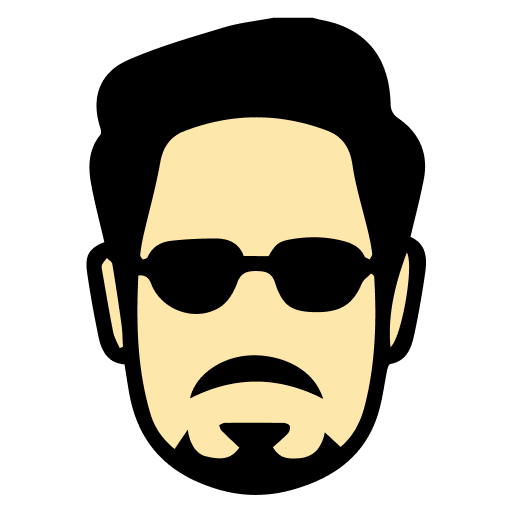
Python is one of the most sought-after skills in the tech industry, making it a popular choice for interviews. Whether you're applying for a software developer, data analyst, or machine learning role, being prepared is key. Stark.ai helps you excel in Python job interviews with curated questions, real-world scenarios, and expert answers.
We couldn't find any categories for technical of type all at the moment.
Please check back later or explore other skills.
We couldn't find any interview questions for technical of type all at the moment.
Please check back later or explore other skills.
Solve Python coding challenges designed to mirror interview questions.
Explore MoreEnsure your Python expertise is highlighted with ATS-friendly resumes.
Explore MoreMaster Python syntax, data types, and core libraries.
Regularly solve Python problems on platforms like Stark.ai.
Discuss your hands-on experience with Python.
Highlight how Python helped you solve specific problems.
Join thousands of successful candidates who prepared with Stark.ai. Practice Python questions, mock interviews, and more to secure your dream job.
Start Preparing now