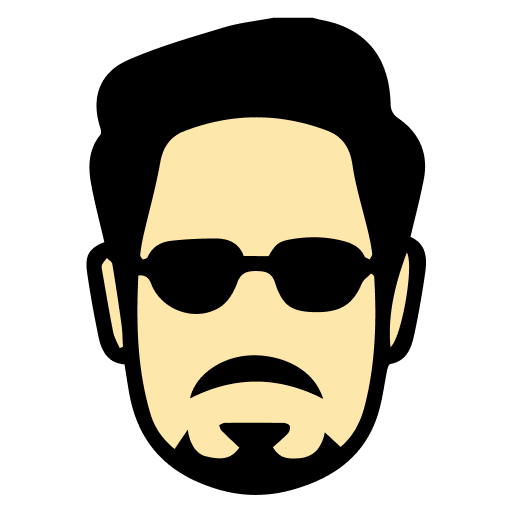
Solve Array Increment Operations using JavaScript to enhance your skills with javascript coding practice , master coding concepts, and prepare for interviews with practical exercises and detailed solutions.
Difficulty : Medium
Categories :
Design a data structure that supports incrementing subsequence of elements by a value. Implement the CustomArray class:
Input: ["CustomArray", "increment", "increment", "getArray"] [[5], [0, 2, 100], [1, 4, 100], []] Output: [null, null, null, [100,200,200,100,100]] Explanation: CustomArray arr = new CustomArray(5); arr.increment(0, 2, 100); // [100,100,100,0,0] arr.increment(1, 4, 100); // [100,200,200,100,100] arr.getArray(); // [100,200,200,100,100]
Hands-On Exercises Work on coding problems inspired by real-world scenarios.
Detailed Explanations Break down complex solutions into easy-to-understand steps.
Interactive Learning Test your skills in an engaging and fun way.