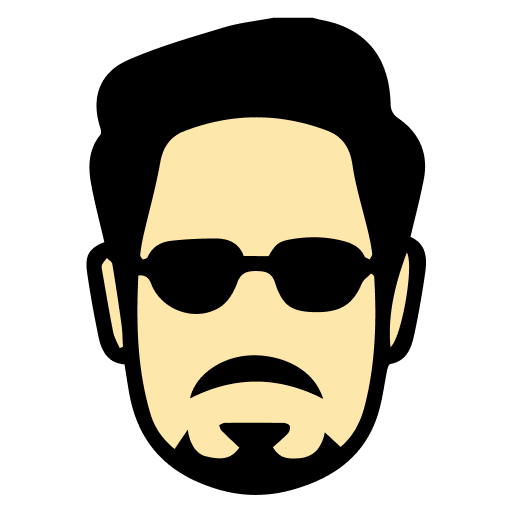
Laravel's elegant syntax and robust features make it a leading PHP framework for web development. Stark.ai offers a comprehensive collection of Laravel interview questions, real-world scenarios, and expert guidance to help you excel in your next technical interview.
Routing in Laravel refers to defining URL paths that users can access and mapping them to specific controller...
Laravel supports common HTTP methods: GET for retrieving data, POST for creating new resources, PUT/PATCH for...
A controller is a PHP class that handles business logic and acts as an intermediary between Models and Views....
Controllers can be created using the Artisan command: php artisan make:controller ControllerName. This creates a new...
Route parameters are dynamic segments in URLs that capture and pass values to controller methods. They are defined...
Routes can be named using the name() method: Route::get('/profile', [ProfileController::class,...
web.php contains routes for web interface and includes session state and CSRF protection. api.php is for stateless...
Request data can be accessed using the Request facade or by type-hinting Request in controller methods. Example:...
Route grouping allows you to share route attributes (middleware, prefixes, namespaces) across multiple routes....
Redirects can be performed using redirect() helper or Route::redirect(). Examples: return redirect('/home') or...
Blade is Laravel's templating engine that combines PHP with HTML templates. It provides convenient shortcuts for...
Variables in Blade templates are displayed using double curly braces syntax {{ $variable }}. The content within...
Blade template inheritance allows creating a base layout template (@extends) with defined sections (@section) that...
Sub-views can be included using @include('view.name') directive. You can also pass data to included views:...
Blade provides convenient directives for control structures: @if, @else, @elseif, @endif for conditionals; @foreach,...
Blade supports loops using @foreach, @for, @while directives. The $loop variable is available inside foreach loops,...
The @yield directive displays content of a specified section. It's typically used in layout templates to define...
Laravel provides the asset() helper function to generate URLs for assets. Assets are stored in the public directory...
Blade comments are written using {{-- comment --}} syntax. Unlike HTML comments, Blade comments are not included in...
Eloquent ORM (Object-Relational Mapping) is Laravel's built-in ORM that allows developers to interact with database...
Models can be created using Artisan command: 'php artisan make:model ModelName'. Adding -m flag creates a migration...
Migrations are like version control for databases, allowing you to define and modify database schema using PHP code....
Basic Eloquent operations include: Model::all() to retrieve all records, Model::find(id) to find by primary key,...
Relationships are defined as methods in model classes. Common types include hasOne(), hasMany(), belongsTo(),...
Seeders are used to populate database tables with sample or initial data. They are created using 'php artisan...
Eloquent provides methods like where(), orWhere(), whereBetween(), orderBy() for querying. Example:...
Mass assignment allows setting multiple model attributes at once. $fillable property in models specifies which...
Database connections are configured in config/database.php and .env file. Multiple connections can be defined for...
Model factories generate fake data for testing and seeding. Created using 'php artisan make:factory'. They use Faker...
Laravel provides a complete authentication system out of the box using the Auth facade. It includes features for...
Basic authentication can be implemented using Auth::attempt(['email' => $email, 'password' => $password]) for login,...
Auth middleware (auth) protects routes by ensuring users are authenticated. Can be applied to routes or controllers...
Use Auth::check() to verify authentication status, Auth::user() to get current user, or @auth/@guest Blade...
Guards define how users are authenticated for each request. Laravel supports multiple authentication guards (web,...
Laravel includes password reset using Password facade. Uses notifications system to send reset links. Requires...
Remember me allows users to stay logged in across sessions using secure cookie. Implemented by passing true as...
