazy loading, responsive sizes, modern formats....
Next.js automatically determines which pages can be statically generated. Improves page load performance. Supports...
Next.js Script component optimizes third-party script loading. Supports loading strategies (defer, lazy). Prevents...
Next.js automatically prefetches links in viewport. Reduces page load time. Supports custom prefetch behavior. Uses...
Next.js automatically optimizes font loading. Reduces layout shift. Supports CSS size-adjust. Implements font...
Next.js supports multiple caching strategies: build-time cache, server-side cache, client-side cache. Improves...
Middleware runs before request is completed. Enables custom code execution between request and response. Can modify...
next.config.js is used for custom Next.js configuration. Supports various options like rewrites, redirects,...
Environment variables configured in .env files. Support different environments (.env.local, .env.production). Access...
Configure redirects in next.config.js using redirects array. Support permanent/temporary redirects. Handle path...
Rewrites allow URL mapping without path change. Configured in next.config.js. Support external rewrites. Handle path...
Add custom headers using headers in next.config.js. Support security headers, CORS headers. Handle header...
Matcher defines paths where middleware runs. Uses path matching patterns. Support multiple matchers. Handle path...
Customize webpack config in next.config.js. Modify loaders, plugins, optimization settings. Support module...
basePath sets base URL path for application. Useful for sub-path deployments. Handle path prefixing. Support path...
Configure allowed image domains in next.config.js. Support external image optimization. Handle domain whitelist....
Jest and React Testing Library are recommended for unit and integration testing. Cypress or Playwright for...
Configure jest.config.js for Next.js. Set up environment and transforms. Handle module mocking. Support TypeScript...
Use React Testing Library to render pages. Test page components. Handle data fetching. Support routing tests. Test...
Use Chrome DevTools or VS Code debugger. Set breakpoints. Inspect component state. Handle error tracing. Support source maps.
Mock HTTP requests. Test API endpoints. Handle response validation. Support API testing. Implement test scenarios.
Captures component output. Compares against stored snapshots. Detects UI changes. Support snapshot updates. Handle...
Use Node.js debugger. Handle server breakpoints. Inspect server state. Support server-side debugging. Implement logging.
Test individual components/functions. Handle isolated testing. Support test coverage. Implement unit test cases....
Create test fixtures. Handle mock data. Support test databases. Implement data generation. Handle test state.
Next.js is a React framework that provides features like server-side rendering, static site generation, file-based routing, API routes, and built-in optimizations. It simplifies building production-ready React applications with improved performance and SEO capabilities.
Create a Next.js project using 'npx create-next-app@latest' or 'yarn create next-app'. This sets up a new project with default configuration including TypeScript support, ESLint, and basic project structure.
Next.js project structure includes pages directory for routes, public for static assets, styles for CSS, components for reusable UI components, and next.config.js for configuration. The app directory is used for the App Router in newer versions.
Core dependencies include React, React DOM, and Next.js itself. These are automatically included in package.json when creating a new project. Additional dependencies can be added based on project requirements.
Default scripts include 'dev' for development server, 'build' for production build, 'start' for production server, and 'lint' for linting. These are defined in package.json and can be run using npm or yarn.
Run development server using 'npm run dev' or 'yarn dev'. This starts the application with hot reloading at localhost:3000 by default. Changes are automatically reflected without manual refresh.
next.config.js is a configuration file for customizing Next.js behavior. It can configure webpack, environment variables, redirects, rewrites, image optimization, and other build-time features.
Environment variables are handled using .env files (.env.local, .env.development, .env.production). Variables prefixed with NEXT_PUBLIC_ are exposed to the browser. Others are only available server-side.
The pages directory uses the Pages Router, while app directory uses the newer App Router. App Router supports React Server Components, nested layouts, and improved routing patterns by default.
TypeScript is supported out of the box. Create project with --typescript flag or add manually by creating tsconfig.json and installing typescript and @types/react. Next.js automatically detects and configures TypeScript.
Next.js 13+ provides async Server Components, fetch() with caching options, server actions, and client-side fetching methods. Supports static and dynamic data fetching with options for revalidation.
Use async/await directly in Server Components. Example: async function Page() { const data = await fetch('api/data'); return <Component data={data} />}. Supports automatic caching and revalidation.
Static data fetching occurs at build time using fetch with cache: 'force-cache' option. Data is cached and reused across requests. Suitable for content that doesn't change frequently.
Use fetch with cache: 'no-store' option or revalidate: 0 for dynamic data. Data fetched on every request. Suitable for real-time or frequently changing data.
ISR allows static pages to be updated after build time. Use fetch with revalidate option. Combines benefits of static and dynamic rendering. Pages regenerated based on time interval.
