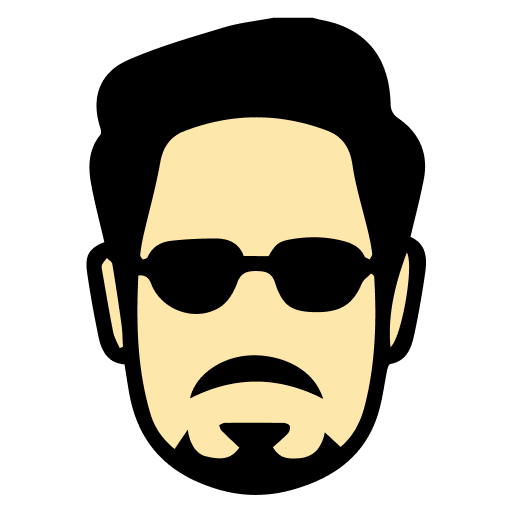
Solve DFS of Graph using JavaScript to enhance your skills with javascript coding practice , master coding concepts, and prepare for interviews with practical exercises and detailed solutions.
Difficulty : Easy
Categories :
Given a connected undirected graph represented as an adjacency list adj
, perform a Depth First Search (DFS) traversal starting from vertex 0. Return a list containing the DFS traversal of the graph.
The traversal should visit neighbors in the same order as they appear in the adjacency list.
Input: adj = [[2,3,1], [0], [0,4], [0], [2]] Output: [0,2,4,3,1] Explanation: DFS path: 1. Start at 0 2. Visit first neighbor 2, then its neighbor 4 3. Backtrack to 0, visit 3 4. Backtrack to 0, visit 1
Input: adj = [[1,2], [0,2], [0,1,3,4], [2], [2]] Output: [0,1,2,3,4] Explanation: Follow adjacency list order for neighbor selection
Hands-On Exercises Work on coding problems inspired by real-world scenarios.
Detailed Explanations Break down complex solutions into easy-to-understand steps.
Interactive Learning Test your skills in an engaging and fun way.