Email verification uses MustVerifyEmail interface and VerifiesEmails trait. Sends verification email on...
Sanctum provides lightweight authentication for SPAs and mobile applications. Issues API tokens, handles SPA...
Policies organize authorization logic around models or resources. Created using make:policy command. Methods...
Request handling in Laravel manages HTTP requests using the Request class. It provides methods to access input data,...
Request input data can be accessed using methods like $request->input('name'), $request->get('email'), or...
Form Request Validation is a custom request class that encapsulates validation logic. Created using 'php artisan...
Request data can be validated using validate() method, Validator facade, or Form Request classes. Basic syntax:...
Common validation rules include required, string, email, min, max, between, unique, exists, regex, date, file,...
File uploads are handled using $request->file() or $request->hasFile(). Files can be validated using file, image,...
Validation errors are automatically stored in session and can be accessed in views using $errors variable. Custom...
Old input data is accessible using old() helper function or @old() Blade directive. Data is automatically flashed to...
JSON requests can be handled using $request->json() method. Content-Type should be application/json. Use json()...
CSRF (Cross-Site Request Forgery) protection in Laravel automatically generates and validates tokens for each active...
Laravel provides XSS (Cross-Site Scripting) protection by automatically escaping output using {{ }} Blade syntax....
Laravel prevents SQL injection using PDO parameter binding in the query builder and Eloquent ORM. Query parameters...
Laravel automatically hashes passwords using the Hash facade and bcrypt or Argon2 algorithms. Never store plain-text...
Signed routes are URLs with a signature that ensures they haven't been modified. Created using URL::signedRoute() or...
Laravel sets HTTP-only flag on cookies by default to prevent JavaScript access. Session cookies are automatically...
Mass assignment protection prevents unintended attribute modification through $fillable and $guarded properties in...
Laravel includes security headers through middleware. Headers like X-Frame-Options, X-XSS-Protection, and...
Laravel provides encryption using the Crypt facade. Data is encrypted using OpenSSL and AES-256-CBC. Encryption key...
Laravel secures sessions using encrypted cookies, CSRF protection, and secure configuration options. Sessions can be...
Artisan is Laravel's command-line interface that provides helpful commands for development. It's accessed using 'php...
Basic Artisan commands include: 'php artisan list' to show all commands, 'php artisan help' for command details,...
Custom commands are created using 'php artisan make:command CommandName'. This generates a command class in...
Command signature defines command name and arguments/options. Format: 'name:command {argument} {--option}'. Required...
Custom commands are registered in app/Console/Kernel.php in the commands property or commands() method. They can...
Arguments are required input values, options are optional flags. Define using {argument} and {--option}. Access...
Use methods like line(), info(), comment(), question(), error() for different colored output. table() for tabular...
Command scheduling allows automated command execution at specified intervals. Defined in app/Console/Kernel.php...
Use Artisan facade: Artisan::call('command:name', ['argument' => 'value']). Can queue commands using...
Command isolation ensures each command runs independently. Use separate service providers, handle dependencies...
PHPUnit is the default testing framework in Laravel. It provides a suite of tools for writing and running automated...
Tests are created using 'php artisan make:test TestName'. Two types available: Feature tests (--test suffix) and...
Feature tests focus on larger portions of code and test application behavior from user perspective. Unit tests focus...
Tests are run using 'php artisan test' or './vendor/bin/phpunit'. Can filter tests using --filter flag. Support...
Assertions verify expected outcomes in tests. Common assertions include assertTrue(), assertEquals(),...
Use get(), post(), put(), patch(), delete() methods in tests. Can chain assertions like ->assertOk(),...
Database testing uses RefreshDatabase or DatabaseTransactions traits. Tests run in transactions to prevent test data...
Tinker is an REPL for Laravel. Access using 'php artisan tinker'. Test code, interact with models, execute queries...
Factories generate fake data for testing using Faker library. Created with 'php artisan make:factory'. Define model...
Use .env.testing file for test environment. Configure test database, mail settings, queues. Use config:clear before...
Caching in Laravel stores and retrieves data for faster access. Laravel supports multiple cache drivers (file,...
Basic cache operations include Cache::get() to retrieve, Cache::put() to store, Cache::has() to check existence,...
Route caching improves routing performance using 'php artisan route:cache'. Creates a single file of compiled...
Config caching combines all configuration files into single cached file using 'php artisan config:cache'. Improves...
View caching compiles Blade templates into PHP code. Happens automatically and stored in storage/framework/views....