Use hooks like useState and useEffect in Client Components, or data fetching libraries like SWR or React Query. Handle loading states and errors. Support real-time updates.
Server Actions allow form handling and data mutations directly from Server Components. Use 'use server' directive. Support progressive enhancement. Handle form submissions securely.
Use fetch cache options or React cache function. Configure cache behavior in fetch requests. Support cache revalidation. Handle cache invalidation.
Fetch multiple data sources simultaneously using Promise.all or parallel routes. Improve performance by avoiding waterfall requests. Handle loading states independently.
Create loading.js files for automatic loading UI. Use Suspense boundaries. Support streaming and progressive rendering. Implement loading skeletons.
SSR generates HTML on the server for each request. Provides better initial page load and SEO. Next.js handles SSR automatically. Combines with client-side hydration for interactivity.
Server Components run only on server, reduce client-side JavaScript. Support async data fetching. Cannot use client-side hooks or browser APIs. Improve performance and bundle size.
Hydration attaches JavaScript functionality to server-rendered HTML. Happens automatically after initial page load. Makes static content interactive. Preserves server-rendered state.
Streaming SSR progressively sends HTML chunks as they're generated. Uses <Suspense> boundaries. Improves Time To First Byte (TTFB). Supports progressive rendering.
Use async Server Components or getServerSideProps. Data available during render. Support server-only operations. Handle loading states.
Better SEO, faster initial page load, improved performance on slow devices. Support social media previews. Handle browser without JavaScript. Improve accessibility.
Use 'use server' directive or .server.js files. Handle sensitive operations. Access server-side APIs. Support secure data handling.
SSR cache stores rendered pages on server. Improves performance for subsequent requests. Supports cache invalidation. Handle cache strategies.
Use error.js files for error boundaries. Handle server-side errors. Support fallback content. Implement error reporting.
SSR generates HTML per request, SSG at build time. SSR better for dynamic content, SSG for static. Trade-off between freshness and performance.
SSG generates HTML at build time instead of runtime. Pages are pre-rendered and can be served from CDN. Provides fastest possible performance. Ideal for content that doesn't change frequently.
Use generateStaticParams for App Router or getStaticProps/getStaticPaths for Pages Router. Pages are built at build time. Support static data fetching. Content cached and reused.
Define dynamic paths using generateStaticParams or getStaticPaths. Specify which paths to pre-render. Support fallback behavior. Handle path generation.
Fallback controls behavior for non-generated paths. Options: false (404), true (loading), or 'blocking' (SSR). Affects user experience and build time.
Fetch data during build using async components or getStaticProps. Data available at build time. Support external APIs. Handle build-time data requirements.
Fastest page loads, better SEO, reduced server load, improved security. Pages can be served from CDN. Support global deployment. Lower hosting costs.
Implement path validation during build. Handle invalid paths. Support custom validation. Implement error handling for path generation.
Data fetched during npm run build. Available for static page generation. Support external data sources. Handle build-time operations.
Place assets in public directory. Support automatic optimization. Handle asset references. Implement asset management strategies.
API Routes are serverless endpoints built into Next.js. Created in pages/api directory (Pages Router) or app/api directory (App Router). Handle HTTP requests and provide backend functionality.
Create a file in app/api directory that exports default async function. Handle request methods (GET, POST, etc.). Return Response object. Example: export async function GET() { return Response.json({ data: 'hello' }) }
Export functions named after HTTP methods (GET, POST, PUT, DELETE). Or use conditional logic in Pages Router. Support method-specific logic. Handle unsupported methods.
Access query params through request.nextUrl.searchParams in App Router or req.query in Pages Router. Parse and validate parameters. Handle missing parameters.
Access request body using await request.json() or similar methods. Validate request data. Process POST data. Return appropriate response.
Use square brackets for dynamic segments [param]. Access parameters through route object. Support multiple dynamic segments. Handle parameter validation.
Return appropriate status codes and error messages. Use try-catch blocks. Implement error handling middleware. Support error logging.
Configure CORS headers using middleware or within route handlers. Set Access-Control-Allow-Origin and other headers. Handle preflight requests.
Middleware processes requests before reaching route handlers. Handle authentication, logging, CORS. Support middleware chains. Implement custom middleware.
Process multipart/form-data using appropriate middleware. Handle file storage. Validate file types and sizes. Implement upload progress.
Pages are special components that become routes automatically when placed in pages/ or app/ directory. Components are reusable UI pieces that don't create routes. Pages can use getStaticProps/getServerSideProps while components cannot.
Server Components are rendered on server by default in App Router. Cannot use browser APIs or React hooks. Better performance and bundle size. Support async operations directly.
