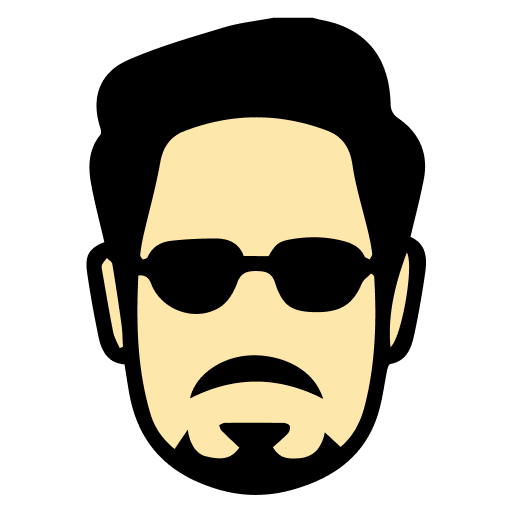
Solve Rat Maze With Multiple Jumps using Python to enhance your skills with python coding practice , master coding concepts, and prepare for interviews with practical exercises and detailed solutions.
Difficulty : Medium
Categories :
Given an n×n maze matrix where a rat needs to reach from source (0,0) to destination (n-1,n-1). The rat can move in two directions:
Each cell contains a number indicating the maximum number of steps the rat can jump from that cell. A value of 0 indicates a blocked cell.
Return a matrix of the same size where 1 indicates cells in the path taken and 0 for unused cells. If no path exists, return a 1×1 matrix containing -1.
Input: matrix = [ [2,1,0,0], [3,0,0,1], [0,1,0,1], [0,0,0,1] ] Output: [ [1,0,0,0], [1,0,0,1], [0,0,0,1], [0,0,0,1] ] Explanation: Path takes jumps according to allowed max steps in each cell
Interactive Exercises Practice coding with problems designed for beginners and experts.
Step-by-Step Solutions Understand every step of the solution process.
Real-World Scenarios Apply your skills to real-world problems and boost your confidence.