Database queries can be cached using remember() method on query builder or Eloquent models. Example:...
Cache tags group related items for easy manipulation. Use Cache::tags(['tag'])->put() for storage. Can flush all...
Eager loading reduces N+1 query problems by loading relationships in advance using with() method. Example:...
Response caching stores HTTP responses using middleware. Configure using Cache-Control headers. Supports client-side...
Cache keys uniquely identify cached items. Use meaningful names and version prefixes. Handle key collisions. Support...
Queues allow deferring time-consuming tasks for background processing. Laravel supports various queue drivers...
Jobs are created using 'php artisan make:job JobName'. Jobs implement ShouldQueue interface. Define handle() method...
Queue workers are run using 'php artisan queue:work'. Can specify connection, queue name, and other options. Should...
Job dispatch sends jobs to queue for processing. Can use dispatch() helper, Job::dispatch(), or DispatchesJobs...
Failed jobs are tracked in failed_jobs table. Handle failures using failed() method in job class. Can retry failed...
Job middleware intercept job processing. Define middleware in job's middleware() method. Can rate limit, throttle,...
Job chains execute jobs in sequence using Chain::with(). Later jobs run only if previous ones succeed. Can set chain...
Queue connections are configured in config/queue.php. Define driver, connection parameters. Support multiple...
Job batching processes multiple jobs as group using Bus::batch(). Track batch progress. Handle batch completion and...
Job events track job lifecycle. Listen for job processed, failed events. Handle queue events in...
Routing in Laravel refers to defining URL paths that users can access and mapping them to specific controller actions or closure callbacks. Routes are defined in files within the routes directory, primarily in web.php and api.php.
Laravel supports common HTTP methods: GET for retrieving data, POST for creating new resources, PUT/PATCH for updating existing resources, DELETE for removing resources. These can be defined using Route::get(), Route::post(), Route::put(), Route::patch(), and Route::delete().
A controller is a PHP class that handles business logic and acts as an intermediary between Models and Views. Controllers group related request handling logic into a single class, organizing code better than closure routes.
Controllers can be created using the Artisan command: php artisan make:controller ControllerName. This creates a new controller class in app/Http/Controllers directory with basic structure and necessary imports.
Route parameters are dynamic segments in URLs that capture and pass values to controller methods. They are defined using curly braces in route definitions, like '/user/{id}' where {id} is the parameter.
Routes can be named using the name() method: Route::get('/profile', [ProfileController::class, 'show'])->name('profile'). Named routes provide a convenient way to generate URLs or redirects using route('profile').
web.php contains routes for web interface and includes session state and CSRF protection. api.php is for stateless API routes, includes rate limiting, and is prefixed with '/api'. api.php routes don't include session or CSRF middleware.
Request data can be accessed using the Request facade or by type-hinting Request in controller methods. Example: public function store(Request $request) { $name = $request->input('name'); }
Route grouping allows you to share route attributes (middleware, prefixes, namespaces) across multiple routes. Routes can be grouped using Route::group() or using the Route::prefix() method.
Redirects can be performed using redirect() helper or Route::redirect(). Examples: return redirect('/home') or Route::redirect('/here', '/there'). You can also redirect to named routes using redirect()->route('name').
Blade is Laravel's templating engine that combines PHP with HTML templates. It provides convenient shortcuts for common PHP control structures, template inheritance, and component features while being compiled into plain PHP code for better performance.
Variables in Blade templates are displayed using double curly braces syntax {{ $variable }}. The content within braces is automatically escaped to prevent XSS attacks. For unescaped content, use {!! $variable !!}.
Blade template inheritance allows creating a base layout template (@extends) with defined sections (@section) that child templates can override or extend. Child templates use @extends('layout') to inherit and @section to provide content.
Sub-views can be included using @include('view.name') directive. You can also pass data to included views: @include('view.name', ['data' => $data]). For performance, use @includeIf, @includeWhen, or @includeUnless.
Blade provides convenient directives for control structures: @if, @else, @elseif, @endif for conditionals; @foreach, @while for loops; @switch, @case for switch statements. These compile to regular PHP control structures.
Blade supports loops using @foreach, @for, @while directives. The $loop variable is available inside foreach loops, providing information like index, iteration, first, last, and depth for nested loops.
The @yield directive displays content of a specified section. It's typically used in layout templates to define places where child views can inject content. It can also specify default content if section is not defined.
Laravel provides the asset() helper function to generate URLs for assets. Assets are stored in the public directory and can be referenced using asset('css/app.css'). For versioning, use mix() with Laravel Mix.
