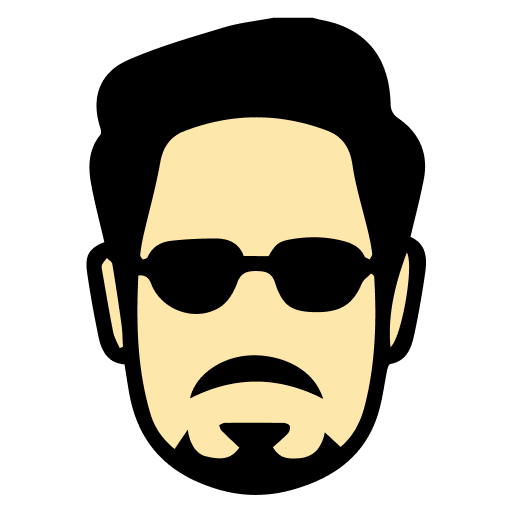
Solve Longest Alternating Path Search using Rust to enhance your skills with rust coding practice , master coding concepts, and prepare for interviews with practical exercises and detailed solutions.
Difficulty : Hard
Categories :
You are given a 2D matrix where each cell is either 0 or 1. A path in the matrix is called a 'zigzag path' if:
Find the length of the longest possible zigzag path. If no such path exists, return -1.
Input: matrix = [ [1,0,1,0], [0,1,1,0], [1,0,1,1], [0,1,0,1] ] Output: 4 Explanation: One possible path is (0,1) -> (1,1) -> (2,2) -> (3,1) Values along path: 0 -> 1 -> 0 -> 1
Input: matrix = [ [1,1], [1,1], [0,1] ] Output: 2 Explanation: Path can be (0,0) -> (1,1)
Real-World Challenges Solve problems that help you master Rust's unique features.
Detailed Explanations Break down complex concepts into manageable steps.
Industry-Ready Skills Prepare for systems programming and performance-critical applications.