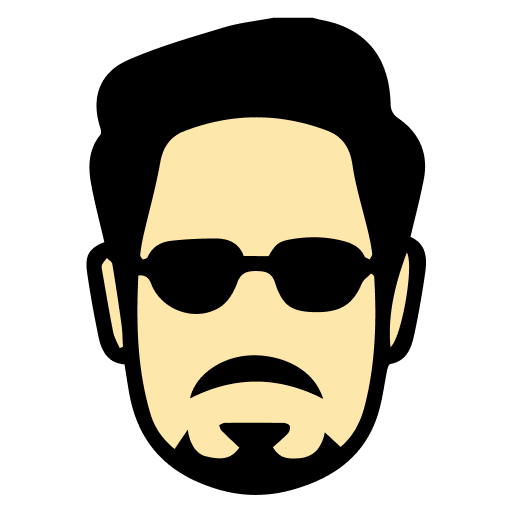
Solve Minimize Array Maximum with Bit Operations using Lua to enhance your skills with lua coding practice , master coding concepts, and prepare for interviews with practical exercises and detailed solutions.
Difficulty : Hard
Categories :
You are given an array of non-negative integers nums. Your task is to minimize the maximum value in the array by performing at most k operations, where in each operation you can:
Return the minimum possible maximum value in the array after at most k operations.
Input: nums = [3,5,2,6], k = 2 Output: 2 Explanation: Operation 1: Right shift 6 >> 1 = 3, array becomes [3,5,2,3] Operation 2: Right shift 5 >> 2 = 1, array becomes [3,1,2,3] Maximum value is now 3, which is minimum possible
Input: nums = [10,8,6,12], k = 1 Output: 8 Explanation: Optimal operation: Right shift 12 >> 1 = 6 Array becomes [10,8,6,6], maximum is 8
Real-World Applications Solve problems inspired by Lua's common use cases, such as game development and embedded systems.
Step-by-Step Guidance Break down Lua's concepts into digestible lessons.
Practical Skills Build hands-on experience with Lua for real-world projects.