Blade comments are written using {{-- comment --}} syntax. Unlike HTML comments, Blade comments are not included in the HTML rendered to the browser, making them useful for developer notes.
Eloquent ORM (Object-Relational Mapping) is Laravel's built-in ORM that allows developers to interact with database tables using elegant object-oriented models. Each database table has a corresponding Model that is used for interacting with that table.
Models can be created using Artisan command: 'php artisan make:model ModelName'. Adding -m flag creates a migration file too. Models are placed in app/Models directory and extend Illuminate\Database\Eloquent\Model class.
Migrations are like version control for databases, allowing you to define and modify database schema using PHP code. They enable team collaboration by maintaining consistent database structure across different development environments.
Basic Eloquent operations include: Model::all() to retrieve all records, Model::find(id) to find by primary key, Model::create([]) to create new records, save() to update, and delete() to remove records.
Relationships are defined as methods in model classes. Common types include hasOne(), hasMany(), belongsTo(), belongsToMany(). These methods specify how models are related to each other in the database.
Seeders are used to populate database tables with sample or initial data. They are created using 'php artisan make:seeder' and can be run using 'php artisan db:seed'. Useful for testing and initial application setup.
Eloquent provides methods like where(), orWhere(), whereBetween(), orderBy() for querying. Example: User::where('active', 1)->orderBy('name')->get(). Queries return collections of model instances.
Mass assignment allows setting multiple model attributes at once. $fillable property in models specifies which attributes can be mass assigned, while $guarded specifies which cannot. This prevents unintended attribute modifications.
Database connections are configured in config/database.php and .env file. Multiple connections can be defined for different databases. The default connection is specified in .env using DB_CONNECTION.
Model factories generate fake data for testing and seeding. Created using 'php artisan make:factory'. They use Faker library to generate realistic test data. Factories can define states for different scenarios.
Laravel provides a complete authentication system out of the box using the Auth facade. It includes features for user registration, login, password reset, and remember me functionality. Can be scaffolded using laravel/ui or breeze/jetstream packages.
Basic authentication can be implemented using Auth::attempt(['email' => $email, 'password' => $password]) for login, Auth::login($user) for manual login, and Auth::logout() for logging out. Session-based authentication is default.
Auth middleware (auth) protects routes by ensuring users are authenticated. Can be applied to routes or controllers using middleware('auth'). Redirects unauthenticated users to login page or returns 401 for API routes.
Use Auth::check() to verify authentication status, Auth::user() to get current user, or @auth/@guest Blade directives in views. Request object also provides auth()->user() helper.
Guards define how users are authenticated for each request. Laravel supports multiple authentication guards (web, api) configured in config/auth.php. Each guard specifies provider and driver for authentication.
Laravel includes password reset using Password facade. Uses notifications system to send reset links. Requires password_resets table. Can customize views, expiration time, and throttling.
Remember me allows users to stay logged in across sessions using secure cookie. Implemented by passing true as second parameter to Auth::attempt() or using remember() method. Requires remember_token column.
Email verification uses MustVerifyEmail interface and VerifiesEmails trait. Sends verification email on registration. Can protect routes with verified middleware. Customizable verification notice and email.
Sanctum provides lightweight authentication for SPAs and mobile applications. Issues API tokens, handles SPA authentication through cookies. Supports multiple tokens per user with different abilities.
Policies organize authorization logic around models or resources. Created using make:policy command. Methods correspond to actions (view, create, update, delete). Used with Gate facade or @can directive.
Request handling in Laravel manages HTTP requests using the Request class. It provides methods to access input data, files, headers, and server variables. Request handling is the foundation of processing user input in Laravel applications.
Request input data can be accessed using methods like $request->input('name'), $request->get('email'), or $request->all(). For specific input types, use $request->query() for GET parameters and $request->post() for POST data.
Form Request Validation is a custom request class that encapsulates validation logic. Created using 'php artisan make:request'. Contains rules() method for validation rules and authorize() method for authorization checks.
Request data can be validated using validate() method, Validator facade, or Form Request classes. Basic syntax: $validated = $request->validate(['field' => 'rule|rule2']). Failed validation redirects back with errors.
Common validation rules include required, string, email, min, max, between, unique, exists, regex, date, file, image, and numeric. Rules can be combined using pipe (|) or array syntax.
File uploads are handled using $request->file() or $request->hasFile(). Files can be validated using file, image, mimes rules. Use store() or storeAs() methods to save uploaded files.
