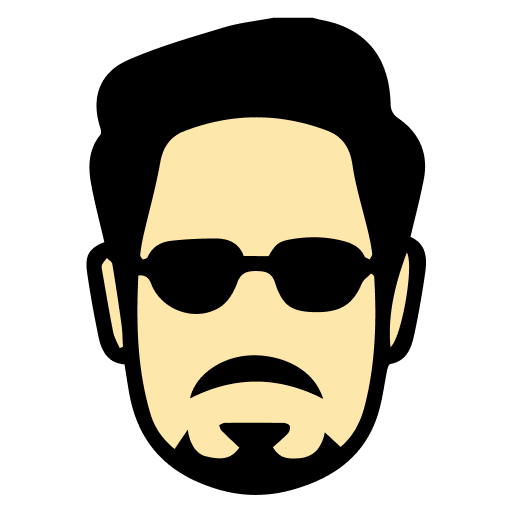
Solve Print Adjacency List using Java to enhance your skills with java coding practice , master coding concepts, and prepare for interviews with practical exercises and detailed solutions.
Difficulty : Easy
Categories :
Given an undirected graph with V
vertices and E
edges, create and return its adjacency list representation. Use 0-based indexing for the vertices.
An adjacency list is a data structure where for each vertex, we maintain a list of all other vertices it is connected to.
Input: V = 5, E = 7 edges = [[0,1],[0,4],[4,1],[4,3],[1,3],[1,2],[3,2]] Output: [[1,4],[0,2,3,4],[1,3],[1,2,4],[0,1,3]] Explanation: For each vertex i, output[i] contains all vertices j where an edge exists between i and j.
Input: V = 4, E = 3 edges = [[0,3],[0,2],[2,1]] Output: [[2,3],[2],[0,1],[0]] Explanation: The list for each vertex contains all vertices it has edges to.
Real-World Problems Solve problems designed to simulate workplace challenges.
Comprehensive Solutions Gain a deep understanding of Java concepts through detailed explanations.
Industry-Ready Skills Prepare for top tech roles with targeted exercises.