Client Components use 'use client' directive. Can use browser APIs and React hooks. Enable interactive features. Run on client side after hydration.
Create layout.js file in app directory. Wraps child pages/components. Shares UI across routes. Supports nested layouts. Uses children prop for content injection.
Hydration attaches JavaScript event handlers to server-rendered HTML. Makes static content interactive. Happens automatically after initial load. Preserves server-rendered state.
Use metadata object or generateMetadata function in page files. Set title, description, open graph data. Support dynamic metadata. Handle SEO requirements.
Create error.js files for error boundaries. Handle component errors. Support fallback content. Implement error reporting. Manage error states.
Use [param] syntax for dynamic routes. Access parameters through props. Support multiple segments. Handle parameter validation. Implement dynamic routing.
Use React Context, state management libraries, or lift state up. Handle component communication. Support state updates. Implement state management patterns.
Layout components are created using layout.js files. Share UI between pages. Support nested layouts. Handle persistent navigation and UI elements. Available in App Router.
Use .module.css files for component-scoped CSS. Import styles as objects. Support local class naming. Automatic unique class generation. Built-in support in Next.js.
Import global CSS in app/layout.js or pages/_app.js. Apply styles across all components. Support reset styles and base themes. Handle global styling patterns.
Use media queries, CSS Grid, Flexbox. Support mobile-first design. Handle breakpoints. Implement responsive patterns. Support different screen sizes.
Create multiple layout.js files in route segments. Support layout hierarchy. Share UI between related routes. Handle layout composition.
Install and configure Tailwind CSS. Use utility classes. Support JIT mode. Handle Tailwind configuration. Implement responsive design.
Use (group) folders to organize routes. Don't affect URL structure. Share layouts within groups. Support multiple groups.
Use CSS-in-JS solutions or dynamic class names. Support runtime styles. Handle style variables. Implement dynamic theming.
Support styled-components, Emotion, and other CSS-in-JS libraries. Handle server-side rendering. Support dynamic styles. Implement styling patterns.
Implement page and layout transitions. Support animation effects. Handle transition states. Implement smooth navigation experiences.
Next.js supports multiple state management options: React's built-in useState and useContext, external libraries like Redux and Zustand, server state with React Query/SWR. Choose based on application needs.
Use useState hook for component-level state. Handle state updates. Support state initialization. Implement local state patterns. Manage state lifecycle.
Context API shares state between components without prop drilling. Create providers and consumers. Handle context updates. Support global state patterns.
Configure Redux store with Next.js. Handle server-side state hydration. Support Redux middleware. Implement Redux patterns. Manage store configuration.
SWR is a React hooks library for data fetching. Handles caching, revalidation, and real-time updates. Support optimistic updates. Implement data fetching patterns.
Use form libraries like React Hook Form or Formik. Handle form validation. Support form submission. Implement form state patterns. Manage form data.
Store state in localStorage or sessionStorage. Handle state recovery. Support persistence strategies. Implement state serialization. Manage persistent data.
Use query parameters and URL segments for state. Handle URL updates. Support navigation state. Implement URL-based patterns. Manage route state.
Initialize state during server-side rendering. Handle state hydration. Support initial data loading. Implement SSR state patterns.
Manage loading, error, and success states. Handle async operations. Support state transitions. Implement async patterns. Manage async flow.
Next.js Image component is an extension of HTML <img> tag. Provides automatic image optimization. Supports lazy loading, responsive sizing, and modern image formats. Handles image optimization on-demand.
Import Image from 'next/image'. Add src, alt, width, and height props. Component optimizes and serves images automatically. Example: <Image src='/img.png' alt='image' width={500} height={300} />
Reduces image file size, improves page load speed, supports responsive images, optimizes for different devices, reduces bandwidth usage, improves Core Web Vitals scores.
Images load only when they enter viewport. Reduces initial page load time. Uses loading='lazy' attribute automatically. Supports intersection observer API.
Images adapt to different screen sizes. Uses sizes prop for viewport-based sizing. Supports srcset generation. Implements responsive design patterns.
Use quality prop to set compression level. Default is 75. Balance between quality and file size. Support different quality levels for different images.
Shows blurred version of image while loading. Use placeholder='blur' prop. Supports blurDataURL for custom blur. Improves perceived performance.
Configure domains in next.config.js. Use loader prop for custom image service. Support remote image optimization. Handle remote image domains.
Import images as modules. Get width and height automatically. Support webpack optimization. Handle static image assets.
Support WebP, AVIF formats. Use formats prop for specific formats. Handle browser compatibility. Implement format strategies.
Next.js supports multiple authentication methods: JWT, session-based, OAuth providers, NextAuth.js library. Can implement custom authentication or use third-party solutions. Supports both client and server-side authentication.