Validation errors are automatically stored in session and can be accessed in views using $errors variable. Custom error messages can be defined in validation rules or language files.
Old input data is accessible using old() helper function or @old() Blade directive. Data is automatically flashed to session on validation failure. Useful for repopulating forms.
JSON requests can be handled using $request->json() method. Content-Type should be application/json. Use json() method for responses. APIs typically return JSON responses by default.
CSRF (Cross-Site Request Forgery) protection in Laravel automatically generates and validates tokens for each active user session. It's implemented through the VerifyCsrfToken middleware and @csrf Blade directive in forms.
Laravel provides XSS (Cross-Site Scripting) protection by automatically escaping output using {{ }} Blade syntax. HTML entities are converted to prevent script injection. Use {!! !!} for trusted content that needs to render HTML.
Laravel prevents SQL injection using PDO parameter binding in the query builder and Eloquent ORM. Query parameters are automatically escaped. Never concatenate strings directly into queries.
Laravel automatically hashes passwords using the Hash facade and bcrypt or Argon2 algorithms. Never store plain-text passwords. Password hashing is handled by the HashedAttributes trait in the User model.
Signed routes are URLs with a signature that ensures they haven't been modified. Created using URL::signedRoute() or URL::temporarySignedRoute(). Useful for email verification or temporary access links.
Laravel sets HTTP-only flag on cookies by default to prevent JavaScript access. Session cookies are automatically HTTP-only. Config can be modified in config/session.php.
Mass assignment protection prevents unintended attribute modification through $fillable and $guarded properties in models. Attributes must be explicitly marked as fillable to allow mass assignment.
Laravel includes security headers through middleware. Headers like X-Frame-Options, X-XSS-Protection, and X-Content-Type-Options are set by default. Additional headers can be added via middleware.
Laravel provides encryption using the Crypt facade. Data is encrypted using OpenSSL and AES-256-CBC. Encryption key is stored in .env file. All encrypted values are signed to prevent tampering.
Laravel secures sessions using encrypted cookies, CSRF protection, and secure configuration options. Sessions can be stored in various drivers (file, database, Redis). Session IDs are regularly rotated.
Artisan is Laravel's command-line interface that provides helpful commands for development. It's accessed using 'php artisan' and includes commands for database migrations, cache clearing, job processing, and other common tasks.
Basic Artisan commands include: 'php artisan list' to show all commands, 'php artisan help' for command details, 'php artisan serve' to start development server, 'php artisan tinker' for REPL, and 'php artisan make' for generating files.
Custom commands are created using 'php artisan make:command CommandName'. This generates a command class in app/Console/Commands. Define command signature and description, implement handle() method for command logic.
Command signature defines command name and arguments/options. Format: 'name:command {argument} {--option}'. Required arguments in curly braces, optional in square brackets. Options prefixed with --.
Custom commands are registered in app/Console/Kernel.php in the commands property or commands() method. They can also be registered using $this->load() method to auto-register all commands in a directory.
Arguments are required input values, options are optional flags. Define using {argument} and {--option}. Access using $this->argument() and $this->option() in handle() method. Can have default values.
Use methods like line(), info(), comment(), question(), error() for different colored output. table() for tabular data, progressBar() for progress indicators. All methods available through Command class.
Command scheduling allows automated command execution at specified intervals. Defined in app/Console/Kernel.php schedule() method. Uses cron expressions or fluent interface. Requires cron entry for schedule:run.
Use Artisan facade: Artisan::call('command:name', ['argument' => 'value']). Can queue commands using Artisan::queue(). Get command output using Artisan::output().
Command isolation ensures each command runs independently. Use separate service providers, handle dependencies properly. Important for testing and avoiding side effects between commands.
PHPUnit is the default testing framework in Laravel. It provides a suite of tools for writing and running automated tests. Laravel extends PHPUnit with additional assertions and helper methods for testing applications.
Tests are created using 'php artisan make:test TestName'. Two types available: Feature tests (--test suffix) and Unit tests (--unit flag). Tests extend TestCase class and are stored in tests directory.
Feature tests focus on larger portions of code and test application behavior from user perspective. Unit tests focus on individual classes or methods in isolation. Feature tests typically test HTTP requests, while unit tests verify specific functionality.
Tests are run using 'php artisan test' or './vendor/bin/phpunit'. Can filter tests using --filter flag. Support parallel testing with --parallel option. Generate coverage reports with --coverage flag.
