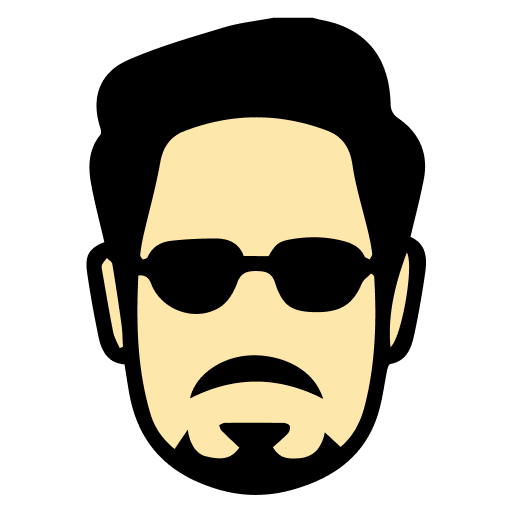
Solve Longest Equal Subarray After Bit Flips using Java to enhance your skills with java coding practice , master coding concepts, and prepare for interviews with practical exercises and detailed solutions.
Difficulty : Hard
Categories :
You are given an array of integers nums and an integer k. You can perform any of the following operations at most k times:
Your task is to maximize the length of the longest subarray that contains all equal elements after at most k operations.
Input: nums = [4,2,4,4], k = 1 Output: 4 Explanation: Change 2 (binary: 10) to 4 (binary: 100) with one bit flip Array becomes [4,4,4,4], longest equal subarray length is 4
Input: nums = [1,2,4,8], k = 2 Output: 2 Explanation: Two bit flips needed to make any two numbers equal Can change 1 (001) to 2 (010) with one bit flip Array can become [2,2,4,8], longest equal subarray length is 2
Real-World Problems Solve problems designed to simulate workplace challenges.
Comprehensive Solutions Gain a deep understanding of Java concepts through detailed explanations.
Industry-Ready Skills Prepare for top tech roles with targeted exercises.