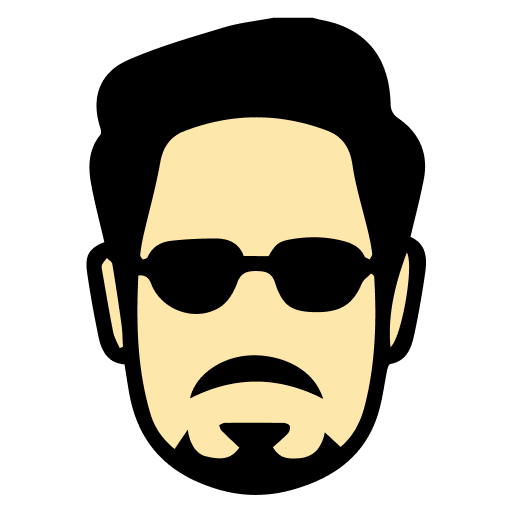
Solve Sort by Bit Count using JavaScript to enhance your skills with javascript coding practice , master coding concepts, and prepare for interviews with practical exercises and detailed solutions.
Difficulty : Medium
Categories :
Given an array of integers nums, sort it according to the following rules:
Return the sorted array.
Input: nums = [3,5,2,7,8] Output: [2,8,3,5,7] Explanation: 2 = 10 (1 bit) 8 = 1000 (1 bit) 3 = 11 (2 bits) 5 = 101 (2 bits) 7 = 111 (3 bits)
Input: nums = [10,12,15,9] Output: [12,10,9,15] Explanation: 12 = 1100 (2 bits) 10 = 1010 (2 bits) 9 = 1001 (2 bits) 15 = 1111 (4 bits) Within 2-bit group, sort by value
Hands-On Exercises Work on coding problems inspired by real-world scenarios.
Detailed Explanations Break down complex solutions into easy-to-understand steps.
Interactive Learning Test your skills in an engaging and fun way.