Assertions verify expected outcomes in tests. Common assertions include assertTrue(), assertEquals(), assertDatabaseHas(). Laravel adds web-specific assertions like assertStatus(), assertViewIs(), assertJson().
Use get(), post(), put(), patch(), delete() methods in tests. Can chain assertions like ->assertOk(), ->assertRedirect(). Submit forms using call() method with request data.
Database testing uses RefreshDatabase or DatabaseTransactions traits. Tests run in transactions to prevent test data persistence. Use factories to generate test data. Assert database state using assertDatabaseHas().
Tinker is an REPL for Laravel. Access using 'php artisan tinker'. Test code, interact with models, execute queries interactively. Useful for debugging and exploring application state.
Factories generate fake data for testing using Faker library. Created with 'php artisan make:factory'. Define model attributes and relationships. Support states for different scenarios.
Use .env.testing file for test environment. Configure test database, mail settings, queues. Use config:clear before testing. Support different configurations per test suite.
Caching in Laravel stores and retrieves data for faster access. Laravel supports multiple cache drivers (file, database, Redis, Memcached) configured in config/cache.php. Caching improves application performance by reducing database queries and computation.
Basic cache operations include Cache::get() to retrieve, Cache::put() to store, Cache::has() to check existence, Cache::forget() to remove items. Also supports Cache::remember() for compute-and-store operations.
Route caching improves routing performance using 'php artisan route:cache'. Creates a single file of compiled routes. Should be used in production. Must be cleared when routes change using route:clear.
Config caching combines all configuration files into single cached file using 'php artisan config:cache'. Improves performance by reducing file loading. Must be cleared when configs change using config:clear.
View caching compiles Blade templates into PHP code. Happens automatically and stored in storage/framework/views. Can be cleared using view:clear. Improves rendering performance.
Database queries can be cached using remember() method on query builder or Eloquent models. Example: User::remember(60)->get(). Also supports tags and cache invalidation strategies.
Cache tags group related items for easy manipulation. Use Cache::tags(['tag'])->put() for storage. Can flush all tagged cache using Cache::tags(['tag'])->flush(). Not all drivers support tagging.
Eager loading reduces N+1 query problems by loading relationships in advance using with() method. Example: User::with('posts')->get(). Improves performance by reducing database queries.
Response caching stores HTTP responses using middleware. Configure using Cache-Control headers. Supports client-side and server-side caching. Improves response times for static content.
Cache keys uniquely identify cached items. Use meaningful names and version prefixes. Handle key collisions. Support cache namespacing. Consider key length limits.
Queues allow deferring time-consuming tasks for background processing. Laravel supports various queue drivers (database, Redis, SQS, etc.). Queues improve application response time by handling heavy tasks asynchronously.
Jobs are created using 'php artisan make:job JobName'. Jobs implement ShouldQueue interface. Define handle() method for job logic. Jobs can be dispatched using dispatch() helper or Job::dispatch().
Queue workers are run using 'php artisan queue:work'. Can specify connection, queue name, and other options. Should be monitored using supervisor or similar process manager in production.
Job dispatch sends jobs to queue for processing. Can use dispatch() helper, Job::dispatch(), or DispatchesJobs trait. Supports delayed dispatch and customizing queue/connection.
Failed jobs are tracked in failed_jobs table. Handle failures using failed() method in job class. Can retry failed jobs using queue:retry command. Support custom failure handling.
Job middleware intercept job processing. Define middleware in job's middleware() method. Can rate limit, throttle, or modify job behavior. Support global and per-job middleware.
Job chains execute jobs in sequence using Chain::with(). Later jobs run only if previous ones succeed. Can set chain catch callback for failure handling.
Queue connections are configured in config/queue.php. Define driver, connection parameters. Support multiple connections. Can set default connection. Handle queue priorities.
Job batching processes multiple jobs as group using Bus::batch(). Track batch progress. Handle batch completion and failures. Support adding jobs to existing batch.
Job events track job lifecycle. Listen for job processed, failed events. Handle queue events in EventServiceProvider. Support custom event listeners.
Understand concepts like MVC, Eloquent ORM, routing, and middleware.
Work on creating APIs, database migrations, and authentication systems.
Dive into service containers, events, queues, and testing.
Expect hands-on challenges to build, secure, and optimize Laravel applications.
Join thousands of successful candidates preparing with Stark.ai. Start practicing Laravel questions, mock interviews, and more to secure your dream role.
Start Preparing now