NextAuth.js is a complete authentication solution for Next.js applications. Provides built-in support for multiple providers (OAuth, email, credentials). Handles sessions, JWT, and database integration.
Store JWT in HTTP-only cookies or local storage. Implement token verification. Handle token expiration. Support refresh tokens. Manage token lifecycle.
Store session data on server. Use session cookies for client identification. Handle session expiration. Support session persistence. Implement session management.
Configure OAuth providers. Handle OAuth flow. Support callback URLs. Implement user profile retrieval. Manage OAuth tokens.
Implement role checking middleware. Define user roles. Handle permission checks. Support role hierarchies. Implement access control.
Implement authentication middleware. Verify tokens or sessions. Handle unauthorized requests. Support API security. Implement rate limiting.
Implement CSRF tokens. Handle token validation. Support form submissions. Implement security headers. Prevent cross-site request forgery.
Manage user authentication state. Handle state persistence. Support state updates. Implement state management. Handle state synchronization.
Core Web Vitals are key metrics measuring user experience: LCP (Largest Contentful Paint), FID (First Input Delay), CLS (Cumulative Layout Shift). Next.js provides built-in optimizations for these metrics.
Code splitting automatically splits JavaScript bundles by route. Reduces initial bundle size. Supports dynamic imports. Improves page load performance. Built into Next.js by default.
Next.js Image component automatically optimizes images. Supports lazy loading, responsive sizes, modern formats. Reduces image file size. Improves loading performance.
Next.js automatically determines which pages can be statically generated. Improves page load performance. Supports hybrid static and dynamic pages. No configuration required.
Next.js Script component optimizes third-party script loading. Supports loading strategies (defer, lazy). Prevents render blocking. Improves page performance.
Next.js automatically prefetches links in viewport. Reduces page load time. Supports custom prefetch behavior. Uses intersection observer API.
Next.js automatically optimizes font loading. Reduces layout shift. Supports CSS size-adjust. Implements font display strategies.
Next.js supports multiple caching strategies: build-time cache, server-side cache, client-side cache. Improves response times. Supports cache invalidation.
Middleware runs before request is completed. Enables custom code execution between request and response. Can modify response, redirect requests, add headers. Defined in middleware.ts file.
next.config.js is used for custom Next.js configuration. Supports various options like rewrites, redirects, environment variables. Exports configuration object or function.
Environment variables configured in .env files. Support different environments (.env.local, .env.production). Access via process.env. NEXT_PUBLIC_ prefix for client-side access.
Configure redirects in next.config.js using redirects array. Support permanent/temporary redirects. Handle path matching. Implement redirect conditions.
Rewrites allow URL mapping without path change. Configured in next.config.js. Support external rewrites. Handle path transformation. Maintain URL appearance.
Add custom headers using headers in next.config.js. Support security headers, CORS headers. Handle header conditions. Implement header policies.
Matcher defines paths where middleware runs. Uses path matching patterns. Support multiple matchers. Handle path exclusions. Configure middleware scope.
Customize webpack config in next.config.js. Modify loaders, plugins, optimization settings. Support module customization. Handle build process.
basePath sets base URL path for application. Useful for sub-path deployments. Handle path prefixing. Support path configuration.
Configure allowed image domains in next.config.js. Support external image optimization. Handle domain whitelist. Implement image security.
Jest and React Testing Library are recommended for unit and integration testing. Cypress or Playwright for end-to-end testing. Vitest gaining popularity for faster test execution. Built-in Next.js testing support.
Configure jest.config.js for Next.js. Set up environment and transforms. Handle module mocking. Support TypeScript testing. Default configuration available with next/jest.
Use React Testing Library to render pages. Test page components. Handle data fetching. Support routing tests. Test page lifecycle.
Use Chrome DevTools or VS Code debugger. Set breakpoints. Inspect component state. Handle error tracing. Support source maps.
Mock HTTP requests. Test API endpoints. Handle response validation. Support API testing. Implement test scenarios.
Captures component output. Compares against stored snapshots. Detects UI changes. Support snapshot updates. Handle snapshot maintenance.
Use Node.js debugger. Handle server breakpoints. Inspect server state. Support server-side debugging. Implement logging.
Test individual components/functions. Handle isolated testing. Support test coverage. Implement unit test cases. Handle component logic.
Create test fixtures. Handle mock data. Support test databases. Implement data generation. Handle test state.
Understand SSR, SSG, and ISR strategies.
Work with getStaticProps, getServerSideProps, and SWR.
Explore file-based routing and app directory structure.
Expect discussions about optimization and caching strategies.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Next.js questions, mock interviews, and more to secure your dream role.
Start Preparing now